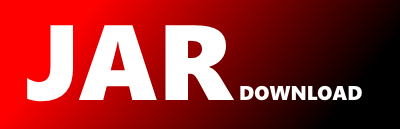
eraftpb.Eraftpb Maven / Gradle / Ivy
// Generated by the protocol buffer compiler. DO NOT EDIT!
// source: eraftpb.proto
package eraftpb;
public final class Eraftpb {
private Eraftpb() {}
public static void registerAllExtensions(
com.google.protobuf.ExtensionRegistryLite registry) {
}
public static void registerAllExtensions(
com.google.protobuf.ExtensionRegistry registry) {
registerAllExtensions(
(com.google.protobuf.ExtensionRegistryLite) registry);
}
/**
* Protobuf enum {@code eraftpb.EntryType}
*/
public enum EntryType
implements com.google.protobuf.ProtocolMessageEnum {
/**
* EntryNormal = 0;
*/
EntryNormal(0),
/**
* EntryConfChange = 1;
*/
EntryConfChange(1),
/**
* EntryConfChangeV2 = 2;
*/
EntryConfChangeV2(2),
UNRECOGNIZED(-1),
;
/**
* EntryNormal = 0;
*/
public static final int EntryNormal_VALUE = 0;
/**
* EntryConfChange = 1;
*/
public static final int EntryConfChange_VALUE = 1;
/**
* EntryConfChangeV2 = 2;
*/
public static final int EntryConfChangeV2_VALUE = 2;
public final int getNumber() {
if (this == UNRECOGNIZED) {
throw new java.lang.IllegalArgumentException(
"Can't get the number of an unknown enum value.");
}
return value;
}
/**
* @deprecated Use {@link #forNumber(int)} instead.
*/
@java.lang.Deprecated
public static EntryType valueOf(int value) {
return forNumber(value);
}
public static EntryType forNumber(int value) {
switch (value) {
case 0: return EntryNormal;
case 1: return EntryConfChange;
case 2: return EntryConfChangeV2;
default: return null;
}
}
public static com.google.protobuf.Internal.EnumLiteMap
internalGetValueMap() {
return internalValueMap;
}
private static final com.google.protobuf.Internal.EnumLiteMap<
EntryType> internalValueMap =
new com.google.protobuf.Internal.EnumLiteMap() {
public EntryType findValueByNumber(int number) {
return EntryType.forNumber(number);
}
};
public final com.google.protobuf.Descriptors.EnumValueDescriptor
getValueDescriptor() {
return getDescriptor().getValues().get(ordinal());
}
public final com.google.protobuf.Descriptors.EnumDescriptor
getDescriptorForType() {
return getDescriptor();
}
public static final com.google.protobuf.Descriptors.EnumDescriptor
getDescriptor() {
return eraftpb.Eraftpb.getDescriptor().getEnumTypes().get(0);
}
private static final EntryType[] VALUES = values();
public static EntryType valueOf(
com.google.protobuf.Descriptors.EnumValueDescriptor desc) {
if (desc.getType() != getDescriptor()) {
throw new java.lang.IllegalArgumentException(
"EnumValueDescriptor is not for this type.");
}
if (desc.getIndex() == -1) {
return UNRECOGNIZED;
}
return VALUES[desc.getIndex()];
}
private final int value;
private EntryType(int value) {
this.value = value;
}
// @@protoc_insertion_point(enum_scope:eraftpb.EntryType)
}
/**
* Protobuf enum {@code eraftpb.MessageType}
*/
public enum MessageType
implements com.google.protobuf.ProtocolMessageEnum {
/**
* MsgHup = 0;
*/
MsgHup(0),
/**
* MsgBeat = 1;
*/
MsgBeat(1),
/**
* MsgPropose = 2;
*/
MsgPropose(2),
/**
* MsgAppend = 3;
*/
MsgAppend(3),
/**
* MsgAppendResponse = 4;
*/
MsgAppendResponse(4),
/**
* MsgRequestVote = 5;
*/
MsgRequestVote(5),
/**
* MsgRequestVoteResponse = 6;
*/
MsgRequestVoteResponse(6),
/**
* MsgSnapshot = 7;
*/
MsgSnapshot(7),
/**
* MsgHeartbeat = 8;
*/
MsgHeartbeat(8),
/**
* MsgHeartbeatResponse = 9;
*/
MsgHeartbeatResponse(9),
/**
* MsgUnreachable = 10;
*/
MsgUnreachable(10),
/**
* MsgSnapStatus = 11;
*/
MsgSnapStatus(11),
/**
* MsgCheckQuorum = 12;
*/
MsgCheckQuorum(12),
/**
* MsgTransferLeader = 13;
*/
MsgTransferLeader(13),
/**
* MsgTimeoutNow = 14;
*/
MsgTimeoutNow(14),
/**
* MsgReadIndex = 15;
*/
MsgReadIndex(15),
/**
* MsgReadIndexResp = 16;
*/
MsgReadIndexResp(16),
/**
* MsgRequestPreVote = 17;
*/
MsgRequestPreVote(17),
/**
* MsgRequestPreVoteResponse = 18;
*/
MsgRequestPreVoteResponse(18),
UNRECOGNIZED(-1),
;
/**
* MsgHup = 0;
*/
public static final int MsgHup_VALUE = 0;
/**
* MsgBeat = 1;
*/
public static final int MsgBeat_VALUE = 1;
/**
* MsgPropose = 2;
*/
public static final int MsgPropose_VALUE = 2;
/**
* MsgAppend = 3;
*/
public static final int MsgAppend_VALUE = 3;
/**
* MsgAppendResponse = 4;
*/
public static final int MsgAppendResponse_VALUE = 4;
/**
* MsgRequestVote = 5;
*/
public static final int MsgRequestVote_VALUE = 5;
/**
* MsgRequestVoteResponse = 6;
*/
public static final int MsgRequestVoteResponse_VALUE = 6;
/**
* MsgSnapshot = 7;
*/
public static final int MsgSnapshot_VALUE = 7;
/**
* MsgHeartbeat = 8;
*/
public static final int MsgHeartbeat_VALUE = 8;
/**
* MsgHeartbeatResponse = 9;
*/
public static final int MsgHeartbeatResponse_VALUE = 9;
/**
* MsgUnreachable = 10;
*/
public static final int MsgUnreachable_VALUE = 10;
/**
* MsgSnapStatus = 11;
*/
public static final int MsgSnapStatus_VALUE = 11;
/**
* MsgCheckQuorum = 12;
*/
public static final int MsgCheckQuorum_VALUE = 12;
/**
* MsgTransferLeader = 13;
*/
public static final int MsgTransferLeader_VALUE = 13;
/**
* MsgTimeoutNow = 14;
*/
public static final int MsgTimeoutNow_VALUE = 14;
/**
* MsgReadIndex = 15;
*/
public static final int MsgReadIndex_VALUE = 15;
/**
* MsgReadIndexResp = 16;
*/
public static final int MsgReadIndexResp_VALUE = 16;
/**
* MsgRequestPreVote = 17;
*/
public static final int MsgRequestPreVote_VALUE = 17;
/**
* MsgRequestPreVoteResponse = 18;
*/
public static final int MsgRequestPreVoteResponse_VALUE = 18;
public final int getNumber() {
if (this == UNRECOGNIZED) {
throw new java.lang.IllegalArgumentException(
"Can't get the number of an unknown enum value.");
}
return value;
}
/**
* @deprecated Use {@link #forNumber(int)} instead.
*/
@java.lang.Deprecated
public static MessageType valueOf(int value) {
return forNumber(value);
}
public static MessageType forNumber(int value) {
switch (value) {
case 0: return MsgHup;
case 1: return MsgBeat;
case 2: return MsgPropose;
case 3: return MsgAppend;
case 4: return MsgAppendResponse;
case 5: return MsgRequestVote;
case 6: return MsgRequestVoteResponse;
case 7: return MsgSnapshot;
case 8: return MsgHeartbeat;
case 9: return MsgHeartbeatResponse;
case 10: return MsgUnreachable;
case 11: return MsgSnapStatus;
case 12: return MsgCheckQuorum;
case 13: return MsgTransferLeader;
case 14: return MsgTimeoutNow;
case 15: return MsgReadIndex;
case 16: return MsgReadIndexResp;
case 17: return MsgRequestPreVote;
case 18: return MsgRequestPreVoteResponse;
default: return null;
}
}
public static com.google.protobuf.Internal.EnumLiteMap
internalGetValueMap() {
return internalValueMap;
}
private static final com.google.protobuf.Internal.EnumLiteMap<
MessageType> internalValueMap =
new com.google.protobuf.Internal.EnumLiteMap() {
public MessageType findValueByNumber(int number) {
return MessageType.forNumber(number);
}
};
public final com.google.protobuf.Descriptors.EnumValueDescriptor
getValueDescriptor() {
return getDescriptor().getValues().get(ordinal());
}
public final com.google.protobuf.Descriptors.EnumDescriptor
getDescriptorForType() {
return getDescriptor();
}
public static final com.google.protobuf.Descriptors.EnumDescriptor
getDescriptor() {
return eraftpb.Eraftpb.getDescriptor().getEnumTypes().get(1);
}
private static final MessageType[] VALUES = values();
public static MessageType valueOf(
com.google.protobuf.Descriptors.EnumValueDescriptor desc) {
if (desc.getType() != getDescriptor()) {
throw new java.lang.IllegalArgumentException(
"EnumValueDescriptor is not for this type.");
}
if (desc.getIndex() == -1) {
return UNRECOGNIZED;
}
return VALUES[desc.getIndex()];
}
private final int value;
private MessageType(int value) {
this.value = value;
}
// @@protoc_insertion_point(enum_scope:eraftpb.MessageType)
}
/**
* Protobuf enum {@code eraftpb.ConfChangeTransition}
*/
public enum ConfChangeTransition
implements com.google.protobuf.ProtocolMessageEnum {
/**
*
* Automatically use the simple protocol if possible, otherwise fall back
* to ConfChangeType::Implicit. Most applications will want to use this.
*
*
* Auto = 0;
*/
Auto(0),
/**
*
* Use joint consensus unconditionally, and transition out of them
* automatically (by proposing a zero configuration change).
* This option is suitable for applications that want to minimize the time
* spent in the joint configuration and do not store the joint configuration
* in the state machine (outside of InitialState).
*
*
* Implicit = 1;
*/
Implicit(1),
/**
*
* Use joint consensus and remain in the joint configuration until the
* application proposes a no-op configuration change. This is suitable for
* applications that want to explicitly control the transitions, for example
* to use a custom payload (via the Context field).
*
*
* Explicit = 2;
*/
Explicit(2),
UNRECOGNIZED(-1),
;
/**
*
* Automatically use the simple protocol if possible, otherwise fall back
* to ConfChangeType::Implicit. Most applications will want to use this.
*
*
* Auto = 0;
*/
public static final int Auto_VALUE = 0;
/**
*
* Use joint consensus unconditionally, and transition out of them
* automatically (by proposing a zero configuration change).
* This option is suitable for applications that want to minimize the time
* spent in the joint configuration and do not store the joint configuration
* in the state machine (outside of InitialState).
*
*
* Implicit = 1;
*/
public static final int Implicit_VALUE = 1;
/**
*
* Use joint consensus and remain in the joint configuration until the
* application proposes a no-op configuration change. This is suitable for
* applications that want to explicitly control the transitions, for example
* to use a custom payload (via the Context field).
*
*
* Explicit = 2;
*/
public static final int Explicit_VALUE = 2;
public final int getNumber() {
if (this == UNRECOGNIZED) {
throw new java.lang.IllegalArgumentException(
"Can't get the number of an unknown enum value.");
}
return value;
}
/**
* @deprecated Use {@link #forNumber(int)} instead.
*/
@java.lang.Deprecated
public static ConfChangeTransition valueOf(int value) {
return forNumber(value);
}
public static ConfChangeTransition forNumber(int value) {
switch (value) {
case 0: return Auto;
case 1: return Implicit;
case 2: return Explicit;
default: return null;
}
}
public static com.google.protobuf.Internal.EnumLiteMap
internalGetValueMap() {
return internalValueMap;
}
private static final com.google.protobuf.Internal.EnumLiteMap<
ConfChangeTransition> internalValueMap =
new com.google.protobuf.Internal.EnumLiteMap() {
public ConfChangeTransition findValueByNumber(int number) {
return ConfChangeTransition.forNumber(number);
}
};
public final com.google.protobuf.Descriptors.EnumValueDescriptor
getValueDescriptor() {
return getDescriptor().getValues().get(ordinal());
}
public final com.google.protobuf.Descriptors.EnumDescriptor
getDescriptorForType() {
return getDescriptor();
}
public static final com.google.protobuf.Descriptors.EnumDescriptor
getDescriptor() {
return eraftpb.Eraftpb.getDescriptor().getEnumTypes().get(2);
}
private static final ConfChangeTransition[] VALUES = values();
public static ConfChangeTransition valueOf(
com.google.protobuf.Descriptors.EnumValueDescriptor desc) {
if (desc.getType() != getDescriptor()) {
throw new java.lang.IllegalArgumentException(
"EnumValueDescriptor is not for this type.");
}
if (desc.getIndex() == -1) {
return UNRECOGNIZED;
}
return VALUES[desc.getIndex()];
}
private final int value;
private ConfChangeTransition(int value) {
this.value = value;
}
// @@protoc_insertion_point(enum_scope:eraftpb.ConfChangeTransition)
}
/**
* Protobuf enum {@code eraftpb.ConfChangeType}
*/
public enum ConfChangeType
implements com.google.protobuf.ProtocolMessageEnum {
/**
* AddNode = 0;
*/
AddNode(0),
/**
* RemoveNode = 1;
*/
RemoveNode(1),
/**
* AddLearnerNode = 2;
*/
AddLearnerNode(2),
UNRECOGNIZED(-1),
;
/**
* AddNode = 0;
*/
public static final int AddNode_VALUE = 0;
/**
* RemoveNode = 1;
*/
public static final int RemoveNode_VALUE = 1;
/**
* AddLearnerNode = 2;
*/
public static final int AddLearnerNode_VALUE = 2;
public final int getNumber() {
if (this == UNRECOGNIZED) {
throw new java.lang.IllegalArgumentException(
"Can't get the number of an unknown enum value.");
}
return value;
}
/**
* @deprecated Use {@link #forNumber(int)} instead.
*/
@java.lang.Deprecated
public static ConfChangeType valueOf(int value) {
return forNumber(value);
}
public static ConfChangeType forNumber(int value) {
switch (value) {
case 0: return AddNode;
case 1: return RemoveNode;
case 2: return AddLearnerNode;
default: return null;
}
}
public static com.google.protobuf.Internal.EnumLiteMap
internalGetValueMap() {
return internalValueMap;
}
private static final com.google.protobuf.Internal.EnumLiteMap<
ConfChangeType> internalValueMap =
new com.google.protobuf.Internal.EnumLiteMap() {
public ConfChangeType findValueByNumber(int number) {
return ConfChangeType.forNumber(number);
}
};
public final com.google.protobuf.Descriptors.EnumValueDescriptor
getValueDescriptor() {
return getDescriptor().getValues().get(ordinal());
}
public final com.google.protobuf.Descriptors.EnumDescriptor
getDescriptorForType() {
return getDescriptor();
}
public static final com.google.protobuf.Descriptors.EnumDescriptor
getDescriptor() {
return eraftpb.Eraftpb.getDescriptor().getEnumTypes().get(3);
}
private static final ConfChangeType[] VALUES = values();
public static ConfChangeType valueOf(
com.google.protobuf.Descriptors.EnumValueDescriptor desc) {
if (desc.getType() != getDescriptor()) {
throw new java.lang.IllegalArgumentException(
"EnumValueDescriptor is not for this type.");
}
if (desc.getIndex() == -1) {
return UNRECOGNIZED;
}
return VALUES[desc.getIndex()];
}
private final int value;
private ConfChangeType(int value) {
this.value = value;
}
// @@protoc_insertion_point(enum_scope:eraftpb.ConfChangeType)
}
public interface EntryOrBuilder extends
// @@protoc_insertion_point(interface_extends:eraftpb.Entry)
com.google.protobuf.MessageOrBuilder {
/**
* .eraftpb.EntryType entry_type = 1;
*/
int getEntryTypeValue();
/**
* .eraftpb.EntryType entry_type = 1;
*/
eraftpb.Eraftpb.EntryType getEntryType();
/**
* uint64 term = 2;
*/
long getTerm();
/**
* uint64 index = 3;
*/
long getIndex();
/**
* bytes data = 4;
*/
com.google.protobuf.ByteString getData();
/**
* bytes context = 6;
*/
com.google.protobuf.ByteString getContext();
/**
*
* Deprecated! It is kept for backward compatibility.
* TODO: remove it in the next major release.
*
*
* bool sync_log = 5;
*/
boolean getSyncLog();
}
/**
*
* The entry is a type of change that needs to be applied. It contains two data fields.
* While the fields are built into the model; their usage is determined by the entry_type.
* For normal entries, the data field should contain the data change that should be applied.
* The context field can be used for any contextual data that might be relevant to the
* application of the data.
* For configuration changes, the data will contain the ConfChange message and the
* context will provide anything needed to assist the configuration change. The context
* if for the user to set and use in this case.
*
*
* Protobuf type {@code eraftpb.Entry}
*/
public static final class Entry extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:eraftpb.Entry)
EntryOrBuilder {
private static final long serialVersionUID = 0L;
// Use Entry.newBuilder() to construct.
private Entry(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private Entry() {
entryType_ = 0;
term_ = 0L;
index_ = 0L;
data_ = com.google.protobuf.ByteString.EMPTY;
context_ = com.google.protobuf.ByteString.EMPTY;
syncLog_ = false;
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private Entry(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
default: {
if (!parseUnknownFieldProto3(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
case 8: {
int rawValue = input.readEnum();
entryType_ = rawValue;
break;
}
case 16: {
term_ = input.readUInt64();
break;
}
case 24: {
index_ = input.readUInt64();
break;
}
case 34: {
data_ = input.readBytes();
break;
}
case 40: {
syncLog_ = input.readBool();
break;
}
case 50: {
context_ = input.readBytes();
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return eraftpb.Eraftpb.internal_static_eraftpb_Entry_descriptor;
}
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return eraftpb.Eraftpb.internal_static_eraftpb_Entry_fieldAccessorTable
.ensureFieldAccessorsInitialized(
eraftpb.Eraftpb.Entry.class, eraftpb.Eraftpb.Entry.Builder.class);
}
public static final int ENTRY_TYPE_FIELD_NUMBER = 1;
private int entryType_;
/**
* .eraftpb.EntryType entry_type = 1;
*/
public int getEntryTypeValue() {
return entryType_;
}
/**
* .eraftpb.EntryType entry_type = 1;
*/
public eraftpb.Eraftpb.EntryType getEntryType() {
eraftpb.Eraftpb.EntryType result = eraftpb.Eraftpb.EntryType.valueOf(entryType_);
return result == null ? eraftpb.Eraftpb.EntryType.UNRECOGNIZED : result;
}
public static final int TERM_FIELD_NUMBER = 2;
private long term_;
/**
* uint64 term = 2;
*/
public long getTerm() {
return term_;
}
public static final int INDEX_FIELD_NUMBER = 3;
private long index_;
/**
* uint64 index = 3;
*/
public long getIndex() {
return index_;
}
public static final int DATA_FIELD_NUMBER = 4;
private com.google.protobuf.ByteString data_;
/**
* bytes data = 4;
*/
public com.google.protobuf.ByteString getData() {
return data_;
}
public static final int CONTEXT_FIELD_NUMBER = 6;
private com.google.protobuf.ByteString context_;
/**
* bytes context = 6;
*/
public com.google.protobuf.ByteString getContext() {
return context_;
}
public static final int SYNC_LOG_FIELD_NUMBER = 5;
private boolean syncLog_;
/**
*
* Deprecated! It is kept for backward compatibility.
* TODO: remove it in the next major release.
*
*
* bool sync_log = 5;
*/
public boolean getSyncLog() {
return syncLog_;
}
private byte memoizedIsInitialized = -1;
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (entryType_ != eraftpb.Eraftpb.EntryType.EntryNormal.getNumber()) {
output.writeEnum(1, entryType_);
}
if (term_ != 0L) {
output.writeUInt64(2, term_);
}
if (index_ != 0L) {
output.writeUInt64(3, index_);
}
if (!data_.isEmpty()) {
output.writeBytes(4, data_);
}
if (syncLog_ != false) {
output.writeBool(5, syncLog_);
}
if (!context_.isEmpty()) {
output.writeBytes(6, context_);
}
unknownFields.writeTo(output);
}
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (entryType_ != eraftpb.Eraftpb.EntryType.EntryNormal.getNumber()) {
size += com.google.protobuf.CodedOutputStream
.computeEnumSize(1, entryType_);
}
if (term_ != 0L) {
size += com.google.protobuf.CodedOutputStream
.computeUInt64Size(2, term_);
}
if (index_ != 0L) {
size += com.google.protobuf.CodedOutputStream
.computeUInt64Size(3, index_);
}
if (!data_.isEmpty()) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(4, data_);
}
if (syncLog_ != false) {
size += com.google.protobuf.CodedOutputStream
.computeBoolSize(5, syncLog_);
}
if (!context_.isEmpty()) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(6, context_);
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof eraftpb.Eraftpb.Entry)) {
return super.equals(obj);
}
eraftpb.Eraftpb.Entry other = (eraftpb.Eraftpb.Entry) obj;
boolean result = true;
result = result && entryType_ == other.entryType_;
result = result && (getTerm()
== other.getTerm());
result = result && (getIndex()
== other.getIndex());
result = result && getData()
.equals(other.getData());
result = result && getContext()
.equals(other.getContext());
result = result && (getSyncLog()
== other.getSyncLog());
result = result && unknownFields.equals(other.unknownFields);
return result;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
hash = (37 * hash) + ENTRY_TYPE_FIELD_NUMBER;
hash = (53 * hash) + entryType_;
hash = (37 * hash) + TERM_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
getTerm());
hash = (37 * hash) + INDEX_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
getIndex());
hash = (37 * hash) + DATA_FIELD_NUMBER;
hash = (53 * hash) + getData().hashCode();
hash = (37 * hash) + CONTEXT_FIELD_NUMBER;
hash = (53 * hash) + getContext().hashCode();
hash = (37 * hash) + SYNC_LOG_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashBoolean(
getSyncLog());
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static eraftpb.Eraftpb.Entry parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static eraftpb.Eraftpb.Entry parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static eraftpb.Eraftpb.Entry parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static eraftpb.Eraftpb.Entry parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static eraftpb.Eraftpb.Entry parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static eraftpb.Eraftpb.Entry parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static eraftpb.Eraftpb.Entry parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static eraftpb.Eraftpb.Entry parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static eraftpb.Eraftpb.Entry parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static eraftpb.Eraftpb.Entry parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static eraftpb.Eraftpb.Entry parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static eraftpb.Eraftpb.Entry parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(eraftpb.Eraftpb.Entry prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
* The entry is a type of change that needs to be applied. It contains two data fields.
* While the fields are built into the model; their usage is determined by the entry_type.
* For normal entries, the data field should contain the data change that should be applied.
* The context field can be used for any contextual data that might be relevant to the
* application of the data.
* For configuration changes, the data will contain the ConfChange message and the
* context will provide anything needed to assist the configuration change. The context
* if for the user to set and use in this case.
*
*
* Protobuf type {@code eraftpb.Entry}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:eraftpb.Entry)
eraftpb.Eraftpb.EntryOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return eraftpb.Eraftpb.internal_static_eraftpb_Entry_descriptor;
}
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return eraftpb.Eraftpb.internal_static_eraftpb_Entry_fieldAccessorTable
.ensureFieldAccessorsInitialized(
eraftpb.Eraftpb.Entry.class, eraftpb.Eraftpb.Entry.Builder.class);
}
// Construct using eraftpb.Eraftpb.Entry.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
public Builder clear() {
super.clear();
entryType_ = 0;
term_ = 0L;
index_ = 0L;
data_ = com.google.protobuf.ByteString.EMPTY;
context_ = com.google.protobuf.ByteString.EMPTY;
syncLog_ = false;
return this;
}
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return eraftpb.Eraftpb.internal_static_eraftpb_Entry_descriptor;
}
public eraftpb.Eraftpb.Entry getDefaultInstanceForType() {
return eraftpb.Eraftpb.Entry.getDefaultInstance();
}
public eraftpb.Eraftpb.Entry build() {
eraftpb.Eraftpb.Entry result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
public eraftpb.Eraftpb.Entry buildPartial() {
eraftpb.Eraftpb.Entry result = new eraftpb.Eraftpb.Entry(this);
result.entryType_ = entryType_;
result.term_ = term_;
result.index_ = index_;
result.data_ = data_;
result.context_ = context_;
result.syncLog_ = syncLog_;
onBuilt();
return result;
}
public Builder clone() {
return (Builder) super.clone();
}
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.setField(field, value);
}
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return (Builder) super.clearField(field);
}
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return (Builder) super.clearOneof(oneof);
}
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return (Builder) super.setRepeatedField(field, index, value);
}
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.addRepeatedField(field, value);
}
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof eraftpb.Eraftpb.Entry) {
return mergeFrom((eraftpb.Eraftpb.Entry)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(eraftpb.Eraftpb.Entry other) {
if (other == eraftpb.Eraftpb.Entry.getDefaultInstance()) return this;
if (other.entryType_ != 0) {
setEntryTypeValue(other.getEntryTypeValue());
}
if (other.getTerm() != 0L) {
setTerm(other.getTerm());
}
if (other.getIndex() != 0L) {
setIndex(other.getIndex());
}
if (other.getData() != com.google.protobuf.ByteString.EMPTY) {
setData(other.getData());
}
if (other.getContext() != com.google.protobuf.ByteString.EMPTY) {
setContext(other.getContext());
}
if (other.getSyncLog() != false) {
setSyncLog(other.getSyncLog());
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
public final boolean isInitialized() {
return true;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
eraftpb.Eraftpb.Entry parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (eraftpb.Eraftpb.Entry) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int entryType_ = 0;
/**
* .eraftpb.EntryType entry_type = 1;
*/
public int getEntryTypeValue() {
return entryType_;
}
/**
* .eraftpb.EntryType entry_type = 1;
*/
public Builder setEntryTypeValue(int value) {
entryType_ = value;
onChanged();
return this;
}
/**
* .eraftpb.EntryType entry_type = 1;
*/
public eraftpb.Eraftpb.EntryType getEntryType() {
eraftpb.Eraftpb.EntryType result = eraftpb.Eraftpb.EntryType.valueOf(entryType_);
return result == null ? eraftpb.Eraftpb.EntryType.UNRECOGNIZED : result;
}
/**
* .eraftpb.EntryType entry_type = 1;
*/
public Builder setEntryType(eraftpb.Eraftpb.EntryType value) {
if (value == null) {
throw new NullPointerException();
}
entryType_ = value.getNumber();
onChanged();
return this;
}
/**
* .eraftpb.EntryType entry_type = 1;
*/
public Builder clearEntryType() {
entryType_ = 0;
onChanged();
return this;
}
private long term_ ;
/**
* uint64 term = 2;
*/
public long getTerm() {
return term_;
}
/**
* uint64 term = 2;
*/
public Builder setTerm(long value) {
term_ = value;
onChanged();
return this;
}
/**
* uint64 term = 2;
*/
public Builder clearTerm() {
term_ = 0L;
onChanged();
return this;
}
private long index_ ;
/**
* uint64 index = 3;
*/
public long getIndex() {
return index_;
}
/**
* uint64 index = 3;
*/
public Builder setIndex(long value) {
index_ = value;
onChanged();
return this;
}
/**
* uint64 index = 3;
*/
public Builder clearIndex() {
index_ = 0L;
onChanged();
return this;
}
private com.google.protobuf.ByteString data_ = com.google.protobuf.ByteString.EMPTY;
/**
* bytes data = 4;
*/
public com.google.protobuf.ByteString getData() {
return data_;
}
/**
* bytes data = 4;
*/
public Builder setData(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
data_ = value;
onChanged();
return this;
}
/**
* bytes data = 4;
*/
public Builder clearData() {
data_ = getDefaultInstance().getData();
onChanged();
return this;
}
private com.google.protobuf.ByteString context_ = com.google.protobuf.ByteString.EMPTY;
/**
* bytes context = 6;
*/
public com.google.protobuf.ByteString getContext() {
return context_;
}
/**
* bytes context = 6;
*/
public Builder setContext(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
context_ = value;
onChanged();
return this;
}
/**
* bytes context = 6;
*/
public Builder clearContext() {
context_ = getDefaultInstance().getContext();
onChanged();
return this;
}
private boolean syncLog_ ;
/**
*
* Deprecated! It is kept for backward compatibility.
* TODO: remove it in the next major release.
*
*
* bool sync_log = 5;
*/
public boolean getSyncLog() {
return syncLog_;
}
/**
*
* Deprecated! It is kept for backward compatibility.
* TODO: remove it in the next major release.
*
*
* bool sync_log = 5;
*/
public Builder setSyncLog(boolean value) {
syncLog_ = value;
onChanged();
return this;
}
/**
*
* Deprecated! It is kept for backward compatibility.
* TODO: remove it in the next major release.
*
*
* bool sync_log = 5;
*/
public Builder clearSyncLog() {
syncLog_ = false;
onChanged();
return this;
}
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFieldsProto3(unknownFields);
}
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:eraftpb.Entry)
}
// @@protoc_insertion_point(class_scope:eraftpb.Entry)
private static final eraftpb.Eraftpb.Entry DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new eraftpb.Eraftpb.Entry();
}
public static eraftpb.Eraftpb.Entry getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
public Entry parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new Entry(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
public eraftpb.Eraftpb.Entry getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface SnapshotMetadataOrBuilder extends
// @@protoc_insertion_point(interface_extends:eraftpb.SnapshotMetadata)
com.google.protobuf.MessageOrBuilder {
/**
*
* The current `ConfState`.
*
*
* .eraftpb.ConfState conf_state = 1;
*/
boolean hasConfState();
/**
*
* The current `ConfState`.
*
*
* .eraftpb.ConfState conf_state = 1;
*/
eraftpb.Eraftpb.ConfState getConfState();
/**
*
* The current `ConfState`.
*
*
* .eraftpb.ConfState conf_state = 1;
*/
eraftpb.Eraftpb.ConfStateOrBuilder getConfStateOrBuilder();
/**
*
* The applied index.
*
*
* uint64 index = 2;
*/
long getIndex();
/**
*
* The term of the applied index.
*
*
* uint64 term = 3;
*/
long getTerm();
}
/**
* Protobuf type {@code eraftpb.SnapshotMetadata}
*/
public static final class SnapshotMetadata extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:eraftpb.SnapshotMetadata)
SnapshotMetadataOrBuilder {
private static final long serialVersionUID = 0L;
// Use SnapshotMetadata.newBuilder() to construct.
private SnapshotMetadata(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private SnapshotMetadata() {
index_ = 0L;
term_ = 0L;
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private SnapshotMetadata(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
default: {
if (!parseUnknownFieldProto3(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
case 10: {
eraftpb.Eraftpb.ConfState.Builder subBuilder = null;
if (confState_ != null) {
subBuilder = confState_.toBuilder();
}
confState_ = input.readMessage(eraftpb.Eraftpb.ConfState.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(confState_);
confState_ = subBuilder.buildPartial();
}
break;
}
case 16: {
index_ = input.readUInt64();
break;
}
case 24: {
term_ = input.readUInt64();
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return eraftpb.Eraftpb.internal_static_eraftpb_SnapshotMetadata_descriptor;
}
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return eraftpb.Eraftpb.internal_static_eraftpb_SnapshotMetadata_fieldAccessorTable
.ensureFieldAccessorsInitialized(
eraftpb.Eraftpb.SnapshotMetadata.class, eraftpb.Eraftpb.SnapshotMetadata.Builder.class);
}
public static final int CONF_STATE_FIELD_NUMBER = 1;
private eraftpb.Eraftpb.ConfState confState_;
/**
*
* The current `ConfState`.
*
*
* .eraftpb.ConfState conf_state = 1;
*/
public boolean hasConfState() {
return confState_ != null;
}
/**
*
* The current `ConfState`.
*
*
* .eraftpb.ConfState conf_state = 1;
*/
public eraftpb.Eraftpb.ConfState getConfState() {
return confState_ == null ? eraftpb.Eraftpb.ConfState.getDefaultInstance() : confState_;
}
/**
*
* The current `ConfState`.
*
*
* .eraftpb.ConfState conf_state = 1;
*/
public eraftpb.Eraftpb.ConfStateOrBuilder getConfStateOrBuilder() {
return getConfState();
}
public static final int INDEX_FIELD_NUMBER = 2;
private long index_;
/**
*
* The applied index.
*
*
* uint64 index = 2;
*/
public long getIndex() {
return index_;
}
public static final int TERM_FIELD_NUMBER = 3;
private long term_;
/**
*
* The term of the applied index.
*
*
* uint64 term = 3;
*/
public long getTerm() {
return term_;
}
private byte memoizedIsInitialized = -1;
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (confState_ != null) {
output.writeMessage(1, getConfState());
}
if (index_ != 0L) {
output.writeUInt64(2, index_);
}
if (term_ != 0L) {
output.writeUInt64(3, term_);
}
unknownFields.writeTo(output);
}
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (confState_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(1, getConfState());
}
if (index_ != 0L) {
size += com.google.protobuf.CodedOutputStream
.computeUInt64Size(2, index_);
}
if (term_ != 0L) {
size += com.google.protobuf.CodedOutputStream
.computeUInt64Size(3, term_);
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof eraftpb.Eraftpb.SnapshotMetadata)) {
return super.equals(obj);
}
eraftpb.Eraftpb.SnapshotMetadata other = (eraftpb.Eraftpb.SnapshotMetadata) obj;
boolean result = true;
result = result && (hasConfState() == other.hasConfState());
if (hasConfState()) {
result = result && getConfState()
.equals(other.getConfState());
}
result = result && (getIndex()
== other.getIndex());
result = result && (getTerm()
== other.getTerm());
result = result && unknownFields.equals(other.unknownFields);
return result;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (hasConfState()) {
hash = (37 * hash) + CONF_STATE_FIELD_NUMBER;
hash = (53 * hash) + getConfState().hashCode();
}
hash = (37 * hash) + INDEX_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
getIndex());
hash = (37 * hash) + TERM_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
getTerm());
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static eraftpb.Eraftpb.SnapshotMetadata parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static eraftpb.Eraftpb.SnapshotMetadata parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static eraftpb.Eraftpb.SnapshotMetadata parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static eraftpb.Eraftpb.SnapshotMetadata parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static eraftpb.Eraftpb.SnapshotMetadata parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static eraftpb.Eraftpb.SnapshotMetadata parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static eraftpb.Eraftpb.SnapshotMetadata parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static eraftpb.Eraftpb.SnapshotMetadata parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static eraftpb.Eraftpb.SnapshotMetadata parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static eraftpb.Eraftpb.SnapshotMetadata parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static eraftpb.Eraftpb.SnapshotMetadata parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static eraftpb.Eraftpb.SnapshotMetadata parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(eraftpb.Eraftpb.SnapshotMetadata prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code eraftpb.SnapshotMetadata}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:eraftpb.SnapshotMetadata)
eraftpb.Eraftpb.SnapshotMetadataOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return eraftpb.Eraftpb.internal_static_eraftpb_SnapshotMetadata_descriptor;
}
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return eraftpb.Eraftpb.internal_static_eraftpb_SnapshotMetadata_fieldAccessorTable
.ensureFieldAccessorsInitialized(
eraftpb.Eraftpb.SnapshotMetadata.class, eraftpb.Eraftpb.SnapshotMetadata.Builder.class);
}
// Construct using eraftpb.Eraftpb.SnapshotMetadata.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
public Builder clear() {
super.clear();
if (confStateBuilder_ == null) {
confState_ = null;
} else {
confState_ = null;
confStateBuilder_ = null;
}
index_ = 0L;
term_ = 0L;
return this;
}
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return eraftpb.Eraftpb.internal_static_eraftpb_SnapshotMetadata_descriptor;
}
public eraftpb.Eraftpb.SnapshotMetadata getDefaultInstanceForType() {
return eraftpb.Eraftpb.SnapshotMetadata.getDefaultInstance();
}
public eraftpb.Eraftpb.SnapshotMetadata build() {
eraftpb.Eraftpb.SnapshotMetadata result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
public eraftpb.Eraftpb.SnapshotMetadata buildPartial() {
eraftpb.Eraftpb.SnapshotMetadata result = new eraftpb.Eraftpb.SnapshotMetadata(this);
if (confStateBuilder_ == null) {
result.confState_ = confState_;
} else {
result.confState_ = confStateBuilder_.build();
}
result.index_ = index_;
result.term_ = term_;
onBuilt();
return result;
}
public Builder clone() {
return (Builder) super.clone();
}
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.setField(field, value);
}
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return (Builder) super.clearField(field);
}
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return (Builder) super.clearOneof(oneof);
}
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return (Builder) super.setRepeatedField(field, index, value);
}
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.addRepeatedField(field, value);
}
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof eraftpb.Eraftpb.SnapshotMetadata) {
return mergeFrom((eraftpb.Eraftpb.SnapshotMetadata)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(eraftpb.Eraftpb.SnapshotMetadata other) {
if (other == eraftpb.Eraftpb.SnapshotMetadata.getDefaultInstance()) return this;
if (other.hasConfState()) {
mergeConfState(other.getConfState());
}
if (other.getIndex() != 0L) {
setIndex(other.getIndex());
}
if (other.getTerm() != 0L) {
setTerm(other.getTerm());
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
public final boolean isInitialized() {
return true;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
eraftpb.Eraftpb.SnapshotMetadata parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (eraftpb.Eraftpb.SnapshotMetadata) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private eraftpb.Eraftpb.ConfState confState_ = null;
private com.google.protobuf.SingleFieldBuilderV3<
eraftpb.Eraftpb.ConfState, eraftpb.Eraftpb.ConfState.Builder, eraftpb.Eraftpb.ConfStateOrBuilder> confStateBuilder_;
/**
*
* The current `ConfState`.
*
*
* .eraftpb.ConfState conf_state = 1;
*/
public boolean hasConfState() {
return confStateBuilder_ != null || confState_ != null;
}
/**
*
* The current `ConfState`.
*
*
* .eraftpb.ConfState conf_state = 1;
*/
public eraftpb.Eraftpb.ConfState getConfState() {
if (confStateBuilder_ == null) {
return confState_ == null ? eraftpb.Eraftpb.ConfState.getDefaultInstance() : confState_;
} else {
return confStateBuilder_.getMessage();
}
}
/**
*
* The current `ConfState`.
*
*
* .eraftpb.ConfState conf_state = 1;
*/
public Builder setConfState(eraftpb.Eraftpb.ConfState value) {
if (confStateBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
confState_ = value;
onChanged();
} else {
confStateBuilder_.setMessage(value);
}
return this;
}
/**
*
* The current `ConfState`.
*
*
* .eraftpb.ConfState conf_state = 1;
*/
public Builder setConfState(
eraftpb.Eraftpb.ConfState.Builder builderForValue) {
if (confStateBuilder_ == null) {
confState_ = builderForValue.build();
onChanged();
} else {
confStateBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
*
* The current `ConfState`.
*
*
* .eraftpb.ConfState conf_state = 1;
*/
public Builder mergeConfState(eraftpb.Eraftpb.ConfState value) {
if (confStateBuilder_ == null) {
if (confState_ != null) {
confState_ =
eraftpb.Eraftpb.ConfState.newBuilder(confState_).mergeFrom(value).buildPartial();
} else {
confState_ = value;
}
onChanged();
} else {
confStateBuilder_.mergeFrom(value);
}
return this;
}
/**
*
* The current `ConfState`.
*
*
* .eraftpb.ConfState conf_state = 1;
*/
public Builder clearConfState() {
if (confStateBuilder_ == null) {
confState_ = null;
onChanged();
} else {
confState_ = null;
confStateBuilder_ = null;
}
return this;
}
/**
*
* The current `ConfState`.
*
*
* .eraftpb.ConfState conf_state = 1;
*/
public eraftpb.Eraftpb.ConfState.Builder getConfStateBuilder() {
onChanged();
return getConfStateFieldBuilder().getBuilder();
}
/**
*
* The current `ConfState`.
*
*
* .eraftpb.ConfState conf_state = 1;
*/
public eraftpb.Eraftpb.ConfStateOrBuilder getConfStateOrBuilder() {
if (confStateBuilder_ != null) {
return confStateBuilder_.getMessageOrBuilder();
} else {
return confState_ == null ?
eraftpb.Eraftpb.ConfState.getDefaultInstance() : confState_;
}
}
/**
*
* The current `ConfState`.
*
*
* .eraftpb.ConfState conf_state = 1;
*/
private com.google.protobuf.SingleFieldBuilderV3<
eraftpb.Eraftpb.ConfState, eraftpb.Eraftpb.ConfState.Builder, eraftpb.Eraftpb.ConfStateOrBuilder>
getConfStateFieldBuilder() {
if (confStateBuilder_ == null) {
confStateBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
eraftpb.Eraftpb.ConfState, eraftpb.Eraftpb.ConfState.Builder, eraftpb.Eraftpb.ConfStateOrBuilder>(
getConfState(),
getParentForChildren(),
isClean());
confState_ = null;
}
return confStateBuilder_;
}
private long index_ ;
/**
*
* The applied index.
*
*
* uint64 index = 2;
*/
public long getIndex() {
return index_;
}
/**
*
* The applied index.
*
*
* uint64 index = 2;
*/
public Builder setIndex(long value) {
index_ = value;
onChanged();
return this;
}
/**
*
* The applied index.
*
*
* uint64 index = 2;
*/
public Builder clearIndex() {
index_ = 0L;
onChanged();
return this;
}
private long term_ ;
/**
*
* The term of the applied index.
*
*
* uint64 term = 3;
*/
public long getTerm() {
return term_;
}
/**
*
* The term of the applied index.
*
*
* uint64 term = 3;
*/
public Builder setTerm(long value) {
term_ = value;
onChanged();
return this;
}
/**
*
* The term of the applied index.
*
*
* uint64 term = 3;
*/
public Builder clearTerm() {
term_ = 0L;
onChanged();
return this;
}
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFieldsProto3(unknownFields);
}
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:eraftpb.SnapshotMetadata)
}
// @@protoc_insertion_point(class_scope:eraftpb.SnapshotMetadata)
private static final eraftpb.Eraftpb.SnapshotMetadata DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new eraftpb.Eraftpb.SnapshotMetadata();
}
public static eraftpb.Eraftpb.SnapshotMetadata getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
public SnapshotMetadata parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new SnapshotMetadata(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
public eraftpb.Eraftpb.SnapshotMetadata getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface SnapshotOrBuilder extends
// @@protoc_insertion_point(interface_extends:eraftpb.Snapshot)
com.google.protobuf.MessageOrBuilder {
/**
* bytes data = 1;
*/
com.google.protobuf.ByteString getData();
/**
* .eraftpb.SnapshotMetadata metadata = 2;
*/
boolean hasMetadata();
/**
* .eraftpb.SnapshotMetadata metadata = 2;
*/
eraftpb.Eraftpb.SnapshotMetadata getMetadata();
/**
* .eraftpb.SnapshotMetadata metadata = 2;
*/
eraftpb.Eraftpb.SnapshotMetadataOrBuilder getMetadataOrBuilder();
}
/**
* Protobuf type {@code eraftpb.Snapshot}
*/
public static final class Snapshot extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:eraftpb.Snapshot)
SnapshotOrBuilder {
private static final long serialVersionUID = 0L;
// Use Snapshot.newBuilder() to construct.
private Snapshot(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private Snapshot() {
data_ = com.google.protobuf.ByteString.EMPTY;
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private Snapshot(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
default: {
if (!parseUnknownFieldProto3(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
case 10: {
data_ = input.readBytes();
break;
}
case 18: {
eraftpb.Eraftpb.SnapshotMetadata.Builder subBuilder = null;
if (metadata_ != null) {
subBuilder = metadata_.toBuilder();
}
metadata_ = input.readMessage(eraftpb.Eraftpb.SnapshotMetadata.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(metadata_);
metadata_ = subBuilder.buildPartial();
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return eraftpb.Eraftpb.internal_static_eraftpb_Snapshot_descriptor;
}
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return eraftpb.Eraftpb.internal_static_eraftpb_Snapshot_fieldAccessorTable
.ensureFieldAccessorsInitialized(
eraftpb.Eraftpb.Snapshot.class, eraftpb.Eraftpb.Snapshot.Builder.class);
}
public static final int DATA_FIELD_NUMBER = 1;
private com.google.protobuf.ByteString data_;
/**
* bytes data = 1;
*/
public com.google.protobuf.ByteString getData() {
return data_;
}
public static final int METADATA_FIELD_NUMBER = 2;
private eraftpb.Eraftpb.SnapshotMetadata metadata_;
/**
* .eraftpb.SnapshotMetadata metadata = 2;
*/
public boolean hasMetadata() {
return metadata_ != null;
}
/**
* .eraftpb.SnapshotMetadata metadata = 2;
*/
public eraftpb.Eraftpb.SnapshotMetadata getMetadata() {
return metadata_ == null ? eraftpb.Eraftpb.SnapshotMetadata.getDefaultInstance() : metadata_;
}
/**
* .eraftpb.SnapshotMetadata metadata = 2;
*/
public eraftpb.Eraftpb.SnapshotMetadataOrBuilder getMetadataOrBuilder() {
return getMetadata();
}
private byte memoizedIsInitialized = -1;
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (!data_.isEmpty()) {
output.writeBytes(1, data_);
}
if (metadata_ != null) {
output.writeMessage(2, getMetadata());
}
unknownFields.writeTo(output);
}
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (!data_.isEmpty()) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(1, data_);
}
if (metadata_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(2, getMetadata());
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof eraftpb.Eraftpb.Snapshot)) {
return super.equals(obj);
}
eraftpb.Eraftpb.Snapshot other = (eraftpb.Eraftpb.Snapshot) obj;
boolean result = true;
result = result && getData()
.equals(other.getData());
result = result && (hasMetadata() == other.hasMetadata());
if (hasMetadata()) {
result = result && getMetadata()
.equals(other.getMetadata());
}
result = result && unknownFields.equals(other.unknownFields);
return result;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
hash = (37 * hash) + DATA_FIELD_NUMBER;
hash = (53 * hash) + getData().hashCode();
if (hasMetadata()) {
hash = (37 * hash) + METADATA_FIELD_NUMBER;
hash = (53 * hash) + getMetadata().hashCode();
}
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static eraftpb.Eraftpb.Snapshot parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static eraftpb.Eraftpb.Snapshot parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static eraftpb.Eraftpb.Snapshot parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static eraftpb.Eraftpb.Snapshot parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static eraftpb.Eraftpb.Snapshot parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static eraftpb.Eraftpb.Snapshot parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static eraftpb.Eraftpb.Snapshot parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static eraftpb.Eraftpb.Snapshot parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static eraftpb.Eraftpb.Snapshot parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static eraftpb.Eraftpb.Snapshot parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static eraftpb.Eraftpb.Snapshot parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static eraftpb.Eraftpb.Snapshot parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(eraftpb.Eraftpb.Snapshot prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code eraftpb.Snapshot}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:eraftpb.Snapshot)
eraftpb.Eraftpb.SnapshotOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return eraftpb.Eraftpb.internal_static_eraftpb_Snapshot_descriptor;
}
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return eraftpb.Eraftpb.internal_static_eraftpb_Snapshot_fieldAccessorTable
.ensureFieldAccessorsInitialized(
eraftpb.Eraftpb.Snapshot.class, eraftpb.Eraftpb.Snapshot.Builder.class);
}
// Construct using eraftpb.Eraftpb.Snapshot.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
public Builder clear() {
super.clear();
data_ = com.google.protobuf.ByteString.EMPTY;
if (metadataBuilder_ == null) {
metadata_ = null;
} else {
metadata_ = null;
metadataBuilder_ = null;
}
return this;
}
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return eraftpb.Eraftpb.internal_static_eraftpb_Snapshot_descriptor;
}
public eraftpb.Eraftpb.Snapshot getDefaultInstanceForType() {
return eraftpb.Eraftpb.Snapshot.getDefaultInstance();
}
public eraftpb.Eraftpb.Snapshot build() {
eraftpb.Eraftpb.Snapshot result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
public eraftpb.Eraftpb.Snapshot buildPartial() {
eraftpb.Eraftpb.Snapshot result = new eraftpb.Eraftpb.Snapshot(this);
result.data_ = data_;
if (metadataBuilder_ == null) {
result.metadata_ = metadata_;
} else {
result.metadata_ = metadataBuilder_.build();
}
onBuilt();
return result;
}
public Builder clone() {
return (Builder) super.clone();
}
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.setField(field, value);
}
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return (Builder) super.clearField(field);
}
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return (Builder) super.clearOneof(oneof);
}
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return (Builder) super.setRepeatedField(field, index, value);
}
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.addRepeatedField(field, value);
}
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof eraftpb.Eraftpb.Snapshot) {
return mergeFrom((eraftpb.Eraftpb.Snapshot)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(eraftpb.Eraftpb.Snapshot other) {
if (other == eraftpb.Eraftpb.Snapshot.getDefaultInstance()) return this;
if (other.getData() != com.google.protobuf.ByteString.EMPTY) {
setData(other.getData());
}
if (other.hasMetadata()) {
mergeMetadata(other.getMetadata());
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
public final boolean isInitialized() {
return true;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
eraftpb.Eraftpb.Snapshot parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (eraftpb.Eraftpb.Snapshot) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private com.google.protobuf.ByteString data_ = com.google.protobuf.ByteString.EMPTY;
/**
* bytes data = 1;
*/
public com.google.protobuf.ByteString getData() {
return data_;
}
/**
* bytes data = 1;
*/
public Builder setData(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
data_ = value;
onChanged();
return this;
}
/**
* bytes data = 1;
*/
public Builder clearData() {
data_ = getDefaultInstance().getData();
onChanged();
return this;
}
private eraftpb.Eraftpb.SnapshotMetadata metadata_ = null;
private com.google.protobuf.SingleFieldBuilderV3<
eraftpb.Eraftpb.SnapshotMetadata, eraftpb.Eraftpb.SnapshotMetadata.Builder, eraftpb.Eraftpb.SnapshotMetadataOrBuilder> metadataBuilder_;
/**
* .eraftpb.SnapshotMetadata metadata = 2;
*/
public boolean hasMetadata() {
return metadataBuilder_ != null || metadata_ != null;
}
/**
* .eraftpb.SnapshotMetadata metadata = 2;
*/
public eraftpb.Eraftpb.SnapshotMetadata getMetadata() {
if (metadataBuilder_ == null) {
return metadata_ == null ? eraftpb.Eraftpb.SnapshotMetadata.getDefaultInstance() : metadata_;
} else {
return metadataBuilder_.getMessage();
}
}
/**
* .eraftpb.SnapshotMetadata metadata = 2;
*/
public Builder setMetadata(eraftpb.Eraftpb.SnapshotMetadata value) {
if (metadataBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
metadata_ = value;
onChanged();
} else {
metadataBuilder_.setMessage(value);
}
return this;
}
/**
* .eraftpb.SnapshotMetadata metadata = 2;
*/
public Builder setMetadata(
eraftpb.Eraftpb.SnapshotMetadata.Builder builderForValue) {
if (metadataBuilder_ == null) {
metadata_ = builderForValue.build();
onChanged();
} else {
metadataBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
* .eraftpb.SnapshotMetadata metadata = 2;
*/
public Builder mergeMetadata(eraftpb.Eraftpb.SnapshotMetadata value) {
if (metadataBuilder_ == null) {
if (metadata_ != null) {
metadata_ =
eraftpb.Eraftpb.SnapshotMetadata.newBuilder(metadata_).mergeFrom(value).buildPartial();
} else {
metadata_ = value;
}
onChanged();
} else {
metadataBuilder_.mergeFrom(value);
}
return this;
}
/**
* .eraftpb.SnapshotMetadata metadata = 2;
*/
public Builder clearMetadata() {
if (metadataBuilder_ == null) {
metadata_ = null;
onChanged();
} else {
metadata_ = null;
metadataBuilder_ = null;
}
return this;
}
/**
* .eraftpb.SnapshotMetadata metadata = 2;
*/
public eraftpb.Eraftpb.SnapshotMetadata.Builder getMetadataBuilder() {
onChanged();
return getMetadataFieldBuilder().getBuilder();
}
/**
* .eraftpb.SnapshotMetadata metadata = 2;
*/
public eraftpb.Eraftpb.SnapshotMetadataOrBuilder getMetadataOrBuilder() {
if (metadataBuilder_ != null) {
return metadataBuilder_.getMessageOrBuilder();
} else {
return metadata_ == null ?
eraftpb.Eraftpb.SnapshotMetadata.getDefaultInstance() : metadata_;
}
}
/**
* .eraftpb.SnapshotMetadata metadata = 2;
*/
private com.google.protobuf.SingleFieldBuilderV3<
eraftpb.Eraftpb.SnapshotMetadata, eraftpb.Eraftpb.SnapshotMetadata.Builder, eraftpb.Eraftpb.SnapshotMetadataOrBuilder>
getMetadataFieldBuilder() {
if (metadataBuilder_ == null) {
metadataBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
eraftpb.Eraftpb.SnapshotMetadata, eraftpb.Eraftpb.SnapshotMetadata.Builder, eraftpb.Eraftpb.SnapshotMetadataOrBuilder>(
getMetadata(),
getParentForChildren(),
isClean());
metadata_ = null;
}
return metadataBuilder_;
}
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFieldsProto3(unknownFields);
}
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:eraftpb.Snapshot)
}
// @@protoc_insertion_point(class_scope:eraftpb.Snapshot)
private static final eraftpb.Eraftpb.Snapshot DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new eraftpb.Eraftpb.Snapshot();
}
public static eraftpb.Eraftpb.Snapshot getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
public Snapshot parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new Snapshot(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
public eraftpb.Eraftpb.Snapshot getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface MessageOrBuilder extends
// @@protoc_insertion_point(interface_extends:eraftpb.Message)
com.google.protobuf.MessageOrBuilder {
/**
* .eraftpb.MessageType msg_type = 1;
*/
int getMsgTypeValue();
/**
* .eraftpb.MessageType msg_type = 1;
*/
eraftpb.Eraftpb.MessageType getMsgType();
/**
* uint64 to = 2;
*/
long getTo();
/**
* uint64 from = 3;
*/
long getFrom();
/**
* uint64 term = 4;
*/
long getTerm();
/**
* uint64 log_term = 5;
*/
long getLogTerm();
/**
* uint64 index = 6;
*/
long getIndex();
/**
* repeated .eraftpb.Entry entries = 7;
*/
java.util.List
getEntriesList();
/**
* repeated .eraftpb.Entry entries = 7;
*/
eraftpb.Eraftpb.Entry getEntries(int index);
/**
* repeated .eraftpb.Entry entries = 7;
*/
int getEntriesCount();
/**
* repeated .eraftpb.Entry entries = 7;
*/
java.util.List extends eraftpb.Eraftpb.EntryOrBuilder>
getEntriesOrBuilderList();
/**
* repeated .eraftpb.Entry entries = 7;
*/
eraftpb.Eraftpb.EntryOrBuilder getEntriesOrBuilder(
int index);
/**
* uint64 commit = 8;
*/
long getCommit();
/**
* .eraftpb.Snapshot snapshot = 9;
*/
boolean hasSnapshot();
/**
* .eraftpb.Snapshot snapshot = 9;
*/
eraftpb.Eraftpb.Snapshot getSnapshot();
/**
* .eraftpb.Snapshot snapshot = 9;
*/
eraftpb.Eraftpb.SnapshotOrBuilder getSnapshotOrBuilder();
/**
* uint64 request_snapshot = 13;
*/
long getRequestSnapshot();
/**
* bool reject = 10;
*/
boolean getReject();
/**
* uint64 reject_hint = 11;
*/
long getRejectHint();
/**
* bytes context = 12;
*/
com.google.protobuf.ByteString getContext();
}
/**
* Protobuf type {@code eraftpb.Message}
*/
public static final class Message extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:eraftpb.Message)
MessageOrBuilder {
private static final long serialVersionUID = 0L;
// Use Message.newBuilder() to construct.
private Message(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private Message() {
msgType_ = 0;
to_ = 0L;
from_ = 0L;
term_ = 0L;
logTerm_ = 0L;
index_ = 0L;
entries_ = java.util.Collections.emptyList();
commit_ = 0L;
requestSnapshot_ = 0L;
reject_ = false;
rejectHint_ = 0L;
context_ = com.google.protobuf.ByteString.EMPTY;
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private Message(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
default: {
if (!parseUnknownFieldProto3(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
case 8: {
int rawValue = input.readEnum();
msgType_ = rawValue;
break;
}
case 16: {
to_ = input.readUInt64();
break;
}
case 24: {
from_ = input.readUInt64();
break;
}
case 32: {
term_ = input.readUInt64();
break;
}
case 40: {
logTerm_ = input.readUInt64();
break;
}
case 48: {
index_ = input.readUInt64();
break;
}
case 58: {
if (!((mutable_bitField0_ & 0x00000040) == 0x00000040)) {
entries_ = new java.util.ArrayList();
mutable_bitField0_ |= 0x00000040;
}
entries_.add(
input.readMessage(eraftpb.Eraftpb.Entry.parser(), extensionRegistry));
break;
}
case 64: {
commit_ = input.readUInt64();
break;
}
case 74: {
eraftpb.Eraftpb.Snapshot.Builder subBuilder = null;
if (snapshot_ != null) {
subBuilder = snapshot_.toBuilder();
}
snapshot_ = input.readMessage(eraftpb.Eraftpb.Snapshot.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(snapshot_);
snapshot_ = subBuilder.buildPartial();
}
break;
}
case 80: {
reject_ = input.readBool();
break;
}
case 88: {
rejectHint_ = input.readUInt64();
break;
}
case 98: {
context_ = input.readBytes();
break;
}
case 104: {
requestSnapshot_ = input.readUInt64();
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
if (((mutable_bitField0_ & 0x00000040) == 0x00000040)) {
entries_ = java.util.Collections.unmodifiableList(entries_);
}
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return eraftpb.Eraftpb.internal_static_eraftpb_Message_descriptor;
}
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return eraftpb.Eraftpb.internal_static_eraftpb_Message_fieldAccessorTable
.ensureFieldAccessorsInitialized(
eraftpb.Eraftpb.Message.class, eraftpb.Eraftpb.Message.Builder.class);
}
private int bitField0_;
public static final int MSG_TYPE_FIELD_NUMBER = 1;
private int msgType_;
/**
* .eraftpb.MessageType msg_type = 1;
*/
public int getMsgTypeValue() {
return msgType_;
}
/**
* .eraftpb.MessageType msg_type = 1;
*/
public eraftpb.Eraftpb.MessageType getMsgType() {
eraftpb.Eraftpb.MessageType result = eraftpb.Eraftpb.MessageType.valueOf(msgType_);
return result == null ? eraftpb.Eraftpb.MessageType.UNRECOGNIZED : result;
}
public static final int TO_FIELD_NUMBER = 2;
private long to_;
/**
* uint64 to = 2;
*/
public long getTo() {
return to_;
}
public static final int FROM_FIELD_NUMBER = 3;
private long from_;
/**
* uint64 from = 3;
*/
public long getFrom() {
return from_;
}
public static final int TERM_FIELD_NUMBER = 4;
private long term_;
/**
* uint64 term = 4;
*/
public long getTerm() {
return term_;
}
public static final int LOG_TERM_FIELD_NUMBER = 5;
private long logTerm_;
/**
* uint64 log_term = 5;
*/
public long getLogTerm() {
return logTerm_;
}
public static final int INDEX_FIELD_NUMBER = 6;
private long index_;
/**
* uint64 index = 6;
*/
public long getIndex() {
return index_;
}
public static final int ENTRIES_FIELD_NUMBER = 7;
private java.util.List entries_;
/**
* repeated .eraftpb.Entry entries = 7;
*/
public java.util.List getEntriesList() {
return entries_;
}
/**
* repeated .eraftpb.Entry entries = 7;
*/
public java.util.List extends eraftpb.Eraftpb.EntryOrBuilder>
getEntriesOrBuilderList() {
return entries_;
}
/**
* repeated .eraftpb.Entry entries = 7;
*/
public int getEntriesCount() {
return entries_.size();
}
/**
* repeated .eraftpb.Entry entries = 7;
*/
public eraftpb.Eraftpb.Entry getEntries(int index) {
return entries_.get(index);
}
/**
* repeated .eraftpb.Entry entries = 7;
*/
public eraftpb.Eraftpb.EntryOrBuilder getEntriesOrBuilder(
int index) {
return entries_.get(index);
}
public static final int COMMIT_FIELD_NUMBER = 8;
private long commit_;
/**
* uint64 commit = 8;
*/
public long getCommit() {
return commit_;
}
public static final int SNAPSHOT_FIELD_NUMBER = 9;
private eraftpb.Eraftpb.Snapshot snapshot_;
/**
* .eraftpb.Snapshot snapshot = 9;
*/
public boolean hasSnapshot() {
return snapshot_ != null;
}
/**
* .eraftpb.Snapshot snapshot = 9;
*/
public eraftpb.Eraftpb.Snapshot getSnapshot() {
return snapshot_ == null ? eraftpb.Eraftpb.Snapshot.getDefaultInstance() : snapshot_;
}
/**
* .eraftpb.Snapshot snapshot = 9;
*/
public eraftpb.Eraftpb.SnapshotOrBuilder getSnapshotOrBuilder() {
return getSnapshot();
}
public static final int REQUEST_SNAPSHOT_FIELD_NUMBER = 13;
private long requestSnapshot_;
/**
* uint64 request_snapshot = 13;
*/
public long getRequestSnapshot() {
return requestSnapshot_;
}
public static final int REJECT_FIELD_NUMBER = 10;
private boolean reject_;
/**
* bool reject = 10;
*/
public boolean getReject() {
return reject_;
}
public static final int REJECT_HINT_FIELD_NUMBER = 11;
private long rejectHint_;
/**
* uint64 reject_hint = 11;
*/
public long getRejectHint() {
return rejectHint_;
}
public static final int CONTEXT_FIELD_NUMBER = 12;
private com.google.protobuf.ByteString context_;
/**
* bytes context = 12;
*/
public com.google.protobuf.ByteString getContext() {
return context_;
}
private byte memoizedIsInitialized = -1;
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (msgType_ != eraftpb.Eraftpb.MessageType.MsgHup.getNumber()) {
output.writeEnum(1, msgType_);
}
if (to_ != 0L) {
output.writeUInt64(2, to_);
}
if (from_ != 0L) {
output.writeUInt64(3, from_);
}
if (term_ != 0L) {
output.writeUInt64(4, term_);
}
if (logTerm_ != 0L) {
output.writeUInt64(5, logTerm_);
}
if (index_ != 0L) {
output.writeUInt64(6, index_);
}
for (int i = 0; i < entries_.size(); i++) {
output.writeMessage(7, entries_.get(i));
}
if (commit_ != 0L) {
output.writeUInt64(8, commit_);
}
if (snapshot_ != null) {
output.writeMessage(9, getSnapshot());
}
if (reject_ != false) {
output.writeBool(10, reject_);
}
if (rejectHint_ != 0L) {
output.writeUInt64(11, rejectHint_);
}
if (!context_.isEmpty()) {
output.writeBytes(12, context_);
}
if (requestSnapshot_ != 0L) {
output.writeUInt64(13, requestSnapshot_);
}
unknownFields.writeTo(output);
}
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (msgType_ != eraftpb.Eraftpb.MessageType.MsgHup.getNumber()) {
size += com.google.protobuf.CodedOutputStream
.computeEnumSize(1, msgType_);
}
if (to_ != 0L) {
size += com.google.protobuf.CodedOutputStream
.computeUInt64Size(2, to_);
}
if (from_ != 0L) {
size += com.google.protobuf.CodedOutputStream
.computeUInt64Size(3, from_);
}
if (term_ != 0L) {
size += com.google.protobuf.CodedOutputStream
.computeUInt64Size(4, term_);
}
if (logTerm_ != 0L) {
size += com.google.protobuf.CodedOutputStream
.computeUInt64Size(5, logTerm_);
}
if (index_ != 0L) {
size += com.google.protobuf.CodedOutputStream
.computeUInt64Size(6, index_);
}
for (int i = 0; i < entries_.size(); i++) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(7, entries_.get(i));
}
if (commit_ != 0L) {
size += com.google.protobuf.CodedOutputStream
.computeUInt64Size(8, commit_);
}
if (snapshot_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(9, getSnapshot());
}
if (reject_ != false) {
size += com.google.protobuf.CodedOutputStream
.computeBoolSize(10, reject_);
}
if (rejectHint_ != 0L) {
size += com.google.protobuf.CodedOutputStream
.computeUInt64Size(11, rejectHint_);
}
if (!context_.isEmpty()) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(12, context_);
}
if (requestSnapshot_ != 0L) {
size += com.google.protobuf.CodedOutputStream
.computeUInt64Size(13, requestSnapshot_);
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof eraftpb.Eraftpb.Message)) {
return super.equals(obj);
}
eraftpb.Eraftpb.Message other = (eraftpb.Eraftpb.Message) obj;
boolean result = true;
result = result && msgType_ == other.msgType_;
result = result && (getTo()
== other.getTo());
result = result && (getFrom()
== other.getFrom());
result = result && (getTerm()
== other.getTerm());
result = result && (getLogTerm()
== other.getLogTerm());
result = result && (getIndex()
== other.getIndex());
result = result && getEntriesList()
.equals(other.getEntriesList());
result = result && (getCommit()
== other.getCommit());
result = result && (hasSnapshot() == other.hasSnapshot());
if (hasSnapshot()) {
result = result && getSnapshot()
.equals(other.getSnapshot());
}
result = result && (getRequestSnapshot()
== other.getRequestSnapshot());
result = result && (getReject()
== other.getReject());
result = result && (getRejectHint()
== other.getRejectHint());
result = result && getContext()
.equals(other.getContext());
result = result && unknownFields.equals(other.unknownFields);
return result;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
hash = (37 * hash) + MSG_TYPE_FIELD_NUMBER;
hash = (53 * hash) + msgType_;
hash = (37 * hash) + TO_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
getTo());
hash = (37 * hash) + FROM_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
getFrom());
hash = (37 * hash) + TERM_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
getTerm());
hash = (37 * hash) + LOG_TERM_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
getLogTerm());
hash = (37 * hash) + INDEX_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
getIndex());
if (getEntriesCount() > 0) {
hash = (37 * hash) + ENTRIES_FIELD_NUMBER;
hash = (53 * hash) + getEntriesList().hashCode();
}
hash = (37 * hash) + COMMIT_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
getCommit());
if (hasSnapshot()) {
hash = (37 * hash) + SNAPSHOT_FIELD_NUMBER;
hash = (53 * hash) + getSnapshot().hashCode();
}
hash = (37 * hash) + REQUEST_SNAPSHOT_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
getRequestSnapshot());
hash = (37 * hash) + REJECT_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashBoolean(
getReject());
hash = (37 * hash) + REJECT_HINT_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
getRejectHint());
hash = (37 * hash) + CONTEXT_FIELD_NUMBER;
hash = (53 * hash) + getContext().hashCode();
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static eraftpb.Eraftpb.Message parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static eraftpb.Eraftpb.Message parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static eraftpb.Eraftpb.Message parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static eraftpb.Eraftpb.Message parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static eraftpb.Eraftpb.Message parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static eraftpb.Eraftpb.Message parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static eraftpb.Eraftpb.Message parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static eraftpb.Eraftpb.Message parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static eraftpb.Eraftpb.Message parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static eraftpb.Eraftpb.Message parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static eraftpb.Eraftpb.Message parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static eraftpb.Eraftpb.Message parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(eraftpb.Eraftpb.Message prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code eraftpb.Message}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:eraftpb.Message)
eraftpb.Eraftpb.MessageOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return eraftpb.Eraftpb.internal_static_eraftpb_Message_descriptor;
}
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return eraftpb.Eraftpb.internal_static_eraftpb_Message_fieldAccessorTable
.ensureFieldAccessorsInitialized(
eraftpb.Eraftpb.Message.class, eraftpb.Eraftpb.Message.Builder.class);
}
// Construct using eraftpb.Eraftpb.Message.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
getEntriesFieldBuilder();
}
}
public Builder clear() {
super.clear();
msgType_ = 0;
to_ = 0L;
from_ = 0L;
term_ = 0L;
logTerm_ = 0L;
index_ = 0L;
if (entriesBuilder_ == null) {
entries_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000040);
} else {
entriesBuilder_.clear();
}
commit_ = 0L;
if (snapshotBuilder_ == null) {
snapshot_ = null;
} else {
snapshot_ = null;
snapshotBuilder_ = null;
}
requestSnapshot_ = 0L;
reject_ = false;
rejectHint_ = 0L;
context_ = com.google.protobuf.ByteString.EMPTY;
return this;
}
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return eraftpb.Eraftpb.internal_static_eraftpb_Message_descriptor;
}
public eraftpb.Eraftpb.Message getDefaultInstanceForType() {
return eraftpb.Eraftpb.Message.getDefaultInstance();
}
public eraftpb.Eraftpb.Message build() {
eraftpb.Eraftpb.Message result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
public eraftpb.Eraftpb.Message buildPartial() {
eraftpb.Eraftpb.Message result = new eraftpb.Eraftpb.Message(this);
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
result.msgType_ = msgType_;
result.to_ = to_;
result.from_ = from_;
result.term_ = term_;
result.logTerm_ = logTerm_;
result.index_ = index_;
if (entriesBuilder_ == null) {
if (((bitField0_ & 0x00000040) == 0x00000040)) {
entries_ = java.util.Collections.unmodifiableList(entries_);
bitField0_ = (bitField0_ & ~0x00000040);
}
result.entries_ = entries_;
} else {
result.entries_ = entriesBuilder_.build();
}
result.commit_ = commit_;
if (snapshotBuilder_ == null) {
result.snapshot_ = snapshot_;
} else {
result.snapshot_ = snapshotBuilder_.build();
}
result.requestSnapshot_ = requestSnapshot_;
result.reject_ = reject_;
result.rejectHint_ = rejectHint_;
result.context_ = context_;
result.bitField0_ = to_bitField0_;
onBuilt();
return result;
}
public Builder clone() {
return (Builder) super.clone();
}
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.setField(field, value);
}
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return (Builder) super.clearField(field);
}
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return (Builder) super.clearOneof(oneof);
}
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return (Builder) super.setRepeatedField(field, index, value);
}
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.addRepeatedField(field, value);
}
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof eraftpb.Eraftpb.Message) {
return mergeFrom((eraftpb.Eraftpb.Message)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(eraftpb.Eraftpb.Message other) {
if (other == eraftpb.Eraftpb.Message.getDefaultInstance()) return this;
if (other.msgType_ != 0) {
setMsgTypeValue(other.getMsgTypeValue());
}
if (other.getTo() != 0L) {
setTo(other.getTo());
}
if (other.getFrom() != 0L) {
setFrom(other.getFrom());
}
if (other.getTerm() != 0L) {
setTerm(other.getTerm());
}
if (other.getLogTerm() != 0L) {
setLogTerm(other.getLogTerm());
}
if (other.getIndex() != 0L) {
setIndex(other.getIndex());
}
if (entriesBuilder_ == null) {
if (!other.entries_.isEmpty()) {
if (entries_.isEmpty()) {
entries_ = other.entries_;
bitField0_ = (bitField0_ & ~0x00000040);
} else {
ensureEntriesIsMutable();
entries_.addAll(other.entries_);
}
onChanged();
}
} else {
if (!other.entries_.isEmpty()) {
if (entriesBuilder_.isEmpty()) {
entriesBuilder_.dispose();
entriesBuilder_ = null;
entries_ = other.entries_;
bitField0_ = (bitField0_ & ~0x00000040);
entriesBuilder_ =
com.google.protobuf.GeneratedMessageV3.alwaysUseFieldBuilders ?
getEntriesFieldBuilder() : null;
} else {
entriesBuilder_.addAllMessages(other.entries_);
}
}
}
if (other.getCommit() != 0L) {
setCommit(other.getCommit());
}
if (other.hasSnapshot()) {
mergeSnapshot(other.getSnapshot());
}
if (other.getRequestSnapshot() != 0L) {
setRequestSnapshot(other.getRequestSnapshot());
}
if (other.getReject() != false) {
setReject(other.getReject());
}
if (other.getRejectHint() != 0L) {
setRejectHint(other.getRejectHint());
}
if (other.getContext() != com.google.protobuf.ByteString.EMPTY) {
setContext(other.getContext());
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
public final boolean isInitialized() {
return true;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
eraftpb.Eraftpb.Message parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (eraftpb.Eraftpb.Message) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
private int msgType_ = 0;
/**
* .eraftpb.MessageType msg_type = 1;
*/
public int getMsgTypeValue() {
return msgType_;
}
/**
* .eraftpb.MessageType msg_type = 1;
*/
public Builder setMsgTypeValue(int value) {
msgType_ = value;
onChanged();
return this;
}
/**
* .eraftpb.MessageType msg_type = 1;
*/
public eraftpb.Eraftpb.MessageType getMsgType() {
eraftpb.Eraftpb.MessageType result = eraftpb.Eraftpb.MessageType.valueOf(msgType_);
return result == null ? eraftpb.Eraftpb.MessageType.UNRECOGNIZED : result;
}
/**
* .eraftpb.MessageType msg_type = 1;
*/
public Builder setMsgType(eraftpb.Eraftpb.MessageType value) {
if (value == null) {
throw new NullPointerException();
}
msgType_ = value.getNumber();
onChanged();
return this;
}
/**
* .eraftpb.MessageType msg_type = 1;
*/
public Builder clearMsgType() {
msgType_ = 0;
onChanged();
return this;
}
private long to_ ;
/**
* uint64 to = 2;
*/
public long getTo() {
return to_;
}
/**
* uint64 to = 2;
*/
public Builder setTo(long value) {
to_ = value;
onChanged();
return this;
}
/**
* uint64 to = 2;
*/
public Builder clearTo() {
to_ = 0L;
onChanged();
return this;
}
private long from_ ;
/**
* uint64 from = 3;
*/
public long getFrom() {
return from_;
}
/**
* uint64 from = 3;
*/
public Builder setFrom(long value) {
from_ = value;
onChanged();
return this;
}
/**
* uint64 from = 3;
*/
public Builder clearFrom() {
from_ = 0L;
onChanged();
return this;
}
private long term_ ;
/**
* uint64 term = 4;
*/
public long getTerm() {
return term_;
}
/**
* uint64 term = 4;
*/
public Builder setTerm(long value) {
term_ = value;
onChanged();
return this;
}
/**
* uint64 term = 4;
*/
public Builder clearTerm() {
term_ = 0L;
onChanged();
return this;
}
private long logTerm_ ;
/**
* uint64 log_term = 5;
*/
public long getLogTerm() {
return logTerm_;
}
/**
* uint64 log_term = 5;
*/
public Builder setLogTerm(long value) {
logTerm_ = value;
onChanged();
return this;
}
/**
* uint64 log_term = 5;
*/
public Builder clearLogTerm() {
logTerm_ = 0L;
onChanged();
return this;
}
private long index_ ;
/**
* uint64 index = 6;
*/
public long getIndex() {
return index_;
}
/**
* uint64 index = 6;
*/
public Builder setIndex(long value) {
index_ = value;
onChanged();
return this;
}
/**
* uint64 index = 6;
*/
public Builder clearIndex() {
index_ = 0L;
onChanged();
return this;
}
private java.util.List entries_ =
java.util.Collections.emptyList();
private void ensureEntriesIsMutable() {
if (!((bitField0_ & 0x00000040) == 0x00000040)) {
entries_ = new java.util.ArrayList(entries_);
bitField0_ |= 0x00000040;
}
}
private com.google.protobuf.RepeatedFieldBuilderV3<
eraftpb.Eraftpb.Entry, eraftpb.Eraftpb.Entry.Builder, eraftpb.Eraftpb.EntryOrBuilder> entriesBuilder_;
/**
* repeated .eraftpb.Entry entries = 7;
*/
public java.util.List getEntriesList() {
if (entriesBuilder_ == null) {
return java.util.Collections.unmodifiableList(entries_);
} else {
return entriesBuilder_.getMessageList();
}
}
/**
* repeated .eraftpb.Entry entries = 7;
*/
public int getEntriesCount() {
if (entriesBuilder_ == null) {
return entries_.size();
} else {
return entriesBuilder_.getCount();
}
}
/**
* repeated .eraftpb.Entry entries = 7;
*/
public eraftpb.Eraftpb.Entry getEntries(int index) {
if (entriesBuilder_ == null) {
return entries_.get(index);
} else {
return entriesBuilder_.getMessage(index);
}
}
/**
* repeated .eraftpb.Entry entries = 7;
*/
public Builder setEntries(
int index, eraftpb.Eraftpb.Entry value) {
if (entriesBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureEntriesIsMutable();
entries_.set(index, value);
onChanged();
} else {
entriesBuilder_.setMessage(index, value);
}
return this;
}
/**
* repeated .eraftpb.Entry entries = 7;
*/
public Builder setEntries(
int index, eraftpb.Eraftpb.Entry.Builder builderForValue) {
if (entriesBuilder_ == null) {
ensureEntriesIsMutable();
entries_.set(index, builderForValue.build());
onChanged();
} else {
entriesBuilder_.setMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .eraftpb.Entry entries = 7;
*/
public Builder addEntries(eraftpb.Eraftpb.Entry value) {
if (entriesBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureEntriesIsMutable();
entries_.add(value);
onChanged();
} else {
entriesBuilder_.addMessage(value);
}
return this;
}
/**
* repeated .eraftpb.Entry entries = 7;
*/
public Builder addEntries(
int index, eraftpb.Eraftpb.Entry value) {
if (entriesBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureEntriesIsMutable();
entries_.add(index, value);
onChanged();
} else {
entriesBuilder_.addMessage(index, value);
}
return this;
}
/**
* repeated .eraftpb.Entry entries = 7;
*/
public Builder addEntries(
eraftpb.Eraftpb.Entry.Builder builderForValue) {
if (entriesBuilder_ == null) {
ensureEntriesIsMutable();
entries_.add(builderForValue.build());
onChanged();
} else {
entriesBuilder_.addMessage(builderForValue.build());
}
return this;
}
/**
* repeated .eraftpb.Entry entries = 7;
*/
public Builder addEntries(
int index, eraftpb.Eraftpb.Entry.Builder builderForValue) {
if (entriesBuilder_ == null) {
ensureEntriesIsMutable();
entries_.add(index, builderForValue.build());
onChanged();
} else {
entriesBuilder_.addMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .eraftpb.Entry entries = 7;
*/
public Builder addAllEntries(
java.lang.Iterable extends eraftpb.Eraftpb.Entry> values) {
if (entriesBuilder_ == null) {
ensureEntriesIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, entries_);
onChanged();
} else {
entriesBuilder_.addAllMessages(values);
}
return this;
}
/**
* repeated .eraftpb.Entry entries = 7;
*/
public Builder clearEntries() {
if (entriesBuilder_ == null) {
entries_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000040);
onChanged();
} else {
entriesBuilder_.clear();
}
return this;
}
/**
* repeated .eraftpb.Entry entries = 7;
*/
public Builder removeEntries(int index) {
if (entriesBuilder_ == null) {
ensureEntriesIsMutable();
entries_.remove(index);
onChanged();
} else {
entriesBuilder_.remove(index);
}
return this;
}
/**
* repeated .eraftpb.Entry entries = 7;
*/
public eraftpb.Eraftpb.Entry.Builder getEntriesBuilder(
int index) {
return getEntriesFieldBuilder().getBuilder(index);
}
/**
* repeated .eraftpb.Entry entries = 7;
*/
public eraftpb.Eraftpb.EntryOrBuilder getEntriesOrBuilder(
int index) {
if (entriesBuilder_ == null) {
return entries_.get(index); } else {
return entriesBuilder_.getMessageOrBuilder(index);
}
}
/**
* repeated .eraftpb.Entry entries = 7;
*/
public java.util.List extends eraftpb.Eraftpb.EntryOrBuilder>
getEntriesOrBuilderList() {
if (entriesBuilder_ != null) {
return entriesBuilder_.getMessageOrBuilderList();
} else {
return java.util.Collections.unmodifiableList(entries_);
}
}
/**
* repeated .eraftpb.Entry entries = 7;
*/
public eraftpb.Eraftpb.Entry.Builder addEntriesBuilder() {
return getEntriesFieldBuilder().addBuilder(
eraftpb.Eraftpb.Entry.getDefaultInstance());
}
/**
* repeated .eraftpb.Entry entries = 7;
*/
public eraftpb.Eraftpb.Entry.Builder addEntriesBuilder(
int index) {
return getEntriesFieldBuilder().addBuilder(
index, eraftpb.Eraftpb.Entry.getDefaultInstance());
}
/**
* repeated .eraftpb.Entry entries = 7;
*/
public java.util.List
getEntriesBuilderList() {
return getEntriesFieldBuilder().getBuilderList();
}
private com.google.protobuf.RepeatedFieldBuilderV3<
eraftpb.Eraftpb.Entry, eraftpb.Eraftpb.Entry.Builder, eraftpb.Eraftpb.EntryOrBuilder>
getEntriesFieldBuilder() {
if (entriesBuilder_ == null) {
entriesBuilder_ = new com.google.protobuf.RepeatedFieldBuilderV3<
eraftpb.Eraftpb.Entry, eraftpb.Eraftpb.Entry.Builder, eraftpb.Eraftpb.EntryOrBuilder>(
entries_,
((bitField0_ & 0x00000040) == 0x00000040),
getParentForChildren(),
isClean());
entries_ = null;
}
return entriesBuilder_;
}
private long commit_ ;
/**
* uint64 commit = 8;
*/
public long getCommit() {
return commit_;
}
/**
* uint64 commit = 8;
*/
public Builder setCommit(long value) {
commit_ = value;
onChanged();
return this;
}
/**
* uint64 commit = 8;
*/
public Builder clearCommit() {
commit_ = 0L;
onChanged();
return this;
}
private eraftpb.Eraftpb.Snapshot snapshot_ = null;
private com.google.protobuf.SingleFieldBuilderV3<
eraftpb.Eraftpb.Snapshot, eraftpb.Eraftpb.Snapshot.Builder, eraftpb.Eraftpb.SnapshotOrBuilder> snapshotBuilder_;
/**
* .eraftpb.Snapshot snapshot = 9;
*/
public boolean hasSnapshot() {
return snapshotBuilder_ != null || snapshot_ != null;
}
/**
* .eraftpb.Snapshot snapshot = 9;
*/
public eraftpb.Eraftpb.Snapshot getSnapshot() {
if (snapshotBuilder_ == null) {
return snapshot_ == null ? eraftpb.Eraftpb.Snapshot.getDefaultInstance() : snapshot_;
} else {
return snapshotBuilder_.getMessage();
}
}
/**
* .eraftpb.Snapshot snapshot = 9;
*/
public Builder setSnapshot(eraftpb.Eraftpb.Snapshot value) {
if (snapshotBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
snapshot_ = value;
onChanged();
} else {
snapshotBuilder_.setMessage(value);
}
return this;
}
/**
* .eraftpb.Snapshot snapshot = 9;
*/
public Builder setSnapshot(
eraftpb.Eraftpb.Snapshot.Builder builderForValue) {
if (snapshotBuilder_ == null) {
snapshot_ = builderForValue.build();
onChanged();
} else {
snapshotBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
* .eraftpb.Snapshot snapshot = 9;
*/
public Builder mergeSnapshot(eraftpb.Eraftpb.Snapshot value) {
if (snapshotBuilder_ == null) {
if (snapshot_ != null) {
snapshot_ =
eraftpb.Eraftpb.Snapshot.newBuilder(snapshot_).mergeFrom(value).buildPartial();
} else {
snapshot_ = value;
}
onChanged();
} else {
snapshotBuilder_.mergeFrom(value);
}
return this;
}
/**
* .eraftpb.Snapshot snapshot = 9;
*/
public Builder clearSnapshot() {
if (snapshotBuilder_ == null) {
snapshot_ = null;
onChanged();
} else {
snapshot_ = null;
snapshotBuilder_ = null;
}
return this;
}
/**
* .eraftpb.Snapshot snapshot = 9;
*/
public eraftpb.Eraftpb.Snapshot.Builder getSnapshotBuilder() {
onChanged();
return getSnapshotFieldBuilder().getBuilder();
}
/**
* .eraftpb.Snapshot snapshot = 9;
*/
public eraftpb.Eraftpb.SnapshotOrBuilder getSnapshotOrBuilder() {
if (snapshotBuilder_ != null) {
return snapshotBuilder_.getMessageOrBuilder();
} else {
return snapshot_ == null ?
eraftpb.Eraftpb.Snapshot.getDefaultInstance() : snapshot_;
}
}
/**
* .eraftpb.Snapshot snapshot = 9;
*/
private com.google.protobuf.SingleFieldBuilderV3<
eraftpb.Eraftpb.Snapshot, eraftpb.Eraftpb.Snapshot.Builder, eraftpb.Eraftpb.SnapshotOrBuilder>
getSnapshotFieldBuilder() {
if (snapshotBuilder_ == null) {
snapshotBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
eraftpb.Eraftpb.Snapshot, eraftpb.Eraftpb.Snapshot.Builder, eraftpb.Eraftpb.SnapshotOrBuilder>(
getSnapshot(),
getParentForChildren(),
isClean());
snapshot_ = null;
}
return snapshotBuilder_;
}
private long requestSnapshot_ ;
/**
* uint64 request_snapshot = 13;
*/
public long getRequestSnapshot() {
return requestSnapshot_;
}
/**
* uint64 request_snapshot = 13;
*/
public Builder setRequestSnapshot(long value) {
requestSnapshot_ = value;
onChanged();
return this;
}
/**
* uint64 request_snapshot = 13;
*/
public Builder clearRequestSnapshot() {
requestSnapshot_ = 0L;
onChanged();
return this;
}
private boolean reject_ ;
/**
* bool reject = 10;
*/
public boolean getReject() {
return reject_;
}
/**
* bool reject = 10;
*/
public Builder setReject(boolean value) {
reject_ = value;
onChanged();
return this;
}
/**
* bool reject = 10;
*/
public Builder clearReject() {
reject_ = false;
onChanged();
return this;
}
private long rejectHint_ ;
/**
* uint64 reject_hint = 11;
*/
public long getRejectHint() {
return rejectHint_;
}
/**
* uint64 reject_hint = 11;
*/
public Builder setRejectHint(long value) {
rejectHint_ = value;
onChanged();
return this;
}
/**
* uint64 reject_hint = 11;
*/
public Builder clearRejectHint() {
rejectHint_ = 0L;
onChanged();
return this;
}
private com.google.protobuf.ByteString context_ = com.google.protobuf.ByteString.EMPTY;
/**
* bytes context = 12;
*/
public com.google.protobuf.ByteString getContext() {
return context_;
}
/**
* bytes context = 12;
*/
public Builder setContext(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
context_ = value;
onChanged();
return this;
}
/**
* bytes context = 12;
*/
public Builder clearContext() {
context_ = getDefaultInstance().getContext();
onChanged();
return this;
}
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFieldsProto3(unknownFields);
}
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:eraftpb.Message)
}
// @@protoc_insertion_point(class_scope:eraftpb.Message)
private static final eraftpb.Eraftpb.Message DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new eraftpb.Eraftpb.Message();
}
public static eraftpb.Eraftpb.Message getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
public Message parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new Message(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
public eraftpb.Eraftpb.Message getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface HardStateOrBuilder extends
// @@protoc_insertion_point(interface_extends:eraftpb.HardState)
com.google.protobuf.MessageOrBuilder {
/**
* uint64 term = 1;
*/
long getTerm();
/**
* uint64 vote = 2;
*/
long getVote();
/**
* uint64 commit = 3;
*/
long getCommit();
}
/**
* Protobuf type {@code eraftpb.HardState}
*/
public static final class HardState extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:eraftpb.HardState)
HardStateOrBuilder {
private static final long serialVersionUID = 0L;
// Use HardState.newBuilder() to construct.
private HardState(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private HardState() {
term_ = 0L;
vote_ = 0L;
commit_ = 0L;
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private HardState(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
default: {
if (!parseUnknownFieldProto3(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
case 8: {
term_ = input.readUInt64();
break;
}
case 16: {
vote_ = input.readUInt64();
break;
}
case 24: {
commit_ = input.readUInt64();
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return eraftpb.Eraftpb.internal_static_eraftpb_HardState_descriptor;
}
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return eraftpb.Eraftpb.internal_static_eraftpb_HardState_fieldAccessorTable
.ensureFieldAccessorsInitialized(
eraftpb.Eraftpb.HardState.class, eraftpb.Eraftpb.HardState.Builder.class);
}
public static final int TERM_FIELD_NUMBER = 1;
private long term_;
/**
* uint64 term = 1;
*/
public long getTerm() {
return term_;
}
public static final int VOTE_FIELD_NUMBER = 2;
private long vote_;
/**
* uint64 vote = 2;
*/
public long getVote() {
return vote_;
}
public static final int COMMIT_FIELD_NUMBER = 3;
private long commit_;
/**
* uint64 commit = 3;
*/
public long getCommit() {
return commit_;
}
private byte memoizedIsInitialized = -1;
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (term_ != 0L) {
output.writeUInt64(1, term_);
}
if (vote_ != 0L) {
output.writeUInt64(2, vote_);
}
if (commit_ != 0L) {
output.writeUInt64(3, commit_);
}
unknownFields.writeTo(output);
}
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (term_ != 0L) {
size += com.google.protobuf.CodedOutputStream
.computeUInt64Size(1, term_);
}
if (vote_ != 0L) {
size += com.google.protobuf.CodedOutputStream
.computeUInt64Size(2, vote_);
}
if (commit_ != 0L) {
size += com.google.protobuf.CodedOutputStream
.computeUInt64Size(3, commit_);
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof eraftpb.Eraftpb.HardState)) {
return super.equals(obj);
}
eraftpb.Eraftpb.HardState other = (eraftpb.Eraftpb.HardState) obj;
boolean result = true;
result = result && (getTerm()
== other.getTerm());
result = result && (getVote()
== other.getVote());
result = result && (getCommit()
== other.getCommit());
result = result && unknownFields.equals(other.unknownFields);
return result;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
hash = (37 * hash) + TERM_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
getTerm());
hash = (37 * hash) + VOTE_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
getVote());
hash = (37 * hash) + COMMIT_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
getCommit());
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static eraftpb.Eraftpb.HardState parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static eraftpb.Eraftpb.HardState parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static eraftpb.Eraftpb.HardState parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static eraftpb.Eraftpb.HardState parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static eraftpb.Eraftpb.HardState parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static eraftpb.Eraftpb.HardState parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static eraftpb.Eraftpb.HardState parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static eraftpb.Eraftpb.HardState parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static eraftpb.Eraftpb.HardState parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static eraftpb.Eraftpb.HardState parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static eraftpb.Eraftpb.HardState parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static eraftpb.Eraftpb.HardState parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(eraftpb.Eraftpb.HardState prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code eraftpb.HardState}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:eraftpb.HardState)
eraftpb.Eraftpb.HardStateOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return eraftpb.Eraftpb.internal_static_eraftpb_HardState_descriptor;
}
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return eraftpb.Eraftpb.internal_static_eraftpb_HardState_fieldAccessorTable
.ensureFieldAccessorsInitialized(
eraftpb.Eraftpb.HardState.class, eraftpb.Eraftpb.HardState.Builder.class);
}
// Construct using eraftpb.Eraftpb.HardState.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
public Builder clear() {
super.clear();
term_ = 0L;
vote_ = 0L;
commit_ = 0L;
return this;
}
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return eraftpb.Eraftpb.internal_static_eraftpb_HardState_descriptor;
}
public eraftpb.Eraftpb.HardState getDefaultInstanceForType() {
return eraftpb.Eraftpb.HardState.getDefaultInstance();
}
public eraftpb.Eraftpb.HardState build() {
eraftpb.Eraftpb.HardState result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
public eraftpb.Eraftpb.HardState buildPartial() {
eraftpb.Eraftpb.HardState result = new eraftpb.Eraftpb.HardState(this);
result.term_ = term_;
result.vote_ = vote_;
result.commit_ = commit_;
onBuilt();
return result;
}
public Builder clone() {
return (Builder) super.clone();
}
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.setField(field, value);
}
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return (Builder) super.clearField(field);
}
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return (Builder) super.clearOneof(oneof);
}
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return (Builder) super.setRepeatedField(field, index, value);
}
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.addRepeatedField(field, value);
}
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof eraftpb.Eraftpb.HardState) {
return mergeFrom((eraftpb.Eraftpb.HardState)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(eraftpb.Eraftpb.HardState other) {
if (other == eraftpb.Eraftpb.HardState.getDefaultInstance()) return this;
if (other.getTerm() != 0L) {
setTerm(other.getTerm());
}
if (other.getVote() != 0L) {
setVote(other.getVote());
}
if (other.getCommit() != 0L) {
setCommit(other.getCommit());
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
public final boolean isInitialized() {
return true;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
eraftpb.Eraftpb.HardState parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (eraftpb.Eraftpb.HardState) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private long term_ ;
/**
* uint64 term = 1;
*/
public long getTerm() {
return term_;
}
/**
* uint64 term = 1;
*/
public Builder setTerm(long value) {
term_ = value;
onChanged();
return this;
}
/**
* uint64 term = 1;
*/
public Builder clearTerm() {
term_ = 0L;
onChanged();
return this;
}
private long vote_ ;
/**
* uint64 vote = 2;
*/
public long getVote() {
return vote_;
}
/**
* uint64 vote = 2;
*/
public Builder setVote(long value) {
vote_ = value;
onChanged();
return this;
}
/**
* uint64 vote = 2;
*/
public Builder clearVote() {
vote_ = 0L;
onChanged();
return this;
}
private long commit_ ;
/**
* uint64 commit = 3;
*/
public long getCommit() {
return commit_;
}
/**
* uint64 commit = 3;
*/
public Builder setCommit(long value) {
commit_ = value;
onChanged();
return this;
}
/**
* uint64 commit = 3;
*/
public Builder clearCommit() {
commit_ = 0L;
onChanged();
return this;
}
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFieldsProto3(unknownFields);
}
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:eraftpb.HardState)
}
// @@protoc_insertion_point(class_scope:eraftpb.HardState)
private static final eraftpb.Eraftpb.HardState DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new eraftpb.Eraftpb.HardState();
}
public static eraftpb.Eraftpb.HardState getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
public HardState parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new HardState(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
public eraftpb.Eraftpb.HardState getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface ConfStateOrBuilder extends
// @@protoc_insertion_point(interface_extends:eraftpb.ConfState)
com.google.protobuf.MessageOrBuilder {
/**
* repeated uint64 voters = 1;
*/
java.util.List getVotersList();
/**
* repeated uint64 voters = 1;
*/
int getVotersCount();
/**
* repeated uint64 voters = 1;
*/
long getVoters(int index);
/**
* repeated uint64 learners = 2;
*/
java.util.List getLearnersList();
/**
* repeated uint64 learners = 2;
*/
int getLearnersCount();
/**
* repeated uint64 learners = 2;
*/
long getLearners(int index);
/**
*
* The voters in the outgoing config. If not empty the node is in joint consensus.
*
*
* repeated uint64 voters_outgoing = 3;
*/
java.util.List getVotersOutgoingList();
/**
*
* The voters in the outgoing config. If not empty the node is in joint consensus.
*
*
* repeated uint64 voters_outgoing = 3;
*/
int getVotersOutgoingCount();
/**
*
* The voters in the outgoing config. If not empty the node is in joint consensus.
*
*
* repeated uint64 voters_outgoing = 3;
*/
long getVotersOutgoing(int index);
/**
*
* The nodes that will become learners when the outgoing config is removed.
* These nodes are necessarily currently in nodes_joint (or they would have
* been added to the incoming config right away).
*
*
* repeated uint64 learners_next = 4;
*/
java.util.List getLearnersNextList();
/**
*
* The nodes that will become learners when the outgoing config is removed.
* These nodes are necessarily currently in nodes_joint (or they would have
* been added to the incoming config right away).
*
*
* repeated uint64 learners_next = 4;
*/
int getLearnersNextCount();
/**
*
* The nodes that will become learners when the outgoing config is removed.
* These nodes are necessarily currently in nodes_joint (or they would have
* been added to the incoming config right away).
*
*
* repeated uint64 learners_next = 4;
*/
long getLearnersNext(int index);
/**
*
* If set, the config is joint and Raft will automatically transition into
* the final config (i.e. remove the outgoing config) when this is safe.
*
*
* bool auto_leave = 5;
*/
boolean getAutoLeave();
}
/**
* Protobuf type {@code eraftpb.ConfState}
*/
public static final class ConfState extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:eraftpb.ConfState)
ConfStateOrBuilder {
private static final long serialVersionUID = 0L;
// Use ConfState.newBuilder() to construct.
private ConfState(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private ConfState() {
voters_ = java.util.Collections.emptyList();
learners_ = java.util.Collections.emptyList();
votersOutgoing_ = java.util.Collections.emptyList();
learnersNext_ = java.util.Collections.emptyList();
autoLeave_ = false;
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private ConfState(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
default: {
if (!parseUnknownFieldProto3(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
case 8: {
if (!((mutable_bitField0_ & 0x00000001) == 0x00000001)) {
voters_ = new java.util.ArrayList();
mutable_bitField0_ |= 0x00000001;
}
voters_.add(input.readUInt64());
break;
}
case 10: {
int length = input.readRawVarint32();
int limit = input.pushLimit(length);
if (!((mutable_bitField0_ & 0x00000001) == 0x00000001) && input.getBytesUntilLimit() > 0) {
voters_ = new java.util.ArrayList();
mutable_bitField0_ |= 0x00000001;
}
while (input.getBytesUntilLimit() > 0) {
voters_.add(input.readUInt64());
}
input.popLimit(limit);
break;
}
case 16: {
if (!((mutable_bitField0_ & 0x00000002) == 0x00000002)) {
learners_ = new java.util.ArrayList();
mutable_bitField0_ |= 0x00000002;
}
learners_.add(input.readUInt64());
break;
}
case 18: {
int length = input.readRawVarint32();
int limit = input.pushLimit(length);
if (!((mutable_bitField0_ & 0x00000002) == 0x00000002) && input.getBytesUntilLimit() > 0) {
learners_ = new java.util.ArrayList();
mutable_bitField0_ |= 0x00000002;
}
while (input.getBytesUntilLimit() > 0) {
learners_.add(input.readUInt64());
}
input.popLimit(limit);
break;
}
case 24: {
if (!((mutable_bitField0_ & 0x00000004) == 0x00000004)) {
votersOutgoing_ = new java.util.ArrayList();
mutable_bitField0_ |= 0x00000004;
}
votersOutgoing_.add(input.readUInt64());
break;
}
case 26: {
int length = input.readRawVarint32();
int limit = input.pushLimit(length);
if (!((mutable_bitField0_ & 0x00000004) == 0x00000004) && input.getBytesUntilLimit() > 0) {
votersOutgoing_ = new java.util.ArrayList();
mutable_bitField0_ |= 0x00000004;
}
while (input.getBytesUntilLimit() > 0) {
votersOutgoing_.add(input.readUInt64());
}
input.popLimit(limit);
break;
}
case 32: {
if (!((mutable_bitField0_ & 0x00000008) == 0x00000008)) {
learnersNext_ = new java.util.ArrayList();
mutable_bitField0_ |= 0x00000008;
}
learnersNext_.add(input.readUInt64());
break;
}
case 34: {
int length = input.readRawVarint32();
int limit = input.pushLimit(length);
if (!((mutable_bitField0_ & 0x00000008) == 0x00000008) && input.getBytesUntilLimit() > 0) {
learnersNext_ = new java.util.ArrayList();
mutable_bitField0_ |= 0x00000008;
}
while (input.getBytesUntilLimit() > 0) {
learnersNext_.add(input.readUInt64());
}
input.popLimit(limit);
break;
}
case 40: {
autoLeave_ = input.readBool();
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
if (((mutable_bitField0_ & 0x00000001) == 0x00000001)) {
voters_ = java.util.Collections.unmodifiableList(voters_);
}
if (((mutable_bitField0_ & 0x00000002) == 0x00000002)) {
learners_ = java.util.Collections.unmodifiableList(learners_);
}
if (((mutable_bitField0_ & 0x00000004) == 0x00000004)) {
votersOutgoing_ = java.util.Collections.unmodifiableList(votersOutgoing_);
}
if (((mutable_bitField0_ & 0x00000008) == 0x00000008)) {
learnersNext_ = java.util.Collections.unmodifiableList(learnersNext_);
}
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return eraftpb.Eraftpb.internal_static_eraftpb_ConfState_descriptor;
}
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return eraftpb.Eraftpb.internal_static_eraftpb_ConfState_fieldAccessorTable
.ensureFieldAccessorsInitialized(
eraftpb.Eraftpb.ConfState.class, eraftpb.Eraftpb.ConfState.Builder.class);
}
private int bitField0_;
public static final int VOTERS_FIELD_NUMBER = 1;
private java.util.List voters_;
/**
* repeated uint64 voters = 1;
*/
public java.util.List
getVotersList() {
return voters_;
}
/**
* repeated uint64 voters = 1;
*/
public int getVotersCount() {
return voters_.size();
}
/**
* repeated uint64 voters = 1;
*/
public long getVoters(int index) {
return voters_.get(index);
}
private int votersMemoizedSerializedSize = -1;
public static final int LEARNERS_FIELD_NUMBER = 2;
private java.util.List learners_;
/**
* repeated uint64 learners = 2;
*/
public java.util.List
getLearnersList() {
return learners_;
}
/**
* repeated uint64 learners = 2;
*/
public int getLearnersCount() {
return learners_.size();
}
/**
* repeated uint64 learners = 2;
*/
public long getLearners(int index) {
return learners_.get(index);
}
private int learnersMemoizedSerializedSize = -1;
public static final int VOTERS_OUTGOING_FIELD_NUMBER = 3;
private java.util.List votersOutgoing_;
/**
*
* The voters in the outgoing config. If not empty the node is in joint consensus.
*
*
* repeated uint64 voters_outgoing = 3;
*/
public java.util.List
getVotersOutgoingList() {
return votersOutgoing_;
}
/**
*
* The voters in the outgoing config. If not empty the node is in joint consensus.
*
*
* repeated uint64 voters_outgoing = 3;
*/
public int getVotersOutgoingCount() {
return votersOutgoing_.size();
}
/**
*
* The voters in the outgoing config. If not empty the node is in joint consensus.
*
*
* repeated uint64 voters_outgoing = 3;
*/
public long getVotersOutgoing(int index) {
return votersOutgoing_.get(index);
}
private int votersOutgoingMemoizedSerializedSize = -1;
public static final int LEARNERS_NEXT_FIELD_NUMBER = 4;
private java.util.List learnersNext_;
/**
*
* The nodes that will become learners when the outgoing config is removed.
* These nodes are necessarily currently in nodes_joint (or they would have
* been added to the incoming config right away).
*
*
* repeated uint64 learners_next = 4;
*/
public java.util.List
getLearnersNextList() {
return learnersNext_;
}
/**
*
* The nodes that will become learners when the outgoing config is removed.
* These nodes are necessarily currently in nodes_joint (or they would have
* been added to the incoming config right away).
*
*
* repeated uint64 learners_next = 4;
*/
public int getLearnersNextCount() {
return learnersNext_.size();
}
/**
*
* The nodes that will become learners when the outgoing config is removed.
* These nodes are necessarily currently in nodes_joint (or they would have
* been added to the incoming config right away).
*
*
* repeated uint64 learners_next = 4;
*/
public long getLearnersNext(int index) {
return learnersNext_.get(index);
}
private int learnersNextMemoizedSerializedSize = -1;
public static final int AUTO_LEAVE_FIELD_NUMBER = 5;
private boolean autoLeave_;
/**
*
* If set, the config is joint and Raft will automatically transition into
* the final config (i.e. remove the outgoing config) when this is safe.
*
*
* bool auto_leave = 5;
*/
public boolean getAutoLeave() {
return autoLeave_;
}
private byte memoizedIsInitialized = -1;
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
getSerializedSize();
if (getVotersList().size() > 0) {
output.writeUInt32NoTag(10);
output.writeUInt32NoTag(votersMemoizedSerializedSize);
}
for (int i = 0; i < voters_.size(); i++) {
output.writeUInt64NoTag(voters_.get(i));
}
if (getLearnersList().size() > 0) {
output.writeUInt32NoTag(18);
output.writeUInt32NoTag(learnersMemoizedSerializedSize);
}
for (int i = 0; i < learners_.size(); i++) {
output.writeUInt64NoTag(learners_.get(i));
}
if (getVotersOutgoingList().size() > 0) {
output.writeUInt32NoTag(26);
output.writeUInt32NoTag(votersOutgoingMemoizedSerializedSize);
}
for (int i = 0; i < votersOutgoing_.size(); i++) {
output.writeUInt64NoTag(votersOutgoing_.get(i));
}
if (getLearnersNextList().size() > 0) {
output.writeUInt32NoTag(34);
output.writeUInt32NoTag(learnersNextMemoizedSerializedSize);
}
for (int i = 0; i < learnersNext_.size(); i++) {
output.writeUInt64NoTag(learnersNext_.get(i));
}
if (autoLeave_ != false) {
output.writeBool(5, autoLeave_);
}
unknownFields.writeTo(output);
}
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
{
int dataSize = 0;
for (int i = 0; i < voters_.size(); i++) {
dataSize += com.google.protobuf.CodedOutputStream
.computeUInt64SizeNoTag(voters_.get(i));
}
size += dataSize;
if (!getVotersList().isEmpty()) {
size += 1;
size += com.google.protobuf.CodedOutputStream
.computeInt32SizeNoTag(dataSize);
}
votersMemoizedSerializedSize = dataSize;
}
{
int dataSize = 0;
for (int i = 0; i < learners_.size(); i++) {
dataSize += com.google.protobuf.CodedOutputStream
.computeUInt64SizeNoTag(learners_.get(i));
}
size += dataSize;
if (!getLearnersList().isEmpty()) {
size += 1;
size += com.google.protobuf.CodedOutputStream
.computeInt32SizeNoTag(dataSize);
}
learnersMemoizedSerializedSize = dataSize;
}
{
int dataSize = 0;
for (int i = 0; i < votersOutgoing_.size(); i++) {
dataSize += com.google.protobuf.CodedOutputStream
.computeUInt64SizeNoTag(votersOutgoing_.get(i));
}
size += dataSize;
if (!getVotersOutgoingList().isEmpty()) {
size += 1;
size += com.google.protobuf.CodedOutputStream
.computeInt32SizeNoTag(dataSize);
}
votersOutgoingMemoizedSerializedSize = dataSize;
}
{
int dataSize = 0;
for (int i = 0; i < learnersNext_.size(); i++) {
dataSize += com.google.protobuf.CodedOutputStream
.computeUInt64SizeNoTag(learnersNext_.get(i));
}
size += dataSize;
if (!getLearnersNextList().isEmpty()) {
size += 1;
size += com.google.protobuf.CodedOutputStream
.computeInt32SizeNoTag(dataSize);
}
learnersNextMemoizedSerializedSize = dataSize;
}
if (autoLeave_ != false) {
size += com.google.protobuf.CodedOutputStream
.computeBoolSize(5, autoLeave_);
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof eraftpb.Eraftpb.ConfState)) {
return super.equals(obj);
}
eraftpb.Eraftpb.ConfState other = (eraftpb.Eraftpb.ConfState) obj;
boolean result = true;
result = result && getVotersList()
.equals(other.getVotersList());
result = result && getLearnersList()
.equals(other.getLearnersList());
result = result && getVotersOutgoingList()
.equals(other.getVotersOutgoingList());
result = result && getLearnersNextList()
.equals(other.getLearnersNextList());
result = result && (getAutoLeave()
== other.getAutoLeave());
result = result && unknownFields.equals(other.unknownFields);
return result;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (getVotersCount() > 0) {
hash = (37 * hash) + VOTERS_FIELD_NUMBER;
hash = (53 * hash) + getVotersList().hashCode();
}
if (getLearnersCount() > 0) {
hash = (37 * hash) + LEARNERS_FIELD_NUMBER;
hash = (53 * hash) + getLearnersList().hashCode();
}
if (getVotersOutgoingCount() > 0) {
hash = (37 * hash) + VOTERS_OUTGOING_FIELD_NUMBER;
hash = (53 * hash) + getVotersOutgoingList().hashCode();
}
if (getLearnersNextCount() > 0) {
hash = (37 * hash) + LEARNERS_NEXT_FIELD_NUMBER;
hash = (53 * hash) + getLearnersNextList().hashCode();
}
hash = (37 * hash) + AUTO_LEAVE_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashBoolean(
getAutoLeave());
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static eraftpb.Eraftpb.ConfState parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static eraftpb.Eraftpb.ConfState parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static eraftpb.Eraftpb.ConfState parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static eraftpb.Eraftpb.ConfState parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static eraftpb.Eraftpb.ConfState parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static eraftpb.Eraftpb.ConfState parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static eraftpb.Eraftpb.ConfState parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static eraftpb.Eraftpb.ConfState parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static eraftpb.Eraftpb.ConfState parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static eraftpb.Eraftpb.ConfState parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static eraftpb.Eraftpb.ConfState parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static eraftpb.Eraftpb.ConfState parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(eraftpb.Eraftpb.ConfState prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code eraftpb.ConfState}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:eraftpb.ConfState)
eraftpb.Eraftpb.ConfStateOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return eraftpb.Eraftpb.internal_static_eraftpb_ConfState_descriptor;
}
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return eraftpb.Eraftpb.internal_static_eraftpb_ConfState_fieldAccessorTable
.ensureFieldAccessorsInitialized(
eraftpb.Eraftpb.ConfState.class, eraftpb.Eraftpb.ConfState.Builder.class);
}
// Construct using eraftpb.Eraftpb.ConfState.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
public Builder clear() {
super.clear();
voters_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000001);
learners_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000002);
votersOutgoing_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000004);
learnersNext_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000008);
autoLeave_ = false;
return this;
}
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return eraftpb.Eraftpb.internal_static_eraftpb_ConfState_descriptor;
}
public eraftpb.Eraftpb.ConfState getDefaultInstanceForType() {
return eraftpb.Eraftpb.ConfState.getDefaultInstance();
}
public eraftpb.Eraftpb.ConfState build() {
eraftpb.Eraftpb.ConfState result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
public eraftpb.Eraftpb.ConfState buildPartial() {
eraftpb.Eraftpb.ConfState result = new eraftpb.Eraftpb.ConfState(this);
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (((bitField0_ & 0x00000001) == 0x00000001)) {
voters_ = java.util.Collections.unmodifiableList(voters_);
bitField0_ = (bitField0_ & ~0x00000001);
}
result.voters_ = voters_;
if (((bitField0_ & 0x00000002) == 0x00000002)) {
learners_ = java.util.Collections.unmodifiableList(learners_);
bitField0_ = (bitField0_ & ~0x00000002);
}
result.learners_ = learners_;
if (((bitField0_ & 0x00000004) == 0x00000004)) {
votersOutgoing_ = java.util.Collections.unmodifiableList(votersOutgoing_);
bitField0_ = (bitField0_ & ~0x00000004);
}
result.votersOutgoing_ = votersOutgoing_;
if (((bitField0_ & 0x00000008) == 0x00000008)) {
learnersNext_ = java.util.Collections.unmodifiableList(learnersNext_);
bitField0_ = (bitField0_ & ~0x00000008);
}
result.learnersNext_ = learnersNext_;
result.autoLeave_ = autoLeave_;
result.bitField0_ = to_bitField0_;
onBuilt();
return result;
}
public Builder clone() {
return (Builder) super.clone();
}
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.setField(field, value);
}
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return (Builder) super.clearField(field);
}
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return (Builder) super.clearOneof(oneof);
}
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return (Builder) super.setRepeatedField(field, index, value);
}
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.addRepeatedField(field, value);
}
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof eraftpb.Eraftpb.ConfState) {
return mergeFrom((eraftpb.Eraftpb.ConfState)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(eraftpb.Eraftpb.ConfState other) {
if (other == eraftpb.Eraftpb.ConfState.getDefaultInstance()) return this;
if (!other.voters_.isEmpty()) {
if (voters_.isEmpty()) {
voters_ = other.voters_;
bitField0_ = (bitField0_ & ~0x00000001);
} else {
ensureVotersIsMutable();
voters_.addAll(other.voters_);
}
onChanged();
}
if (!other.learners_.isEmpty()) {
if (learners_.isEmpty()) {
learners_ = other.learners_;
bitField0_ = (bitField0_ & ~0x00000002);
} else {
ensureLearnersIsMutable();
learners_.addAll(other.learners_);
}
onChanged();
}
if (!other.votersOutgoing_.isEmpty()) {
if (votersOutgoing_.isEmpty()) {
votersOutgoing_ = other.votersOutgoing_;
bitField0_ = (bitField0_ & ~0x00000004);
} else {
ensureVotersOutgoingIsMutable();
votersOutgoing_.addAll(other.votersOutgoing_);
}
onChanged();
}
if (!other.learnersNext_.isEmpty()) {
if (learnersNext_.isEmpty()) {
learnersNext_ = other.learnersNext_;
bitField0_ = (bitField0_ & ~0x00000008);
} else {
ensureLearnersNextIsMutable();
learnersNext_.addAll(other.learnersNext_);
}
onChanged();
}
if (other.getAutoLeave() != false) {
setAutoLeave(other.getAutoLeave());
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
public final boolean isInitialized() {
return true;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
eraftpb.Eraftpb.ConfState parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (eraftpb.Eraftpb.ConfState) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
private java.util.List voters_ = java.util.Collections.emptyList();
private void ensureVotersIsMutable() {
if (!((bitField0_ & 0x00000001) == 0x00000001)) {
voters_ = new java.util.ArrayList(voters_);
bitField0_ |= 0x00000001;
}
}
/**
* repeated uint64 voters = 1;
*/
public java.util.List
getVotersList() {
return java.util.Collections.unmodifiableList(voters_);
}
/**
* repeated uint64 voters = 1;
*/
public int getVotersCount() {
return voters_.size();
}
/**
* repeated uint64 voters = 1;
*/
public long getVoters(int index) {
return voters_.get(index);
}
/**
* repeated uint64 voters = 1;
*/
public Builder setVoters(
int index, long value) {
ensureVotersIsMutable();
voters_.set(index, value);
onChanged();
return this;
}
/**
* repeated uint64 voters = 1;
*/
public Builder addVoters(long value) {
ensureVotersIsMutable();
voters_.add(value);
onChanged();
return this;
}
/**
* repeated uint64 voters = 1;
*/
public Builder addAllVoters(
java.lang.Iterable extends java.lang.Long> values) {
ensureVotersIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, voters_);
onChanged();
return this;
}
/**
* repeated uint64 voters = 1;
*/
public Builder clearVoters() {
voters_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000001);
onChanged();
return this;
}
private java.util.List learners_ = java.util.Collections.emptyList();
private void ensureLearnersIsMutable() {
if (!((bitField0_ & 0x00000002) == 0x00000002)) {
learners_ = new java.util.ArrayList(learners_);
bitField0_ |= 0x00000002;
}
}
/**
* repeated uint64 learners = 2;
*/
public java.util.List
getLearnersList() {
return java.util.Collections.unmodifiableList(learners_);
}
/**
* repeated uint64 learners = 2;
*/
public int getLearnersCount() {
return learners_.size();
}
/**
* repeated uint64 learners = 2;
*/
public long getLearners(int index) {
return learners_.get(index);
}
/**
* repeated uint64 learners = 2;
*/
public Builder setLearners(
int index, long value) {
ensureLearnersIsMutable();
learners_.set(index, value);
onChanged();
return this;
}
/**
* repeated uint64 learners = 2;
*/
public Builder addLearners(long value) {
ensureLearnersIsMutable();
learners_.add(value);
onChanged();
return this;
}
/**
* repeated uint64 learners = 2;
*/
public Builder addAllLearners(
java.lang.Iterable extends java.lang.Long> values) {
ensureLearnersIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, learners_);
onChanged();
return this;
}
/**
* repeated uint64 learners = 2;
*/
public Builder clearLearners() {
learners_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000002);
onChanged();
return this;
}
private java.util.List votersOutgoing_ = java.util.Collections.emptyList();
private void ensureVotersOutgoingIsMutable() {
if (!((bitField0_ & 0x00000004) == 0x00000004)) {
votersOutgoing_ = new java.util.ArrayList(votersOutgoing_);
bitField0_ |= 0x00000004;
}
}
/**
*
* The voters in the outgoing config. If not empty the node is in joint consensus.
*
*
* repeated uint64 voters_outgoing = 3;
*/
public java.util.List
getVotersOutgoingList() {
return java.util.Collections.unmodifiableList(votersOutgoing_);
}
/**
*
* The voters in the outgoing config. If not empty the node is in joint consensus.
*
*
* repeated uint64 voters_outgoing = 3;
*/
public int getVotersOutgoingCount() {
return votersOutgoing_.size();
}
/**
*
* The voters in the outgoing config. If not empty the node is in joint consensus.
*
*
* repeated uint64 voters_outgoing = 3;
*/
public long getVotersOutgoing(int index) {
return votersOutgoing_.get(index);
}
/**
*
* The voters in the outgoing config. If not empty the node is in joint consensus.
*
*
* repeated uint64 voters_outgoing = 3;
*/
public Builder setVotersOutgoing(
int index, long value) {
ensureVotersOutgoingIsMutable();
votersOutgoing_.set(index, value);
onChanged();
return this;
}
/**
*
* The voters in the outgoing config. If not empty the node is in joint consensus.
*
*
* repeated uint64 voters_outgoing = 3;
*/
public Builder addVotersOutgoing(long value) {
ensureVotersOutgoingIsMutable();
votersOutgoing_.add(value);
onChanged();
return this;
}
/**
*
* The voters in the outgoing config. If not empty the node is in joint consensus.
*
*
* repeated uint64 voters_outgoing = 3;
*/
public Builder addAllVotersOutgoing(
java.lang.Iterable extends java.lang.Long> values) {
ensureVotersOutgoingIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, votersOutgoing_);
onChanged();
return this;
}
/**
*
* The voters in the outgoing config. If not empty the node is in joint consensus.
*
*
* repeated uint64 voters_outgoing = 3;
*/
public Builder clearVotersOutgoing() {
votersOutgoing_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000004);
onChanged();
return this;
}
private java.util.List learnersNext_ = java.util.Collections.emptyList();
private void ensureLearnersNextIsMutable() {
if (!((bitField0_ & 0x00000008) == 0x00000008)) {
learnersNext_ = new java.util.ArrayList(learnersNext_);
bitField0_ |= 0x00000008;
}
}
/**
*
* The nodes that will become learners when the outgoing config is removed.
* These nodes are necessarily currently in nodes_joint (or they would have
* been added to the incoming config right away).
*
*
* repeated uint64 learners_next = 4;
*/
public java.util.List
getLearnersNextList() {
return java.util.Collections.unmodifiableList(learnersNext_);
}
/**
*
* The nodes that will become learners when the outgoing config is removed.
* These nodes are necessarily currently in nodes_joint (or they would have
* been added to the incoming config right away).
*
*
* repeated uint64 learners_next = 4;
*/
public int getLearnersNextCount() {
return learnersNext_.size();
}
/**
*
* The nodes that will become learners when the outgoing config is removed.
* These nodes are necessarily currently in nodes_joint (or they would have
* been added to the incoming config right away).
*
*
* repeated uint64 learners_next = 4;
*/
public long getLearnersNext(int index) {
return learnersNext_.get(index);
}
/**
*
* The nodes that will become learners when the outgoing config is removed.
* These nodes are necessarily currently in nodes_joint (or they would have
* been added to the incoming config right away).
*
*
* repeated uint64 learners_next = 4;
*/
public Builder setLearnersNext(
int index, long value) {
ensureLearnersNextIsMutable();
learnersNext_.set(index, value);
onChanged();
return this;
}
/**
*
* The nodes that will become learners when the outgoing config is removed.
* These nodes are necessarily currently in nodes_joint (or they would have
* been added to the incoming config right away).
*
*
* repeated uint64 learners_next = 4;
*/
public Builder addLearnersNext(long value) {
ensureLearnersNextIsMutable();
learnersNext_.add(value);
onChanged();
return this;
}
/**
*
* The nodes that will become learners when the outgoing config is removed.
* These nodes are necessarily currently in nodes_joint (or they would have
* been added to the incoming config right away).
*
*
* repeated uint64 learners_next = 4;
*/
public Builder addAllLearnersNext(
java.lang.Iterable extends java.lang.Long> values) {
ensureLearnersNextIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, learnersNext_);
onChanged();
return this;
}
/**
*
* The nodes that will become learners when the outgoing config is removed.
* These nodes are necessarily currently in nodes_joint (or they would have
* been added to the incoming config right away).
*
*
* repeated uint64 learners_next = 4;
*/
public Builder clearLearnersNext() {
learnersNext_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000008);
onChanged();
return this;
}
private boolean autoLeave_ ;
/**
*
* If set, the config is joint and Raft will automatically transition into
* the final config (i.e. remove the outgoing config) when this is safe.
*
*
* bool auto_leave = 5;
*/
public boolean getAutoLeave() {
return autoLeave_;
}
/**
*
* If set, the config is joint and Raft will automatically transition into
* the final config (i.e. remove the outgoing config) when this is safe.
*
*
* bool auto_leave = 5;
*/
public Builder setAutoLeave(boolean value) {
autoLeave_ = value;
onChanged();
return this;
}
/**
*
* If set, the config is joint and Raft will automatically transition into
* the final config (i.e. remove the outgoing config) when this is safe.
*
*
* bool auto_leave = 5;
*/
public Builder clearAutoLeave() {
autoLeave_ = false;
onChanged();
return this;
}
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFieldsProto3(unknownFields);
}
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:eraftpb.ConfState)
}
// @@protoc_insertion_point(class_scope:eraftpb.ConfState)
private static final eraftpb.Eraftpb.ConfState DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new eraftpb.Eraftpb.ConfState();
}
public static eraftpb.Eraftpb.ConfState getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
public ConfState parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new ConfState(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
public eraftpb.Eraftpb.ConfState getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface ConfChangeOrBuilder extends
// @@protoc_insertion_point(interface_extends:eraftpb.ConfChange)
com.google.protobuf.MessageOrBuilder {
/**
* .eraftpb.ConfChangeType change_type = 2;
*/
int getChangeTypeValue();
/**
* .eraftpb.ConfChangeType change_type = 2;
*/
eraftpb.Eraftpb.ConfChangeType getChangeType();
/**
* uint64 node_id = 3;
*/
long getNodeId();
/**
* bytes context = 4;
*/
com.google.protobuf.ByteString getContext();
/**
* uint64 id = 1;
*/
long getId();
}
/**
* Protobuf type {@code eraftpb.ConfChange}
*/
public static final class ConfChange extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:eraftpb.ConfChange)
ConfChangeOrBuilder {
private static final long serialVersionUID = 0L;
// Use ConfChange.newBuilder() to construct.
private ConfChange(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private ConfChange() {
changeType_ = 0;
nodeId_ = 0L;
context_ = com.google.protobuf.ByteString.EMPTY;
id_ = 0L;
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private ConfChange(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
default: {
if (!parseUnknownFieldProto3(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
case 8: {
id_ = input.readUInt64();
break;
}
case 16: {
int rawValue = input.readEnum();
changeType_ = rawValue;
break;
}
case 24: {
nodeId_ = input.readUInt64();
break;
}
case 34: {
context_ = input.readBytes();
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return eraftpb.Eraftpb.internal_static_eraftpb_ConfChange_descriptor;
}
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return eraftpb.Eraftpb.internal_static_eraftpb_ConfChange_fieldAccessorTable
.ensureFieldAccessorsInitialized(
eraftpb.Eraftpb.ConfChange.class, eraftpb.Eraftpb.ConfChange.Builder.class);
}
public static final int CHANGE_TYPE_FIELD_NUMBER = 2;
private int changeType_;
/**
* .eraftpb.ConfChangeType change_type = 2;
*/
public int getChangeTypeValue() {
return changeType_;
}
/**
* .eraftpb.ConfChangeType change_type = 2;
*/
public eraftpb.Eraftpb.ConfChangeType getChangeType() {
eraftpb.Eraftpb.ConfChangeType result = eraftpb.Eraftpb.ConfChangeType.valueOf(changeType_);
return result == null ? eraftpb.Eraftpb.ConfChangeType.UNRECOGNIZED : result;
}
public static final int NODE_ID_FIELD_NUMBER = 3;
private long nodeId_;
/**
* uint64 node_id = 3;
*/
public long getNodeId() {
return nodeId_;
}
public static final int CONTEXT_FIELD_NUMBER = 4;
private com.google.protobuf.ByteString context_;
/**
* bytes context = 4;
*/
public com.google.protobuf.ByteString getContext() {
return context_;
}
public static final int ID_FIELD_NUMBER = 1;
private long id_;
/**
* uint64 id = 1;
*/
public long getId() {
return id_;
}
private byte memoizedIsInitialized = -1;
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (id_ != 0L) {
output.writeUInt64(1, id_);
}
if (changeType_ != eraftpb.Eraftpb.ConfChangeType.AddNode.getNumber()) {
output.writeEnum(2, changeType_);
}
if (nodeId_ != 0L) {
output.writeUInt64(3, nodeId_);
}
if (!context_.isEmpty()) {
output.writeBytes(4, context_);
}
unknownFields.writeTo(output);
}
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (id_ != 0L) {
size += com.google.protobuf.CodedOutputStream
.computeUInt64Size(1, id_);
}
if (changeType_ != eraftpb.Eraftpb.ConfChangeType.AddNode.getNumber()) {
size += com.google.protobuf.CodedOutputStream
.computeEnumSize(2, changeType_);
}
if (nodeId_ != 0L) {
size += com.google.protobuf.CodedOutputStream
.computeUInt64Size(3, nodeId_);
}
if (!context_.isEmpty()) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(4, context_);
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof eraftpb.Eraftpb.ConfChange)) {
return super.equals(obj);
}
eraftpb.Eraftpb.ConfChange other = (eraftpb.Eraftpb.ConfChange) obj;
boolean result = true;
result = result && changeType_ == other.changeType_;
result = result && (getNodeId()
== other.getNodeId());
result = result && getContext()
.equals(other.getContext());
result = result && (getId()
== other.getId());
result = result && unknownFields.equals(other.unknownFields);
return result;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
hash = (37 * hash) + CHANGE_TYPE_FIELD_NUMBER;
hash = (53 * hash) + changeType_;
hash = (37 * hash) + NODE_ID_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
getNodeId());
hash = (37 * hash) + CONTEXT_FIELD_NUMBER;
hash = (53 * hash) + getContext().hashCode();
hash = (37 * hash) + ID_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
getId());
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static eraftpb.Eraftpb.ConfChange parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static eraftpb.Eraftpb.ConfChange parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static eraftpb.Eraftpb.ConfChange parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static eraftpb.Eraftpb.ConfChange parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static eraftpb.Eraftpb.ConfChange parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static eraftpb.Eraftpb.ConfChange parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static eraftpb.Eraftpb.ConfChange parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static eraftpb.Eraftpb.ConfChange parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static eraftpb.Eraftpb.ConfChange parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static eraftpb.Eraftpb.ConfChange parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static eraftpb.Eraftpb.ConfChange parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static eraftpb.Eraftpb.ConfChange parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(eraftpb.Eraftpb.ConfChange prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code eraftpb.ConfChange}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:eraftpb.ConfChange)
eraftpb.Eraftpb.ConfChangeOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return eraftpb.Eraftpb.internal_static_eraftpb_ConfChange_descriptor;
}
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return eraftpb.Eraftpb.internal_static_eraftpb_ConfChange_fieldAccessorTable
.ensureFieldAccessorsInitialized(
eraftpb.Eraftpb.ConfChange.class, eraftpb.Eraftpb.ConfChange.Builder.class);
}
// Construct using eraftpb.Eraftpb.ConfChange.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
public Builder clear() {
super.clear();
changeType_ = 0;
nodeId_ = 0L;
context_ = com.google.protobuf.ByteString.EMPTY;
id_ = 0L;
return this;
}
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return eraftpb.Eraftpb.internal_static_eraftpb_ConfChange_descriptor;
}
public eraftpb.Eraftpb.ConfChange getDefaultInstanceForType() {
return eraftpb.Eraftpb.ConfChange.getDefaultInstance();
}
public eraftpb.Eraftpb.ConfChange build() {
eraftpb.Eraftpb.ConfChange result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
public eraftpb.Eraftpb.ConfChange buildPartial() {
eraftpb.Eraftpb.ConfChange result = new eraftpb.Eraftpb.ConfChange(this);
result.changeType_ = changeType_;
result.nodeId_ = nodeId_;
result.context_ = context_;
result.id_ = id_;
onBuilt();
return result;
}
public Builder clone() {
return (Builder) super.clone();
}
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.setField(field, value);
}
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return (Builder) super.clearField(field);
}
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return (Builder) super.clearOneof(oneof);
}
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return (Builder) super.setRepeatedField(field, index, value);
}
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.addRepeatedField(field, value);
}
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof eraftpb.Eraftpb.ConfChange) {
return mergeFrom((eraftpb.Eraftpb.ConfChange)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(eraftpb.Eraftpb.ConfChange other) {
if (other == eraftpb.Eraftpb.ConfChange.getDefaultInstance()) return this;
if (other.changeType_ != 0) {
setChangeTypeValue(other.getChangeTypeValue());
}
if (other.getNodeId() != 0L) {
setNodeId(other.getNodeId());
}
if (other.getContext() != com.google.protobuf.ByteString.EMPTY) {
setContext(other.getContext());
}
if (other.getId() != 0L) {
setId(other.getId());
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
public final boolean isInitialized() {
return true;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
eraftpb.Eraftpb.ConfChange parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (eraftpb.Eraftpb.ConfChange) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int changeType_ = 0;
/**
* .eraftpb.ConfChangeType change_type = 2;
*/
public int getChangeTypeValue() {
return changeType_;
}
/**
* .eraftpb.ConfChangeType change_type = 2;
*/
public Builder setChangeTypeValue(int value) {
changeType_ = value;
onChanged();
return this;
}
/**
* .eraftpb.ConfChangeType change_type = 2;
*/
public eraftpb.Eraftpb.ConfChangeType getChangeType() {
eraftpb.Eraftpb.ConfChangeType result = eraftpb.Eraftpb.ConfChangeType.valueOf(changeType_);
return result == null ? eraftpb.Eraftpb.ConfChangeType.UNRECOGNIZED : result;
}
/**
* .eraftpb.ConfChangeType change_type = 2;
*/
public Builder setChangeType(eraftpb.Eraftpb.ConfChangeType value) {
if (value == null) {
throw new NullPointerException();
}
changeType_ = value.getNumber();
onChanged();
return this;
}
/**
* .eraftpb.ConfChangeType change_type = 2;
*/
public Builder clearChangeType() {
changeType_ = 0;
onChanged();
return this;
}
private long nodeId_ ;
/**
* uint64 node_id = 3;
*/
public long getNodeId() {
return nodeId_;
}
/**
* uint64 node_id = 3;
*/
public Builder setNodeId(long value) {
nodeId_ = value;
onChanged();
return this;
}
/**
* uint64 node_id = 3;
*/
public Builder clearNodeId() {
nodeId_ = 0L;
onChanged();
return this;
}
private com.google.protobuf.ByteString context_ = com.google.protobuf.ByteString.EMPTY;
/**
* bytes context = 4;
*/
public com.google.protobuf.ByteString getContext() {
return context_;
}
/**
* bytes context = 4;
*/
public Builder setContext(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
context_ = value;
onChanged();
return this;
}
/**
* bytes context = 4;
*/
public Builder clearContext() {
context_ = getDefaultInstance().getContext();
onChanged();
return this;
}
private long id_ ;
/**
* uint64 id = 1;
*/
public long getId() {
return id_;
}
/**
* uint64 id = 1;
*/
public Builder setId(long value) {
id_ = value;
onChanged();
return this;
}
/**
* uint64 id = 1;
*/
public Builder clearId() {
id_ = 0L;
onChanged();
return this;
}
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFieldsProto3(unknownFields);
}
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:eraftpb.ConfChange)
}
// @@protoc_insertion_point(class_scope:eraftpb.ConfChange)
private static final eraftpb.Eraftpb.ConfChange DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new eraftpb.Eraftpb.ConfChange();
}
public static eraftpb.Eraftpb.ConfChange getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
public ConfChange parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new ConfChange(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
public eraftpb.Eraftpb.ConfChange getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface ConfChangeSingleOrBuilder extends
// @@protoc_insertion_point(interface_extends:eraftpb.ConfChangeSingle)
com.google.protobuf.MessageOrBuilder {
/**
* .eraftpb.ConfChangeType type = 1;
*/
int getTypeValue();
/**
* .eraftpb.ConfChangeType type = 1;
*/
eraftpb.Eraftpb.ConfChangeType getType();
/**
* uint64 node_id = 2;
*/
long getNodeId();
}
/**
*
* ConfChangeSingle is an individual configuration change operation. Multiple
* such operations can be carried out atomically via a ConfChangeV2.
*
*
* Protobuf type {@code eraftpb.ConfChangeSingle}
*/
public static final class ConfChangeSingle extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:eraftpb.ConfChangeSingle)
ConfChangeSingleOrBuilder {
private static final long serialVersionUID = 0L;
// Use ConfChangeSingle.newBuilder() to construct.
private ConfChangeSingle(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private ConfChangeSingle() {
type_ = 0;
nodeId_ = 0L;
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private ConfChangeSingle(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
default: {
if (!parseUnknownFieldProto3(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
case 8: {
int rawValue = input.readEnum();
type_ = rawValue;
break;
}
case 16: {
nodeId_ = input.readUInt64();
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return eraftpb.Eraftpb.internal_static_eraftpb_ConfChangeSingle_descriptor;
}
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return eraftpb.Eraftpb.internal_static_eraftpb_ConfChangeSingle_fieldAccessorTable
.ensureFieldAccessorsInitialized(
eraftpb.Eraftpb.ConfChangeSingle.class, eraftpb.Eraftpb.ConfChangeSingle.Builder.class);
}
public static final int TYPE_FIELD_NUMBER = 1;
private int type_;
/**
* .eraftpb.ConfChangeType type = 1;
*/
public int getTypeValue() {
return type_;
}
/**
* .eraftpb.ConfChangeType type = 1;
*/
public eraftpb.Eraftpb.ConfChangeType getType() {
eraftpb.Eraftpb.ConfChangeType result = eraftpb.Eraftpb.ConfChangeType.valueOf(type_);
return result == null ? eraftpb.Eraftpb.ConfChangeType.UNRECOGNIZED : result;
}
public static final int NODE_ID_FIELD_NUMBER = 2;
private long nodeId_;
/**
* uint64 node_id = 2;
*/
public long getNodeId() {
return nodeId_;
}
private byte memoizedIsInitialized = -1;
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (type_ != eraftpb.Eraftpb.ConfChangeType.AddNode.getNumber()) {
output.writeEnum(1, type_);
}
if (nodeId_ != 0L) {
output.writeUInt64(2, nodeId_);
}
unknownFields.writeTo(output);
}
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (type_ != eraftpb.Eraftpb.ConfChangeType.AddNode.getNumber()) {
size += com.google.protobuf.CodedOutputStream
.computeEnumSize(1, type_);
}
if (nodeId_ != 0L) {
size += com.google.protobuf.CodedOutputStream
.computeUInt64Size(2, nodeId_);
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof eraftpb.Eraftpb.ConfChangeSingle)) {
return super.equals(obj);
}
eraftpb.Eraftpb.ConfChangeSingle other = (eraftpb.Eraftpb.ConfChangeSingle) obj;
boolean result = true;
result = result && type_ == other.type_;
result = result && (getNodeId()
== other.getNodeId());
result = result && unknownFields.equals(other.unknownFields);
return result;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
hash = (37 * hash) + TYPE_FIELD_NUMBER;
hash = (53 * hash) + type_;
hash = (37 * hash) + NODE_ID_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
getNodeId());
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static eraftpb.Eraftpb.ConfChangeSingle parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static eraftpb.Eraftpb.ConfChangeSingle parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static eraftpb.Eraftpb.ConfChangeSingle parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static eraftpb.Eraftpb.ConfChangeSingle parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static eraftpb.Eraftpb.ConfChangeSingle parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static eraftpb.Eraftpb.ConfChangeSingle parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static eraftpb.Eraftpb.ConfChangeSingle parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static eraftpb.Eraftpb.ConfChangeSingle parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static eraftpb.Eraftpb.ConfChangeSingle parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static eraftpb.Eraftpb.ConfChangeSingle parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static eraftpb.Eraftpb.ConfChangeSingle parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static eraftpb.Eraftpb.ConfChangeSingle parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(eraftpb.Eraftpb.ConfChangeSingle prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
* ConfChangeSingle is an individual configuration change operation. Multiple
* such operations can be carried out atomically via a ConfChangeV2.
*
*
* Protobuf type {@code eraftpb.ConfChangeSingle}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:eraftpb.ConfChangeSingle)
eraftpb.Eraftpb.ConfChangeSingleOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return eraftpb.Eraftpb.internal_static_eraftpb_ConfChangeSingle_descriptor;
}
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return eraftpb.Eraftpb.internal_static_eraftpb_ConfChangeSingle_fieldAccessorTable
.ensureFieldAccessorsInitialized(
eraftpb.Eraftpb.ConfChangeSingle.class, eraftpb.Eraftpb.ConfChangeSingle.Builder.class);
}
// Construct using eraftpb.Eraftpb.ConfChangeSingle.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
public Builder clear() {
super.clear();
type_ = 0;
nodeId_ = 0L;
return this;
}
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return eraftpb.Eraftpb.internal_static_eraftpb_ConfChangeSingle_descriptor;
}
public eraftpb.Eraftpb.ConfChangeSingle getDefaultInstanceForType() {
return eraftpb.Eraftpb.ConfChangeSingle.getDefaultInstance();
}
public eraftpb.Eraftpb.ConfChangeSingle build() {
eraftpb.Eraftpb.ConfChangeSingle result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
public eraftpb.Eraftpb.ConfChangeSingle buildPartial() {
eraftpb.Eraftpb.ConfChangeSingle result = new eraftpb.Eraftpb.ConfChangeSingle(this);
result.type_ = type_;
result.nodeId_ = nodeId_;
onBuilt();
return result;
}
public Builder clone() {
return (Builder) super.clone();
}
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.setField(field, value);
}
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return (Builder) super.clearField(field);
}
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return (Builder) super.clearOneof(oneof);
}
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return (Builder) super.setRepeatedField(field, index, value);
}
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.addRepeatedField(field, value);
}
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof eraftpb.Eraftpb.ConfChangeSingle) {
return mergeFrom((eraftpb.Eraftpb.ConfChangeSingle)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(eraftpb.Eraftpb.ConfChangeSingle other) {
if (other == eraftpb.Eraftpb.ConfChangeSingle.getDefaultInstance()) return this;
if (other.type_ != 0) {
setTypeValue(other.getTypeValue());
}
if (other.getNodeId() != 0L) {
setNodeId(other.getNodeId());
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
public final boolean isInitialized() {
return true;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
eraftpb.Eraftpb.ConfChangeSingle parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (eraftpb.Eraftpb.ConfChangeSingle) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int type_ = 0;
/**
* .eraftpb.ConfChangeType type = 1;
*/
public int getTypeValue() {
return type_;
}
/**
* .eraftpb.ConfChangeType type = 1;
*/
public Builder setTypeValue(int value) {
type_ = value;
onChanged();
return this;
}
/**
* .eraftpb.ConfChangeType type = 1;
*/
public eraftpb.Eraftpb.ConfChangeType getType() {
eraftpb.Eraftpb.ConfChangeType result = eraftpb.Eraftpb.ConfChangeType.valueOf(type_);
return result == null ? eraftpb.Eraftpb.ConfChangeType.UNRECOGNIZED : result;
}
/**
* .eraftpb.ConfChangeType type = 1;
*/
public Builder setType(eraftpb.Eraftpb.ConfChangeType value) {
if (value == null) {
throw new NullPointerException();
}
type_ = value.getNumber();
onChanged();
return this;
}
/**
* .eraftpb.ConfChangeType type = 1;
*/
public Builder clearType() {
type_ = 0;
onChanged();
return this;
}
private long nodeId_ ;
/**
* uint64 node_id = 2;
*/
public long getNodeId() {
return nodeId_;
}
/**
* uint64 node_id = 2;
*/
public Builder setNodeId(long value) {
nodeId_ = value;
onChanged();
return this;
}
/**
* uint64 node_id = 2;
*/
public Builder clearNodeId() {
nodeId_ = 0L;
onChanged();
return this;
}
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFieldsProto3(unknownFields);
}
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:eraftpb.ConfChangeSingle)
}
// @@protoc_insertion_point(class_scope:eraftpb.ConfChangeSingle)
private static final eraftpb.Eraftpb.ConfChangeSingle DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new eraftpb.Eraftpb.ConfChangeSingle();
}
public static eraftpb.Eraftpb.ConfChangeSingle getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
public ConfChangeSingle parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new ConfChangeSingle(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
public eraftpb.Eraftpb.ConfChangeSingle getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface ConfChangeV2OrBuilder extends
// @@protoc_insertion_point(interface_extends:eraftpb.ConfChangeV2)
com.google.protobuf.MessageOrBuilder {
/**
* .eraftpb.ConfChangeTransition transition = 1;
*/
int getTransitionValue();
/**
* .eraftpb.ConfChangeTransition transition = 1;
*/
eraftpb.Eraftpb.ConfChangeTransition getTransition();
/**
* repeated .eraftpb.ConfChangeSingle changes = 2;
*/
java.util.List
getChangesList();
/**
* repeated .eraftpb.ConfChangeSingle changes = 2;
*/
eraftpb.Eraftpb.ConfChangeSingle getChanges(int index);
/**
* repeated .eraftpb.ConfChangeSingle changes = 2;
*/
int getChangesCount();
/**
* repeated .eraftpb.ConfChangeSingle changes = 2;
*/
java.util.List extends eraftpb.Eraftpb.ConfChangeSingleOrBuilder>
getChangesOrBuilderList();
/**
* repeated .eraftpb.ConfChangeSingle changes = 2;
*/
eraftpb.Eraftpb.ConfChangeSingleOrBuilder getChangesOrBuilder(
int index);
/**
* bytes context = 3;
*/
com.google.protobuf.ByteString getContext();
}
/**
*
* ConfChangeV2 messages initiate configuration changes. They support both the
* simple "one at a time" membership change protocol and full Joint Consensus
* allowing for arbitrary changes in membership.
* The supplied context is treated as an opaque payload and can be used to
* attach an action on the state machine to the application of the config change
* proposal. Note that contrary to Joint Consensus as outlined in the Raft
* paper[1], configuration changes become active when they are *applied* to the
* state machine (not when they are appended to the log).
* The simple protocol can be used whenever only a single change is made.
* Non-simple changes require the use of Joint Consensus, for which two
* configuration changes are run. The first configuration change specifies the
* desired changes and transitions the Raft group into the joint configuration,
* in which quorum requires a majority of both the pre-changes and post-changes
* configuration. Joint Consensus avoids entering fragile intermediate
* configurations that could compromise survivability. For example, without the
* use of Joint Consensus and running across three availability zones with a
* replication factor of three, it is not possible to replace a voter without
* entering an intermediate configuration that does not survive the outage of
* one availability zone.
* The provided ConfChangeTransition specifies how (and whether) Joint Consensus
* is used, and assigns the task of leaving the joint configuration either to
* Raft or the application. Leaving the joint configuration is accomplished by
* proposing a ConfChangeV2 with only and optionally the Context field
* populated.
* For details on Raft membership changes, see:
* [1]: https://github.com/ongardie/dissertation/blob/master/online-trim.pdf
*
*
* Protobuf type {@code eraftpb.ConfChangeV2}
*/
public static final class ConfChangeV2 extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:eraftpb.ConfChangeV2)
ConfChangeV2OrBuilder {
private static final long serialVersionUID = 0L;
// Use ConfChangeV2.newBuilder() to construct.
private ConfChangeV2(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private ConfChangeV2() {
transition_ = 0;
changes_ = java.util.Collections.emptyList();
context_ = com.google.protobuf.ByteString.EMPTY;
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private ConfChangeV2(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
default: {
if (!parseUnknownFieldProto3(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
case 8: {
int rawValue = input.readEnum();
transition_ = rawValue;
break;
}
case 18: {
if (!((mutable_bitField0_ & 0x00000002) == 0x00000002)) {
changes_ = new java.util.ArrayList();
mutable_bitField0_ |= 0x00000002;
}
changes_.add(
input.readMessage(eraftpb.Eraftpb.ConfChangeSingle.parser(), extensionRegistry));
break;
}
case 26: {
context_ = input.readBytes();
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
if (((mutable_bitField0_ & 0x00000002) == 0x00000002)) {
changes_ = java.util.Collections.unmodifiableList(changes_);
}
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return eraftpb.Eraftpb.internal_static_eraftpb_ConfChangeV2_descriptor;
}
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return eraftpb.Eraftpb.internal_static_eraftpb_ConfChangeV2_fieldAccessorTable
.ensureFieldAccessorsInitialized(
eraftpb.Eraftpb.ConfChangeV2.class, eraftpb.Eraftpb.ConfChangeV2.Builder.class);
}
private int bitField0_;
public static final int TRANSITION_FIELD_NUMBER = 1;
private int transition_;
/**
* .eraftpb.ConfChangeTransition transition = 1;
*/
public int getTransitionValue() {
return transition_;
}
/**
* .eraftpb.ConfChangeTransition transition = 1;
*/
public eraftpb.Eraftpb.ConfChangeTransition getTransition() {
eraftpb.Eraftpb.ConfChangeTransition result = eraftpb.Eraftpb.ConfChangeTransition.valueOf(transition_);
return result == null ? eraftpb.Eraftpb.ConfChangeTransition.UNRECOGNIZED : result;
}
public static final int CHANGES_FIELD_NUMBER = 2;
private java.util.List changes_;
/**
* repeated .eraftpb.ConfChangeSingle changes = 2;
*/
public java.util.List getChangesList() {
return changes_;
}
/**
* repeated .eraftpb.ConfChangeSingle changes = 2;
*/
public java.util.List extends eraftpb.Eraftpb.ConfChangeSingleOrBuilder>
getChangesOrBuilderList() {
return changes_;
}
/**
* repeated .eraftpb.ConfChangeSingle changes = 2;
*/
public int getChangesCount() {
return changes_.size();
}
/**
* repeated .eraftpb.ConfChangeSingle changes = 2;
*/
public eraftpb.Eraftpb.ConfChangeSingle getChanges(int index) {
return changes_.get(index);
}
/**
* repeated .eraftpb.ConfChangeSingle changes = 2;
*/
public eraftpb.Eraftpb.ConfChangeSingleOrBuilder getChangesOrBuilder(
int index) {
return changes_.get(index);
}
public static final int CONTEXT_FIELD_NUMBER = 3;
private com.google.protobuf.ByteString context_;
/**
* bytes context = 3;
*/
public com.google.protobuf.ByteString getContext() {
return context_;
}
private byte memoizedIsInitialized = -1;
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (transition_ != eraftpb.Eraftpb.ConfChangeTransition.Auto.getNumber()) {
output.writeEnum(1, transition_);
}
for (int i = 0; i < changes_.size(); i++) {
output.writeMessage(2, changes_.get(i));
}
if (!context_.isEmpty()) {
output.writeBytes(3, context_);
}
unknownFields.writeTo(output);
}
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (transition_ != eraftpb.Eraftpb.ConfChangeTransition.Auto.getNumber()) {
size += com.google.protobuf.CodedOutputStream
.computeEnumSize(1, transition_);
}
for (int i = 0; i < changes_.size(); i++) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(2, changes_.get(i));
}
if (!context_.isEmpty()) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(3, context_);
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof eraftpb.Eraftpb.ConfChangeV2)) {
return super.equals(obj);
}
eraftpb.Eraftpb.ConfChangeV2 other = (eraftpb.Eraftpb.ConfChangeV2) obj;
boolean result = true;
result = result && transition_ == other.transition_;
result = result && getChangesList()
.equals(other.getChangesList());
result = result && getContext()
.equals(other.getContext());
result = result && unknownFields.equals(other.unknownFields);
return result;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
hash = (37 * hash) + TRANSITION_FIELD_NUMBER;
hash = (53 * hash) + transition_;
if (getChangesCount() > 0) {
hash = (37 * hash) + CHANGES_FIELD_NUMBER;
hash = (53 * hash) + getChangesList().hashCode();
}
hash = (37 * hash) + CONTEXT_FIELD_NUMBER;
hash = (53 * hash) + getContext().hashCode();
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static eraftpb.Eraftpb.ConfChangeV2 parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static eraftpb.Eraftpb.ConfChangeV2 parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static eraftpb.Eraftpb.ConfChangeV2 parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static eraftpb.Eraftpb.ConfChangeV2 parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static eraftpb.Eraftpb.ConfChangeV2 parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static eraftpb.Eraftpb.ConfChangeV2 parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static eraftpb.Eraftpb.ConfChangeV2 parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static eraftpb.Eraftpb.ConfChangeV2 parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static eraftpb.Eraftpb.ConfChangeV2 parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static eraftpb.Eraftpb.ConfChangeV2 parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static eraftpb.Eraftpb.ConfChangeV2 parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static eraftpb.Eraftpb.ConfChangeV2 parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(eraftpb.Eraftpb.ConfChangeV2 prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
* ConfChangeV2 messages initiate configuration changes. They support both the
* simple "one at a time" membership change protocol and full Joint Consensus
* allowing for arbitrary changes in membership.
* The supplied context is treated as an opaque payload and can be used to
* attach an action on the state machine to the application of the config change
* proposal. Note that contrary to Joint Consensus as outlined in the Raft
* paper[1], configuration changes become active when they are *applied* to the
* state machine (not when they are appended to the log).
* The simple protocol can be used whenever only a single change is made.
* Non-simple changes require the use of Joint Consensus, for which two
* configuration changes are run. The first configuration change specifies the
* desired changes and transitions the Raft group into the joint configuration,
* in which quorum requires a majority of both the pre-changes and post-changes
* configuration. Joint Consensus avoids entering fragile intermediate
* configurations that could compromise survivability. For example, without the
* use of Joint Consensus and running across three availability zones with a
* replication factor of three, it is not possible to replace a voter without
* entering an intermediate configuration that does not survive the outage of
* one availability zone.
* The provided ConfChangeTransition specifies how (and whether) Joint Consensus
* is used, and assigns the task of leaving the joint configuration either to
* Raft or the application. Leaving the joint configuration is accomplished by
* proposing a ConfChangeV2 with only and optionally the Context field
* populated.
* For details on Raft membership changes, see:
* [1]: https://github.com/ongardie/dissertation/blob/master/online-trim.pdf
*
*
* Protobuf type {@code eraftpb.ConfChangeV2}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:eraftpb.ConfChangeV2)
eraftpb.Eraftpb.ConfChangeV2OrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return eraftpb.Eraftpb.internal_static_eraftpb_ConfChangeV2_descriptor;
}
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return eraftpb.Eraftpb.internal_static_eraftpb_ConfChangeV2_fieldAccessorTable
.ensureFieldAccessorsInitialized(
eraftpb.Eraftpb.ConfChangeV2.class, eraftpb.Eraftpb.ConfChangeV2.Builder.class);
}
// Construct using eraftpb.Eraftpb.ConfChangeV2.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
getChangesFieldBuilder();
}
}
public Builder clear() {
super.clear();
transition_ = 0;
if (changesBuilder_ == null) {
changes_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000002);
} else {
changesBuilder_.clear();
}
context_ = com.google.protobuf.ByteString.EMPTY;
return this;
}
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return eraftpb.Eraftpb.internal_static_eraftpb_ConfChangeV2_descriptor;
}
public eraftpb.Eraftpb.ConfChangeV2 getDefaultInstanceForType() {
return eraftpb.Eraftpb.ConfChangeV2.getDefaultInstance();
}
public eraftpb.Eraftpb.ConfChangeV2 build() {
eraftpb.Eraftpb.ConfChangeV2 result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
public eraftpb.Eraftpb.ConfChangeV2 buildPartial() {
eraftpb.Eraftpb.ConfChangeV2 result = new eraftpb.Eraftpb.ConfChangeV2(this);
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
result.transition_ = transition_;
if (changesBuilder_ == null) {
if (((bitField0_ & 0x00000002) == 0x00000002)) {
changes_ = java.util.Collections.unmodifiableList(changes_);
bitField0_ = (bitField0_ & ~0x00000002);
}
result.changes_ = changes_;
} else {
result.changes_ = changesBuilder_.build();
}
result.context_ = context_;
result.bitField0_ = to_bitField0_;
onBuilt();
return result;
}
public Builder clone() {
return (Builder) super.clone();
}
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.setField(field, value);
}
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return (Builder) super.clearField(field);
}
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return (Builder) super.clearOneof(oneof);
}
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return (Builder) super.setRepeatedField(field, index, value);
}
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.addRepeatedField(field, value);
}
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof eraftpb.Eraftpb.ConfChangeV2) {
return mergeFrom((eraftpb.Eraftpb.ConfChangeV2)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(eraftpb.Eraftpb.ConfChangeV2 other) {
if (other == eraftpb.Eraftpb.ConfChangeV2.getDefaultInstance()) return this;
if (other.transition_ != 0) {
setTransitionValue(other.getTransitionValue());
}
if (changesBuilder_ == null) {
if (!other.changes_.isEmpty()) {
if (changes_.isEmpty()) {
changes_ = other.changes_;
bitField0_ = (bitField0_ & ~0x00000002);
} else {
ensureChangesIsMutable();
changes_.addAll(other.changes_);
}
onChanged();
}
} else {
if (!other.changes_.isEmpty()) {
if (changesBuilder_.isEmpty()) {
changesBuilder_.dispose();
changesBuilder_ = null;
changes_ = other.changes_;
bitField0_ = (bitField0_ & ~0x00000002);
changesBuilder_ =
com.google.protobuf.GeneratedMessageV3.alwaysUseFieldBuilders ?
getChangesFieldBuilder() : null;
} else {
changesBuilder_.addAllMessages(other.changes_);
}
}
}
if (other.getContext() != com.google.protobuf.ByteString.EMPTY) {
setContext(other.getContext());
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
public final boolean isInitialized() {
return true;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
eraftpb.Eraftpb.ConfChangeV2 parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (eraftpb.Eraftpb.ConfChangeV2) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
private int transition_ = 0;
/**
* .eraftpb.ConfChangeTransition transition = 1;
*/
public int getTransitionValue() {
return transition_;
}
/**
* .eraftpb.ConfChangeTransition transition = 1;
*/
public Builder setTransitionValue(int value) {
transition_ = value;
onChanged();
return this;
}
/**
* .eraftpb.ConfChangeTransition transition = 1;
*/
public eraftpb.Eraftpb.ConfChangeTransition getTransition() {
eraftpb.Eraftpb.ConfChangeTransition result = eraftpb.Eraftpb.ConfChangeTransition.valueOf(transition_);
return result == null ? eraftpb.Eraftpb.ConfChangeTransition.UNRECOGNIZED : result;
}
/**
* .eraftpb.ConfChangeTransition transition = 1;
*/
public Builder setTransition(eraftpb.Eraftpb.ConfChangeTransition value) {
if (value == null) {
throw new NullPointerException();
}
transition_ = value.getNumber();
onChanged();
return this;
}
/**
* .eraftpb.ConfChangeTransition transition = 1;
*/
public Builder clearTransition() {
transition_ = 0;
onChanged();
return this;
}
private java.util.List changes_ =
java.util.Collections.emptyList();
private void ensureChangesIsMutable() {
if (!((bitField0_ & 0x00000002) == 0x00000002)) {
changes_ = new java.util.ArrayList(changes_);
bitField0_ |= 0x00000002;
}
}
private com.google.protobuf.RepeatedFieldBuilderV3<
eraftpb.Eraftpb.ConfChangeSingle, eraftpb.Eraftpb.ConfChangeSingle.Builder, eraftpb.Eraftpb.ConfChangeSingleOrBuilder> changesBuilder_;
/**
* repeated .eraftpb.ConfChangeSingle changes = 2;
*/
public java.util.List getChangesList() {
if (changesBuilder_ == null) {
return java.util.Collections.unmodifiableList(changes_);
} else {
return changesBuilder_.getMessageList();
}
}
/**
* repeated .eraftpb.ConfChangeSingle changes = 2;
*/
public int getChangesCount() {
if (changesBuilder_ == null) {
return changes_.size();
} else {
return changesBuilder_.getCount();
}
}
/**
* repeated .eraftpb.ConfChangeSingle changes = 2;
*/
public eraftpb.Eraftpb.ConfChangeSingle getChanges(int index) {
if (changesBuilder_ == null) {
return changes_.get(index);
} else {
return changesBuilder_.getMessage(index);
}
}
/**
* repeated .eraftpb.ConfChangeSingle changes = 2;
*/
public Builder setChanges(
int index, eraftpb.Eraftpb.ConfChangeSingle value) {
if (changesBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureChangesIsMutable();
changes_.set(index, value);
onChanged();
} else {
changesBuilder_.setMessage(index, value);
}
return this;
}
/**
* repeated .eraftpb.ConfChangeSingle changes = 2;
*/
public Builder setChanges(
int index, eraftpb.Eraftpb.ConfChangeSingle.Builder builderForValue) {
if (changesBuilder_ == null) {
ensureChangesIsMutable();
changes_.set(index, builderForValue.build());
onChanged();
} else {
changesBuilder_.setMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .eraftpb.ConfChangeSingle changes = 2;
*/
public Builder addChanges(eraftpb.Eraftpb.ConfChangeSingle value) {
if (changesBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureChangesIsMutable();
changes_.add(value);
onChanged();
} else {
changesBuilder_.addMessage(value);
}
return this;
}
/**
* repeated .eraftpb.ConfChangeSingle changes = 2;
*/
public Builder addChanges(
int index, eraftpb.Eraftpb.ConfChangeSingle value) {
if (changesBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureChangesIsMutable();
changes_.add(index, value);
onChanged();
} else {
changesBuilder_.addMessage(index, value);
}
return this;
}
/**
* repeated .eraftpb.ConfChangeSingle changes = 2;
*/
public Builder addChanges(
eraftpb.Eraftpb.ConfChangeSingle.Builder builderForValue) {
if (changesBuilder_ == null) {
ensureChangesIsMutable();
changes_.add(builderForValue.build());
onChanged();
} else {
changesBuilder_.addMessage(builderForValue.build());
}
return this;
}
/**
* repeated .eraftpb.ConfChangeSingle changes = 2;
*/
public Builder addChanges(
int index, eraftpb.Eraftpb.ConfChangeSingle.Builder builderForValue) {
if (changesBuilder_ == null) {
ensureChangesIsMutable();
changes_.add(index, builderForValue.build());
onChanged();
} else {
changesBuilder_.addMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .eraftpb.ConfChangeSingle changes = 2;
*/
public Builder addAllChanges(
java.lang.Iterable extends eraftpb.Eraftpb.ConfChangeSingle> values) {
if (changesBuilder_ == null) {
ensureChangesIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, changes_);
onChanged();
} else {
changesBuilder_.addAllMessages(values);
}
return this;
}
/**
* repeated .eraftpb.ConfChangeSingle changes = 2;
*/
public Builder clearChanges() {
if (changesBuilder_ == null) {
changes_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000002);
onChanged();
} else {
changesBuilder_.clear();
}
return this;
}
/**
* repeated .eraftpb.ConfChangeSingle changes = 2;
*/
public Builder removeChanges(int index) {
if (changesBuilder_ == null) {
ensureChangesIsMutable();
changes_.remove(index);
onChanged();
} else {
changesBuilder_.remove(index);
}
return this;
}
/**
* repeated .eraftpb.ConfChangeSingle changes = 2;
*/
public eraftpb.Eraftpb.ConfChangeSingle.Builder getChangesBuilder(
int index) {
return getChangesFieldBuilder().getBuilder(index);
}
/**
* repeated .eraftpb.ConfChangeSingle changes = 2;
*/
public eraftpb.Eraftpb.ConfChangeSingleOrBuilder getChangesOrBuilder(
int index) {
if (changesBuilder_ == null) {
return changes_.get(index); } else {
return changesBuilder_.getMessageOrBuilder(index);
}
}
/**
* repeated .eraftpb.ConfChangeSingle changes = 2;
*/
public java.util.List extends eraftpb.Eraftpb.ConfChangeSingleOrBuilder>
getChangesOrBuilderList() {
if (changesBuilder_ != null) {
return changesBuilder_.getMessageOrBuilderList();
} else {
return java.util.Collections.unmodifiableList(changes_);
}
}
/**
* repeated .eraftpb.ConfChangeSingle changes = 2;
*/
public eraftpb.Eraftpb.ConfChangeSingle.Builder addChangesBuilder() {
return getChangesFieldBuilder().addBuilder(
eraftpb.Eraftpb.ConfChangeSingle.getDefaultInstance());
}
/**
* repeated .eraftpb.ConfChangeSingle changes = 2;
*/
public eraftpb.Eraftpb.ConfChangeSingle.Builder addChangesBuilder(
int index) {
return getChangesFieldBuilder().addBuilder(
index, eraftpb.Eraftpb.ConfChangeSingle.getDefaultInstance());
}
/**
* repeated .eraftpb.ConfChangeSingle changes = 2;
*/
public java.util.List
getChangesBuilderList() {
return getChangesFieldBuilder().getBuilderList();
}
private com.google.protobuf.RepeatedFieldBuilderV3<
eraftpb.Eraftpb.ConfChangeSingle, eraftpb.Eraftpb.ConfChangeSingle.Builder, eraftpb.Eraftpb.ConfChangeSingleOrBuilder>
getChangesFieldBuilder() {
if (changesBuilder_ == null) {
changesBuilder_ = new com.google.protobuf.RepeatedFieldBuilderV3<
eraftpb.Eraftpb.ConfChangeSingle, eraftpb.Eraftpb.ConfChangeSingle.Builder, eraftpb.Eraftpb.ConfChangeSingleOrBuilder>(
changes_,
((bitField0_ & 0x00000002) == 0x00000002),
getParentForChildren(),
isClean());
changes_ = null;
}
return changesBuilder_;
}
private com.google.protobuf.ByteString context_ = com.google.protobuf.ByteString.EMPTY;
/**
* bytes context = 3;
*/
public com.google.protobuf.ByteString getContext() {
return context_;
}
/**
* bytes context = 3;
*/
public Builder setContext(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
context_ = value;
onChanged();
return this;
}
/**
* bytes context = 3;
*/
public Builder clearContext() {
context_ = getDefaultInstance().getContext();
onChanged();
return this;
}
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFieldsProto3(unknownFields);
}
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:eraftpb.ConfChangeV2)
}
// @@protoc_insertion_point(class_scope:eraftpb.ConfChangeV2)
private static final eraftpb.Eraftpb.ConfChangeV2 DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new eraftpb.Eraftpb.ConfChangeV2();
}
public static eraftpb.Eraftpb.ConfChangeV2 getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
public ConfChangeV2 parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new ConfChangeV2(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
public eraftpb.Eraftpb.ConfChangeV2 getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_eraftpb_Entry_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_eraftpb_Entry_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_eraftpb_SnapshotMetadata_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_eraftpb_SnapshotMetadata_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_eraftpb_Snapshot_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_eraftpb_Snapshot_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_eraftpb_Message_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_eraftpb_Message_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_eraftpb_HardState_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_eraftpb_HardState_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_eraftpb_ConfState_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_eraftpb_ConfState_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_eraftpb_ConfChange_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_eraftpb_ConfChange_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_eraftpb_ConfChangeSingle_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_eraftpb_ConfChangeSingle_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_eraftpb_ConfChangeV2_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_eraftpb_ConfChangeV2_fieldAccessorTable;
public static com.google.protobuf.Descriptors.FileDescriptor
getDescriptor() {
return descriptor;
}
private static com.google.protobuf.Descriptors.FileDescriptor
descriptor;
static {
java.lang.String[] descriptorData = {
"\n\reraftpb.proto\022\007eraftpb\"}\n\005Entry\022&\n\nent" +
"ry_type\030\001 \001(\0162\022.eraftpb.EntryType\022\014\n\004ter" +
"m\030\002 \001(\004\022\r\n\005index\030\003 \001(\004\022\014\n\004data\030\004 \001(\014\022\017\n\007" +
"context\030\006 \001(\014\022\020\n\010sync_log\030\005 \001(\010\"W\n\020Snaps" +
"hotMetadata\022&\n\nconf_state\030\001 \001(\0132\022.eraftp" +
"b.ConfState\022\r\n\005index\030\002 \001(\004\022\014\n\004term\030\003 \001(\004" +
"\"E\n\010Snapshot\022\014\n\004data\030\001 \001(\014\022+\n\010metadata\030\002" +
" \001(\0132\031.eraftpb.SnapshotMetadata\"\240\002\n\007Mess" +
"age\022&\n\010msg_type\030\001 \001(\0162\024.eraftpb.MessageT" +
"ype\022\n\n\002to\030\002 \001(\004\022\014\n\004from\030\003 \001(\004\022\014\n\004term\030\004 " +
"\001(\004\022\020\n\010log_term\030\005 \001(\004\022\r\n\005index\030\006 \001(\004\022\037\n\007" +
"entries\030\007 \003(\0132\016.eraftpb.Entry\022\016\n\006commit\030" +
"\010 \001(\004\022#\n\010snapshot\030\t \001(\0132\021.eraftpb.Snapsh" +
"ot\022\030\n\020request_snapshot\030\r \001(\004\022\016\n\006reject\030\n" +
" \001(\010\022\023\n\013reject_hint\030\013 \001(\004\022\017\n\007context\030\014 \001" +
"(\014\"7\n\tHardState\022\014\n\004term\030\001 \001(\004\022\014\n\004vote\030\002 " +
"\001(\004\022\016\n\006commit\030\003 \001(\004\"q\n\tConfState\022\016\n\006vote" +
"rs\030\001 \003(\004\022\020\n\010learners\030\002 \003(\004\022\027\n\017voters_out" +
"going\030\003 \003(\004\022\025\n\rlearners_next\030\004 \003(\004\022\022\n\nau" +
"to_leave\030\005 \001(\010\"h\n\nConfChange\022,\n\013change_t" +
"ype\030\002 \001(\0162\027.eraftpb.ConfChangeType\022\017\n\007no" +
"de_id\030\003 \001(\004\022\017\n\007context\030\004 \001(\014\022\n\n\002id\030\001 \001(\004" +
"\"J\n\020ConfChangeSingle\022%\n\004type\030\001 \001(\0162\027.era" +
"ftpb.ConfChangeType\022\017\n\007node_id\030\002 \001(\004\"~\n\014" +
"ConfChangeV2\0221\n\ntransition\030\001 \001(\0162\035.eraft" +
"pb.ConfChangeTransition\022*\n\007changes\030\002 \003(\013" +
"2\031.eraftpb.ConfChangeSingle\022\017\n\007context\030\003" +
" \001(\014*H\n\tEntryType\022\017\n\013EntryNormal\020\000\022\023\n\017En" +
"tryConfChange\020\001\022\025\n\021EntryConfChangeV2\020\002*\214" +
"\003\n\013MessageType\022\n\n\006MsgHup\020\000\022\013\n\007MsgBeat\020\001\022" +
"\016\n\nMsgPropose\020\002\022\r\n\tMsgAppend\020\003\022\025\n\021MsgApp" +
"endResponse\020\004\022\022\n\016MsgRequestVote\020\005\022\032\n\026Msg" +
"RequestVoteResponse\020\006\022\017\n\013MsgSnapshot\020\007\022\020" +
"\n\014MsgHeartbeat\020\010\022\030\n\024MsgHeartbeatResponse" +
"\020\t\022\022\n\016MsgUnreachable\020\n\022\021\n\rMsgSnapStatus\020" +
"\013\022\022\n\016MsgCheckQuorum\020\014\022\025\n\021MsgTransferLead" +
"er\020\r\022\021\n\rMsgTimeoutNow\020\016\022\020\n\014MsgReadIndex\020" +
"\017\022\024\n\020MsgReadIndexResp\020\020\022\025\n\021MsgRequestPre" +
"Vote\020\021\022\035\n\031MsgRequestPreVoteResponse\020\022*<\n" +
"\024ConfChangeTransition\022\010\n\004Auto\020\000\022\014\n\010Impli" +
"cit\020\001\022\014\n\010Explicit\020\002*A\n\016ConfChangeType\022\013\n" +
"\007AddNode\020\000\022\016\n\nRemoveNode\020\001\022\022\n\016AddLearner" +
"Node\020\002b\006proto3"
};
com.google.protobuf.Descriptors.FileDescriptor.InternalDescriptorAssigner assigner =
new com.google.protobuf.Descriptors.FileDescriptor. InternalDescriptorAssigner() {
public com.google.protobuf.ExtensionRegistry assignDescriptors(
com.google.protobuf.Descriptors.FileDescriptor root) {
descriptor = root;
return null;
}
};
com.google.protobuf.Descriptors.FileDescriptor
.internalBuildGeneratedFileFrom(descriptorData,
new com.google.protobuf.Descriptors.FileDescriptor[] {
}, assigner);
internal_static_eraftpb_Entry_descriptor =
getDescriptor().getMessageTypes().get(0);
internal_static_eraftpb_Entry_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_eraftpb_Entry_descriptor,
new java.lang.String[] { "EntryType", "Term", "Index", "Data", "Context", "SyncLog", });
internal_static_eraftpb_SnapshotMetadata_descriptor =
getDescriptor().getMessageTypes().get(1);
internal_static_eraftpb_SnapshotMetadata_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_eraftpb_SnapshotMetadata_descriptor,
new java.lang.String[] { "ConfState", "Index", "Term", });
internal_static_eraftpb_Snapshot_descriptor =
getDescriptor().getMessageTypes().get(2);
internal_static_eraftpb_Snapshot_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_eraftpb_Snapshot_descriptor,
new java.lang.String[] { "Data", "Metadata", });
internal_static_eraftpb_Message_descriptor =
getDescriptor().getMessageTypes().get(3);
internal_static_eraftpb_Message_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_eraftpb_Message_descriptor,
new java.lang.String[] { "MsgType", "To", "From", "Term", "LogTerm", "Index", "Entries", "Commit", "Snapshot", "RequestSnapshot", "Reject", "RejectHint", "Context", });
internal_static_eraftpb_HardState_descriptor =
getDescriptor().getMessageTypes().get(4);
internal_static_eraftpb_HardState_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_eraftpb_HardState_descriptor,
new java.lang.String[] { "Term", "Vote", "Commit", });
internal_static_eraftpb_ConfState_descriptor =
getDescriptor().getMessageTypes().get(5);
internal_static_eraftpb_ConfState_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_eraftpb_ConfState_descriptor,
new java.lang.String[] { "Voters", "Learners", "VotersOutgoing", "LearnersNext", "AutoLeave", });
internal_static_eraftpb_ConfChange_descriptor =
getDescriptor().getMessageTypes().get(6);
internal_static_eraftpb_ConfChange_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_eraftpb_ConfChange_descriptor,
new java.lang.String[] { "ChangeType", "NodeId", "Context", "Id", });
internal_static_eraftpb_ConfChangeSingle_descriptor =
getDescriptor().getMessageTypes().get(7);
internal_static_eraftpb_ConfChangeSingle_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_eraftpb_ConfChangeSingle_descriptor,
new java.lang.String[] { "Type", "NodeId", });
internal_static_eraftpb_ConfChangeV2_descriptor =
getDescriptor().getMessageTypes().get(8);
internal_static_eraftpb_ConfChangeV2_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_eraftpb_ConfChangeV2_descriptor,
new java.lang.String[] { "Transition", "Changes", "Context", });
}
// @@protoc_insertion_point(outer_class_scope)
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy