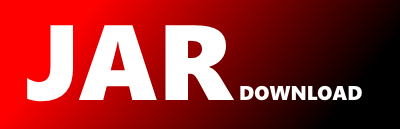
com.pig4cloud.plugin.cache.support.RedisCaffeineCache Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of multilevel-cache-spring-boot-starter Show documentation
Show all versions of multilevel-cache-spring-boot-starter Show documentation
support L1 caffeine and L2 redis cache
package com.pig4cloud.plugin.cache.support;
import com.github.benmanes.caffeine.cache.Cache;
import com.pig4cloud.plugin.cache.properties.CacheConfigProperties;
import lombok.Getter;
import lombok.extern.slf4j.Slf4j;
import org.springframework.cache.support.AbstractValueAdaptingCache;
import org.springframework.data.redis.core.RedisTemplate;
import org.springframework.data.redis.serializer.StringRedisSerializer;
import org.springframework.util.CollectionUtils;
import org.springframework.util.StringUtils;
import java.util.Map;
import java.util.Set;
import java.util.concurrent.Callable;
import java.util.concurrent.ConcurrentHashMap;
import java.util.concurrent.TimeUnit;
import java.util.concurrent.locks.ReentrantLock;
/**
* @author fuwei.deng
* @version 1.0.0
*/
@Slf4j
public class RedisCaffeineCache extends AbstractValueAdaptingCache {
@Getter
private final String name;
@Getter
private final Cache
© 2015 - 2025 Weber Informatics LLC | Privacy Policy