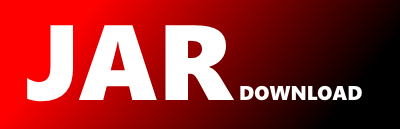
com.pingcap.tidb.tipb.TopN Maven / Gradle / Ivy
// Generated by the protocol buffer compiler. DO NOT EDIT!
// source: executor.proto
package com.pingcap.tidb.tipb;
/**
* Protobuf type {@code tipb.TopN}
*/
public final class TopN extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:tipb.TopN)
TopNOrBuilder {
private static final long serialVersionUID = 0L;
// Use TopN.newBuilder() to construct.
private TopN(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private TopN() {
orderBy_ = java.util.Collections.emptyList();
limit_ = 0L;
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private TopN(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
default: {
if (!parseUnknownField(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
case 10: {
if (!((mutable_bitField0_ & 0x00000001) == 0x00000001)) {
orderBy_ = new java.util.ArrayList();
mutable_bitField0_ |= 0x00000001;
}
orderBy_.add(
input.readMessage(com.pingcap.tidb.tipb.ByItem.PARSER, extensionRegistry));
break;
}
case 16: {
bitField0_ |= 0x00000001;
limit_ = input.readUInt64();
break;
}
case 26: {
com.pingcap.tidb.tipb.Executor.Builder subBuilder = null;
if (((bitField0_ & 0x00000002) == 0x00000002)) {
subBuilder = child_.toBuilder();
}
child_ = input.readMessage(com.pingcap.tidb.tipb.Executor.PARSER, extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(child_);
child_ = subBuilder.buildPartial();
}
bitField0_ |= 0x00000002;
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
if (((mutable_bitField0_ & 0x00000001) == 0x00000001)) {
orderBy_ = java.util.Collections.unmodifiableList(orderBy_);
}
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.pingcap.tidb.tipb.ExecutorOuterClass.internal_static_tipb_TopN_descriptor;
}
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.pingcap.tidb.tipb.ExecutorOuterClass.internal_static_tipb_TopN_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.pingcap.tidb.tipb.TopN.class, com.pingcap.tidb.tipb.TopN.Builder.class);
}
private int bitField0_;
public static final int ORDER_BY_FIELD_NUMBER = 1;
private java.util.List orderBy_;
/**
*
* Order by clause.
*
*
* repeated .tipb.ByItem order_by = 1;
*/
public java.util.List getOrderByList() {
return orderBy_;
}
/**
*
* Order by clause.
*
*
* repeated .tipb.ByItem order_by = 1;
*/
public java.util.List extends com.pingcap.tidb.tipb.ByItemOrBuilder>
getOrderByOrBuilderList() {
return orderBy_;
}
/**
*
* Order by clause.
*
*
* repeated .tipb.ByItem order_by = 1;
*/
public int getOrderByCount() {
return orderBy_.size();
}
/**
*
* Order by clause.
*
*
* repeated .tipb.ByItem order_by = 1;
*/
public com.pingcap.tidb.tipb.ByItem getOrderBy(int index) {
return orderBy_.get(index);
}
/**
*
* Order by clause.
*
*
* repeated .tipb.ByItem order_by = 1;
*/
public com.pingcap.tidb.tipb.ByItemOrBuilder getOrderByOrBuilder(
int index) {
return orderBy_.get(index);
}
public static final int LIMIT_FIELD_NUMBER = 2;
private long limit_;
/**
* optional uint64 limit = 2 [(.gogoproto.nullable) = false];
*/
public boolean hasLimit() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
/**
* optional uint64 limit = 2 [(.gogoproto.nullable) = false];
*/
public long getLimit() {
return limit_;
}
public static final int CHILD_FIELD_NUMBER = 3;
private com.pingcap.tidb.tipb.Executor child_;
/**
* optional .tipb.Executor child = 3;
*/
public boolean hasChild() {
return ((bitField0_ & 0x00000002) == 0x00000002);
}
/**
* optional .tipb.Executor child = 3;
*/
public com.pingcap.tidb.tipb.Executor getChild() {
return child_ == null ? com.pingcap.tidb.tipb.Executor.getDefaultInstance() : child_;
}
/**
* optional .tipb.Executor child = 3;
*/
public com.pingcap.tidb.tipb.ExecutorOrBuilder getChildOrBuilder() {
return child_ == null ? com.pingcap.tidb.tipb.Executor.getDefaultInstance() : child_;
}
private byte memoizedIsInitialized = -1;
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
for (int i = 0; i < orderBy_.size(); i++) {
output.writeMessage(1, orderBy_.get(i));
}
if (((bitField0_ & 0x00000001) == 0x00000001)) {
output.writeUInt64(2, limit_);
}
if (((bitField0_ & 0x00000002) == 0x00000002)) {
output.writeMessage(3, getChild());
}
unknownFields.writeTo(output);
}
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
for (int i = 0; i < orderBy_.size(); i++) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(1, orderBy_.get(i));
}
if (((bitField0_ & 0x00000001) == 0x00000001)) {
size += com.google.protobuf.CodedOutputStream
.computeUInt64Size(2, limit_);
}
if (((bitField0_ & 0x00000002) == 0x00000002)) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(3, getChild());
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof com.pingcap.tidb.tipb.TopN)) {
return super.equals(obj);
}
com.pingcap.tidb.tipb.TopN other = (com.pingcap.tidb.tipb.TopN) obj;
boolean result = true;
result = result && getOrderByList()
.equals(other.getOrderByList());
result = result && (hasLimit() == other.hasLimit());
if (hasLimit()) {
result = result && (getLimit()
== other.getLimit());
}
result = result && (hasChild() == other.hasChild());
if (hasChild()) {
result = result && getChild()
.equals(other.getChild());
}
result = result && unknownFields.equals(other.unknownFields);
return result;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (getOrderByCount() > 0) {
hash = (37 * hash) + ORDER_BY_FIELD_NUMBER;
hash = (53 * hash) + getOrderByList().hashCode();
}
if (hasLimit()) {
hash = (37 * hash) + LIMIT_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
getLimit());
}
if (hasChild()) {
hash = (37 * hash) + CHILD_FIELD_NUMBER;
hash = (53 * hash) + getChild().hashCode();
}
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static com.pingcap.tidb.tipb.TopN parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.pingcap.tidb.tipb.TopN parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.pingcap.tidb.tipb.TopN parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.pingcap.tidb.tipb.TopN parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.pingcap.tidb.tipb.TopN parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.pingcap.tidb.tipb.TopN parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.pingcap.tidb.tipb.TopN parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.pingcap.tidb.tipb.TopN parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static com.pingcap.tidb.tipb.TopN parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static com.pingcap.tidb.tipb.TopN parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static com.pingcap.tidb.tipb.TopN parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.pingcap.tidb.tipb.TopN parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(com.pingcap.tidb.tipb.TopN prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code tipb.TopN}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:tipb.TopN)
com.pingcap.tidb.tipb.TopNOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.pingcap.tidb.tipb.ExecutorOuterClass.internal_static_tipb_TopN_descriptor;
}
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.pingcap.tidb.tipb.ExecutorOuterClass.internal_static_tipb_TopN_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.pingcap.tidb.tipb.TopN.class, com.pingcap.tidb.tipb.TopN.Builder.class);
}
// Construct using com.pingcap.tidb.tipb.TopN.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
getOrderByFieldBuilder();
getChildFieldBuilder();
}
}
public Builder clear() {
super.clear();
if (orderByBuilder_ == null) {
orderBy_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000001);
} else {
orderByBuilder_.clear();
}
limit_ = 0L;
bitField0_ = (bitField0_ & ~0x00000002);
if (childBuilder_ == null) {
child_ = null;
} else {
childBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000004);
return this;
}
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return com.pingcap.tidb.tipb.ExecutorOuterClass.internal_static_tipb_TopN_descriptor;
}
public com.pingcap.tidb.tipb.TopN getDefaultInstanceForType() {
return com.pingcap.tidb.tipb.TopN.getDefaultInstance();
}
public com.pingcap.tidb.tipb.TopN build() {
com.pingcap.tidb.tipb.TopN result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
public com.pingcap.tidb.tipb.TopN buildPartial() {
com.pingcap.tidb.tipb.TopN result = new com.pingcap.tidb.tipb.TopN(this);
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (orderByBuilder_ == null) {
if (((bitField0_ & 0x00000001) == 0x00000001)) {
orderBy_ = java.util.Collections.unmodifiableList(orderBy_);
bitField0_ = (bitField0_ & ~0x00000001);
}
result.orderBy_ = orderBy_;
} else {
result.orderBy_ = orderByBuilder_.build();
}
if (((from_bitField0_ & 0x00000002) == 0x00000002)) {
to_bitField0_ |= 0x00000001;
}
result.limit_ = limit_;
if (((from_bitField0_ & 0x00000004) == 0x00000004)) {
to_bitField0_ |= 0x00000002;
}
if (childBuilder_ == null) {
result.child_ = child_;
} else {
result.child_ = childBuilder_.build();
}
result.bitField0_ = to_bitField0_;
onBuilt();
return result;
}
public Builder clone() {
return (Builder) super.clone();
}
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.setField(field, value);
}
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return (Builder) super.clearField(field);
}
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return (Builder) super.clearOneof(oneof);
}
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return (Builder) super.setRepeatedField(field, index, value);
}
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.addRepeatedField(field, value);
}
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.pingcap.tidb.tipb.TopN) {
return mergeFrom((com.pingcap.tidb.tipb.TopN)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.pingcap.tidb.tipb.TopN other) {
if (other == com.pingcap.tidb.tipb.TopN.getDefaultInstance()) return this;
if (orderByBuilder_ == null) {
if (!other.orderBy_.isEmpty()) {
if (orderBy_.isEmpty()) {
orderBy_ = other.orderBy_;
bitField0_ = (bitField0_ & ~0x00000001);
} else {
ensureOrderByIsMutable();
orderBy_.addAll(other.orderBy_);
}
onChanged();
}
} else {
if (!other.orderBy_.isEmpty()) {
if (orderByBuilder_.isEmpty()) {
orderByBuilder_.dispose();
orderByBuilder_ = null;
orderBy_ = other.orderBy_;
bitField0_ = (bitField0_ & ~0x00000001);
orderByBuilder_ =
com.google.protobuf.GeneratedMessageV3.alwaysUseFieldBuilders ?
getOrderByFieldBuilder() : null;
} else {
orderByBuilder_.addAllMessages(other.orderBy_);
}
}
}
if (other.hasLimit()) {
setLimit(other.getLimit());
}
if (other.hasChild()) {
mergeChild(other.getChild());
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
public final boolean isInitialized() {
return true;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
com.pingcap.tidb.tipb.TopN parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (com.pingcap.tidb.tipb.TopN) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
private java.util.List orderBy_ =
java.util.Collections.emptyList();
private void ensureOrderByIsMutable() {
if (!((bitField0_ & 0x00000001) == 0x00000001)) {
orderBy_ = new java.util.ArrayList(orderBy_);
bitField0_ |= 0x00000001;
}
}
private com.google.protobuf.RepeatedFieldBuilderV3<
com.pingcap.tidb.tipb.ByItem, com.pingcap.tidb.tipb.ByItem.Builder, com.pingcap.tidb.tipb.ByItemOrBuilder> orderByBuilder_;
/**
*
* Order by clause.
*
*
* repeated .tipb.ByItem order_by = 1;
*/
public java.util.List getOrderByList() {
if (orderByBuilder_ == null) {
return java.util.Collections.unmodifiableList(orderBy_);
} else {
return orderByBuilder_.getMessageList();
}
}
/**
*
* Order by clause.
*
*
* repeated .tipb.ByItem order_by = 1;
*/
public int getOrderByCount() {
if (orderByBuilder_ == null) {
return orderBy_.size();
} else {
return orderByBuilder_.getCount();
}
}
/**
*
* Order by clause.
*
*
* repeated .tipb.ByItem order_by = 1;
*/
public com.pingcap.tidb.tipb.ByItem getOrderBy(int index) {
if (orderByBuilder_ == null) {
return orderBy_.get(index);
} else {
return orderByBuilder_.getMessage(index);
}
}
/**
*
* Order by clause.
*
*
* repeated .tipb.ByItem order_by = 1;
*/
public Builder setOrderBy(
int index, com.pingcap.tidb.tipb.ByItem value) {
if (orderByBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureOrderByIsMutable();
orderBy_.set(index, value);
onChanged();
} else {
orderByBuilder_.setMessage(index, value);
}
return this;
}
/**
*
* Order by clause.
*
*
* repeated .tipb.ByItem order_by = 1;
*/
public Builder setOrderBy(
int index, com.pingcap.tidb.tipb.ByItem.Builder builderForValue) {
if (orderByBuilder_ == null) {
ensureOrderByIsMutable();
orderBy_.set(index, builderForValue.build());
onChanged();
} else {
orderByBuilder_.setMessage(index, builderForValue.build());
}
return this;
}
/**
*
* Order by clause.
*
*
* repeated .tipb.ByItem order_by = 1;
*/
public Builder addOrderBy(com.pingcap.tidb.tipb.ByItem value) {
if (orderByBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureOrderByIsMutable();
orderBy_.add(value);
onChanged();
} else {
orderByBuilder_.addMessage(value);
}
return this;
}
/**
*
* Order by clause.
*
*
* repeated .tipb.ByItem order_by = 1;
*/
public Builder addOrderBy(
int index, com.pingcap.tidb.tipb.ByItem value) {
if (orderByBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureOrderByIsMutable();
orderBy_.add(index, value);
onChanged();
} else {
orderByBuilder_.addMessage(index, value);
}
return this;
}
/**
*
* Order by clause.
*
*
* repeated .tipb.ByItem order_by = 1;
*/
public Builder addOrderBy(
com.pingcap.tidb.tipb.ByItem.Builder builderForValue) {
if (orderByBuilder_ == null) {
ensureOrderByIsMutable();
orderBy_.add(builderForValue.build());
onChanged();
} else {
orderByBuilder_.addMessage(builderForValue.build());
}
return this;
}
/**
*
* Order by clause.
*
*
* repeated .tipb.ByItem order_by = 1;
*/
public Builder addOrderBy(
int index, com.pingcap.tidb.tipb.ByItem.Builder builderForValue) {
if (orderByBuilder_ == null) {
ensureOrderByIsMutable();
orderBy_.add(index, builderForValue.build());
onChanged();
} else {
orderByBuilder_.addMessage(index, builderForValue.build());
}
return this;
}
/**
*
* Order by clause.
*
*
* repeated .tipb.ByItem order_by = 1;
*/
public Builder addAllOrderBy(
java.lang.Iterable extends com.pingcap.tidb.tipb.ByItem> values) {
if (orderByBuilder_ == null) {
ensureOrderByIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, orderBy_);
onChanged();
} else {
orderByBuilder_.addAllMessages(values);
}
return this;
}
/**
*
* Order by clause.
*
*
* repeated .tipb.ByItem order_by = 1;
*/
public Builder clearOrderBy() {
if (orderByBuilder_ == null) {
orderBy_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000001);
onChanged();
} else {
orderByBuilder_.clear();
}
return this;
}
/**
*
* Order by clause.
*
*
* repeated .tipb.ByItem order_by = 1;
*/
public Builder removeOrderBy(int index) {
if (orderByBuilder_ == null) {
ensureOrderByIsMutable();
orderBy_.remove(index);
onChanged();
} else {
orderByBuilder_.remove(index);
}
return this;
}
/**
*
* Order by clause.
*
*
* repeated .tipb.ByItem order_by = 1;
*/
public com.pingcap.tidb.tipb.ByItem.Builder getOrderByBuilder(
int index) {
return getOrderByFieldBuilder().getBuilder(index);
}
/**
*
* Order by clause.
*
*
* repeated .tipb.ByItem order_by = 1;
*/
public com.pingcap.tidb.tipb.ByItemOrBuilder getOrderByOrBuilder(
int index) {
if (orderByBuilder_ == null) {
return orderBy_.get(index); } else {
return orderByBuilder_.getMessageOrBuilder(index);
}
}
/**
*
* Order by clause.
*
*
* repeated .tipb.ByItem order_by = 1;
*/
public java.util.List extends com.pingcap.tidb.tipb.ByItemOrBuilder>
getOrderByOrBuilderList() {
if (orderByBuilder_ != null) {
return orderByBuilder_.getMessageOrBuilderList();
} else {
return java.util.Collections.unmodifiableList(orderBy_);
}
}
/**
*
* Order by clause.
*
*
* repeated .tipb.ByItem order_by = 1;
*/
public com.pingcap.tidb.tipb.ByItem.Builder addOrderByBuilder() {
return getOrderByFieldBuilder().addBuilder(
com.pingcap.tidb.tipb.ByItem.getDefaultInstance());
}
/**
*
* Order by clause.
*
*
* repeated .tipb.ByItem order_by = 1;
*/
public com.pingcap.tidb.tipb.ByItem.Builder addOrderByBuilder(
int index) {
return getOrderByFieldBuilder().addBuilder(
index, com.pingcap.tidb.tipb.ByItem.getDefaultInstance());
}
/**
*
* Order by clause.
*
*
* repeated .tipb.ByItem order_by = 1;
*/
public java.util.List
getOrderByBuilderList() {
return getOrderByFieldBuilder().getBuilderList();
}
private com.google.protobuf.RepeatedFieldBuilderV3<
com.pingcap.tidb.tipb.ByItem, com.pingcap.tidb.tipb.ByItem.Builder, com.pingcap.tidb.tipb.ByItemOrBuilder>
getOrderByFieldBuilder() {
if (orderByBuilder_ == null) {
orderByBuilder_ = new com.google.protobuf.RepeatedFieldBuilderV3<
com.pingcap.tidb.tipb.ByItem, com.pingcap.tidb.tipb.ByItem.Builder, com.pingcap.tidb.tipb.ByItemOrBuilder>(
orderBy_,
((bitField0_ & 0x00000001) == 0x00000001),
getParentForChildren(),
isClean());
orderBy_ = null;
}
return orderByBuilder_;
}
private long limit_ ;
/**
* optional uint64 limit = 2 [(.gogoproto.nullable) = false];
*/
public boolean hasLimit() {
return ((bitField0_ & 0x00000002) == 0x00000002);
}
/**
* optional uint64 limit = 2 [(.gogoproto.nullable) = false];
*/
public long getLimit() {
return limit_;
}
/**
* optional uint64 limit = 2 [(.gogoproto.nullable) = false];
*/
public Builder setLimit(long value) {
bitField0_ |= 0x00000002;
limit_ = value;
onChanged();
return this;
}
/**
* optional uint64 limit = 2 [(.gogoproto.nullable) = false];
*/
public Builder clearLimit() {
bitField0_ = (bitField0_ & ~0x00000002);
limit_ = 0L;
onChanged();
return this;
}
private com.pingcap.tidb.tipb.Executor child_ = null;
private com.google.protobuf.SingleFieldBuilderV3<
com.pingcap.tidb.tipb.Executor, com.pingcap.tidb.tipb.Executor.Builder, com.pingcap.tidb.tipb.ExecutorOrBuilder> childBuilder_;
/**
* optional .tipb.Executor child = 3;
*/
public boolean hasChild() {
return ((bitField0_ & 0x00000004) == 0x00000004);
}
/**
* optional .tipb.Executor child = 3;
*/
public com.pingcap.tidb.tipb.Executor getChild() {
if (childBuilder_ == null) {
return child_ == null ? com.pingcap.tidb.tipb.Executor.getDefaultInstance() : child_;
} else {
return childBuilder_.getMessage();
}
}
/**
* optional .tipb.Executor child = 3;
*/
public Builder setChild(com.pingcap.tidb.tipb.Executor value) {
if (childBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
child_ = value;
onChanged();
} else {
childBuilder_.setMessage(value);
}
bitField0_ |= 0x00000004;
return this;
}
/**
* optional .tipb.Executor child = 3;
*/
public Builder setChild(
com.pingcap.tidb.tipb.Executor.Builder builderForValue) {
if (childBuilder_ == null) {
child_ = builderForValue.build();
onChanged();
} else {
childBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x00000004;
return this;
}
/**
* optional .tipb.Executor child = 3;
*/
public Builder mergeChild(com.pingcap.tidb.tipb.Executor value) {
if (childBuilder_ == null) {
if (((bitField0_ & 0x00000004) == 0x00000004) &&
child_ != null &&
child_ != com.pingcap.tidb.tipb.Executor.getDefaultInstance()) {
child_ =
com.pingcap.tidb.tipb.Executor.newBuilder(child_).mergeFrom(value).buildPartial();
} else {
child_ = value;
}
onChanged();
} else {
childBuilder_.mergeFrom(value);
}
bitField0_ |= 0x00000004;
return this;
}
/**
* optional .tipb.Executor child = 3;
*/
public Builder clearChild() {
if (childBuilder_ == null) {
child_ = null;
onChanged();
} else {
childBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000004);
return this;
}
/**
* optional .tipb.Executor child = 3;
*/
public com.pingcap.tidb.tipb.Executor.Builder getChildBuilder() {
bitField0_ |= 0x00000004;
onChanged();
return getChildFieldBuilder().getBuilder();
}
/**
* optional .tipb.Executor child = 3;
*/
public com.pingcap.tidb.tipb.ExecutorOrBuilder getChildOrBuilder() {
if (childBuilder_ != null) {
return childBuilder_.getMessageOrBuilder();
} else {
return child_ == null ?
com.pingcap.tidb.tipb.Executor.getDefaultInstance() : child_;
}
}
/**
* optional .tipb.Executor child = 3;
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.pingcap.tidb.tipb.Executor, com.pingcap.tidb.tipb.Executor.Builder, com.pingcap.tidb.tipb.ExecutorOrBuilder>
getChildFieldBuilder() {
if (childBuilder_ == null) {
childBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
com.pingcap.tidb.tipb.Executor, com.pingcap.tidb.tipb.Executor.Builder, com.pingcap.tidb.tipb.ExecutorOrBuilder>(
getChild(),
getParentForChildren(),
isClean());
child_ = null;
}
return childBuilder_;
}
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:tipb.TopN)
}
// @@protoc_insertion_point(class_scope:tipb.TopN)
private static final com.pingcap.tidb.tipb.TopN DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new com.pingcap.tidb.tipb.TopN();
}
public static com.pingcap.tidb.tipb.TopN getDefaultInstance() {
return DEFAULT_INSTANCE;
}
@java.lang.Deprecated public static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
public TopN parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new TopN(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
public com.pingcap.tidb.tipb.TopN getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy