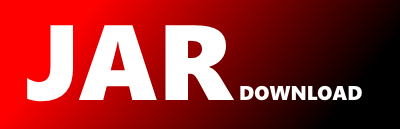
com.pingcap.tidb.tipb.Executor Maven / Gradle / Ivy
// Generated by the protocol buffer compiler. DO NOT EDIT!
// source: executor.proto
package com.pingcap.tidb.tipb;
/**
*
* It represents a Executor.
*
*
* Protobuf type {@code tipb.Executor}
*/
public final class Executor extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:tipb.Executor)
ExecutorOrBuilder {
private static final long serialVersionUID = 0L;
// Use Executor.newBuilder() to construct.
private Executor(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private Executor() {
tp_ = 0;
executorId_ = "";
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private Executor(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
default: {
if (!parseUnknownField(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
case 8: {
int rawValue = input.readEnum();
com.pingcap.tidb.tipb.ExecType value = com.pingcap.tidb.tipb.ExecType.valueOf(rawValue);
if (value == null) {
unknownFields.mergeVarintField(1, rawValue);
} else {
bitField0_ |= 0x00000001;
tp_ = rawValue;
}
break;
}
case 18: {
com.pingcap.tidb.tipb.TableScan.Builder subBuilder = null;
if (((bitField0_ & 0x00000002) == 0x00000002)) {
subBuilder = tblScan_.toBuilder();
}
tblScan_ = input.readMessage(com.pingcap.tidb.tipb.TableScan.PARSER, extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(tblScan_);
tblScan_ = subBuilder.buildPartial();
}
bitField0_ |= 0x00000002;
break;
}
case 26: {
com.pingcap.tidb.tipb.IndexScan.Builder subBuilder = null;
if (((bitField0_ & 0x00000004) == 0x00000004)) {
subBuilder = idxScan_.toBuilder();
}
idxScan_ = input.readMessage(com.pingcap.tidb.tipb.IndexScan.PARSER, extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(idxScan_);
idxScan_ = subBuilder.buildPartial();
}
bitField0_ |= 0x00000004;
break;
}
case 34: {
com.pingcap.tidb.tipb.Selection.Builder subBuilder = null;
if (((bitField0_ & 0x00000008) == 0x00000008)) {
subBuilder = selection_.toBuilder();
}
selection_ = input.readMessage(com.pingcap.tidb.tipb.Selection.PARSER, extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(selection_);
selection_ = subBuilder.buildPartial();
}
bitField0_ |= 0x00000008;
break;
}
case 42: {
com.pingcap.tidb.tipb.Aggregation.Builder subBuilder = null;
if (((bitField0_ & 0x00000010) == 0x00000010)) {
subBuilder = aggregation_.toBuilder();
}
aggregation_ = input.readMessage(com.pingcap.tidb.tipb.Aggregation.PARSER, extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(aggregation_);
aggregation_ = subBuilder.buildPartial();
}
bitField0_ |= 0x00000010;
break;
}
case 50: {
com.pingcap.tidb.tipb.TopN.Builder subBuilder = null;
if (((bitField0_ & 0x00000020) == 0x00000020)) {
subBuilder = topN_.toBuilder();
}
topN_ = input.readMessage(com.pingcap.tidb.tipb.TopN.PARSER, extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(topN_);
topN_ = subBuilder.buildPartial();
}
bitField0_ |= 0x00000020;
break;
}
case 58: {
com.pingcap.tidb.tipb.Limit.Builder subBuilder = null;
if (((bitField0_ & 0x00000040) == 0x00000040)) {
subBuilder = limit_.toBuilder();
}
limit_ = input.readMessage(com.pingcap.tidb.tipb.Limit.PARSER, extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(limit_);
limit_ = subBuilder.buildPartial();
}
bitField0_ |= 0x00000040;
break;
}
case 66: {
com.pingcap.tidb.tipb.ExchangeReceiver.Builder subBuilder = null;
if (((bitField0_ & 0x00000080) == 0x00000080)) {
subBuilder = exchangeReceiver_.toBuilder();
}
exchangeReceiver_ = input.readMessage(com.pingcap.tidb.tipb.ExchangeReceiver.PARSER, extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(exchangeReceiver_);
exchangeReceiver_ = subBuilder.buildPartial();
}
bitField0_ |= 0x00000080;
break;
}
case 74: {
com.pingcap.tidb.tipb.Join.Builder subBuilder = null;
if (((bitField0_ & 0x00000100) == 0x00000100)) {
subBuilder = join_.toBuilder();
}
join_ = input.readMessage(com.pingcap.tidb.tipb.Join.PARSER, extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(join_);
join_ = subBuilder.buildPartial();
}
bitField0_ |= 0x00000100;
break;
}
case 82: {
com.google.protobuf.ByteString bs = input.readBytes();
bitField0_ |= 0x00000200;
executorId_ = bs;
break;
}
case 90: {
com.pingcap.tidb.tipb.Kill.Builder subBuilder = null;
if (((bitField0_ & 0x00000400) == 0x00000400)) {
subBuilder = kill_.toBuilder();
}
kill_ = input.readMessage(com.pingcap.tidb.tipb.Kill.PARSER, extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(kill_);
kill_ = subBuilder.buildPartial();
}
bitField0_ |= 0x00000400;
break;
}
case 98: {
com.pingcap.tidb.tipb.ExchangeSender.Builder subBuilder = null;
if (((bitField0_ & 0x00000800) == 0x00000800)) {
subBuilder = exchangeSender_.toBuilder();
}
exchangeSender_ = input.readMessage(com.pingcap.tidb.tipb.ExchangeSender.PARSER, extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(exchangeSender_);
exchangeSender_ = subBuilder.buildPartial();
}
bitField0_ |= 0x00000800;
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.pingcap.tidb.tipb.ExecutorOuterClass.internal_static_tipb_Executor_descriptor;
}
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.pingcap.tidb.tipb.ExecutorOuterClass.internal_static_tipb_Executor_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.pingcap.tidb.tipb.Executor.class, com.pingcap.tidb.tipb.Executor.Builder.class);
}
private int bitField0_;
public static final int TP_FIELD_NUMBER = 1;
private int tp_;
/**
* optional .tipb.ExecType tp = 1 [(.gogoproto.nullable) = false];
*/
public boolean hasTp() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
/**
* optional .tipb.ExecType tp = 1 [(.gogoproto.nullable) = false];
*/
public com.pingcap.tidb.tipb.ExecType getTp() {
com.pingcap.tidb.tipb.ExecType result = com.pingcap.tidb.tipb.ExecType.valueOf(tp_);
return result == null ? com.pingcap.tidb.tipb.ExecType.TypeTableScan : result;
}
public static final int TBL_SCAN_FIELD_NUMBER = 2;
private com.pingcap.tidb.tipb.TableScan tblScan_;
/**
* optional .tipb.TableScan tbl_scan = 2;
*/
public boolean hasTblScan() {
return ((bitField0_ & 0x00000002) == 0x00000002);
}
/**
* optional .tipb.TableScan tbl_scan = 2;
*/
public com.pingcap.tidb.tipb.TableScan getTblScan() {
return tblScan_ == null ? com.pingcap.tidb.tipb.TableScan.getDefaultInstance() : tblScan_;
}
/**
* optional .tipb.TableScan tbl_scan = 2;
*/
public com.pingcap.tidb.tipb.TableScanOrBuilder getTblScanOrBuilder() {
return tblScan_ == null ? com.pingcap.tidb.tipb.TableScan.getDefaultInstance() : tblScan_;
}
public static final int IDX_SCAN_FIELD_NUMBER = 3;
private com.pingcap.tidb.tipb.IndexScan idxScan_;
/**
* optional .tipb.IndexScan idx_scan = 3;
*/
public boolean hasIdxScan() {
return ((bitField0_ & 0x00000004) == 0x00000004);
}
/**
* optional .tipb.IndexScan idx_scan = 3;
*/
public com.pingcap.tidb.tipb.IndexScan getIdxScan() {
return idxScan_ == null ? com.pingcap.tidb.tipb.IndexScan.getDefaultInstance() : idxScan_;
}
/**
* optional .tipb.IndexScan idx_scan = 3;
*/
public com.pingcap.tidb.tipb.IndexScanOrBuilder getIdxScanOrBuilder() {
return idxScan_ == null ? com.pingcap.tidb.tipb.IndexScan.getDefaultInstance() : idxScan_;
}
public static final int SELECTION_FIELD_NUMBER = 4;
private com.pingcap.tidb.tipb.Selection selection_;
/**
* optional .tipb.Selection selection = 4;
*/
public boolean hasSelection() {
return ((bitField0_ & 0x00000008) == 0x00000008);
}
/**
* optional .tipb.Selection selection = 4;
*/
public com.pingcap.tidb.tipb.Selection getSelection() {
return selection_ == null ? com.pingcap.tidb.tipb.Selection.getDefaultInstance() : selection_;
}
/**
* optional .tipb.Selection selection = 4;
*/
public com.pingcap.tidb.tipb.SelectionOrBuilder getSelectionOrBuilder() {
return selection_ == null ? com.pingcap.tidb.tipb.Selection.getDefaultInstance() : selection_;
}
public static final int AGGREGATION_FIELD_NUMBER = 5;
private com.pingcap.tidb.tipb.Aggregation aggregation_;
/**
* optional .tipb.Aggregation aggregation = 5;
*/
public boolean hasAggregation() {
return ((bitField0_ & 0x00000010) == 0x00000010);
}
/**
* optional .tipb.Aggregation aggregation = 5;
*/
public com.pingcap.tidb.tipb.Aggregation getAggregation() {
return aggregation_ == null ? com.pingcap.tidb.tipb.Aggregation.getDefaultInstance() : aggregation_;
}
/**
* optional .tipb.Aggregation aggregation = 5;
*/
public com.pingcap.tidb.tipb.AggregationOrBuilder getAggregationOrBuilder() {
return aggregation_ == null ? com.pingcap.tidb.tipb.Aggregation.getDefaultInstance() : aggregation_;
}
public static final int TOPN_FIELD_NUMBER = 6;
private com.pingcap.tidb.tipb.TopN topN_;
/**
* optional .tipb.TopN topN = 6;
*/
public boolean hasTopN() {
return ((bitField0_ & 0x00000020) == 0x00000020);
}
/**
* optional .tipb.TopN topN = 6;
*/
public com.pingcap.tidb.tipb.TopN getTopN() {
return topN_ == null ? com.pingcap.tidb.tipb.TopN.getDefaultInstance() : topN_;
}
/**
* optional .tipb.TopN topN = 6;
*/
public com.pingcap.tidb.tipb.TopNOrBuilder getTopNOrBuilder() {
return topN_ == null ? com.pingcap.tidb.tipb.TopN.getDefaultInstance() : topN_;
}
public static final int LIMIT_FIELD_NUMBER = 7;
private com.pingcap.tidb.tipb.Limit limit_;
/**
* optional .tipb.Limit limit = 7;
*/
public boolean hasLimit() {
return ((bitField0_ & 0x00000040) == 0x00000040);
}
/**
* optional .tipb.Limit limit = 7;
*/
public com.pingcap.tidb.tipb.Limit getLimit() {
return limit_ == null ? com.pingcap.tidb.tipb.Limit.getDefaultInstance() : limit_;
}
/**
* optional .tipb.Limit limit = 7;
*/
public com.pingcap.tidb.tipb.LimitOrBuilder getLimitOrBuilder() {
return limit_ == null ? com.pingcap.tidb.tipb.Limit.getDefaultInstance() : limit_;
}
public static final int EXCHANGE_RECEIVER_FIELD_NUMBER = 8;
private com.pingcap.tidb.tipb.ExchangeReceiver exchangeReceiver_;
/**
* optional .tipb.ExchangeReceiver exchange_receiver = 8;
*/
public boolean hasExchangeReceiver() {
return ((bitField0_ & 0x00000080) == 0x00000080);
}
/**
* optional .tipb.ExchangeReceiver exchange_receiver = 8;
*/
public com.pingcap.tidb.tipb.ExchangeReceiver getExchangeReceiver() {
return exchangeReceiver_ == null ? com.pingcap.tidb.tipb.ExchangeReceiver.getDefaultInstance() : exchangeReceiver_;
}
/**
* optional .tipb.ExchangeReceiver exchange_receiver = 8;
*/
public com.pingcap.tidb.tipb.ExchangeReceiverOrBuilder getExchangeReceiverOrBuilder() {
return exchangeReceiver_ == null ? com.pingcap.tidb.tipb.ExchangeReceiver.getDefaultInstance() : exchangeReceiver_;
}
public static final int JOIN_FIELD_NUMBER = 9;
private com.pingcap.tidb.tipb.Join join_;
/**
* optional .tipb.Join join = 9;
*/
public boolean hasJoin() {
return ((bitField0_ & 0x00000100) == 0x00000100);
}
/**
* optional .tipb.Join join = 9;
*/
public com.pingcap.tidb.tipb.Join getJoin() {
return join_ == null ? com.pingcap.tidb.tipb.Join.getDefaultInstance() : join_;
}
/**
* optional .tipb.Join join = 9;
*/
public com.pingcap.tidb.tipb.JoinOrBuilder getJoinOrBuilder() {
return join_ == null ? com.pingcap.tidb.tipb.Join.getDefaultInstance() : join_;
}
public static final int EXECUTOR_ID_FIELD_NUMBER = 10;
private volatile java.lang.Object executorId_;
/**
* optional string executor_id = 10;
*/
public boolean hasExecutorId() {
return ((bitField0_ & 0x00000200) == 0x00000200);
}
/**
* optional string executor_id = 10;
*/
public java.lang.String getExecutorId() {
java.lang.Object ref = executorId_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
executorId_ = s;
}
return s;
}
}
/**
* optional string executor_id = 10;
*/
public com.google.protobuf.ByteString
getExecutorIdBytes() {
java.lang.Object ref = executorId_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
executorId_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int KILL_FIELD_NUMBER = 11;
private com.pingcap.tidb.tipb.Kill kill_;
/**
* optional .tipb.Kill kill = 11;
*/
public boolean hasKill() {
return ((bitField0_ & 0x00000400) == 0x00000400);
}
/**
* optional .tipb.Kill kill = 11;
*/
public com.pingcap.tidb.tipb.Kill getKill() {
return kill_ == null ? com.pingcap.tidb.tipb.Kill.getDefaultInstance() : kill_;
}
/**
* optional .tipb.Kill kill = 11;
*/
public com.pingcap.tidb.tipb.KillOrBuilder getKillOrBuilder() {
return kill_ == null ? com.pingcap.tidb.tipb.Kill.getDefaultInstance() : kill_;
}
public static final int EXCHANGE_SENDER_FIELD_NUMBER = 12;
private com.pingcap.tidb.tipb.ExchangeSender exchangeSender_;
/**
* optional .tipb.ExchangeSender exchange_sender = 12;
*/
public boolean hasExchangeSender() {
return ((bitField0_ & 0x00000800) == 0x00000800);
}
/**
* optional .tipb.ExchangeSender exchange_sender = 12;
*/
public com.pingcap.tidb.tipb.ExchangeSender getExchangeSender() {
return exchangeSender_ == null ? com.pingcap.tidb.tipb.ExchangeSender.getDefaultInstance() : exchangeSender_;
}
/**
* optional .tipb.ExchangeSender exchange_sender = 12;
*/
public com.pingcap.tidb.tipb.ExchangeSenderOrBuilder getExchangeSenderOrBuilder() {
return exchangeSender_ == null ? com.pingcap.tidb.tipb.ExchangeSender.getDefaultInstance() : exchangeSender_;
}
private byte memoizedIsInitialized = -1;
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (((bitField0_ & 0x00000001) == 0x00000001)) {
output.writeEnum(1, tp_);
}
if (((bitField0_ & 0x00000002) == 0x00000002)) {
output.writeMessage(2, getTblScan());
}
if (((bitField0_ & 0x00000004) == 0x00000004)) {
output.writeMessage(3, getIdxScan());
}
if (((bitField0_ & 0x00000008) == 0x00000008)) {
output.writeMessage(4, getSelection());
}
if (((bitField0_ & 0x00000010) == 0x00000010)) {
output.writeMessage(5, getAggregation());
}
if (((bitField0_ & 0x00000020) == 0x00000020)) {
output.writeMessage(6, getTopN());
}
if (((bitField0_ & 0x00000040) == 0x00000040)) {
output.writeMessage(7, getLimit());
}
if (((bitField0_ & 0x00000080) == 0x00000080)) {
output.writeMessage(8, getExchangeReceiver());
}
if (((bitField0_ & 0x00000100) == 0x00000100)) {
output.writeMessage(9, getJoin());
}
if (((bitField0_ & 0x00000200) == 0x00000200)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 10, executorId_);
}
if (((bitField0_ & 0x00000400) == 0x00000400)) {
output.writeMessage(11, getKill());
}
if (((bitField0_ & 0x00000800) == 0x00000800)) {
output.writeMessage(12, getExchangeSender());
}
unknownFields.writeTo(output);
}
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (((bitField0_ & 0x00000001) == 0x00000001)) {
size += com.google.protobuf.CodedOutputStream
.computeEnumSize(1, tp_);
}
if (((bitField0_ & 0x00000002) == 0x00000002)) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(2, getTblScan());
}
if (((bitField0_ & 0x00000004) == 0x00000004)) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(3, getIdxScan());
}
if (((bitField0_ & 0x00000008) == 0x00000008)) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(4, getSelection());
}
if (((bitField0_ & 0x00000010) == 0x00000010)) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(5, getAggregation());
}
if (((bitField0_ & 0x00000020) == 0x00000020)) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(6, getTopN());
}
if (((bitField0_ & 0x00000040) == 0x00000040)) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(7, getLimit());
}
if (((bitField0_ & 0x00000080) == 0x00000080)) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(8, getExchangeReceiver());
}
if (((bitField0_ & 0x00000100) == 0x00000100)) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(9, getJoin());
}
if (((bitField0_ & 0x00000200) == 0x00000200)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(10, executorId_);
}
if (((bitField0_ & 0x00000400) == 0x00000400)) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(11, getKill());
}
if (((bitField0_ & 0x00000800) == 0x00000800)) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(12, getExchangeSender());
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof com.pingcap.tidb.tipb.Executor)) {
return super.equals(obj);
}
com.pingcap.tidb.tipb.Executor other = (com.pingcap.tidb.tipb.Executor) obj;
boolean result = true;
result = result && (hasTp() == other.hasTp());
if (hasTp()) {
result = result && tp_ == other.tp_;
}
result = result && (hasTblScan() == other.hasTblScan());
if (hasTblScan()) {
result = result && getTblScan()
.equals(other.getTblScan());
}
result = result && (hasIdxScan() == other.hasIdxScan());
if (hasIdxScan()) {
result = result && getIdxScan()
.equals(other.getIdxScan());
}
result = result && (hasSelection() == other.hasSelection());
if (hasSelection()) {
result = result && getSelection()
.equals(other.getSelection());
}
result = result && (hasAggregation() == other.hasAggregation());
if (hasAggregation()) {
result = result && getAggregation()
.equals(other.getAggregation());
}
result = result && (hasTopN() == other.hasTopN());
if (hasTopN()) {
result = result && getTopN()
.equals(other.getTopN());
}
result = result && (hasLimit() == other.hasLimit());
if (hasLimit()) {
result = result && getLimit()
.equals(other.getLimit());
}
result = result && (hasExchangeReceiver() == other.hasExchangeReceiver());
if (hasExchangeReceiver()) {
result = result && getExchangeReceiver()
.equals(other.getExchangeReceiver());
}
result = result && (hasJoin() == other.hasJoin());
if (hasJoin()) {
result = result && getJoin()
.equals(other.getJoin());
}
result = result && (hasExecutorId() == other.hasExecutorId());
if (hasExecutorId()) {
result = result && getExecutorId()
.equals(other.getExecutorId());
}
result = result && (hasKill() == other.hasKill());
if (hasKill()) {
result = result && getKill()
.equals(other.getKill());
}
result = result && (hasExchangeSender() == other.hasExchangeSender());
if (hasExchangeSender()) {
result = result && getExchangeSender()
.equals(other.getExchangeSender());
}
result = result && unknownFields.equals(other.unknownFields);
return result;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (hasTp()) {
hash = (37 * hash) + TP_FIELD_NUMBER;
hash = (53 * hash) + tp_;
}
if (hasTblScan()) {
hash = (37 * hash) + TBL_SCAN_FIELD_NUMBER;
hash = (53 * hash) + getTblScan().hashCode();
}
if (hasIdxScan()) {
hash = (37 * hash) + IDX_SCAN_FIELD_NUMBER;
hash = (53 * hash) + getIdxScan().hashCode();
}
if (hasSelection()) {
hash = (37 * hash) + SELECTION_FIELD_NUMBER;
hash = (53 * hash) + getSelection().hashCode();
}
if (hasAggregation()) {
hash = (37 * hash) + AGGREGATION_FIELD_NUMBER;
hash = (53 * hash) + getAggregation().hashCode();
}
if (hasTopN()) {
hash = (37 * hash) + TOPN_FIELD_NUMBER;
hash = (53 * hash) + getTopN().hashCode();
}
if (hasLimit()) {
hash = (37 * hash) + LIMIT_FIELD_NUMBER;
hash = (53 * hash) + getLimit().hashCode();
}
if (hasExchangeReceiver()) {
hash = (37 * hash) + EXCHANGE_RECEIVER_FIELD_NUMBER;
hash = (53 * hash) + getExchangeReceiver().hashCode();
}
if (hasJoin()) {
hash = (37 * hash) + JOIN_FIELD_NUMBER;
hash = (53 * hash) + getJoin().hashCode();
}
if (hasExecutorId()) {
hash = (37 * hash) + EXECUTOR_ID_FIELD_NUMBER;
hash = (53 * hash) + getExecutorId().hashCode();
}
if (hasKill()) {
hash = (37 * hash) + KILL_FIELD_NUMBER;
hash = (53 * hash) + getKill().hashCode();
}
if (hasExchangeSender()) {
hash = (37 * hash) + EXCHANGE_SENDER_FIELD_NUMBER;
hash = (53 * hash) + getExchangeSender().hashCode();
}
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static com.pingcap.tidb.tipb.Executor parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.pingcap.tidb.tipb.Executor parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.pingcap.tidb.tipb.Executor parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.pingcap.tidb.tipb.Executor parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.pingcap.tidb.tipb.Executor parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.pingcap.tidb.tipb.Executor parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.pingcap.tidb.tipb.Executor parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.pingcap.tidb.tipb.Executor parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static com.pingcap.tidb.tipb.Executor parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static com.pingcap.tidb.tipb.Executor parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static com.pingcap.tidb.tipb.Executor parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.pingcap.tidb.tipb.Executor parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(com.pingcap.tidb.tipb.Executor prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
* It represents a Executor.
*
*
* Protobuf type {@code tipb.Executor}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:tipb.Executor)
com.pingcap.tidb.tipb.ExecutorOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.pingcap.tidb.tipb.ExecutorOuterClass.internal_static_tipb_Executor_descriptor;
}
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.pingcap.tidb.tipb.ExecutorOuterClass.internal_static_tipb_Executor_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.pingcap.tidb.tipb.Executor.class, com.pingcap.tidb.tipb.Executor.Builder.class);
}
// Construct using com.pingcap.tidb.tipb.Executor.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
getTblScanFieldBuilder();
getIdxScanFieldBuilder();
getSelectionFieldBuilder();
getAggregationFieldBuilder();
getTopNFieldBuilder();
getLimitFieldBuilder();
getExchangeReceiverFieldBuilder();
getJoinFieldBuilder();
getKillFieldBuilder();
getExchangeSenderFieldBuilder();
}
}
public Builder clear() {
super.clear();
tp_ = 0;
bitField0_ = (bitField0_ & ~0x00000001);
if (tblScanBuilder_ == null) {
tblScan_ = null;
} else {
tblScanBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000002);
if (idxScanBuilder_ == null) {
idxScan_ = null;
} else {
idxScanBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000004);
if (selectionBuilder_ == null) {
selection_ = null;
} else {
selectionBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000008);
if (aggregationBuilder_ == null) {
aggregation_ = null;
} else {
aggregationBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000010);
if (topNBuilder_ == null) {
topN_ = null;
} else {
topNBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000020);
if (limitBuilder_ == null) {
limit_ = null;
} else {
limitBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000040);
if (exchangeReceiverBuilder_ == null) {
exchangeReceiver_ = null;
} else {
exchangeReceiverBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000080);
if (joinBuilder_ == null) {
join_ = null;
} else {
joinBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000100);
executorId_ = "";
bitField0_ = (bitField0_ & ~0x00000200);
if (killBuilder_ == null) {
kill_ = null;
} else {
killBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000400);
if (exchangeSenderBuilder_ == null) {
exchangeSender_ = null;
} else {
exchangeSenderBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000800);
return this;
}
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return com.pingcap.tidb.tipb.ExecutorOuterClass.internal_static_tipb_Executor_descriptor;
}
public com.pingcap.tidb.tipb.Executor getDefaultInstanceForType() {
return com.pingcap.tidb.tipb.Executor.getDefaultInstance();
}
public com.pingcap.tidb.tipb.Executor build() {
com.pingcap.tidb.tipb.Executor result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
public com.pingcap.tidb.tipb.Executor buildPartial() {
com.pingcap.tidb.tipb.Executor result = new com.pingcap.tidb.tipb.Executor(this);
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (((from_bitField0_ & 0x00000001) == 0x00000001)) {
to_bitField0_ |= 0x00000001;
}
result.tp_ = tp_;
if (((from_bitField0_ & 0x00000002) == 0x00000002)) {
to_bitField0_ |= 0x00000002;
}
if (tblScanBuilder_ == null) {
result.tblScan_ = tblScan_;
} else {
result.tblScan_ = tblScanBuilder_.build();
}
if (((from_bitField0_ & 0x00000004) == 0x00000004)) {
to_bitField0_ |= 0x00000004;
}
if (idxScanBuilder_ == null) {
result.idxScan_ = idxScan_;
} else {
result.idxScan_ = idxScanBuilder_.build();
}
if (((from_bitField0_ & 0x00000008) == 0x00000008)) {
to_bitField0_ |= 0x00000008;
}
if (selectionBuilder_ == null) {
result.selection_ = selection_;
} else {
result.selection_ = selectionBuilder_.build();
}
if (((from_bitField0_ & 0x00000010) == 0x00000010)) {
to_bitField0_ |= 0x00000010;
}
if (aggregationBuilder_ == null) {
result.aggregation_ = aggregation_;
} else {
result.aggregation_ = aggregationBuilder_.build();
}
if (((from_bitField0_ & 0x00000020) == 0x00000020)) {
to_bitField0_ |= 0x00000020;
}
if (topNBuilder_ == null) {
result.topN_ = topN_;
} else {
result.topN_ = topNBuilder_.build();
}
if (((from_bitField0_ & 0x00000040) == 0x00000040)) {
to_bitField0_ |= 0x00000040;
}
if (limitBuilder_ == null) {
result.limit_ = limit_;
} else {
result.limit_ = limitBuilder_.build();
}
if (((from_bitField0_ & 0x00000080) == 0x00000080)) {
to_bitField0_ |= 0x00000080;
}
if (exchangeReceiverBuilder_ == null) {
result.exchangeReceiver_ = exchangeReceiver_;
} else {
result.exchangeReceiver_ = exchangeReceiverBuilder_.build();
}
if (((from_bitField0_ & 0x00000100) == 0x00000100)) {
to_bitField0_ |= 0x00000100;
}
if (joinBuilder_ == null) {
result.join_ = join_;
} else {
result.join_ = joinBuilder_.build();
}
if (((from_bitField0_ & 0x00000200) == 0x00000200)) {
to_bitField0_ |= 0x00000200;
}
result.executorId_ = executorId_;
if (((from_bitField0_ & 0x00000400) == 0x00000400)) {
to_bitField0_ |= 0x00000400;
}
if (killBuilder_ == null) {
result.kill_ = kill_;
} else {
result.kill_ = killBuilder_.build();
}
if (((from_bitField0_ & 0x00000800) == 0x00000800)) {
to_bitField0_ |= 0x00000800;
}
if (exchangeSenderBuilder_ == null) {
result.exchangeSender_ = exchangeSender_;
} else {
result.exchangeSender_ = exchangeSenderBuilder_.build();
}
result.bitField0_ = to_bitField0_;
onBuilt();
return result;
}
public Builder clone() {
return (Builder) super.clone();
}
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.setField(field, value);
}
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return (Builder) super.clearField(field);
}
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return (Builder) super.clearOneof(oneof);
}
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return (Builder) super.setRepeatedField(field, index, value);
}
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.addRepeatedField(field, value);
}
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.pingcap.tidb.tipb.Executor) {
return mergeFrom((com.pingcap.tidb.tipb.Executor)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.pingcap.tidb.tipb.Executor other) {
if (other == com.pingcap.tidb.tipb.Executor.getDefaultInstance()) return this;
if (other.hasTp()) {
setTp(other.getTp());
}
if (other.hasTblScan()) {
mergeTblScan(other.getTblScan());
}
if (other.hasIdxScan()) {
mergeIdxScan(other.getIdxScan());
}
if (other.hasSelection()) {
mergeSelection(other.getSelection());
}
if (other.hasAggregation()) {
mergeAggregation(other.getAggregation());
}
if (other.hasTopN()) {
mergeTopN(other.getTopN());
}
if (other.hasLimit()) {
mergeLimit(other.getLimit());
}
if (other.hasExchangeReceiver()) {
mergeExchangeReceiver(other.getExchangeReceiver());
}
if (other.hasJoin()) {
mergeJoin(other.getJoin());
}
if (other.hasExecutorId()) {
bitField0_ |= 0x00000200;
executorId_ = other.executorId_;
onChanged();
}
if (other.hasKill()) {
mergeKill(other.getKill());
}
if (other.hasExchangeSender()) {
mergeExchangeSender(other.getExchangeSender());
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
public final boolean isInitialized() {
return true;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
com.pingcap.tidb.tipb.Executor parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (com.pingcap.tidb.tipb.Executor) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
private int tp_ = 0;
/**
* optional .tipb.ExecType tp = 1 [(.gogoproto.nullable) = false];
*/
public boolean hasTp() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
/**
* optional .tipb.ExecType tp = 1 [(.gogoproto.nullable) = false];
*/
public com.pingcap.tidb.tipb.ExecType getTp() {
com.pingcap.tidb.tipb.ExecType result = com.pingcap.tidb.tipb.ExecType.valueOf(tp_);
return result == null ? com.pingcap.tidb.tipb.ExecType.TypeTableScan : result;
}
/**
* optional .tipb.ExecType tp = 1 [(.gogoproto.nullable) = false];
*/
public Builder setTp(com.pingcap.tidb.tipb.ExecType value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000001;
tp_ = value.getNumber();
onChanged();
return this;
}
/**
* optional .tipb.ExecType tp = 1 [(.gogoproto.nullable) = false];
*/
public Builder clearTp() {
bitField0_ = (bitField0_ & ~0x00000001);
tp_ = 0;
onChanged();
return this;
}
private com.pingcap.tidb.tipb.TableScan tblScan_ = null;
private com.google.protobuf.SingleFieldBuilderV3<
com.pingcap.tidb.tipb.TableScan, com.pingcap.tidb.tipb.TableScan.Builder, com.pingcap.tidb.tipb.TableScanOrBuilder> tblScanBuilder_;
/**
* optional .tipb.TableScan tbl_scan = 2;
*/
public boolean hasTblScan() {
return ((bitField0_ & 0x00000002) == 0x00000002);
}
/**
* optional .tipb.TableScan tbl_scan = 2;
*/
public com.pingcap.tidb.tipb.TableScan getTblScan() {
if (tblScanBuilder_ == null) {
return tblScan_ == null ? com.pingcap.tidb.tipb.TableScan.getDefaultInstance() : tblScan_;
} else {
return tblScanBuilder_.getMessage();
}
}
/**
* optional .tipb.TableScan tbl_scan = 2;
*/
public Builder setTblScan(com.pingcap.tidb.tipb.TableScan value) {
if (tblScanBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
tblScan_ = value;
onChanged();
} else {
tblScanBuilder_.setMessage(value);
}
bitField0_ |= 0x00000002;
return this;
}
/**
* optional .tipb.TableScan tbl_scan = 2;
*/
public Builder setTblScan(
com.pingcap.tidb.tipb.TableScan.Builder builderForValue) {
if (tblScanBuilder_ == null) {
tblScan_ = builderForValue.build();
onChanged();
} else {
tblScanBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x00000002;
return this;
}
/**
* optional .tipb.TableScan tbl_scan = 2;
*/
public Builder mergeTblScan(com.pingcap.tidb.tipb.TableScan value) {
if (tblScanBuilder_ == null) {
if (((bitField0_ & 0x00000002) == 0x00000002) &&
tblScan_ != null &&
tblScan_ != com.pingcap.tidb.tipb.TableScan.getDefaultInstance()) {
tblScan_ =
com.pingcap.tidb.tipb.TableScan.newBuilder(tblScan_).mergeFrom(value).buildPartial();
} else {
tblScan_ = value;
}
onChanged();
} else {
tblScanBuilder_.mergeFrom(value);
}
bitField0_ |= 0x00000002;
return this;
}
/**
* optional .tipb.TableScan tbl_scan = 2;
*/
public Builder clearTblScan() {
if (tblScanBuilder_ == null) {
tblScan_ = null;
onChanged();
} else {
tblScanBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000002);
return this;
}
/**
* optional .tipb.TableScan tbl_scan = 2;
*/
public com.pingcap.tidb.tipb.TableScan.Builder getTblScanBuilder() {
bitField0_ |= 0x00000002;
onChanged();
return getTblScanFieldBuilder().getBuilder();
}
/**
* optional .tipb.TableScan tbl_scan = 2;
*/
public com.pingcap.tidb.tipb.TableScanOrBuilder getTblScanOrBuilder() {
if (tblScanBuilder_ != null) {
return tblScanBuilder_.getMessageOrBuilder();
} else {
return tblScan_ == null ?
com.pingcap.tidb.tipb.TableScan.getDefaultInstance() : tblScan_;
}
}
/**
* optional .tipb.TableScan tbl_scan = 2;
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.pingcap.tidb.tipb.TableScan, com.pingcap.tidb.tipb.TableScan.Builder, com.pingcap.tidb.tipb.TableScanOrBuilder>
getTblScanFieldBuilder() {
if (tblScanBuilder_ == null) {
tblScanBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
com.pingcap.tidb.tipb.TableScan, com.pingcap.tidb.tipb.TableScan.Builder, com.pingcap.tidb.tipb.TableScanOrBuilder>(
getTblScan(),
getParentForChildren(),
isClean());
tblScan_ = null;
}
return tblScanBuilder_;
}
private com.pingcap.tidb.tipb.IndexScan idxScan_ = null;
private com.google.protobuf.SingleFieldBuilderV3<
com.pingcap.tidb.tipb.IndexScan, com.pingcap.tidb.tipb.IndexScan.Builder, com.pingcap.tidb.tipb.IndexScanOrBuilder> idxScanBuilder_;
/**
* optional .tipb.IndexScan idx_scan = 3;
*/
public boolean hasIdxScan() {
return ((bitField0_ & 0x00000004) == 0x00000004);
}
/**
* optional .tipb.IndexScan idx_scan = 3;
*/
public com.pingcap.tidb.tipb.IndexScan getIdxScan() {
if (idxScanBuilder_ == null) {
return idxScan_ == null ? com.pingcap.tidb.tipb.IndexScan.getDefaultInstance() : idxScan_;
} else {
return idxScanBuilder_.getMessage();
}
}
/**
* optional .tipb.IndexScan idx_scan = 3;
*/
public Builder setIdxScan(com.pingcap.tidb.tipb.IndexScan value) {
if (idxScanBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
idxScan_ = value;
onChanged();
} else {
idxScanBuilder_.setMessage(value);
}
bitField0_ |= 0x00000004;
return this;
}
/**
* optional .tipb.IndexScan idx_scan = 3;
*/
public Builder setIdxScan(
com.pingcap.tidb.tipb.IndexScan.Builder builderForValue) {
if (idxScanBuilder_ == null) {
idxScan_ = builderForValue.build();
onChanged();
} else {
idxScanBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x00000004;
return this;
}
/**
* optional .tipb.IndexScan idx_scan = 3;
*/
public Builder mergeIdxScan(com.pingcap.tidb.tipb.IndexScan value) {
if (idxScanBuilder_ == null) {
if (((bitField0_ & 0x00000004) == 0x00000004) &&
idxScan_ != null &&
idxScan_ != com.pingcap.tidb.tipb.IndexScan.getDefaultInstance()) {
idxScan_ =
com.pingcap.tidb.tipb.IndexScan.newBuilder(idxScan_).mergeFrom(value).buildPartial();
} else {
idxScan_ = value;
}
onChanged();
} else {
idxScanBuilder_.mergeFrom(value);
}
bitField0_ |= 0x00000004;
return this;
}
/**
* optional .tipb.IndexScan idx_scan = 3;
*/
public Builder clearIdxScan() {
if (idxScanBuilder_ == null) {
idxScan_ = null;
onChanged();
} else {
idxScanBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000004);
return this;
}
/**
* optional .tipb.IndexScan idx_scan = 3;
*/
public com.pingcap.tidb.tipb.IndexScan.Builder getIdxScanBuilder() {
bitField0_ |= 0x00000004;
onChanged();
return getIdxScanFieldBuilder().getBuilder();
}
/**
* optional .tipb.IndexScan idx_scan = 3;
*/
public com.pingcap.tidb.tipb.IndexScanOrBuilder getIdxScanOrBuilder() {
if (idxScanBuilder_ != null) {
return idxScanBuilder_.getMessageOrBuilder();
} else {
return idxScan_ == null ?
com.pingcap.tidb.tipb.IndexScan.getDefaultInstance() : idxScan_;
}
}
/**
* optional .tipb.IndexScan idx_scan = 3;
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.pingcap.tidb.tipb.IndexScan, com.pingcap.tidb.tipb.IndexScan.Builder, com.pingcap.tidb.tipb.IndexScanOrBuilder>
getIdxScanFieldBuilder() {
if (idxScanBuilder_ == null) {
idxScanBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
com.pingcap.tidb.tipb.IndexScan, com.pingcap.tidb.tipb.IndexScan.Builder, com.pingcap.tidb.tipb.IndexScanOrBuilder>(
getIdxScan(),
getParentForChildren(),
isClean());
idxScan_ = null;
}
return idxScanBuilder_;
}
private com.pingcap.tidb.tipb.Selection selection_ = null;
private com.google.protobuf.SingleFieldBuilderV3<
com.pingcap.tidb.tipb.Selection, com.pingcap.tidb.tipb.Selection.Builder, com.pingcap.tidb.tipb.SelectionOrBuilder> selectionBuilder_;
/**
* optional .tipb.Selection selection = 4;
*/
public boolean hasSelection() {
return ((bitField0_ & 0x00000008) == 0x00000008);
}
/**
* optional .tipb.Selection selection = 4;
*/
public com.pingcap.tidb.tipb.Selection getSelection() {
if (selectionBuilder_ == null) {
return selection_ == null ? com.pingcap.tidb.tipb.Selection.getDefaultInstance() : selection_;
} else {
return selectionBuilder_.getMessage();
}
}
/**
* optional .tipb.Selection selection = 4;
*/
public Builder setSelection(com.pingcap.tidb.tipb.Selection value) {
if (selectionBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
selection_ = value;
onChanged();
} else {
selectionBuilder_.setMessage(value);
}
bitField0_ |= 0x00000008;
return this;
}
/**
* optional .tipb.Selection selection = 4;
*/
public Builder setSelection(
com.pingcap.tidb.tipb.Selection.Builder builderForValue) {
if (selectionBuilder_ == null) {
selection_ = builderForValue.build();
onChanged();
} else {
selectionBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x00000008;
return this;
}
/**
* optional .tipb.Selection selection = 4;
*/
public Builder mergeSelection(com.pingcap.tidb.tipb.Selection value) {
if (selectionBuilder_ == null) {
if (((bitField0_ & 0x00000008) == 0x00000008) &&
selection_ != null &&
selection_ != com.pingcap.tidb.tipb.Selection.getDefaultInstance()) {
selection_ =
com.pingcap.tidb.tipb.Selection.newBuilder(selection_).mergeFrom(value).buildPartial();
} else {
selection_ = value;
}
onChanged();
} else {
selectionBuilder_.mergeFrom(value);
}
bitField0_ |= 0x00000008;
return this;
}
/**
* optional .tipb.Selection selection = 4;
*/
public Builder clearSelection() {
if (selectionBuilder_ == null) {
selection_ = null;
onChanged();
} else {
selectionBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000008);
return this;
}
/**
* optional .tipb.Selection selection = 4;
*/
public com.pingcap.tidb.tipb.Selection.Builder getSelectionBuilder() {
bitField0_ |= 0x00000008;
onChanged();
return getSelectionFieldBuilder().getBuilder();
}
/**
* optional .tipb.Selection selection = 4;
*/
public com.pingcap.tidb.tipb.SelectionOrBuilder getSelectionOrBuilder() {
if (selectionBuilder_ != null) {
return selectionBuilder_.getMessageOrBuilder();
} else {
return selection_ == null ?
com.pingcap.tidb.tipb.Selection.getDefaultInstance() : selection_;
}
}
/**
* optional .tipb.Selection selection = 4;
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.pingcap.tidb.tipb.Selection, com.pingcap.tidb.tipb.Selection.Builder, com.pingcap.tidb.tipb.SelectionOrBuilder>
getSelectionFieldBuilder() {
if (selectionBuilder_ == null) {
selectionBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
com.pingcap.tidb.tipb.Selection, com.pingcap.tidb.tipb.Selection.Builder, com.pingcap.tidb.tipb.SelectionOrBuilder>(
getSelection(),
getParentForChildren(),
isClean());
selection_ = null;
}
return selectionBuilder_;
}
private com.pingcap.tidb.tipb.Aggregation aggregation_ = null;
private com.google.protobuf.SingleFieldBuilderV3<
com.pingcap.tidb.tipb.Aggregation, com.pingcap.tidb.tipb.Aggregation.Builder, com.pingcap.tidb.tipb.AggregationOrBuilder> aggregationBuilder_;
/**
* optional .tipb.Aggregation aggregation = 5;
*/
public boolean hasAggregation() {
return ((bitField0_ & 0x00000010) == 0x00000010);
}
/**
* optional .tipb.Aggregation aggregation = 5;
*/
public com.pingcap.tidb.tipb.Aggregation getAggregation() {
if (aggregationBuilder_ == null) {
return aggregation_ == null ? com.pingcap.tidb.tipb.Aggregation.getDefaultInstance() : aggregation_;
} else {
return aggregationBuilder_.getMessage();
}
}
/**
* optional .tipb.Aggregation aggregation = 5;
*/
public Builder setAggregation(com.pingcap.tidb.tipb.Aggregation value) {
if (aggregationBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
aggregation_ = value;
onChanged();
} else {
aggregationBuilder_.setMessage(value);
}
bitField0_ |= 0x00000010;
return this;
}
/**
* optional .tipb.Aggregation aggregation = 5;
*/
public Builder setAggregation(
com.pingcap.tidb.tipb.Aggregation.Builder builderForValue) {
if (aggregationBuilder_ == null) {
aggregation_ = builderForValue.build();
onChanged();
} else {
aggregationBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x00000010;
return this;
}
/**
* optional .tipb.Aggregation aggregation = 5;
*/
public Builder mergeAggregation(com.pingcap.tidb.tipb.Aggregation value) {
if (aggregationBuilder_ == null) {
if (((bitField0_ & 0x00000010) == 0x00000010) &&
aggregation_ != null &&
aggregation_ != com.pingcap.tidb.tipb.Aggregation.getDefaultInstance()) {
aggregation_ =
com.pingcap.tidb.tipb.Aggregation.newBuilder(aggregation_).mergeFrom(value).buildPartial();
} else {
aggregation_ = value;
}
onChanged();
} else {
aggregationBuilder_.mergeFrom(value);
}
bitField0_ |= 0x00000010;
return this;
}
/**
* optional .tipb.Aggregation aggregation = 5;
*/
public Builder clearAggregation() {
if (aggregationBuilder_ == null) {
aggregation_ = null;
onChanged();
} else {
aggregationBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000010);
return this;
}
/**
* optional .tipb.Aggregation aggregation = 5;
*/
public com.pingcap.tidb.tipb.Aggregation.Builder getAggregationBuilder() {
bitField0_ |= 0x00000010;
onChanged();
return getAggregationFieldBuilder().getBuilder();
}
/**
* optional .tipb.Aggregation aggregation = 5;
*/
public com.pingcap.tidb.tipb.AggregationOrBuilder getAggregationOrBuilder() {
if (aggregationBuilder_ != null) {
return aggregationBuilder_.getMessageOrBuilder();
} else {
return aggregation_ == null ?
com.pingcap.tidb.tipb.Aggregation.getDefaultInstance() : aggregation_;
}
}
/**
* optional .tipb.Aggregation aggregation = 5;
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.pingcap.tidb.tipb.Aggregation, com.pingcap.tidb.tipb.Aggregation.Builder, com.pingcap.tidb.tipb.AggregationOrBuilder>
getAggregationFieldBuilder() {
if (aggregationBuilder_ == null) {
aggregationBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
com.pingcap.tidb.tipb.Aggregation, com.pingcap.tidb.tipb.Aggregation.Builder, com.pingcap.tidb.tipb.AggregationOrBuilder>(
getAggregation(),
getParentForChildren(),
isClean());
aggregation_ = null;
}
return aggregationBuilder_;
}
private com.pingcap.tidb.tipb.TopN topN_ = null;
private com.google.protobuf.SingleFieldBuilderV3<
com.pingcap.tidb.tipb.TopN, com.pingcap.tidb.tipb.TopN.Builder, com.pingcap.tidb.tipb.TopNOrBuilder> topNBuilder_;
/**
* optional .tipb.TopN topN = 6;
*/
public boolean hasTopN() {
return ((bitField0_ & 0x00000020) == 0x00000020);
}
/**
* optional .tipb.TopN topN = 6;
*/
public com.pingcap.tidb.tipb.TopN getTopN() {
if (topNBuilder_ == null) {
return topN_ == null ? com.pingcap.tidb.tipb.TopN.getDefaultInstance() : topN_;
} else {
return topNBuilder_.getMessage();
}
}
/**
* optional .tipb.TopN topN = 6;
*/
public Builder setTopN(com.pingcap.tidb.tipb.TopN value) {
if (topNBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
topN_ = value;
onChanged();
} else {
topNBuilder_.setMessage(value);
}
bitField0_ |= 0x00000020;
return this;
}
/**
* optional .tipb.TopN topN = 6;
*/
public Builder setTopN(
com.pingcap.tidb.tipb.TopN.Builder builderForValue) {
if (topNBuilder_ == null) {
topN_ = builderForValue.build();
onChanged();
} else {
topNBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x00000020;
return this;
}
/**
* optional .tipb.TopN topN = 6;
*/
public Builder mergeTopN(com.pingcap.tidb.tipb.TopN value) {
if (topNBuilder_ == null) {
if (((bitField0_ & 0x00000020) == 0x00000020) &&
topN_ != null &&
topN_ != com.pingcap.tidb.tipb.TopN.getDefaultInstance()) {
topN_ =
com.pingcap.tidb.tipb.TopN.newBuilder(topN_).mergeFrom(value).buildPartial();
} else {
topN_ = value;
}
onChanged();
} else {
topNBuilder_.mergeFrom(value);
}
bitField0_ |= 0x00000020;
return this;
}
/**
* optional .tipb.TopN topN = 6;
*/
public Builder clearTopN() {
if (topNBuilder_ == null) {
topN_ = null;
onChanged();
} else {
topNBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000020);
return this;
}
/**
* optional .tipb.TopN topN = 6;
*/
public com.pingcap.tidb.tipb.TopN.Builder getTopNBuilder() {
bitField0_ |= 0x00000020;
onChanged();
return getTopNFieldBuilder().getBuilder();
}
/**
* optional .tipb.TopN topN = 6;
*/
public com.pingcap.tidb.tipb.TopNOrBuilder getTopNOrBuilder() {
if (topNBuilder_ != null) {
return topNBuilder_.getMessageOrBuilder();
} else {
return topN_ == null ?
com.pingcap.tidb.tipb.TopN.getDefaultInstance() : topN_;
}
}
/**
* optional .tipb.TopN topN = 6;
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.pingcap.tidb.tipb.TopN, com.pingcap.tidb.tipb.TopN.Builder, com.pingcap.tidb.tipb.TopNOrBuilder>
getTopNFieldBuilder() {
if (topNBuilder_ == null) {
topNBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
com.pingcap.tidb.tipb.TopN, com.pingcap.tidb.tipb.TopN.Builder, com.pingcap.tidb.tipb.TopNOrBuilder>(
getTopN(),
getParentForChildren(),
isClean());
topN_ = null;
}
return topNBuilder_;
}
private com.pingcap.tidb.tipb.Limit limit_ = null;
private com.google.protobuf.SingleFieldBuilderV3<
com.pingcap.tidb.tipb.Limit, com.pingcap.tidb.tipb.Limit.Builder, com.pingcap.tidb.tipb.LimitOrBuilder> limitBuilder_;
/**
* optional .tipb.Limit limit = 7;
*/
public boolean hasLimit() {
return ((bitField0_ & 0x00000040) == 0x00000040);
}
/**
* optional .tipb.Limit limit = 7;
*/
public com.pingcap.tidb.tipb.Limit getLimit() {
if (limitBuilder_ == null) {
return limit_ == null ? com.pingcap.tidb.tipb.Limit.getDefaultInstance() : limit_;
} else {
return limitBuilder_.getMessage();
}
}
/**
* optional .tipb.Limit limit = 7;
*/
public Builder setLimit(com.pingcap.tidb.tipb.Limit value) {
if (limitBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
limit_ = value;
onChanged();
} else {
limitBuilder_.setMessage(value);
}
bitField0_ |= 0x00000040;
return this;
}
/**
* optional .tipb.Limit limit = 7;
*/
public Builder setLimit(
com.pingcap.tidb.tipb.Limit.Builder builderForValue) {
if (limitBuilder_ == null) {
limit_ = builderForValue.build();
onChanged();
} else {
limitBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x00000040;
return this;
}
/**
* optional .tipb.Limit limit = 7;
*/
public Builder mergeLimit(com.pingcap.tidb.tipb.Limit value) {
if (limitBuilder_ == null) {
if (((bitField0_ & 0x00000040) == 0x00000040) &&
limit_ != null &&
limit_ != com.pingcap.tidb.tipb.Limit.getDefaultInstance()) {
limit_ =
com.pingcap.tidb.tipb.Limit.newBuilder(limit_).mergeFrom(value).buildPartial();
} else {
limit_ = value;
}
onChanged();
} else {
limitBuilder_.mergeFrom(value);
}
bitField0_ |= 0x00000040;
return this;
}
/**
* optional .tipb.Limit limit = 7;
*/
public Builder clearLimit() {
if (limitBuilder_ == null) {
limit_ = null;
onChanged();
} else {
limitBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000040);
return this;
}
/**
* optional .tipb.Limit limit = 7;
*/
public com.pingcap.tidb.tipb.Limit.Builder getLimitBuilder() {
bitField0_ |= 0x00000040;
onChanged();
return getLimitFieldBuilder().getBuilder();
}
/**
* optional .tipb.Limit limit = 7;
*/
public com.pingcap.tidb.tipb.LimitOrBuilder getLimitOrBuilder() {
if (limitBuilder_ != null) {
return limitBuilder_.getMessageOrBuilder();
} else {
return limit_ == null ?
com.pingcap.tidb.tipb.Limit.getDefaultInstance() : limit_;
}
}
/**
* optional .tipb.Limit limit = 7;
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.pingcap.tidb.tipb.Limit, com.pingcap.tidb.tipb.Limit.Builder, com.pingcap.tidb.tipb.LimitOrBuilder>
getLimitFieldBuilder() {
if (limitBuilder_ == null) {
limitBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
com.pingcap.tidb.tipb.Limit, com.pingcap.tidb.tipb.Limit.Builder, com.pingcap.tidb.tipb.LimitOrBuilder>(
getLimit(),
getParentForChildren(),
isClean());
limit_ = null;
}
return limitBuilder_;
}
private com.pingcap.tidb.tipb.ExchangeReceiver exchangeReceiver_ = null;
private com.google.protobuf.SingleFieldBuilderV3<
com.pingcap.tidb.tipb.ExchangeReceiver, com.pingcap.tidb.tipb.ExchangeReceiver.Builder, com.pingcap.tidb.tipb.ExchangeReceiverOrBuilder> exchangeReceiverBuilder_;
/**
* optional .tipb.ExchangeReceiver exchange_receiver = 8;
*/
public boolean hasExchangeReceiver() {
return ((bitField0_ & 0x00000080) == 0x00000080);
}
/**
* optional .tipb.ExchangeReceiver exchange_receiver = 8;
*/
public com.pingcap.tidb.tipb.ExchangeReceiver getExchangeReceiver() {
if (exchangeReceiverBuilder_ == null) {
return exchangeReceiver_ == null ? com.pingcap.tidb.tipb.ExchangeReceiver.getDefaultInstance() : exchangeReceiver_;
} else {
return exchangeReceiverBuilder_.getMessage();
}
}
/**
* optional .tipb.ExchangeReceiver exchange_receiver = 8;
*/
public Builder setExchangeReceiver(com.pingcap.tidb.tipb.ExchangeReceiver value) {
if (exchangeReceiverBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
exchangeReceiver_ = value;
onChanged();
} else {
exchangeReceiverBuilder_.setMessage(value);
}
bitField0_ |= 0x00000080;
return this;
}
/**
* optional .tipb.ExchangeReceiver exchange_receiver = 8;
*/
public Builder setExchangeReceiver(
com.pingcap.tidb.tipb.ExchangeReceiver.Builder builderForValue) {
if (exchangeReceiverBuilder_ == null) {
exchangeReceiver_ = builderForValue.build();
onChanged();
} else {
exchangeReceiverBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x00000080;
return this;
}
/**
* optional .tipb.ExchangeReceiver exchange_receiver = 8;
*/
public Builder mergeExchangeReceiver(com.pingcap.tidb.tipb.ExchangeReceiver value) {
if (exchangeReceiverBuilder_ == null) {
if (((bitField0_ & 0x00000080) == 0x00000080) &&
exchangeReceiver_ != null &&
exchangeReceiver_ != com.pingcap.tidb.tipb.ExchangeReceiver.getDefaultInstance()) {
exchangeReceiver_ =
com.pingcap.tidb.tipb.ExchangeReceiver.newBuilder(exchangeReceiver_).mergeFrom(value).buildPartial();
} else {
exchangeReceiver_ = value;
}
onChanged();
} else {
exchangeReceiverBuilder_.mergeFrom(value);
}
bitField0_ |= 0x00000080;
return this;
}
/**
* optional .tipb.ExchangeReceiver exchange_receiver = 8;
*/
public Builder clearExchangeReceiver() {
if (exchangeReceiverBuilder_ == null) {
exchangeReceiver_ = null;
onChanged();
} else {
exchangeReceiverBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000080);
return this;
}
/**
* optional .tipb.ExchangeReceiver exchange_receiver = 8;
*/
public com.pingcap.tidb.tipb.ExchangeReceiver.Builder getExchangeReceiverBuilder() {
bitField0_ |= 0x00000080;
onChanged();
return getExchangeReceiverFieldBuilder().getBuilder();
}
/**
* optional .tipb.ExchangeReceiver exchange_receiver = 8;
*/
public com.pingcap.tidb.tipb.ExchangeReceiverOrBuilder getExchangeReceiverOrBuilder() {
if (exchangeReceiverBuilder_ != null) {
return exchangeReceiverBuilder_.getMessageOrBuilder();
} else {
return exchangeReceiver_ == null ?
com.pingcap.tidb.tipb.ExchangeReceiver.getDefaultInstance() : exchangeReceiver_;
}
}
/**
* optional .tipb.ExchangeReceiver exchange_receiver = 8;
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.pingcap.tidb.tipb.ExchangeReceiver, com.pingcap.tidb.tipb.ExchangeReceiver.Builder, com.pingcap.tidb.tipb.ExchangeReceiverOrBuilder>
getExchangeReceiverFieldBuilder() {
if (exchangeReceiverBuilder_ == null) {
exchangeReceiverBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
com.pingcap.tidb.tipb.ExchangeReceiver, com.pingcap.tidb.tipb.ExchangeReceiver.Builder, com.pingcap.tidb.tipb.ExchangeReceiverOrBuilder>(
getExchangeReceiver(),
getParentForChildren(),
isClean());
exchangeReceiver_ = null;
}
return exchangeReceiverBuilder_;
}
private com.pingcap.tidb.tipb.Join join_ = null;
private com.google.protobuf.SingleFieldBuilderV3<
com.pingcap.tidb.tipb.Join, com.pingcap.tidb.tipb.Join.Builder, com.pingcap.tidb.tipb.JoinOrBuilder> joinBuilder_;
/**
* optional .tipb.Join join = 9;
*/
public boolean hasJoin() {
return ((bitField0_ & 0x00000100) == 0x00000100);
}
/**
* optional .tipb.Join join = 9;
*/
public com.pingcap.tidb.tipb.Join getJoin() {
if (joinBuilder_ == null) {
return join_ == null ? com.pingcap.tidb.tipb.Join.getDefaultInstance() : join_;
} else {
return joinBuilder_.getMessage();
}
}
/**
* optional .tipb.Join join = 9;
*/
public Builder setJoin(com.pingcap.tidb.tipb.Join value) {
if (joinBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
join_ = value;
onChanged();
} else {
joinBuilder_.setMessage(value);
}
bitField0_ |= 0x00000100;
return this;
}
/**
* optional .tipb.Join join = 9;
*/
public Builder setJoin(
com.pingcap.tidb.tipb.Join.Builder builderForValue) {
if (joinBuilder_ == null) {
join_ = builderForValue.build();
onChanged();
} else {
joinBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x00000100;
return this;
}
/**
* optional .tipb.Join join = 9;
*/
public Builder mergeJoin(com.pingcap.tidb.tipb.Join value) {
if (joinBuilder_ == null) {
if (((bitField0_ & 0x00000100) == 0x00000100) &&
join_ != null &&
join_ != com.pingcap.tidb.tipb.Join.getDefaultInstance()) {
join_ =
com.pingcap.tidb.tipb.Join.newBuilder(join_).mergeFrom(value).buildPartial();
} else {
join_ = value;
}
onChanged();
} else {
joinBuilder_.mergeFrom(value);
}
bitField0_ |= 0x00000100;
return this;
}
/**
* optional .tipb.Join join = 9;
*/
public Builder clearJoin() {
if (joinBuilder_ == null) {
join_ = null;
onChanged();
} else {
joinBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000100);
return this;
}
/**
* optional .tipb.Join join = 9;
*/
public com.pingcap.tidb.tipb.Join.Builder getJoinBuilder() {
bitField0_ |= 0x00000100;
onChanged();
return getJoinFieldBuilder().getBuilder();
}
/**
* optional .tipb.Join join = 9;
*/
public com.pingcap.tidb.tipb.JoinOrBuilder getJoinOrBuilder() {
if (joinBuilder_ != null) {
return joinBuilder_.getMessageOrBuilder();
} else {
return join_ == null ?
com.pingcap.tidb.tipb.Join.getDefaultInstance() : join_;
}
}
/**
* optional .tipb.Join join = 9;
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.pingcap.tidb.tipb.Join, com.pingcap.tidb.tipb.Join.Builder, com.pingcap.tidb.tipb.JoinOrBuilder>
getJoinFieldBuilder() {
if (joinBuilder_ == null) {
joinBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
com.pingcap.tidb.tipb.Join, com.pingcap.tidb.tipb.Join.Builder, com.pingcap.tidb.tipb.JoinOrBuilder>(
getJoin(),
getParentForChildren(),
isClean());
join_ = null;
}
return joinBuilder_;
}
private java.lang.Object executorId_ = "";
/**
* optional string executor_id = 10;
*/
public boolean hasExecutorId() {
return ((bitField0_ & 0x00000200) == 0x00000200);
}
/**
* optional string executor_id = 10;
*/
public java.lang.String getExecutorId() {
java.lang.Object ref = executorId_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
executorId_ = s;
}
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* optional string executor_id = 10;
*/
public com.google.protobuf.ByteString
getExecutorIdBytes() {
java.lang.Object ref = executorId_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
executorId_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* optional string executor_id = 10;
*/
public Builder setExecutorId(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000200;
executorId_ = value;
onChanged();
return this;
}
/**
* optional string executor_id = 10;
*/
public Builder clearExecutorId() {
bitField0_ = (bitField0_ & ~0x00000200);
executorId_ = getDefaultInstance().getExecutorId();
onChanged();
return this;
}
/**
* optional string executor_id = 10;
*/
public Builder setExecutorIdBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000200;
executorId_ = value;
onChanged();
return this;
}
private com.pingcap.tidb.tipb.Kill kill_ = null;
private com.google.protobuf.SingleFieldBuilderV3<
com.pingcap.tidb.tipb.Kill, com.pingcap.tidb.tipb.Kill.Builder, com.pingcap.tidb.tipb.KillOrBuilder> killBuilder_;
/**
* optional .tipb.Kill kill = 11;
*/
public boolean hasKill() {
return ((bitField0_ & 0x00000400) == 0x00000400);
}
/**
* optional .tipb.Kill kill = 11;
*/
public com.pingcap.tidb.tipb.Kill getKill() {
if (killBuilder_ == null) {
return kill_ == null ? com.pingcap.tidb.tipb.Kill.getDefaultInstance() : kill_;
} else {
return killBuilder_.getMessage();
}
}
/**
* optional .tipb.Kill kill = 11;
*/
public Builder setKill(com.pingcap.tidb.tipb.Kill value) {
if (killBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
kill_ = value;
onChanged();
} else {
killBuilder_.setMessage(value);
}
bitField0_ |= 0x00000400;
return this;
}
/**
* optional .tipb.Kill kill = 11;
*/
public Builder setKill(
com.pingcap.tidb.tipb.Kill.Builder builderForValue) {
if (killBuilder_ == null) {
kill_ = builderForValue.build();
onChanged();
} else {
killBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x00000400;
return this;
}
/**
* optional .tipb.Kill kill = 11;
*/
public Builder mergeKill(com.pingcap.tidb.tipb.Kill value) {
if (killBuilder_ == null) {
if (((bitField0_ & 0x00000400) == 0x00000400) &&
kill_ != null &&
kill_ != com.pingcap.tidb.tipb.Kill.getDefaultInstance()) {
kill_ =
com.pingcap.tidb.tipb.Kill.newBuilder(kill_).mergeFrom(value).buildPartial();
} else {
kill_ = value;
}
onChanged();
} else {
killBuilder_.mergeFrom(value);
}
bitField0_ |= 0x00000400;
return this;
}
/**
* optional .tipb.Kill kill = 11;
*/
public Builder clearKill() {
if (killBuilder_ == null) {
kill_ = null;
onChanged();
} else {
killBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000400);
return this;
}
/**
* optional .tipb.Kill kill = 11;
*/
public com.pingcap.tidb.tipb.Kill.Builder getKillBuilder() {
bitField0_ |= 0x00000400;
onChanged();
return getKillFieldBuilder().getBuilder();
}
/**
* optional .tipb.Kill kill = 11;
*/
public com.pingcap.tidb.tipb.KillOrBuilder getKillOrBuilder() {
if (killBuilder_ != null) {
return killBuilder_.getMessageOrBuilder();
} else {
return kill_ == null ?
com.pingcap.tidb.tipb.Kill.getDefaultInstance() : kill_;
}
}
/**
* optional .tipb.Kill kill = 11;
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.pingcap.tidb.tipb.Kill, com.pingcap.tidb.tipb.Kill.Builder, com.pingcap.tidb.tipb.KillOrBuilder>
getKillFieldBuilder() {
if (killBuilder_ == null) {
killBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
com.pingcap.tidb.tipb.Kill, com.pingcap.tidb.tipb.Kill.Builder, com.pingcap.tidb.tipb.KillOrBuilder>(
getKill(),
getParentForChildren(),
isClean());
kill_ = null;
}
return killBuilder_;
}
private com.pingcap.tidb.tipb.ExchangeSender exchangeSender_ = null;
private com.google.protobuf.SingleFieldBuilderV3<
com.pingcap.tidb.tipb.ExchangeSender, com.pingcap.tidb.tipb.ExchangeSender.Builder, com.pingcap.tidb.tipb.ExchangeSenderOrBuilder> exchangeSenderBuilder_;
/**
* optional .tipb.ExchangeSender exchange_sender = 12;
*/
public boolean hasExchangeSender() {
return ((bitField0_ & 0x00000800) == 0x00000800);
}
/**
* optional .tipb.ExchangeSender exchange_sender = 12;
*/
public com.pingcap.tidb.tipb.ExchangeSender getExchangeSender() {
if (exchangeSenderBuilder_ == null) {
return exchangeSender_ == null ? com.pingcap.tidb.tipb.ExchangeSender.getDefaultInstance() : exchangeSender_;
} else {
return exchangeSenderBuilder_.getMessage();
}
}
/**
* optional .tipb.ExchangeSender exchange_sender = 12;
*/
public Builder setExchangeSender(com.pingcap.tidb.tipb.ExchangeSender value) {
if (exchangeSenderBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
exchangeSender_ = value;
onChanged();
} else {
exchangeSenderBuilder_.setMessage(value);
}
bitField0_ |= 0x00000800;
return this;
}
/**
* optional .tipb.ExchangeSender exchange_sender = 12;
*/
public Builder setExchangeSender(
com.pingcap.tidb.tipb.ExchangeSender.Builder builderForValue) {
if (exchangeSenderBuilder_ == null) {
exchangeSender_ = builderForValue.build();
onChanged();
} else {
exchangeSenderBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x00000800;
return this;
}
/**
* optional .tipb.ExchangeSender exchange_sender = 12;
*/
public Builder mergeExchangeSender(com.pingcap.tidb.tipb.ExchangeSender value) {
if (exchangeSenderBuilder_ == null) {
if (((bitField0_ & 0x00000800) == 0x00000800) &&
exchangeSender_ != null &&
exchangeSender_ != com.pingcap.tidb.tipb.ExchangeSender.getDefaultInstance()) {
exchangeSender_ =
com.pingcap.tidb.tipb.ExchangeSender.newBuilder(exchangeSender_).mergeFrom(value).buildPartial();
} else {
exchangeSender_ = value;
}
onChanged();
} else {
exchangeSenderBuilder_.mergeFrom(value);
}
bitField0_ |= 0x00000800;
return this;
}
/**
* optional .tipb.ExchangeSender exchange_sender = 12;
*/
public Builder clearExchangeSender() {
if (exchangeSenderBuilder_ == null) {
exchangeSender_ = null;
onChanged();
} else {
exchangeSenderBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000800);
return this;
}
/**
* optional .tipb.ExchangeSender exchange_sender = 12;
*/
public com.pingcap.tidb.tipb.ExchangeSender.Builder getExchangeSenderBuilder() {
bitField0_ |= 0x00000800;
onChanged();
return getExchangeSenderFieldBuilder().getBuilder();
}
/**
* optional .tipb.ExchangeSender exchange_sender = 12;
*/
public com.pingcap.tidb.tipb.ExchangeSenderOrBuilder getExchangeSenderOrBuilder() {
if (exchangeSenderBuilder_ != null) {
return exchangeSenderBuilder_.getMessageOrBuilder();
} else {
return exchangeSender_ == null ?
com.pingcap.tidb.tipb.ExchangeSender.getDefaultInstance() : exchangeSender_;
}
}
/**
* optional .tipb.ExchangeSender exchange_sender = 12;
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.pingcap.tidb.tipb.ExchangeSender, com.pingcap.tidb.tipb.ExchangeSender.Builder, com.pingcap.tidb.tipb.ExchangeSenderOrBuilder>
getExchangeSenderFieldBuilder() {
if (exchangeSenderBuilder_ == null) {
exchangeSenderBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
com.pingcap.tidb.tipb.ExchangeSender, com.pingcap.tidb.tipb.ExchangeSender.Builder, com.pingcap.tidb.tipb.ExchangeSenderOrBuilder>(
getExchangeSender(),
getParentForChildren(),
isClean());
exchangeSender_ = null;
}
return exchangeSenderBuilder_;
}
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:tipb.Executor)
}
// @@protoc_insertion_point(class_scope:tipb.Executor)
private static final com.pingcap.tidb.tipb.Executor DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new com.pingcap.tidb.tipb.Executor();
}
public static com.pingcap.tidb.tipb.Executor getDefaultInstance() {
return DEFAULT_INSTANCE;
}
@java.lang.Deprecated public static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
public Executor parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new Executor(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
public com.pingcap.tidb.tipb.Executor getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy