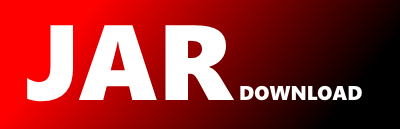
org.tikv.kvproto.DebugGrpc Maven / Gradle / Ivy
package org.tikv.kvproto;
import static io.grpc.MethodDescriptor.generateFullMethodName;
import static io.grpc.stub.ClientCalls.asyncBidiStreamingCall;
import static io.grpc.stub.ClientCalls.asyncClientStreamingCall;
import static io.grpc.stub.ClientCalls.asyncServerStreamingCall;
import static io.grpc.stub.ClientCalls.asyncUnaryCall;
import static io.grpc.stub.ClientCalls.blockingServerStreamingCall;
import static io.grpc.stub.ClientCalls.blockingUnaryCall;
import static io.grpc.stub.ClientCalls.futureUnaryCall;
import static io.grpc.stub.ServerCalls.asyncBidiStreamingCall;
import static io.grpc.stub.ServerCalls.asyncClientStreamingCall;
import static io.grpc.stub.ServerCalls.asyncServerStreamingCall;
import static io.grpc.stub.ServerCalls.asyncUnaryCall;
import static io.grpc.stub.ServerCalls.asyncUnimplementedStreamingCall;
import static io.grpc.stub.ServerCalls.asyncUnimplementedUnaryCall;
/**
*
* Debug service for TiKV.
* Errors are defined as follow:
* - OK: Okay, we are good!
* - UNKNOWN: For unknown error.
* - INVALID_ARGUMENT: Something goes wrong within requests.
* - NOT_FOUND: It is key or region not found, it's based on context, detailed
* reason can be found in grpc message.
* Note: It bypasses raft layer.
*
*/
@javax.annotation.Generated(
value = "by gRPC proto compiler (version 1.29.0)",
comments = "Source: debugpb.proto")
public final class DebugGrpc {
private DebugGrpc() {}
public static final String SERVICE_NAME = "debugpb.Debug";
// Static method descriptors that strictly reflect the proto.
private static volatile io.grpc.MethodDescriptor getGetMethod;
@io.grpc.stub.annotations.RpcMethod(
fullMethodName = SERVICE_NAME + '/' + "Get",
requestType = org.tikv.kvproto.Debugpb.GetRequest.class,
responseType = org.tikv.kvproto.Debugpb.GetResponse.class,
methodType = io.grpc.MethodDescriptor.MethodType.UNARY)
public static io.grpc.MethodDescriptor getGetMethod() {
io.grpc.MethodDescriptor getGetMethod;
if ((getGetMethod = DebugGrpc.getGetMethod) == null) {
synchronized (DebugGrpc.class) {
if ((getGetMethod = DebugGrpc.getGetMethod) == null) {
DebugGrpc.getGetMethod = getGetMethod =
io.grpc.MethodDescriptor.newBuilder()
.setType(io.grpc.MethodDescriptor.MethodType.UNARY)
.setFullMethodName(generateFullMethodName(SERVICE_NAME, "Get"))
.setSampledToLocalTracing(true)
.setRequestMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
org.tikv.kvproto.Debugpb.GetRequest.getDefaultInstance()))
.setResponseMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
org.tikv.kvproto.Debugpb.GetResponse.getDefaultInstance()))
.setSchemaDescriptor(new DebugMethodDescriptorSupplier("Get"))
.build();
}
}
}
return getGetMethod;
}
private static volatile io.grpc.MethodDescriptor getRaftLogMethod;
@io.grpc.stub.annotations.RpcMethod(
fullMethodName = SERVICE_NAME + '/' + "RaftLog",
requestType = org.tikv.kvproto.Debugpb.RaftLogRequest.class,
responseType = org.tikv.kvproto.Debugpb.RaftLogResponse.class,
methodType = io.grpc.MethodDescriptor.MethodType.UNARY)
public static io.grpc.MethodDescriptor getRaftLogMethod() {
io.grpc.MethodDescriptor getRaftLogMethod;
if ((getRaftLogMethod = DebugGrpc.getRaftLogMethod) == null) {
synchronized (DebugGrpc.class) {
if ((getRaftLogMethod = DebugGrpc.getRaftLogMethod) == null) {
DebugGrpc.getRaftLogMethod = getRaftLogMethod =
io.grpc.MethodDescriptor.newBuilder()
.setType(io.grpc.MethodDescriptor.MethodType.UNARY)
.setFullMethodName(generateFullMethodName(SERVICE_NAME, "RaftLog"))
.setSampledToLocalTracing(true)
.setRequestMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
org.tikv.kvproto.Debugpb.RaftLogRequest.getDefaultInstance()))
.setResponseMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
org.tikv.kvproto.Debugpb.RaftLogResponse.getDefaultInstance()))
.setSchemaDescriptor(new DebugMethodDescriptorSupplier("RaftLog"))
.build();
}
}
}
return getRaftLogMethod;
}
private static volatile io.grpc.MethodDescriptor getRegionInfoMethod;
@io.grpc.stub.annotations.RpcMethod(
fullMethodName = SERVICE_NAME + '/' + "RegionInfo",
requestType = org.tikv.kvproto.Debugpb.RegionInfoRequest.class,
responseType = org.tikv.kvproto.Debugpb.RegionInfoResponse.class,
methodType = io.grpc.MethodDescriptor.MethodType.UNARY)
public static io.grpc.MethodDescriptor getRegionInfoMethod() {
io.grpc.MethodDescriptor getRegionInfoMethod;
if ((getRegionInfoMethod = DebugGrpc.getRegionInfoMethod) == null) {
synchronized (DebugGrpc.class) {
if ((getRegionInfoMethod = DebugGrpc.getRegionInfoMethod) == null) {
DebugGrpc.getRegionInfoMethod = getRegionInfoMethod =
io.grpc.MethodDescriptor.newBuilder()
.setType(io.grpc.MethodDescriptor.MethodType.UNARY)
.setFullMethodName(generateFullMethodName(SERVICE_NAME, "RegionInfo"))
.setSampledToLocalTracing(true)
.setRequestMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
org.tikv.kvproto.Debugpb.RegionInfoRequest.getDefaultInstance()))
.setResponseMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
org.tikv.kvproto.Debugpb.RegionInfoResponse.getDefaultInstance()))
.setSchemaDescriptor(new DebugMethodDescriptorSupplier("RegionInfo"))
.build();
}
}
}
return getRegionInfoMethod;
}
private static volatile io.grpc.MethodDescriptor getRegionSizeMethod;
@io.grpc.stub.annotations.RpcMethod(
fullMethodName = SERVICE_NAME + '/' + "RegionSize",
requestType = org.tikv.kvproto.Debugpb.RegionSizeRequest.class,
responseType = org.tikv.kvproto.Debugpb.RegionSizeResponse.class,
methodType = io.grpc.MethodDescriptor.MethodType.UNARY)
public static io.grpc.MethodDescriptor getRegionSizeMethod() {
io.grpc.MethodDescriptor getRegionSizeMethod;
if ((getRegionSizeMethod = DebugGrpc.getRegionSizeMethod) == null) {
synchronized (DebugGrpc.class) {
if ((getRegionSizeMethod = DebugGrpc.getRegionSizeMethod) == null) {
DebugGrpc.getRegionSizeMethod = getRegionSizeMethod =
io.grpc.MethodDescriptor.newBuilder()
.setType(io.grpc.MethodDescriptor.MethodType.UNARY)
.setFullMethodName(generateFullMethodName(SERVICE_NAME, "RegionSize"))
.setSampledToLocalTracing(true)
.setRequestMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
org.tikv.kvproto.Debugpb.RegionSizeRequest.getDefaultInstance()))
.setResponseMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
org.tikv.kvproto.Debugpb.RegionSizeResponse.getDefaultInstance()))
.setSchemaDescriptor(new DebugMethodDescriptorSupplier("RegionSize"))
.build();
}
}
}
return getRegionSizeMethod;
}
private static volatile io.grpc.MethodDescriptor getScanMvccMethod;
@io.grpc.stub.annotations.RpcMethod(
fullMethodName = SERVICE_NAME + '/' + "ScanMvcc",
requestType = org.tikv.kvproto.Debugpb.ScanMvccRequest.class,
responseType = org.tikv.kvproto.Debugpb.ScanMvccResponse.class,
methodType = io.grpc.MethodDescriptor.MethodType.SERVER_STREAMING)
public static io.grpc.MethodDescriptor getScanMvccMethod() {
io.grpc.MethodDescriptor getScanMvccMethod;
if ((getScanMvccMethod = DebugGrpc.getScanMvccMethod) == null) {
synchronized (DebugGrpc.class) {
if ((getScanMvccMethod = DebugGrpc.getScanMvccMethod) == null) {
DebugGrpc.getScanMvccMethod = getScanMvccMethod =
io.grpc.MethodDescriptor.newBuilder()
.setType(io.grpc.MethodDescriptor.MethodType.SERVER_STREAMING)
.setFullMethodName(generateFullMethodName(SERVICE_NAME, "ScanMvcc"))
.setSampledToLocalTracing(true)
.setRequestMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
org.tikv.kvproto.Debugpb.ScanMvccRequest.getDefaultInstance()))
.setResponseMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
org.tikv.kvproto.Debugpb.ScanMvccResponse.getDefaultInstance()))
.setSchemaDescriptor(new DebugMethodDescriptorSupplier("ScanMvcc"))
.build();
}
}
}
return getScanMvccMethod;
}
private static volatile io.grpc.MethodDescriptor getCompactMethod;
@io.grpc.stub.annotations.RpcMethod(
fullMethodName = SERVICE_NAME + '/' + "Compact",
requestType = org.tikv.kvproto.Debugpb.CompactRequest.class,
responseType = org.tikv.kvproto.Debugpb.CompactResponse.class,
methodType = io.grpc.MethodDescriptor.MethodType.UNARY)
public static io.grpc.MethodDescriptor getCompactMethod() {
io.grpc.MethodDescriptor getCompactMethod;
if ((getCompactMethod = DebugGrpc.getCompactMethod) == null) {
synchronized (DebugGrpc.class) {
if ((getCompactMethod = DebugGrpc.getCompactMethod) == null) {
DebugGrpc.getCompactMethod = getCompactMethod =
io.grpc.MethodDescriptor.newBuilder()
.setType(io.grpc.MethodDescriptor.MethodType.UNARY)
.setFullMethodName(generateFullMethodName(SERVICE_NAME, "Compact"))
.setSampledToLocalTracing(true)
.setRequestMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
org.tikv.kvproto.Debugpb.CompactRequest.getDefaultInstance()))
.setResponseMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
org.tikv.kvproto.Debugpb.CompactResponse.getDefaultInstance()))
.setSchemaDescriptor(new DebugMethodDescriptorSupplier("Compact"))
.build();
}
}
}
return getCompactMethod;
}
private static volatile io.grpc.MethodDescriptor getInjectFailPointMethod;
@io.grpc.stub.annotations.RpcMethod(
fullMethodName = SERVICE_NAME + '/' + "InjectFailPoint",
requestType = org.tikv.kvproto.Debugpb.InjectFailPointRequest.class,
responseType = org.tikv.kvproto.Debugpb.InjectFailPointResponse.class,
methodType = io.grpc.MethodDescriptor.MethodType.UNARY)
public static io.grpc.MethodDescriptor getInjectFailPointMethod() {
io.grpc.MethodDescriptor getInjectFailPointMethod;
if ((getInjectFailPointMethod = DebugGrpc.getInjectFailPointMethod) == null) {
synchronized (DebugGrpc.class) {
if ((getInjectFailPointMethod = DebugGrpc.getInjectFailPointMethod) == null) {
DebugGrpc.getInjectFailPointMethod = getInjectFailPointMethod =
io.grpc.MethodDescriptor.newBuilder()
.setType(io.grpc.MethodDescriptor.MethodType.UNARY)
.setFullMethodName(generateFullMethodName(SERVICE_NAME, "InjectFailPoint"))
.setSampledToLocalTracing(true)
.setRequestMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
org.tikv.kvproto.Debugpb.InjectFailPointRequest.getDefaultInstance()))
.setResponseMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
org.tikv.kvproto.Debugpb.InjectFailPointResponse.getDefaultInstance()))
.setSchemaDescriptor(new DebugMethodDescriptorSupplier("InjectFailPoint"))
.build();
}
}
}
return getInjectFailPointMethod;
}
private static volatile io.grpc.MethodDescriptor getRecoverFailPointMethod;
@io.grpc.stub.annotations.RpcMethod(
fullMethodName = SERVICE_NAME + '/' + "RecoverFailPoint",
requestType = org.tikv.kvproto.Debugpb.RecoverFailPointRequest.class,
responseType = org.tikv.kvproto.Debugpb.RecoverFailPointResponse.class,
methodType = io.grpc.MethodDescriptor.MethodType.UNARY)
public static io.grpc.MethodDescriptor getRecoverFailPointMethod() {
io.grpc.MethodDescriptor getRecoverFailPointMethod;
if ((getRecoverFailPointMethod = DebugGrpc.getRecoverFailPointMethod) == null) {
synchronized (DebugGrpc.class) {
if ((getRecoverFailPointMethod = DebugGrpc.getRecoverFailPointMethod) == null) {
DebugGrpc.getRecoverFailPointMethod = getRecoverFailPointMethod =
io.grpc.MethodDescriptor.newBuilder()
.setType(io.grpc.MethodDescriptor.MethodType.UNARY)
.setFullMethodName(generateFullMethodName(SERVICE_NAME, "RecoverFailPoint"))
.setSampledToLocalTracing(true)
.setRequestMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
org.tikv.kvproto.Debugpb.RecoverFailPointRequest.getDefaultInstance()))
.setResponseMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
org.tikv.kvproto.Debugpb.RecoverFailPointResponse.getDefaultInstance()))
.setSchemaDescriptor(new DebugMethodDescriptorSupplier("RecoverFailPoint"))
.build();
}
}
}
return getRecoverFailPointMethod;
}
private static volatile io.grpc.MethodDescriptor getListFailPointsMethod;
@io.grpc.stub.annotations.RpcMethod(
fullMethodName = SERVICE_NAME + '/' + "ListFailPoints",
requestType = org.tikv.kvproto.Debugpb.ListFailPointsRequest.class,
responseType = org.tikv.kvproto.Debugpb.ListFailPointsResponse.class,
methodType = io.grpc.MethodDescriptor.MethodType.UNARY)
public static io.grpc.MethodDescriptor getListFailPointsMethod() {
io.grpc.MethodDescriptor getListFailPointsMethod;
if ((getListFailPointsMethod = DebugGrpc.getListFailPointsMethod) == null) {
synchronized (DebugGrpc.class) {
if ((getListFailPointsMethod = DebugGrpc.getListFailPointsMethod) == null) {
DebugGrpc.getListFailPointsMethod = getListFailPointsMethod =
io.grpc.MethodDescriptor.newBuilder()
.setType(io.grpc.MethodDescriptor.MethodType.UNARY)
.setFullMethodName(generateFullMethodName(SERVICE_NAME, "ListFailPoints"))
.setSampledToLocalTracing(true)
.setRequestMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
org.tikv.kvproto.Debugpb.ListFailPointsRequest.getDefaultInstance()))
.setResponseMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
org.tikv.kvproto.Debugpb.ListFailPointsResponse.getDefaultInstance()))
.setSchemaDescriptor(new DebugMethodDescriptorSupplier("ListFailPoints"))
.build();
}
}
}
return getListFailPointsMethod;
}
private static volatile io.grpc.MethodDescriptor getGetMetricsMethod;
@io.grpc.stub.annotations.RpcMethod(
fullMethodName = SERVICE_NAME + '/' + "GetMetrics",
requestType = org.tikv.kvproto.Debugpb.GetMetricsRequest.class,
responseType = org.tikv.kvproto.Debugpb.GetMetricsResponse.class,
methodType = io.grpc.MethodDescriptor.MethodType.UNARY)
public static io.grpc.MethodDescriptor getGetMetricsMethod() {
io.grpc.MethodDescriptor getGetMetricsMethod;
if ((getGetMetricsMethod = DebugGrpc.getGetMetricsMethod) == null) {
synchronized (DebugGrpc.class) {
if ((getGetMetricsMethod = DebugGrpc.getGetMetricsMethod) == null) {
DebugGrpc.getGetMetricsMethod = getGetMetricsMethod =
io.grpc.MethodDescriptor.newBuilder()
.setType(io.grpc.MethodDescriptor.MethodType.UNARY)
.setFullMethodName(generateFullMethodName(SERVICE_NAME, "GetMetrics"))
.setSampledToLocalTracing(true)
.setRequestMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
org.tikv.kvproto.Debugpb.GetMetricsRequest.getDefaultInstance()))
.setResponseMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
org.tikv.kvproto.Debugpb.GetMetricsResponse.getDefaultInstance()))
.setSchemaDescriptor(new DebugMethodDescriptorSupplier("GetMetrics"))
.build();
}
}
}
return getGetMetricsMethod;
}
private static volatile io.grpc.MethodDescriptor getCheckRegionConsistencyMethod;
@io.grpc.stub.annotations.RpcMethod(
fullMethodName = SERVICE_NAME + '/' + "CheckRegionConsistency",
requestType = org.tikv.kvproto.Debugpb.RegionConsistencyCheckRequest.class,
responseType = org.tikv.kvproto.Debugpb.RegionConsistencyCheckResponse.class,
methodType = io.grpc.MethodDescriptor.MethodType.UNARY)
public static io.grpc.MethodDescriptor getCheckRegionConsistencyMethod() {
io.grpc.MethodDescriptor getCheckRegionConsistencyMethod;
if ((getCheckRegionConsistencyMethod = DebugGrpc.getCheckRegionConsistencyMethod) == null) {
synchronized (DebugGrpc.class) {
if ((getCheckRegionConsistencyMethod = DebugGrpc.getCheckRegionConsistencyMethod) == null) {
DebugGrpc.getCheckRegionConsistencyMethod = getCheckRegionConsistencyMethod =
io.grpc.MethodDescriptor.newBuilder()
.setType(io.grpc.MethodDescriptor.MethodType.UNARY)
.setFullMethodName(generateFullMethodName(SERVICE_NAME, "CheckRegionConsistency"))
.setSampledToLocalTracing(true)
.setRequestMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
org.tikv.kvproto.Debugpb.RegionConsistencyCheckRequest.getDefaultInstance()))
.setResponseMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
org.tikv.kvproto.Debugpb.RegionConsistencyCheckResponse.getDefaultInstance()))
.setSchemaDescriptor(new DebugMethodDescriptorSupplier("CheckRegionConsistency"))
.build();
}
}
}
return getCheckRegionConsistencyMethod;
}
private static volatile io.grpc.MethodDescriptor getModifyTikvConfigMethod;
@io.grpc.stub.annotations.RpcMethod(
fullMethodName = SERVICE_NAME + '/' + "ModifyTikvConfig",
requestType = org.tikv.kvproto.Debugpb.ModifyTikvConfigRequest.class,
responseType = org.tikv.kvproto.Debugpb.ModifyTikvConfigResponse.class,
methodType = io.grpc.MethodDescriptor.MethodType.UNARY)
public static io.grpc.MethodDescriptor getModifyTikvConfigMethod() {
io.grpc.MethodDescriptor getModifyTikvConfigMethod;
if ((getModifyTikvConfigMethod = DebugGrpc.getModifyTikvConfigMethod) == null) {
synchronized (DebugGrpc.class) {
if ((getModifyTikvConfigMethod = DebugGrpc.getModifyTikvConfigMethod) == null) {
DebugGrpc.getModifyTikvConfigMethod = getModifyTikvConfigMethod =
io.grpc.MethodDescriptor.newBuilder()
.setType(io.grpc.MethodDescriptor.MethodType.UNARY)
.setFullMethodName(generateFullMethodName(SERVICE_NAME, "ModifyTikvConfig"))
.setSampledToLocalTracing(true)
.setRequestMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
org.tikv.kvproto.Debugpb.ModifyTikvConfigRequest.getDefaultInstance()))
.setResponseMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
org.tikv.kvproto.Debugpb.ModifyTikvConfigResponse.getDefaultInstance()))
.setSchemaDescriptor(new DebugMethodDescriptorSupplier("ModifyTikvConfig"))
.build();
}
}
}
return getModifyTikvConfigMethod;
}
private static volatile io.grpc.MethodDescriptor getGetRegionPropertiesMethod;
@io.grpc.stub.annotations.RpcMethod(
fullMethodName = SERVICE_NAME + '/' + "GetRegionProperties",
requestType = org.tikv.kvproto.Debugpb.GetRegionPropertiesRequest.class,
responseType = org.tikv.kvproto.Debugpb.GetRegionPropertiesResponse.class,
methodType = io.grpc.MethodDescriptor.MethodType.UNARY)
public static io.grpc.MethodDescriptor getGetRegionPropertiesMethod() {
io.grpc.MethodDescriptor getGetRegionPropertiesMethod;
if ((getGetRegionPropertiesMethod = DebugGrpc.getGetRegionPropertiesMethod) == null) {
synchronized (DebugGrpc.class) {
if ((getGetRegionPropertiesMethod = DebugGrpc.getGetRegionPropertiesMethod) == null) {
DebugGrpc.getGetRegionPropertiesMethod = getGetRegionPropertiesMethod =
io.grpc.MethodDescriptor.newBuilder()
.setType(io.grpc.MethodDescriptor.MethodType.UNARY)
.setFullMethodName(generateFullMethodName(SERVICE_NAME, "GetRegionProperties"))
.setSampledToLocalTracing(true)
.setRequestMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
org.tikv.kvproto.Debugpb.GetRegionPropertiesRequest.getDefaultInstance()))
.setResponseMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
org.tikv.kvproto.Debugpb.GetRegionPropertiesResponse.getDefaultInstance()))
.setSchemaDescriptor(new DebugMethodDescriptorSupplier("GetRegionProperties"))
.build();
}
}
}
return getGetRegionPropertiesMethod;
}
private static volatile io.grpc.MethodDescriptor getGetStoreInfoMethod;
@io.grpc.stub.annotations.RpcMethod(
fullMethodName = SERVICE_NAME + '/' + "GetStoreInfo",
requestType = org.tikv.kvproto.Debugpb.GetStoreInfoRequest.class,
responseType = org.tikv.kvproto.Debugpb.GetStoreInfoResponse.class,
methodType = io.grpc.MethodDescriptor.MethodType.UNARY)
public static io.grpc.MethodDescriptor getGetStoreInfoMethod() {
io.grpc.MethodDescriptor getGetStoreInfoMethod;
if ((getGetStoreInfoMethod = DebugGrpc.getGetStoreInfoMethod) == null) {
synchronized (DebugGrpc.class) {
if ((getGetStoreInfoMethod = DebugGrpc.getGetStoreInfoMethod) == null) {
DebugGrpc.getGetStoreInfoMethod = getGetStoreInfoMethod =
io.grpc.MethodDescriptor.newBuilder()
.setType(io.grpc.MethodDescriptor.MethodType.UNARY)
.setFullMethodName(generateFullMethodName(SERVICE_NAME, "GetStoreInfo"))
.setSampledToLocalTracing(true)
.setRequestMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
org.tikv.kvproto.Debugpb.GetStoreInfoRequest.getDefaultInstance()))
.setResponseMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
org.tikv.kvproto.Debugpb.GetStoreInfoResponse.getDefaultInstance()))
.setSchemaDescriptor(new DebugMethodDescriptorSupplier("GetStoreInfo"))
.build();
}
}
}
return getGetStoreInfoMethod;
}
private static volatile io.grpc.MethodDescriptor getGetClusterInfoMethod;
@io.grpc.stub.annotations.RpcMethod(
fullMethodName = SERVICE_NAME + '/' + "GetClusterInfo",
requestType = org.tikv.kvproto.Debugpb.GetClusterInfoRequest.class,
responseType = org.tikv.kvproto.Debugpb.GetClusterInfoResponse.class,
methodType = io.grpc.MethodDescriptor.MethodType.UNARY)
public static io.grpc.MethodDescriptor getGetClusterInfoMethod() {
io.grpc.MethodDescriptor getGetClusterInfoMethod;
if ((getGetClusterInfoMethod = DebugGrpc.getGetClusterInfoMethod) == null) {
synchronized (DebugGrpc.class) {
if ((getGetClusterInfoMethod = DebugGrpc.getGetClusterInfoMethod) == null) {
DebugGrpc.getGetClusterInfoMethod = getGetClusterInfoMethod =
io.grpc.MethodDescriptor.newBuilder()
.setType(io.grpc.MethodDescriptor.MethodType.UNARY)
.setFullMethodName(generateFullMethodName(SERVICE_NAME, "GetClusterInfo"))
.setSampledToLocalTracing(true)
.setRequestMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
org.tikv.kvproto.Debugpb.GetClusterInfoRequest.getDefaultInstance()))
.setResponseMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
org.tikv.kvproto.Debugpb.GetClusterInfoResponse.getDefaultInstance()))
.setSchemaDescriptor(new DebugMethodDescriptorSupplier("GetClusterInfo"))
.build();
}
}
}
return getGetClusterInfoMethod;
}
/**
* Creates a new async stub that supports all call types for the service
*/
public static DebugStub newStub(io.grpc.Channel channel) {
io.grpc.stub.AbstractStub.StubFactory factory =
new io.grpc.stub.AbstractStub.StubFactory() {
@java.lang.Override
public DebugStub newStub(io.grpc.Channel channel, io.grpc.CallOptions callOptions) {
return new DebugStub(channel, callOptions);
}
};
return DebugStub.newStub(factory, channel);
}
/**
* Creates a new blocking-style stub that supports unary and streaming output calls on the service
*/
public static DebugBlockingStub newBlockingStub(
io.grpc.Channel channel) {
io.grpc.stub.AbstractStub.StubFactory factory =
new io.grpc.stub.AbstractStub.StubFactory() {
@java.lang.Override
public DebugBlockingStub newStub(io.grpc.Channel channel, io.grpc.CallOptions callOptions) {
return new DebugBlockingStub(channel, callOptions);
}
};
return DebugBlockingStub.newStub(factory, channel);
}
/**
* Creates a new ListenableFuture-style stub that supports unary calls on the service
*/
public static DebugFutureStub newFutureStub(
io.grpc.Channel channel) {
io.grpc.stub.AbstractStub.StubFactory factory =
new io.grpc.stub.AbstractStub.StubFactory() {
@java.lang.Override
public DebugFutureStub newStub(io.grpc.Channel channel, io.grpc.CallOptions callOptions) {
return new DebugFutureStub(channel, callOptions);
}
};
return DebugFutureStub.newStub(factory, channel);
}
/**
*
* Debug service for TiKV.
* Errors are defined as follow:
* - OK: Okay, we are good!
* - UNKNOWN: For unknown error.
* - INVALID_ARGUMENT: Something goes wrong within requests.
* - NOT_FOUND: It is key or region not found, it's based on context, detailed
* reason can be found in grpc message.
* Note: It bypasses raft layer.
*
*/
public static abstract class DebugImplBase implements io.grpc.BindableService {
/**
*
* Read a value arbitrarily for a key.
* Note: Server uses key directly w/o any encoding.
*
*/
public void get(org.tikv.kvproto.Debugpb.GetRequest request,
io.grpc.stub.StreamObserver responseObserver) {
asyncUnimplementedUnaryCall(getGetMethod(), responseObserver);
}
/**
*
* Read raft info.
*
*/
public void raftLog(org.tikv.kvproto.Debugpb.RaftLogRequest request,
io.grpc.stub.StreamObserver responseObserver) {
asyncUnimplementedUnaryCall(getRaftLogMethod(), responseObserver);
}
/**
*/
public void regionInfo(org.tikv.kvproto.Debugpb.RegionInfoRequest request,
io.grpc.stub.StreamObserver responseObserver) {
asyncUnimplementedUnaryCall(getRegionInfoMethod(), responseObserver);
}
/**
*
* Calculate size of a region.
* Note: DO NOT CALL IT IN PRODUCTION, it's really expensive.
*
*/
public void regionSize(org.tikv.kvproto.Debugpb.RegionSizeRequest request,
io.grpc.stub.StreamObserver responseObserver) {
asyncUnimplementedUnaryCall(getRegionSizeMethod(), responseObserver);
}
/**
*
* Scan a specific range.
* Note: DO NOT CALL IT IN PRODUCTION, it's really expensive.
* Server uses keys directly w/o any encoding.
*
*/
public void scanMvcc(org.tikv.kvproto.Debugpb.ScanMvccRequest request,
io.grpc.stub.StreamObserver responseObserver) {
asyncUnimplementedUnaryCall(getScanMvccMethod(), responseObserver);
}
/**
*
* Compact a column family in a specified range.
* Note: Server uses keys directly w/o any encoding.
*
*/
public void compact(org.tikv.kvproto.Debugpb.CompactRequest request,
io.grpc.stub.StreamObserver responseObserver) {
asyncUnimplementedUnaryCall(getCompactMethod(), responseObserver);
}
/**
*
* Inject a fail point. Currently, it's only used in tests.
* Note: DO NOT CALL IT IN PRODUCTION.
*
*/
public void injectFailPoint(org.tikv.kvproto.Debugpb.InjectFailPointRequest request,
io.grpc.stub.StreamObserver responseObserver) {
asyncUnimplementedUnaryCall(getInjectFailPointMethod(), responseObserver);
}
/**
*
* Recover from a fail point.
*
*/
public void recoverFailPoint(org.tikv.kvproto.Debugpb.RecoverFailPointRequest request,
io.grpc.stub.StreamObserver responseObserver) {
asyncUnimplementedUnaryCall(getRecoverFailPointMethod(), responseObserver);
}
/**
*
* List all fail points.
*
*/
public void listFailPoints(org.tikv.kvproto.Debugpb.ListFailPointsRequest request,
io.grpc.stub.StreamObserver responseObserver) {
asyncUnimplementedUnaryCall(getListFailPointsMethod(), responseObserver);
}
/**
*
* Get Metrics
*
*/
public void getMetrics(org.tikv.kvproto.Debugpb.GetMetricsRequest request,
io.grpc.stub.StreamObserver responseObserver) {
asyncUnimplementedUnaryCall(getGetMetricsMethod(), responseObserver);
}
/**
*
* Do a consistent check for a region.
*
*/
public void checkRegionConsistency(org.tikv.kvproto.Debugpb.RegionConsistencyCheckRequest request,
io.grpc.stub.StreamObserver responseObserver) {
asyncUnimplementedUnaryCall(getCheckRegionConsistencyMethod(), responseObserver);
}
/**
*
* dynamically modify tikv's config
*
*/
public void modifyTikvConfig(org.tikv.kvproto.Debugpb.ModifyTikvConfigRequest request,
io.grpc.stub.StreamObserver responseObserver) {
asyncUnimplementedUnaryCall(getModifyTikvConfigMethod(), responseObserver);
}
/**
*
* Get region properties
*
*/
public void getRegionProperties(org.tikv.kvproto.Debugpb.GetRegionPropertiesRequest request,
io.grpc.stub.StreamObserver responseObserver) {
asyncUnimplementedUnaryCall(getGetRegionPropertiesMethod(), responseObserver);
}
/**
*
* Get store ID
*
*/
public void getStoreInfo(org.tikv.kvproto.Debugpb.GetStoreInfoRequest request,
io.grpc.stub.StreamObserver responseObserver) {
asyncUnimplementedUnaryCall(getGetStoreInfoMethod(), responseObserver);
}
/**
*
* Get cluster ID
*
*/
public void getClusterInfo(org.tikv.kvproto.Debugpb.GetClusterInfoRequest request,
io.grpc.stub.StreamObserver responseObserver) {
asyncUnimplementedUnaryCall(getGetClusterInfoMethod(), responseObserver);
}
@java.lang.Override public final io.grpc.ServerServiceDefinition bindService() {
return io.grpc.ServerServiceDefinition.builder(getServiceDescriptor())
.addMethod(
getGetMethod(),
asyncUnaryCall(
new MethodHandlers<
org.tikv.kvproto.Debugpb.GetRequest,
org.tikv.kvproto.Debugpb.GetResponse>(
this, METHODID_GET)))
.addMethod(
getRaftLogMethod(),
asyncUnaryCall(
new MethodHandlers<
org.tikv.kvproto.Debugpb.RaftLogRequest,
org.tikv.kvproto.Debugpb.RaftLogResponse>(
this, METHODID_RAFT_LOG)))
.addMethod(
getRegionInfoMethod(),
asyncUnaryCall(
new MethodHandlers<
org.tikv.kvproto.Debugpb.RegionInfoRequest,
org.tikv.kvproto.Debugpb.RegionInfoResponse>(
this, METHODID_REGION_INFO)))
.addMethod(
getRegionSizeMethod(),
asyncUnaryCall(
new MethodHandlers<
org.tikv.kvproto.Debugpb.RegionSizeRequest,
org.tikv.kvproto.Debugpb.RegionSizeResponse>(
this, METHODID_REGION_SIZE)))
.addMethod(
getScanMvccMethod(),
asyncServerStreamingCall(
new MethodHandlers<
org.tikv.kvproto.Debugpb.ScanMvccRequest,
org.tikv.kvproto.Debugpb.ScanMvccResponse>(
this, METHODID_SCAN_MVCC)))
.addMethod(
getCompactMethod(),
asyncUnaryCall(
new MethodHandlers<
org.tikv.kvproto.Debugpb.CompactRequest,
org.tikv.kvproto.Debugpb.CompactResponse>(
this, METHODID_COMPACT)))
.addMethod(
getInjectFailPointMethod(),
asyncUnaryCall(
new MethodHandlers<
org.tikv.kvproto.Debugpb.InjectFailPointRequest,
org.tikv.kvproto.Debugpb.InjectFailPointResponse>(
this, METHODID_INJECT_FAIL_POINT)))
.addMethod(
getRecoverFailPointMethod(),
asyncUnaryCall(
new MethodHandlers<
org.tikv.kvproto.Debugpb.RecoverFailPointRequest,
org.tikv.kvproto.Debugpb.RecoverFailPointResponse>(
this, METHODID_RECOVER_FAIL_POINT)))
.addMethod(
getListFailPointsMethod(),
asyncUnaryCall(
new MethodHandlers<
org.tikv.kvproto.Debugpb.ListFailPointsRequest,
org.tikv.kvproto.Debugpb.ListFailPointsResponse>(
this, METHODID_LIST_FAIL_POINTS)))
.addMethod(
getGetMetricsMethod(),
asyncUnaryCall(
new MethodHandlers<
org.tikv.kvproto.Debugpb.GetMetricsRequest,
org.tikv.kvproto.Debugpb.GetMetricsResponse>(
this, METHODID_GET_METRICS)))
.addMethod(
getCheckRegionConsistencyMethod(),
asyncUnaryCall(
new MethodHandlers<
org.tikv.kvproto.Debugpb.RegionConsistencyCheckRequest,
org.tikv.kvproto.Debugpb.RegionConsistencyCheckResponse>(
this, METHODID_CHECK_REGION_CONSISTENCY)))
.addMethod(
getModifyTikvConfigMethod(),
asyncUnaryCall(
new MethodHandlers<
org.tikv.kvproto.Debugpb.ModifyTikvConfigRequest,
org.tikv.kvproto.Debugpb.ModifyTikvConfigResponse>(
this, METHODID_MODIFY_TIKV_CONFIG)))
.addMethod(
getGetRegionPropertiesMethod(),
asyncUnaryCall(
new MethodHandlers<
org.tikv.kvproto.Debugpb.GetRegionPropertiesRequest,
org.tikv.kvproto.Debugpb.GetRegionPropertiesResponse>(
this, METHODID_GET_REGION_PROPERTIES)))
.addMethod(
getGetStoreInfoMethod(),
asyncUnaryCall(
new MethodHandlers<
org.tikv.kvproto.Debugpb.GetStoreInfoRequest,
org.tikv.kvproto.Debugpb.GetStoreInfoResponse>(
this, METHODID_GET_STORE_INFO)))
.addMethod(
getGetClusterInfoMethod(),
asyncUnaryCall(
new MethodHandlers<
org.tikv.kvproto.Debugpb.GetClusterInfoRequest,
org.tikv.kvproto.Debugpb.GetClusterInfoResponse>(
this, METHODID_GET_CLUSTER_INFO)))
.build();
}
}
/**
*
* Debug service for TiKV.
* Errors are defined as follow:
* - OK: Okay, we are good!
* - UNKNOWN: For unknown error.
* - INVALID_ARGUMENT: Something goes wrong within requests.
* - NOT_FOUND: It is key or region not found, it's based on context, detailed
* reason can be found in grpc message.
* Note: It bypasses raft layer.
*
*/
public static final class DebugStub extends io.grpc.stub.AbstractAsyncStub {
private DebugStub(
io.grpc.Channel channel, io.grpc.CallOptions callOptions) {
super(channel, callOptions);
}
@java.lang.Override
protected DebugStub build(
io.grpc.Channel channel, io.grpc.CallOptions callOptions) {
return new DebugStub(channel, callOptions);
}
/**
*
* Read a value arbitrarily for a key.
* Note: Server uses key directly w/o any encoding.
*
*/
public void get(org.tikv.kvproto.Debugpb.GetRequest request,
io.grpc.stub.StreamObserver responseObserver) {
asyncUnaryCall(
getChannel().newCall(getGetMethod(), getCallOptions()), request, responseObserver);
}
/**
*
* Read raft info.
*
*/
public void raftLog(org.tikv.kvproto.Debugpb.RaftLogRequest request,
io.grpc.stub.StreamObserver responseObserver) {
asyncUnaryCall(
getChannel().newCall(getRaftLogMethod(), getCallOptions()), request, responseObserver);
}
/**
*/
public void regionInfo(org.tikv.kvproto.Debugpb.RegionInfoRequest request,
io.grpc.stub.StreamObserver responseObserver) {
asyncUnaryCall(
getChannel().newCall(getRegionInfoMethod(), getCallOptions()), request, responseObserver);
}
/**
*
* Calculate size of a region.
* Note: DO NOT CALL IT IN PRODUCTION, it's really expensive.
*
*/
public void regionSize(org.tikv.kvproto.Debugpb.RegionSizeRequest request,
io.grpc.stub.StreamObserver responseObserver) {
asyncUnaryCall(
getChannel().newCall(getRegionSizeMethod(), getCallOptions()), request, responseObserver);
}
/**
*
* Scan a specific range.
* Note: DO NOT CALL IT IN PRODUCTION, it's really expensive.
* Server uses keys directly w/o any encoding.
*
*/
public void scanMvcc(org.tikv.kvproto.Debugpb.ScanMvccRequest request,
io.grpc.stub.StreamObserver responseObserver) {
asyncServerStreamingCall(
getChannel().newCall(getScanMvccMethod(), getCallOptions()), request, responseObserver);
}
/**
*
* Compact a column family in a specified range.
* Note: Server uses keys directly w/o any encoding.
*
*/
public void compact(org.tikv.kvproto.Debugpb.CompactRequest request,
io.grpc.stub.StreamObserver responseObserver) {
asyncUnaryCall(
getChannel().newCall(getCompactMethod(), getCallOptions()), request, responseObserver);
}
/**
*
* Inject a fail point. Currently, it's only used in tests.
* Note: DO NOT CALL IT IN PRODUCTION.
*
*/
public void injectFailPoint(org.tikv.kvproto.Debugpb.InjectFailPointRequest request,
io.grpc.stub.StreamObserver responseObserver) {
asyncUnaryCall(
getChannel().newCall(getInjectFailPointMethod(), getCallOptions()), request, responseObserver);
}
/**
*
* Recover from a fail point.
*
*/
public void recoverFailPoint(org.tikv.kvproto.Debugpb.RecoverFailPointRequest request,
io.grpc.stub.StreamObserver responseObserver) {
asyncUnaryCall(
getChannel().newCall(getRecoverFailPointMethod(), getCallOptions()), request, responseObserver);
}
/**
*
* List all fail points.
*
*/
public void listFailPoints(org.tikv.kvproto.Debugpb.ListFailPointsRequest request,
io.grpc.stub.StreamObserver responseObserver) {
asyncUnaryCall(
getChannel().newCall(getListFailPointsMethod(), getCallOptions()), request, responseObserver);
}
/**
*
* Get Metrics
*
*/
public void getMetrics(org.tikv.kvproto.Debugpb.GetMetricsRequest request,
io.grpc.stub.StreamObserver responseObserver) {
asyncUnaryCall(
getChannel().newCall(getGetMetricsMethod(), getCallOptions()), request, responseObserver);
}
/**
*
* Do a consistent check for a region.
*
*/
public void checkRegionConsistency(org.tikv.kvproto.Debugpb.RegionConsistencyCheckRequest request,
io.grpc.stub.StreamObserver responseObserver) {
asyncUnaryCall(
getChannel().newCall(getCheckRegionConsistencyMethod(), getCallOptions()), request, responseObserver);
}
/**
*
* dynamically modify tikv's config
*
*/
public void modifyTikvConfig(org.tikv.kvproto.Debugpb.ModifyTikvConfigRequest request,
io.grpc.stub.StreamObserver responseObserver) {
asyncUnaryCall(
getChannel().newCall(getModifyTikvConfigMethod(), getCallOptions()), request, responseObserver);
}
/**
*
* Get region properties
*
*/
public void getRegionProperties(org.tikv.kvproto.Debugpb.GetRegionPropertiesRequest request,
io.grpc.stub.StreamObserver responseObserver) {
asyncUnaryCall(
getChannel().newCall(getGetRegionPropertiesMethod(), getCallOptions()), request, responseObserver);
}
/**
*
* Get store ID
*
*/
public void getStoreInfo(org.tikv.kvproto.Debugpb.GetStoreInfoRequest request,
io.grpc.stub.StreamObserver responseObserver) {
asyncUnaryCall(
getChannel().newCall(getGetStoreInfoMethod(), getCallOptions()), request, responseObserver);
}
/**
*
* Get cluster ID
*
*/
public void getClusterInfo(org.tikv.kvproto.Debugpb.GetClusterInfoRequest request,
io.grpc.stub.StreamObserver responseObserver) {
asyncUnaryCall(
getChannel().newCall(getGetClusterInfoMethod(), getCallOptions()), request, responseObserver);
}
}
/**
*
* Debug service for TiKV.
* Errors are defined as follow:
* - OK: Okay, we are good!
* - UNKNOWN: For unknown error.
* - INVALID_ARGUMENT: Something goes wrong within requests.
* - NOT_FOUND: It is key or region not found, it's based on context, detailed
* reason can be found in grpc message.
* Note: It bypasses raft layer.
*
*/
public static final class DebugBlockingStub extends io.grpc.stub.AbstractBlockingStub {
private DebugBlockingStub(
io.grpc.Channel channel, io.grpc.CallOptions callOptions) {
super(channel, callOptions);
}
@java.lang.Override
protected DebugBlockingStub build(
io.grpc.Channel channel, io.grpc.CallOptions callOptions) {
return new DebugBlockingStub(channel, callOptions);
}
/**
*
* Read a value arbitrarily for a key.
* Note: Server uses key directly w/o any encoding.
*
*/
public org.tikv.kvproto.Debugpb.GetResponse get(org.tikv.kvproto.Debugpb.GetRequest request) {
return blockingUnaryCall(
getChannel(), getGetMethod(), getCallOptions(), request);
}
/**
*
* Read raft info.
*
*/
public org.tikv.kvproto.Debugpb.RaftLogResponse raftLog(org.tikv.kvproto.Debugpb.RaftLogRequest request) {
return blockingUnaryCall(
getChannel(), getRaftLogMethod(), getCallOptions(), request);
}
/**
*/
public org.tikv.kvproto.Debugpb.RegionInfoResponse regionInfo(org.tikv.kvproto.Debugpb.RegionInfoRequest request) {
return blockingUnaryCall(
getChannel(), getRegionInfoMethod(), getCallOptions(), request);
}
/**
*
* Calculate size of a region.
* Note: DO NOT CALL IT IN PRODUCTION, it's really expensive.
*
*/
public org.tikv.kvproto.Debugpb.RegionSizeResponse regionSize(org.tikv.kvproto.Debugpb.RegionSizeRequest request) {
return blockingUnaryCall(
getChannel(), getRegionSizeMethod(), getCallOptions(), request);
}
/**
*
* Scan a specific range.
* Note: DO NOT CALL IT IN PRODUCTION, it's really expensive.
* Server uses keys directly w/o any encoding.
*
*/
public java.util.Iterator scanMvcc(
org.tikv.kvproto.Debugpb.ScanMvccRequest request) {
return blockingServerStreamingCall(
getChannel(), getScanMvccMethod(), getCallOptions(), request);
}
/**
*
* Compact a column family in a specified range.
* Note: Server uses keys directly w/o any encoding.
*
*/
public org.tikv.kvproto.Debugpb.CompactResponse compact(org.tikv.kvproto.Debugpb.CompactRequest request) {
return blockingUnaryCall(
getChannel(), getCompactMethod(), getCallOptions(), request);
}
/**
*
* Inject a fail point. Currently, it's only used in tests.
* Note: DO NOT CALL IT IN PRODUCTION.
*
*/
public org.tikv.kvproto.Debugpb.InjectFailPointResponse injectFailPoint(org.tikv.kvproto.Debugpb.InjectFailPointRequest request) {
return blockingUnaryCall(
getChannel(), getInjectFailPointMethod(), getCallOptions(), request);
}
/**
*
* Recover from a fail point.
*
*/
public org.tikv.kvproto.Debugpb.RecoverFailPointResponse recoverFailPoint(org.tikv.kvproto.Debugpb.RecoverFailPointRequest request) {
return blockingUnaryCall(
getChannel(), getRecoverFailPointMethod(), getCallOptions(), request);
}
/**
*
* List all fail points.
*
*/
public org.tikv.kvproto.Debugpb.ListFailPointsResponse listFailPoints(org.tikv.kvproto.Debugpb.ListFailPointsRequest request) {
return blockingUnaryCall(
getChannel(), getListFailPointsMethod(), getCallOptions(), request);
}
/**
*
* Get Metrics
*
*/
public org.tikv.kvproto.Debugpb.GetMetricsResponse getMetrics(org.tikv.kvproto.Debugpb.GetMetricsRequest request) {
return blockingUnaryCall(
getChannel(), getGetMetricsMethod(), getCallOptions(), request);
}
/**
*
* Do a consistent check for a region.
*
*/
public org.tikv.kvproto.Debugpb.RegionConsistencyCheckResponse checkRegionConsistency(org.tikv.kvproto.Debugpb.RegionConsistencyCheckRequest request) {
return blockingUnaryCall(
getChannel(), getCheckRegionConsistencyMethod(), getCallOptions(), request);
}
/**
*
* dynamically modify tikv's config
*
*/
public org.tikv.kvproto.Debugpb.ModifyTikvConfigResponse modifyTikvConfig(org.tikv.kvproto.Debugpb.ModifyTikvConfigRequest request) {
return blockingUnaryCall(
getChannel(), getModifyTikvConfigMethod(), getCallOptions(), request);
}
/**
*
* Get region properties
*
*/
public org.tikv.kvproto.Debugpb.GetRegionPropertiesResponse getRegionProperties(org.tikv.kvproto.Debugpb.GetRegionPropertiesRequest request) {
return blockingUnaryCall(
getChannel(), getGetRegionPropertiesMethod(), getCallOptions(), request);
}
/**
*
* Get store ID
*
*/
public org.tikv.kvproto.Debugpb.GetStoreInfoResponse getStoreInfo(org.tikv.kvproto.Debugpb.GetStoreInfoRequest request) {
return blockingUnaryCall(
getChannel(), getGetStoreInfoMethod(), getCallOptions(), request);
}
/**
*
* Get cluster ID
*
*/
public org.tikv.kvproto.Debugpb.GetClusterInfoResponse getClusterInfo(org.tikv.kvproto.Debugpb.GetClusterInfoRequest request) {
return blockingUnaryCall(
getChannel(), getGetClusterInfoMethod(), getCallOptions(), request);
}
}
/**
*
* Debug service for TiKV.
* Errors are defined as follow:
* - OK: Okay, we are good!
* - UNKNOWN: For unknown error.
* - INVALID_ARGUMENT: Something goes wrong within requests.
* - NOT_FOUND: It is key or region not found, it's based on context, detailed
* reason can be found in grpc message.
* Note: It bypasses raft layer.
*
*/
public static final class DebugFutureStub extends io.grpc.stub.AbstractFutureStub {
private DebugFutureStub(
io.grpc.Channel channel, io.grpc.CallOptions callOptions) {
super(channel, callOptions);
}
@java.lang.Override
protected DebugFutureStub build(
io.grpc.Channel channel, io.grpc.CallOptions callOptions) {
return new DebugFutureStub(channel, callOptions);
}
/**
*
* Read a value arbitrarily for a key.
* Note: Server uses key directly w/o any encoding.
*
*/
public com.google.common.util.concurrent.ListenableFuture get(
org.tikv.kvproto.Debugpb.GetRequest request) {
return futureUnaryCall(
getChannel().newCall(getGetMethod(), getCallOptions()), request);
}
/**
*
* Read raft info.
*
*/
public com.google.common.util.concurrent.ListenableFuture raftLog(
org.tikv.kvproto.Debugpb.RaftLogRequest request) {
return futureUnaryCall(
getChannel().newCall(getRaftLogMethod(), getCallOptions()), request);
}
/**
*/
public com.google.common.util.concurrent.ListenableFuture regionInfo(
org.tikv.kvproto.Debugpb.RegionInfoRequest request) {
return futureUnaryCall(
getChannel().newCall(getRegionInfoMethod(), getCallOptions()), request);
}
/**
*
* Calculate size of a region.
* Note: DO NOT CALL IT IN PRODUCTION, it's really expensive.
*
*/
public com.google.common.util.concurrent.ListenableFuture regionSize(
org.tikv.kvproto.Debugpb.RegionSizeRequest request) {
return futureUnaryCall(
getChannel().newCall(getRegionSizeMethod(), getCallOptions()), request);
}
/**
*
* Compact a column family in a specified range.
* Note: Server uses keys directly w/o any encoding.
*
*/
public com.google.common.util.concurrent.ListenableFuture compact(
org.tikv.kvproto.Debugpb.CompactRequest request) {
return futureUnaryCall(
getChannel().newCall(getCompactMethod(), getCallOptions()), request);
}
/**
*
* Inject a fail point. Currently, it's only used in tests.
* Note: DO NOT CALL IT IN PRODUCTION.
*
*/
public com.google.common.util.concurrent.ListenableFuture injectFailPoint(
org.tikv.kvproto.Debugpb.InjectFailPointRequest request) {
return futureUnaryCall(
getChannel().newCall(getInjectFailPointMethod(), getCallOptions()), request);
}
/**
*
* Recover from a fail point.
*
*/
public com.google.common.util.concurrent.ListenableFuture recoverFailPoint(
org.tikv.kvproto.Debugpb.RecoverFailPointRequest request) {
return futureUnaryCall(
getChannel().newCall(getRecoverFailPointMethod(), getCallOptions()), request);
}
/**
*
* List all fail points.
*
*/
public com.google.common.util.concurrent.ListenableFuture listFailPoints(
org.tikv.kvproto.Debugpb.ListFailPointsRequest request) {
return futureUnaryCall(
getChannel().newCall(getListFailPointsMethod(), getCallOptions()), request);
}
/**
*
* Get Metrics
*
*/
public com.google.common.util.concurrent.ListenableFuture getMetrics(
org.tikv.kvproto.Debugpb.GetMetricsRequest request) {
return futureUnaryCall(
getChannel().newCall(getGetMetricsMethod(), getCallOptions()), request);
}
/**
*
* Do a consistent check for a region.
*
*/
public com.google.common.util.concurrent.ListenableFuture checkRegionConsistency(
org.tikv.kvproto.Debugpb.RegionConsistencyCheckRequest request) {
return futureUnaryCall(
getChannel().newCall(getCheckRegionConsistencyMethod(), getCallOptions()), request);
}
/**
*
* dynamically modify tikv's config
*
*/
public com.google.common.util.concurrent.ListenableFuture modifyTikvConfig(
org.tikv.kvproto.Debugpb.ModifyTikvConfigRequest request) {
return futureUnaryCall(
getChannel().newCall(getModifyTikvConfigMethod(), getCallOptions()), request);
}
/**
*
* Get region properties
*
*/
public com.google.common.util.concurrent.ListenableFuture getRegionProperties(
org.tikv.kvproto.Debugpb.GetRegionPropertiesRequest request) {
return futureUnaryCall(
getChannel().newCall(getGetRegionPropertiesMethod(), getCallOptions()), request);
}
/**
*
* Get store ID
*
*/
public com.google.common.util.concurrent.ListenableFuture getStoreInfo(
org.tikv.kvproto.Debugpb.GetStoreInfoRequest request) {
return futureUnaryCall(
getChannel().newCall(getGetStoreInfoMethod(), getCallOptions()), request);
}
/**
*
* Get cluster ID
*
*/
public com.google.common.util.concurrent.ListenableFuture getClusterInfo(
org.tikv.kvproto.Debugpb.GetClusterInfoRequest request) {
return futureUnaryCall(
getChannel().newCall(getGetClusterInfoMethod(), getCallOptions()), request);
}
}
private static final int METHODID_GET = 0;
private static final int METHODID_RAFT_LOG = 1;
private static final int METHODID_REGION_INFO = 2;
private static final int METHODID_REGION_SIZE = 3;
private static final int METHODID_SCAN_MVCC = 4;
private static final int METHODID_COMPACT = 5;
private static final int METHODID_INJECT_FAIL_POINT = 6;
private static final int METHODID_RECOVER_FAIL_POINT = 7;
private static final int METHODID_LIST_FAIL_POINTS = 8;
private static final int METHODID_GET_METRICS = 9;
private static final int METHODID_CHECK_REGION_CONSISTENCY = 10;
private static final int METHODID_MODIFY_TIKV_CONFIG = 11;
private static final int METHODID_GET_REGION_PROPERTIES = 12;
private static final int METHODID_GET_STORE_INFO = 13;
private static final int METHODID_GET_CLUSTER_INFO = 14;
private static final class MethodHandlers implements
io.grpc.stub.ServerCalls.UnaryMethod,
io.grpc.stub.ServerCalls.ServerStreamingMethod,
io.grpc.stub.ServerCalls.ClientStreamingMethod,
io.grpc.stub.ServerCalls.BidiStreamingMethod {
private final DebugImplBase serviceImpl;
private final int methodId;
MethodHandlers(DebugImplBase serviceImpl, int methodId) {
this.serviceImpl = serviceImpl;
this.methodId = methodId;
}
@java.lang.Override
@java.lang.SuppressWarnings("unchecked")
public void invoke(Req request, io.grpc.stub.StreamObserver responseObserver) {
switch (methodId) {
case METHODID_GET:
serviceImpl.get((org.tikv.kvproto.Debugpb.GetRequest) request,
(io.grpc.stub.StreamObserver) responseObserver);
break;
case METHODID_RAFT_LOG:
serviceImpl.raftLog((org.tikv.kvproto.Debugpb.RaftLogRequest) request,
(io.grpc.stub.StreamObserver) responseObserver);
break;
case METHODID_REGION_INFO:
serviceImpl.regionInfo((org.tikv.kvproto.Debugpb.RegionInfoRequest) request,
(io.grpc.stub.StreamObserver) responseObserver);
break;
case METHODID_REGION_SIZE:
serviceImpl.regionSize((org.tikv.kvproto.Debugpb.RegionSizeRequest) request,
(io.grpc.stub.StreamObserver) responseObserver);
break;
case METHODID_SCAN_MVCC:
serviceImpl.scanMvcc((org.tikv.kvproto.Debugpb.ScanMvccRequest) request,
(io.grpc.stub.StreamObserver) responseObserver);
break;
case METHODID_COMPACT:
serviceImpl.compact((org.tikv.kvproto.Debugpb.CompactRequest) request,
(io.grpc.stub.StreamObserver) responseObserver);
break;
case METHODID_INJECT_FAIL_POINT:
serviceImpl.injectFailPoint((org.tikv.kvproto.Debugpb.InjectFailPointRequest) request,
(io.grpc.stub.StreamObserver) responseObserver);
break;
case METHODID_RECOVER_FAIL_POINT:
serviceImpl.recoverFailPoint((org.tikv.kvproto.Debugpb.RecoverFailPointRequest) request,
(io.grpc.stub.StreamObserver) responseObserver);
break;
case METHODID_LIST_FAIL_POINTS:
serviceImpl.listFailPoints((org.tikv.kvproto.Debugpb.ListFailPointsRequest) request,
(io.grpc.stub.StreamObserver) responseObserver);
break;
case METHODID_GET_METRICS:
serviceImpl.getMetrics((org.tikv.kvproto.Debugpb.GetMetricsRequest) request,
(io.grpc.stub.StreamObserver) responseObserver);
break;
case METHODID_CHECK_REGION_CONSISTENCY:
serviceImpl.checkRegionConsistency((org.tikv.kvproto.Debugpb.RegionConsistencyCheckRequest) request,
(io.grpc.stub.StreamObserver) responseObserver);
break;
case METHODID_MODIFY_TIKV_CONFIG:
serviceImpl.modifyTikvConfig((org.tikv.kvproto.Debugpb.ModifyTikvConfigRequest) request,
(io.grpc.stub.StreamObserver) responseObserver);
break;
case METHODID_GET_REGION_PROPERTIES:
serviceImpl.getRegionProperties((org.tikv.kvproto.Debugpb.GetRegionPropertiesRequest) request,
(io.grpc.stub.StreamObserver) responseObserver);
break;
case METHODID_GET_STORE_INFO:
serviceImpl.getStoreInfo((org.tikv.kvproto.Debugpb.GetStoreInfoRequest) request,
(io.grpc.stub.StreamObserver) responseObserver);
break;
case METHODID_GET_CLUSTER_INFO:
serviceImpl.getClusterInfo((org.tikv.kvproto.Debugpb.GetClusterInfoRequest) request,
(io.grpc.stub.StreamObserver) responseObserver);
break;
default:
throw new AssertionError();
}
}
@java.lang.Override
@java.lang.SuppressWarnings("unchecked")
public io.grpc.stub.StreamObserver invoke(
io.grpc.stub.StreamObserver responseObserver) {
switch (methodId) {
default:
throw new AssertionError();
}
}
}
private static abstract class DebugBaseDescriptorSupplier
implements io.grpc.protobuf.ProtoFileDescriptorSupplier, io.grpc.protobuf.ProtoServiceDescriptorSupplier {
DebugBaseDescriptorSupplier() {}
@java.lang.Override
public com.google.protobuf.Descriptors.FileDescriptor getFileDescriptor() {
return org.tikv.kvproto.Debugpb.getDescriptor();
}
@java.lang.Override
public com.google.protobuf.Descriptors.ServiceDescriptor getServiceDescriptor() {
return getFileDescriptor().findServiceByName("Debug");
}
}
private static final class DebugFileDescriptorSupplier
extends DebugBaseDescriptorSupplier {
DebugFileDescriptorSupplier() {}
}
private static final class DebugMethodDescriptorSupplier
extends DebugBaseDescriptorSupplier
implements io.grpc.protobuf.ProtoMethodDescriptorSupplier {
private final String methodName;
DebugMethodDescriptorSupplier(String methodName) {
this.methodName = methodName;
}
@java.lang.Override
public com.google.protobuf.Descriptors.MethodDescriptor getMethodDescriptor() {
return getServiceDescriptor().findMethodByName(methodName);
}
}
private static volatile io.grpc.ServiceDescriptor serviceDescriptor;
public static io.grpc.ServiceDescriptor getServiceDescriptor() {
io.grpc.ServiceDescriptor result = serviceDescriptor;
if (result == null) {
synchronized (DebugGrpc.class) {
result = serviceDescriptor;
if (result == null) {
serviceDescriptor = result = io.grpc.ServiceDescriptor.newBuilder(SERVICE_NAME)
.setSchemaDescriptor(new DebugFileDescriptorSupplier())
.addMethod(getGetMethod())
.addMethod(getRaftLogMethod())
.addMethod(getRegionInfoMethod())
.addMethod(getRegionSizeMethod())
.addMethod(getScanMvccMethod())
.addMethod(getCompactMethod())
.addMethod(getInjectFailPointMethod())
.addMethod(getRecoverFailPointMethod())
.addMethod(getListFailPointsMethod())
.addMethod(getGetMetricsMethod())
.addMethod(getCheckRegionConsistencyMethod())
.addMethod(getModifyTikvConfigMethod())
.addMethod(getGetRegionPropertiesMethod())
.addMethod(getGetStoreInfoMethod())
.addMethod(getGetClusterInfoMethod())
.build();
}
}
}
return result;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy