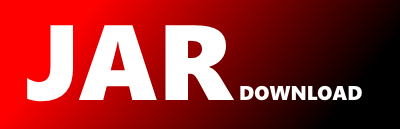
com.pingcap.tidb.tipb.AnalyzeReq Maven / Gradle / Ivy
// Generated by the protocol buffer compiler. DO NOT EDIT!
// source: analyze.proto
package com.pingcap.tidb.tipb;
/**
* Protobuf type {@code tipb.AnalyzeReq}
*/
public final class AnalyzeReq extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:tipb.AnalyzeReq)
AnalyzeReqOrBuilder {
private static final long serialVersionUID = 0L;
// Use AnalyzeReq.newBuilder() to construct.
private AnalyzeReq(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private AnalyzeReq() {
tp_ = 0;
startTsFallback_ = 0L;
flags_ = 0L;
timeZoneOffset_ = 0L;
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private AnalyzeReq(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
default: {
if (!parseUnknownField(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
case 8: {
int rawValue = input.readEnum();
com.pingcap.tidb.tipb.AnalyzeType value = com.pingcap.tidb.tipb.AnalyzeType.valueOf(rawValue);
if (value == null) {
unknownFields.mergeVarintField(1, rawValue);
} else {
bitField0_ |= 0x00000001;
tp_ = rawValue;
}
break;
}
case 16: {
bitField0_ |= 0x00000002;
startTsFallback_ = input.readUInt64();
break;
}
case 24: {
bitField0_ |= 0x00000004;
flags_ = input.readUInt64();
break;
}
case 32: {
bitField0_ |= 0x00000008;
timeZoneOffset_ = input.readInt64();
break;
}
case 42: {
com.pingcap.tidb.tipb.AnalyzeIndexReq.Builder subBuilder = null;
if (((bitField0_ & 0x00000010) == 0x00000010)) {
subBuilder = idxReq_.toBuilder();
}
idxReq_ = input.readMessage(com.pingcap.tidb.tipb.AnalyzeIndexReq.PARSER, extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(idxReq_);
idxReq_ = subBuilder.buildPartial();
}
bitField0_ |= 0x00000010;
break;
}
case 50: {
com.pingcap.tidb.tipb.AnalyzeColumnsReq.Builder subBuilder = null;
if (((bitField0_ & 0x00000020) == 0x00000020)) {
subBuilder = colReq_.toBuilder();
}
colReq_ = input.readMessage(com.pingcap.tidb.tipb.AnalyzeColumnsReq.PARSER, extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(colReq_);
colReq_ = subBuilder.buildPartial();
}
bitField0_ |= 0x00000020;
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.pingcap.tidb.tipb.Analyze.internal_static_tipb_AnalyzeReq_descriptor;
}
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.pingcap.tidb.tipb.Analyze.internal_static_tipb_AnalyzeReq_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.pingcap.tidb.tipb.AnalyzeReq.class, com.pingcap.tidb.tipb.AnalyzeReq.Builder.class);
}
private int bitField0_;
public static final int TP_FIELD_NUMBER = 1;
private int tp_;
/**
* optional .tipb.AnalyzeType tp = 1 [(.gogoproto.nullable) = false];
*/
public boolean hasTp() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
/**
* optional .tipb.AnalyzeType tp = 1 [(.gogoproto.nullable) = false];
*/
public com.pingcap.tidb.tipb.AnalyzeType getTp() {
com.pingcap.tidb.tipb.AnalyzeType result = com.pingcap.tidb.tipb.AnalyzeType.valueOf(tp_);
return result == null ? com.pingcap.tidb.tipb.AnalyzeType.TypeIndex : result;
}
public static final int START_TS_FALLBACK_FIELD_NUMBER = 2;
private long startTsFallback_;
/**
*
* Deprecated. Start Ts has been moved to coprocessor.Request.
*
*
* optional uint64 start_ts_fallback = 2;
*/
public boolean hasStartTsFallback() {
return ((bitField0_ & 0x00000002) == 0x00000002);
}
/**
*
* Deprecated. Start Ts has been moved to coprocessor.Request.
*
*
* optional uint64 start_ts_fallback = 2;
*/
public long getStartTsFallback() {
return startTsFallback_;
}
public static final int FLAGS_FIELD_NUMBER = 3;
private long flags_;
/**
* optional uint64 flags = 3 [(.gogoproto.nullable) = false];
*/
public boolean hasFlags() {
return ((bitField0_ & 0x00000004) == 0x00000004);
}
/**
* optional uint64 flags = 3 [(.gogoproto.nullable) = false];
*/
public long getFlags() {
return flags_;
}
public static final int TIME_ZONE_OFFSET_FIELD_NUMBER = 4;
private long timeZoneOffset_;
/**
* optional int64 time_zone_offset = 4 [(.gogoproto.nullable) = false];
*/
public boolean hasTimeZoneOffset() {
return ((bitField0_ & 0x00000008) == 0x00000008);
}
/**
* optional int64 time_zone_offset = 4 [(.gogoproto.nullable) = false];
*/
public long getTimeZoneOffset() {
return timeZoneOffset_;
}
public static final int IDX_REQ_FIELD_NUMBER = 5;
private com.pingcap.tidb.tipb.AnalyzeIndexReq idxReq_;
/**
* optional .tipb.AnalyzeIndexReq idx_req = 5;
*/
public boolean hasIdxReq() {
return ((bitField0_ & 0x00000010) == 0x00000010);
}
/**
* optional .tipb.AnalyzeIndexReq idx_req = 5;
*/
public com.pingcap.tidb.tipb.AnalyzeIndexReq getIdxReq() {
return idxReq_ == null ? com.pingcap.tidb.tipb.AnalyzeIndexReq.getDefaultInstance() : idxReq_;
}
/**
* optional .tipb.AnalyzeIndexReq idx_req = 5;
*/
public com.pingcap.tidb.tipb.AnalyzeIndexReqOrBuilder getIdxReqOrBuilder() {
return idxReq_ == null ? com.pingcap.tidb.tipb.AnalyzeIndexReq.getDefaultInstance() : idxReq_;
}
public static final int COL_REQ_FIELD_NUMBER = 6;
private com.pingcap.tidb.tipb.AnalyzeColumnsReq colReq_;
/**
* optional .tipb.AnalyzeColumnsReq col_req = 6;
*/
public boolean hasColReq() {
return ((bitField0_ & 0x00000020) == 0x00000020);
}
/**
* optional .tipb.AnalyzeColumnsReq col_req = 6;
*/
public com.pingcap.tidb.tipb.AnalyzeColumnsReq getColReq() {
return colReq_ == null ? com.pingcap.tidb.tipb.AnalyzeColumnsReq.getDefaultInstance() : colReq_;
}
/**
* optional .tipb.AnalyzeColumnsReq col_req = 6;
*/
public com.pingcap.tidb.tipb.AnalyzeColumnsReqOrBuilder getColReqOrBuilder() {
return colReq_ == null ? com.pingcap.tidb.tipb.AnalyzeColumnsReq.getDefaultInstance() : colReq_;
}
private byte memoizedIsInitialized = -1;
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (((bitField0_ & 0x00000001) == 0x00000001)) {
output.writeEnum(1, tp_);
}
if (((bitField0_ & 0x00000002) == 0x00000002)) {
output.writeUInt64(2, startTsFallback_);
}
if (((bitField0_ & 0x00000004) == 0x00000004)) {
output.writeUInt64(3, flags_);
}
if (((bitField0_ & 0x00000008) == 0x00000008)) {
output.writeInt64(4, timeZoneOffset_);
}
if (((bitField0_ & 0x00000010) == 0x00000010)) {
output.writeMessage(5, getIdxReq());
}
if (((bitField0_ & 0x00000020) == 0x00000020)) {
output.writeMessage(6, getColReq());
}
unknownFields.writeTo(output);
}
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (((bitField0_ & 0x00000001) == 0x00000001)) {
size += com.google.protobuf.CodedOutputStream
.computeEnumSize(1, tp_);
}
if (((bitField0_ & 0x00000002) == 0x00000002)) {
size += com.google.protobuf.CodedOutputStream
.computeUInt64Size(2, startTsFallback_);
}
if (((bitField0_ & 0x00000004) == 0x00000004)) {
size += com.google.protobuf.CodedOutputStream
.computeUInt64Size(3, flags_);
}
if (((bitField0_ & 0x00000008) == 0x00000008)) {
size += com.google.protobuf.CodedOutputStream
.computeInt64Size(4, timeZoneOffset_);
}
if (((bitField0_ & 0x00000010) == 0x00000010)) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(5, getIdxReq());
}
if (((bitField0_ & 0x00000020) == 0x00000020)) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(6, getColReq());
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof com.pingcap.tidb.tipb.AnalyzeReq)) {
return super.equals(obj);
}
com.pingcap.tidb.tipb.AnalyzeReq other = (com.pingcap.tidb.tipb.AnalyzeReq) obj;
boolean result = true;
result = result && (hasTp() == other.hasTp());
if (hasTp()) {
result = result && tp_ == other.tp_;
}
result = result && (hasStartTsFallback() == other.hasStartTsFallback());
if (hasStartTsFallback()) {
result = result && (getStartTsFallback()
== other.getStartTsFallback());
}
result = result && (hasFlags() == other.hasFlags());
if (hasFlags()) {
result = result && (getFlags()
== other.getFlags());
}
result = result && (hasTimeZoneOffset() == other.hasTimeZoneOffset());
if (hasTimeZoneOffset()) {
result = result && (getTimeZoneOffset()
== other.getTimeZoneOffset());
}
result = result && (hasIdxReq() == other.hasIdxReq());
if (hasIdxReq()) {
result = result && getIdxReq()
.equals(other.getIdxReq());
}
result = result && (hasColReq() == other.hasColReq());
if (hasColReq()) {
result = result && getColReq()
.equals(other.getColReq());
}
result = result && unknownFields.equals(other.unknownFields);
return result;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (hasTp()) {
hash = (37 * hash) + TP_FIELD_NUMBER;
hash = (53 * hash) + tp_;
}
if (hasStartTsFallback()) {
hash = (37 * hash) + START_TS_FALLBACK_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
getStartTsFallback());
}
if (hasFlags()) {
hash = (37 * hash) + FLAGS_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
getFlags());
}
if (hasTimeZoneOffset()) {
hash = (37 * hash) + TIME_ZONE_OFFSET_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
getTimeZoneOffset());
}
if (hasIdxReq()) {
hash = (37 * hash) + IDX_REQ_FIELD_NUMBER;
hash = (53 * hash) + getIdxReq().hashCode();
}
if (hasColReq()) {
hash = (37 * hash) + COL_REQ_FIELD_NUMBER;
hash = (53 * hash) + getColReq().hashCode();
}
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static com.pingcap.tidb.tipb.AnalyzeReq parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.pingcap.tidb.tipb.AnalyzeReq parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.pingcap.tidb.tipb.AnalyzeReq parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.pingcap.tidb.tipb.AnalyzeReq parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.pingcap.tidb.tipb.AnalyzeReq parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.pingcap.tidb.tipb.AnalyzeReq parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.pingcap.tidb.tipb.AnalyzeReq parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.pingcap.tidb.tipb.AnalyzeReq parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static com.pingcap.tidb.tipb.AnalyzeReq parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static com.pingcap.tidb.tipb.AnalyzeReq parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static com.pingcap.tidb.tipb.AnalyzeReq parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.pingcap.tidb.tipb.AnalyzeReq parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(com.pingcap.tidb.tipb.AnalyzeReq prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code tipb.AnalyzeReq}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:tipb.AnalyzeReq)
com.pingcap.tidb.tipb.AnalyzeReqOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.pingcap.tidb.tipb.Analyze.internal_static_tipb_AnalyzeReq_descriptor;
}
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.pingcap.tidb.tipb.Analyze.internal_static_tipb_AnalyzeReq_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.pingcap.tidb.tipb.AnalyzeReq.class, com.pingcap.tidb.tipb.AnalyzeReq.Builder.class);
}
// Construct using com.pingcap.tidb.tipb.AnalyzeReq.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
getIdxReqFieldBuilder();
getColReqFieldBuilder();
}
}
public Builder clear() {
super.clear();
tp_ = 0;
bitField0_ = (bitField0_ & ~0x00000001);
startTsFallback_ = 0L;
bitField0_ = (bitField0_ & ~0x00000002);
flags_ = 0L;
bitField0_ = (bitField0_ & ~0x00000004);
timeZoneOffset_ = 0L;
bitField0_ = (bitField0_ & ~0x00000008);
if (idxReqBuilder_ == null) {
idxReq_ = null;
} else {
idxReqBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000010);
if (colReqBuilder_ == null) {
colReq_ = null;
} else {
colReqBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000020);
return this;
}
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return com.pingcap.tidb.tipb.Analyze.internal_static_tipb_AnalyzeReq_descriptor;
}
public com.pingcap.tidb.tipb.AnalyzeReq getDefaultInstanceForType() {
return com.pingcap.tidb.tipb.AnalyzeReq.getDefaultInstance();
}
public com.pingcap.tidb.tipb.AnalyzeReq build() {
com.pingcap.tidb.tipb.AnalyzeReq result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
public com.pingcap.tidb.tipb.AnalyzeReq buildPartial() {
com.pingcap.tidb.tipb.AnalyzeReq result = new com.pingcap.tidb.tipb.AnalyzeReq(this);
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (((from_bitField0_ & 0x00000001) == 0x00000001)) {
to_bitField0_ |= 0x00000001;
}
result.tp_ = tp_;
if (((from_bitField0_ & 0x00000002) == 0x00000002)) {
to_bitField0_ |= 0x00000002;
}
result.startTsFallback_ = startTsFallback_;
if (((from_bitField0_ & 0x00000004) == 0x00000004)) {
to_bitField0_ |= 0x00000004;
}
result.flags_ = flags_;
if (((from_bitField0_ & 0x00000008) == 0x00000008)) {
to_bitField0_ |= 0x00000008;
}
result.timeZoneOffset_ = timeZoneOffset_;
if (((from_bitField0_ & 0x00000010) == 0x00000010)) {
to_bitField0_ |= 0x00000010;
}
if (idxReqBuilder_ == null) {
result.idxReq_ = idxReq_;
} else {
result.idxReq_ = idxReqBuilder_.build();
}
if (((from_bitField0_ & 0x00000020) == 0x00000020)) {
to_bitField0_ |= 0x00000020;
}
if (colReqBuilder_ == null) {
result.colReq_ = colReq_;
} else {
result.colReq_ = colReqBuilder_.build();
}
result.bitField0_ = to_bitField0_;
onBuilt();
return result;
}
public Builder clone() {
return (Builder) super.clone();
}
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.setField(field, value);
}
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return (Builder) super.clearField(field);
}
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return (Builder) super.clearOneof(oneof);
}
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return (Builder) super.setRepeatedField(field, index, value);
}
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.addRepeatedField(field, value);
}
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.pingcap.tidb.tipb.AnalyzeReq) {
return mergeFrom((com.pingcap.tidb.tipb.AnalyzeReq)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.pingcap.tidb.tipb.AnalyzeReq other) {
if (other == com.pingcap.tidb.tipb.AnalyzeReq.getDefaultInstance()) return this;
if (other.hasTp()) {
setTp(other.getTp());
}
if (other.hasStartTsFallback()) {
setStartTsFallback(other.getStartTsFallback());
}
if (other.hasFlags()) {
setFlags(other.getFlags());
}
if (other.hasTimeZoneOffset()) {
setTimeZoneOffset(other.getTimeZoneOffset());
}
if (other.hasIdxReq()) {
mergeIdxReq(other.getIdxReq());
}
if (other.hasColReq()) {
mergeColReq(other.getColReq());
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
public final boolean isInitialized() {
return true;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
com.pingcap.tidb.tipb.AnalyzeReq parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (com.pingcap.tidb.tipb.AnalyzeReq) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
private int tp_ = 0;
/**
* optional .tipb.AnalyzeType tp = 1 [(.gogoproto.nullable) = false];
*/
public boolean hasTp() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
/**
* optional .tipb.AnalyzeType tp = 1 [(.gogoproto.nullable) = false];
*/
public com.pingcap.tidb.tipb.AnalyzeType getTp() {
com.pingcap.tidb.tipb.AnalyzeType result = com.pingcap.tidb.tipb.AnalyzeType.valueOf(tp_);
return result == null ? com.pingcap.tidb.tipb.AnalyzeType.TypeIndex : result;
}
/**
* optional .tipb.AnalyzeType tp = 1 [(.gogoproto.nullable) = false];
*/
public Builder setTp(com.pingcap.tidb.tipb.AnalyzeType value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000001;
tp_ = value.getNumber();
onChanged();
return this;
}
/**
* optional .tipb.AnalyzeType tp = 1 [(.gogoproto.nullable) = false];
*/
public Builder clearTp() {
bitField0_ = (bitField0_ & ~0x00000001);
tp_ = 0;
onChanged();
return this;
}
private long startTsFallback_ ;
/**
*
* Deprecated. Start Ts has been moved to coprocessor.Request.
*
*
* optional uint64 start_ts_fallback = 2;
*/
public boolean hasStartTsFallback() {
return ((bitField0_ & 0x00000002) == 0x00000002);
}
/**
*
* Deprecated. Start Ts has been moved to coprocessor.Request.
*
*
* optional uint64 start_ts_fallback = 2;
*/
public long getStartTsFallback() {
return startTsFallback_;
}
/**
*
* Deprecated. Start Ts has been moved to coprocessor.Request.
*
*
* optional uint64 start_ts_fallback = 2;
*/
public Builder setStartTsFallback(long value) {
bitField0_ |= 0x00000002;
startTsFallback_ = value;
onChanged();
return this;
}
/**
*
* Deprecated. Start Ts has been moved to coprocessor.Request.
*
*
* optional uint64 start_ts_fallback = 2;
*/
public Builder clearStartTsFallback() {
bitField0_ = (bitField0_ & ~0x00000002);
startTsFallback_ = 0L;
onChanged();
return this;
}
private long flags_ ;
/**
* optional uint64 flags = 3 [(.gogoproto.nullable) = false];
*/
public boolean hasFlags() {
return ((bitField0_ & 0x00000004) == 0x00000004);
}
/**
* optional uint64 flags = 3 [(.gogoproto.nullable) = false];
*/
public long getFlags() {
return flags_;
}
/**
* optional uint64 flags = 3 [(.gogoproto.nullable) = false];
*/
public Builder setFlags(long value) {
bitField0_ |= 0x00000004;
flags_ = value;
onChanged();
return this;
}
/**
* optional uint64 flags = 3 [(.gogoproto.nullable) = false];
*/
public Builder clearFlags() {
bitField0_ = (bitField0_ & ~0x00000004);
flags_ = 0L;
onChanged();
return this;
}
private long timeZoneOffset_ ;
/**
* optional int64 time_zone_offset = 4 [(.gogoproto.nullable) = false];
*/
public boolean hasTimeZoneOffset() {
return ((bitField0_ & 0x00000008) == 0x00000008);
}
/**
* optional int64 time_zone_offset = 4 [(.gogoproto.nullable) = false];
*/
public long getTimeZoneOffset() {
return timeZoneOffset_;
}
/**
* optional int64 time_zone_offset = 4 [(.gogoproto.nullable) = false];
*/
public Builder setTimeZoneOffset(long value) {
bitField0_ |= 0x00000008;
timeZoneOffset_ = value;
onChanged();
return this;
}
/**
* optional int64 time_zone_offset = 4 [(.gogoproto.nullable) = false];
*/
public Builder clearTimeZoneOffset() {
bitField0_ = (bitField0_ & ~0x00000008);
timeZoneOffset_ = 0L;
onChanged();
return this;
}
private com.pingcap.tidb.tipb.AnalyzeIndexReq idxReq_ = null;
private com.google.protobuf.SingleFieldBuilderV3<
com.pingcap.tidb.tipb.AnalyzeIndexReq, com.pingcap.tidb.tipb.AnalyzeIndexReq.Builder, com.pingcap.tidb.tipb.AnalyzeIndexReqOrBuilder> idxReqBuilder_;
/**
* optional .tipb.AnalyzeIndexReq idx_req = 5;
*/
public boolean hasIdxReq() {
return ((bitField0_ & 0x00000010) == 0x00000010);
}
/**
* optional .tipb.AnalyzeIndexReq idx_req = 5;
*/
public com.pingcap.tidb.tipb.AnalyzeIndexReq getIdxReq() {
if (idxReqBuilder_ == null) {
return idxReq_ == null ? com.pingcap.tidb.tipb.AnalyzeIndexReq.getDefaultInstance() : idxReq_;
} else {
return idxReqBuilder_.getMessage();
}
}
/**
* optional .tipb.AnalyzeIndexReq idx_req = 5;
*/
public Builder setIdxReq(com.pingcap.tidb.tipb.AnalyzeIndexReq value) {
if (idxReqBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
idxReq_ = value;
onChanged();
} else {
idxReqBuilder_.setMessage(value);
}
bitField0_ |= 0x00000010;
return this;
}
/**
* optional .tipb.AnalyzeIndexReq idx_req = 5;
*/
public Builder setIdxReq(
com.pingcap.tidb.tipb.AnalyzeIndexReq.Builder builderForValue) {
if (idxReqBuilder_ == null) {
idxReq_ = builderForValue.build();
onChanged();
} else {
idxReqBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x00000010;
return this;
}
/**
* optional .tipb.AnalyzeIndexReq idx_req = 5;
*/
public Builder mergeIdxReq(com.pingcap.tidb.tipb.AnalyzeIndexReq value) {
if (idxReqBuilder_ == null) {
if (((bitField0_ & 0x00000010) == 0x00000010) &&
idxReq_ != null &&
idxReq_ != com.pingcap.tidb.tipb.AnalyzeIndexReq.getDefaultInstance()) {
idxReq_ =
com.pingcap.tidb.tipb.AnalyzeIndexReq.newBuilder(idxReq_).mergeFrom(value).buildPartial();
} else {
idxReq_ = value;
}
onChanged();
} else {
idxReqBuilder_.mergeFrom(value);
}
bitField0_ |= 0x00000010;
return this;
}
/**
* optional .tipb.AnalyzeIndexReq idx_req = 5;
*/
public Builder clearIdxReq() {
if (idxReqBuilder_ == null) {
idxReq_ = null;
onChanged();
} else {
idxReqBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000010);
return this;
}
/**
* optional .tipb.AnalyzeIndexReq idx_req = 5;
*/
public com.pingcap.tidb.tipb.AnalyzeIndexReq.Builder getIdxReqBuilder() {
bitField0_ |= 0x00000010;
onChanged();
return getIdxReqFieldBuilder().getBuilder();
}
/**
* optional .tipb.AnalyzeIndexReq idx_req = 5;
*/
public com.pingcap.tidb.tipb.AnalyzeIndexReqOrBuilder getIdxReqOrBuilder() {
if (idxReqBuilder_ != null) {
return idxReqBuilder_.getMessageOrBuilder();
} else {
return idxReq_ == null ?
com.pingcap.tidb.tipb.AnalyzeIndexReq.getDefaultInstance() : idxReq_;
}
}
/**
* optional .tipb.AnalyzeIndexReq idx_req = 5;
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.pingcap.tidb.tipb.AnalyzeIndexReq, com.pingcap.tidb.tipb.AnalyzeIndexReq.Builder, com.pingcap.tidb.tipb.AnalyzeIndexReqOrBuilder>
getIdxReqFieldBuilder() {
if (idxReqBuilder_ == null) {
idxReqBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
com.pingcap.tidb.tipb.AnalyzeIndexReq, com.pingcap.tidb.tipb.AnalyzeIndexReq.Builder, com.pingcap.tidb.tipb.AnalyzeIndexReqOrBuilder>(
getIdxReq(),
getParentForChildren(),
isClean());
idxReq_ = null;
}
return idxReqBuilder_;
}
private com.pingcap.tidb.tipb.AnalyzeColumnsReq colReq_ = null;
private com.google.protobuf.SingleFieldBuilderV3<
com.pingcap.tidb.tipb.AnalyzeColumnsReq, com.pingcap.tidb.tipb.AnalyzeColumnsReq.Builder, com.pingcap.tidb.tipb.AnalyzeColumnsReqOrBuilder> colReqBuilder_;
/**
* optional .tipb.AnalyzeColumnsReq col_req = 6;
*/
public boolean hasColReq() {
return ((bitField0_ & 0x00000020) == 0x00000020);
}
/**
* optional .tipb.AnalyzeColumnsReq col_req = 6;
*/
public com.pingcap.tidb.tipb.AnalyzeColumnsReq getColReq() {
if (colReqBuilder_ == null) {
return colReq_ == null ? com.pingcap.tidb.tipb.AnalyzeColumnsReq.getDefaultInstance() : colReq_;
} else {
return colReqBuilder_.getMessage();
}
}
/**
* optional .tipb.AnalyzeColumnsReq col_req = 6;
*/
public Builder setColReq(com.pingcap.tidb.tipb.AnalyzeColumnsReq value) {
if (colReqBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
colReq_ = value;
onChanged();
} else {
colReqBuilder_.setMessage(value);
}
bitField0_ |= 0x00000020;
return this;
}
/**
* optional .tipb.AnalyzeColumnsReq col_req = 6;
*/
public Builder setColReq(
com.pingcap.tidb.tipb.AnalyzeColumnsReq.Builder builderForValue) {
if (colReqBuilder_ == null) {
colReq_ = builderForValue.build();
onChanged();
} else {
colReqBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x00000020;
return this;
}
/**
* optional .tipb.AnalyzeColumnsReq col_req = 6;
*/
public Builder mergeColReq(com.pingcap.tidb.tipb.AnalyzeColumnsReq value) {
if (colReqBuilder_ == null) {
if (((bitField0_ & 0x00000020) == 0x00000020) &&
colReq_ != null &&
colReq_ != com.pingcap.tidb.tipb.AnalyzeColumnsReq.getDefaultInstance()) {
colReq_ =
com.pingcap.tidb.tipb.AnalyzeColumnsReq.newBuilder(colReq_).mergeFrom(value).buildPartial();
} else {
colReq_ = value;
}
onChanged();
} else {
colReqBuilder_.mergeFrom(value);
}
bitField0_ |= 0x00000020;
return this;
}
/**
* optional .tipb.AnalyzeColumnsReq col_req = 6;
*/
public Builder clearColReq() {
if (colReqBuilder_ == null) {
colReq_ = null;
onChanged();
} else {
colReqBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000020);
return this;
}
/**
* optional .tipb.AnalyzeColumnsReq col_req = 6;
*/
public com.pingcap.tidb.tipb.AnalyzeColumnsReq.Builder getColReqBuilder() {
bitField0_ |= 0x00000020;
onChanged();
return getColReqFieldBuilder().getBuilder();
}
/**
* optional .tipb.AnalyzeColumnsReq col_req = 6;
*/
public com.pingcap.tidb.tipb.AnalyzeColumnsReqOrBuilder getColReqOrBuilder() {
if (colReqBuilder_ != null) {
return colReqBuilder_.getMessageOrBuilder();
} else {
return colReq_ == null ?
com.pingcap.tidb.tipb.AnalyzeColumnsReq.getDefaultInstance() : colReq_;
}
}
/**
* optional .tipb.AnalyzeColumnsReq col_req = 6;
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.pingcap.tidb.tipb.AnalyzeColumnsReq, com.pingcap.tidb.tipb.AnalyzeColumnsReq.Builder, com.pingcap.tidb.tipb.AnalyzeColumnsReqOrBuilder>
getColReqFieldBuilder() {
if (colReqBuilder_ == null) {
colReqBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
com.pingcap.tidb.tipb.AnalyzeColumnsReq, com.pingcap.tidb.tipb.AnalyzeColumnsReq.Builder, com.pingcap.tidb.tipb.AnalyzeColumnsReqOrBuilder>(
getColReq(),
getParentForChildren(),
isClean());
colReq_ = null;
}
return colReqBuilder_;
}
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:tipb.AnalyzeReq)
}
// @@protoc_insertion_point(class_scope:tipb.AnalyzeReq)
private static final com.pingcap.tidb.tipb.AnalyzeReq DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new com.pingcap.tidb.tipb.AnalyzeReq();
}
public static com.pingcap.tidb.tipb.AnalyzeReq getDefaultInstance() {
return DEFAULT_INSTANCE;
}
@java.lang.Deprecated public static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
public AnalyzeReq parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new AnalyzeReq(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
public com.pingcap.tidb.tipb.AnalyzeReq getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy