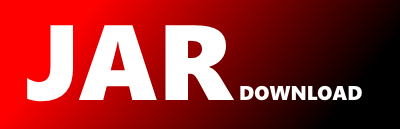
binlog.BinlogOuterClass Maven / Gradle / Ivy
// Generated by the protocol buffer compiler. DO NOT EDIT!
// source: binlog/binlog.proto
package binlog;
public final class BinlogOuterClass {
private BinlogOuterClass() {}
public static void registerAllExtensions(
com.google.protobuf.ExtensionRegistryLite registry) {
}
public static void registerAllExtensions(
com.google.protobuf.ExtensionRegistry registry) {
registerAllExtensions(
(com.google.protobuf.ExtensionRegistryLite) registry);
}
/**
* Protobuf enum {@code binlog.MutationType}
*/
public enum MutationType
implements com.google.protobuf.ProtocolMessageEnum {
/**
* Insert = 0;
*/
Insert(0),
/**
* Update = 1;
*/
Update(1),
/**
*
* Obsolete field.
*
*
* DeleteID = 2;
*/
DeleteID(2),
/**
*
* Obsolete field.
*
*
* DeletePK = 3;
*/
DeletePK(3),
/**
* DeleteRow = 4;
*/
DeleteRow(4),
;
/**
* Insert = 0;
*/
public static final int Insert_VALUE = 0;
/**
* Update = 1;
*/
public static final int Update_VALUE = 1;
/**
*
* Obsolete field.
*
*
* DeleteID = 2;
*/
public static final int DeleteID_VALUE = 2;
/**
*
* Obsolete field.
*
*
* DeletePK = 3;
*/
public static final int DeletePK_VALUE = 3;
/**
* DeleteRow = 4;
*/
public static final int DeleteRow_VALUE = 4;
public final int getNumber() {
return value;
}
/**
* @deprecated Use {@link #forNumber(int)} instead.
*/
@java.lang.Deprecated
public static MutationType valueOf(int value) {
return forNumber(value);
}
public static MutationType forNumber(int value) {
switch (value) {
case 0: return Insert;
case 1: return Update;
case 2: return DeleteID;
case 3: return DeletePK;
case 4: return DeleteRow;
default: return null;
}
}
public static com.google.protobuf.Internal.EnumLiteMap
internalGetValueMap() {
return internalValueMap;
}
private static final com.google.protobuf.Internal.EnumLiteMap<
MutationType> internalValueMap =
new com.google.protobuf.Internal.EnumLiteMap() {
public MutationType findValueByNumber(int number) {
return MutationType.forNumber(number);
}
};
public final com.google.protobuf.Descriptors.EnumValueDescriptor
getValueDescriptor() {
return getDescriptor().getValues().get(ordinal());
}
public final com.google.protobuf.Descriptors.EnumDescriptor
getDescriptorForType() {
return getDescriptor();
}
public static final com.google.protobuf.Descriptors.EnumDescriptor
getDescriptor() {
return binlog.BinlogOuterClass.getDescriptor().getEnumTypes().get(0);
}
private static final MutationType[] VALUES = values();
public static MutationType valueOf(
com.google.protobuf.Descriptors.EnumValueDescriptor desc) {
if (desc.getType() != getDescriptor()) {
throw new java.lang.IllegalArgumentException(
"EnumValueDescriptor is not for this type.");
}
return VALUES[desc.getIndex()];
}
private final int value;
private MutationType(int value) {
this.value = value;
}
// @@protoc_insertion_point(enum_scope:binlog.MutationType)
}
/**
* Protobuf enum {@code binlog.BinlogType}
*/
public enum BinlogType
implements com.google.protobuf.ProtocolMessageEnum {
/**
*
* has start_ts, prewrite_key, prewrite_value.
*
*
* Prewrite = 0;
*/
Prewrite(0),
/**
*
* has start_ts, commit_ts.
*
*
* Commit = 1;
*/
Commit(1),
/**
*
* has start_ts.
*
*
* Rollback = 2;
*/
Rollback(2),
/**
*
* Obsolete field.
*
*
* PreDDL = 3;
*/
PreDDL(3),
/**
*
* Obsolete field.
*
*
* PostDDL = 4;
*/
PostDDL(4),
;
/**
*
* has start_ts, prewrite_key, prewrite_value.
*
*
* Prewrite = 0;
*/
public static final int Prewrite_VALUE = 0;
/**
*
* has start_ts, commit_ts.
*
*
* Commit = 1;
*/
public static final int Commit_VALUE = 1;
/**
*
* has start_ts.
*
*
* Rollback = 2;
*/
public static final int Rollback_VALUE = 2;
/**
*
* Obsolete field.
*
*
* PreDDL = 3;
*/
public static final int PreDDL_VALUE = 3;
/**
*
* Obsolete field.
*
*
* PostDDL = 4;
*/
public static final int PostDDL_VALUE = 4;
public final int getNumber() {
return value;
}
/**
* @deprecated Use {@link #forNumber(int)} instead.
*/
@java.lang.Deprecated
public static BinlogType valueOf(int value) {
return forNumber(value);
}
public static BinlogType forNumber(int value) {
switch (value) {
case 0: return Prewrite;
case 1: return Commit;
case 2: return Rollback;
case 3: return PreDDL;
case 4: return PostDDL;
default: return null;
}
}
public static com.google.protobuf.Internal.EnumLiteMap
internalGetValueMap() {
return internalValueMap;
}
private static final com.google.protobuf.Internal.EnumLiteMap<
BinlogType> internalValueMap =
new com.google.protobuf.Internal.EnumLiteMap() {
public BinlogType findValueByNumber(int number) {
return BinlogType.forNumber(number);
}
};
public final com.google.protobuf.Descriptors.EnumValueDescriptor
getValueDescriptor() {
return getDescriptor().getValues().get(ordinal());
}
public final com.google.protobuf.Descriptors.EnumDescriptor
getDescriptorForType() {
return getDescriptor();
}
public static final com.google.protobuf.Descriptors.EnumDescriptor
getDescriptor() {
return binlog.BinlogOuterClass.getDescriptor().getEnumTypes().get(1);
}
private static final BinlogType[] VALUES = values();
public static BinlogType valueOf(
com.google.protobuf.Descriptors.EnumValueDescriptor desc) {
if (desc.getType() != getDescriptor()) {
throw new java.lang.IllegalArgumentException(
"EnumValueDescriptor is not for this type.");
}
return VALUES[desc.getIndex()];
}
private final int value;
private BinlogType(int value) {
this.value = value;
}
// @@protoc_insertion_point(enum_scope:binlog.BinlogType)
}
public interface TableMutationOrBuilder extends
// @@protoc_insertion_point(interface_extends:binlog.TableMutation)
com.google.protobuf.MessageOrBuilder {
/**
* optional int64 table_id = 1 [(.gogoproto.nullable) = false];
*/
boolean hasTableId();
/**
* optional int64 table_id = 1 [(.gogoproto.nullable) = false];
*/
long getTableId();
/**
*
* The inserted row contains all column values.
*
*
* repeated bytes inserted_rows = 2;
*/
java.util.List getInsertedRowsList();
/**
*
* The inserted row contains all column values.
*
*
* repeated bytes inserted_rows = 2;
*/
int getInsertedRowsCount();
/**
*
* The inserted row contains all column values.
*
*
* repeated bytes inserted_rows = 2;
*/
com.google.protobuf.ByteString getInsertedRows(int index);
/**
*
* The updated row contains old values and new values of the row.
*
*
* repeated bytes updated_rows = 3;
*/
java.util.List getUpdatedRowsList();
/**
*
* The updated row contains old values and new values of the row.
*
*
* repeated bytes updated_rows = 3;
*/
int getUpdatedRowsCount();
/**
*
* The updated row contains old values and new values of the row.
*
*
* repeated bytes updated_rows = 3;
*/
com.google.protobuf.ByteString getUpdatedRows(int index);
/**
*
* Obsolete field.
*
*
* repeated int64 deleted_ids = 4;
*/
java.util.List getDeletedIdsList();
/**
*
* Obsolete field.
*
*
* repeated int64 deleted_ids = 4;
*/
int getDeletedIdsCount();
/**
*
* Obsolete field.
*
*
* repeated int64 deleted_ids = 4;
*/
long getDeletedIds(int index);
/**
*
* Obsolete field.
*
*
* repeated bytes deleted_pks = 5;
*/
java.util.List getDeletedPksList();
/**
*
* Obsolete field.
*
*
* repeated bytes deleted_pks = 5;
*/
int getDeletedPksCount();
/**
*
* Obsolete field.
*
*
* repeated bytes deleted_pks = 5;
*/
com.google.protobuf.ByteString getDeletedPks(int index);
/**
*
* The row value of the deleted row.
*
*
* repeated bytes deleted_rows = 6;
*/
java.util.List getDeletedRowsList();
/**
*
* The row value of the deleted row.
*
*
* repeated bytes deleted_rows = 6;
*/
int getDeletedRowsCount();
/**
*
* The row value of the deleted row.
*
*
* repeated bytes deleted_rows = 6;
*/
com.google.protobuf.ByteString getDeletedRows(int index);
/**
*
* Used to apply table mutations in original sequence.
*
*
* repeated .binlog.MutationType sequence = 7;
*/
java.util.List getSequenceList();
/**
*
* Used to apply table mutations in original sequence.
*
*
* repeated .binlog.MutationType sequence = 7;
*/
int getSequenceCount();
/**
*
* Used to apply table mutations in original sequence.
*
*
* repeated .binlog.MutationType sequence = 7;
*/
binlog.BinlogOuterClass.MutationType getSequence(int index);
}
/**
*
* TableMutation contains mutations in a table.
*
*
* Protobuf type {@code binlog.TableMutation}
*/
public static final class TableMutation extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:binlog.TableMutation)
TableMutationOrBuilder {
private static final long serialVersionUID = 0L;
// Use TableMutation.newBuilder() to construct.
private TableMutation(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private TableMutation() {
tableId_ = 0L;
insertedRows_ = java.util.Collections.emptyList();
updatedRows_ = java.util.Collections.emptyList();
deletedIds_ = java.util.Collections.emptyList();
deletedPks_ = java.util.Collections.emptyList();
deletedRows_ = java.util.Collections.emptyList();
sequence_ = java.util.Collections.emptyList();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private TableMutation(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
default: {
if (!parseUnknownField(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
case 8: {
bitField0_ |= 0x00000001;
tableId_ = input.readInt64();
break;
}
case 18: {
if (!((mutable_bitField0_ & 0x00000002) == 0x00000002)) {
insertedRows_ = new java.util.ArrayList();
mutable_bitField0_ |= 0x00000002;
}
insertedRows_.add(input.readBytes());
break;
}
case 26: {
if (!((mutable_bitField0_ & 0x00000004) == 0x00000004)) {
updatedRows_ = new java.util.ArrayList();
mutable_bitField0_ |= 0x00000004;
}
updatedRows_.add(input.readBytes());
break;
}
case 32: {
if (!((mutable_bitField0_ & 0x00000008) == 0x00000008)) {
deletedIds_ = new java.util.ArrayList();
mutable_bitField0_ |= 0x00000008;
}
deletedIds_.add(input.readInt64());
break;
}
case 34: {
int length = input.readRawVarint32();
int limit = input.pushLimit(length);
if (!((mutable_bitField0_ & 0x00000008) == 0x00000008) && input.getBytesUntilLimit() > 0) {
deletedIds_ = new java.util.ArrayList();
mutable_bitField0_ |= 0x00000008;
}
while (input.getBytesUntilLimit() > 0) {
deletedIds_.add(input.readInt64());
}
input.popLimit(limit);
break;
}
case 42: {
if (!((mutable_bitField0_ & 0x00000010) == 0x00000010)) {
deletedPks_ = new java.util.ArrayList();
mutable_bitField0_ |= 0x00000010;
}
deletedPks_.add(input.readBytes());
break;
}
case 50: {
if (!((mutable_bitField0_ & 0x00000020) == 0x00000020)) {
deletedRows_ = new java.util.ArrayList();
mutable_bitField0_ |= 0x00000020;
}
deletedRows_.add(input.readBytes());
break;
}
case 56: {
int rawValue = input.readEnum();
binlog.BinlogOuterClass.MutationType value = binlog.BinlogOuterClass.MutationType.valueOf(rawValue);
if (value == null) {
unknownFields.mergeVarintField(7, rawValue);
} else {
if (!((mutable_bitField0_ & 0x00000040) == 0x00000040)) {
sequence_ = new java.util.ArrayList();
mutable_bitField0_ |= 0x00000040;
}
sequence_.add(rawValue);
}
break;
}
case 58: {
int length = input.readRawVarint32();
int oldLimit = input.pushLimit(length);
while(input.getBytesUntilLimit() > 0) {
int rawValue = input.readEnum();
binlog.BinlogOuterClass.MutationType value = binlog.BinlogOuterClass.MutationType.valueOf(rawValue);
if (value == null) {
unknownFields.mergeVarintField(7, rawValue);
} else {
if (!((mutable_bitField0_ & 0x00000040) == 0x00000040)) {
sequence_ = new java.util.ArrayList();
mutable_bitField0_ |= 0x00000040;
}
sequence_.add(rawValue);
}
}
input.popLimit(oldLimit);
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
if (((mutable_bitField0_ & 0x00000002) == 0x00000002)) {
insertedRows_ = java.util.Collections.unmodifiableList(insertedRows_);
}
if (((mutable_bitField0_ & 0x00000004) == 0x00000004)) {
updatedRows_ = java.util.Collections.unmodifiableList(updatedRows_);
}
if (((mutable_bitField0_ & 0x00000008) == 0x00000008)) {
deletedIds_ = java.util.Collections.unmodifiableList(deletedIds_);
}
if (((mutable_bitField0_ & 0x00000010) == 0x00000010)) {
deletedPks_ = java.util.Collections.unmodifiableList(deletedPks_);
}
if (((mutable_bitField0_ & 0x00000020) == 0x00000020)) {
deletedRows_ = java.util.Collections.unmodifiableList(deletedRows_);
}
if (((mutable_bitField0_ & 0x00000040) == 0x00000040)) {
sequence_ = java.util.Collections.unmodifiableList(sequence_);
}
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return binlog.BinlogOuterClass.internal_static_binlog_TableMutation_descriptor;
}
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return binlog.BinlogOuterClass.internal_static_binlog_TableMutation_fieldAccessorTable
.ensureFieldAccessorsInitialized(
binlog.BinlogOuterClass.TableMutation.class, binlog.BinlogOuterClass.TableMutation.Builder.class);
}
private int bitField0_;
public static final int TABLE_ID_FIELD_NUMBER = 1;
private long tableId_;
/**
* optional int64 table_id = 1 [(.gogoproto.nullable) = false];
*/
public boolean hasTableId() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
/**
* optional int64 table_id = 1 [(.gogoproto.nullable) = false];
*/
public long getTableId() {
return tableId_;
}
public static final int INSERTED_ROWS_FIELD_NUMBER = 2;
private java.util.List insertedRows_;
/**
*
* The inserted row contains all column values.
*
*
* repeated bytes inserted_rows = 2;
*/
public java.util.List
getInsertedRowsList() {
return insertedRows_;
}
/**
*
* The inserted row contains all column values.
*
*
* repeated bytes inserted_rows = 2;
*/
public int getInsertedRowsCount() {
return insertedRows_.size();
}
/**
*
* The inserted row contains all column values.
*
*
* repeated bytes inserted_rows = 2;
*/
public com.google.protobuf.ByteString getInsertedRows(int index) {
return insertedRows_.get(index);
}
public static final int UPDATED_ROWS_FIELD_NUMBER = 3;
private java.util.List updatedRows_;
/**
*
* The updated row contains old values and new values of the row.
*
*
* repeated bytes updated_rows = 3;
*/
public java.util.List
getUpdatedRowsList() {
return updatedRows_;
}
/**
*
* The updated row contains old values and new values of the row.
*
*
* repeated bytes updated_rows = 3;
*/
public int getUpdatedRowsCount() {
return updatedRows_.size();
}
/**
*
* The updated row contains old values and new values of the row.
*
*
* repeated bytes updated_rows = 3;
*/
public com.google.protobuf.ByteString getUpdatedRows(int index) {
return updatedRows_.get(index);
}
public static final int DELETED_IDS_FIELD_NUMBER = 4;
private java.util.List deletedIds_;
/**
*
* Obsolete field.
*
*
* repeated int64 deleted_ids = 4;
*/
public java.util.List
getDeletedIdsList() {
return deletedIds_;
}
/**
*
* Obsolete field.
*
*
* repeated int64 deleted_ids = 4;
*/
public int getDeletedIdsCount() {
return deletedIds_.size();
}
/**
*
* Obsolete field.
*
*
* repeated int64 deleted_ids = 4;
*/
public long getDeletedIds(int index) {
return deletedIds_.get(index);
}
public static final int DELETED_PKS_FIELD_NUMBER = 5;
private java.util.List deletedPks_;
/**
*
* Obsolete field.
*
*
* repeated bytes deleted_pks = 5;
*/
public java.util.List
getDeletedPksList() {
return deletedPks_;
}
/**
*
* Obsolete field.
*
*
* repeated bytes deleted_pks = 5;
*/
public int getDeletedPksCount() {
return deletedPks_.size();
}
/**
*
* Obsolete field.
*
*
* repeated bytes deleted_pks = 5;
*/
public com.google.protobuf.ByteString getDeletedPks(int index) {
return deletedPks_.get(index);
}
public static final int DELETED_ROWS_FIELD_NUMBER = 6;
private java.util.List deletedRows_;
/**
*
* The row value of the deleted row.
*
*
* repeated bytes deleted_rows = 6;
*/
public java.util.List
getDeletedRowsList() {
return deletedRows_;
}
/**
*
* The row value of the deleted row.
*
*
* repeated bytes deleted_rows = 6;
*/
public int getDeletedRowsCount() {
return deletedRows_.size();
}
/**
*
* The row value of the deleted row.
*
*
* repeated bytes deleted_rows = 6;
*/
public com.google.protobuf.ByteString getDeletedRows(int index) {
return deletedRows_.get(index);
}
public static final int SEQUENCE_FIELD_NUMBER = 7;
private java.util.List sequence_;
private static final com.google.protobuf.Internal.ListAdapter.Converter<
java.lang.Integer, binlog.BinlogOuterClass.MutationType> sequence_converter_ =
new com.google.protobuf.Internal.ListAdapter.Converter<
java.lang.Integer, binlog.BinlogOuterClass.MutationType>() {
public binlog.BinlogOuterClass.MutationType convert(java.lang.Integer from) {
binlog.BinlogOuterClass.MutationType result = binlog.BinlogOuterClass.MutationType.valueOf(from);
return result == null ? binlog.BinlogOuterClass.MutationType.Insert : result;
}
};
/**
*
* Used to apply table mutations in original sequence.
*
*
* repeated .binlog.MutationType sequence = 7;
*/
public java.util.List getSequenceList() {
return new com.google.protobuf.Internal.ListAdapter<
java.lang.Integer, binlog.BinlogOuterClass.MutationType>(sequence_, sequence_converter_);
}
/**
*
* Used to apply table mutations in original sequence.
*
*
* repeated .binlog.MutationType sequence = 7;
*/
public int getSequenceCount() {
return sequence_.size();
}
/**
*
* Used to apply table mutations in original sequence.
*
*
* repeated .binlog.MutationType sequence = 7;
*/
public binlog.BinlogOuterClass.MutationType getSequence(int index) {
return sequence_converter_.convert(sequence_.get(index));
}
private byte memoizedIsInitialized = -1;
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (((bitField0_ & 0x00000001) == 0x00000001)) {
output.writeInt64(1, tableId_);
}
for (int i = 0; i < insertedRows_.size(); i++) {
output.writeBytes(2, insertedRows_.get(i));
}
for (int i = 0; i < updatedRows_.size(); i++) {
output.writeBytes(3, updatedRows_.get(i));
}
for (int i = 0; i < deletedIds_.size(); i++) {
output.writeInt64(4, deletedIds_.get(i));
}
for (int i = 0; i < deletedPks_.size(); i++) {
output.writeBytes(5, deletedPks_.get(i));
}
for (int i = 0; i < deletedRows_.size(); i++) {
output.writeBytes(6, deletedRows_.get(i));
}
for (int i = 0; i < sequence_.size(); i++) {
output.writeEnum(7, sequence_.get(i));
}
unknownFields.writeTo(output);
}
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (((bitField0_ & 0x00000001) == 0x00000001)) {
size += com.google.protobuf.CodedOutputStream
.computeInt64Size(1, tableId_);
}
{
int dataSize = 0;
for (int i = 0; i < insertedRows_.size(); i++) {
dataSize += com.google.protobuf.CodedOutputStream
.computeBytesSizeNoTag(insertedRows_.get(i));
}
size += dataSize;
size += 1 * getInsertedRowsList().size();
}
{
int dataSize = 0;
for (int i = 0; i < updatedRows_.size(); i++) {
dataSize += com.google.protobuf.CodedOutputStream
.computeBytesSizeNoTag(updatedRows_.get(i));
}
size += dataSize;
size += 1 * getUpdatedRowsList().size();
}
{
int dataSize = 0;
for (int i = 0; i < deletedIds_.size(); i++) {
dataSize += com.google.protobuf.CodedOutputStream
.computeInt64SizeNoTag(deletedIds_.get(i));
}
size += dataSize;
size += 1 * getDeletedIdsList().size();
}
{
int dataSize = 0;
for (int i = 0; i < deletedPks_.size(); i++) {
dataSize += com.google.protobuf.CodedOutputStream
.computeBytesSizeNoTag(deletedPks_.get(i));
}
size += dataSize;
size += 1 * getDeletedPksList().size();
}
{
int dataSize = 0;
for (int i = 0; i < deletedRows_.size(); i++) {
dataSize += com.google.protobuf.CodedOutputStream
.computeBytesSizeNoTag(deletedRows_.get(i));
}
size += dataSize;
size += 1 * getDeletedRowsList().size();
}
{
int dataSize = 0;
for (int i = 0; i < sequence_.size(); i++) {
dataSize += com.google.protobuf.CodedOutputStream
.computeEnumSizeNoTag(sequence_.get(i));
}
size += dataSize;
size += 1 * sequence_.size();
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof binlog.BinlogOuterClass.TableMutation)) {
return super.equals(obj);
}
binlog.BinlogOuterClass.TableMutation other = (binlog.BinlogOuterClass.TableMutation) obj;
boolean result = true;
result = result && (hasTableId() == other.hasTableId());
if (hasTableId()) {
result = result && (getTableId()
== other.getTableId());
}
result = result && getInsertedRowsList()
.equals(other.getInsertedRowsList());
result = result && getUpdatedRowsList()
.equals(other.getUpdatedRowsList());
result = result && getDeletedIdsList()
.equals(other.getDeletedIdsList());
result = result && getDeletedPksList()
.equals(other.getDeletedPksList());
result = result && getDeletedRowsList()
.equals(other.getDeletedRowsList());
result = result && sequence_.equals(other.sequence_);
result = result && unknownFields.equals(other.unknownFields);
return result;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (hasTableId()) {
hash = (37 * hash) + TABLE_ID_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
getTableId());
}
if (getInsertedRowsCount() > 0) {
hash = (37 * hash) + INSERTED_ROWS_FIELD_NUMBER;
hash = (53 * hash) + getInsertedRowsList().hashCode();
}
if (getUpdatedRowsCount() > 0) {
hash = (37 * hash) + UPDATED_ROWS_FIELD_NUMBER;
hash = (53 * hash) + getUpdatedRowsList().hashCode();
}
if (getDeletedIdsCount() > 0) {
hash = (37 * hash) + DELETED_IDS_FIELD_NUMBER;
hash = (53 * hash) + getDeletedIdsList().hashCode();
}
if (getDeletedPksCount() > 0) {
hash = (37 * hash) + DELETED_PKS_FIELD_NUMBER;
hash = (53 * hash) + getDeletedPksList().hashCode();
}
if (getDeletedRowsCount() > 0) {
hash = (37 * hash) + DELETED_ROWS_FIELD_NUMBER;
hash = (53 * hash) + getDeletedRowsList().hashCode();
}
if (getSequenceCount() > 0) {
hash = (37 * hash) + SEQUENCE_FIELD_NUMBER;
hash = (53 * hash) + sequence_.hashCode();
}
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static binlog.BinlogOuterClass.TableMutation parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static binlog.BinlogOuterClass.TableMutation parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static binlog.BinlogOuterClass.TableMutation parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static binlog.BinlogOuterClass.TableMutation parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static binlog.BinlogOuterClass.TableMutation parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static binlog.BinlogOuterClass.TableMutation parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static binlog.BinlogOuterClass.TableMutation parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static binlog.BinlogOuterClass.TableMutation parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static binlog.BinlogOuterClass.TableMutation parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static binlog.BinlogOuterClass.TableMutation parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static binlog.BinlogOuterClass.TableMutation parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static binlog.BinlogOuterClass.TableMutation parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(binlog.BinlogOuterClass.TableMutation prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
* TableMutation contains mutations in a table.
*
*
* Protobuf type {@code binlog.TableMutation}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:binlog.TableMutation)
binlog.BinlogOuterClass.TableMutationOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return binlog.BinlogOuterClass.internal_static_binlog_TableMutation_descriptor;
}
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return binlog.BinlogOuterClass.internal_static_binlog_TableMutation_fieldAccessorTable
.ensureFieldAccessorsInitialized(
binlog.BinlogOuterClass.TableMutation.class, binlog.BinlogOuterClass.TableMutation.Builder.class);
}
// Construct using binlog.BinlogOuterClass.TableMutation.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
public Builder clear() {
super.clear();
tableId_ = 0L;
bitField0_ = (bitField0_ & ~0x00000001);
insertedRows_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000002);
updatedRows_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000004);
deletedIds_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000008);
deletedPks_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000010);
deletedRows_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000020);
sequence_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000040);
return this;
}
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return binlog.BinlogOuterClass.internal_static_binlog_TableMutation_descriptor;
}
public binlog.BinlogOuterClass.TableMutation getDefaultInstanceForType() {
return binlog.BinlogOuterClass.TableMutation.getDefaultInstance();
}
public binlog.BinlogOuterClass.TableMutation build() {
binlog.BinlogOuterClass.TableMutation result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
public binlog.BinlogOuterClass.TableMutation buildPartial() {
binlog.BinlogOuterClass.TableMutation result = new binlog.BinlogOuterClass.TableMutation(this);
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (((from_bitField0_ & 0x00000001) == 0x00000001)) {
to_bitField0_ |= 0x00000001;
}
result.tableId_ = tableId_;
if (((bitField0_ & 0x00000002) == 0x00000002)) {
insertedRows_ = java.util.Collections.unmodifiableList(insertedRows_);
bitField0_ = (bitField0_ & ~0x00000002);
}
result.insertedRows_ = insertedRows_;
if (((bitField0_ & 0x00000004) == 0x00000004)) {
updatedRows_ = java.util.Collections.unmodifiableList(updatedRows_);
bitField0_ = (bitField0_ & ~0x00000004);
}
result.updatedRows_ = updatedRows_;
if (((bitField0_ & 0x00000008) == 0x00000008)) {
deletedIds_ = java.util.Collections.unmodifiableList(deletedIds_);
bitField0_ = (bitField0_ & ~0x00000008);
}
result.deletedIds_ = deletedIds_;
if (((bitField0_ & 0x00000010) == 0x00000010)) {
deletedPks_ = java.util.Collections.unmodifiableList(deletedPks_);
bitField0_ = (bitField0_ & ~0x00000010);
}
result.deletedPks_ = deletedPks_;
if (((bitField0_ & 0x00000020) == 0x00000020)) {
deletedRows_ = java.util.Collections.unmodifiableList(deletedRows_);
bitField0_ = (bitField0_ & ~0x00000020);
}
result.deletedRows_ = deletedRows_;
if (((bitField0_ & 0x00000040) == 0x00000040)) {
sequence_ = java.util.Collections.unmodifiableList(sequence_);
bitField0_ = (bitField0_ & ~0x00000040);
}
result.sequence_ = sequence_;
result.bitField0_ = to_bitField0_;
onBuilt();
return result;
}
public Builder clone() {
return (Builder) super.clone();
}
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.setField(field, value);
}
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return (Builder) super.clearField(field);
}
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return (Builder) super.clearOneof(oneof);
}
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return (Builder) super.setRepeatedField(field, index, value);
}
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.addRepeatedField(field, value);
}
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof binlog.BinlogOuterClass.TableMutation) {
return mergeFrom((binlog.BinlogOuterClass.TableMutation)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(binlog.BinlogOuterClass.TableMutation other) {
if (other == binlog.BinlogOuterClass.TableMutation.getDefaultInstance()) return this;
if (other.hasTableId()) {
setTableId(other.getTableId());
}
if (!other.insertedRows_.isEmpty()) {
if (insertedRows_.isEmpty()) {
insertedRows_ = other.insertedRows_;
bitField0_ = (bitField0_ & ~0x00000002);
} else {
ensureInsertedRowsIsMutable();
insertedRows_.addAll(other.insertedRows_);
}
onChanged();
}
if (!other.updatedRows_.isEmpty()) {
if (updatedRows_.isEmpty()) {
updatedRows_ = other.updatedRows_;
bitField0_ = (bitField0_ & ~0x00000004);
} else {
ensureUpdatedRowsIsMutable();
updatedRows_.addAll(other.updatedRows_);
}
onChanged();
}
if (!other.deletedIds_.isEmpty()) {
if (deletedIds_.isEmpty()) {
deletedIds_ = other.deletedIds_;
bitField0_ = (bitField0_ & ~0x00000008);
} else {
ensureDeletedIdsIsMutable();
deletedIds_.addAll(other.deletedIds_);
}
onChanged();
}
if (!other.deletedPks_.isEmpty()) {
if (deletedPks_.isEmpty()) {
deletedPks_ = other.deletedPks_;
bitField0_ = (bitField0_ & ~0x00000010);
} else {
ensureDeletedPksIsMutable();
deletedPks_.addAll(other.deletedPks_);
}
onChanged();
}
if (!other.deletedRows_.isEmpty()) {
if (deletedRows_.isEmpty()) {
deletedRows_ = other.deletedRows_;
bitField0_ = (bitField0_ & ~0x00000020);
} else {
ensureDeletedRowsIsMutable();
deletedRows_.addAll(other.deletedRows_);
}
onChanged();
}
if (!other.sequence_.isEmpty()) {
if (sequence_.isEmpty()) {
sequence_ = other.sequence_;
bitField0_ = (bitField0_ & ~0x00000040);
} else {
ensureSequenceIsMutable();
sequence_.addAll(other.sequence_);
}
onChanged();
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
public final boolean isInitialized() {
return true;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
binlog.BinlogOuterClass.TableMutation parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (binlog.BinlogOuterClass.TableMutation) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
private long tableId_ ;
/**
* optional int64 table_id = 1 [(.gogoproto.nullable) = false];
*/
public boolean hasTableId() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
/**
* optional int64 table_id = 1 [(.gogoproto.nullable) = false];
*/
public long getTableId() {
return tableId_;
}
/**
* optional int64 table_id = 1 [(.gogoproto.nullable) = false];
*/
public Builder setTableId(long value) {
bitField0_ |= 0x00000001;
tableId_ = value;
onChanged();
return this;
}
/**
* optional int64 table_id = 1 [(.gogoproto.nullable) = false];
*/
public Builder clearTableId() {
bitField0_ = (bitField0_ & ~0x00000001);
tableId_ = 0L;
onChanged();
return this;
}
private java.util.List insertedRows_ = java.util.Collections.emptyList();
private void ensureInsertedRowsIsMutable() {
if (!((bitField0_ & 0x00000002) == 0x00000002)) {
insertedRows_ = new java.util.ArrayList(insertedRows_);
bitField0_ |= 0x00000002;
}
}
/**
*
* The inserted row contains all column values.
*
*
* repeated bytes inserted_rows = 2;
*/
public java.util.List
getInsertedRowsList() {
return java.util.Collections.unmodifiableList(insertedRows_);
}
/**
*
* The inserted row contains all column values.
*
*
* repeated bytes inserted_rows = 2;
*/
public int getInsertedRowsCount() {
return insertedRows_.size();
}
/**
*
* The inserted row contains all column values.
*
*
* repeated bytes inserted_rows = 2;
*/
public com.google.protobuf.ByteString getInsertedRows(int index) {
return insertedRows_.get(index);
}
/**
*
* The inserted row contains all column values.
*
*
* repeated bytes inserted_rows = 2;
*/
public Builder setInsertedRows(
int index, com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
ensureInsertedRowsIsMutable();
insertedRows_.set(index, value);
onChanged();
return this;
}
/**
*
* The inserted row contains all column values.
*
*
* repeated bytes inserted_rows = 2;
*/
public Builder addInsertedRows(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
ensureInsertedRowsIsMutable();
insertedRows_.add(value);
onChanged();
return this;
}
/**
*
* The inserted row contains all column values.
*
*
* repeated bytes inserted_rows = 2;
*/
public Builder addAllInsertedRows(
java.lang.Iterable extends com.google.protobuf.ByteString> values) {
ensureInsertedRowsIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, insertedRows_);
onChanged();
return this;
}
/**
*
* The inserted row contains all column values.
*
*
* repeated bytes inserted_rows = 2;
*/
public Builder clearInsertedRows() {
insertedRows_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000002);
onChanged();
return this;
}
private java.util.List updatedRows_ = java.util.Collections.emptyList();
private void ensureUpdatedRowsIsMutable() {
if (!((bitField0_ & 0x00000004) == 0x00000004)) {
updatedRows_ = new java.util.ArrayList(updatedRows_);
bitField0_ |= 0x00000004;
}
}
/**
*
* The updated row contains old values and new values of the row.
*
*
* repeated bytes updated_rows = 3;
*/
public java.util.List
getUpdatedRowsList() {
return java.util.Collections.unmodifiableList(updatedRows_);
}
/**
*
* The updated row contains old values and new values of the row.
*
*
* repeated bytes updated_rows = 3;
*/
public int getUpdatedRowsCount() {
return updatedRows_.size();
}
/**
*
* The updated row contains old values and new values of the row.
*
*
* repeated bytes updated_rows = 3;
*/
public com.google.protobuf.ByteString getUpdatedRows(int index) {
return updatedRows_.get(index);
}
/**
*
* The updated row contains old values and new values of the row.
*
*
* repeated bytes updated_rows = 3;
*/
public Builder setUpdatedRows(
int index, com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
ensureUpdatedRowsIsMutable();
updatedRows_.set(index, value);
onChanged();
return this;
}
/**
*
* The updated row contains old values and new values of the row.
*
*
* repeated bytes updated_rows = 3;
*/
public Builder addUpdatedRows(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
ensureUpdatedRowsIsMutable();
updatedRows_.add(value);
onChanged();
return this;
}
/**
*
* The updated row contains old values and new values of the row.
*
*
* repeated bytes updated_rows = 3;
*/
public Builder addAllUpdatedRows(
java.lang.Iterable extends com.google.protobuf.ByteString> values) {
ensureUpdatedRowsIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, updatedRows_);
onChanged();
return this;
}
/**
*
* The updated row contains old values and new values of the row.
*
*
* repeated bytes updated_rows = 3;
*/
public Builder clearUpdatedRows() {
updatedRows_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000004);
onChanged();
return this;
}
private java.util.List deletedIds_ = java.util.Collections.emptyList();
private void ensureDeletedIdsIsMutable() {
if (!((bitField0_ & 0x00000008) == 0x00000008)) {
deletedIds_ = new java.util.ArrayList(deletedIds_);
bitField0_ |= 0x00000008;
}
}
/**
*
* Obsolete field.
*
*
* repeated int64 deleted_ids = 4;
*/
public java.util.List
getDeletedIdsList() {
return java.util.Collections.unmodifiableList(deletedIds_);
}
/**
*
* Obsolete field.
*
*
* repeated int64 deleted_ids = 4;
*/
public int getDeletedIdsCount() {
return deletedIds_.size();
}
/**
*
* Obsolete field.
*
*
* repeated int64 deleted_ids = 4;
*/
public long getDeletedIds(int index) {
return deletedIds_.get(index);
}
/**
*
* Obsolete field.
*
*
* repeated int64 deleted_ids = 4;
*/
public Builder setDeletedIds(
int index, long value) {
ensureDeletedIdsIsMutable();
deletedIds_.set(index, value);
onChanged();
return this;
}
/**
*
* Obsolete field.
*
*
* repeated int64 deleted_ids = 4;
*/
public Builder addDeletedIds(long value) {
ensureDeletedIdsIsMutable();
deletedIds_.add(value);
onChanged();
return this;
}
/**
*
* Obsolete field.
*
*
* repeated int64 deleted_ids = 4;
*/
public Builder addAllDeletedIds(
java.lang.Iterable extends java.lang.Long> values) {
ensureDeletedIdsIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, deletedIds_);
onChanged();
return this;
}
/**
*
* Obsolete field.
*
*
* repeated int64 deleted_ids = 4;
*/
public Builder clearDeletedIds() {
deletedIds_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000008);
onChanged();
return this;
}
private java.util.List deletedPks_ = java.util.Collections.emptyList();
private void ensureDeletedPksIsMutable() {
if (!((bitField0_ & 0x00000010) == 0x00000010)) {
deletedPks_ = new java.util.ArrayList(deletedPks_);
bitField0_ |= 0x00000010;
}
}
/**
*
* Obsolete field.
*
*
* repeated bytes deleted_pks = 5;
*/
public java.util.List
getDeletedPksList() {
return java.util.Collections.unmodifiableList(deletedPks_);
}
/**
*
* Obsolete field.
*
*
* repeated bytes deleted_pks = 5;
*/
public int getDeletedPksCount() {
return deletedPks_.size();
}
/**
*
* Obsolete field.
*
*
* repeated bytes deleted_pks = 5;
*/
public com.google.protobuf.ByteString getDeletedPks(int index) {
return deletedPks_.get(index);
}
/**
*
* Obsolete field.
*
*
* repeated bytes deleted_pks = 5;
*/
public Builder setDeletedPks(
int index, com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
ensureDeletedPksIsMutable();
deletedPks_.set(index, value);
onChanged();
return this;
}
/**
*
* Obsolete field.
*
*
* repeated bytes deleted_pks = 5;
*/
public Builder addDeletedPks(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
ensureDeletedPksIsMutable();
deletedPks_.add(value);
onChanged();
return this;
}
/**
*
* Obsolete field.
*
*
* repeated bytes deleted_pks = 5;
*/
public Builder addAllDeletedPks(
java.lang.Iterable extends com.google.protobuf.ByteString> values) {
ensureDeletedPksIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, deletedPks_);
onChanged();
return this;
}
/**
*
* Obsolete field.
*
*
* repeated bytes deleted_pks = 5;
*/
public Builder clearDeletedPks() {
deletedPks_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000010);
onChanged();
return this;
}
private java.util.List deletedRows_ = java.util.Collections.emptyList();
private void ensureDeletedRowsIsMutable() {
if (!((bitField0_ & 0x00000020) == 0x00000020)) {
deletedRows_ = new java.util.ArrayList(deletedRows_);
bitField0_ |= 0x00000020;
}
}
/**
*
* The row value of the deleted row.
*
*
* repeated bytes deleted_rows = 6;
*/
public java.util.List
getDeletedRowsList() {
return java.util.Collections.unmodifiableList(deletedRows_);
}
/**
*
* The row value of the deleted row.
*
*
* repeated bytes deleted_rows = 6;
*/
public int getDeletedRowsCount() {
return deletedRows_.size();
}
/**
*
* The row value of the deleted row.
*
*
* repeated bytes deleted_rows = 6;
*/
public com.google.protobuf.ByteString getDeletedRows(int index) {
return deletedRows_.get(index);
}
/**
*
* The row value of the deleted row.
*
*
* repeated bytes deleted_rows = 6;
*/
public Builder setDeletedRows(
int index, com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
ensureDeletedRowsIsMutable();
deletedRows_.set(index, value);
onChanged();
return this;
}
/**
*
* The row value of the deleted row.
*
*
* repeated bytes deleted_rows = 6;
*/
public Builder addDeletedRows(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
ensureDeletedRowsIsMutable();
deletedRows_.add(value);
onChanged();
return this;
}
/**
*
* The row value of the deleted row.
*
*
* repeated bytes deleted_rows = 6;
*/
public Builder addAllDeletedRows(
java.lang.Iterable extends com.google.protobuf.ByteString> values) {
ensureDeletedRowsIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, deletedRows_);
onChanged();
return this;
}
/**
*
* The row value of the deleted row.
*
*
* repeated bytes deleted_rows = 6;
*/
public Builder clearDeletedRows() {
deletedRows_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000020);
onChanged();
return this;
}
private java.util.List sequence_ =
java.util.Collections.emptyList();
private void ensureSequenceIsMutable() {
if (!((bitField0_ & 0x00000040) == 0x00000040)) {
sequence_ = new java.util.ArrayList(sequence_);
bitField0_ |= 0x00000040;
}
}
/**
*
* Used to apply table mutations in original sequence.
*
*
* repeated .binlog.MutationType sequence = 7;
*/
public java.util.List getSequenceList() {
return new com.google.protobuf.Internal.ListAdapter<
java.lang.Integer, binlog.BinlogOuterClass.MutationType>(sequence_, sequence_converter_);
}
/**
*
* Used to apply table mutations in original sequence.
*
*
* repeated .binlog.MutationType sequence = 7;
*/
public int getSequenceCount() {
return sequence_.size();
}
/**
*
* Used to apply table mutations in original sequence.
*
*
* repeated .binlog.MutationType sequence = 7;
*/
public binlog.BinlogOuterClass.MutationType getSequence(int index) {
return sequence_converter_.convert(sequence_.get(index));
}
/**
*
* Used to apply table mutations in original sequence.
*
*
* repeated .binlog.MutationType sequence = 7;
*/
public Builder setSequence(
int index, binlog.BinlogOuterClass.MutationType value) {
if (value == null) {
throw new NullPointerException();
}
ensureSequenceIsMutable();
sequence_.set(index, value.getNumber());
onChanged();
return this;
}
/**
*
* Used to apply table mutations in original sequence.
*
*
* repeated .binlog.MutationType sequence = 7;
*/
public Builder addSequence(binlog.BinlogOuterClass.MutationType value) {
if (value == null) {
throw new NullPointerException();
}
ensureSequenceIsMutable();
sequence_.add(value.getNumber());
onChanged();
return this;
}
/**
*
* Used to apply table mutations in original sequence.
*
*
* repeated .binlog.MutationType sequence = 7;
*/
public Builder addAllSequence(
java.lang.Iterable extends binlog.BinlogOuterClass.MutationType> values) {
ensureSequenceIsMutable();
for (binlog.BinlogOuterClass.MutationType value : values) {
sequence_.add(value.getNumber());
}
onChanged();
return this;
}
/**
*
* Used to apply table mutations in original sequence.
*
*
* repeated .binlog.MutationType sequence = 7;
*/
public Builder clearSequence() {
sequence_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000040);
onChanged();
return this;
}
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:binlog.TableMutation)
}
// @@protoc_insertion_point(class_scope:binlog.TableMutation)
private static final binlog.BinlogOuterClass.TableMutation DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new binlog.BinlogOuterClass.TableMutation();
}
public static binlog.BinlogOuterClass.TableMutation getDefaultInstance() {
return DEFAULT_INSTANCE;
}
@java.lang.Deprecated public static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
public TableMutation parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new TableMutation(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
public binlog.BinlogOuterClass.TableMutation getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface PrewriteValueOrBuilder extends
// @@protoc_insertion_point(interface_extends:binlog.PrewriteValue)
com.google.protobuf.MessageOrBuilder {
/**
* optional int64 schema_version = 1 [(.gogoproto.nullable) = false];
*/
boolean hasSchemaVersion();
/**
* optional int64 schema_version = 1 [(.gogoproto.nullable) = false];
*/
long getSchemaVersion();
/**
* repeated .binlog.TableMutation mutations = 2 [(.gogoproto.nullable) = false];
*/
java.util.List
getMutationsList();
/**
* repeated .binlog.TableMutation mutations = 2 [(.gogoproto.nullable) = false];
*/
binlog.BinlogOuterClass.TableMutation getMutations(int index);
/**
* repeated .binlog.TableMutation mutations = 2 [(.gogoproto.nullable) = false];
*/
int getMutationsCount();
/**
* repeated .binlog.TableMutation mutations = 2 [(.gogoproto.nullable) = false];
*/
java.util.List extends binlog.BinlogOuterClass.TableMutationOrBuilder>
getMutationsOrBuilderList();
/**
* repeated .binlog.TableMutation mutations = 2 [(.gogoproto.nullable) = false];
*/
binlog.BinlogOuterClass.TableMutationOrBuilder getMutationsOrBuilder(
int index);
}
/**
* Protobuf type {@code binlog.PrewriteValue}
*/
public static final class PrewriteValue extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:binlog.PrewriteValue)
PrewriteValueOrBuilder {
private static final long serialVersionUID = 0L;
// Use PrewriteValue.newBuilder() to construct.
private PrewriteValue(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private PrewriteValue() {
schemaVersion_ = 0L;
mutations_ = java.util.Collections.emptyList();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private PrewriteValue(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
default: {
if (!parseUnknownField(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
case 8: {
bitField0_ |= 0x00000001;
schemaVersion_ = input.readInt64();
break;
}
case 18: {
if (!((mutable_bitField0_ & 0x00000002) == 0x00000002)) {
mutations_ = new java.util.ArrayList();
mutable_bitField0_ |= 0x00000002;
}
mutations_.add(
input.readMessage(binlog.BinlogOuterClass.TableMutation.PARSER, extensionRegistry));
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
if (((mutable_bitField0_ & 0x00000002) == 0x00000002)) {
mutations_ = java.util.Collections.unmodifiableList(mutations_);
}
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return binlog.BinlogOuterClass.internal_static_binlog_PrewriteValue_descriptor;
}
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return binlog.BinlogOuterClass.internal_static_binlog_PrewriteValue_fieldAccessorTable
.ensureFieldAccessorsInitialized(
binlog.BinlogOuterClass.PrewriteValue.class, binlog.BinlogOuterClass.PrewriteValue.Builder.class);
}
private int bitField0_;
public static final int SCHEMA_VERSION_FIELD_NUMBER = 1;
private long schemaVersion_;
/**
* optional int64 schema_version = 1 [(.gogoproto.nullable) = false];
*/
public boolean hasSchemaVersion() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
/**
* optional int64 schema_version = 1 [(.gogoproto.nullable) = false];
*/
public long getSchemaVersion() {
return schemaVersion_;
}
public static final int MUTATIONS_FIELD_NUMBER = 2;
private java.util.List mutations_;
/**
* repeated .binlog.TableMutation mutations = 2 [(.gogoproto.nullable) = false];
*/
public java.util.List getMutationsList() {
return mutations_;
}
/**
* repeated .binlog.TableMutation mutations = 2 [(.gogoproto.nullable) = false];
*/
public java.util.List extends binlog.BinlogOuterClass.TableMutationOrBuilder>
getMutationsOrBuilderList() {
return mutations_;
}
/**
* repeated .binlog.TableMutation mutations = 2 [(.gogoproto.nullable) = false];
*/
public int getMutationsCount() {
return mutations_.size();
}
/**
* repeated .binlog.TableMutation mutations = 2 [(.gogoproto.nullable) = false];
*/
public binlog.BinlogOuterClass.TableMutation getMutations(int index) {
return mutations_.get(index);
}
/**
* repeated .binlog.TableMutation mutations = 2 [(.gogoproto.nullable) = false];
*/
public binlog.BinlogOuterClass.TableMutationOrBuilder getMutationsOrBuilder(
int index) {
return mutations_.get(index);
}
private byte memoizedIsInitialized = -1;
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (((bitField0_ & 0x00000001) == 0x00000001)) {
output.writeInt64(1, schemaVersion_);
}
for (int i = 0; i < mutations_.size(); i++) {
output.writeMessage(2, mutations_.get(i));
}
unknownFields.writeTo(output);
}
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (((bitField0_ & 0x00000001) == 0x00000001)) {
size += com.google.protobuf.CodedOutputStream
.computeInt64Size(1, schemaVersion_);
}
for (int i = 0; i < mutations_.size(); i++) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(2, mutations_.get(i));
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof binlog.BinlogOuterClass.PrewriteValue)) {
return super.equals(obj);
}
binlog.BinlogOuterClass.PrewriteValue other = (binlog.BinlogOuterClass.PrewriteValue) obj;
boolean result = true;
result = result && (hasSchemaVersion() == other.hasSchemaVersion());
if (hasSchemaVersion()) {
result = result && (getSchemaVersion()
== other.getSchemaVersion());
}
result = result && getMutationsList()
.equals(other.getMutationsList());
result = result && unknownFields.equals(other.unknownFields);
return result;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (hasSchemaVersion()) {
hash = (37 * hash) + SCHEMA_VERSION_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
getSchemaVersion());
}
if (getMutationsCount() > 0) {
hash = (37 * hash) + MUTATIONS_FIELD_NUMBER;
hash = (53 * hash) + getMutationsList().hashCode();
}
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static binlog.BinlogOuterClass.PrewriteValue parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static binlog.BinlogOuterClass.PrewriteValue parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static binlog.BinlogOuterClass.PrewriteValue parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static binlog.BinlogOuterClass.PrewriteValue parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static binlog.BinlogOuterClass.PrewriteValue parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static binlog.BinlogOuterClass.PrewriteValue parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static binlog.BinlogOuterClass.PrewriteValue parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static binlog.BinlogOuterClass.PrewriteValue parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static binlog.BinlogOuterClass.PrewriteValue parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static binlog.BinlogOuterClass.PrewriteValue parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static binlog.BinlogOuterClass.PrewriteValue parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static binlog.BinlogOuterClass.PrewriteValue parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(binlog.BinlogOuterClass.PrewriteValue prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code binlog.PrewriteValue}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:binlog.PrewriteValue)
binlog.BinlogOuterClass.PrewriteValueOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return binlog.BinlogOuterClass.internal_static_binlog_PrewriteValue_descriptor;
}
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return binlog.BinlogOuterClass.internal_static_binlog_PrewriteValue_fieldAccessorTable
.ensureFieldAccessorsInitialized(
binlog.BinlogOuterClass.PrewriteValue.class, binlog.BinlogOuterClass.PrewriteValue.Builder.class);
}
// Construct using binlog.BinlogOuterClass.PrewriteValue.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
getMutationsFieldBuilder();
}
}
public Builder clear() {
super.clear();
schemaVersion_ = 0L;
bitField0_ = (bitField0_ & ~0x00000001);
if (mutationsBuilder_ == null) {
mutations_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000002);
} else {
mutationsBuilder_.clear();
}
return this;
}
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return binlog.BinlogOuterClass.internal_static_binlog_PrewriteValue_descriptor;
}
public binlog.BinlogOuterClass.PrewriteValue getDefaultInstanceForType() {
return binlog.BinlogOuterClass.PrewriteValue.getDefaultInstance();
}
public binlog.BinlogOuterClass.PrewriteValue build() {
binlog.BinlogOuterClass.PrewriteValue result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
public binlog.BinlogOuterClass.PrewriteValue buildPartial() {
binlog.BinlogOuterClass.PrewriteValue result = new binlog.BinlogOuterClass.PrewriteValue(this);
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (((from_bitField0_ & 0x00000001) == 0x00000001)) {
to_bitField0_ |= 0x00000001;
}
result.schemaVersion_ = schemaVersion_;
if (mutationsBuilder_ == null) {
if (((bitField0_ & 0x00000002) == 0x00000002)) {
mutations_ = java.util.Collections.unmodifiableList(mutations_);
bitField0_ = (bitField0_ & ~0x00000002);
}
result.mutations_ = mutations_;
} else {
result.mutations_ = mutationsBuilder_.build();
}
result.bitField0_ = to_bitField0_;
onBuilt();
return result;
}
public Builder clone() {
return (Builder) super.clone();
}
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.setField(field, value);
}
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return (Builder) super.clearField(field);
}
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return (Builder) super.clearOneof(oneof);
}
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return (Builder) super.setRepeatedField(field, index, value);
}
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.addRepeatedField(field, value);
}
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof binlog.BinlogOuterClass.PrewriteValue) {
return mergeFrom((binlog.BinlogOuterClass.PrewriteValue)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(binlog.BinlogOuterClass.PrewriteValue other) {
if (other == binlog.BinlogOuterClass.PrewriteValue.getDefaultInstance()) return this;
if (other.hasSchemaVersion()) {
setSchemaVersion(other.getSchemaVersion());
}
if (mutationsBuilder_ == null) {
if (!other.mutations_.isEmpty()) {
if (mutations_.isEmpty()) {
mutations_ = other.mutations_;
bitField0_ = (bitField0_ & ~0x00000002);
} else {
ensureMutationsIsMutable();
mutations_.addAll(other.mutations_);
}
onChanged();
}
} else {
if (!other.mutations_.isEmpty()) {
if (mutationsBuilder_.isEmpty()) {
mutationsBuilder_.dispose();
mutationsBuilder_ = null;
mutations_ = other.mutations_;
bitField0_ = (bitField0_ & ~0x00000002);
mutationsBuilder_ =
com.google.protobuf.GeneratedMessageV3.alwaysUseFieldBuilders ?
getMutationsFieldBuilder() : null;
} else {
mutationsBuilder_.addAllMessages(other.mutations_);
}
}
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
public final boolean isInitialized() {
return true;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
binlog.BinlogOuterClass.PrewriteValue parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (binlog.BinlogOuterClass.PrewriteValue) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
private long schemaVersion_ ;
/**
* optional int64 schema_version = 1 [(.gogoproto.nullable) = false];
*/
public boolean hasSchemaVersion() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
/**
* optional int64 schema_version = 1 [(.gogoproto.nullable) = false];
*/
public long getSchemaVersion() {
return schemaVersion_;
}
/**
* optional int64 schema_version = 1 [(.gogoproto.nullable) = false];
*/
public Builder setSchemaVersion(long value) {
bitField0_ |= 0x00000001;
schemaVersion_ = value;
onChanged();
return this;
}
/**
* optional int64 schema_version = 1 [(.gogoproto.nullable) = false];
*/
public Builder clearSchemaVersion() {
bitField0_ = (bitField0_ & ~0x00000001);
schemaVersion_ = 0L;
onChanged();
return this;
}
private java.util.List mutations_ =
java.util.Collections.emptyList();
private void ensureMutationsIsMutable() {
if (!((bitField0_ & 0x00000002) == 0x00000002)) {
mutations_ = new java.util.ArrayList(mutations_);
bitField0_ |= 0x00000002;
}
}
private com.google.protobuf.RepeatedFieldBuilderV3<
binlog.BinlogOuterClass.TableMutation, binlog.BinlogOuterClass.TableMutation.Builder, binlog.BinlogOuterClass.TableMutationOrBuilder> mutationsBuilder_;
/**
* repeated .binlog.TableMutation mutations = 2 [(.gogoproto.nullable) = false];
*/
public java.util.List getMutationsList() {
if (mutationsBuilder_ == null) {
return java.util.Collections.unmodifiableList(mutations_);
} else {
return mutationsBuilder_.getMessageList();
}
}
/**
* repeated .binlog.TableMutation mutations = 2 [(.gogoproto.nullable) = false];
*/
public int getMutationsCount() {
if (mutationsBuilder_ == null) {
return mutations_.size();
} else {
return mutationsBuilder_.getCount();
}
}
/**
* repeated .binlog.TableMutation mutations = 2 [(.gogoproto.nullable) = false];
*/
public binlog.BinlogOuterClass.TableMutation getMutations(int index) {
if (mutationsBuilder_ == null) {
return mutations_.get(index);
} else {
return mutationsBuilder_.getMessage(index);
}
}
/**
* repeated .binlog.TableMutation mutations = 2 [(.gogoproto.nullable) = false];
*/
public Builder setMutations(
int index, binlog.BinlogOuterClass.TableMutation value) {
if (mutationsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureMutationsIsMutable();
mutations_.set(index, value);
onChanged();
} else {
mutationsBuilder_.setMessage(index, value);
}
return this;
}
/**
* repeated .binlog.TableMutation mutations = 2 [(.gogoproto.nullable) = false];
*/
public Builder setMutations(
int index, binlog.BinlogOuterClass.TableMutation.Builder builderForValue) {
if (mutationsBuilder_ == null) {
ensureMutationsIsMutable();
mutations_.set(index, builderForValue.build());
onChanged();
} else {
mutationsBuilder_.setMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .binlog.TableMutation mutations = 2 [(.gogoproto.nullable) = false];
*/
public Builder addMutations(binlog.BinlogOuterClass.TableMutation value) {
if (mutationsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureMutationsIsMutable();
mutations_.add(value);
onChanged();
} else {
mutationsBuilder_.addMessage(value);
}
return this;
}
/**
* repeated .binlog.TableMutation mutations = 2 [(.gogoproto.nullable) = false];
*/
public Builder addMutations(
int index, binlog.BinlogOuterClass.TableMutation value) {
if (mutationsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureMutationsIsMutable();
mutations_.add(index, value);
onChanged();
} else {
mutationsBuilder_.addMessage(index, value);
}
return this;
}
/**
* repeated .binlog.TableMutation mutations = 2 [(.gogoproto.nullable) = false];
*/
public Builder addMutations(
binlog.BinlogOuterClass.TableMutation.Builder builderForValue) {
if (mutationsBuilder_ == null) {
ensureMutationsIsMutable();
mutations_.add(builderForValue.build());
onChanged();
} else {
mutationsBuilder_.addMessage(builderForValue.build());
}
return this;
}
/**
* repeated .binlog.TableMutation mutations = 2 [(.gogoproto.nullable) = false];
*/
public Builder addMutations(
int index, binlog.BinlogOuterClass.TableMutation.Builder builderForValue) {
if (mutationsBuilder_ == null) {
ensureMutationsIsMutable();
mutations_.add(index, builderForValue.build());
onChanged();
} else {
mutationsBuilder_.addMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .binlog.TableMutation mutations = 2 [(.gogoproto.nullable) = false];
*/
public Builder addAllMutations(
java.lang.Iterable extends binlog.BinlogOuterClass.TableMutation> values) {
if (mutationsBuilder_ == null) {
ensureMutationsIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, mutations_);
onChanged();
} else {
mutationsBuilder_.addAllMessages(values);
}
return this;
}
/**
* repeated .binlog.TableMutation mutations = 2 [(.gogoproto.nullable) = false];
*/
public Builder clearMutations() {
if (mutationsBuilder_ == null) {
mutations_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000002);
onChanged();
} else {
mutationsBuilder_.clear();
}
return this;
}
/**
* repeated .binlog.TableMutation mutations = 2 [(.gogoproto.nullable) = false];
*/
public Builder removeMutations(int index) {
if (mutationsBuilder_ == null) {
ensureMutationsIsMutable();
mutations_.remove(index);
onChanged();
} else {
mutationsBuilder_.remove(index);
}
return this;
}
/**
* repeated .binlog.TableMutation mutations = 2 [(.gogoproto.nullable) = false];
*/
public binlog.BinlogOuterClass.TableMutation.Builder getMutationsBuilder(
int index) {
return getMutationsFieldBuilder().getBuilder(index);
}
/**
* repeated .binlog.TableMutation mutations = 2 [(.gogoproto.nullable) = false];
*/
public binlog.BinlogOuterClass.TableMutationOrBuilder getMutationsOrBuilder(
int index) {
if (mutationsBuilder_ == null) {
return mutations_.get(index); } else {
return mutationsBuilder_.getMessageOrBuilder(index);
}
}
/**
* repeated .binlog.TableMutation mutations = 2 [(.gogoproto.nullable) = false];
*/
public java.util.List extends binlog.BinlogOuterClass.TableMutationOrBuilder>
getMutationsOrBuilderList() {
if (mutationsBuilder_ != null) {
return mutationsBuilder_.getMessageOrBuilderList();
} else {
return java.util.Collections.unmodifiableList(mutations_);
}
}
/**
* repeated .binlog.TableMutation mutations = 2 [(.gogoproto.nullable) = false];
*/
public binlog.BinlogOuterClass.TableMutation.Builder addMutationsBuilder() {
return getMutationsFieldBuilder().addBuilder(
binlog.BinlogOuterClass.TableMutation.getDefaultInstance());
}
/**
* repeated .binlog.TableMutation mutations = 2 [(.gogoproto.nullable) = false];
*/
public binlog.BinlogOuterClass.TableMutation.Builder addMutationsBuilder(
int index) {
return getMutationsFieldBuilder().addBuilder(
index, binlog.BinlogOuterClass.TableMutation.getDefaultInstance());
}
/**
* repeated .binlog.TableMutation mutations = 2 [(.gogoproto.nullable) = false];
*/
public java.util.List
getMutationsBuilderList() {
return getMutationsFieldBuilder().getBuilderList();
}
private com.google.protobuf.RepeatedFieldBuilderV3<
binlog.BinlogOuterClass.TableMutation, binlog.BinlogOuterClass.TableMutation.Builder, binlog.BinlogOuterClass.TableMutationOrBuilder>
getMutationsFieldBuilder() {
if (mutationsBuilder_ == null) {
mutationsBuilder_ = new com.google.protobuf.RepeatedFieldBuilderV3<
binlog.BinlogOuterClass.TableMutation, binlog.BinlogOuterClass.TableMutation.Builder, binlog.BinlogOuterClass.TableMutationOrBuilder>(
mutations_,
((bitField0_ & 0x00000002) == 0x00000002),
getParentForChildren(),
isClean());
mutations_ = null;
}
return mutationsBuilder_;
}
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:binlog.PrewriteValue)
}
// @@protoc_insertion_point(class_scope:binlog.PrewriteValue)
private static final binlog.BinlogOuterClass.PrewriteValue DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new binlog.BinlogOuterClass.PrewriteValue();
}
public static binlog.BinlogOuterClass.PrewriteValue getDefaultInstance() {
return DEFAULT_INSTANCE;
}
@java.lang.Deprecated public static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
public PrewriteValue parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new PrewriteValue(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
public binlog.BinlogOuterClass.PrewriteValue getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface BinlogOrBuilder extends
// @@protoc_insertion_point(interface_extends:binlog.Binlog)
com.google.protobuf.MessageOrBuilder {
/**
* optional .binlog.BinlogType tp = 1 [(.gogoproto.nullable) = false];
*/
boolean hasTp();
/**
* optional .binlog.BinlogType tp = 1 [(.gogoproto.nullable) = false];
*/
binlog.BinlogOuterClass.BinlogType getTp();
/**
*
* start_ts is used in Prewrite, Commit and Rollback binlog Type.
* It is used for pairing prewrite log to commit log or rollback log.
*
*
* optional int64 start_ts = 2 [(.gogoproto.nullable) = false];
*/
boolean hasStartTs();
/**
*
* start_ts is used in Prewrite, Commit and Rollback binlog Type.
* It is used for pairing prewrite log to commit log or rollback log.
*
*
* optional int64 start_ts = 2 [(.gogoproto.nullable) = false];
*/
long getStartTs();
/**
*
* commit_ts is used only in binlog type Commit.
*
*
* optional int64 commit_ts = 3 [(.gogoproto.nullable) = false];
*/
boolean hasCommitTs();
/**
*
* commit_ts is used only in binlog type Commit.
*
*
* optional int64 commit_ts = 3 [(.gogoproto.nullable) = false];
*/
long getCommitTs();
/**
*
* prewrite key is used only in Prewrite binlog type.
* It is the primary key of the transaction, is used to check that the transaction is
* commited or not if it failed to pair to commit log or rollback log within a time window.
*
*
* optional bytes prewrite_key = 4;
*/
boolean hasPrewriteKey();
/**
*
* prewrite key is used only in Prewrite binlog type.
* It is the primary key of the transaction, is used to check that the transaction is
* commited or not if it failed to pair to commit log or rollback log within a time window.
*
*
* optional bytes prewrite_key = 4;
*/
com.google.protobuf.ByteString getPrewriteKey();
/**
*
* prewrite_data is marshalled from PrewriteData type,
* we do not need to unmarshal prewrite data before the binlog have been successfully paired.
*
*
* optional bytes prewrite_value = 5;
*/
boolean hasPrewriteValue();
/**
*
* prewrite_data is marshalled from PrewriteData type,
* we do not need to unmarshal prewrite data before the binlog have been successfully paired.
*
*
* optional bytes prewrite_value = 5;
*/
com.google.protobuf.ByteString getPrewriteValue();
/**
*
* ddl_query is the original DDL statement query.
*
*
* optional bytes ddl_query = 6;
*/
boolean hasDdlQuery();
/**
*
* ddl_query is the original DDL statement query.
*
*
* optional bytes ddl_query = 6;
*/
com.google.protobuf.ByteString getDdlQuery();
/**
*
* ddl_job_id is used for DDL Binlog.
* If ddl_job_id is setted, this is a DDL Binlog and ddl_query contains the DDL query, we can query the informations about this job from TiKV.
*
*
* optional int64 ddl_job_id = 7 [(.gogoproto.nullable) = false];
*/
boolean hasDdlJobId();
/**
*
* ddl_job_id is used for DDL Binlog.
* If ddl_job_id is setted, this is a DDL Binlog and ddl_query contains the DDL query, we can query the informations about this job from TiKV.
*
*
* optional int64 ddl_job_id = 7 [(.gogoproto.nullable) = false];
*/
long getDdlJobId();
/**
*
* ddl_schema_state is used for DDL Binlog.
*
*
* optional int32 ddl_schema_state = 8 [(.gogoproto.nullable) = false];
*/
boolean hasDdlSchemaState();
/**
*
* ddl_schema_state is used for DDL Binlog.
*
*
* optional int32 ddl_schema_state = 8 [(.gogoproto.nullable) = false];
*/
int getDdlSchemaState();
}
/**
*
* Binlog contains all the changes in a transaction, which can be used to reconstruct SQL statement, then export to
* other systems.
*
*
* Protobuf type {@code binlog.Binlog}
*/
public static final class Binlog extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:binlog.Binlog)
BinlogOrBuilder {
private static final long serialVersionUID = 0L;
// Use Binlog.newBuilder() to construct.
private Binlog(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private Binlog() {
tp_ = 0;
startTs_ = 0L;
commitTs_ = 0L;
prewriteKey_ = com.google.protobuf.ByteString.EMPTY;
prewriteValue_ = com.google.protobuf.ByteString.EMPTY;
ddlQuery_ = com.google.protobuf.ByteString.EMPTY;
ddlJobId_ = 0L;
ddlSchemaState_ = 0;
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private Binlog(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
default: {
if (!parseUnknownField(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
case 8: {
int rawValue = input.readEnum();
binlog.BinlogOuterClass.BinlogType value = binlog.BinlogOuterClass.BinlogType.valueOf(rawValue);
if (value == null) {
unknownFields.mergeVarintField(1, rawValue);
} else {
bitField0_ |= 0x00000001;
tp_ = rawValue;
}
break;
}
case 16: {
bitField0_ |= 0x00000002;
startTs_ = input.readInt64();
break;
}
case 24: {
bitField0_ |= 0x00000004;
commitTs_ = input.readInt64();
break;
}
case 34: {
bitField0_ |= 0x00000008;
prewriteKey_ = input.readBytes();
break;
}
case 42: {
bitField0_ |= 0x00000010;
prewriteValue_ = input.readBytes();
break;
}
case 50: {
bitField0_ |= 0x00000020;
ddlQuery_ = input.readBytes();
break;
}
case 56: {
bitField0_ |= 0x00000040;
ddlJobId_ = input.readInt64();
break;
}
case 64: {
bitField0_ |= 0x00000080;
ddlSchemaState_ = input.readInt32();
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return binlog.BinlogOuterClass.internal_static_binlog_Binlog_descriptor;
}
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return binlog.BinlogOuterClass.internal_static_binlog_Binlog_fieldAccessorTable
.ensureFieldAccessorsInitialized(
binlog.BinlogOuterClass.Binlog.class, binlog.BinlogOuterClass.Binlog.Builder.class);
}
private int bitField0_;
public static final int TP_FIELD_NUMBER = 1;
private int tp_;
/**
* optional .binlog.BinlogType tp = 1 [(.gogoproto.nullable) = false];
*/
public boolean hasTp() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
/**
* optional .binlog.BinlogType tp = 1 [(.gogoproto.nullable) = false];
*/
public binlog.BinlogOuterClass.BinlogType getTp() {
binlog.BinlogOuterClass.BinlogType result = binlog.BinlogOuterClass.BinlogType.valueOf(tp_);
return result == null ? binlog.BinlogOuterClass.BinlogType.Prewrite : result;
}
public static final int START_TS_FIELD_NUMBER = 2;
private long startTs_;
/**
*
* start_ts is used in Prewrite, Commit and Rollback binlog Type.
* It is used for pairing prewrite log to commit log or rollback log.
*
*
* optional int64 start_ts = 2 [(.gogoproto.nullable) = false];
*/
public boolean hasStartTs() {
return ((bitField0_ & 0x00000002) == 0x00000002);
}
/**
*
* start_ts is used in Prewrite, Commit and Rollback binlog Type.
* It is used for pairing prewrite log to commit log or rollback log.
*
*
* optional int64 start_ts = 2 [(.gogoproto.nullable) = false];
*/
public long getStartTs() {
return startTs_;
}
public static final int COMMIT_TS_FIELD_NUMBER = 3;
private long commitTs_;
/**
*
* commit_ts is used only in binlog type Commit.
*
*
* optional int64 commit_ts = 3 [(.gogoproto.nullable) = false];
*/
public boolean hasCommitTs() {
return ((bitField0_ & 0x00000004) == 0x00000004);
}
/**
*
* commit_ts is used only in binlog type Commit.
*
*
* optional int64 commit_ts = 3 [(.gogoproto.nullable) = false];
*/
public long getCommitTs() {
return commitTs_;
}
public static final int PREWRITE_KEY_FIELD_NUMBER = 4;
private com.google.protobuf.ByteString prewriteKey_;
/**
*
* prewrite key is used only in Prewrite binlog type.
* It is the primary key of the transaction, is used to check that the transaction is
* commited or not if it failed to pair to commit log or rollback log within a time window.
*
*
* optional bytes prewrite_key = 4;
*/
public boolean hasPrewriteKey() {
return ((bitField0_ & 0x00000008) == 0x00000008);
}
/**
*
* prewrite key is used only in Prewrite binlog type.
* It is the primary key of the transaction, is used to check that the transaction is
* commited or not if it failed to pair to commit log or rollback log within a time window.
*
*
* optional bytes prewrite_key = 4;
*/
public com.google.protobuf.ByteString getPrewriteKey() {
return prewriteKey_;
}
public static final int PREWRITE_VALUE_FIELD_NUMBER = 5;
private com.google.protobuf.ByteString prewriteValue_;
/**
*
* prewrite_data is marshalled from PrewriteData type,
* we do not need to unmarshal prewrite data before the binlog have been successfully paired.
*
*
* optional bytes prewrite_value = 5;
*/
public boolean hasPrewriteValue() {
return ((bitField0_ & 0x00000010) == 0x00000010);
}
/**
*
* prewrite_data is marshalled from PrewriteData type,
* we do not need to unmarshal prewrite data before the binlog have been successfully paired.
*
*
* optional bytes prewrite_value = 5;
*/
public com.google.protobuf.ByteString getPrewriteValue() {
return prewriteValue_;
}
public static final int DDL_QUERY_FIELD_NUMBER = 6;
private com.google.protobuf.ByteString ddlQuery_;
/**
*
* ddl_query is the original DDL statement query.
*
*
* optional bytes ddl_query = 6;
*/
public boolean hasDdlQuery() {
return ((bitField0_ & 0x00000020) == 0x00000020);
}
/**
*
* ddl_query is the original DDL statement query.
*
*
* optional bytes ddl_query = 6;
*/
public com.google.protobuf.ByteString getDdlQuery() {
return ddlQuery_;
}
public static final int DDL_JOB_ID_FIELD_NUMBER = 7;
private long ddlJobId_;
/**
*
* ddl_job_id is used for DDL Binlog.
* If ddl_job_id is setted, this is a DDL Binlog and ddl_query contains the DDL query, we can query the informations about this job from TiKV.
*
*
* optional int64 ddl_job_id = 7 [(.gogoproto.nullable) = false];
*/
public boolean hasDdlJobId() {
return ((bitField0_ & 0x00000040) == 0x00000040);
}
/**
*
* ddl_job_id is used for DDL Binlog.
* If ddl_job_id is setted, this is a DDL Binlog and ddl_query contains the DDL query, we can query the informations about this job from TiKV.
*
*
* optional int64 ddl_job_id = 7 [(.gogoproto.nullable) = false];
*/
public long getDdlJobId() {
return ddlJobId_;
}
public static final int DDL_SCHEMA_STATE_FIELD_NUMBER = 8;
private int ddlSchemaState_;
/**
*
* ddl_schema_state is used for DDL Binlog.
*
*
* optional int32 ddl_schema_state = 8 [(.gogoproto.nullable) = false];
*/
public boolean hasDdlSchemaState() {
return ((bitField0_ & 0x00000080) == 0x00000080);
}
/**
*
* ddl_schema_state is used for DDL Binlog.
*
*
* optional int32 ddl_schema_state = 8 [(.gogoproto.nullable) = false];
*/
public int getDdlSchemaState() {
return ddlSchemaState_;
}
private byte memoizedIsInitialized = -1;
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (((bitField0_ & 0x00000001) == 0x00000001)) {
output.writeEnum(1, tp_);
}
if (((bitField0_ & 0x00000002) == 0x00000002)) {
output.writeInt64(2, startTs_);
}
if (((bitField0_ & 0x00000004) == 0x00000004)) {
output.writeInt64(3, commitTs_);
}
if (((bitField0_ & 0x00000008) == 0x00000008)) {
output.writeBytes(4, prewriteKey_);
}
if (((bitField0_ & 0x00000010) == 0x00000010)) {
output.writeBytes(5, prewriteValue_);
}
if (((bitField0_ & 0x00000020) == 0x00000020)) {
output.writeBytes(6, ddlQuery_);
}
if (((bitField0_ & 0x00000040) == 0x00000040)) {
output.writeInt64(7, ddlJobId_);
}
if (((bitField0_ & 0x00000080) == 0x00000080)) {
output.writeInt32(8, ddlSchemaState_);
}
unknownFields.writeTo(output);
}
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (((bitField0_ & 0x00000001) == 0x00000001)) {
size += com.google.protobuf.CodedOutputStream
.computeEnumSize(1, tp_);
}
if (((bitField0_ & 0x00000002) == 0x00000002)) {
size += com.google.protobuf.CodedOutputStream
.computeInt64Size(2, startTs_);
}
if (((bitField0_ & 0x00000004) == 0x00000004)) {
size += com.google.protobuf.CodedOutputStream
.computeInt64Size(3, commitTs_);
}
if (((bitField0_ & 0x00000008) == 0x00000008)) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(4, prewriteKey_);
}
if (((bitField0_ & 0x00000010) == 0x00000010)) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(5, prewriteValue_);
}
if (((bitField0_ & 0x00000020) == 0x00000020)) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(6, ddlQuery_);
}
if (((bitField0_ & 0x00000040) == 0x00000040)) {
size += com.google.protobuf.CodedOutputStream
.computeInt64Size(7, ddlJobId_);
}
if (((bitField0_ & 0x00000080) == 0x00000080)) {
size += com.google.protobuf.CodedOutputStream
.computeInt32Size(8, ddlSchemaState_);
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof binlog.BinlogOuterClass.Binlog)) {
return super.equals(obj);
}
binlog.BinlogOuterClass.Binlog other = (binlog.BinlogOuterClass.Binlog) obj;
boolean result = true;
result = result && (hasTp() == other.hasTp());
if (hasTp()) {
result = result && tp_ == other.tp_;
}
result = result && (hasStartTs() == other.hasStartTs());
if (hasStartTs()) {
result = result && (getStartTs()
== other.getStartTs());
}
result = result && (hasCommitTs() == other.hasCommitTs());
if (hasCommitTs()) {
result = result && (getCommitTs()
== other.getCommitTs());
}
result = result && (hasPrewriteKey() == other.hasPrewriteKey());
if (hasPrewriteKey()) {
result = result && getPrewriteKey()
.equals(other.getPrewriteKey());
}
result = result && (hasPrewriteValue() == other.hasPrewriteValue());
if (hasPrewriteValue()) {
result = result && getPrewriteValue()
.equals(other.getPrewriteValue());
}
result = result && (hasDdlQuery() == other.hasDdlQuery());
if (hasDdlQuery()) {
result = result && getDdlQuery()
.equals(other.getDdlQuery());
}
result = result && (hasDdlJobId() == other.hasDdlJobId());
if (hasDdlJobId()) {
result = result && (getDdlJobId()
== other.getDdlJobId());
}
result = result && (hasDdlSchemaState() == other.hasDdlSchemaState());
if (hasDdlSchemaState()) {
result = result && (getDdlSchemaState()
== other.getDdlSchemaState());
}
result = result && unknownFields.equals(other.unknownFields);
return result;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (hasTp()) {
hash = (37 * hash) + TP_FIELD_NUMBER;
hash = (53 * hash) + tp_;
}
if (hasStartTs()) {
hash = (37 * hash) + START_TS_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
getStartTs());
}
if (hasCommitTs()) {
hash = (37 * hash) + COMMIT_TS_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
getCommitTs());
}
if (hasPrewriteKey()) {
hash = (37 * hash) + PREWRITE_KEY_FIELD_NUMBER;
hash = (53 * hash) + getPrewriteKey().hashCode();
}
if (hasPrewriteValue()) {
hash = (37 * hash) + PREWRITE_VALUE_FIELD_NUMBER;
hash = (53 * hash) + getPrewriteValue().hashCode();
}
if (hasDdlQuery()) {
hash = (37 * hash) + DDL_QUERY_FIELD_NUMBER;
hash = (53 * hash) + getDdlQuery().hashCode();
}
if (hasDdlJobId()) {
hash = (37 * hash) + DDL_JOB_ID_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
getDdlJobId());
}
if (hasDdlSchemaState()) {
hash = (37 * hash) + DDL_SCHEMA_STATE_FIELD_NUMBER;
hash = (53 * hash) + getDdlSchemaState();
}
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static binlog.BinlogOuterClass.Binlog parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static binlog.BinlogOuterClass.Binlog parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static binlog.BinlogOuterClass.Binlog parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static binlog.BinlogOuterClass.Binlog parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static binlog.BinlogOuterClass.Binlog parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static binlog.BinlogOuterClass.Binlog parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static binlog.BinlogOuterClass.Binlog parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static binlog.BinlogOuterClass.Binlog parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static binlog.BinlogOuterClass.Binlog parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static binlog.BinlogOuterClass.Binlog parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static binlog.BinlogOuterClass.Binlog parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static binlog.BinlogOuterClass.Binlog parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(binlog.BinlogOuterClass.Binlog prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
* Binlog contains all the changes in a transaction, which can be used to reconstruct SQL statement, then export to
* other systems.
*
*
* Protobuf type {@code binlog.Binlog}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:binlog.Binlog)
binlog.BinlogOuterClass.BinlogOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return binlog.BinlogOuterClass.internal_static_binlog_Binlog_descriptor;
}
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return binlog.BinlogOuterClass.internal_static_binlog_Binlog_fieldAccessorTable
.ensureFieldAccessorsInitialized(
binlog.BinlogOuterClass.Binlog.class, binlog.BinlogOuterClass.Binlog.Builder.class);
}
// Construct using binlog.BinlogOuterClass.Binlog.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
public Builder clear() {
super.clear();
tp_ = 0;
bitField0_ = (bitField0_ & ~0x00000001);
startTs_ = 0L;
bitField0_ = (bitField0_ & ~0x00000002);
commitTs_ = 0L;
bitField0_ = (bitField0_ & ~0x00000004);
prewriteKey_ = com.google.protobuf.ByteString.EMPTY;
bitField0_ = (bitField0_ & ~0x00000008);
prewriteValue_ = com.google.protobuf.ByteString.EMPTY;
bitField0_ = (bitField0_ & ~0x00000010);
ddlQuery_ = com.google.protobuf.ByteString.EMPTY;
bitField0_ = (bitField0_ & ~0x00000020);
ddlJobId_ = 0L;
bitField0_ = (bitField0_ & ~0x00000040);
ddlSchemaState_ = 0;
bitField0_ = (bitField0_ & ~0x00000080);
return this;
}
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return binlog.BinlogOuterClass.internal_static_binlog_Binlog_descriptor;
}
public binlog.BinlogOuterClass.Binlog getDefaultInstanceForType() {
return binlog.BinlogOuterClass.Binlog.getDefaultInstance();
}
public binlog.BinlogOuterClass.Binlog build() {
binlog.BinlogOuterClass.Binlog result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
public binlog.BinlogOuterClass.Binlog buildPartial() {
binlog.BinlogOuterClass.Binlog result = new binlog.BinlogOuterClass.Binlog(this);
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (((from_bitField0_ & 0x00000001) == 0x00000001)) {
to_bitField0_ |= 0x00000001;
}
result.tp_ = tp_;
if (((from_bitField0_ & 0x00000002) == 0x00000002)) {
to_bitField0_ |= 0x00000002;
}
result.startTs_ = startTs_;
if (((from_bitField0_ & 0x00000004) == 0x00000004)) {
to_bitField0_ |= 0x00000004;
}
result.commitTs_ = commitTs_;
if (((from_bitField0_ & 0x00000008) == 0x00000008)) {
to_bitField0_ |= 0x00000008;
}
result.prewriteKey_ = prewriteKey_;
if (((from_bitField0_ & 0x00000010) == 0x00000010)) {
to_bitField0_ |= 0x00000010;
}
result.prewriteValue_ = prewriteValue_;
if (((from_bitField0_ & 0x00000020) == 0x00000020)) {
to_bitField0_ |= 0x00000020;
}
result.ddlQuery_ = ddlQuery_;
if (((from_bitField0_ & 0x00000040) == 0x00000040)) {
to_bitField0_ |= 0x00000040;
}
result.ddlJobId_ = ddlJobId_;
if (((from_bitField0_ & 0x00000080) == 0x00000080)) {
to_bitField0_ |= 0x00000080;
}
result.ddlSchemaState_ = ddlSchemaState_;
result.bitField0_ = to_bitField0_;
onBuilt();
return result;
}
public Builder clone() {
return (Builder) super.clone();
}
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.setField(field, value);
}
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return (Builder) super.clearField(field);
}
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return (Builder) super.clearOneof(oneof);
}
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return (Builder) super.setRepeatedField(field, index, value);
}
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.addRepeatedField(field, value);
}
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof binlog.BinlogOuterClass.Binlog) {
return mergeFrom((binlog.BinlogOuterClass.Binlog)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(binlog.BinlogOuterClass.Binlog other) {
if (other == binlog.BinlogOuterClass.Binlog.getDefaultInstance()) return this;
if (other.hasTp()) {
setTp(other.getTp());
}
if (other.hasStartTs()) {
setStartTs(other.getStartTs());
}
if (other.hasCommitTs()) {
setCommitTs(other.getCommitTs());
}
if (other.hasPrewriteKey()) {
setPrewriteKey(other.getPrewriteKey());
}
if (other.hasPrewriteValue()) {
setPrewriteValue(other.getPrewriteValue());
}
if (other.hasDdlQuery()) {
setDdlQuery(other.getDdlQuery());
}
if (other.hasDdlJobId()) {
setDdlJobId(other.getDdlJobId());
}
if (other.hasDdlSchemaState()) {
setDdlSchemaState(other.getDdlSchemaState());
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
public final boolean isInitialized() {
return true;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
binlog.BinlogOuterClass.Binlog parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (binlog.BinlogOuterClass.Binlog) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
private int tp_ = 0;
/**
* optional .binlog.BinlogType tp = 1 [(.gogoproto.nullable) = false];
*/
public boolean hasTp() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
/**
* optional .binlog.BinlogType tp = 1 [(.gogoproto.nullable) = false];
*/
public binlog.BinlogOuterClass.BinlogType getTp() {
binlog.BinlogOuterClass.BinlogType result = binlog.BinlogOuterClass.BinlogType.valueOf(tp_);
return result == null ? binlog.BinlogOuterClass.BinlogType.Prewrite : result;
}
/**
* optional .binlog.BinlogType tp = 1 [(.gogoproto.nullable) = false];
*/
public Builder setTp(binlog.BinlogOuterClass.BinlogType value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000001;
tp_ = value.getNumber();
onChanged();
return this;
}
/**
* optional .binlog.BinlogType tp = 1 [(.gogoproto.nullable) = false];
*/
public Builder clearTp() {
bitField0_ = (bitField0_ & ~0x00000001);
tp_ = 0;
onChanged();
return this;
}
private long startTs_ ;
/**
*
* start_ts is used in Prewrite, Commit and Rollback binlog Type.
* It is used for pairing prewrite log to commit log or rollback log.
*
*
* optional int64 start_ts = 2 [(.gogoproto.nullable) = false];
*/
public boolean hasStartTs() {
return ((bitField0_ & 0x00000002) == 0x00000002);
}
/**
*
* start_ts is used in Prewrite, Commit and Rollback binlog Type.
* It is used for pairing prewrite log to commit log or rollback log.
*
*
* optional int64 start_ts = 2 [(.gogoproto.nullable) = false];
*/
public long getStartTs() {
return startTs_;
}
/**
*
* start_ts is used in Prewrite, Commit and Rollback binlog Type.
* It is used for pairing prewrite log to commit log or rollback log.
*
*
* optional int64 start_ts = 2 [(.gogoproto.nullable) = false];
*/
public Builder setStartTs(long value) {
bitField0_ |= 0x00000002;
startTs_ = value;
onChanged();
return this;
}
/**
*
* start_ts is used in Prewrite, Commit and Rollback binlog Type.
* It is used for pairing prewrite log to commit log or rollback log.
*
*
* optional int64 start_ts = 2 [(.gogoproto.nullable) = false];
*/
public Builder clearStartTs() {
bitField0_ = (bitField0_ & ~0x00000002);
startTs_ = 0L;
onChanged();
return this;
}
private long commitTs_ ;
/**
*
* commit_ts is used only in binlog type Commit.
*
*
* optional int64 commit_ts = 3 [(.gogoproto.nullable) = false];
*/
public boolean hasCommitTs() {
return ((bitField0_ & 0x00000004) == 0x00000004);
}
/**
*
* commit_ts is used only in binlog type Commit.
*
*
* optional int64 commit_ts = 3 [(.gogoproto.nullable) = false];
*/
public long getCommitTs() {
return commitTs_;
}
/**
*
* commit_ts is used only in binlog type Commit.
*
*
* optional int64 commit_ts = 3 [(.gogoproto.nullable) = false];
*/
public Builder setCommitTs(long value) {
bitField0_ |= 0x00000004;
commitTs_ = value;
onChanged();
return this;
}
/**
*
* commit_ts is used only in binlog type Commit.
*
*
* optional int64 commit_ts = 3 [(.gogoproto.nullable) = false];
*/
public Builder clearCommitTs() {
bitField0_ = (bitField0_ & ~0x00000004);
commitTs_ = 0L;
onChanged();
return this;
}
private com.google.protobuf.ByteString prewriteKey_ = com.google.protobuf.ByteString.EMPTY;
/**
*
* prewrite key is used only in Prewrite binlog type.
* It is the primary key of the transaction, is used to check that the transaction is
* commited or not if it failed to pair to commit log or rollback log within a time window.
*
*
* optional bytes prewrite_key = 4;
*/
public boolean hasPrewriteKey() {
return ((bitField0_ & 0x00000008) == 0x00000008);
}
/**
*
* prewrite key is used only in Prewrite binlog type.
* It is the primary key of the transaction, is used to check that the transaction is
* commited or not if it failed to pair to commit log or rollback log within a time window.
*
*
* optional bytes prewrite_key = 4;
*/
public com.google.protobuf.ByteString getPrewriteKey() {
return prewriteKey_;
}
/**
*
* prewrite key is used only in Prewrite binlog type.
* It is the primary key of the transaction, is used to check that the transaction is
* commited or not if it failed to pair to commit log or rollback log within a time window.
*
*
* optional bytes prewrite_key = 4;
*/
public Builder setPrewriteKey(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000008;
prewriteKey_ = value;
onChanged();
return this;
}
/**
*
* prewrite key is used only in Prewrite binlog type.
* It is the primary key of the transaction, is used to check that the transaction is
* commited or not if it failed to pair to commit log or rollback log within a time window.
*
*
* optional bytes prewrite_key = 4;
*/
public Builder clearPrewriteKey() {
bitField0_ = (bitField0_ & ~0x00000008);
prewriteKey_ = getDefaultInstance().getPrewriteKey();
onChanged();
return this;
}
private com.google.protobuf.ByteString prewriteValue_ = com.google.protobuf.ByteString.EMPTY;
/**
*
* prewrite_data is marshalled from PrewriteData type,
* we do not need to unmarshal prewrite data before the binlog have been successfully paired.
*
*
* optional bytes prewrite_value = 5;
*/
public boolean hasPrewriteValue() {
return ((bitField0_ & 0x00000010) == 0x00000010);
}
/**
*
* prewrite_data is marshalled from PrewriteData type,
* we do not need to unmarshal prewrite data before the binlog have been successfully paired.
*
*
* optional bytes prewrite_value = 5;
*/
public com.google.protobuf.ByteString getPrewriteValue() {
return prewriteValue_;
}
/**
*
* prewrite_data is marshalled from PrewriteData type,
* we do not need to unmarshal prewrite data before the binlog have been successfully paired.
*
*
* optional bytes prewrite_value = 5;
*/
public Builder setPrewriteValue(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000010;
prewriteValue_ = value;
onChanged();
return this;
}
/**
*
* prewrite_data is marshalled from PrewriteData type,
* we do not need to unmarshal prewrite data before the binlog have been successfully paired.
*
*
* optional bytes prewrite_value = 5;
*/
public Builder clearPrewriteValue() {
bitField0_ = (bitField0_ & ~0x00000010);
prewriteValue_ = getDefaultInstance().getPrewriteValue();
onChanged();
return this;
}
private com.google.protobuf.ByteString ddlQuery_ = com.google.protobuf.ByteString.EMPTY;
/**
*
* ddl_query is the original DDL statement query.
*
*
* optional bytes ddl_query = 6;
*/
public boolean hasDdlQuery() {
return ((bitField0_ & 0x00000020) == 0x00000020);
}
/**
*
* ddl_query is the original DDL statement query.
*
*
* optional bytes ddl_query = 6;
*/
public com.google.protobuf.ByteString getDdlQuery() {
return ddlQuery_;
}
/**
*
* ddl_query is the original DDL statement query.
*
*
* optional bytes ddl_query = 6;
*/
public Builder setDdlQuery(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000020;
ddlQuery_ = value;
onChanged();
return this;
}
/**
*
* ddl_query is the original DDL statement query.
*
*
* optional bytes ddl_query = 6;
*/
public Builder clearDdlQuery() {
bitField0_ = (bitField0_ & ~0x00000020);
ddlQuery_ = getDefaultInstance().getDdlQuery();
onChanged();
return this;
}
private long ddlJobId_ ;
/**
*
* ddl_job_id is used for DDL Binlog.
* If ddl_job_id is setted, this is a DDL Binlog and ddl_query contains the DDL query, we can query the informations about this job from TiKV.
*
*
* optional int64 ddl_job_id = 7 [(.gogoproto.nullable) = false];
*/
public boolean hasDdlJobId() {
return ((bitField0_ & 0x00000040) == 0x00000040);
}
/**
*
* ddl_job_id is used for DDL Binlog.
* If ddl_job_id is setted, this is a DDL Binlog and ddl_query contains the DDL query, we can query the informations about this job from TiKV.
*
*
* optional int64 ddl_job_id = 7 [(.gogoproto.nullable) = false];
*/
public long getDdlJobId() {
return ddlJobId_;
}
/**
*
* ddl_job_id is used for DDL Binlog.
* If ddl_job_id is setted, this is a DDL Binlog and ddl_query contains the DDL query, we can query the informations about this job from TiKV.
*
*
* optional int64 ddl_job_id = 7 [(.gogoproto.nullable) = false];
*/
public Builder setDdlJobId(long value) {
bitField0_ |= 0x00000040;
ddlJobId_ = value;
onChanged();
return this;
}
/**
*
* ddl_job_id is used for DDL Binlog.
* If ddl_job_id is setted, this is a DDL Binlog and ddl_query contains the DDL query, we can query the informations about this job from TiKV.
*
*
* optional int64 ddl_job_id = 7 [(.gogoproto.nullable) = false];
*/
public Builder clearDdlJobId() {
bitField0_ = (bitField0_ & ~0x00000040);
ddlJobId_ = 0L;
onChanged();
return this;
}
private int ddlSchemaState_ ;
/**
*
* ddl_schema_state is used for DDL Binlog.
*
*
* optional int32 ddl_schema_state = 8 [(.gogoproto.nullable) = false];
*/
public boolean hasDdlSchemaState() {
return ((bitField0_ & 0x00000080) == 0x00000080);
}
/**
*
* ddl_schema_state is used for DDL Binlog.
*
*
* optional int32 ddl_schema_state = 8 [(.gogoproto.nullable) = false];
*/
public int getDdlSchemaState() {
return ddlSchemaState_;
}
/**
*
* ddl_schema_state is used for DDL Binlog.
*
*
* optional int32 ddl_schema_state = 8 [(.gogoproto.nullable) = false];
*/
public Builder setDdlSchemaState(int value) {
bitField0_ |= 0x00000080;
ddlSchemaState_ = value;
onChanged();
return this;
}
/**
*
* ddl_schema_state is used for DDL Binlog.
*
*
* optional int32 ddl_schema_state = 8 [(.gogoproto.nullable) = false];
*/
public Builder clearDdlSchemaState() {
bitField0_ = (bitField0_ & ~0x00000080);
ddlSchemaState_ = 0;
onChanged();
return this;
}
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:binlog.Binlog)
}
// @@protoc_insertion_point(class_scope:binlog.Binlog)
private static final binlog.BinlogOuterClass.Binlog DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new binlog.BinlogOuterClass.Binlog();
}
public static binlog.BinlogOuterClass.Binlog getDefaultInstance() {
return DEFAULT_INSTANCE;
}
@java.lang.Deprecated public static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
public Binlog parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new Binlog(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
public binlog.BinlogOuterClass.Binlog getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_binlog_TableMutation_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_binlog_TableMutation_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_binlog_PrewriteValue_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_binlog_PrewriteValue_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_binlog_Binlog_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_binlog_Binlog_fieldAccessorTable;
public static com.google.protobuf.Descriptors.FileDescriptor
getDescriptor() {
return descriptor;
}
private static com.google.protobuf.Descriptors.FileDescriptor
descriptor;
static {
java.lang.String[] descriptorData = {
"\n\023binlog/binlog.proto\022\006binlog\032\024gogoproto" +
"/gogo.proto\"\274\001\n\rTableMutation\022\026\n\010table_i" +
"d\030\001 \001(\003B\004\310\336\037\000\022\025\n\rinserted_rows\030\002 \003(\014\022\024\n\014" +
"updated_rows\030\003 \003(\014\022\023\n\013deleted_ids\030\004 \003(\003\022" +
"\023\n\013deleted_pks\030\005 \003(\014\022\024\n\014deleted_rows\030\006 \003" +
"(\014\022&\n\010sequence\030\007 \003(\0162\024.binlog.MutationTy" +
"pe\"]\n\rPrewriteValue\022\034\n\016schema_version\030\001 " +
"\001(\003B\004\310\336\037\000\022.\n\tmutations\030\002 \003(\0132\025.binlog.Ta" +
"bleMutationB\004\310\336\037\000\"\332\001\n\006Binlog\022$\n\002tp\030\001 \001(\016" +
"2\022.binlog.BinlogTypeB\004\310\336\037\000\022\026\n\010start_ts\030\002" +
" \001(\003B\004\310\336\037\000\022\027\n\tcommit_ts\030\003 \001(\003B\004\310\336\037\000\022\024\n\014p" +
"rewrite_key\030\004 \001(\014\022\026\n\016prewrite_value\030\005 \001(" +
"\014\022\021\n\tddl_query\030\006 \001(\014\022\030\n\nddl_job_id\030\007 \001(\003" +
"B\004\310\336\037\000\022\036\n\020ddl_schema_state\030\010 \001(\005B\004\310\336\037\000*Q" +
"\n\014MutationType\022\n\n\006Insert\020\000\022\n\n\006Update\020\001\022\014" +
"\n\010DeleteID\020\002\022\014\n\010DeletePK\020\003\022\r\n\tDeleteRow\020" +
"\004*M\n\nBinlogType\022\014\n\010Prewrite\020\000\022\n\n\006Commit\020" +
"\001\022\014\n\010Rollback\020\002\022\n\n\006PreDDL\020\003\022\013\n\007PostDDL\020\004" +
"B\014\310\342\036\001\340\342\036\001\320\342\036\001"
};
com.google.protobuf.Descriptors.FileDescriptor.InternalDescriptorAssigner assigner =
new com.google.protobuf.Descriptors.FileDescriptor. InternalDescriptorAssigner() {
public com.google.protobuf.ExtensionRegistry assignDescriptors(
com.google.protobuf.Descriptors.FileDescriptor root) {
descriptor = root;
return null;
}
};
com.google.protobuf.Descriptors.FileDescriptor
.internalBuildGeneratedFileFrom(descriptorData,
new com.google.protobuf.Descriptors.FileDescriptor[] {
com.google.protobuf.GoGoProtos.getDescriptor(),
}, assigner);
internal_static_binlog_TableMutation_descriptor =
getDescriptor().getMessageTypes().get(0);
internal_static_binlog_TableMutation_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_binlog_TableMutation_descriptor,
new java.lang.String[] { "TableId", "InsertedRows", "UpdatedRows", "DeletedIds", "DeletedPks", "DeletedRows", "Sequence", });
internal_static_binlog_PrewriteValue_descriptor =
getDescriptor().getMessageTypes().get(1);
internal_static_binlog_PrewriteValue_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_binlog_PrewriteValue_descriptor,
new java.lang.String[] { "SchemaVersion", "Mutations", });
internal_static_binlog_Binlog_descriptor =
getDescriptor().getMessageTypes().get(2);
internal_static_binlog_Binlog_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_binlog_Binlog_descriptor,
new java.lang.String[] { "Tp", "StartTs", "CommitTs", "PrewriteKey", "PrewriteValue", "DdlQuery", "DdlJobId", "DdlSchemaState", });
com.google.protobuf.ExtensionRegistry registry =
com.google.protobuf.ExtensionRegistry.newInstance();
registry.add(com.google.protobuf.GoGoProtos.marshalerAll);
registry.add(com.google.protobuf.GoGoProtos.nullable);
registry.add(com.google.protobuf.GoGoProtos.sizerAll);
registry.add(com.google.protobuf.GoGoProtos.unmarshalerAll);
com.google.protobuf.Descriptors.FileDescriptor
.internalUpdateFileDescriptor(descriptor, registry);
com.google.protobuf.GoGoProtos.getDescriptor();
}
// @@protoc_insertion_point(outer_class_scope)
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy