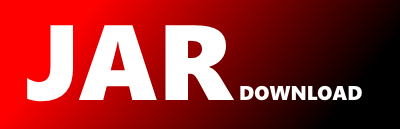
binlog.CisternOuterClass Maven / Gradle / Ivy
// Generated by the protocol buffer compiler. DO NOT EDIT!
// source: binlog/cistern.proto
package binlog;
public final class CisternOuterClass {
private CisternOuterClass() {}
public static void registerAllExtensions(
com.google.protobuf.ExtensionRegistryLite registry) {
}
public static void registerAllExtensions(
com.google.protobuf.ExtensionRegistry registry) {
registerAllExtensions(
(com.google.protobuf.ExtensionRegistryLite) registry);
}
public interface DumpBinlogReqOrBuilder extends
// @@protoc_insertion_point(interface_extends:binlog.DumpBinlogReq)
com.google.protobuf.MessageOrBuilder {
/**
*
* beginCommitTS speicifies the position from which begin to dump binlogs.
* note that actually the result of dump starts from the one next to beginCommitTS
* it should be zero in case of the first request.
*
*
* optional int64 beginCommitTS = 1;
*/
long getBeginCommitTS();
}
/**
* Protobuf type {@code binlog.DumpBinlogReq}
*/
public static final class DumpBinlogReq extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:binlog.DumpBinlogReq)
DumpBinlogReqOrBuilder {
// Use DumpBinlogReq.newBuilder() to construct.
private DumpBinlogReq(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private DumpBinlogReq() {
beginCommitTS_ = 0L;
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return com.google.protobuf.UnknownFieldSet.getDefaultInstance();
}
private DumpBinlogReq(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
int mutable_bitField0_ = 0;
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
default: {
if (!input.skipField(tag)) {
done = true;
}
break;
}
case 8: {
beginCommitTS_ = input.readInt64();
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return binlog.CisternOuterClass.internal_static_binlog_DumpBinlogReq_descriptor;
}
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return binlog.CisternOuterClass.internal_static_binlog_DumpBinlogReq_fieldAccessorTable
.ensureFieldAccessorsInitialized(
binlog.CisternOuterClass.DumpBinlogReq.class, binlog.CisternOuterClass.DumpBinlogReq.Builder.class);
}
public static final int BEGINCOMMITTS_FIELD_NUMBER = 1;
private long beginCommitTS_;
/**
*
* beginCommitTS speicifies the position from which begin to dump binlogs.
* note that actually the result of dump starts from the one next to beginCommitTS
* it should be zero in case of the first request.
*
*
* optional int64 beginCommitTS = 1;
*/
public long getBeginCommitTS() {
return beginCommitTS_;
}
private byte memoizedIsInitialized = -1;
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (beginCommitTS_ != 0L) {
output.writeInt64(1, beginCommitTS_);
}
}
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (beginCommitTS_ != 0L) {
size += com.google.protobuf.CodedOutputStream
.computeInt64Size(1, beginCommitTS_);
}
memoizedSize = size;
return size;
}
private static final long serialVersionUID = 0L;
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof binlog.CisternOuterClass.DumpBinlogReq)) {
return super.equals(obj);
}
binlog.CisternOuterClass.DumpBinlogReq other = (binlog.CisternOuterClass.DumpBinlogReq) obj;
boolean result = true;
result = result && (getBeginCommitTS()
== other.getBeginCommitTS());
return result;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptorForType().hashCode();
hash = (37 * hash) + BEGINCOMMITTS_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
getBeginCommitTS());
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static binlog.CisternOuterClass.DumpBinlogReq parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static binlog.CisternOuterClass.DumpBinlogReq parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static binlog.CisternOuterClass.DumpBinlogReq parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static binlog.CisternOuterClass.DumpBinlogReq parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static binlog.CisternOuterClass.DumpBinlogReq parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static binlog.CisternOuterClass.DumpBinlogReq parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static binlog.CisternOuterClass.DumpBinlogReq parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static binlog.CisternOuterClass.DumpBinlogReq parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static binlog.CisternOuterClass.DumpBinlogReq parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static binlog.CisternOuterClass.DumpBinlogReq parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(binlog.CisternOuterClass.DumpBinlogReq prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code binlog.DumpBinlogReq}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:binlog.DumpBinlogReq)
binlog.CisternOuterClass.DumpBinlogReqOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return binlog.CisternOuterClass.internal_static_binlog_DumpBinlogReq_descriptor;
}
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return binlog.CisternOuterClass.internal_static_binlog_DumpBinlogReq_fieldAccessorTable
.ensureFieldAccessorsInitialized(
binlog.CisternOuterClass.DumpBinlogReq.class, binlog.CisternOuterClass.DumpBinlogReq.Builder.class);
}
// Construct using binlog.CisternOuterClass.DumpBinlogReq.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
public Builder clear() {
super.clear();
beginCommitTS_ = 0L;
return this;
}
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return binlog.CisternOuterClass.internal_static_binlog_DumpBinlogReq_descriptor;
}
public binlog.CisternOuterClass.DumpBinlogReq getDefaultInstanceForType() {
return binlog.CisternOuterClass.DumpBinlogReq.getDefaultInstance();
}
public binlog.CisternOuterClass.DumpBinlogReq build() {
binlog.CisternOuterClass.DumpBinlogReq result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
public binlog.CisternOuterClass.DumpBinlogReq buildPartial() {
binlog.CisternOuterClass.DumpBinlogReq result = new binlog.CisternOuterClass.DumpBinlogReq(this);
result.beginCommitTS_ = beginCommitTS_;
onBuilt();
return result;
}
public Builder clone() {
return (Builder) super.clone();
}
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
Object value) {
return (Builder) super.setField(field, value);
}
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return (Builder) super.clearField(field);
}
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return (Builder) super.clearOneof(oneof);
}
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, Object value) {
return (Builder) super.setRepeatedField(field, index, value);
}
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
Object value) {
return (Builder) super.addRepeatedField(field, value);
}
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof binlog.CisternOuterClass.DumpBinlogReq) {
return mergeFrom((binlog.CisternOuterClass.DumpBinlogReq)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(binlog.CisternOuterClass.DumpBinlogReq other) {
if (other == binlog.CisternOuterClass.DumpBinlogReq.getDefaultInstance()) return this;
if (other.getBeginCommitTS() != 0L) {
setBeginCommitTS(other.getBeginCommitTS());
}
onChanged();
return this;
}
public final boolean isInitialized() {
return true;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
binlog.CisternOuterClass.DumpBinlogReq parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (binlog.CisternOuterClass.DumpBinlogReq) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private long beginCommitTS_ ;
/**
*
* beginCommitTS speicifies the position from which begin to dump binlogs.
* note that actually the result of dump starts from the one next to beginCommitTS
* it should be zero in case of the first request.
*
*
* optional int64 beginCommitTS = 1;
*/
public long getBeginCommitTS() {
return beginCommitTS_;
}
/**
*
* beginCommitTS speicifies the position from which begin to dump binlogs.
* note that actually the result of dump starts from the one next to beginCommitTS
* it should be zero in case of the first request.
*
*
* optional int64 beginCommitTS = 1;
*/
public Builder setBeginCommitTS(long value) {
beginCommitTS_ = value;
onChanged();
return this;
}
/**
*
* beginCommitTS speicifies the position from which begin to dump binlogs.
* note that actually the result of dump starts from the one next to beginCommitTS
* it should be zero in case of the first request.
*
*
* optional int64 beginCommitTS = 1;
*/
public Builder clearBeginCommitTS() {
beginCommitTS_ = 0L;
onChanged();
return this;
}
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return this;
}
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return this;
}
// @@protoc_insertion_point(builder_scope:binlog.DumpBinlogReq)
}
// @@protoc_insertion_point(class_scope:binlog.DumpBinlogReq)
private static final binlog.CisternOuterClass.DumpBinlogReq DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new binlog.CisternOuterClass.DumpBinlogReq();
}
public static binlog.CisternOuterClass.DumpBinlogReq getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
public DumpBinlogReq parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new DumpBinlogReq(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
public binlog.CisternOuterClass.DumpBinlogReq getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface DumpBinlogRespOrBuilder extends
// @@protoc_insertion_point(interface_extends:binlog.DumpBinlogResp)
com.google.protobuf.MessageOrBuilder {
/**
*
* CommitTS specifies the commitTS of binlog
*
*
* optional int64 commitTS = 1;
*/
long getCommitTS();
/**
*
* payloads is bytecodes encoded from binlog item
*
*
* optional bytes payload = 2;
*/
com.google.protobuf.ByteString getPayload();
/**
*
* ddljob is json bytes marshaled from corresponding ddljob struct if payload is a DDL type of binlog
*
*
* optional bytes ddljob = 3;
*/
com.google.protobuf.ByteString getDdljob();
}
/**
* Protobuf type {@code binlog.DumpBinlogResp}
*/
public static final class DumpBinlogResp extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:binlog.DumpBinlogResp)
DumpBinlogRespOrBuilder {
// Use DumpBinlogResp.newBuilder() to construct.
private DumpBinlogResp(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private DumpBinlogResp() {
commitTS_ = 0L;
payload_ = com.google.protobuf.ByteString.EMPTY;
ddljob_ = com.google.protobuf.ByteString.EMPTY;
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return com.google.protobuf.UnknownFieldSet.getDefaultInstance();
}
private DumpBinlogResp(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
int mutable_bitField0_ = 0;
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
default: {
if (!input.skipField(tag)) {
done = true;
}
break;
}
case 8: {
commitTS_ = input.readInt64();
break;
}
case 18: {
payload_ = input.readBytes();
break;
}
case 26: {
ddljob_ = input.readBytes();
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return binlog.CisternOuterClass.internal_static_binlog_DumpBinlogResp_descriptor;
}
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return binlog.CisternOuterClass.internal_static_binlog_DumpBinlogResp_fieldAccessorTable
.ensureFieldAccessorsInitialized(
binlog.CisternOuterClass.DumpBinlogResp.class, binlog.CisternOuterClass.DumpBinlogResp.Builder.class);
}
public static final int COMMITTS_FIELD_NUMBER = 1;
private long commitTS_;
/**
*
* CommitTS specifies the commitTS of binlog
*
*
* optional int64 commitTS = 1;
*/
public long getCommitTS() {
return commitTS_;
}
public static final int PAYLOAD_FIELD_NUMBER = 2;
private com.google.protobuf.ByteString payload_;
/**
*
* payloads is bytecodes encoded from binlog item
*
*
* optional bytes payload = 2;
*/
public com.google.protobuf.ByteString getPayload() {
return payload_;
}
public static final int DDLJOB_FIELD_NUMBER = 3;
private com.google.protobuf.ByteString ddljob_;
/**
*
* ddljob is json bytes marshaled from corresponding ddljob struct if payload is a DDL type of binlog
*
*
* optional bytes ddljob = 3;
*/
public com.google.protobuf.ByteString getDdljob() {
return ddljob_;
}
private byte memoizedIsInitialized = -1;
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (commitTS_ != 0L) {
output.writeInt64(1, commitTS_);
}
if (!payload_.isEmpty()) {
output.writeBytes(2, payload_);
}
if (!ddljob_.isEmpty()) {
output.writeBytes(3, ddljob_);
}
}
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (commitTS_ != 0L) {
size += com.google.protobuf.CodedOutputStream
.computeInt64Size(1, commitTS_);
}
if (!payload_.isEmpty()) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(2, payload_);
}
if (!ddljob_.isEmpty()) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(3, ddljob_);
}
memoizedSize = size;
return size;
}
private static final long serialVersionUID = 0L;
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof binlog.CisternOuterClass.DumpBinlogResp)) {
return super.equals(obj);
}
binlog.CisternOuterClass.DumpBinlogResp other = (binlog.CisternOuterClass.DumpBinlogResp) obj;
boolean result = true;
result = result && (getCommitTS()
== other.getCommitTS());
result = result && getPayload()
.equals(other.getPayload());
result = result && getDdljob()
.equals(other.getDdljob());
return result;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptorForType().hashCode();
hash = (37 * hash) + COMMITTS_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
getCommitTS());
hash = (37 * hash) + PAYLOAD_FIELD_NUMBER;
hash = (53 * hash) + getPayload().hashCode();
hash = (37 * hash) + DDLJOB_FIELD_NUMBER;
hash = (53 * hash) + getDdljob().hashCode();
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static binlog.CisternOuterClass.DumpBinlogResp parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static binlog.CisternOuterClass.DumpBinlogResp parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static binlog.CisternOuterClass.DumpBinlogResp parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static binlog.CisternOuterClass.DumpBinlogResp parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static binlog.CisternOuterClass.DumpBinlogResp parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static binlog.CisternOuterClass.DumpBinlogResp parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static binlog.CisternOuterClass.DumpBinlogResp parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static binlog.CisternOuterClass.DumpBinlogResp parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static binlog.CisternOuterClass.DumpBinlogResp parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static binlog.CisternOuterClass.DumpBinlogResp parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(binlog.CisternOuterClass.DumpBinlogResp prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code binlog.DumpBinlogResp}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:binlog.DumpBinlogResp)
binlog.CisternOuterClass.DumpBinlogRespOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return binlog.CisternOuterClass.internal_static_binlog_DumpBinlogResp_descriptor;
}
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return binlog.CisternOuterClass.internal_static_binlog_DumpBinlogResp_fieldAccessorTable
.ensureFieldAccessorsInitialized(
binlog.CisternOuterClass.DumpBinlogResp.class, binlog.CisternOuterClass.DumpBinlogResp.Builder.class);
}
// Construct using binlog.CisternOuterClass.DumpBinlogResp.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
public Builder clear() {
super.clear();
commitTS_ = 0L;
payload_ = com.google.protobuf.ByteString.EMPTY;
ddljob_ = com.google.protobuf.ByteString.EMPTY;
return this;
}
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return binlog.CisternOuterClass.internal_static_binlog_DumpBinlogResp_descriptor;
}
public binlog.CisternOuterClass.DumpBinlogResp getDefaultInstanceForType() {
return binlog.CisternOuterClass.DumpBinlogResp.getDefaultInstance();
}
public binlog.CisternOuterClass.DumpBinlogResp build() {
binlog.CisternOuterClass.DumpBinlogResp result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
public binlog.CisternOuterClass.DumpBinlogResp buildPartial() {
binlog.CisternOuterClass.DumpBinlogResp result = new binlog.CisternOuterClass.DumpBinlogResp(this);
result.commitTS_ = commitTS_;
result.payload_ = payload_;
result.ddljob_ = ddljob_;
onBuilt();
return result;
}
public Builder clone() {
return (Builder) super.clone();
}
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
Object value) {
return (Builder) super.setField(field, value);
}
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return (Builder) super.clearField(field);
}
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return (Builder) super.clearOneof(oneof);
}
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, Object value) {
return (Builder) super.setRepeatedField(field, index, value);
}
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
Object value) {
return (Builder) super.addRepeatedField(field, value);
}
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof binlog.CisternOuterClass.DumpBinlogResp) {
return mergeFrom((binlog.CisternOuterClass.DumpBinlogResp)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(binlog.CisternOuterClass.DumpBinlogResp other) {
if (other == binlog.CisternOuterClass.DumpBinlogResp.getDefaultInstance()) return this;
if (other.getCommitTS() != 0L) {
setCommitTS(other.getCommitTS());
}
if (other.getPayload() != com.google.protobuf.ByteString.EMPTY) {
setPayload(other.getPayload());
}
if (other.getDdljob() != com.google.protobuf.ByteString.EMPTY) {
setDdljob(other.getDdljob());
}
onChanged();
return this;
}
public final boolean isInitialized() {
return true;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
binlog.CisternOuterClass.DumpBinlogResp parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (binlog.CisternOuterClass.DumpBinlogResp) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private long commitTS_ ;
/**
*
* CommitTS specifies the commitTS of binlog
*
*
* optional int64 commitTS = 1;
*/
public long getCommitTS() {
return commitTS_;
}
/**
*
* CommitTS specifies the commitTS of binlog
*
*
* optional int64 commitTS = 1;
*/
public Builder setCommitTS(long value) {
commitTS_ = value;
onChanged();
return this;
}
/**
*
* CommitTS specifies the commitTS of binlog
*
*
* optional int64 commitTS = 1;
*/
public Builder clearCommitTS() {
commitTS_ = 0L;
onChanged();
return this;
}
private com.google.protobuf.ByteString payload_ = com.google.protobuf.ByteString.EMPTY;
/**
*
* payloads is bytecodes encoded from binlog item
*
*
* optional bytes payload = 2;
*/
public com.google.protobuf.ByteString getPayload() {
return payload_;
}
/**
*
* payloads is bytecodes encoded from binlog item
*
*
* optional bytes payload = 2;
*/
public Builder setPayload(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
payload_ = value;
onChanged();
return this;
}
/**
*
* payloads is bytecodes encoded from binlog item
*
*
* optional bytes payload = 2;
*/
public Builder clearPayload() {
payload_ = getDefaultInstance().getPayload();
onChanged();
return this;
}
private com.google.protobuf.ByteString ddljob_ = com.google.protobuf.ByteString.EMPTY;
/**
*
* ddljob is json bytes marshaled from corresponding ddljob struct if payload is a DDL type of binlog
*
*
* optional bytes ddljob = 3;
*/
public com.google.protobuf.ByteString getDdljob() {
return ddljob_;
}
/**
*
* ddljob is json bytes marshaled from corresponding ddljob struct if payload is a DDL type of binlog
*
*
* optional bytes ddljob = 3;
*/
public Builder setDdljob(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
ddljob_ = value;
onChanged();
return this;
}
/**
*
* ddljob is json bytes marshaled from corresponding ddljob struct if payload is a DDL type of binlog
*
*
* optional bytes ddljob = 3;
*/
public Builder clearDdljob() {
ddljob_ = getDefaultInstance().getDdljob();
onChanged();
return this;
}
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return this;
}
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return this;
}
// @@protoc_insertion_point(builder_scope:binlog.DumpBinlogResp)
}
// @@protoc_insertion_point(class_scope:binlog.DumpBinlogResp)
private static final binlog.CisternOuterClass.DumpBinlogResp DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new binlog.CisternOuterClass.DumpBinlogResp();
}
public static binlog.CisternOuterClass.DumpBinlogResp getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
public DumpBinlogResp parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new DumpBinlogResp(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
public binlog.CisternOuterClass.DumpBinlogResp getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface DumpDDLJobsReqOrBuilder extends
// @@protoc_insertion_point(interface_extends:binlog.DumpDDLJobsReq)
com.google.protobuf.MessageOrBuilder {
/**
*
* beginCommitTS is the start point of drainer processing binlog, DumpDDLJobs() returns
* all history DDL jobs before this position, then drainer will apply these DDL jobs
* in order of job ID to restore the whole schema info at that moment.
*
*
* optional int64 beginCommitTS = 1;
*/
long getBeginCommitTS();
}
/**
* Protobuf type {@code binlog.DumpDDLJobsReq}
*/
public static final class DumpDDLJobsReq extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:binlog.DumpDDLJobsReq)
DumpDDLJobsReqOrBuilder {
// Use DumpDDLJobsReq.newBuilder() to construct.
private DumpDDLJobsReq(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private DumpDDLJobsReq() {
beginCommitTS_ = 0L;
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return com.google.protobuf.UnknownFieldSet.getDefaultInstance();
}
private DumpDDLJobsReq(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
int mutable_bitField0_ = 0;
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
default: {
if (!input.skipField(tag)) {
done = true;
}
break;
}
case 8: {
beginCommitTS_ = input.readInt64();
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return binlog.CisternOuterClass.internal_static_binlog_DumpDDLJobsReq_descriptor;
}
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return binlog.CisternOuterClass.internal_static_binlog_DumpDDLJobsReq_fieldAccessorTable
.ensureFieldAccessorsInitialized(
binlog.CisternOuterClass.DumpDDLJobsReq.class, binlog.CisternOuterClass.DumpDDLJobsReq.Builder.class);
}
public static final int BEGINCOMMITTS_FIELD_NUMBER = 1;
private long beginCommitTS_;
/**
*
* beginCommitTS is the start point of drainer processing binlog, DumpDDLJobs() returns
* all history DDL jobs before this position, then drainer will apply these DDL jobs
* in order of job ID to restore the whole schema info at that moment.
*
*
* optional int64 beginCommitTS = 1;
*/
public long getBeginCommitTS() {
return beginCommitTS_;
}
private byte memoizedIsInitialized = -1;
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (beginCommitTS_ != 0L) {
output.writeInt64(1, beginCommitTS_);
}
}
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (beginCommitTS_ != 0L) {
size += com.google.protobuf.CodedOutputStream
.computeInt64Size(1, beginCommitTS_);
}
memoizedSize = size;
return size;
}
private static final long serialVersionUID = 0L;
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof binlog.CisternOuterClass.DumpDDLJobsReq)) {
return super.equals(obj);
}
binlog.CisternOuterClass.DumpDDLJobsReq other = (binlog.CisternOuterClass.DumpDDLJobsReq) obj;
boolean result = true;
result = result && (getBeginCommitTS()
== other.getBeginCommitTS());
return result;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptorForType().hashCode();
hash = (37 * hash) + BEGINCOMMITTS_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
getBeginCommitTS());
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static binlog.CisternOuterClass.DumpDDLJobsReq parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static binlog.CisternOuterClass.DumpDDLJobsReq parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static binlog.CisternOuterClass.DumpDDLJobsReq parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static binlog.CisternOuterClass.DumpDDLJobsReq parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static binlog.CisternOuterClass.DumpDDLJobsReq parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static binlog.CisternOuterClass.DumpDDLJobsReq parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static binlog.CisternOuterClass.DumpDDLJobsReq parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static binlog.CisternOuterClass.DumpDDLJobsReq parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static binlog.CisternOuterClass.DumpDDLJobsReq parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static binlog.CisternOuterClass.DumpDDLJobsReq parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(binlog.CisternOuterClass.DumpDDLJobsReq prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code binlog.DumpDDLJobsReq}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:binlog.DumpDDLJobsReq)
binlog.CisternOuterClass.DumpDDLJobsReqOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return binlog.CisternOuterClass.internal_static_binlog_DumpDDLJobsReq_descriptor;
}
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return binlog.CisternOuterClass.internal_static_binlog_DumpDDLJobsReq_fieldAccessorTable
.ensureFieldAccessorsInitialized(
binlog.CisternOuterClass.DumpDDLJobsReq.class, binlog.CisternOuterClass.DumpDDLJobsReq.Builder.class);
}
// Construct using binlog.CisternOuterClass.DumpDDLJobsReq.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
public Builder clear() {
super.clear();
beginCommitTS_ = 0L;
return this;
}
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return binlog.CisternOuterClass.internal_static_binlog_DumpDDLJobsReq_descriptor;
}
public binlog.CisternOuterClass.DumpDDLJobsReq getDefaultInstanceForType() {
return binlog.CisternOuterClass.DumpDDLJobsReq.getDefaultInstance();
}
public binlog.CisternOuterClass.DumpDDLJobsReq build() {
binlog.CisternOuterClass.DumpDDLJobsReq result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
public binlog.CisternOuterClass.DumpDDLJobsReq buildPartial() {
binlog.CisternOuterClass.DumpDDLJobsReq result = new binlog.CisternOuterClass.DumpDDLJobsReq(this);
result.beginCommitTS_ = beginCommitTS_;
onBuilt();
return result;
}
public Builder clone() {
return (Builder) super.clone();
}
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
Object value) {
return (Builder) super.setField(field, value);
}
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return (Builder) super.clearField(field);
}
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return (Builder) super.clearOneof(oneof);
}
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, Object value) {
return (Builder) super.setRepeatedField(field, index, value);
}
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
Object value) {
return (Builder) super.addRepeatedField(field, value);
}
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof binlog.CisternOuterClass.DumpDDLJobsReq) {
return mergeFrom((binlog.CisternOuterClass.DumpDDLJobsReq)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(binlog.CisternOuterClass.DumpDDLJobsReq other) {
if (other == binlog.CisternOuterClass.DumpDDLJobsReq.getDefaultInstance()) return this;
if (other.getBeginCommitTS() != 0L) {
setBeginCommitTS(other.getBeginCommitTS());
}
onChanged();
return this;
}
public final boolean isInitialized() {
return true;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
binlog.CisternOuterClass.DumpDDLJobsReq parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (binlog.CisternOuterClass.DumpDDLJobsReq) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private long beginCommitTS_ ;
/**
*
* beginCommitTS is the start point of drainer processing binlog, DumpDDLJobs() returns
* all history DDL jobs before this position, then drainer will apply these DDL jobs
* in order of job ID to restore the whole schema info at that moment.
*
*
* optional int64 beginCommitTS = 1;
*/
public long getBeginCommitTS() {
return beginCommitTS_;
}
/**
*
* beginCommitTS is the start point of drainer processing binlog, DumpDDLJobs() returns
* all history DDL jobs before this position, then drainer will apply these DDL jobs
* in order of job ID to restore the whole schema info at that moment.
*
*
* optional int64 beginCommitTS = 1;
*/
public Builder setBeginCommitTS(long value) {
beginCommitTS_ = value;
onChanged();
return this;
}
/**
*
* beginCommitTS is the start point of drainer processing binlog, DumpDDLJobs() returns
* all history DDL jobs before this position, then drainer will apply these DDL jobs
* in order of job ID to restore the whole schema info at that moment.
*
*
* optional int64 beginCommitTS = 1;
*/
public Builder clearBeginCommitTS() {
beginCommitTS_ = 0L;
onChanged();
return this;
}
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return this;
}
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return this;
}
// @@protoc_insertion_point(builder_scope:binlog.DumpDDLJobsReq)
}
// @@protoc_insertion_point(class_scope:binlog.DumpDDLJobsReq)
private static final binlog.CisternOuterClass.DumpDDLJobsReq DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new binlog.CisternOuterClass.DumpDDLJobsReq();
}
public static binlog.CisternOuterClass.DumpDDLJobsReq getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
public DumpDDLJobsReq parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new DumpDDLJobsReq(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
public binlog.CisternOuterClass.DumpDDLJobsReq getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface DumpDDLJobsRespOrBuilder extends
// @@protoc_insertion_point(interface_extends:binlog.DumpDDLJobsResp)
com.google.protobuf.MessageOrBuilder {
/**
*
* ddljobs is an array of JSON encoded history DDL jobs
*
*
* repeated bytes ddljobs = 1;
*/
java.util.List getDdljobsList();
/**
*
* ddljobs is an array of JSON encoded history DDL jobs
*
*
* repeated bytes ddljobs = 1;
*/
int getDdljobsCount();
/**
*
* ddljobs is an array of JSON encoded history DDL jobs
*
*
* repeated bytes ddljobs = 1;
*/
com.google.protobuf.ByteString getDdljobs(int index);
}
/**
* Protobuf type {@code binlog.DumpDDLJobsResp}
*/
public static final class DumpDDLJobsResp extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:binlog.DumpDDLJobsResp)
DumpDDLJobsRespOrBuilder {
// Use DumpDDLJobsResp.newBuilder() to construct.
private DumpDDLJobsResp(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private DumpDDLJobsResp() {
ddljobs_ = java.util.Collections.emptyList();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return com.google.protobuf.UnknownFieldSet.getDefaultInstance();
}
private DumpDDLJobsResp(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
int mutable_bitField0_ = 0;
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
default: {
if (!input.skipField(tag)) {
done = true;
}
break;
}
case 10: {
if (!((mutable_bitField0_ & 0x00000001) == 0x00000001)) {
ddljobs_ = new java.util.ArrayList();
mutable_bitField0_ |= 0x00000001;
}
ddljobs_.add(input.readBytes());
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
if (((mutable_bitField0_ & 0x00000001) == 0x00000001)) {
ddljobs_ = java.util.Collections.unmodifiableList(ddljobs_);
}
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return binlog.CisternOuterClass.internal_static_binlog_DumpDDLJobsResp_descriptor;
}
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return binlog.CisternOuterClass.internal_static_binlog_DumpDDLJobsResp_fieldAccessorTable
.ensureFieldAccessorsInitialized(
binlog.CisternOuterClass.DumpDDLJobsResp.class, binlog.CisternOuterClass.DumpDDLJobsResp.Builder.class);
}
public static final int DDLJOBS_FIELD_NUMBER = 1;
private java.util.List ddljobs_;
/**
*
* ddljobs is an array of JSON encoded history DDL jobs
*
*
* repeated bytes ddljobs = 1;
*/
public java.util.List
getDdljobsList() {
return ddljobs_;
}
/**
*
* ddljobs is an array of JSON encoded history DDL jobs
*
*
* repeated bytes ddljobs = 1;
*/
public int getDdljobsCount() {
return ddljobs_.size();
}
/**
*
* ddljobs is an array of JSON encoded history DDL jobs
*
*
* repeated bytes ddljobs = 1;
*/
public com.google.protobuf.ByteString getDdljobs(int index) {
return ddljobs_.get(index);
}
private byte memoizedIsInitialized = -1;
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
for (int i = 0; i < ddljobs_.size(); i++) {
output.writeBytes(1, ddljobs_.get(i));
}
}
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
{
int dataSize = 0;
for (int i = 0; i < ddljobs_.size(); i++) {
dataSize += com.google.protobuf.CodedOutputStream
.computeBytesSizeNoTag(ddljobs_.get(i));
}
size += dataSize;
size += 1 * getDdljobsList().size();
}
memoizedSize = size;
return size;
}
private static final long serialVersionUID = 0L;
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof binlog.CisternOuterClass.DumpDDLJobsResp)) {
return super.equals(obj);
}
binlog.CisternOuterClass.DumpDDLJobsResp other = (binlog.CisternOuterClass.DumpDDLJobsResp) obj;
boolean result = true;
result = result && getDdljobsList()
.equals(other.getDdljobsList());
return result;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptorForType().hashCode();
if (getDdljobsCount() > 0) {
hash = (37 * hash) + DDLJOBS_FIELD_NUMBER;
hash = (53 * hash) + getDdljobsList().hashCode();
}
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static binlog.CisternOuterClass.DumpDDLJobsResp parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static binlog.CisternOuterClass.DumpDDLJobsResp parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static binlog.CisternOuterClass.DumpDDLJobsResp parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static binlog.CisternOuterClass.DumpDDLJobsResp parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static binlog.CisternOuterClass.DumpDDLJobsResp parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static binlog.CisternOuterClass.DumpDDLJobsResp parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static binlog.CisternOuterClass.DumpDDLJobsResp parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static binlog.CisternOuterClass.DumpDDLJobsResp parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static binlog.CisternOuterClass.DumpDDLJobsResp parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static binlog.CisternOuterClass.DumpDDLJobsResp parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(binlog.CisternOuterClass.DumpDDLJobsResp prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code binlog.DumpDDLJobsResp}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:binlog.DumpDDLJobsResp)
binlog.CisternOuterClass.DumpDDLJobsRespOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return binlog.CisternOuterClass.internal_static_binlog_DumpDDLJobsResp_descriptor;
}
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return binlog.CisternOuterClass.internal_static_binlog_DumpDDLJobsResp_fieldAccessorTable
.ensureFieldAccessorsInitialized(
binlog.CisternOuterClass.DumpDDLJobsResp.class, binlog.CisternOuterClass.DumpDDLJobsResp.Builder.class);
}
// Construct using binlog.CisternOuterClass.DumpDDLJobsResp.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
public Builder clear() {
super.clear();
ddljobs_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000001);
return this;
}
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return binlog.CisternOuterClass.internal_static_binlog_DumpDDLJobsResp_descriptor;
}
public binlog.CisternOuterClass.DumpDDLJobsResp getDefaultInstanceForType() {
return binlog.CisternOuterClass.DumpDDLJobsResp.getDefaultInstance();
}
public binlog.CisternOuterClass.DumpDDLJobsResp build() {
binlog.CisternOuterClass.DumpDDLJobsResp result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
public binlog.CisternOuterClass.DumpDDLJobsResp buildPartial() {
binlog.CisternOuterClass.DumpDDLJobsResp result = new binlog.CisternOuterClass.DumpDDLJobsResp(this);
int from_bitField0_ = bitField0_;
if (((bitField0_ & 0x00000001) == 0x00000001)) {
ddljobs_ = java.util.Collections.unmodifiableList(ddljobs_);
bitField0_ = (bitField0_ & ~0x00000001);
}
result.ddljobs_ = ddljobs_;
onBuilt();
return result;
}
public Builder clone() {
return (Builder) super.clone();
}
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
Object value) {
return (Builder) super.setField(field, value);
}
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return (Builder) super.clearField(field);
}
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return (Builder) super.clearOneof(oneof);
}
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, Object value) {
return (Builder) super.setRepeatedField(field, index, value);
}
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
Object value) {
return (Builder) super.addRepeatedField(field, value);
}
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof binlog.CisternOuterClass.DumpDDLJobsResp) {
return mergeFrom((binlog.CisternOuterClass.DumpDDLJobsResp)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(binlog.CisternOuterClass.DumpDDLJobsResp other) {
if (other == binlog.CisternOuterClass.DumpDDLJobsResp.getDefaultInstance()) return this;
if (!other.ddljobs_.isEmpty()) {
if (ddljobs_.isEmpty()) {
ddljobs_ = other.ddljobs_;
bitField0_ = (bitField0_ & ~0x00000001);
} else {
ensureDdljobsIsMutable();
ddljobs_.addAll(other.ddljobs_);
}
onChanged();
}
onChanged();
return this;
}
public final boolean isInitialized() {
return true;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
binlog.CisternOuterClass.DumpDDLJobsResp parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (binlog.CisternOuterClass.DumpDDLJobsResp) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
private java.util.List ddljobs_ = java.util.Collections.emptyList();
private void ensureDdljobsIsMutable() {
if (!((bitField0_ & 0x00000001) == 0x00000001)) {
ddljobs_ = new java.util.ArrayList(ddljobs_);
bitField0_ |= 0x00000001;
}
}
/**
*
* ddljobs is an array of JSON encoded history DDL jobs
*
*
* repeated bytes ddljobs = 1;
*/
public java.util.List
getDdljobsList() {
return java.util.Collections.unmodifiableList(ddljobs_);
}
/**
*
* ddljobs is an array of JSON encoded history DDL jobs
*
*
* repeated bytes ddljobs = 1;
*/
public int getDdljobsCount() {
return ddljobs_.size();
}
/**
*
* ddljobs is an array of JSON encoded history DDL jobs
*
*
* repeated bytes ddljobs = 1;
*/
public com.google.protobuf.ByteString getDdljobs(int index) {
return ddljobs_.get(index);
}
/**
*
* ddljobs is an array of JSON encoded history DDL jobs
*
*
* repeated bytes ddljobs = 1;
*/
public Builder setDdljobs(
int index, com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
ensureDdljobsIsMutable();
ddljobs_.set(index, value);
onChanged();
return this;
}
/**
*
* ddljobs is an array of JSON encoded history DDL jobs
*
*
* repeated bytes ddljobs = 1;
*/
public Builder addDdljobs(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
ensureDdljobsIsMutable();
ddljobs_.add(value);
onChanged();
return this;
}
/**
*
* ddljobs is an array of JSON encoded history DDL jobs
*
*
* repeated bytes ddljobs = 1;
*/
public Builder addAllDdljobs(
java.lang.Iterable extends com.google.protobuf.ByteString> values) {
ensureDdljobsIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, ddljobs_);
onChanged();
return this;
}
/**
*
* ddljobs is an array of JSON encoded history DDL jobs
*
*
* repeated bytes ddljobs = 1;
*/
public Builder clearDdljobs() {
ddljobs_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000001);
onChanged();
return this;
}
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return this;
}
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return this;
}
// @@protoc_insertion_point(builder_scope:binlog.DumpDDLJobsResp)
}
// @@protoc_insertion_point(class_scope:binlog.DumpDDLJobsResp)
private static final binlog.CisternOuterClass.DumpDDLJobsResp DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new binlog.CisternOuterClass.DumpDDLJobsResp();
}
public static binlog.CisternOuterClass.DumpDDLJobsResp getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
public DumpDDLJobsResp parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new DumpDDLJobsResp(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
public binlog.CisternOuterClass.DumpDDLJobsResp getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface NotifyReqOrBuilder extends
// @@protoc_insertion_point(interface_extends:binlog.NotifyReq)
com.google.protobuf.MessageOrBuilder {
}
/**
* Protobuf type {@code binlog.NotifyReq}
*/
public static final class NotifyReq extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:binlog.NotifyReq)
NotifyReqOrBuilder {
// Use NotifyReq.newBuilder() to construct.
private NotifyReq(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private NotifyReq() {
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return com.google.protobuf.UnknownFieldSet.getDefaultInstance();
}
private NotifyReq(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
default: {
if (!input.skipField(tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return binlog.CisternOuterClass.internal_static_binlog_NotifyReq_descriptor;
}
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return binlog.CisternOuterClass.internal_static_binlog_NotifyReq_fieldAccessorTable
.ensureFieldAccessorsInitialized(
binlog.CisternOuterClass.NotifyReq.class, binlog.CisternOuterClass.NotifyReq.Builder.class);
}
private byte memoizedIsInitialized = -1;
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
}
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
memoizedSize = size;
return size;
}
private static final long serialVersionUID = 0L;
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof binlog.CisternOuterClass.NotifyReq)) {
return super.equals(obj);
}
binlog.CisternOuterClass.NotifyReq other = (binlog.CisternOuterClass.NotifyReq) obj;
boolean result = true;
return result;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptorForType().hashCode();
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static binlog.CisternOuterClass.NotifyReq parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static binlog.CisternOuterClass.NotifyReq parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static binlog.CisternOuterClass.NotifyReq parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static binlog.CisternOuterClass.NotifyReq parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static binlog.CisternOuterClass.NotifyReq parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static binlog.CisternOuterClass.NotifyReq parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static binlog.CisternOuterClass.NotifyReq parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static binlog.CisternOuterClass.NotifyReq parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static binlog.CisternOuterClass.NotifyReq parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static binlog.CisternOuterClass.NotifyReq parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(binlog.CisternOuterClass.NotifyReq prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code binlog.NotifyReq}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:binlog.NotifyReq)
binlog.CisternOuterClass.NotifyReqOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return binlog.CisternOuterClass.internal_static_binlog_NotifyReq_descriptor;
}
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return binlog.CisternOuterClass.internal_static_binlog_NotifyReq_fieldAccessorTable
.ensureFieldAccessorsInitialized(
binlog.CisternOuterClass.NotifyReq.class, binlog.CisternOuterClass.NotifyReq.Builder.class);
}
// Construct using binlog.CisternOuterClass.NotifyReq.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
public Builder clear() {
super.clear();
return this;
}
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return binlog.CisternOuterClass.internal_static_binlog_NotifyReq_descriptor;
}
public binlog.CisternOuterClass.NotifyReq getDefaultInstanceForType() {
return binlog.CisternOuterClass.NotifyReq.getDefaultInstance();
}
public binlog.CisternOuterClass.NotifyReq build() {
binlog.CisternOuterClass.NotifyReq result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
public binlog.CisternOuterClass.NotifyReq buildPartial() {
binlog.CisternOuterClass.NotifyReq result = new binlog.CisternOuterClass.NotifyReq(this);
onBuilt();
return result;
}
public Builder clone() {
return (Builder) super.clone();
}
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
Object value) {
return (Builder) super.setField(field, value);
}
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return (Builder) super.clearField(field);
}
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return (Builder) super.clearOneof(oneof);
}
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, Object value) {
return (Builder) super.setRepeatedField(field, index, value);
}
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
Object value) {
return (Builder) super.addRepeatedField(field, value);
}
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof binlog.CisternOuterClass.NotifyReq) {
return mergeFrom((binlog.CisternOuterClass.NotifyReq)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(binlog.CisternOuterClass.NotifyReq other) {
if (other == binlog.CisternOuterClass.NotifyReq.getDefaultInstance()) return this;
onChanged();
return this;
}
public final boolean isInitialized() {
return true;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
binlog.CisternOuterClass.NotifyReq parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (binlog.CisternOuterClass.NotifyReq) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return this;
}
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return this;
}
// @@protoc_insertion_point(builder_scope:binlog.NotifyReq)
}
// @@protoc_insertion_point(class_scope:binlog.NotifyReq)
private static final binlog.CisternOuterClass.NotifyReq DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new binlog.CisternOuterClass.NotifyReq();
}
public static binlog.CisternOuterClass.NotifyReq getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
public NotifyReq parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new NotifyReq(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
public binlog.CisternOuterClass.NotifyReq getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface NotifyRespOrBuilder extends
// @@protoc_insertion_point(interface_extends:binlog.NotifyResp)
com.google.protobuf.MessageOrBuilder {
}
/**
* Protobuf type {@code binlog.NotifyResp}
*/
public static final class NotifyResp extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:binlog.NotifyResp)
NotifyRespOrBuilder {
// Use NotifyResp.newBuilder() to construct.
private NotifyResp(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private NotifyResp() {
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return com.google.protobuf.UnknownFieldSet.getDefaultInstance();
}
private NotifyResp(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
default: {
if (!input.skipField(tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return binlog.CisternOuterClass.internal_static_binlog_NotifyResp_descriptor;
}
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return binlog.CisternOuterClass.internal_static_binlog_NotifyResp_fieldAccessorTable
.ensureFieldAccessorsInitialized(
binlog.CisternOuterClass.NotifyResp.class, binlog.CisternOuterClass.NotifyResp.Builder.class);
}
private byte memoizedIsInitialized = -1;
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
}
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
memoizedSize = size;
return size;
}
private static final long serialVersionUID = 0L;
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof binlog.CisternOuterClass.NotifyResp)) {
return super.equals(obj);
}
binlog.CisternOuterClass.NotifyResp other = (binlog.CisternOuterClass.NotifyResp) obj;
boolean result = true;
return result;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptorForType().hashCode();
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static binlog.CisternOuterClass.NotifyResp parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static binlog.CisternOuterClass.NotifyResp parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static binlog.CisternOuterClass.NotifyResp parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static binlog.CisternOuterClass.NotifyResp parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static binlog.CisternOuterClass.NotifyResp parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static binlog.CisternOuterClass.NotifyResp parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static binlog.CisternOuterClass.NotifyResp parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static binlog.CisternOuterClass.NotifyResp parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static binlog.CisternOuterClass.NotifyResp parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static binlog.CisternOuterClass.NotifyResp parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(binlog.CisternOuterClass.NotifyResp prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code binlog.NotifyResp}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:binlog.NotifyResp)
binlog.CisternOuterClass.NotifyRespOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return binlog.CisternOuterClass.internal_static_binlog_NotifyResp_descriptor;
}
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return binlog.CisternOuterClass.internal_static_binlog_NotifyResp_fieldAccessorTable
.ensureFieldAccessorsInitialized(
binlog.CisternOuterClass.NotifyResp.class, binlog.CisternOuterClass.NotifyResp.Builder.class);
}
// Construct using binlog.CisternOuterClass.NotifyResp.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
public Builder clear() {
super.clear();
return this;
}
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return binlog.CisternOuterClass.internal_static_binlog_NotifyResp_descriptor;
}
public binlog.CisternOuterClass.NotifyResp getDefaultInstanceForType() {
return binlog.CisternOuterClass.NotifyResp.getDefaultInstance();
}
public binlog.CisternOuterClass.NotifyResp build() {
binlog.CisternOuterClass.NotifyResp result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
public binlog.CisternOuterClass.NotifyResp buildPartial() {
binlog.CisternOuterClass.NotifyResp result = new binlog.CisternOuterClass.NotifyResp(this);
onBuilt();
return result;
}
public Builder clone() {
return (Builder) super.clone();
}
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
Object value) {
return (Builder) super.setField(field, value);
}
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return (Builder) super.clearField(field);
}
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return (Builder) super.clearOneof(oneof);
}
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, Object value) {
return (Builder) super.setRepeatedField(field, index, value);
}
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
Object value) {
return (Builder) super.addRepeatedField(field, value);
}
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof binlog.CisternOuterClass.NotifyResp) {
return mergeFrom((binlog.CisternOuterClass.NotifyResp)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(binlog.CisternOuterClass.NotifyResp other) {
if (other == binlog.CisternOuterClass.NotifyResp.getDefaultInstance()) return this;
onChanged();
return this;
}
public final boolean isInitialized() {
return true;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
binlog.CisternOuterClass.NotifyResp parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (binlog.CisternOuterClass.NotifyResp) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return this;
}
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return this;
}
// @@protoc_insertion_point(builder_scope:binlog.NotifyResp)
}
// @@protoc_insertion_point(class_scope:binlog.NotifyResp)
private static final binlog.CisternOuterClass.NotifyResp DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new binlog.CisternOuterClass.NotifyResp();
}
public static binlog.CisternOuterClass.NotifyResp getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
public NotifyResp parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new NotifyResp(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
public binlog.CisternOuterClass.NotifyResp getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_binlog_DumpBinlogReq_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_binlog_DumpBinlogReq_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_binlog_DumpBinlogResp_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_binlog_DumpBinlogResp_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_binlog_DumpDDLJobsReq_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_binlog_DumpDDLJobsReq_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_binlog_DumpDDLJobsResp_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_binlog_DumpDDLJobsResp_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_binlog_NotifyReq_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_binlog_NotifyReq_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_binlog_NotifyResp_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_binlog_NotifyResp_fieldAccessorTable;
public static com.google.protobuf.Descriptors.FileDescriptor
getDescriptor() {
return descriptor;
}
private static com.google.protobuf.Descriptors.FileDescriptor
descriptor;
static {
java.lang.String[] descriptorData = {
"\n\024binlog/cistern.proto\022\006binlog\032\024gogoprot" +
"o/gogo.proto\"&\n\rDumpBinlogReq\022\025\n\rbeginCo" +
"mmitTS\030\001 \001(\003\"C\n\016DumpBinlogResp\022\020\n\010commit" +
"TS\030\001 \001(\003\022\017\n\007payload\030\002 \001(\014\022\016\n\006ddljob\030\003 \001(" +
"\014\"\'\n\016DumpDDLJobsReq\022\025\n\rbeginCommitTS\030\001 \001" +
"(\003\"\"\n\017DumpDDLJobsResp\022\017\n\007ddljobs\030\001 \003(\014\"\013" +
"\n\tNotifyReq\"\014\n\nNotifyResp2\277\001\n\007Cistern\022?\n" +
"\nDumpBinlog\022\025.binlog.DumpBinlogReq\032\026.bin" +
"log.DumpBinlogResp\"\0000\001\022@\n\013DumpDDLJobs\022\026." +
"binlog.DumpDDLJobsReq\032\027.binlog.DumpDDLJo",
"bsResp\"\000\0221\n\006Notify\022\021.binlog.NotifyReq\032\022." +
"binlog.NotifyResp\"\000B\014\310\342\036\001\320\342\036\001\340\342\036\001b\006proto" +
"3"
};
com.google.protobuf.Descriptors.FileDescriptor.InternalDescriptorAssigner assigner =
new com.google.protobuf.Descriptors.FileDescriptor. InternalDescriptorAssigner() {
public com.google.protobuf.ExtensionRegistry assignDescriptors(
com.google.protobuf.Descriptors.FileDescriptor root) {
descriptor = root;
return null;
}
};
com.google.protobuf.Descriptors.FileDescriptor
.internalBuildGeneratedFileFrom(descriptorData,
new com.google.protobuf.Descriptors.FileDescriptor[] {
com.google.protobuf.GoGoProtos.getDescriptor(),
}, assigner);
internal_static_binlog_DumpBinlogReq_descriptor =
getDescriptor().getMessageTypes().get(0);
internal_static_binlog_DumpBinlogReq_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_binlog_DumpBinlogReq_descriptor,
new java.lang.String[] { "BeginCommitTS", });
internal_static_binlog_DumpBinlogResp_descriptor =
getDescriptor().getMessageTypes().get(1);
internal_static_binlog_DumpBinlogResp_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_binlog_DumpBinlogResp_descriptor,
new java.lang.String[] { "CommitTS", "Payload", "Ddljob", });
internal_static_binlog_DumpDDLJobsReq_descriptor =
getDescriptor().getMessageTypes().get(2);
internal_static_binlog_DumpDDLJobsReq_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_binlog_DumpDDLJobsReq_descriptor,
new java.lang.String[] { "BeginCommitTS", });
internal_static_binlog_DumpDDLJobsResp_descriptor =
getDescriptor().getMessageTypes().get(3);
internal_static_binlog_DumpDDLJobsResp_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_binlog_DumpDDLJobsResp_descriptor,
new java.lang.String[] { "Ddljobs", });
internal_static_binlog_NotifyReq_descriptor =
getDescriptor().getMessageTypes().get(4);
internal_static_binlog_NotifyReq_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_binlog_NotifyReq_descriptor,
new java.lang.String[] { });
internal_static_binlog_NotifyResp_descriptor =
getDescriptor().getMessageTypes().get(5);
internal_static_binlog_NotifyResp_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_binlog_NotifyResp_descriptor,
new java.lang.String[] { });
com.google.protobuf.ExtensionRegistry registry =
com.google.protobuf.ExtensionRegistry.newInstance();
registry.add(com.google.protobuf.GoGoProtos.marshalerAll);
registry.add(com.google.protobuf.GoGoProtos.sizerAll);
registry.add(com.google.protobuf.GoGoProtos.unmarshalerAll);
com.google.protobuf.Descriptors.FileDescriptor
.internalUpdateFileDescriptor(descriptor, registry);
com.google.protobuf.GoGoProtos.getDescriptor();
}
// @@protoc_insertion_point(outer_class_scope)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy