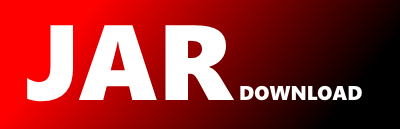
com.pingcap.tikv.codec.TableCodec Maven / Gradle / Ivy
/*
* Copyright 2020 PingCAP, Inc.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.pingcap.tikv.codec;
import com.pingcap.tikv.exception.CodecException;
import com.pingcap.tikv.key.CommonHandle;
import com.pingcap.tikv.key.Handle;
import com.pingcap.tikv.key.IntHandle;
import com.pingcap.tikv.meta.TiColumnInfo;
import com.pingcap.tikv.meta.TiIndexInfo;
import com.pingcap.tikv.meta.TiTableInfo;
import com.pingcap.tikv.row.Row;
import java.util.List;
public class TableCodec {
public static byte IndexVersionFlag = 125;
public static byte CommonHandleFlag = 127;
public static byte[] encodeRow(
List columnInfos,
Object[] values,
boolean isPkHandle,
boolean encodeWithNewRowFormat)
throws IllegalAccessException {
if (columnInfos.size() != values.length) {
throw new IllegalAccessException(
String.format(
"encodeRow error: data and columnID count not " + "match %d vs %d",
columnInfos.size(), values.length));
}
if (encodeWithNewRowFormat) {
return TableCodecV2.encodeRow(columnInfos, values, isPkHandle);
}
return TableCodecV1.encodeRow(columnInfos, values, isPkHandle);
}
public static Row decodeRow(byte[] value, Handle handle, TiTableInfo tableInfo) {
if (value.length == 0) {
throw new CodecException("Decode fails: value length is zero");
}
if ((value[0] & 0xff) == RowV2.CODEC_VER) {
return TableCodecV2.decodeRow(value, handle, tableInfo);
}
return TableCodecV1.decodeRow(value, handle, tableInfo);
}
public static Handle decodeHandle(byte[] value, boolean isCommonHandle) {
if (isCommonHandle) {
return new CommonHandle(value);
}
return new IntHandle(new CodecDataInput(value).readLong());
}
/* only for unique index */
public static byte[] genIndexValueForClusteredIndexVersion1(TiIndexInfo index, Handle handle) {
CodecDataOutput cdo = new CodecDataOutput();
cdo.writeByte(0);
cdo.writeByte(IndexVersionFlag);
cdo.writeByte(1);
assert (index.isUnique());
encodeCommonHandle(cdo, handle);
return cdo.toBytes();
}
private static void encodeCommonHandle(CodecDataOutput cdo, Handle handle) {
cdo.write(CommonHandleFlag);
byte[] encoded = handle.encoded();
int hLen = encoded.length;
cdo.writeShort(hLen);
cdo.write(encoded);
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy