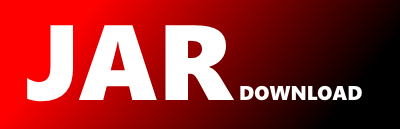
com.pingcap.tikv.codec.TableCodecV2 Maven / Gradle / Ivy
/*
* Copyright 2020 PingCAP, Inc.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.pingcap.tikv.codec;
import com.pingcap.tikv.key.Handle;
import com.pingcap.tikv.meta.TiColumnInfo;
import com.pingcap.tikv.meta.TiIndexColumn;
import com.pingcap.tikv.meta.TiIndexInfo;
import com.pingcap.tikv.meta.TiTableInfo;
import com.pingcap.tikv.row.ObjectRowImpl;
import com.pingcap.tikv.row.Row;
import java.util.ArrayList;
import java.util.HashMap;
import java.util.List;
public class TableCodecV2 {
/**
* New Row Format: Reference
* https://github.com/pingcap/tidb/blob/952d1d7541a8e86be0af58f5b7e3d5e982bab34e/docs/design/2018-07-19-row-format.md
*
* - version, flag, numOfNotNullCols, numOfNullCols, notNullCols, nullCols, notNullOffsets,
* notNullValues
*/
protected static byte[] encodeRow(
List columnInfos, Object[] values, boolean isPkHandle) {
RowEncoderV2 encoder = new RowEncoderV2();
List columnInfoList = new ArrayList<>();
List
© 2015 - 2024 Weber Informatics LLC | Privacy Policy