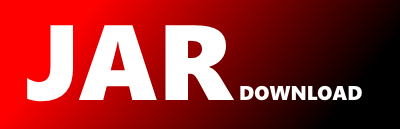
org.tikv.kvproto.Debugpb Maven / Gradle / Ivy
// Generated by the protocol buffer compiler. DO NOT EDIT!
// source: debugpb.proto
package org.tikv.kvproto;
public final class Debugpb {
private Debugpb() {}
public static void registerAllExtensions(
com.google.protobuf.ExtensionRegistryLite registry) {
}
public static void registerAllExtensions(
com.google.protobuf.ExtensionRegistry registry) {
registerAllExtensions(
(com.google.protobuf.ExtensionRegistryLite) registry);
}
/**
* Protobuf enum {@code debugpb.DB}
*/
public enum DB
implements com.google.protobuf.ProtocolMessageEnum {
/**
* INVALID = 0;
*/
INVALID(0),
/**
* KV = 1;
*/
KV(1),
/**
* RAFT = 2;
*/
RAFT(2),
UNRECOGNIZED(-1),
;
/**
* INVALID = 0;
*/
public static final int INVALID_VALUE = 0;
/**
* KV = 1;
*/
public static final int KV_VALUE = 1;
/**
* RAFT = 2;
*/
public static final int RAFT_VALUE = 2;
public final int getNumber() {
if (this == UNRECOGNIZED) {
throw new java.lang.IllegalArgumentException(
"Can't get the number of an unknown enum value.");
}
return value;
}
/**
* @deprecated Use {@link #forNumber(int)} instead.
*/
@java.lang.Deprecated
public static DB valueOf(int value) {
return forNumber(value);
}
public static DB forNumber(int value) {
switch (value) {
case 0: return INVALID;
case 1: return KV;
case 2: return RAFT;
default: return null;
}
}
public static com.google.protobuf.Internal.EnumLiteMap
internalGetValueMap() {
return internalValueMap;
}
private static final com.google.protobuf.Internal.EnumLiteMap<
DB> internalValueMap =
new com.google.protobuf.Internal.EnumLiteMap() {
public DB findValueByNumber(int number) {
return DB.forNumber(number);
}
};
public final com.google.protobuf.Descriptors.EnumValueDescriptor
getValueDescriptor() {
return getDescriptor().getValues().get(ordinal());
}
public final com.google.protobuf.Descriptors.EnumDescriptor
getDescriptorForType() {
return getDescriptor();
}
public static final com.google.protobuf.Descriptors.EnumDescriptor
getDescriptor() {
return org.tikv.kvproto.Debugpb.getDescriptor().getEnumTypes().get(0);
}
private static final DB[] VALUES = values();
public static DB valueOf(
com.google.protobuf.Descriptors.EnumValueDescriptor desc) {
if (desc.getType() != getDescriptor()) {
throw new java.lang.IllegalArgumentException(
"EnumValueDescriptor is not for this type.");
}
if (desc.getIndex() == -1) {
return UNRECOGNIZED;
}
return VALUES[desc.getIndex()];
}
private final int value;
private DB(int value) {
this.value = value;
}
// @@protoc_insertion_point(enum_scope:debugpb.DB)
}
/**
* Protobuf enum {@code debugpb.MODULE}
*/
public enum MODULE
implements com.google.protobuf.ProtocolMessageEnum {
/**
* UNUSED = 0;
*/
UNUSED(0),
/**
* KVDB = 1;
*/
KVDB(1),
/**
* RAFTDB = 2;
*/
RAFTDB(2),
/**
* READPOOL = 3;
*/
READPOOL(3),
/**
* SERVER = 4;
*/
SERVER(4),
/**
* STORAGE = 5;
*/
STORAGE(5),
/**
* PD = 6;
*/
PD(6),
/**
* METRIC = 7;
*/
METRIC(7),
/**
* COPROCESSOR = 8;
*/
COPROCESSOR(8),
/**
* SECURITY = 9;
*/
SECURITY(9),
/**
* IMPORT = 10;
*/
IMPORT(10),
UNRECOGNIZED(-1),
;
/**
* UNUSED = 0;
*/
public static final int UNUSED_VALUE = 0;
/**
* KVDB = 1;
*/
public static final int KVDB_VALUE = 1;
/**
* RAFTDB = 2;
*/
public static final int RAFTDB_VALUE = 2;
/**
* READPOOL = 3;
*/
public static final int READPOOL_VALUE = 3;
/**
* SERVER = 4;
*/
public static final int SERVER_VALUE = 4;
/**
* STORAGE = 5;
*/
public static final int STORAGE_VALUE = 5;
/**
* PD = 6;
*/
public static final int PD_VALUE = 6;
/**
* METRIC = 7;
*/
public static final int METRIC_VALUE = 7;
/**
* COPROCESSOR = 8;
*/
public static final int COPROCESSOR_VALUE = 8;
/**
* SECURITY = 9;
*/
public static final int SECURITY_VALUE = 9;
/**
* IMPORT = 10;
*/
public static final int IMPORT_VALUE = 10;
public final int getNumber() {
if (this == UNRECOGNIZED) {
throw new java.lang.IllegalArgumentException(
"Can't get the number of an unknown enum value.");
}
return value;
}
/**
* @deprecated Use {@link #forNumber(int)} instead.
*/
@java.lang.Deprecated
public static MODULE valueOf(int value) {
return forNumber(value);
}
public static MODULE forNumber(int value) {
switch (value) {
case 0: return UNUSED;
case 1: return KVDB;
case 2: return RAFTDB;
case 3: return READPOOL;
case 4: return SERVER;
case 5: return STORAGE;
case 6: return PD;
case 7: return METRIC;
case 8: return COPROCESSOR;
case 9: return SECURITY;
case 10: return IMPORT;
default: return null;
}
}
public static com.google.protobuf.Internal.EnumLiteMap
internalGetValueMap() {
return internalValueMap;
}
private static final com.google.protobuf.Internal.EnumLiteMap<
MODULE> internalValueMap =
new com.google.protobuf.Internal.EnumLiteMap() {
public MODULE findValueByNumber(int number) {
return MODULE.forNumber(number);
}
};
public final com.google.protobuf.Descriptors.EnumValueDescriptor
getValueDescriptor() {
return getDescriptor().getValues().get(ordinal());
}
public final com.google.protobuf.Descriptors.EnumDescriptor
getDescriptorForType() {
return getDescriptor();
}
public static final com.google.protobuf.Descriptors.EnumDescriptor
getDescriptor() {
return org.tikv.kvproto.Debugpb.getDescriptor().getEnumTypes().get(1);
}
private static final MODULE[] VALUES = values();
public static MODULE valueOf(
com.google.protobuf.Descriptors.EnumValueDescriptor desc) {
if (desc.getType() != getDescriptor()) {
throw new java.lang.IllegalArgumentException(
"EnumValueDescriptor is not for this type.");
}
if (desc.getIndex() == -1) {
return UNRECOGNIZED;
}
return VALUES[desc.getIndex()];
}
private final int value;
private MODULE(int value) {
this.value = value;
}
// @@protoc_insertion_point(enum_scope:debugpb.MODULE)
}
/**
* Protobuf enum {@code debugpb.BottommostLevelCompaction}
*/
public enum BottommostLevelCompaction
implements com.google.protobuf.ProtocolMessageEnum {
/**
*
* Skip bottommost level compaction
*
*
* Skip = 0;
*/
Skip(0),
/**
*
* Force bottommost level compaction
*
*
* Force = 1;
*/
Force(1),
/**
*
* Compact bottommost level if there is a compaction filter.
*
*
* IfHaveCompactionFilter = 2;
*/
IfHaveCompactionFilter(2),
UNRECOGNIZED(-1),
;
/**
*
* Skip bottommost level compaction
*
*
* Skip = 0;
*/
public static final int Skip_VALUE = 0;
/**
*
* Force bottommost level compaction
*
*
* Force = 1;
*/
public static final int Force_VALUE = 1;
/**
*
* Compact bottommost level if there is a compaction filter.
*
*
* IfHaveCompactionFilter = 2;
*/
public static final int IfHaveCompactionFilter_VALUE = 2;
public final int getNumber() {
if (this == UNRECOGNIZED) {
throw new java.lang.IllegalArgumentException(
"Can't get the number of an unknown enum value.");
}
return value;
}
/**
* @deprecated Use {@link #forNumber(int)} instead.
*/
@java.lang.Deprecated
public static BottommostLevelCompaction valueOf(int value) {
return forNumber(value);
}
public static BottommostLevelCompaction forNumber(int value) {
switch (value) {
case 0: return Skip;
case 1: return Force;
case 2: return IfHaveCompactionFilter;
default: return null;
}
}
public static com.google.protobuf.Internal.EnumLiteMap
internalGetValueMap() {
return internalValueMap;
}
private static final com.google.protobuf.Internal.EnumLiteMap<
BottommostLevelCompaction> internalValueMap =
new com.google.protobuf.Internal.EnumLiteMap() {
public BottommostLevelCompaction findValueByNumber(int number) {
return BottommostLevelCompaction.forNumber(number);
}
};
public final com.google.protobuf.Descriptors.EnumValueDescriptor
getValueDescriptor() {
return getDescriptor().getValues().get(ordinal());
}
public final com.google.protobuf.Descriptors.EnumDescriptor
getDescriptorForType() {
return getDescriptor();
}
public static final com.google.protobuf.Descriptors.EnumDescriptor
getDescriptor() {
return org.tikv.kvproto.Debugpb.getDescriptor().getEnumTypes().get(2);
}
private static final BottommostLevelCompaction[] VALUES = values();
public static BottommostLevelCompaction valueOf(
com.google.protobuf.Descriptors.EnumValueDescriptor desc) {
if (desc.getType() != getDescriptor()) {
throw new java.lang.IllegalArgumentException(
"EnumValueDescriptor is not for this type.");
}
if (desc.getIndex() == -1) {
return UNRECOGNIZED;
}
return VALUES[desc.getIndex()];
}
private final int value;
private BottommostLevelCompaction(int value) {
this.value = value;
}
// @@protoc_insertion_point(enum_scope:debugpb.BottommostLevelCompaction)
}
public interface GetRequestOrBuilder extends
// @@protoc_insertion_point(interface_extends:debugpb.GetRequest)
com.google.protobuf.MessageOrBuilder {
/**
* .debugpb.DB db = 1;
*/
int getDbValue();
/**
* .debugpb.DB db = 1;
*/
org.tikv.kvproto.Debugpb.DB getDb();
/**
* string cf = 2;
*/
java.lang.String getCf();
/**
* string cf = 2;
*/
com.google.protobuf.ByteString
getCfBytes();
/**
* bytes key = 3;
*/
com.google.protobuf.ByteString getKey();
}
/**
* Protobuf type {@code debugpb.GetRequest}
*/
public static final class GetRequest extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:debugpb.GetRequest)
GetRequestOrBuilder {
private static final long serialVersionUID = 0L;
// Use GetRequest.newBuilder() to construct.
private GetRequest(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private GetRequest() {
db_ = 0;
cf_ = "";
key_ = com.google.protobuf.ByteString.EMPTY;
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private GetRequest(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
default: {
if (!parseUnknownFieldProto3(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
case 8: {
int rawValue = input.readEnum();
db_ = rawValue;
break;
}
case 18: {
java.lang.String s = input.readStringRequireUtf8();
cf_ = s;
break;
}
case 26: {
key_ = input.readBytes();
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.tikv.kvproto.Debugpb.internal_static_debugpb_GetRequest_descriptor;
}
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.tikv.kvproto.Debugpb.internal_static_debugpb_GetRequest_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.tikv.kvproto.Debugpb.GetRequest.class, org.tikv.kvproto.Debugpb.GetRequest.Builder.class);
}
public static final int DB_FIELD_NUMBER = 1;
private int db_;
/**
* .debugpb.DB db = 1;
*/
public int getDbValue() {
return db_;
}
/**
* .debugpb.DB db = 1;
*/
public org.tikv.kvproto.Debugpb.DB getDb() {
org.tikv.kvproto.Debugpb.DB result = org.tikv.kvproto.Debugpb.DB.valueOf(db_);
return result == null ? org.tikv.kvproto.Debugpb.DB.UNRECOGNIZED : result;
}
public static final int CF_FIELD_NUMBER = 2;
private volatile java.lang.Object cf_;
/**
* string cf = 2;
*/
public java.lang.String getCf() {
java.lang.Object ref = cf_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
cf_ = s;
return s;
}
}
/**
* string cf = 2;
*/
public com.google.protobuf.ByteString
getCfBytes() {
java.lang.Object ref = cf_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
cf_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int KEY_FIELD_NUMBER = 3;
private com.google.protobuf.ByteString key_;
/**
* bytes key = 3;
*/
public com.google.protobuf.ByteString getKey() {
return key_;
}
private byte memoizedIsInitialized = -1;
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (db_ != org.tikv.kvproto.Debugpb.DB.INVALID.getNumber()) {
output.writeEnum(1, db_);
}
if (!getCfBytes().isEmpty()) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 2, cf_);
}
if (!key_.isEmpty()) {
output.writeBytes(3, key_);
}
unknownFields.writeTo(output);
}
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (db_ != org.tikv.kvproto.Debugpb.DB.INVALID.getNumber()) {
size += com.google.protobuf.CodedOutputStream
.computeEnumSize(1, db_);
}
if (!getCfBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(2, cf_);
}
if (!key_.isEmpty()) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(3, key_);
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof org.tikv.kvproto.Debugpb.GetRequest)) {
return super.equals(obj);
}
org.tikv.kvproto.Debugpb.GetRequest other = (org.tikv.kvproto.Debugpb.GetRequest) obj;
boolean result = true;
result = result && db_ == other.db_;
result = result && getCf()
.equals(other.getCf());
result = result && getKey()
.equals(other.getKey());
result = result && unknownFields.equals(other.unknownFields);
return result;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
hash = (37 * hash) + DB_FIELD_NUMBER;
hash = (53 * hash) + db_;
hash = (37 * hash) + CF_FIELD_NUMBER;
hash = (53 * hash) + getCf().hashCode();
hash = (37 * hash) + KEY_FIELD_NUMBER;
hash = (53 * hash) + getKey().hashCode();
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static org.tikv.kvproto.Debugpb.GetRequest parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.tikv.kvproto.Debugpb.GetRequest parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.tikv.kvproto.Debugpb.GetRequest parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.tikv.kvproto.Debugpb.GetRequest parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.tikv.kvproto.Debugpb.GetRequest parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.tikv.kvproto.Debugpb.GetRequest parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.tikv.kvproto.Debugpb.GetRequest parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static org.tikv.kvproto.Debugpb.GetRequest parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static org.tikv.kvproto.Debugpb.GetRequest parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static org.tikv.kvproto.Debugpb.GetRequest parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static org.tikv.kvproto.Debugpb.GetRequest parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static org.tikv.kvproto.Debugpb.GetRequest parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(org.tikv.kvproto.Debugpb.GetRequest prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code debugpb.GetRequest}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:debugpb.GetRequest)
org.tikv.kvproto.Debugpb.GetRequestOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.tikv.kvproto.Debugpb.internal_static_debugpb_GetRequest_descriptor;
}
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.tikv.kvproto.Debugpb.internal_static_debugpb_GetRequest_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.tikv.kvproto.Debugpb.GetRequest.class, org.tikv.kvproto.Debugpb.GetRequest.Builder.class);
}
// Construct using org.tikv.kvproto.Debugpb.GetRequest.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
public Builder clear() {
super.clear();
db_ = 0;
cf_ = "";
key_ = com.google.protobuf.ByteString.EMPTY;
return this;
}
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return org.tikv.kvproto.Debugpb.internal_static_debugpb_GetRequest_descriptor;
}
public org.tikv.kvproto.Debugpb.GetRequest getDefaultInstanceForType() {
return org.tikv.kvproto.Debugpb.GetRequest.getDefaultInstance();
}
public org.tikv.kvproto.Debugpb.GetRequest build() {
org.tikv.kvproto.Debugpb.GetRequest result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
public org.tikv.kvproto.Debugpb.GetRequest buildPartial() {
org.tikv.kvproto.Debugpb.GetRequest result = new org.tikv.kvproto.Debugpb.GetRequest(this);
result.db_ = db_;
result.cf_ = cf_;
result.key_ = key_;
onBuilt();
return result;
}
public Builder clone() {
return (Builder) super.clone();
}
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.setField(field, value);
}
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return (Builder) super.clearField(field);
}
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return (Builder) super.clearOneof(oneof);
}
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return (Builder) super.setRepeatedField(field, index, value);
}
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.addRepeatedField(field, value);
}
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof org.tikv.kvproto.Debugpb.GetRequest) {
return mergeFrom((org.tikv.kvproto.Debugpb.GetRequest)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(org.tikv.kvproto.Debugpb.GetRequest other) {
if (other == org.tikv.kvproto.Debugpb.GetRequest.getDefaultInstance()) return this;
if (other.db_ != 0) {
setDbValue(other.getDbValue());
}
if (!other.getCf().isEmpty()) {
cf_ = other.cf_;
onChanged();
}
if (other.getKey() != com.google.protobuf.ByteString.EMPTY) {
setKey(other.getKey());
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
public final boolean isInitialized() {
return true;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
org.tikv.kvproto.Debugpb.GetRequest parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (org.tikv.kvproto.Debugpb.GetRequest) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int db_ = 0;
/**
* .debugpb.DB db = 1;
*/
public int getDbValue() {
return db_;
}
/**
* .debugpb.DB db = 1;
*/
public Builder setDbValue(int value) {
db_ = value;
onChanged();
return this;
}
/**
* .debugpb.DB db = 1;
*/
public org.tikv.kvproto.Debugpb.DB getDb() {
org.tikv.kvproto.Debugpb.DB result = org.tikv.kvproto.Debugpb.DB.valueOf(db_);
return result == null ? org.tikv.kvproto.Debugpb.DB.UNRECOGNIZED : result;
}
/**
* .debugpb.DB db = 1;
*/
public Builder setDb(org.tikv.kvproto.Debugpb.DB value) {
if (value == null) {
throw new NullPointerException();
}
db_ = value.getNumber();
onChanged();
return this;
}
/**
* .debugpb.DB db = 1;
*/
public Builder clearDb() {
db_ = 0;
onChanged();
return this;
}
private java.lang.Object cf_ = "";
/**
* string cf = 2;
*/
public java.lang.String getCf() {
java.lang.Object ref = cf_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
cf_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* string cf = 2;
*/
public com.google.protobuf.ByteString
getCfBytes() {
java.lang.Object ref = cf_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
cf_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* string cf = 2;
*/
public Builder setCf(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
cf_ = value;
onChanged();
return this;
}
/**
* string cf = 2;
*/
public Builder clearCf() {
cf_ = getDefaultInstance().getCf();
onChanged();
return this;
}
/**
* string cf = 2;
*/
public Builder setCfBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
cf_ = value;
onChanged();
return this;
}
private com.google.protobuf.ByteString key_ = com.google.protobuf.ByteString.EMPTY;
/**
* bytes key = 3;
*/
public com.google.protobuf.ByteString getKey() {
return key_;
}
/**
* bytes key = 3;
*/
public Builder setKey(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
key_ = value;
onChanged();
return this;
}
/**
* bytes key = 3;
*/
public Builder clearKey() {
key_ = getDefaultInstance().getKey();
onChanged();
return this;
}
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFieldsProto3(unknownFields);
}
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:debugpb.GetRequest)
}
// @@protoc_insertion_point(class_scope:debugpb.GetRequest)
private static final org.tikv.kvproto.Debugpb.GetRequest DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new org.tikv.kvproto.Debugpb.GetRequest();
}
public static org.tikv.kvproto.Debugpb.GetRequest getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
public GetRequest parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new GetRequest(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
public org.tikv.kvproto.Debugpb.GetRequest getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface GetResponseOrBuilder extends
// @@protoc_insertion_point(interface_extends:debugpb.GetResponse)
com.google.protobuf.MessageOrBuilder {
/**
* bytes value = 1;
*/
com.google.protobuf.ByteString getValue();
}
/**
* Protobuf type {@code debugpb.GetResponse}
*/
public static final class GetResponse extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:debugpb.GetResponse)
GetResponseOrBuilder {
private static final long serialVersionUID = 0L;
// Use GetResponse.newBuilder() to construct.
private GetResponse(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private GetResponse() {
value_ = com.google.protobuf.ByteString.EMPTY;
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private GetResponse(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
default: {
if (!parseUnknownFieldProto3(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
case 10: {
value_ = input.readBytes();
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.tikv.kvproto.Debugpb.internal_static_debugpb_GetResponse_descriptor;
}
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.tikv.kvproto.Debugpb.internal_static_debugpb_GetResponse_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.tikv.kvproto.Debugpb.GetResponse.class, org.tikv.kvproto.Debugpb.GetResponse.Builder.class);
}
public static final int VALUE_FIELD_NUMBER = 1;
private com.google.protobuf.ByteString value_;
/**
* bytes value = 1;
*/
public com.google.protobuf.ByteString getValue() {
return value_;
}
private byte memoizedIsInitialized = -1;
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (!value_.isEmpty()) {
output.writeBytes(1, value_);
}
unknownFields.writeTo(output);
}
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (!value_.isEmpty()) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(1, value_);
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof org.tikv.kvproto.Debugpb.GetResponse)) {
return super.equals(obj);
}
org.tikv.kvproto.Debugpb.GetResponse other = (org.tikv.kvproto.Debugpb.GetResponse) obj;
boolean result = true;
result = result && getValue()
.equals(other.getValue());
result = result && unknownFields.equals(other.unknownFields);
return result;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
hash = (37 * hash) + VALUE_FIELD_NUMBER;
hash = (53 * hash) + getValue().hashCode();
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static org.tikv.kvproto.Debugpb.GetResponse parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.tikv.kvproto.Debugpb.GetResponse parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.tikv.kvproto.Debugpb.GetResponse parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.tikv.kvproto.Debugpb.GetResponse parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.tikv.kvproto.Debugpb.GetResponse parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.tikv.kvproto.Debugpb.GetResponse parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.tikv.kvproto.Debugpb.GetResponse parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static org.tikv.kvproto.Debugpb.GetResponse parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static org.tikv.kvproto.Debugpb.GetResponse parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static org.tikv.kvproto.Debugpb.GetResponse parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static org.tikv.kvproto.Debugpb.GetResponse parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static org.tikv.kvproto.Debugpb.GetResponse parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(org.tikv.kvproto.Debugpb.GetResponse prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code debugpb.GetResponse}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:debugpb.GetResponse)
org.tikv.kvproto.Debugpb.GetResponseOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.tikv.kvproto.Debugpb.internal_static_debugpb_GetResponse_descriptor;
}
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.tikv.kvproto.Debugpb.internal_static_debugpb_GetResponse_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.tikv.kvproto.Debugpb.GetResponse.class, org.tikv.kvproto.Debugpb.GetResponse.Builder.class);
}
// Construct using org.tikv.kvproto.Debugpb.GetResponse.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
public Builder clear() {
super.clear();
value_ = com.google.protobuf.ByteString.EMPTY;
return this;
}
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return org.tikv.kvproto.Debugpb.internal_static_debugpb_GetResponse_descriptor;
}
public org.tikv.kvproto.Debugpb.GetResponse getDefaultInstanceForType() {
return org.tikv.kvproto.Debugpb.GetResponse.getDefaultInstance();
}
public org.tikv.kvproto.Debugpb.GetResponse build() {
org.tikv.kvproto.Debugpb.GetResponse result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
public org.tikv.kvproto.Debugpb.GetResponse buildPartial() {
org.tikv.kvproto.Debugpb.GetResponse result = new org.tikv.kvproto.Debugpb.GetResponse(this);
result.value_ = value_;
onBuilt();
return result;
}
public Builder clone() {
return (Builder) super.clone();
}
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.setField(field, value);
}
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return (Builder) super.clearField(field);
}
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return (Builder) super.clearOneof(oneof);
}
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return (Builder) super.setRepeatedField(field, index, value);
}
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.addRepeatedField(field, value);
}
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof org.tikv.kvproto.Debugpb.GetResponse) {
return mergeFrom((org.tikv.kvproto.Debugpb.GetResponse)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(org.tikv.kvproto.Debugpb.GetResponse other) {
if (other == org.tikv.kvproto.Debugpb.GetResponse.getDefaultInstance()) return this;
if (other.getValue() != com.google.protobuf.ByteString.EMPTY) {
setValue(other.getValue());
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
public final boolean isInitialized() {
return true;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
org.tikv.kvproto.Debugpb.GetResponse parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (org.tikv.kvproto.Debugpb.GetResponse) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private com.google.protobuf.ByteString value_ = com.google.protobuf.ByteString.EMPTY;
/**
* bytes value = 1;
*/
public com.google.protobuf.ByteString getValue() {
return value_;
}
/**
* bytes value = 1;
*/
public Builder setValue(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
value_ = value;
onChanged();
return this;
}
/**
* bytes value = 1;
*/
public Builder clearValue() {
value_ = getDefaultInstance().getValue();
onChanged();
return this;
}
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFieldsProto3(unknownFields);
}
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:debugpb.GetResponse)
}
// @@protoc_insertion_point(class_scope:debugpb.GetResponse)
private static final org.tikv.kvproto.Debugpb.GetResponse DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new org.tikv.kvproto.Debugpb.GetResponse();
}
public static org.tikv.kvproto.Debugpb.GetResponse getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
public GetResponse parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new GetResponse(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
public org.tikv.kvproto.Debugpb.GetResponse getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface RaftLogRequestOrBuilder extends
// @@protoc_insertion_point(interface_extends:debugpb.RaftLogRequest)
com.google.protobuf.MessageOrBuilder {
/**
* uint64 region_id = 1;
*/
long getRegionId();
/**
* uint64 log_index = 2;
*/
long getLogIndex();
}
/**
* Protobuf type {@code debugpb.RaftLogRequest}
*/
public static final class RaftLogRequest extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:debugpb.RaftLogRequest)
RaftLogRequestOrBuilder {
private static final long serialVersionUID = 0L;
// Use RaftLogRequest.newBuilder() to construct.
private RaftLogRequest(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private RaftLogRequest() {
regionId_ = 0L;
logIndex_ = 0L;
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private RaftLogRequest(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
default: {
if (!parseUnknownFieldProto3(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
case 8: {
regionId_ = input.readUInt64();
break;
}
case 16: {
logIndex_ = input.readUInt64();
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.tikv.kvproto.Debugpb.internal_static_debugpb_RaftLogRequest_descriptor;
}
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.tikv.kvproto.Debugpb.internal_static_debugpb_RaftLogRequest_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.tikv.kvproto.Debugpb.RaftLogRequest.class, org.tikv.kvproto.Debugpb.RaftLogRequest.Builder.class);
}
public static final int REGION_ID_FIELD_NUMBER = 1;
private long regionId_;
/**
* uint64 region_id = 1;
*/
public long getRegionId() {
return regionId_;
}
public static final int LOG_INDEX_FIELD_NUMBER = 2;
private long logIndex_;
/**
* uint64 log_index = 2;
*/
public long getLogIndex() {
return logIndex_;
}
private byte memoizedIsInitialized = -1;
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (regionId_ != 0L) {
output.writeUInt64(1, regionId_);
}
if (logIndex_ != 0L) {
output.writeUInt64(2, logIndex_);
}
unknownFields.writeTo(output);
}
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (regionId_ != 0L) {
size += com.google.protobuf.CodedOutputStream
.computeUInt64Size(1, regionId_);
}
if (logIndex_ != 0L) {
size += com.google.protobuf.CodedOutputStream
.computeUInt64Size(2, logIndex_);
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof org.tikv.kvproto.Debugpb.RaftLogRequest)) {
return super.equals(obj);
}
org.tikv.kvproto.Debugpb.RaftLogRequest other = (org.tikv.kvproto.Debugpb.RaftLogRequest) obj;
boolean result = true;
result = result && (getRegionId()
== other.getRegionId());
result = result && (getLogIndex()
== other.getLogIndex());
result = result && unknownFields.equals(other.unknownFields);
return result;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
hash = (37 * hash) + REGION_ID_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
getRegionId());
hash = (37 * hash) + LOG_INDEX_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
getLogIndex());
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static org.tikv.kvproto.Debugpb.RaftLogRequest parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.tikv.kvproto.Debugpb.RaftLogRequest parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.tikv.kvproto.Debugpb.RaftLogRequest parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.tikv.kvproto.Debugpb.RaftLogRequest parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.tikv.kvproto.Debugpb.RaftLogRequest parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.tikv.kvproto.Debugpb.RaftLogRequest parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.tikv.kvproto.Debugpb.RaftLogRequest parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static org.tikv.kvproto.Debugpb.RaftLogRequest parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static org.tikv.kvproto.Debugpb.RaftLogRequest parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static org.tikv.kvproto.Debugpb.RaftLogRequest parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static org.tikv.kvproto.Debugpb.RaftLogRequest parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static org.tikv.kvproto.Debugpb.RaftLogRequest parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(org.tikv.kvproto.Debugpb.RaftLogRequest prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code debugpb.RaftLogRequest}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:debugpb.RaftLogRequest)
org.tikv.kvproto.Debugpb.RaftLogRequestOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.tikv.kvproto.Debugpb.internal_static_debugpb_RaftLogRequest_descriptor;
}
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.tikv.kvproto.Debugpb.internal_static_debugpb_RaftLogRequest_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.tikv.kvproto.Debugpb.RaftLogRequest.class, org.tikv.kvproto.Debugpb.RaftLogRequest.Builder.class);
}
// Construct using org.tikv.kvproto.Debugpb.RaftLogRequest.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
public Builder clear() {
super.clear();
regionId_ = 0L;
logIndex_ = 0L;
return this;
}
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return org.tikv.kvproto.Debugpb.internal_static_debugpb_RaftLogRequest_descriptor;
}
public org.tikv.kvproto.Debugpb.RaftLogRequest getDefaultInstanceForType() {
return org.tikv.kvproto.Debugpb.RaftLogRequest.getDefaultInstance();
}
public org.tikv.kvproto.Debugpb.RaftLogRequest build() {
org.tikv.kvproto.Debugpb.RaftLogRequest result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
public org.tikv.kvproto.Debugpb.RaftLogRequest buildPartial() {
org.tikv.kvproto.Debugpb.RaftLogRequest result = new org.tikv.kvproto.Debugpb.RaftLogRequest(this);
result.regionId_ = regionId_;
result.logIndex_ = logIndex_;
onBuilt();
return result;
}
public Builder clone() {
return (Builder) super.clone();
}
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.setField(field, value);
}
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return (Builder) super.clearField(field);
}
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return (Builder) super.clearOneof(oneof);
}
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return (Builder) super.setRepeatedField(field, index, value);
}
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.addRepeatedField(field, value);
}
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof org.tikv.kvproto.Debugpb.RaftLogRequest) {
return mergeFrom((org.tikv.kvproto.Debugpb.RaftLogRequest)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(org.tikv.kvproto.Debugpb.RaftLogRequest other) {
if (other == org.tikv.kvproto.Debugpb.RaftLogRequest.getDefaultInstance()) return this;
if (other.getRegionId() != 0L) {
setRegionId(other.getRegionId());
}
if (other.getLogIndex() != 0L) {
setLogIndex(other.getLogIndex());
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
public final boolean isInitialized() {
return true;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
org.tikv.kvproto.Debugpb.RaftLogRequest parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (org.tikv.kvproto.Debugpb.RaftLogRequest) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private long regionId_ ;
/**
* uint64 region_id = 1;
*/
public long getRegionId() {
return regionId_;
}
/**
* uint64 region_id = 1;
*/
public Builder setRegionId(long value) {
regionId_ = value;
onChanged();
return this;
}
/**
* uint64 region_id = 1;
*/
public Builder clearRegionId() {
regionId_ = 0L;
onChanged();
return this;
}
private long logIndex_ ;
/**
* uint64 log_index = 2;
*/
public long getLogIndex() {
return logIndex_;
}
/**
* uint64 log_index = 2;
*/
public Builder setLogIndex(long value) {
logIndex_ = value;
onChanged();
return this;
}
/**
* uint64 log_index = 2;
*/
public Builder clearLogIndex() {
logIndex_ = 0L;
onChanged();
return this;
}
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFieldsProto3(unknownFields);
}
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:debugpb.RaftLogRequest)
}
// @@protoc_insertion_point(class_scope:debugpb.RaftLogRequest)
private static final org.tikv.kvproto.Debugpb.RaftLogRequest DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new org.tikv.kvproto.Debugpb.RaftLogRequest();
}
public static org.tikv.kvproto.Debugpb.RaftLogRequest getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
public RaftLogRequest parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new RaftLogRequest(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
public org.tikv.kvproto.Debugpb.RaftLogRequest getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface RaftLogResponseOrBuilder extends
// @@protoc_insertion_point(interface_extends:debugpb.RaftLogResponse)
com.google.protobuf.MessageOrBuilder {
/**
* .eraftpb.Entry entry = 1;
*/
boolean hasEntry();
/**
* .eraftpb.Entry entry = 1;
*/
eraftpb.Eraftpb.Entry getEntry();
/**
* .eraftpb.Entry entry = 1;
*/
eraftpb.Eraftpb.EntryOrBuilder getEntryOrBuilder();
}
/**
* Protobuf type {@code debugpb.RaftLogResponse}
*/
public static final class RaftLogResponse extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:debugpb.RaftLogResponse)
RaftLogResponseOrBuilder {
private static final long serialVersionUID = 0L;
// Use RaftLogResponse.newBuilder() to construct.
private RaftLogResponse(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private RaftLogResponse() {
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private RaftLogResponse(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
default: {
if (!parseUnknownFieldProto3(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
case 10: {
eraftpb.Eraftpb.Entry.Builder subBuilder = null;
if (entry_ != null) {
subBuilder = entry_.toBuilder();
}
entry_ = input.readMessage(eraftpb.Eraftpb.Entry.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(entry_);
entry_ = subBuilder.buildPartial();
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.tikv.kvproto.Debugpb.internal_static_debugpb_RaftLogResponse_descriptor;
}
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.tikv.kvproto.Debugpb.internal_static_debugpb_RaftLogResponse_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.tikv.kvproto.Debugpb.RaftLogResponse.class, org.tikv.kvproto.Debugpb.RaftLogResponse.Builder.class);
}
public static final int ENTRY_FIELD_NUMBER = 1;
private eraftpb.Eraftpb.Entry entry_;
/**
* .eraftpb.Entry entry = 1;
*/
public boolean hasEntry() {
return entry_ != null;
}
/**
* .eraftpb.Entry entry = 1;
*/
public eraftpb.Eraftpb.Entry getEntry() {
return entry_ == null ? eraftpb.Eraftpb.Entry.getDefaultInstance() : entry_;
}
/**
* .eraftpb.Entry entry = 1;
*/
public eraftpb.Eraftpb.EntryOrBuilder getEntryOrBuilder() {
return getEntry();
}
private byte memoizedIsInitialized = -1;
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (entry_ != null) {
output.writeMessage(1, getEntry());
}
unknownFields.writeTo(output);
}
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (entry_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(1, getEntry());
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof org.tikv.kvproto.Debugpb.RaftLogResponse)) {
return super.equals(obj);
}
org.tikv.kvproto.Debugpb.RaftLogResponse other = (org.tikv.kvproto.Debugpb.RaftLogResponse) obj;
boolean result = true;
result = result && (hasEntry() == other.hasEntry());
if (hasEntry()) {
result = result && getEntry()
.equals(other.getEntry());
}
result = result && unknownFields.equals(other.unknownFields);
return result;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (hasEntry()) {
hash = (37 * hash) + ENTRY_FIELD_NUMBER;
hash = (53 * hash) + getEntry().hashCode();
}
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static org.tikv.kvproto.Debugpb.RaftLogResponse parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.tikv.kvproto.Debugpb.RaftLogResponse parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.tikv.kvproto.Debugpb.RaftLogResponse parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.tikv.kvproto.Debugpb.RaftLogResponse parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.tikv.kvproto.Debugpb.RaftLogResponse parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.tikv.kvproto.Debugpb.RaftLogResponse parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.tikv.kvproto.Debugpb.RaftLogResponse parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static org.tikv.kvproto.Debugpb.RaftLogResponse parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static org.tikv.kvproto.Debugpb.RaftLogResponse parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static org.tikv.kvproto.Debugpb.RaftLogResponse parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static org.tikv.kvproto.Debugpb.RaftLogResponse parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static org.tikv.kvproto.Debugpb.RaftLogResponse parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(org.tikv.kvproto.Debugpb.RaftLogResponse prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code debugpb.RaftLogResponse}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:debugpb.RaftLogResponse)
org.tikv.kvproto.Debugpb.RaftLogResponseOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.tikv.kvproto.Debugpb.internal_static_debugpb_RaftLogResponse_descriptor;
}
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.tikv.kvproto.Debugpb.internal_static_debugpb_RaftLogResponse_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.tikv.kvproto.Debugpb.RaftLogResponse.class, org.tikv.kvproto.Debugpb.RaftLogResponse.Builder.class);
}
// Construct using org.tikv.kvproto.Debugpb.RaftLogResponse.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
public Builder clear() {
super.clear();
if (entryBuilder_ == null) {
entry_ = null;
} else {
entry_ = null;
entryBuilder_ = null;
}
return this;
}
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return org.tikv.kvproto.Debugpb.internal_static_debugpb_RaftLogResponse_descriptor;
}
public org.tikv.kvproto.Debugpb.RaftLogResponse getDefaultInstanceForType() {
return org.tikv.kvproto.Debugpb.RaftLogResponse.getDefaultInstance();
}
public org.tikv.kvproto.Debugpb.RaftLogResponse build() {
org.tikv.kvproto.Debugpb.RaftLogResponse result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
public org.tikv.kvproto.Debugpb.RaftLogResponse buildPartial() {
org.tikv.kvproto.Debugpb.RaftLogResponse result = new org.tikv.kvproto.Debugpb.RaftLogResponse(this);
if (entryBuilder_ == null) {
result.entry_ = entry_;
} else {
result.entry_ = entryBuilder_.build();
}
onBuilt();
return result;
}
public Builder clone() {
return (Builder) super.clone();
}
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.setField(field, value);
}
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return (Builder) super.clearField(field);
}
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return (Builder) super.clearOneof(oneof);
}
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return (Builder) super.setRepeatedField(field, index, value);
}
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.addRepeatedField(field, value);
}
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof org.tikv.kvproto.Debugpb.RaftLogResponse) {
return mergeFrom((org.tikv.kvproto.Debugpb.RaftLogResponse)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(org.tikv.kvproto.Debugpb.RaftLogResponse other) {
if (other == org.tikv.kvproto.Debugpb.RaftLogResponse.getDefaultInstance()) return this;
if (other.hasEntry()) {
mergeEntry(other.getEntry());
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
public final boolean isInitialized() {
return true;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
org.tikv.kvproto.Debugpb.RaftLogResponse parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (org.tikv.kvproto.Debugpb.RaftLogResponse) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private eraftpb.Eraftpb.Entry entry_ = null;
private com.google.protobuf.SingleFieldBuilderV3<
eraftpb.Eraftpb.Entry, eraftpb.Eraftpb.Entry.Builder, eraftpb.Eraftpb.EntryOrBuilder> entryBuilder_;
/**
* .eraftpb.Entry entry = 1;
*/
public boolean hasEntry() {
return entryBuilder_ != null || entry_ != null;
}
/**
* .eraftpb.Entry entry = 1;
*/
public eraftpb.Eraftpb.Entry getEntry() {
if (entryBuilder_ == null) {
return entry_ == null ? eraftpb.Eraftpb.Entry.getDefaultInstance() : entry_;
} else {
return entryBuilder_.getMessage();
}
}
/**
* .eraftpb.Entry entry = 1;
*/
public Builder setEntry(eraftpb.Eraftpb.Entry value) {
if (entryBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
entry_ = value;
onChanged();
} else {
entryBuilder_.setMessage(value);
}
return this;
}
/**
* .eraftpb.Entry entry = 1;
*/
public Builder setEntry(
eraftpb.Eraftpb.Entry.Builder builderForValue) {
if (entryBuilder_ == null) {
entry_ = builderForValue.build();
onChanged();
} else {
entryBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
* .eraftpb.Entry entry = 1;
*/
public Builder mergeEntry(eraftpb.Eraftpb.Entry value) {
if (entryBuilder_ == null) {
if (entry_ != null) {
entry_ =
eraftpb.Eraftpb.Entry.newBuilder(entry_).mergeFrom(value).buildPartial();
} else {
entry_ = value;
}
onChanged();
} else {
entryBuilder_.mergeFrom(value);
}
return this;
}
/**
* .eraftpb.Entry entry = 1;
*/
public Builder clearEntry() {
if (entryBuilder_ == null) {
entry_ = null;
onChanged();
} else {
entry_ = null;
entryBuilder_ = null;
}
return this;
}
/**
* .eraftpb.Entry entry = 1;
*/
public eraftpb.Eraftpb.Entry.Builder getEntryBuilder() {
onChanged();
return getEntryFieldBuilder().getBuilder();
}
/**
* .eraftpb.Entry entry = 1;
*/
public eraftpb.Eraftpb.EntryOrBuilder getEntryOrBuilder() {
if (entryBuilder_ != null) {
return entryBuilder_.getMessageOrBuilder();
} else {
return entry_ == null ?
eraftpb.Eraftpb.Entry.getDefaultInstance() : entry_;
}
}
/**
* .eraftpb.Entry entry = 1;
*/
private com.google.protobuf.SingleFieldBuilderV3<
eraftpb.Eraftpb.Entry, eraftpb.Eraftpb.Entry.Builder, eraftpb.Eraftpb.EntryOrBuilder>
getEntryFieldBuilder() {
if (entryBuilder_ == null) {
entryBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
eraftpb.Eraftpb.Entry, eraftpb.Eraftpb.Entry.Builder, eraftpb.Eraftpb.EntryOrBuilder>(
getEntry(),
getParentForChildren(),
isClean());
entry_ = null;
}
return entryBuilder_;
}
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFieldsProto3(unknownFields);
}
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:debugpb.RaftLogResponse)
}
// @@protoc_insertion_point(class_scope:debugpb.RaftLogResponse)
private static final org.tikv.kvproto.Debugpb.RaftLogResponse DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new org.tikv.kvproto.Debugpb.RaftLogResponse();
}
public static org.tikv.kvproto.Debugpb.RaftLogResponse getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
public RaftLogResponse parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new RaftLogResponse(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
public org.tikv.kvproto.Debugpb.RaftLogResponse getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface RegionInfoRequestOrBuilder extends
// @@protoc_insertion_point(interface_extends:debugpb.RegionInfoRequest)
com.google.protobuf.MessageOrBuilder {
/**
* uint64 region_id = 1;
*/
long getRegionId();
}
/**
* Protobuf type {@code debugpb.RegionInfoRequest}
*/
public static final class RegionInfoRequest extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:debugpb.RegionInfoRequest)
RegionInfoRequestOrBuilder {
private static final long serialVersionUID = 0L;
// Use RegionInfoRequest.newBuilder() to construct.
private RegionInfoRequest(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private RegionInfoRequest() {
regionId_ = 0L;
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private RegionInfoRequest(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
default: {
if (!parseUnknownFieldProto3(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
case 8: {
regionId_ = input.readUInt64();
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.tikv.kvproto.Debugpb.internal_static_debugpb_RegionInfoRequest_descriptor;
}
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.tikv.kvproto.Debugpb.internal_static_debugpb_RegionInfoRequest_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.tikv.kvproto.Debugpb.RegionInfoRequest.class, org.tikv.kvproto.Debugpb.RegionInfoRequest.Builder.class);
}
public static final int REGION_ID_FIELD_NUMBER = 1;
private long regionId_;
/**
* uint64 region_id = 1;
*/
public long getRegionId() {
return regionId_;
}
private byte memoizedIsInitialized = -1;
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (regionId_ != 0L) {
output.writeUInt64(1, regionId_);
}
unknownFields.writeTo(output);
}
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (regionId_ != 0L) {
size += com.google.protobuf.CodedOutputStream
.computeUInt64Size(1, regionId_);
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof org.tikv.kvproto.Debugpb.RegionInfoRequest)) {
return super.equals(obj);
}
org.tikv.kvproto.Debugpb.RegionInfoRequest other = (org.tikv.kvproto.Debugpb.RegionInfoRequest) obj;
boolean result = true;
result = result && (getRegionId()
== other.getRegionId());
result = result && unknownFields.equals(other.unknownFields);
return result;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
hash = (37 * hash) + REGION_ID_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
getRegionId());
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static org.tikv.kvproto.Debugpb.RegionInfoRequest parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.tikv.kvproto.Debugpb.RegionInfoRequest parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.tikv.kvproto.Debugpb.RegionInfoRequest parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.tikv.kvproto.Debugpb.RegionInfoRequest parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.tikv.kvproto.Debugpb.RegionInfoRequest parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.tikv.kvproto.Debugpb.RegionInfoRequest parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.tikv.kvproto.Debugpb.RegionInfoRequest parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static org.tikv.kvproto.Debugpb.RegionInfoRequest parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static org.tikv.kvproto.Debugpb.RegionInfoRequest parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static org.tikv.kvproto.Debugpb.RegionInfoRequest parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static org.tikv.kvproto.Debugpb.RegionInfoRequest parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static org.tikv.kvproto.Debugpb.RegionInfoRequest parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(org.tikv.kvproto.Debugpb.RegionInfoRequest prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code debugpb.RegionInfoRequest}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:debugpb.RegionInfoRequest)
org.tikv.kvproto.Debugpb.RegionInfoRequestOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.tikv.kvproto.Debugpb.internal_static_debugpb_RegionInfoRequest_descriptor;
}
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.tikv.kvproto.Debugpb.internal_static_debugpb_RegionInfoRequest_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.tikv.kvproto.Debugpb.RegionInfoRequest.class, org.tikv.kvproto.Debugpb.RegionInfoRequest.Builder.class);
}
// Construct using org.tikv.kvproto.Debugpb.RegionInfoRequest.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
public Builder clear() {
super.clear();
regionId_ = 0L;
return this;
}
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return org.tikv.kvproto.Debugpb.internal_static_debugpb_RegionInfoRequest_descriptor;
}
public org.tikv.kvproto.Debugpb.RegionInfoRequest getDefaultInstanceForType() {
return org.tikv.kvproto.Debugpb.RegionInfoRequest.getDefaultInstance();
}
public org.tikv.kvproto.Debugpb.RegionInfoRequest build() {
org.tikv.kvproto.Debugpb.RegionInfoRequest result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
public org.tikv.kvproto.Debugpb.RegionInfoRequest buildPartial() {
org.tikv.kvproto.Debugpb.RegionInfoRequest result = new org.tikv.kvproto.Debugpb.RegionInfoRequest(this);
result.regionId_ = regionId_;
onBuilt();
return result;
}
public Builder clone() {
return (Builder) super.clone();
}
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.setField(field, value);
}
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return (Builder) super.clearField(field);
}
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return (Builder) super.clearOneof(oneof);
}
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return (Builder) super.setRepeatedField(field, index, value);
}
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.addRepeatedField(field, value);
}
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof org.tikv.kvproto.Debugpb.RegionInfoRequest) {
return mergeFrom((org.tikv.kvproto.Debugpb.RegionInfoRequest)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(org.tikv.kvproto.Debugpb.RegionInfoRequest other) {
if (other == org.tikv.kvproto.Debugpb.RegionInfoRequest.getDefaultInstance()) return this;
if (other.getRegionId() != 0L) {
setRegionId(other.getRegionId());
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
public final boolean isInitialized() {
return true;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
org.tikv.kvproto.Debugpb.RegionInfoRequest parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (org.tikv.kvproto.Debugpb.RegionInfoRequest) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private long regionId_ ;
/**
* uint64 region_id = 1;
*/
public long getRegionId() {
return regionId_;
}
/**
* uint64 region_id = 1;
*/
public Builder setRegionId(long value) {
regionId_ = value;
onChanged();
return this;
}
/**
* uint64 region_id = 1;
*/
public Builder clearRegionId() {
regionId_ = 0L;
onChanged();
return this;
}
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFieldsProto3(unknownFields);
}
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:debugpb.RegionInfoRequest)
}
// @@protoc_insertion_point(class_scope:debugpb.RegionInfoRequest)
private static final org.tikv.kvproto.Debugpb.RegionInfoRequest DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new org.tikv.kvproto.Debugpb.RegionInfoRequest();
}
public static org.tikv.kvproto.Debugpb.RegionInfoRequest getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
public RegionInfoRequest parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new RegionInfoRequest(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
public org.tikv.kvproto.Debugpb.RegionInfoRequest getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface RegionInfoResponseOrBuilder extends
// @@protoc_insertion_point(interface_extends:debugpb.RegionInfoResponse)
com.google.protobuf.MessageOrBuilder {
/**
* .raft_serverpb.RaftLocalState raft_local_state = 1;
*/
boolean hasRaftLocalState();
/**
* .raft_serverpb.RaftLocalState raft_local_state = 1;
*/
org.tikv.kvproto.RaftServerpb.RaftLocalState getRaftLocalState();
/**
* .raft_serverpb.RaftLocalState raft_local_state = 1;
*/
org.tikv.kvproto.RaftServerpb.RaftLocalStateOrBuilder getRaftLocalStateOrBuilder();
/**
* .raft_serverpb.RaftApplyState raft_apply_state = 2;
*/
boolean hasRaftApplyState();
/**
* .raft_serverpb.RaftApplyState raft_apply_state = 2;
*/
org.tikv.kvproto.RaftServerpb.RaftApplyState getRaftApplyState();
/**
* .raft_serverpb.RaftApplyState raft_apply_state = 2;
*/
org.tikv.kvproto.RaftServerpb.RaftApplyStateOrBuilder getRaftApplyStateOrBuilder();
/**
* .raft_serverpb.RegionLocalState region_local_state = 3;
*/
boolean hasRegionLocalState();
/**
* .raft_serverpb.RegionLocalState region_local_state = 3;
*/
org.tikv.kvproto.RaftServerpb.RegionLocalState getRegionLocalState();
/**
* .raft_serverpb.RegionLocalState region_local_state = 3;
*/
org.tikv.kvproto.RaftServerpb.RegionLocalStateOrBuilder getRegionLocalStateOrBuilder();
}
/**
* Protobuf type {@code debugpb.RegionInfoResponse}
*/
public static final class RegionInfoResponse extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:debugpb.RegionInfoResponse)
RegionInfoResponseOrBuilder {
private static final long serialVersionUID = 0L;
// Use RegionInfoResponse.newBuilder() to construct.
private RegionInfoResponse(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private RegionInfoResponse() {
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private RegionInfoResponse(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
default: {
if (!parseUnknownFieldProto3(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
case 10: {
org.tikv.kvproto.RaftServerpb.RaftLocalState.Builder subBuilder = null;
if (raftLocalState_ != null) {
subBuilder = raftLocalState_.toBuilder();
}
raftLocalState_ = input.readMessage(org.tikv.kvproto.RaftServerpb.RaftLocalState.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(raftLocalState_);
raftLocalState_ = subBuilder.buildPartial();
}
break;
}
case 18: {
org.tikv.kvproto.RaftServerpb.RaftApplyState.Builder subBuilder = null;
if (raftApplyState_ != null) {
subBuilder = raftApplyState_.toBuilder();
}
raftApplyState_ = input.readMessage(org.tikv.kvproto.RaftServerpb.RaftApplyState.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(raftApplyState_);
raftApplyState_ = subBuilder.buildPartial();
}
break;
}
case 26: {
org.tikv.kvproto.RaftServerpb.RegionLocalState.Builder subBuilder = null;
if (regionLocalState_ != null) {
subBuilder = regionLocalState_.toBuilder();
}
regionLocalState_ = input.readMessage(org.tikv.kvproto.RaftServerpb.RegionLocalState.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(regionLocalState_);
regionLocalState_ = subBuilder.buildPartial();
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.tikv.kvproto.Debugpb.internal_static_debugpb_RegionInfoResponse_descriptor;
}
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.tikv.kvproto.Debugpb.internal_static_debugpb_RegionInfoResponse_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.tikv.kvproto.Debugpb.RegionInfoResponse.class, org.tikv.kvproto.Debugpb.RegionInfoResponse.Builder.class);
}
public static final int RAFT_LOCAL_STATE_FIELD_NUMBER = 1;
private org.tikv.kvproto.RaftServerpb.RaftLocalState raftLocalState_;
/**
* .raft_serverpb.RaftLocalState raft_local_state = 1;
*/
public boolean hasRaftLocalState() {
return raftLocalState_ != null;
}
/**
* .raft_serverpb.RaftLocalState raft_local_state = 1;
*/
public org.tikv.kvproto.RaftServerpb.RaftLocalState getRaftLocalState() {
return raftLocalState_ == null ? org.tikv.kvproto.RaftServerpb.RaftLocalState.getDefaultInstance() : raftLocalState_;
}
/**
* .raft_serverpb.RaftLocalState raft_local_state = 1;
*/
public org.tikv.kvproto.RaftServerpb.RaftLocalStateOrBuilder getRaftLocalStateOrBuilder() {
return getRaftLocalState();
}
public static final int RAFT_APPLY_STATE_FIELD_NUMBER = 2;
private org.tikv.kvproto.RaftServerpb.RaftApplyState raftApplyState_;
/**
* .raft_serverpb.RaftApplyState raft_apply_state = 2;
*/
public boolean hasRaftApplyState() {
return raftApplyState_ != null;
}
/**
* .raft_serverpb.RaftApplyState raft_apply_state = 2;
*/
public org.tikv.kvproto.RaftServerpb.RaftApplyState getRaftApplyState() {
return raftApplyState_ == null ? org.tikv.kvproto.RaftServerpb.RaftApplyState.getDefaultInstance() : raftApplyState_;
}
/**
* .raft_serverpb.RaftApplyState raft_apply_state = 2;
*/
public org.tikv.kvproto.RaftServerpb.RaftApplyStateOrBuilder getRaftApplyStateOrBuilder() {
return getRaftApplyState();
}
public static final int REGION_LOCAL_STATE_FIELD_NUMBER = 3;
private org.tikv.kvproto.RaftServerpb.RegionLocalState regionLocalState_;
/**
* .raft_serverpb.RegionLocalState region_local_state = 3;
*/
public boolean hasRegionLocalState() {
return regionLocalState_ != null;
}
/**
* .raft_serverpb.RegionLocalState region_local_state = 3;
*/
public org.tikv.kvproto.RaftServerpb.RegionLocalState getRegionLocalState() {
return regionLocalState_ == null ? org.tikv.kvproto.RaftServerpb.RegionLocalState.getDefaultInstance() : regionLocalState_;
}
/**
* .raft_serverpb.RegionLocalState region_local_state = 3;
*/
public org.tikv.kvproto.RaftServerpb.RegionLocalStateOrBuilder getRegionLocalStateOrBuilder() {
return getRegionLocalState();
}
private byte memoizedIsInitialized = -1;
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (raftLocalState_ != null) {
output.writeMessage(1, getRaftLocalState());
}
if (raftApplyState_ != null) {
output.writeMessage(2, getRaftApplyState());
}
if (regionLocalState_ != null) {
output.writeMessage(3, getRegionLocalState());
}
unknownFields.writeTo(output);
}
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (raftLocalState_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(1, getRaftLocalState());
}
if (raftApplyState_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(2, getRaftApplyState());
}
if (regionLocalState_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(3, getRegionLocalState());
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof org.tikv.kvproto.Debugpb.RegionInfoResponse)) {
return super.equals(obj);
}
org.tikv.kvproto.Debugpb.RegionInfoResponse other = (org.tikv.kvproto.Debugpb.RegionInfoResponse) obj;
boolean result = true;
result = result && (hasRaftLocalState() == other.hasRaftLocalState());
if (hasRaftLocalState()) {
result = result && getRaftLocalState()
.equals(other.getRaftLocalState());
}
result = result && (hasRaftApplyState() == other.hasRaftApplyState());
if (hasRaftApplyState()) {
result = result && getRaftApplyState()
.equals(other.getRaftApplyState());
}
result = result && (hasRegionLocalState() == other.hasRegionLocalState());
if (hasRegionLocalState()) {
result = result && getRegionLocalState()
.equals(other.getRegionLocalState());
}
result = result && unknownFields.equals(other.unknownFields);
return result;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (hasRaftLocalState()) {
hash = (37 * hash) + RAFT_LOCAL_STATE_FIELD_NUMBER;
hash = (53 * hash) + getRaftLocalState().hashCode();
}
if (hasRaftApplyState()) {
hash = (37 * hash) + RAFT_APPLY_STATE_FIELD_NUMBER;
hash = (53 * hash) + getRaftApplyState().hashCode();
}
if (hasRegionLocalState()) {
hash = (37 * hash) + REGION_LOCAL_STATE_FIELD_NUMBER;
hash = (53 * hash) + getRegionLocalState().hashCode();
}
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static org.tikv.kvproto.Debugpb.RegionInfoResponse parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.tikv.kvproto.Debugpb.RegionInfoResponse parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.tikv.kvproto.Debugpb.RegionInfoResponse parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.tikv.kvproto.Debugpb.RegionInfoResponse parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.tikv.kvproto.Debugpb.RegionInfoResponse parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.tikv.kvproto.Debugpb.RegionInfoResponse parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.tikv.kvproto.Debugpb.RegionInfoResponse parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static org.tikv.kvproto.Debugpb.RegionInfoResponse parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static org.tikv.kvproto.Debugpb.RegionInfoResponse parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static org.tikv.kvproto.Debugpb.RegionInfoResponse parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static org.tikv.kvproto.Debugpb.RegionInfoResponse parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static org.tikv.kvproto.Debugpb.RegionInfoResponse parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(org.tikv.kvproto.Debugpb.RegionInfoResponse prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code debugpb.RegionInfoResponse}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:debugpb.RegionInfoResponse)
org.tikv.kvproto.Debugpb.RegionInfoResponseOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.tikv.kvproto.Debugpb.internal_static_debugpb_RegionInfoResponse_descriptor;
}
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.tikv.kvproto.Debugpb.internal_static_debugpb_RegionInfoResponse_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.tikv.kvproto.Debugpb.RegionInfoResponse.class, org.tikv.kvproto.Debugpb.RegionInfoResponse.Builder.class);
}
// Construct using org.tikv.kvproto.Debugpb.RegionInfoResponse.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
public Builder clear() {
super.clear();
if (raftLocalStateBuilder_ == null) {
raftLocalState_ = null;
} else {
raftLocalState_ = null;
raftLocalStateBuilder_ = null;
}
if (raftApplyStateBuilder_ == null) {
raftApplyState_ = null;
} else {
raftApplyState_ = null;
raftApplyStateBuilder_ = null;
}
if (regionLocalStateBuilder_ == null) {
regionLocalState_ = null;
} else {
regionLocalState_ = null;
regionLocalStateBuilder_ = null;
}
return this;
}
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return org.tikv.kvproto.Debugpb.internal_static_debugpb_RegionInfoResponse_descriptor;
}
public org.tikv.kvproto.Debugpb.RegionInfoResponse getDefaultInstanceForType() {
return org.tikv.kvproto.Debugpb.RegionInfoResponse.getDefaultInstance();
}
public org.tikv.kvproto.Debugpb.RegionInfoResponse build() {
org.tikv.kvproto.Debugpb.RegionInfoResponse result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
public org.tikv.kvproto.Debugpb.RegionInfoResponse buildPartial() {
org.tikv.kvproto.Debugpb.RegionInfoResponse result = new org.tikv.kvproto.Debugpb.RegionInfoResponse(this);
if (raftLocalStateBuilder_ == null) {
result.raftLocalState_ = raftLocalState_;
} else {
result.raftLocalState_ = raftLocalStateBuilder_.build();
}
if (raftApplyStateBuilder_ == null) {
result.raftApplyState_ = raftApplyState_;
} else {
result.raftApplyState_ = raftApplyStateBuilder_.build();
}
if (regionLocalStateBuilder_ == null) {
result.regionLocalState_ = regionLocalState_;
} else {
result.regionLocalState_ = regionLocalStateBuilder_.build();
}
onBuilt();
return result;
}
public Builder clone() {
return (Builder) super.clone();
}
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.setField(field, value);
}
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return (Builder) super.clearField(field);
}
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return (Builder) super.clearOneof(oneof);
}
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return (Builder) super.setRepeatedField(field, index, value);
}
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.addRepeatedField(field, value);
}
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof org.tikv.kvproto.Debugpb.RegionInfoResponse) {
return mergeFrom((org.tikv.kvproto.Debugpb.RegionInfoResponse)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(org.tikv.kvproto.Debugpb.RegionInfoResponse other) {
if (other == org.tikv.kvproto.Debugpb.RegionInfoResponse.getDefaultInstance()) return this;
if (other.hasRaftLocalState()) {
mergeRaftLocalState(other.getRaftLocalState());
}
if (other.hasRaftApplyState()) {
mergeRaftApplyState(other.getRaftApplyState());
}
if (other.hasRegionLocalState()) {
mergeRegionLocalState(other.getRegionLocalState());
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
public final boolean isInitialized() {
return true;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
org.tikv.kvproto.Debugpb.RegionInfoResponse parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (org.tikv.kvproto.Debugpb.RegionInfoResponse) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private org.tikv.kvproto.RaftServerpb.RaftLocalState raftLocalState_ = null;
private com.google.protobuf.SingleFieldBuilderV3<
org.tikv.kvproto.RaftServerpb.RaftLocalState, org.tikv.kvproto.RaftServerpb.RaftLocalState.Builder, org.tikv.kvproto.RaftServerpb.RaftLocalStateOrBuilder> raftLocalStateBuilder_;
/**
* .raft_serverpb.RaftLocalState raft_local_state = 1;
*/
public boolean hasRaftLocalState() {
return raftLocalStateBuilder_ != null || raftLocalState_ != null;
}
/**
* .raft_serverpb.RaftLocalState raft_local_state = 1;
*/
public org.tikv.kvproto.RaftServerpb.RaftLocalState getRaftLocalState() {
if (raftLocalStateBuilder_ == null) {
return raftLocalState_ == null ? org.tikv.kvproto.RaftServerpb.RaftLocalState.getDefaultInstance() : raftLocalState_;
} else {
return raftLocalStateBuilder_.getMessage();
}
}
/**
* .raft_serverpb.RaftLocalState raft_local_state = 1;
*/
public Builder setRaftLocalState(org.tikv.kvproto.RaftServerpb.RaftLocalState value) {
if (raftLocalStateBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
raftLocalState_ = value;
onChanged();
} else {
raftLocalStateBuilder_.setMessage(value);
}
return this;
}
/**
* .raft_serverpb.RaftLocalState raft_local_state = 1;
*/
public Builder setRaftLocalState(
org.tikv.kvproto.RaftServerpb.RaftLocalState.Builder builderForValue) {
if (raftLocalStateBuilder_ == null) {
raftLocalState_ = builderForValue.build();
onChanged();
} else {
raftLocalStateBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
* .raft_serverpb.RaftLocalState raft_local_state = 1;
*/
public Builder mergeRaftLocalState(org.tikv.kvproto.RaftServerpb.RaftLocalState value) {
if (raftLocalStateBuilder_ == null) {
if (raftLocalState_ != null) {
raftLocalState_ =
org.tikv.kvproto.RaftServerpb.RaftLocalState.newBuilder(raftLocalState_).mergeFrom(value).buildPartial();
} else {
raftLocalState_ = value;
}
onChanged();
} else {
raftLocalStateBuilder_.mergeFrom(value);
}
return this;
}
/**
* .raft_serverpb.RaftLocalState raft_local_state = 1;
*/
public Builder clearRaftLocalState() {
if (raftLocalStateBuilder_ == null) {
raftLocalState_ = null;
onChanged();
} else {
raftLocalState_ = null;
raftLocalStateBuilder_ = null;
}
return this;
}
/**
* .raft_serverpb.RaftLocalState raft_local_state = 1;
*/
public org.tikv.kvproto.RaftServerpb.RaftLocalState.Builder getRaftLocalStateBuilder() {
onChanged();
return getRaftLocalStateFieldBuilder().getBuilder();
}
/**
* .raft_serverpb.RaftLocalState raft_local_state = 1;
*/
public org.tikv.kvproto.RaftServerpb.RaftLocalStateOrBuilder getRaftLocalStateOrBuilder() {
if (raftLocalStateBuilder_ != null) {
return raftLocalStateBuilder_.getMessageOrBuilder();
} else {
return raftLocalState_ == null ?
org.tikv.kvproto.RaftServerpb.RaftLocalState.getDefaultInstance() : raftLocalState_;
}
}
/**
* .raft_serverpb.RaftLocalState raft_local_state = 1;
*/
private com.google.protobuf.SingleFieldBuilderV3<
org.tikv.kvproto.RaftServerpb.RaftLocalState, org.tikv.kvproto.RaftServerpb.RaftLocalState.Builder, org.tikv.kvproto.RaftServerpb.RaftLocalStateOrBuilder>
getRaftLocalStateFieldBuilder() {
if (raftLocalStateBuilder_ == null) {
raftLocalStateBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
org.tikv.kvproto.RaftServerpb.RaftLocalState, org.tikv.kvproto.RaftServerpb.RaftLocalState.Builder, org.tikv.kvproto.RaftServerpb.RaftLocalStateOrBuilder>(
getRaftLocalState(),
getParentForChildren(),
isClean());
raftLocalState_ = null;
}
return raftLocalStateBuilder_;
}
private org.tikv.kvproto.RaftServerpb.RaftApplyState raftApplyState_ = null;
private com.google.protobuf.SingleFieldBuilderV3<
org.tikv.kvproto.RaftServerpb.RaftApplyState, org.tikv.kvproto.RaftServerpb.RaftApplyState.Builder, org.tikv.kvproto.RaftServerpb.RaftApplyStateOrBuilder> raftApplyStateBuilder_;
/**
* .raft_serverpb.RaftApplyState raft_apply_state = 2;
*/
public boolean hasRaftApplyState() {
return raftApplyStateBuilder_ != null || raftApplyState_ != null;
}
/**
* .raft_serverpb.RaftApplyState raft_apply_state = 2;
*/
public org.tikv.kvproto.RaftServerpb.RaftApplyState getRaftApplyState() {
if (raftApplyStateBuilder_ == null) {
return raftApplyState_ == null ? org.tikv.kvproto.RaftServerpb.RaftApplyState.getDefaultInstance() : raftApplyState_;
} else {
return raftApplyStateBuilder_.getMessage();
}
}
/**
* .raft_serverpb.RaftApplyState raft_apply_state = 2;
*/
public Builder setRaftApplyState(org.tikv.kvproto.RaftServerpb.RaftApplyState value) {
if (raftApplyStateBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
raftApplyState_ = value;
onChanged();
} else {
raftApplyStateBuilder_.setMessage(value);
}
return this;
}
/**
* .raft_serverpb.RaftApplyState raft_apply_state = 2;
*/
public Builder setRaftApplyState(
org.tikv.kvproto.RaftServerpb.RaftApplyState.Builder builderForValue) {
if (raftApplyStateBuilder_ == null) {
raftApplyState_ = builderForValue.build();
onChanged();
} else {
raftApplyStateBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
* .raft_serverpb.RaftApplyState raft_apply_state = 2;
*/
public Builder mergeRaftApplyState(org.tikv.kvproto.RaftServerpb.RaftApplyState value) {
if (raftApplyStateBuilder_ == null) {
if (raftApplyState_ != null) {
raftApplyState_ =
org.tikv.kvproto.RaftServerpb.RaftApplyState.newBuilder(raftApplyState_).mergeFrom(value).buildPartial();
} else {
raftApplyState_ = value;
}
onChanged();
} else {
raftApplyStateBuilder_.mergeFrom(value);
}
return this;
}
/**
* .raft_serverpb.RaftApplyState raft_apply_state = 2;
*/
public Builder clearRaftApplyState() {
if (raftApplyStateBuilder_ == null) {
raftApplyState_ = null;
onChanged();
} else {
raftApplyState_ = null;
raftApplyStateBuilder_ = null;
}
return this;
}
/**
* .raft_serverpb.RaftApplyState raft_apply_state = 2;
*/
public org.tikv.kvproto.RaftServerpb.RaftApplyState.Builder getRaftApplyStateBuilder() {
onChanged();
return getRaftApplyStateFieldBuilder().getBuilder();
}
/**
* .raft_serverpb.RaftApplyState raft_apply_state = 2;
*/
public org.tikv.kvproto.RaftServerpb.RaftApplyStateOrBuilder getRaftApplyStateOrBuilder() {
if (raftApplyStateBuilder_ != null) {
return raftApplyStateBuilder_.getMessageOrBuilder();
} else {
return raftApplyState_ == null ?
org.tikv.kvproto.RaftServerpb.RaftApplyState.getDefaultInstance() : raftApplyState_;
}
}
/**
* .raft_serverpb.RaftApplyState raft_apply_state = 2;
*/
private com.google.protobuf.SingleFieldBuilderV3<
org.tikv.kvproto.RaftServerpb.RaftApplyState, org.tikv.kvproto.RaftServerpb.RaftApplyState.Builder, org.tikv.kvproto.RaftServerpb.RaftApplyStateOrBuilder>
getRaftApplyStateFieldBuilder() {
if (raftApplyStateBuilder_ == null) {
raftApplyStateBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
org.tikv.kvproto.RaftServerpb.RaftApplyState, org.tikv.kvproto.RaftServerpb.RaftApplyState.Builder, org.tikv.kvproto.RaftServerpb.RaftApplyStateOrBuilder>(
getRaftApplyState(),
getParentForChildren(),
isClean());
raftApplyState_ = null;
}
return raftApplyStateBuilder_;
}
private org.tikv.kvproto.RaftServerpb.RegionLocalState regionLocalState_ = null;
private com.google.protobuf.SingleFieldBuilderV3<
org.tikv.kvproto.RaftServerpb.RegionLocalState, org.tikv.kvproto.RaftServerpb.RegionLocalState.Builder, org.tikv.kvproto.RaftServerpb.RegionLocalStateOrBuilder> regionLocalStateBuilder_;
/**
* .raft_serverpb.RegionLocalState region_local_state = 3;
*/
public boolean hasRegionLocalState() {
return regionLocalStateBuilder_ != null || regionLocalState_ != null;
}
/**
* .raft_serverpb.RegionLocalState region_local_state = 3;
*/
public org.tikv.kvproto.RaftServerpb.RegionLocalState getRegionLocalState() {
if (regionLocalStateBuilder_ == null) {
return regionLocalState_ == null ? org.tikv.kvproto.RaftServerpb.RegionLocalState.getDefaultInstance() : regionLocalState_;
} else {
return regionLocalStateBuilder_.getMessage();
}
}
/**
* .raft_serverpb.RegionLocalState region_local_state = 3;
*/
public Builder setRegionLocalState(org.tikv.kvproto.RaftServerpb.RegionLocalState value) {
if (regionLocalStateBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
regionLocalState_ = value;
onChanged();
} else {
regionLocalStateBuilder_.setMessage(value);
}
return this;
}
/**
* .raft_serverpb.RegionLocalState region_local_state = 3;
*/
public Builder setRegionLocalState(
org.tikv.kvproto.RaftServerpb.RegionLocalState.Builder builderForValue) {
if (regionLocalStateBuilder_ == null) {
regionLocalState_ = builderForValue.build();
onChanged();
} else {
regionLocalStateBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
* .raft_serverpb.RegionLocalState region_local_state = 3;
*/
public Builder mergeRegionLocalState(org.tikv.kvproto.RaftServerpb.RegionLocalState value) {
if (regionLocalStateBuilder_ == null) {
if (regionLocalState_ != null) {
regionLocalState_ =
org.tikv.kvproto.RaftServerpb.RegionLocalState.newBuilder(regionLocalState_).mergeFrom(value).buildPartial();
} else {
regionLocalState_ = value;
}
onChanged();
} else {
regionLocalStateBuilder_.mergeFrom(value);
}
return this;
}
/**
* .raft_serverpb.RegionLocalState region_local_state = 3;
*/
public Builder clearRegionLocalState() {
if (regionLocalStateBuilder_ == null) {
regionLocalState_ = null;
onChanged();
} else {
regionLocalState_ = null;
regionLocalStateBuilder_ = null;
}
return this;
}
/**
* .raft_serverpb.RegionLocalState region_local_state = 3;
*/
public org.tikv.kvproto.RaftServerpb.RegionLocalState.Builder getRegionLocalStateBuilder() {
onChanged();
return getRegionLocalStateFieldBuilder().getBuilder();
}
/**
* .raft_serverpb.RegionLocalState region_local_state = 3;
*/
public org.tikv.kvproto.RaftServerpb.RegionLocalStateOrBuilder getRegionLocalStateOrBuilder() {
if (regionLocalStateBuilder_ != null) {
return regionLocalStateBuilder_.getMessageOrBuilder();
} else {
return regionLocalState_ == null ?
org.tikv.kvproto.RaftServerpb.RegionLocalState.getDefaultInstance() : regionLocalState_;
}
}
/**
* .raft_serverpb.RegionLocalState region_local_state = 3;
*/
private com.google.protobuf.SingleFieldBuilderV3<
org.tikv.kvproto.RaftServerpb.RegionLocalState, org.tikv.kvproto.RaftServerpb.RegionLocalState.Builder, org.tikv.kvproto.RaftServerpb.RegionLocalStateOrBuilder>
getRegionLocalStateFieldBuilder() {
if (regionLocalStateBuilder_ == null) {
regionLocalStateBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
org.tikv.kvproto.RaftServerpb.RegionLocalState, org.tikv.kvproto.RaftServerpb.RegionLocalState.Builder, org.tikv.kvproto.RaftServerpb.RegionLocalStateOrBuilder>(
getRegionLocalState(),
getParentForChildren(),
isClean());
regionLocalState_ = null;
}
return regionLocalStateBuilder_;
}
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFieldsProto3(unknownFields);
}
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:debugpb.RegionInfoResponse)
}
// @@protoc_insertion_point(class_scope:debugpb.RegionInfoResponse)
private static final org.tikv.kvproto.Debugpb.RegionInfoResponse DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new org.tikv.kvproto.Debugpb.RegionInfoResponse();
}
public static org.tikv.kvproto.Debugpb.RegionInfoResponse getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
public RegionInfoResponse parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new RegionInfoResponse(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
public org.tikv.kvproto.Debugpb.RegionInfoResponse getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface RegionSizeRequestOrBuilder extends
// @@protoc_insertion_point(interface_extends:debugpb.RegionSizeRequest)
com.google.protobuf.MessageOrBuilder {
/**
* uint64 region_id = 1;
*/
long getRegionId();
/**
* repeated string cfs = 2;
*/
java.util.List
getCfsList();
/**
* repeated string cfs = 2;
*/
int getCfsCount();
/**
* repeated string cfs = 2;
*/
java.lang.String getCfs(int index);
/**
* repeated string cfs = 2;
*/
com.google.protobuf.ByteString
getCfsBytes(int index);
}
/**
* Protobuf type {@code debugpb.RegionSizeRequest}
*/
public static final class RegionSizeRequest extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:debugpb.RegionSizeRequest)
RegionSizeRequestOrBuilder {
private static final long serialVersionUID = 0L;
// Use RegionSizeRequest.newBuilder() to construct.
private RegionSizeRequest(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private RegionSizeRequest() {
regionId_ = 0L;
cfs_ = com.google.protobuf.LazyStringArrayList.EMPTY;
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private RegionSizeRequest(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
default: {
if (!parseUnknownFieldProto3(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
case 8: {
regionId_ = input.readUInt64();
break;
}
case 18: {
java.lang.String s = input.readStringRequireUtf8();
if (!((mutable_bitField0_ & 0x00000002) == 0x00000002)) {
cfs_ = new com.google.protobuf.LazyStringArrayList();
mutable_bitField0_ |= 0x00000002;
}
cfs_.add(s);
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
if (((mutable_bitField0_ & 0x00000002) == 0x00000002)) {
cfs_ = cfs_.getUnmodifiableView();
}
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.tikv.kvproto.Debugpb.internal_static_debugpb_RegionSizeRequest_descriptor;
}
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.tikv.kvproto.Debugpb.internal_static_debugpb_RegionSizeRequest_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.tikv.kvproto.Debugpb.RegionSizeRequest.class, org.tikv.kvproto.Debugpb.RegionSizeRequest.Builder.class);
}
private int bitField0_;
public static final int REGION_ID_FIELD_NUMBER = 1;
private long regionId_;
/**
* uint64 region_id = 1;
*/
public long getRegionId() {
return regionId_;
}
public static final int CFS_FIELD_NUMBER = 2;
private com.google.protobuf.LazyStringList cfs_;
/**
* repeated string cfs = 2;
*/
public com.google.protobuf.ProtocolStringList
getCfsList() {
return cfs_;
}
/**
* repeated string cfs = 2;
*/
public int getCfsCount() {
return cfs_.size();
}
/**
* repeated string cfs = 2;
*/
public java.lang.String getCfs(int index) {
return cfs_.get(index);
}
/**
* repeated string cfs = 2;
*/
public com.google.protobuf.ByteString
getCfsBytes(int index) {
return cfs_.getByteString(index);
}
private byte memoizedIsInitialized = -1;
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (regionId_ != 0L) {
output.writeUInt64(1, regionId_);
}
for (int i = 0; i < cfs_.size(); i++) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 2, cfs_.getRaw(i));
}
unknownFields.writeTo(output);
}
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (regionId_ != 0L) {
size += com.google.protobuf.CodedOutputStream
.computeUInt64Size(1, regionId_);
}
{
int dataSize = 0;
for (int i = 0; i < cfs_.size(); i++) {
dataSize += computeStringSizeNoTag(cfs_.getRaw(i));
}
size += dataSize;
size += 1 * getCfsList().size();
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof org.tikv.kvproto.Debugpb.RegionSizeRequest)) {
return super.equals(obj);
}
org.tikv.kvproto.Debugpb.RegionSizeRequest other = (org.tikv.kvproto.Debugpb.RegionSizeRequest) obj;
boolean result = true;
result = result && (getRegionId()
== other.getRegionId());
result = result && getCfsList()
.equals(other.getCfsList());
result = result && unknownFields.equals(other.unknownFields);
return result;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
hash = (37 * hash) + REGION_ID_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
getRegionId());
if (getCfsCount() > 0) {
hash = (37 * hash) + CFS_FIELD_NUMBER;
hash = (53 * hash) + getCfsList().hashCode();
}
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static org.tikv.kvproto.Debugpb.RegionSizeRequest parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.tikv.kvproto.Debugpb.RegionSizeRequest parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.tikv.kvproto.Debugpb.RegionSizeRequest parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.tikv.kvproto.Debugpb.RegionSizeRequest parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.tikv.kvproto.Debugpb.RegionSizeRequest parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.tikv.kvproto.Debugpb.RegionSizeRequest parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.tikv.kvproto.Debugpb.RegionSizeRequest parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static org.tikv.kvproto.Debugpb.RegionSizeRequest parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static org.tikv.kvproto.Debugpb.RegionSizeRequest parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static org.tikv.kvproto.Debugpb.RegionSizeRequest parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static org.tikv.kvproto.Debugpb.RegionSizeRequest parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static org.tikv.kvproto.Debugpb.RegionSizeRequest parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(org.tikv.kvproto.Debugpb.RegionSizeRequest prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code debugpb.RegionSizeRequest}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:debugpb.RegionSizeRequest)
org.tikv.kvproto.Debugpb.RegionSizeRequestOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.tikv.kvproto.Debugpb.internal_static_debugpb_RegionSizeRequest_descriptor;
}
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.tikv.kvproto.Debugpb.internal_static_debugpb_RegionSizeRequest_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.tikv.kvproto.Debugpb.RegionSizeRequest.class, org.tikv.kvproto.Debugpb.RegionSizeRequest.Builder.class);
}
// Construct using org.tikv.kvproto.Debugpb.RegionSizeRequest.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
public Builder clear() {
super.clear();
regionId_ = 0L;
cfs_ = com.google.protobuf.LazyStringArrayList.EMPTY;
bitField0_ = (bitField0_ & ~0x00000002);
return this;
}
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return org.tikv.kvproto.Debugpb.internal_static_debugpb_RegionSizeRequest_descriptor;
}
public org.tikv.kvproto.Debugpb.RegionSizeRequest getDefaultInstanceForType() {
return org.tikv.kvproto.Debugpb.RegionSizeRequest.getDefaultInstance();
}
public org.tikv.kvproto.Debugpb.RegionSizeRequest build() {
org.tikv.kvproto.Debugpb.RegionSizeRequest result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
public org.tikv.kvproto.Debugpb.RegionSizeRequest buildPartial() {
org.tikv.kvproto.Debugpb.RegionSizeRequest result = new org.tikv.kvproto.Debugpb.RegionSizeRequest(this);
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
result.regionId_ = regionId_;
if (((bitField0_ & 0x00000002) == 0x00000002)) {
cfs_ = cfs_.getUnmodifiableView();
bitField0_ = (bitField0_ & ~0x00000002);
}
result.cfs_ = cfs_;
result.bitField0_ = to_bitField0_;
onBuilt();
return result;
}
public Builder clone() {
return (Builder) super.clone();
}
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.setField(field, value);
}
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return (Builder) super.clearField(field);
}
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return (Builder) super.clearOneof(oneof);
}
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return (Builder) super.setRepeatedField(field, index, value);
}
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.addRepeatedField(field, value);
}
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof org.tikv.kvproto.Debugpb.RegionSizeRequest) {
return mergeFrom((org.tikv.kvproto.Debugpb.RegionSizeRequest)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(org.tikv.kvproto.Debugpb.RegionSizeRequest other) {
if (other == org.tikv.kvproto.Debugpb.RegionSizeRequest.getDefaultInstance()) return this;
if (other.getRegionId() != 0L) {
setRegionId(other.getRegionId());
}
if (!other.cfs_.isEmpty()) {
if (cfs_.isEmpty()) {
cfs_ = other.cfs_;
bitField0_ = (bitField0_ & ~0x00000002);
} else {
ensureCfsIsMutable();
cfs_.addAll(other.cfs_);
}
onChanged();
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
public final boolean isInitialized() {
return true;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
org.tikv.kvproto.Debugpb.RegionSizeRequest parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (org.tikv.kvproto.Debugpb.RegionSizeRequest) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
private long regionId_ ;
/**
* uint64 region_id = 1;
*/
public long getRegionId() {
return regionId_;
}
/**
* uint64 region_id = 1;
*/
public Builder setRegionId(long value) {
regionId_ = value;
onChanged();
return this;
}
/**
* uint64 region_id = 1;
*/
public Builder clearRegionId() {
regionId_ = 0L;
onChanged();
return this;
}
private com.google.protobuf.LazyStringList cfs_ = com.google.protobuf.LazyStringArrayList.EMPTY;
private void ensureCfsIsMutable() {
if (!((bitField0_ & 0x00000002) == 0x00000002)) {
cfs_ = new com.google.protobuf.LazyStringArrayList(cfs_);
bitField0_ |= 0x00000002;
}
}
/**
* repeated string cfs = 2;
*/
public com.google.protobuf.ProtocolStringList
getCfsList() {
return cfs_.getUnmodifiableView();
}
/**
* repeated string cfs = 2;
*/
public int getCfsCount() {
return cfs_.size();
}
/**
* repeated string cfs = 2;
*/
public java.lang.String getCfs(int index) {
return cfs_.get(index);
}
/**
* repeated string cfs = 2;
*/
public com.google.protobuf.ByteString
getCfsBytes(int index) {
return cfs_.getByteString(index);
}
/**
* repeated string cfs = 2;
*/
public Builder setCfs(
int index, java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
ensureCfsIsMutable();
cfs_.set(index, value);
onChanged();
return this;
}
/**
* repeated string cfs = 2;
*/
public Builder addCfs(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
ensureCfsIsMutable();
cfs_.add(value);
onChanged();
return this;
}
/**
* repeated string cfs = 2;
*/
public Builder addAllCfs(
java.lang.Iterable values) {
ensureCfsIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, cfs_);
onChanged();
return this;
}
/**
* repeated string cfs = 2;
*/
public Builder clearCfs() {
cfs_ = com.google.protobuf.LazyStringArrayList.EMPTY;
bitField0_ = (bitField0_ & ~0x00000002);
onChanged();
return this;
}
/**
* repeated string cfs = 2;
*/
public Builder addCfsBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
ensureCfsIsMutable();
cfs_.add(value);
onChanged();
return this;
}
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFieldsProto3(unknownFields);
}
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:debugpb.RegionSizeRequest)
}
// @@protoc_insertion_point(class_scope:debugpb.RegionSizeRequest)
private static final org.tikv.kvproto.Debugpb.RegionSizeRequest DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new org.tikv.kvproto.Debugpb.RegionSizeRequest();
}
public static org.tikv.kvproto.Debugpb.RegionSizeRequest getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
public RegionSizeRequest parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new RegionSizeRequest(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
public org.tikv.kvproto.Debugpb.RegionSizeRequest getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface RegionSizeResponseOrBuilder extends
// @@protoc_insertion_point(interface_extends:debugpb.RegionSizeResponse)
com.google.protobuf.MessageOrBuilder {
/**
* repeated .debugpb.RegionSizeResponse.Entry entries = 1;
*/
java.util.List
getEntriesList();
/**
* repeated .debugpb.RegionSizeResponse.Entry entries = 1;
*/
org.tikv.kvproto.Debugpb.RegionSizeResponse.Entry getEntries(int index);
/**
* repeated .debugpb.RegionSizeResponse.Entry entries = 1;
*/
int getEntriesCount();
/**
* repeated .debugpb.RegionSizeResponse.Entry entries = 1;
*/
java.util.List extends org.tikv.kvproto.Debugpb.RegionSizeResponse.EntryOrBuilder>
getEntriesOrBuilderList();
/**
* repeated .debugpb.RegionSizeResponse.Entry entries = 1;
*/
org.tikv.kvproto.Debugpb.RegionSizeResponse.EntryOrBuilder getEntriesOrBuilder(
int index);
}
/**
* Protobuf type {@code debugpb.RegionSizeResponse}
*/
public static final class RegionSizeResponse extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:debugpb.RegionSizeResponse)
RegionSizeResponseOrBuilder {
private static final long serialVersionUID = 0L;
// Use RegionSizeResponse.newBuilder() to construct.
private RegionSizeResponse(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private RegionSizeResponse() {
entries_ = java.util.Collections.emptyList();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private RegionSizeResponse(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
default: {
if (!parseUnknownFieldProto3(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
case 10: {
if (!((mutable_bitField0_ & 0x00000001) == 0x00000001)) {
entries_ = new java.util.ArrayList();
mutable_bitField0_ |= 0x00000001;
}
entries_.add(
input.readMessage(org.tikv.kvproto.Debugpb.RegionSizeResponse.Entry.parser(), extensionRegistry));
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
if (((mutable_bitField0_ & 0x00000001) == 0x00000001)) {
entries_ = java.util.Collections.unmodifiableList(entries_);
}
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.tikv.kvproto.Debugpb.internal_static_debugpb_RegionSizeResponse_descriptor;
}
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.tikv.kvproto.Debugpb.internal_static_debugpb_RegionSizeResponse_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.tikv.kvproto.Debugpb.RegionSizeResponse.class, org.tikv.kvproto.Debugpb.RegionSizeResponse.Builder.class);
}
public interface EntryOrBuilder extends
// @@protoc_insertion_point(interface_extends:debugpb.RegionSizeResponse.Entry)
com.google.protobuf.MessageOrBuilder {
/**
* string cf = 1;
*/
java.lang.String getCf();
/**
* string cf = 1;
*/
com.google.protobuf.ByteString
getCfBytes();
/**
* uint64 size = 2;
*/
long getSize();
}
/**
* Protobuf type {@code debugpb.RegionSizeResponse.Entry}
*/
public static final class Entry extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:debugpb.RegionSizeResponse.Entry)
EntryOrBuilder {
private static final long serialVersionUID = 0L;
// Use Entry.newBuilder() to construct.
private Entry(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private Entry() {
cf_ = "";
size_ = 0L;
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private Entry(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
default: {
if (!parseUnknownFieldProto3(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
case 10: {
java.lang.String s = input.readStringRequireUtf8();
cf_ = s;
break;
}
case 16: {
size_ = input.readUInt64();
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.tikv.kvproto.Debugpb.internal_static_debugpb_RegionSizeResponse_Entry_descriptor;
}
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.tikv.kvproto.Debugpb.internal_static_debugpb_RegionSizeResponse_Entry_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.tikv.kvproto.Debugpb.RegionSizeResponse.Entry.class, org.tikv.kvproto.Debugpb.RegionSizeResponse.Entry.Builder.class);
}
public static final int CF_FIELD_NUMBER = 1;
private volatile java.lang.Object cf_;
/**
* string cf = 1;
*/
public java.lang.String getCf() {
java.lang.Object ref = cf_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
cf_ = s;
return s;
}
}
/**
* string cf = 1;
*/
public com.google.protobuf.ByteString
getCfBytes() {
java.lang.Object ref = cf_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
cf_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int SIZE_FIELD_NUMBER = 2;
private long size_;
/**
* uint64 size = 2;
*/
public long getSize() {
return size_;
}
private byte memoizedIsInitialized = -1;
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (!getCfBytes().isEmpty()) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 1, cf_);
}
if (size_ != 0L) {
output.writeUInt64(2, size_);
}
unknownFields.writeTo(output);
}
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (!getCfBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(1, cf_);
}
if (size_ != 0L) {
size += com.google.protobuf.CodedOutputStream
.computeUInt64Size(2, size_);
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof org.tikv.kvproto.Debugpb.RegionSizeResponse.Entry)) {
return super.equals(obj);
}
org.tikv.kvproto.Debugpb.RegionSizeResponse.Entry other = (org.tikv.kvproto.Debugpb.RegionSizeResponse.Entry) obj;
boolean result = true;
result = result && getCf()
.equals(other.getCf());
result = result && (getSize()
== other.getSize());
result = result && unknownFields.equals(other.unknownFields);
return result;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
hash = (37 * hash) + CF_FIELD_NUMBER;
hash = (53 * hash) + getCf().hashCode();
hash = (37 * hash) + SIZE_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
getSize());
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static org.tikv.kvproto.Debugpb.RegionSizeResponse.Entry parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.tikv.kvproto.Debugpb.RegionSizeResponse.Entry parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.tikv.kvproto.Debugpb.RegionSizeResponse.Entry parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.tikv.kvproto.Debugpb.RegionSizeResponse.Entry parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.tikv.kvproto.Debugpb.RegionSizeResponse.Entry parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.tikv.kvproto.Debugpb.RegionSizeResponse.Entry parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.tikv.kvproto.Debugpb.RegionSizeResponse.Entry parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static org.tikv.kvproto.Debugpb.RegionSizeResponse.Entry parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static org.tikv.kvproto.Debugpb.RegionSizeResponse.Entry parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static org.tikv.kvproto.Debugpb.RegionSizeResponse.Entry parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static org.tikv.kvproto.Debugpb.RegionSizeResponse.Entry parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static org.tikv.kvproto.Debugpb.RegionSizeResponse.Entry parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(org.tikv.kvproto.Debugpb.RegionSizeResponse.Entry prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code debugpb.RegionSizeResponse.Entry}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:debugpb.RegionSizeResponse.Entry)
org.tikv.kvproto.Debugpb.RegionSizeResponse.EntryOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.tikv.kvproto.Debugpb.internal_static_debugpb_RegionSizeResponse_Entry_descriptor;
}
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.tikv.kvproto.Debugpb.internal_static_debugpb_RegionSizeResponse_Entry_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.tikv.kvproto.Debugpb.RegionSizeResponse.Entry.class, org.tikv.kvproto.Debugpb.RegionSizeResponse.Entry.Builder.class);
}
// Construct using org.tikv.kvproto.Debugpb.RegionSizeResponse.Entry.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
public Builder clear() {
super.clear();
cf_ = "";
size_ = 0L;
return this;
}
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return org.tikv.kvproto.Debugpb.internal_static_debugpb_RegionSizeResponse_Entry_descriptor;
}
public org.tikv.kvproto.Debugpb.RegionSizeResponse.Entry getDefaultInstanceForType() {
return org.tikv.kvproto.Debugpb.RegionSizeResponse.Entry.getDefaultInstance();
}
public org.tikv.kvproto.Debugpb.RegionSizeResponse.Entry build() {
org.tikv.kvproto.Debugpb.RegionSizeResponse.Entry result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
public org.tikv.kvproto.Debugpb.RegionSizeResponse.Entry buildPartial() {
org.tikv.kvproto.Debugpb.RegionSizeResponse.Entry result = new org.tikv.kvproto.Debugpb.RegionSizeResponse.Entry(this);
result.cf_ = cf_;
result.size_ = size_;
onBuilt();
return result;
}
public Builder clone() {
return (Builder) super.clone();
}
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.setField(field, value);
}
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return (Builder) super.clearField(field);
}
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return (Builder) super.clearOneof(oneof);
}
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return (Builder) super.setRepeatedField(field, index, value);
}
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.addRepeatedField(field, value);
}
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof org.tikv.kvproto.Debugpb.RegionSizeResponse.Entry) {
return mergeFrom((org.tikv.kvproto.Debugpb.RegionSizeResponse.Entry)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(org.tikv.kvproto.Debugpb.RegionSizeResponse.Entry other) {
if (other == org.tikv.kvproto.Debugpb.RegionSizeResponse.Entry.getDefaultInstance()) return this;
if (!other.getCf().isEmpty()) {
cf_ = other.cf_;
onChanged();
}
if (other.getSize() != 0L) {
setSize(other.getSize());
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
public final boolean isInitialized() {
return true;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
org.tikv.kvproto.Debugpb.RegionSizeResponse.Entry parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (org.tikv.kvproto.Debugpb.RegionSizeResponse.Entry) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private java.lang.Object cf_ = "";
/**
* string cf = 1;
*/
public java.lang.String getCf() {
java.lang.Object ref = cf_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
cf_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* string cf = 1;
*/
public com.google.protobuf.ByteString
getCfBytes() {
java.lang.Object ref = cf_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
cf_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* string cf = 1;
*/
public Builder setCf(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
cf_ = value;
onChanged();
return this;
}
/**
* string cf = 1;
*/
public Builder clearCf() {
cf_ = getDefaultInstance().getCf();
onChanged();
return this;
}
/**
* string cf = 1;
*/
public Builder setCfBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
cf_ = value;
onChanged();
return this;
}
private long size_ ;
/**
* uint64 size = 2;
*/
public long getSize() {
return size_;
}
/**
* uint64 size = 2;
*/
public Builder setSize(long value) {
size_ = value;
onChanged();
return this;
}
/**
* uint64 size = 2;
*/
public Builder clearSize() {
size_ = 0L;
onChanged();
return this;
}
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFieldsProto3(unknownFields);
}
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:debugpb.RegionSizeResponse.Entry)
}
// @@protoc_insertion_point(class_scope:debugpb.RegionSizeResponse.Entry)
private static final org.tikv.kvproto.Debugpb.RegionSizeResponse.Entry DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new org.tikv.kvproto.Debugpb.RegionSizeResponse.Entry();
}
public static org.tikv.kvproto.Debugpb.RegionSizeResponse.Entry getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
public Entry parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new Entry(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
public org.tikv.kvproto.Debugpb.RegionSizeResponse.Entry getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public static final int ENTRIES_FIELD_NUMBER = 1;
private java.util.List entries_;
/**
* repeated .debugpb.RegionSizeResponse.Entry entries = 1;
*/
public java.util.List getEntriesList() {
return entries_;
}
/**
* repeated .debugpb.RegionSizeResponse.Entry entries = 1;
*/
public java.util.List extends org.tikv.kvproto.Debugpb.RegionSizeResponse.EntryOrBuilder>
getEntriesOrBuilderList() {
return entries_;
}
/**
* repeated .debugpb.RegionSizeResponse.Entry entries = 1;
*/
public int getEntriesCount() {
return entries_.size();
}
/**
* repeated .debugpb.RegionSizeResponse.Entry entries = 1;
*/
public org.tikv.kvproto.Debugpb.RegionSizeResponse.Entry getEntries(int index) {
return entries_.get(index);
}
/**
* repeated .debugpb.RegionSizeResponse.Entry entries = 1;
*/
public org.tikv.kvproto.Debugpb.RegionSizeResponse.EntryOrBuilder getEntriesOrBuilder(
int index) {
return entries_.get(index);
}
private byte memoizedIsInitialized = -1;
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
for (int i = 0; i < entries_.size(); i++) {
output.writeMessage(1, entries_.get(i));
}
unknownFields.writeTo(output);
}
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
for (int i = 0; i < entries_.size(); i++) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(1, entries_.get(i));
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof org.tikv.kvproto.Debugpb.RegionSizeResponse)) {
return super.equals(obj);
}
org.tikv.kvproto.Debugpb.RegionSizeResponse other = (org.tikv.kvproto.Debugpb.RegionSizeResponse) obj;
boolean result = true;
result = result && getEntriesList()
.equals(other.getEntriesList());
result = result && unknownFields.equals(other.unknownFields);
return result;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (getEntriesCount() > 0) {
hash = (37 * hash) + ENTRIES_FIELD_NUMBER;
hash = (53 * hash) + getEntriesList().hashCode();
}
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static org.tikv.kvproto.Debugpb.RegionSizeResponse parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.tikv.kvproto.Debugpb.RegionSizeResponse parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.tikv.kvproto.Debugpb.RegionSizeResponse parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.tikv.kvproto.Debugpb.RegionSizeResponse parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.tikv.kvproto.Debugpb.RegionSizeResponse parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.tikv.kvproto.Debugpb.RegionSizeResponse parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.tikv.kvproto.Debugpb.RegionSizeResponse parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static org.tikv.kvproto.Debugpb.RegionSizeResponse parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static org.tikv.kvproto.Debugpb.RegionSizeResponse parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static org.tikv.kvproto.Debugpb.RegionSizeResponse parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static org.tikv.kvproto.Debugpb.RegionSizeResponse parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static org.tikv.kvproto.Debugpb.RegionSizeResponse parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(org.tikv.kvproto.Debugpb.RegionSizeResponse prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code debugpb.RegionSizeResponse}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:debugpb.RegionSizeResponse)
org.tikv.kvproto.Debugpb.RegionSizeResponseOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.tikv.kvproto.Debugpb.internal_static_debugpb_RegionSizeResponse_descriptor;
}
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.tikv.kvproto.Debugpb.internal_static_debugpb_RegionSizeResponse_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.tikv.kvproto.Debugpb.RegionSizeResponse.class, org.tikv.kvproto.Debugpb.RegionSizeResponse.Builder.class);
}
// Construct using org.tikv.kvproto.Debugpb.RegionSizeResponse.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
getEntriesFieldBuilder();
}
}
public Builder clear() {
super.clear();
if (entriesBuilder_ == null) {
entries_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000001);
} else {
entriesBuilder_.clear();
}
return this;
}
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return org.tikv.kvproto.Debugpb.internal_static_debugpb_RegionSizeResponse_descriptor;
}
public org.tikv.kvproto.Debugpb.RegionSizeResponse getDefaultInstanceForType() {
return org.tikv.kvproto.Debugpb.RegionSizeResponse.getDefaultInstance();
}
public org.tikv.kvproto.Debugpb.RegionSizeResponse build() {
org.tikv.kvproto.Debugpb.RegionSizeResponse result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
public org.tikv.kvproto.Debugpb.RegionSizeResponse buildPartial() {
org.tikv.kvproto.Debugpb.RegionSizeResponse result = new org.tikv.kvproto.Debugpb.RegionSizeResponse(this);
int from_bitField0_ = bitField0_;
if (entriesBuilder_ == null) {
if (((bitField0_ & 0x00000001) == 0x00000001)) {
entries_ = java.util.Collections.unmodifiableList(entries_);
bitField0_ = (bitField0_ & ~0x00000001);
}
result.entries_ = entries_;
} else {
result.entries_ = entriesBuilder_.build();
}
onBuilt();
return result;
}
public Builder clone() {
return (Builder) super.clone();
}
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.setField(field, value);
}
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return (Builder) super.clearField(field);
}
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return (Builder) super.clearOneof(oneof);
}
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return (Builder) super.setRepeatedField(field, index, value);
}
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.addRepeatedField(field, value);
}
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof org.tikv.kvproto.Debugpb.RegionSizeResponse) {
return mergeFrom((org.tikv.kvproto.Debugpb.RegionSizeResponse)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(org.tikv.kvproto.Debugpb.RegionSizeResponse other) {
if (other == org.tikv.kvproto.Debugpb.RegionSizeResponse.getDefaultInstance()) return this;
if (entriesBuilder_ == null) {
if (!other.entries_.isEmpty()) {
if (entries_.isEmpty()) {
entries_ = other.entries_;
bitField0_ = (bitField0_ & ~0x00000001);
} else {
ensureEntriesIsMutable();
entries_.addAll(other.entries_);
}
onChanged();
}
} else {
if (!other.entries_.isEmpty()) {
if (entriesBuilder_.isEmpty()) {
entriesBuilder_.dispose();
entriesBuilder_ = null;
entries_ = other.entries_;
bitField0_ = (bitField0_ & ~0x00000001);
entriesBuilder_ =
com.google.protobuf.GeneratedMessageV3.alwaysUseFieldBuilders ?
getEntriesFieldBuilder() : null;
} else {
entriesBuilder_.addAllMessages(other.entries_);
}
}
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
public final boolean isInitialized() {
return true;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
org.tikv.kvproto.Debugpb.RegionSizeResponse parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (org.tikv.kvproto.Debugpb.RegionSizeResponse) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
private java.util.List entries_ =
java.util.Collections.emptyList();
private void ensureEntriesIsMutable() {
if (!((bitField0_ & 0x00000001) == 0x00000001)) {
entries_ = new java.util.ArrayList(entries_);
bitField0_ |= 0x00000001;
}
}
private com.google.protobuf.RepeatedFieldBuilderV3<
org.tikv.kvproto.Debugpb.RegionSizeResponse.Entry, org.tikv.kvproto.Debugpb.RegionSizeResponse.Entry.Builder, org.tikv.kvproto.Debugpb.RegionSizeResponse.EntryOrBuilder> entriesBuilder_;
/**
* repeated .debugpb.RegionSizeResponse.Entry entries = 1;
*/
public java.util.List getEntriesList() {
if (entriesBuilder_ == null) {
return java.util.Collections.unmodifiableList(entries_);
} else {
return entriesBuilder_.getMessageList();
}
}
/**
* repeated .debugpb.RegionSizeResponse.Entry entries = 1;
*/
public int getEntriesCount() {
if (entriesBuilder_ == null) {
return entries_.size();
} else {
return entriesBuilder_.getCount();
}
}
/**
* repeated .debugpb.RegionSizeResponse.Entry entries = 1;
*/
public org.tikv.kvproto.Debugpb.RegionSizeResponse.Entry getEntries(int index) {
if (entriesBuilder_ == null) {
return entries_.get(index);
} else {
return entriesBuilder_.getMessage(index);
}
}
/**
* repeated .debugpb.RegionSizeResponse.Entry entries = 1;
*/
public Builder setEntries(
int index, org.tikv.kvproto.Debugpb.RegionSizeResponse.Entry value) {
if (entriesBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureEntriesIsMutable();
entries_.set(index, value);
onChanged();
} else {
entriesBuilder_.setMessage(index, value);
}
return this;
}
/**
* repeated .debugpb.RegionSizeResponse.Entry entries = 1;
*/
public Builder setEntries(
int index, org.tikv.kvproto.Debugpb.RegionSizeResponse.Entry.Builder builderForValue) {
if (entriesBuilder_ == null) {
ensureEntriesIsMutable();
entries_.set(index, builderForValue.build());
onChanged();
} else {
entriesBuilder_.setMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .debugpb.RegionSizeResponse.Entry entries = 1;
*/
public Builder addEntries(org.tikv.kvproto.Debugpb.RegionSizeResponse.Entry value) {
if (entriesBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureEntriesIsMutable();
entries_.add(value);
onChanged();
} else {
entriesBuilder_.addMessage(value);
}
return this;
}
/**
* repeated .debugpb.RegionSizeResponse.Entry entries = 1;
*/
public Builder addEntries(
int index, org.tikv.kvproto.Debugpb.RegionSizeResponse.Entry value) {
if (entriesBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureEntriesIsMutable();
entries_.add(index, value);
onChanged();
} else {
entriesBuilder_.addMessage(index, value);
}
return this;
}
/**
* repeated .debugpb.RegionSizeResponse.Entry entries = 1;
*/
public Builder addEntries(
org.tikv.kvproto.Debugpb.RegionSizeResponse.Entry.Builder builderForValue) {
if (entriesBuilder_ == null) {
ensureEntriesIsMutable();
entries_.add(builderForValue.build());
onChanged();
} else {
entriesBuilder_.addMessage(builderForValue.build());
}
return this;
}
/**
* repeated .debugpb.RegionSizeResponse.Entry entries = 1;
*/
public Builder addEntries(
int index, org.tikv.kvproto.Debugpb.RegionSizeResponse.Entry.Builder builderForValue) {
if (entriesBuilder_ == null) {
ensureEntriesIsMutable();
entries_.add(index, builderForValue.build());
onChanged();
} else {
entriesBuilder_.addMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .debugpb.RegionSizeResponse.Entry entries = 1;
*/
public Builder addAllEntries(
java.lang.Iterable extends org.tikv.kvproto.Debugpb.RegionSizeResponse.Entry> values) {
if (entriesBuilder_ == null) {
ensureEntriesIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, entries_);
onChanged();
} else {
entriesBuilder_.addAllMessages(values);
}
return this;
}
/**
* repeated .debugpb.RegionSizeResponse.Entry entries = 1;
*/
public Builder clearEntries() {
if (entriesBuilder_ == null) {
entries_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000001);
onChanged();
} else {
entriesBuilder_.clear();
}
return this;
}
/**
* repeated .debugpb.RegionSizeResponse.Entry entries = 1;
*/
public Builder removeEntries(int index) {
if (entriesBuilder_ == null) {
ensureEntriesIsMutable();
entries_.remove(index);
onChanged();
} else {
entriesBuilder_.remove(index);
}
return this;
}
/**
* repeated .debugpb.RegionSizeResponse.Entry entries = 1;
*/
public org.tikv.kvproto.Debugpb.RegionSizeResponse.Entry.Builder getEntriesBuilder(
int index) {
return getEntriesFieldBuilder().getBuilder(index);
}
/**
* repeated .debugpb.RegionSizeResponse.Entry entries = 1;
*/
public org.tikv.kvproto.Debugpb.RegionSizeResponse.EntryOrBuilder getEntriesOrBuilder(
int index) {
if (entriesBuilder_ == null) {
return entries_.get(index); } else {
return entriesBuilder_.getMessageOrBuilder(index);
}
}
/**
* repeated .debugpb.RegionSizeResponse.Entry entries = 1;
*/
public java.util.List extends org.tikv.kvproto.Debugpb.RegionSizeResponse.EntryOrBuilder>
getEntriesOrBuilderList() {
if (entriesBuilder_ != null) {
return entriesBuilder_.getMessageOrBuilderList();
} else {
return java.util.Collections.unmodifiableList(entries_);
}
}
/**
* repeated .debugpb.RegionSizeResponse.Entry entries = 1;
*/
public org.tikv.kvproto.Debugpb.RegionSizeResponse.Entry.Builder addEntriesBuilder() {
return getEntriesFieldBuilder().addBuilder(
org.tikv.kvproto.Debugpb.RegionSizeResponse.Entry.getDefaultInstance());
}
/**
* repeated .debugpb.RegionSizeResponse.Entry entries = 1;
*/
public org.tikv.kvproto.Debugpb.RegionSizeResponse.Entry.Builder addEntriesBuilder(
int index) {
return getEntriesFieldBuilder().addBuilder(
index, org.tikv.kvproto.Debugpb.RegionSizeResponse.Entry.getDefaultInstance());
}
/**
* repeated .debugpb.RegionSizeResponse.Entry entries = 1;
*/
public java.util.List
getEntriesBuilderList() {
return getEntriesFieldBuilder().getBuilderList();
}
private com.google.protobuf.RepeatedFieldBuilderV3<
org.tikv.kvproto.Debugpb.RegionSizeResponse.Entry, org.tikv.kvproto.Debugpb.RegionSizeResponse.Entry.Builder, org.tikv.kvproto.Debugpb.RegionSizeResponse.EntryOrBuilder>
getEntriesFieldBuilder() {
if (entriesBuilder_ == null) {
entriesBuilder_ = new com.google.protobuf.RepeatedFieldBuilderV3<
org.tikv.kvproto.Debugpb.RegionSizeResponse.Entry, org.tikv.kvproto.Debugpb.RegionSizeResponse.Entry.Builder, org.tikv.kvproto.Debugpb.RegionSizeResponse.EntryOrBuilder>(
entries_,
((bitField0_ & 0x00000001) == 0x00000001),
getParentForChildren(),
isClean());
entries_ = null;
}
return entriesBuilder_;
}
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFieldsProto3(unknownFields);
}
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:debugpb.RegionSizeResponse)
}
// @@protoc_insertion_point(class_scope:debugpb.RegionSizeResponse)
private static final org.tikv.kvproto.Debugpb.RegionSizeResponse DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new org.tikv.kvproto.Debugpb.RegionSizeResponse();
}
public static org.tikv.kvproto.Debugpb.RegionSizeResponse getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
public RegionSizeResponse parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new RegionSizeResponse(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
public org.tikv.kvproto.Debugpb.RegionSizeResponse getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface ScanMvccRequestOrBuilder extends
// @@protoc_insertion_point(interface_extends:debugpb.ScanMvccRequest)
com.google.protobuf.MessageOrBuilder {
/**
* bytes from_key = 1;
*/
com.google.protobuf.ByteString getFromKey();
/**
* bytes to_key = 2;
*/
com.google.protobuf.ByteString getToKey();
/**
* uint64 limit = 3;
*/
long getLimit();
}
/**
* Protobuf type {@code debugpb.ScanMvccRequest}
*/
public static final class ScanMvccRequest extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:debugpb.ScanMvccRequest)
ScanMvccRequestOrBuilder {
private static final long serialVersionUID = 0L;
// Use ScanMvccRequest.newBuilder() to construct.
private ScanMvccRequest(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private ScanMvccRequest() {
fromKey_ = com.google.protobuf.ByteString.EMPTY;
toKey_ = com.google.protobuf.ByteString.EMPTY;
limit_ = 0L;
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private ScanMvccRequest(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
default: {
if (!parseUnknownFieldProto3(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
case 10: {
fromKey_ = input.readBytes();
break;
}
case 18: {
toKey_ = input.readBytes();
break;
}
case 24: {
limit_ = input.readUInt64();
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.tikv.kvproto.Debugpb.internal_static_debugpb_ScanMvccRequest_descriptor;
}
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.tikv.kvproto.Debugpb.internal_static_debugpb_ScanMvccRequest_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.tikv.kvproto.Debugpb.ScanMvccRequest.class, org.tikv.kvproto.Debugpb.ScanMvccRequest.Builder.class);
}
public static final int FROM_KEY_FIELD_NUMBER = 1;
private com.google.protobuf.ByteString fromKey_;
/**
* bytes from_key = 1;
*/
public com.google.protobuf.ByteString getFromKey() {
return fromKey_;
}
public static final int TO_KEY_FIELD_NUMBER = 2;
private com.google.protobuf.ByteString toKey_;
/**
* bytes to_key = 2;
*/
public com.google.protobuf.ByteString getToKey() {
return toKey_;
}
public static final int LIMIT_FIELD_NUMBER = 3;
private long limit_;
/**
* uint64 limit = 3;
*/
public long getLimit() {
return limit_;
}
private byte memoizedIsInitialized = -1;
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (!fromKey_.isEmpty()) {
output.writeBytes(1, fromKey_);
}
if (!toKey_.isEmpty()) {
output.writeBytes(2, toKey_);
}
if (limit_ != 0L) {
output.writeUInt64(3, limit_);
}
unknownFields.writeTo(output);
}
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (!fromKey_.isEmpty()) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(1, fromKey_);
}
if (!toKey_.isEmpty()) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(2, toKey_);
}
if (limit_ != 0L) {
size += com.google.protobuf.CodedOutputStream
.computeUInt64Size(3, limit_);
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof org.tikv.kvproto.Debugpb.ScanMvccRequest)) {
return super.equals(obj);
}
org.tikv.kvproto.Debugpb.ScanMvccRequest other = (org.tikv.kvproto.Debugpb.ScanMvccRequest) obj;
boolean result = true;
result = result && getFromKey()
.equals(other.getFromKey());
result = result && getToKey()
.equals(other.getToKey());
result = result && (getLimit()
== other.getLimit());
result = result && unknownFields.equals(other.unknownFields);
return result;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
hash = (37 * hash) + FROM_KEY_FIELD_NUMBER;
hash = (53 * hash) + getFromKey().hashCode();
hash = (37 * hash) + TO_KEY_FIELD_NUMBER;
hash = (53 * hash) + getToKey().hashCode();
hash = (37 * hash) + LIMIT_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
getLimit());
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static org.tikv.kvproto.Debugpb.ScanMvccRequest parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.tikv.kvproto.Debugpb.ScanMvccRequest parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.tikv.kvproto.Debugpb.ScanMvccRequest parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.tikv.kvproto.Debugpb.ScanMvccRequest parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.tikv.kvproto.Debugpb.ScanMvccRequest parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.tikv.kvproto.Debugpb.ScanMvccRequest parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.tikv.kvproto.Debugpb.ScanMvccRequest parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static org.tikv.kvproto.Debugpb.ScanMvccRequest parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static org.tikv.kvproto.Debugpb.ScanMvccRequest parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static org.tikv.kvproto.Debugpb.ScanMvccRequest parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static org.tikv.kvproto.Debugpb.ScanMvccRequest parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static org.tikv.kvproto.Debugpb.ScanMvccRequest parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(org.tikv.kvproto.Debugpb.ScanMvccRequest prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code debugpb.ScanMvccRequest}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:debugpb.ScanMvccRequest)
org.tikv.kvproto.Debugpb.ScanMvccRequestOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.tikv.kvproto.Debugpb.internal_static_debugpb_ScanMvccRequest_descriptor;
}
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.tikv.kvproto.Debugpb.internal_static_debugpb_ScanMvccRequest_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.tikv.kvproto.Debugpb.ScanMvccRequest.class, org.tikv.kvproto.Debugpb.ScanMvccRequest.Builder.class);
}
// Construct using org.tikv.kvproto.Debugpb.ScanMvccRequest.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
public Builder clear() {
super.clear();
fromKey_ = com.google.protobuf.ByteString.EMPTY;
toKey_ = com.google.protobuf.ByteString.EMPTY;
limit_ = 0L;
return this;
}
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return org.tikv.kvproto.Debugpb.internal_static_debugpb_ScanMvccRequest_descriptor;
}
public org.tikv.kvproto.Debugpb.ScanMvccRequest getDefaultInstanceForType() {
return org.tikv.kvproto.Debugpb.ScanMvccRequest.getDefaultInstance();
}
public org.tikv.kvproto.Debugpb.ScanMvccRequest build() {
org.tikv.kvproto.Debugpb.ScanMvccRequest result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
public org.tikv.kvproto.Debugpb.ScanMvccRequest buildPartial() {
org.tikv.kvproto.Debugpb.ScanMvccRequest result = new org.tikv.kvproto.Debugpb.ScanMvccRequest(this);
result.fromKey_ = fromKey_;
result.toKey_ = toKey_;
result.limit_ = limit_;
onBuilt();
return result;
}
public Builder clone() {
return (Builder) super.clone();
}
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.setField(field, value);
}
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return (Builder) super.clearField(field);
}
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return (Builder) super.clearOneof(oneof);
}
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return (Builder) super.setRepeatedField(field, index, value);
}
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.addRepeatedField(field, value);
}
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof org.tikv.kvproto.Debugpb.ScanMvccRequest) {
return mergeFrom((org.tikv.kvproto.Debugpb.ScanMvccRequest)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(org.tikv.kvproto.Debugpb.ScanMvccRequest other) {
if (other == org.tikv.kvproto.Debugpb.ScanMvccRequest.getDefaultInstance()) return this;
if (other.getFromKey() != com.google.protobuf.ByteString.EMPTY) {
setFromKey(other.getFromKey());
}
if (other.getToKey() != com.google.protobuf.ByteString.EMPTY) {
setToKey(other.getToKey());
}
if (other.getLimit() != 0L) {
setLimit(other.getLimit());
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
public final boolean isInitialized() {
return true;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
org.tikv.kvproto.Debugpb.ScanMvccRequest parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (org.tikv.kvproto.Debugpb.ScanMvccRequest) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private com.google.protobuf.ByteString fromKey_ = com.google.protobuf.ByteString.EMPTY;
/**
* bytes from_key = 1;
*/
public com.google.protobuf.ByteString getFromKey() {
return fromKey_;
}
/**
* bytes from_key = 1;
*/
public Builder setFromKey(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
fromKey_ = value;
onChanged();
return this;
}
/**
* bytes from_key = 1;
*/
public Builder clearFromKey() {
fromKey_ = getDefaultInstance().getFromKey();
onChanged();
return this;
}
private com.google.protobuf.ByteString toKey_ = com.google.protobuf.ByteString.EMPTY;
/**
* bytes to_key = 2;
*/
public com.google.protobuf.ByteString getToKey() {
return toKey_;
}
/**
* bytes to_key = 2;
*/
public Builder setToKey(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
toKey_ = value;
onChanged();
return this;
}
/**
* bytes to_key = 2;
*/
public Builder clearToKey() {
toKey_ = getDefaultInstance().getToKey();
onChanged();
return this;
}
private long limit_ ;
/**
* uint64 limit = 3;
*/
public long getLimit() {
return limit_;
}
/**
* uint64 limit = 3;
*/
public Builder setLimit(long value) {
limit_ = value;
onChanged();
return this;
}
/**
* uint64 limit = 3;
*/
public Builder clearLimit() {
limit_ = 0L;
onChanged();
return this;
}
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFieldsProto3(unknownFields);
}
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:debugpb.ScanMvccRequest)
}
// @@protoc_insertion_point(class_scope:debugpb.ScanMvccRequest)
private static final org.tikv.kvproto.Debugpb.ScanMvccRequest DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new org.tikv.kvproto.Debugpb.ScanMvccRequest();
}
public static org.tikv.kvproto.Debugpb.ScanMvccRequest getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
public ScanMvccRequest parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new ScanMvccRequest(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
public org.tikv.kvproto.Debugpb.ScanMvccRequest getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface ScanMvccResponseOrBuilder extends
// @@protoc_insertion_point(interface_extends:debugpb.ScanMvccResponse)
com.google.protobuf.MessageOrBuilder {
/**
* bytes key = 1;
*/
com.google.protobuf.ByteString getKey();
/**
* .kvrpcpb.MvccInfo info = 2;
*/
boolean hasInfo();
/**
* .kvrpcpb.MvccInfo info = 2;
*/
org.tikv.kvproto.Kvrpcpb.MvccInfo getInfo();
/**
* .kvrpcpb.MvccInfo info = 2;
*/
org.tikv.kvproto.Kvrpcpb.MvccInfoOrBuilder getInfoOrBuilder();
}
/**
* Protobuf type {@code debugpb.ScanMvccResponse}
*/
public static final class ScanMvccResponse extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:debugpb.ScanMvccResponse)
ScanMvccResponseOrBuilder {
private static final long serialVersionUID = 0L;
// Use ScanMvccResponse.newBuilder() to construct.
private ScanMvccResponse(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private ScanMvccResponse() {
key_ = com.google.protobuf.ByteString.EMPTY;
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private ScanMvccResponse(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
default: {
if (!parseUnknownFieldProto3(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
case 10: {
key_ = input.readBytes();
break;
}
case 18: {
org.tikv.kvproto.Kvrpcpb.MvccInfo.Builder subBuilder = null;
if (info_ != null) {
subBuilder = info_.toBuilder();
}
info_ = input.readMessage(org.tikv.kvproto.Kvrpcpb.MvccInfo.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(info_);
info_ = subBuilder.buildPartial();
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.tikv.kvproto.Debugpb.internal_static_debugpb_ScanMvccResponse_descriptor;
}
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.tikv.kvproto.Debugpb.internal_static_debugpb_ScanMvccResponse_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.tikv.kvproto.Debugpb.ScanMvccResponse.class, org.tikv.kvproto.Debugpb.ScanMvccResponse.Builder.class);
}
public static final int KEY_FIELD_NUMBER = 1;
private com.google.protobuf.ByteString key_;
/**
* bytes key = 1;
*/
public com.google.protobuf.ByteString getKey() {
return key_;
}
public static final int INFO_FIELD_NUMBER = 2;
private org.tikv.kvproto.Kvrpcpb.MvccInfo info_;
/**
* .kvrpcpb.MvccInfo info = 2;
*/
public boolean hasInfo() {
return info_ != null;
}
/**
* .kvrpcpb.MvccInfo info = 2;
*/
public org.tikv.kvproto.Kvrpcpb.MvccInfo getInfo() {
return info_ == null ? org.tikv.kvproto.Kvrpcpb.MvccInfo.getDefaultInstance() : info_;
}
/**
* .kvrpcpb.MvccInfo info = 2;
*/
public org.tikv.kvproto.Kvrpcpb.MvccInfoOrBuilder getInfoOrBuilder() {
return getInfo();
}
private byte memoizedIsInitialized = -1;
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (!key_.isEmpty()) {
output.writeBytes(1, key_);
}
if (info_ != null) {
output.writeMessage(2, getInfo());
}
unknownFields.writeTo(output);
}
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (!key_.isEmpty()) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(1, key_);
}
if (info_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(2, getInfo());
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof org.tikv.kvproto.Debugpb.ScanMvccResponse)) {
return super.equals(obj);
}
org.tikv.kvproto.Debugpb.ScanMvccResponse other = (org.tikv.kvproto.Debugpb.ScanMvccResponse) obj;
boolean result = true;
result = result && getKey()
.equals(other.getKey());
result = result && (hasInfo() == other.hasInfo());
if (hasInfo()) {
result = result && getInfo()
.equals(other.getInfo());
}
result = result && unknownFields.equals(other.unknownFields);
return result;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
hash = (37 * hash) + KEY_FIELD_NUMBER;
hash = (53 * hash) + getKey().hashCode();
if (hasInfo()) {
hash = (37 * hash) + INFO_FIELD_NUMBER;
hash = (53 * hash) + getInfo().hashCode();
}
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static org.tikv.kvproto.Debugpb.ScanMvccResponse parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.tikv.kvproto.Debugpb.ScanMvccResponse parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.tikv.kvproto.Debugpb.ScanMvccResponse parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.tikv.kvproto.Debugpb.ScanMvccResponse parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.tikv.kvproto.Debugpb.ScanMvccResponse parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.tikv.kvproto.Debugpb.ScanMvccResponse parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.tikv.kvproto.Debugpb.ScanMvccResponse parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static org.tikv.kvproto.Debugpb.ScanMvccResponse parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static org.tikv.kvproto.Debugpb.ScanMvccResponse parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static org.tikv.kvproto.Debugpb.ScanMvccResponse parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static org.tikv.kvproto.Debugpb.ScanMvccResponse parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static org.tikv.kvproto.Debugpb.ScanMvccResponse parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(org.tikv.kvproto.Debugpb.ScanMvccResponse prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code debugpb.ScanMvccResponse}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:debugpb.ScanMvccResponse)
org.tikv.kvproto.Debugpb.ScanMvccResponseOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.tikv.kvproto.Debugpb.internal_static_debugpb_ScanMvccResponse_descriptor;
}
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.tikv.kvproto.Debugpb.internal_static_debugpb_ScanMvccResponse_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.tikv.kvproto.Debugpb.ScanMvccResponse.class, org.tikv.kvproto.Debugpb.ScanMvccResponse.Builder.class);
}
// Construct using org.tikv.kvproto.Debugpb.ScanMvccResponse.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
public Builder clear() {
super.clear();
key_ = com.google.protobuf.ByteString.EMPTY;
if (infoBuilder_ == null) {
info_ = null;
} else {
info_ = null;
infoBuilder_ = null;
}
return this;
}
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return org.tikv.kvproto.Debugpb.internal_static_debugpb_ScanMvccResponse_descriptor;
}
public org.tikv.kvproto.Debugpb.ScanMvccResponse getDefaultInstanceForType() {
return org.tikv.kvproto.Debugpb.ScanMvccResponse.getDefaultInstance();
}
public org.tikv.kvproto.Debugpb.ScanMvccResponse build() {
org.tikv.kvproto.Debugpb.ScanMvccResponse result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
public org.tikv.kvproto.Debugpb.ScanMvccResponse buildPartial() {
org.tikv.kvproto.Debugpb.ScanMvccResponse result = new org.tikv.kvproto.Debugpb.ScanMvccResponse(this);
result.key_ = key_;
if (infoBuilder_ == null) {
result.info_ = info_;
} else {
result.info_ = infoBuilder_.build();
}
onBuilt();
return result;
}
public Builder clone() {
return (Builder) super.clone();
}
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.setField(field, value);
}
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return (Builder) super.clearField(field);
}
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return (Builder) super.clearOneof(oneof);
}
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return (Builder) super.setRepeatedField(field, index, value);
}
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.addRepeatedField(field, value);
}
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof org.tikv.kvproto.Debugpb.ScanMvccResponse) {
return mergeFrom((org.tikv.kvproto.Debugpb.ScanMvccResponse)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(org.tikv.kvproto.Debugpb.ScanMvccResponse other) {
if (other == org.tikv.kvproto.Debugpb.ScanMvccResponse.getDefaultInstance()) return this;
if (other.getKey() != com.google.protobuf.ByteString.EMPTY) {
setKey(other.getKey());
}
if (other.hasInfo()) {
mergeInfo(other.getInfo());
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
public final boolean isInitialized() {
return true;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
org.tikv.kvproto.Debugpb.ScanMvccResponse parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (org.tikv.kvproto.Debugpb.ScanMvccResponse) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private com.google.protobuf.ByteString key_ = com.google.protobuf.ByteString.EMPTY;
/**
* bytes key = 1;
*/
public com.google.protobuf.ByteString getKey() {
return key_;
}
/**
* bytes key = 1;
*/
public Builder setKey(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
key_ = value;
onChanged();
return this;
}
/**
* bytes key = 1;
*/
public Builder clearKey() {
key_ = getDefaultInstance().getKey();
onChanged();
return this;
}
private org.tikv.kvproto.Kvrpcpb.MvccInfo info_ = null;
private com.google.protobuf.SingleFieldBuilderV3<
org.tikv.kvproto.Kvrpcpb.MvccInfo, org.tikv.kvproto.Kvrpcpb.MvccInfo.Builder, org.tikv.kvproto.Kvrpcpb.MvccInfoOrBuilder> infoBuilder_;
/**
* .kvrpcpb.MvccInfo info = 2;
*/
public boolean hasInfo() {
return infoBuilder_ != null || info_ != null;
}
/**
* .kvrpcpb.MvccInfo info = 2;
*/
public org.tikv.kvproto.Kvrpcpb.MvccInfo getInfo() {
if (infoBuilder_ == null) {
return info_ == null ? org.tikv.kvproto.Kvrpcpb.MvccInfo.getDefaultInstance() : info_;
} else {
return infoBuilder_.getMessage();
}
}
/**
* .kvrpcpb.MvccInfo info = 2;
*/
public Builder setInfo(org.tikv.kvproto.Kvrpcpb.MvccInfo value) {
if (infoBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
info_ = value;
onChanged();
} else {
infoBuilder_.setMessage(value);
}
return this;
}
/**
* .kvrpcpb.MvccInfo info = 2;
*/
public Builder setInfo(
org.tikv.kvproto.Kvrpcpb.MvccInfo.Builder builderForValue) {
if (infoBuilder_ == null) {
info_ = builderForValue.build();
onChanged();
} else {
infoBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
* .kvrpcpb.MvccInfo info = 2;
*/
public Builder mergeInfo(org.tikv.kvproto.Kvrpcpb.MvccInfo value) {
if (infoBuilder_ == null) {
if (info_ != null) {
info_ =
org.tikv.kvproto.Kvrpcpb.MvccInfo.newBuilder(info_).mergeFrom(value).buildPartial();
} else {
info_ = value;
}
onChanged();
} else {
infoBuilder_.mergeFrom(value);
}
return this;
}
/**
* .kvrpcpb.MvccInfo info = 2;
*/
public Builder clearInfo() {
if (infoBuilder_ == null) {
info_ = null;
onChanged();
} else {
info_ = null;
infoBuilder_ = null;
}
return this;
}
/**
* .kvrpcpb.MvccInfo info = 2;
*/
public org.tikv.kvproto.Kvrpcpb.MvccInfo.Builder getInfoBuilder() {
onChanged();
return getInfoFieldBuilder().getBuilder();
}
/**
* .kvrpcpb.MvccInfo info = 2;
*/
public org.tikv.kvproto.Kvrpcpb.MvccInfoOrBuilder getInfoOrBuilder() {
if (infoBuilder_ != null) {
return infoBuilder_.getMessageOrBuilder();
} else {
return info_ == null ?
org.tikv.kvproto.Kvrpcpb.MvccInfo.getDefaultInstance() : info_;
}
}
/**
* .kvrpcpb.MvccInfo info = 2;
*/
private com.google.protobuf.SingleFieldBuilderV3<
org.tikv.kvproto.Kvrpcpb.MvccInfo, org.tikv.kvproto.Kvrpcpb.MvccInfo.Builder, org.tikv.kvproto.Kvrpcpb.MvccInfoOrBuilder>
getInfoFieldBuilder() {
if (infoBuilder_ == null) {
infoBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
org.tikv.kvproto.Kvrpcpb.MvccInfo, org.tikv.kvproto.Kvrpcpb.MvccInfo.Builder, org.tikv.kvproto.Kvrpcpb.MvccInfoOrBuilder>(
getInfo(),
getParentForChildren(),
isClean());
info_ = null;
}
return infoBuilder_;
}
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFieldsProto3(unknownFields);
}
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:debugpb.ScanMvccResponse)
}
// @@protoc_insertion_point(class_scope:debugpb.ScanMvccResponse)
private static final org.tikv.kvproto.Debugpb.ScanMvccResponse DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new org.tikv.kvproto.Debugpb.ScanMvccResponse();
}
public static org.tikv.kvproto.Debugpb.ScanMvccResponse getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
public ScanMvccResponse parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new ScanMvccResponse(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
public org.tikv.kvproto.Debugpb.ScanMvccResponse getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface CompactRequestOrBuilder extends
// @@protoc_insertion_point(interface_extends:debugpb.CompactRequest)
com.google.protobuf.MessageOrBuilder {
/**
* .debugpb.DB db = 1;
*/
int getDbValue();
/**
* .debugpb.DB db = 1;
*/
org.tikv.kvproto.Debugpb.DB getDb();
/**
* string cf = 2;
*/
java.lang.String getCf();
/**
* string cf = 2;
*/
com.google.protobuf.ByteString
getCfBytes();
/**
* bytes from_key = 3;
*/
com.google.protobuf.ByteString getFromKey();
/**
* bytes to_key = 4;
*/
com.google.protobuf.ByteString getToKey();
/**
* uint32 threads = 5;
*/
int getThreads();
/**
* .debugpb.BottommostLevelCompaction bottommost_level_compaction = 6;
*/
int getBottommostLevelCompactionValue();
/**
* .debugpb.BottommostLevelCompaction bottommost_level_compaction = 6;
*/
org.tikv.kvproto.Debugpb.BottommostLevelCompaction getBottommostLevelCompaction();
}
/**
* Protobuf type {@code debugpb.CompactRequest}
*/
public static final class CompactRequest extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:debugpb.CompactRequest)
CompactRequestOrBuilder {
private static final long serialVersionUID = 0L;
// Use CompactRequest.newBuilder() to construct.
private CompactRequest(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private CompactRequest() {
db_ = 0;
cf_ = "";
fromKey_ = com.google.protobuf.ByteString.EMPTY;
toKey_ = com.google.protobuf.ByteString.EMPTY;
threads_ = 0;
bottommostLevelCompaction_ = 0;
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private CompactRequest(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
default: {
if (!parseUnknownFieldProto3(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
case 8: {
int rawValue = input.readEnum();
db_ = rawValue;
break;
}
case 18: {
java.lang.String s = input.readStringRequireUtf8();
cf_ = s;
break;
}
case 26: {
fromKey_ = input.readBytes();
break;
}
case 34: {
toKey_ = input.readBytes();
break;
}
case 40: {
threads_ = input.readUInt32();
break;
}
case 48: {
int rawValue = input.readEnum();
bottommostLevelCompaction_ = rawValue;
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.tikv.kvproto.Debugpb.internal_static_debugpb_CompactRequest_descriptor;
}
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.tikv.kvproto.Debugpb.internal_static_debugpb_CompactRequest_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.tikv.kvproto.Debugpb.CompactRequest.class, org.tikv.kvproto.Debugpb.CompactRequest.Builder.class);
}
public static final int DB_FIELD_NUMBER = 1;
private int db_;
/**
* .debugpb.DB db = 1;
*/
public int getDbValue() {
return db_;
}
/**
* .debugpb.DB db = 1;
*/
public org.tikv.kvproto.Debugpb.DB getDb() {
org.tikv.kvproto.Debugpb.DB result = org.tikv.kvproto.Debugpb.DB.valueOf(db_);
return result == null ? org.tikv.kvproto.Debugpb.DB.UNRECOGNIZED : result;
}
public static final int CF_FIELD_NUMBER = 2;
private volatile java.lang.Object cf_;
/**
* string cf = 2;
*/
public java.lang.String getCf() {
java.lang.Object ref = cf_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
cf_ = s;
return s;
}
}
/**
* string cf = 2;
*/
public com.google.protobuf.ByteString
getCfBytes() {
java.lang.Object ref = cf_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
cf_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int FROM_KEY_FIELD_NUMBER = 3;
private com.google.protobuf.ByteString fromKey_;
/**
* bytes from_key = 3;
*/
public com.google.protobuf.ByteString getFromKey() {
return fromKey_;
}
public static final int TO_KEY_FIELD_NUMBER = 4;
private com.google.protobuf.ByteString toKey_;
/**
* bytes to_key = 4;
*/
public com.google.protobuf.ByteString getToKey() {
return toKey_;
}
public static final int THREADS_FIELD_NUMBER = 5;
private int threads_;
/**
* uint32 threads = 5;
*/
public int getThreads() {
return threads_;
}
public static final int BOTTOMMOST_LEVEL_COMPACTION_FIELD_NUMBER = 6;
private int bottommostLevelCompaction_;
/**
* .debugpb.BottommostLevelCompaction bottommost_level_compaction = 6;
*/
public int getBottommostLevelCompactionValue() {
return bottommostLevelCompaction_;
}
/**
* .debugpb.BottommostLevelCompaction bottommost_level_compaction = 6;
*/
public org.tikv.kvproto.Debugpb.BottommostLevelCompaction getBottommostLevelCompaction() {
org.tikv.kvproto.Debugpb.BottommostLevelCompaction result = org.tikv.kvproto.Debugpb.BottommostLevelCompaction.valueOf(bottommostLevelCompaction_);
return result == null ? org.tikv.kvproto.Debugpb.BottommostLevelCompaction.UNRECOGNIZED : result;
}
private byte memoizedIsInitialized = -1;
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (db_ != org.tikv.kvproto.Debugpb.DB.INVALID.getNumber()) {
output.writeEnum(1, db_);
}
if (!getCfBytes().isEmpty()) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 2, cf_);
}
if (!fromKey_.isEmpty()) {
output.writeBytes(3, fromKey_);
}
if (!toKey_.isEmpty()) {
output.writeBytes(4, toKey_);
}
if (threads_ != 0) {
output.writeUInt32(5, threads_);
}
if (bottommostLevelCompaction_ != org.tikv.kvproto.Debugpb.BottommostLevelCompaction.Skip.getNumber()) {
output.writeEnum(6, bottommostLevelCompaction_);
}
unknownFields.writeTo(output);
}
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (db_ != org.tikv.kvproto.Debugpb.DB.INVALID.getNumber()) {
size += com.google.protobuf.CodedOutputStream
.computeEnumSize(1, db_);
}
if (!getCfBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(2, cf_);
}
if (!fromKey_.isEmpty()) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(3, fromKey_);
}
if (!toKey_.isEmpty()) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(4, toKey_);
}
if (threads_ != 0) {
size += com.google.protobuf.CodedOutputStream
.computeUInt32Size(5, threads_);
}
if (bottommostLevelCompaction_ != org.tikv.kvproto.Debugpb.BottommostLevelCompaction.Skip.getNumber()) {
size += com.google.protobuf.CodedOutputStream
.computeEnumSize(6, bottommostLevelCompaction_);
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof org.tikv.kvproto.Debugpb.CompactRequest)) {
return super.equals(obj);
}
org.tikv.kvproto.Debugpb.CompactRequest other = (org.tikv.kvproto.Debugpb.CompactRequest) obj;
boolean result = true;
result = result && db_ == other.db_;
result = result && getCf()
.equals(other.getCf());
result = result && getFromKey()
.equals(other.getFromKey());
result = result && getToKey()
.equals(other.getToKey());
result = result && (getThreads()
== other.getThreads());
result = result && bottommostLevelCompaction_ == other.bottommostLevelCompaction_;
result = result && unknownFields.equals(other.unknownFields);
return result;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
hash = (37 * hash) + DB_FIELD_NUMBER;
hash = (53 * hash) + db_;
hash = (37 * hash) + CF_FIELD_NUMBER;
hash = (53 * hash) + getCf().hashCode();
hash = (37 * hash) + FROM_KEY_FIELD_NUMBER;
hash = (53 * hash) + getFromKey().hashCode();
hash = (37 * hash) + TO_KEY_FIELD_NUMBER;
hash = (53 * hash) + getToKey().hashCode();
hash = (37 * hash) + THREADS_FIELD_NUMBER;
hash = (53 * hash) + getThreads();
hash = (37 * hash) + BOTTOMMOST_LEVEL_COMPACTION_FIELD_NUMBER;
hash = (53 * hash) + bottommostLevelCompaction_;
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static org.tikv.kvproto.Debugpb.CompactRequest parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.tikv.kvproto.Debugpb.CompactRequest parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.tikv.kvproto.Debugpb.CompactRequest parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.tikv.kvproto.Debugpb.CompactRequest parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.tikv.kvproto.Debugpb.CompactRequest parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.tikv.kvproto.Debugpb.CompactRequest parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.tikv.kvproto.Debugpb.CompactRequest parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static org.tikv.kvproto.Debugpb.CompactRequest parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static org.tikv.kvproto.Debugpb.CompactRequest parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static org.tikv.kvproto.Debugpb.CompactRequest parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static org.tikv.kvproto.Debugpb.CompactRequest parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static org.tikv.kvproto.Debugpb.CompactRequest parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(org.tikv.kvproto.Debugpb.CompactRequest prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code debugpb.CompactRequest}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:debugpb.CompactRequest)
org.tikv.kvproto.Debugpb.CompactRequestOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.tikv.kvproto.Debugpb.internal_static_debugpb_CompactRequest_descriptor;
}
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.tikv.kvproto.Debugpb.internal_static_debugpb_CompactRequest_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.tikv.kvproto.Debugpb.CompactRequest.class, org.tikv.kvproto.Debugpb.CompactRequest.Builder.class);
}
// Construct using org.tikv.kvproto.Debugpb.CompactRequest.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
public Builder clear() {
super.clear();
db_ = 0;
cf_ = "";
fromKey_ = com.google.protobuf.ByteString.EMPTY;
toKey_ = com.google.protobuf.ByteString.EMPTY;
threads_ = 0;
bottommostLevelCompaction_ = 0;
return this;
}
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return org.tikv.kvproto.Debugpb.internal_static_debugpb_CompactRequest_descriptor;
}
public org.tikv.kvproto.Debugpb.CompactRequest getDefaultInstanceForType() {
return org.tikv.kvproto.Debugpb.CompactRequest.getDefaultInstance();
}
public org.tikv.kvproto.Debugpb.CompactRequest build() {
org.tikv.kvproto.Debugpb.CompactRequest result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
public org.tikv.kvproto.Debugpb.CompactRequest buildPartial() {
org.tikv.kvproto.Debugpb.CompactRequest result = new org.tikv.kvproto.Debugpb.CompactRequest(this);
result.db_ = db_;
result.cf_ = cf_;
result.fromKey_ = fromKey_;
result.toKey_ = toKey_;
result.threads_ = threads_;
result.bottommostLevelCompaction_ = bottommostLevelCompaction_;
onBuilt();
return result;
}
public Builder clone() {
return (Builder) super.clone();
}
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.setField(field, value);
}
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return (Builder) super.clearField(field);
}
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return (Builder) super.clearOneof(oneof);
}
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return (Builder) super.setRepeatedField(field, index, value);
}
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.addRepeatedField(field, value);
}
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof org.tikv.kvproto.Debugpb.CompactRequest) {
return mergeFrom((org.tikv.kvproto.Debugpb.CompactRequest)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(org.tikv.kvproto.Debugpb.CompactRequest other) {
if (other == org.tikv.kvproto.Debugpb.CompactRequest.getDefaultInstance()) return this;
if (other.db_ != 0) {
setDbValue(other.getDbValue());
}
if (!other.getCf().isEmpty()) {
cf_ = other.cf_;
onChanged();
}
if (other.getFromKey() != com.google.protobuf.ByteString.EMPTY) {
setFromKey(other.getFromKey());
}
if (other.getToKey() != com.google.protobuf.ByteString.EMPTY) {
setToKey(other.getToKey());
}
if (other.getThreads() != 0) {
setThreads(other.getThreads());
}
if (other.bottommostLevelCompaction_ != 0) {
setBottommostLevelCompactionValue(other.getBottommostLevelCompactionValue());
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
public final boolean isInitialized() {
return true;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
org.tikv.kvproto.Debugpb.CompactRequest parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (org.tikv.kvproto.Debugpb.CompactRequest) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int db_ = 0;
/**
* .debugpb.DB db = 1;
*/
public int getDbValue() {
return db_;
}
/**
* .debugpb.DB db = 1;
*/
public Builder setDbValue(int value) {
db_ = value;
onChanged();
return this;
}
/**
* .debugpb.DB db = 1;
*/
public org.tikv.kvproto.Debugpb.DB getDb() {
org.tikv.kvproto.Debugpb.DB result = org.tikv.kvproto.Debugpb.DB.valueOf(db_);
return result == null ? org.tikv.kvproto.Debugpb.DB.UNRECOGNIZED : result;
}
/**
* .debugpb.DB db = 1;
*/
public Builder setDb(org.tikv.kvproto.Debugpb.DB value) {
if (value == null) {
throw new NullPointerException();
}
db_ = value.getNumber();
onChanged();
return this;
}
/**
* .debugpb.DB db = 1;
*/
public Builder clearDb() {
db_ = 0;
onChanged();
return this;
}
private java.lang.Object cf_ = "";
/**
* string cf = 2;
*/
public java.lang.String getCf() {
java.lang.Object ref = cf_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
cf_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* string cf = 2;
*/
public com.google.protobuf.ByteString
getCfBytes() {
java.lang.Object ref = cf_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
cf_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* string cf = 2;
*/
public Builder setCf(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
cf_ = value;
onChanged();
return this;
}
/**
* string cf = 2;
*/
public Builder clearCf() {
cf_ = getDefaultInstance().getCf();
onChanged();
return this;
}
/**
* string cf = 2;
*/
public Builder setCfBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
cf_ = value;
onChanged();
return this;
}
private com.google.protobuf.ByteString fromKey_ = com.google.protobuf.ByteString.EMPTY;
/**
* bytes from_key = 3;
*/
public com.google.protobuf.ByteString getFromKey() {
return fromKey_;
}
/**
* bytes from_key = 3;
*/
public Builder setFromKey(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
fromKey_ = value;
onChanged();
return this;
}
/**
* bytes from_key = 3;
*/
public Builder clearFromKey() {
fromKey_ = getDefaultInstance().getFromKey();
onChanged();
return this;
}
private com.google.protobuf.ByteString toKey_ = com.google.protobuf.ByteString.EMPTY;
/**
* bytes to_key = 4;
*/
public com.google.protobuf.ByteString getToKey() {
return toKey_;
}
/**
* bytes to_key = 4;
*/
public Builder setToKey(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
toKey_ = value;
onChanged();
return this;
}
/**
* bytes to_key = 4;
*/
public Builder clearToKey() {
toKey_ = getDefaultInstance().getToKey();
onChanged();
return this;
}
private int threads_ ;
/**
* uint32 threads = 5;
*/
public int getThreads() {
return threads_;
}
/**
* uint32 threads = 5;
*/
public Builder setThreads(int value) {
threads_ = value;
onChanged();
return this;
}
/**
* uint32 threads = 5;
*/
public Builder clearThreads() {
threads_ = 0;
onChanged();
return this;
}
private int bottommostLevelCompaction_ = 0;
/**
* .debugpb.BottommostLevelCompaction bottommost_level_compaction = 6;
*/
public int getBottommostLevelCompactionValue() {
return bottommostLevelCompaction_;
}
/**
* .debugpb.BottommostLevelCompaction bottommost_level_compaction = 6;
*/
public Builder setBottommostLevelCompactionValue(int value) {
bottommostLevelCompaction_ = value;
onChanged();
return this;
}
/**
* .debugpb.BottommostLevelCompaction bottommost_level_compaction = 6;
*/
public org.tikv.kvproto.Debugpb.BottommostLevelCompaction getBottommostLevelCompaction() {
org.tikv.kvproto.Debugpb.BottommostLevelCompaction result = org.tikv.kvproto.Debugpb.BottommostLevelCompaction.valueOf(bottommostLevelCompaction_);
return result == null ? org.tikv.kvproto.Debugpb.BottommostLevelCompaction.UNRECOGNIZED : result;
}
/**
* .debugpb.BottommostLevelCompaction bottommost_level_compaction = 6;
*/
public Builder setBottommostLevelCompaction(org.tikv.kvproto.Debugpb.BottommostLevelCompaction value) {
if (value == null) {
throw new NullPointerException();
}
bottommostLevelCompaction_ = value.getNumber();
onChanged();
return this;
}
/**
* .debugpb.BottommostLevelCompaction bottommost_level_compaction = 6;
*/
public Builder clearBottommostLevelCompaction() {
bottommostLevelCompaction_ = 0;
onChanged();
return this;
}
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFieldsProto3(unknownFields);
}
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:debugpb.CompactRequest)
}
// @@protoc_insertion_point(class_scope:debugpb.CompactRequest)
private static final org.tikv.kvproto.Debugpb.CompactRequest DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new org.tikv.kvproto.Debugpb.CompactRequest();
}
public static org.tikv.kvproto.Debugpb.CompactRequest getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
public CompactRequest parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new CompactRequest(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
public org.tikv.kvproto.Debugpb.CompactRequest getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface CompactResponseOrBuilder extends
// @@protoc_insertion_point(interface_extends:debugpb.CompactResponse)
com.google.protobuf.MessageOrBuilder {
}
/**
* Protobuf type {@code debugpb.CompactResponse}
*/
public static final class CompactResponse extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:debugpb.CompactResponse)
CompactResponseOrBuilder {
private static final long serialVersionUID = 0L;
// Use CompactResponse.newBuilder() to construct.
private CompactResponse(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private CompactResponse() {
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private CompactResponse(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
default: {
if (!parseUnknownFieldProto3(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.tikv.kvproto.Debugpb.internal_static_debugpb_CompactResponse_descriptor;
}
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.tikv.kvproto.Debugpb.internal_static_debugpb_CompactResponse_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.tikv.kvproto.Debugpb.CompactResponse.class, org.tikv.kvproto.Debugpb.CompactResponse.Builder.class);
}
private byte memoizedIsInitialized = -1;
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
unknownFields.writeTo(output);
}
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof org.tikv.kvproto.Debugpb.CompactResponse)) {
return super.equals(obj);
}
org.tikv.kvproto.Debugpb.CompactResponse other = (org.tikv.kvproto.Debugpb.CompactResponse) obj;
boolean result = true;
result = result && unknownFields.equals(other.unknownFields);
return result;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static org.tikv.kvproto.Debugpb.CompactResponse parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.tikv.kvproto.Debugpb.CompactResponse parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.tikv.kvproto.Debugpb.CompactResponse parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.tikv.kvproto.Debugpb.CompactResponse parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.tikv.kvproto.Debugpb.CompactResponse parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.tikv.kvproto.Debugpb.CompactResponse parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.tikv.kvproto.Debugpb.CompactResponse parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static org.tikv.kvproto.Debugpb.CompactResponse parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static org.tikv.kvproto.Debugpb.CompactResponse parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static org.tikv.kvproto.Debugpb.CompactResponse parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static org.tikv.kvproto.Debugpb.CompactResponse parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static org.tikv.kvproto.Debugpb.CompactResponse parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(org.tikv.kvproto.Debugpb.CompactResponse prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code debugpb.CompactResponse}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:debugpb.CompactResponse)
org.tikv.kvproto.Debugpb.CompactResponseOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.tikv.kvproto.Debugpb.internal_static_debugpb_CompactResponse_descriptor;
}
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.tikv.kvproto.Debugpb.internal_static_debugpb_CompactResponse_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.tikv.kvproto.Debugpb.CompactResponse.class, org.tikv.kvproto.Debugpb.CompactResponse.Builder.class);
}
// Construct using org.tikv.kvproto.Debugpb.CompactResponse.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
public Builder clear() {
super.clear();
return this;
}
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return org.tikv.kvproto.Debugpb.internal_static_debugpb_CompactResponse_descriptor;
}
public org.tikv.kvproto.Debugpb.CompactResponse getDefaultInstanceForType() {
return org.tikv.kvproto.Debugpb.CompactResponse.getDefaultInstance();
}
public org.tikv.kvproto.Debugpb.CompactResponse build() {
org.tikv.kvproto.Debugpb.CompactResponse result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
public org.tikv.kvproto.Debugpb.CompactResponse buildPartial() {
org.tikv.kvproto.Debugpb.CompactResponse result = new org.tikv.kvproto.Debugpb.CompactResponse(this);
onBuilt();
return result;
}
public Builder clone() {
return (Builder) super.clone();
}
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.setField(field, value);
}
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return (Builder) super.clearField(field);
}
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return (Builder) super.clearOneof(oneof);
}
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return (Builder) super.setRepeatedField(field, index, value);
}
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.addRepeatedField(field, value);
}
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof org.tikv.kvproto.Debugpb.CompactResponse) {
return mergeFrom((org.tikv.kvproto.Debugpb.CompactResponse)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(org.tikv.kvproto.Debugpb.CompactResponse other) {
if (other == org.tikv.kvproto.Debugpb.CompactResponse.getDefaultInstance()) return this;
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
public final boolean isInitialized() {
return true;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
org.tikv.kvproto.Debugpb.CompactResponse parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (org.tikv.kvproto.Debugpb.CompactResponse) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFieldsProto3(unknownFields);
}
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:debugpb.CompactResponse)
}
// @@protoc_insertion_point(class_scope:debugpb.CompactResponse)
private static final org.tikv.kvproto.Debugpb.CompactResponse DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new org.tikv.kvproto.Debugpb.CompactResponse();
}
public static org.tikv.kvproto.Debugpb.CompactResponse getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
public CompactResponse parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new CompactResponse(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
public org.tikv.kvproto.Debugpb.CompactResponse getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface InjectFailPointRequestOrBuilder extends
// @@protoc_insertion_point(interface_extends:debugpb.InjectFailPointRequest)
com.google.protobuf.MessageOrBuilder {
/**
* string name = 1;
*/
java.lang.String getName();
/**
* string name = 1;
*/
com.google.protobuf.ByteString
getNameBytes();
/**
* string actions = 2;
*/
java.lang.String getActions();
/**
* string actions = 2;
*/
com.google.protobuf.ByteString
getActionsBytes();
}
/**
* Protobuf type {@code debugpb.InjectFailPointRequest}
*/
public static final class InjectFailPointRequest extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:debugpb.InjectFailPointRequest)
InjectFailPointRequestOrBuilder {
private static final long serialVersionUID = 0L;
// Use InjectFailPointRequest.newBuilder() to construct.
private InjectFailPointRequest(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private InjectFailPointRequest() {
name_ = "";
actions_ = "";
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private InjectFailPointRequest(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
default: {
if (!parseUnknownFieldProto3(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
case 10: {
java.lang.String s = input.readStringRequireUtf8();
name_ = s;
break;
}
case 18: {
java.lang.String s = input.readStringRequireUtf8();
actions_ = s;
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.tikv.kvproto.Debugpb.internal_static_debugpb_InjectFailPointRequest_descriptor;
}
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.tikv.kvproto.Debugpb.internal_static_debugpb_InjectFailPointRequest_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.tikv.kvproto.Debugpb.InjectFailPointRequest.class, org.tikv.kvproto.Debugpb.InjectFailPointRequest.Builder.class);
}
public static final int NAME_FIELD_NUMBER = 1;
private volatile java.lang.Object name_;
/**
* string name = 1;
*/
public java.lang.String getName() {
java.lang.Object ref = name_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
name_ = s;
return s;
}
}
/**
* string name = 1;
*/
public com.google.protobuf.ByteString
getNameBytes() {
java.lang.Object ref = name_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
name_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int ACTIONS_FIELD_NUMBER = 2;
private volatile java.lang.Object actions_;
/**
* string actions = 2;
*/
public java.lang.String getActions() {
java.lang.Object ref = actions_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
actions_ = s;
return s;
}
}
/**
* string actions = 2;
*/
public com.google.protobuf.ByteString
getActionsBytes() {
java.lang.Object ref = actions_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
actions_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
private byte memoizedIsInitialized = -1;
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (!getNameBytes().isEmpty()) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 1, name_);
}
if (!getActionsBytes().isEmpty()) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 2, actions_);
}
unknownFields.writeTo(output);
}
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (!getNameBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(1, name_);
}
if (!getActionsBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(2, actions_);
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof org.tikv.kvproto.Debugpb.InjectFailPointRequest)) {
return super.equals(obj);
}
org.tikv.kvproto.Debugpb.InjectFailPointRequest other = (org.tikv.kvproto.Debugpb.InjectFailPointRequest) obj;
boolean result = true;
result = result && getName()
.equals(other.getName());
result = result && getActions()
.equals(other.getActions());
result = result && unknownFields.equals(other.unknownFields);
return result;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
hash = (37 * hash) + NAME_FIELD_NUMBER;
hash = (53 * hash) + getName().hashCode();
hash = (37 * hash) + ACTIONS_FIELD_NUMBER;
hash = (53 * hash) + getActions().hashCode();
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static org.tikv.kvproto.Debugpb.InjectFailPointRequest parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.tikv.kvproto.Debugpb.InjectFailPointRequest parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.tikv.kvproto.Debugpb.InjectFailPointRequest parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.tikv.kvproto.Debugpb.InjectFailPointRequest parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.tikv.kvproto.Debugpb.InjectFailPointRequest parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.tikv.kvproto.Debugpb.InjectFailPointRequest parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.tikv.kvproto.Debugpb.InjectFailPointRequest parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static org.tikv.kvproto.Debugpb.InjectFailPointRequest parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static org.tikv.kvproto.Debugpb.InjectFailPointRequest parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static org.tikv.kvproto.Debugpb.InjectFailPointRequest parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static org.tikv.kvproto.Debugpb.InjectFailPointRequest parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static org.tikv.kvproto.Debugpb.InjectFailPointRequest parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(org.tikv.kvproto.Debugpb.InjectFailPointRequest prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code debugpb.InjectFailPointRequest}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:debugpb.InjectFailPointRequest)
org.tikv.kvproto.Debugpb.InjectFailPointRequestOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.tikv.kvproto.Debugpb.internal_static_debugpb_InjectFailPointRequest_descriptor;
}
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.tikv.kvproto.Debugpb.internal_static_debugpb_InjectFailPointRequest_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.tikv.kvproto.Debugpb.InjectFailPointRequest.class, org.tikv.kvproto.Debugpb.InjectFailPointRequest.Builder.class);
}
// Construct using org.tikv.kvproto.Debugpb.InjectFailPointRequest.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
public Builder clear() {
super.clear();
name_ = "";
actions_ = "";
return this;
}
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return org.tikv.kvproto.Debugpb.internal_static_debugpb_InjectFailPointRequest_descriptor;
}
public org.tikv.kvproto.Debugpb.InjectFailPointRequest getDefaultInstanceForType() {
return org.tikv.kvproto.Debugpb.InjectFailPointRequest.getDefaultInstance();
}
public org.tikv.kvproto.Debugpb.InjectFailPointRequest build() {
org.tikv.kvproto.Debugpb.InjectFailPointRequest result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
public org.tikv.kvproto.Debugpb.InjectFailPointRequest buildPartial() {
org.tikv.kvproto.Debugpb.InjectFailPointRequest result = new org.tikv.kvproto.Debugpb.InjectFailPointRequest(this);
result.name_ = name_;
result.actions_ = actions_;
onBuilt();
return result;
}
public Builder clone() {
return (Builder) super.clone();
}
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.setField(field, value);
}
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return (Builder) super.clearField(field);
}
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return (Builder) super.clearOneof(oneof);
}
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return (Builder) super.setRepeatedField(field, index, value);
}
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.addRepeatedField(field, value);
}
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof org.tikv.kvproto.Debugpb.InjectFailPointRequest) {
return mergeFrom((org.tikv.kvproto.Debugpb.InjectFailPointRequest)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(org.tikv.kvproto.Debugpb.InjectFailPointRequest other) {
if (other == org.tikv.kvproto.Debugpb.InjectFailPointRequest.getDefaultInstance()) return this;
if (!other.getName().isEmpty()) {
name_ = other.name_;
onChanged();
}
if (!other.getActions().isEmpty()) {
actions_ = other.actions_;
onChanged();
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
public final boolean isInitialized() {
return true;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
org.tikv.kvproto.Debugpb.InjectFailPointRequest parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (org.tikv.kvproto.Debugpb.InjectFailPointRequest) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private java.lang.Object name_ = "";
/**
* string name = 1;
*/
public java.lang.String getName() {
java.lang.Object ref = name_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
name_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* string name = 1;
*/
public com.google.protobuf.ByteString
getNameBytes() {
java.lang.Object ref = name_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
name_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* string name = 1;
*/
public Builder setName(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
name_ = value;
onChanged();
return this;
}
/**
* string name = 1;
*/
public Builder clearName() {
name_ = getDefaultInstance().getName();
onChanged();
return this;
}
/**
* string name = 1;
*/
public Builder setNameBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
name_ = value;
onChanged();
return this;
}
private java.lang.Object actions_ = "";
/**
* string actions = 2;
*/
public java.lang.String getActions() {
java.lang.Object ref = actions_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
actions_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* string actions = 2;
*/
public com.google.protobuf.ByteString
getActionsBytes() {
java.lang.Object ref = actions_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
actions_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* string actions = 2;
*/
public Builder setActions(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
actions_ = value;
onChanged();
return this;
}
/**
* string actions = 2;
*/
public Builder clearActions() {
actions_ = getDefaultInstance().getActions();
onChanged();
return this;
}
/**
* string actions = 2;
*/
public Builder setActionsBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
actions_ = value;
onChanged();
return this;
}
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFieldsProto3(unknownFields);
}
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:debugpb.InjectFailPointRequest)
}
// @@protoc_insertion_point(class_scope:debugpb.InjectFailPointRequest)
private static final org.tikv.kvproto.Debugpb.InjectFailPointRequest DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new org.tikv.kvproto.Debugpb.InjectFailPointRequest();
}
public static org.tikv.kvproto.Debugpb.InjectFailPointRequest getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
public InjectFailPointRequest parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new InjectFailPointRequest(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
public org.tikv.kvproto.Debugpb.InjectFailPointRequest getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface InjectFailPointResponseOrBuilder extends
// @@protoc_insertion_point(interface_extends:debugpb.InjectFailPointResponse)
com.google.protobuf.MessageOrBuilder {
}
/**
* Protobuf type {@code debugpb.InjectFailPointResponse}
*/
public static final class InjectFailPointResponse extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:debugpb.InjectFailPointResponse)
InjectFailPointResponseOrBuilder {
private static final long serialVersionUID = 0L;
// Use InjectFailPointResponse.newBuilder() to construct.
private InjectFailPointResponse(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private InjectFailPointResponse() {
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private InjectFailPointResponse(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
default: {
if (!parseUnknownFieldProto3(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.tikv.kvproto.Debugpb.internal_static_debugpb_InjectFailPointResponse_descriptor;
}
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.tikv.kvproto.Debugpb.internal_static_debugpb_InjectFailPointResponse_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.tikv.kvproto.Debugpb.InjectFailPointResponse.class, org.tikv.kvproto.Debugpb.InjectFailPointResponse.Builder.class);
}
private byte memoizedIsInitialized = -1;
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
unknownFields.writeTo(output);
}
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof org.tikv.kvproto.Debugpb.InjectFailPointResponse)) {
return super.equals(obj);
}
org.tikv.kvproto.Debugpb.InjectFailPointResponse other = (org.tikv.kvproto.Debugpb.InjectFailPointResponse) obj;
boolean result = true;
result = result && unknownFields.equals(other.unknownFields);
return result;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static org.tikv.kvproto.Debugpb.InjectFailPointResponse parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.tikv.kvproto.Debugpb.InjectFailPointResponse parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.tikv.kvproto.Debugpb.InjectFailPointResponse parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.tikv.kvproto.Debugpb.InjectFailPointResponse parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.tikv.kvproto.Debugpb.InjectFailPointResponse parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.tikv.kvproto.Debugpb.InjectFailPointResponse parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.tikv.kvproto.Debugpb.InjectFailPointResponse parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static org.tikv.kvproto.Debugpb.InjectFailPointResponse parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static org.tikv.kvproto.Debugpb.InjectFailPointResponse parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static org.tikv.kvproto.Debugpb.InjectFailPointResponse parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static org.tikv.kvproto.Debugpb.InjectFailPointResponse parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static org.tikv.kvproto.Debugpb.InjectFailPointResponse parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(org.tikv.kvproto.Debugpb.InjectFailPointResponse prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code debugpb.InjectFailPointResponse}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:debugpb.InjectFailPointResponse)
org.tikv.kvproto.Debugpb.InjectFailPointResponseOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.tikv.kvproto.Debugpb.internal_static_debugpb_InjectFailPointResponse_descriptor;
}
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.tikv.kvproto.Debugpb.internal_static_debugpb_InjectFailPointResponse_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.tikv.kvproto.Debugpb.InjectFailPointResponse.class, org.tikv.kvproto.Debugpb.InjectFailPointResponse.Builder.class);
}
// Construct using org.tikv.kvproto.Debugpb.InjectFailPointResponse.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
public Builder clear() {
super.clear();
return this;
}
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return org.tikv.kvproto.Debugpb.internal_static_debugpb_InjectFailPointResponse_descriptor;
}
public org.tikv.kvproto.Debugpb.InjectFailPointResponse getDefaultInstanceForType() {
return org.tikv.kvproto.Debugpb.InjectFailPointResponse.getDefaultInstance();
}
public org.tikv.kvproto.Debugpb.InjectFailPointResponse build() {
org.tikv.kvproto.Debugpb.InjectFailPointResponse result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
public org.tikv.kvproto.Debugpb.InjectFailPointResponse buildPartial() {
org.tikv.kvproto.Debugpb.InjectFailPointResponse result = new org.tikv.kvproto.Debugpb.InjectFailPointResponse(this);
onBuilt();
return result;
}
public Builder clone() {
return (Builder) super.clone();
}
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.setField(field, value);
}
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return (Builder) super.clearField(field);
}
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return (Builder) super.clearOneof(oneof);
}
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return (Builder) super.setRepeatedField(field, index, value);
}
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.addRepeatedField(field, value);
}
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof org.tikv.kvproto.Debugpb.InjectFailPointResponse) {
return mergeFrom((org.tikv.kvproto.Debugpb.InjectFailPointResponse)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(org.tikv.kvproto.Debugpb.InjectFailPointResponse other) {
if (other == org.tikv.kvproto.Debugpb.InjectFailPointResponse.getDefaultInstance()) return this;
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
public final boolean isInitialized() {
return true;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
org.tikv.kvproto.Debugpb.InjectFailPointResponse parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (org.tikv.kvproto.Debugpb.InjectFailPointResponse) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFieldsProto3(unknownFields);
}
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:debugpb.InjectFailPointResponse)
}
// @@protoc_insertion_point(class_scope:debugpb.InjectFailPointResponse)
private static final org.tikv.kvproto.Debugpb.InjectFailPointResponse DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new org.tikv.kvproto.Debugpb.InjectFailPointResponse();
}
public static org.tikv.kvproto.Debugpb.InjectFailPointResponse getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
public InjectFailPointResponse parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new InjectFailPointResponse(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
public org.tikv.kvproto.Debugpb.InjectFailPointResponse getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface RecoverFailPointRequestOrBuilder extends
// @@protoc_insertion_point(interface_extends:debugpb.RecoverFailPointRequest)
com.google.protobuf.MessageOrBuilder {
/**
* string name = 1;
*/
java.lang.String getName();
/**
* string name = 1;
*/
com.google.protobuf.ByteString
getNameBytes();
}
/**
* Protobuf type {@code debugpb.RecoverFailPointRequest}
*/
public static final class RecoverFailPointRequest extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:debugpb.RecoverFailPointRequest)
RecoverFailPointRequestOrBuilder {
private static final long serialVersionUID = 0L;
// Use RecoverFailPointRequest.newBuilder() to construct.
private RecoverFailPointRequest(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private RecoverFailPointRequest() {
name_ = "";
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private RecoverFailPointRequest(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
default: {
if (!parseUnknownFieldProto3(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
case 10: {
java.lang.String s = input.readStringRequireUtf8();
name_ = s;
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.tikv.kvproto.Debugpb.internal_static_debugpb_RecoverFailPointRequest_descriptor;
}
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.tikv.kvproto.Debugpb.internal_static_debugpb_RecoverFailPointRequest_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.tikv.kvproto.Debugpb.RecoverFailPointRequest.class, org.tikv.kvproto.Debugpb.RecoverFailPointRequest.Builder.class);
}
public static final int NAME_FIELD_NUMBER = 1;
private volatile java.lang.Object name_;
/**
* string name = 1;
*/
public java.lang.String getName() {
java.lang.Object ref = name_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
name_ = s;
return s;
}
}
/**
* string name = 1;
*/
public com.google.protobuf.ByteString
getNameBytes() {
java.lang.Object ref = name_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
name_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
private byte memoizedIsInitialized = -1;
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (!getNameBytes().isEmpty()) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 1, name_);
}
unknownFields.writeTo(output);
}
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (!getNameBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(1, name_);
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof org.tikv.kvproto.Debugpb.RecoverFailPointRequest)) {
return super.equals(obj);
}
org.tikv.kvproto.Debugpb.RecoverFailPointRequest other = (org.tikv.kvproto.Debugpb.RecoverFailPointRequest) obj;
boolean result = true;
result = result && getName()
.equals(other.getName());
result = result && unknownFields.equals(other.unknownFields);
return result;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
hash = (37 * hash) + NAME_FIELD_NUMBER;
hash = (53 * hash) + getName().hashCode();
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static org.tikv.kvproto.Debugpb.RecoverFailPointRequest parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.tikv.kvproto.Debugpb.RecoverFailPointRequest parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.tikv.kvproto.Debugpb.RecoverFailPointRequest parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.tikv.kvproto.Debugpb.RecoverFailPointRequest parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.tikv.kvproto.Debugpb.RecoverFailPointRequest parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.tikv.kvproto.Debugpb.RecoverFailPointRequest parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.tikv.kvproto.Debugpb.RecoverFailPointRequest parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static org.tikv.kvproto.Debugpb.RecoverFailPointRequest parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static org.tikv.kvproto.Debugpb.RecoverFailPointRequest parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static org.tikv.kvproto.Debugpb.RecoverFailPointRequest parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static org.tikv.kvproto.Debugpb.RecoverFailPointRequest parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static org.tikv.kvproto.Debugpb.RecoverFailPointRequest parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(org.tikv.kvproto.Debugpb.RecoverFailPointRequest prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code debugpb.RecoverFailPointRequest}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:debugpb.RecoverFailPointRequest)
org.tikv.kvproto.Debugpb.RecoverFailPointRequestOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.tikv.kvproto.Debugpb.internal_static_debugpb_RecoverFailPointRequest_descriptor;
}
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.tikv.kvproto.Debugpb.internal_static_debugpb_RecoverFailPointRequest_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.tikv.kvproto.Debugpb.RecoverFailPointRequest.class, org.tikv.kvproto.Debugpb.RecoverFailPointRequest.Builder.class);
}
// Construct using org.tikv.kvproto.Debugpb.RecoverFailPointRequest.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
public Builder clear() {
super.clear();
name_ = "";
return this;
}
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return org.tikv.kvproto.Debugpb.internal_static_debugpb_RecoverFailPointRequest_descriptor;
}
public org.tikv.kvproto.Debugpb.RecoverFailPointRequest getDefaultInstanceForType() {
return org.tikv.kvproto.Debugpb.RecoverFailPointRequest.getDefaultInstance();
}
public org.tikv.kvproto.Debugpb.RecoverFailPointRequest build() {
org.tikv.kvproto.Debugpb.RecoverFailPointRequest result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
public org.tikv.kvproto.Debugpb.RecoverFailPointRequest buildPartial() {
org.tikv.kvproto.Debugpb.RecoverFailPointRequest result = new org.tikv.kvproto.Debugpb.RecoverFailPointRequest(this);
result.name_ = name_;
onBuilt();
return result;
}
public Builder clone() {
return (Builder) super.clone();
}
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.setField(field, value);
}
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return (Builder) super.clearField(field);
}
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return (Builder) super.clearOneof(oneof);
}
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return (Builder) super.setRepeatedField(field, index, value);
}
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.addRepeatedField(field, value);
}
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof org.tikv.kvproto.Debugpb.RecoverFailPointRequest) {
return mergeFrom((org.tikv.kvproto.Debugpb.RecoverFailPointRequest)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(org.tikv.kvproto.Debugpb.RecoverFailPointRequest other) {
if (other == org.tikv.kvproto.Debugpb.RecoverFailPointRequest.getDefaultInstance()) return this;
if (!other.getName().isEmpty()) {
name_ = other.name_;
onChanged();
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
public final boolean isInitialized() {
return true;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
org.tikv.kvproto.Debugpb.RecoverFailPointRequest parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (org.tikv.kvproto.Debugpb.RecoverFailPointRequest) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private java.lang.Object name_ = "";
/**
* string name = 1;
*/
public java.lang.String getName() {
java.lang.Object ref = name_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
name_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* string name = 1;
*/
public com.google.protobuf.ByteString
getNameBytes() {
java.lang.Object ref = name_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
name_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* string name = 1;
*/
public Builder setName(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
name_ = value;
onChanged();
return this;
}
/**
* string name = 1;
*/
public Builder clearName() {
name_ = getDefaultInstance().getName();
onChanged();
return this;
}
/**
* string name = 1;
*/
public Builder setNameBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
name_ = value;
onChanged();
return this;
}
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFieldsProto3(unknownFields);
}
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:debugpb.RecoverFailPointRequest)
}
// @@protoc_insertion_point(class_scope:debugpb.RecoverFailPointRequest)
private static final org.tikv.kvproto.Debugpb.RecoverFailPointRequest DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new org.tikv.kvproto.Debugpb.RecoverFailPointRequest();
}
public static org.tikv.kvproto.Debugpb.RecoverFailPointRequest getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
public RecoverFailPointRequest parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new RecoverFailPointRequest(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
public org.tikv.kvproto.Debugpb.RecoverFailPointRequest getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface RecoverFailPointResponseOrBuilder extends
// @@protoc_insertion_point(interface_extends:debugpb.RecoverFailPointResponse)
com.google.protobuf.MessageOrBuilder {
}
/**
* Protobuf type {@code debugpb.RecoverFailPointResponse}
*/
public static final class RecoverFailPointResponse extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:debugpb.RecoverFailPointResponse)
RecoverFailPointResponseOrBuilder {
private static final long serialVersionUID = 0L;
// Use RecoverFailPointResponse.newBuilder() to construct.
private RecoverFailPointResponse(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private RecoverFailPointResponse() {
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private RecoverFailPointResponse(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
default: {
if (!parseUnknownFieldProto3(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.tikv.kvproto.Debugpb.internal_static_debugpb_RecoverFailPointResponse_descriptor;
}
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.tikv.kvproto.Debugpb.internal_static_debugpb_RecoverFailPointResponse_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.tikv.kvproto.Debugpb.RecoverFailPointResponse.class, org.tikv.kvproto.Debugpb.RecoverFailPointResponse.Builder.class);
}
private byte memoizedIsInitialized = -1;
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
unknownFields.writeTo(output);
}
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof org.tikv.kvproto.Debugpb.RecoverFailPointResponse)) {
return super.equals(obj);
}
org.tikv.kvproto.Debugpb.RecoverFailPointResponse other = (org.tikv.kvproto.Debugpb.RecoverFailPointResponse) obj;
boolean result = true;
result = result && unknownFields.equals(other.unknownFields);
return result;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static org.tikv.kvproto.Debugpb.RecoverFailPointResponse parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.tikv.kvproto.Debugpb.RecoverFailPointResponse parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.tikv.kvproto.Debugpb.RecoverFailPointResponse parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.tikv.kvproto.Debugpb.RecoverFailPointResponse parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.tikv.kvproto.Debugpb.RecoverFailPointResponse parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.tikv.kvproto.Debugpb.RecoverFailPointResponse parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.tikv.kvproto.Debugpb.RecoverFailPointResponse parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static org.tikv.kvproto.Debugpb.RecoverFailPointResponse parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static org.tikv.kvproto.Debugpb.RecoverFailPointResponse parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static org.tikv.kvproto.Debugpb.RecoverFailPointResponse parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static org.tikv.kvproto.Debugpb.RecoverFailPointResponse parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static org.tikv.kvproto.Debugpb.RecoverFailPointResponse parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(org.tikv.kvproto.Debugpb.RecoverFailPointResponse prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code debugpb.RecoverFailPointResponse}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:debugpb.RecoverFailPointResponse)
org.tikv.kvproto.Debugpb.RecoverFailPointResponseOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.tikv.kvproto.Debugpb.internal_static_debugpb_RecoverFailPointResponse_descriptor;
}
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.tikv.kvproto.Debugpb.internal_static_debugpb_RecoverFailPointResponse_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.tikv.kvproto.Debugpb.RecoverFailPointResponse.class, org.tikv.kvproto.Debugpb.RecoverFailPointResponse.Builder.class);
}
// Construct using org.tikv.kvproto.Debugpb.RecoverFailPointResponse.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
public Builder clear() {
super.clear();
return this;
}
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return org.tikv.kvproto.Debugpb.internal_static_debugpb_RecoverFailPointResponse_descriptor;
}
public org.tikv.kvproto.Debugpb.RecoverFailPointResponse getDefaultInstanceForType() {
return org.tikv.kvproto.Debugpb.RecoverFailPointResponse.getDefaultInstance();
}
public org.tikv.kvproto.Debugpb.RecoverFailPointResponse build() {
org.tikv.kvproto.Debugpb.RecoverFailPointResponse result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
public org.tikv.kvproto.Debugpb.RecoverFailPointResponse buildPartial() {
org.tikv.kvproto.Debugpb.RecoverFailPointResponse result = new org.tikv.kvproto.Debugpb.RecoverFailPointResponse(this);
onBuilt();
return result;
}
public Builder clone() {
return (Builder) super.clone();
}
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.setField(field, value);
}
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return (Builder) super.clearField(field);
}
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return (Builder) super.clearOneof(oneof);
}
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return (Builder) super.setRepeatedField(field, index, value);
}
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.addRepeatedField(field, value);
}
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof org.tikv.kvproto.Debugpb.RecoverFailPointResponse) {
return mergeFrom((org.tikv.kvproto.Debugpb.RecoverFailPointResponse)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(org.tikv.kvproto.Debugpb.RecoverFailPointResponse other) {
if (other == org.tikv.kvproto.Debugpb.RecoverFailPointResponse.getDefaultInstance()) return this;
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
public final boolean isInitialized() {
return true;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
org.tikv.kvproto.Debugpb.RecoverFailPointResponse parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (org.tikv.kvproto.Debugpb.RecoverFailPointResponse) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFieldsProto3(unknownFields);
}
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:debugpb.RecoverFailPointResponse)
}
// @@protoc_insertion_point(class_scope:debugpb.RecoverFailPointResponse)
private static final org.tikv.kvproto.Debugpb.RecoverFailPointResponse DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new org.tikv.kvproto.Debugpb.RecoverFailPointResponse();
}
public static org.tikv.kvproto.Debugpb.RecoverFailPointResponse getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
public RecoverFailPointResponse parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new RecoverFailPointResponse(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
public org.tikv.kvproto.Debugpb.RecoverFailPointResponse getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface ListFailPointsRequestOrBuilder extends
// @@protoc_insertion_point(interface_extends:debugpb.ListFailPointsRequest)
com.google.protobuf.MessageOrBuilder {
}
/**
* Protobuf type {@code debugpb.ListFailPointsRequest}
*/
public static final class ListFailPointsRequest extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:debugpb.ListFailPointsRequest)
ListFailPointsRequestOrBuilder {
private static final long serialVersionUID = 0L;
// Use ListFailPointsRequest.newBuilder() to construct.
private ListFailPointsRequest(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private ListFailPointsRequest() {
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private ListFailPointsRequest(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
default: {
if (!parseUnknownFieldProto3(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.tikv.kvproto.Debugpb.internal_static_debugpb_ListFailPointsRequest_descriptor;
}
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.tikv.kvproto.Debugpb.internal_static_debugpb_ListFailPointsRequest_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.tikv.kvproto.Debugpb.ListFailPointsRequest.class, org.tikv.kvproto.Debugpb.ListFailPointsRequest.Builder.class);
}
private byte memoizedIsInitialized = -1;
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
unknownFields.writeTo(output);
}
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof org.tikv.kvproto.Debugpb.ListFailPointsRequest)) {
return super.equals(obj);
}
org.tikv.kvproto.Debugpb.ListFailPointsRequest other = (org.tikv.kvproto.Debugpb.ListFailPointsRequest) obj;
boolean result = true;
result = result && unknownFields.equals(other.unknownFields);
return result;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static org.tikv.kvproto.Debugpb.ListFailPointsRequest parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.tikv.kvproto.Debugpb.ListFailPointsRequest parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.tikv.kvproto.Debugpb.ListFailPointsRequest parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.tikv.kvproto.Debugpb.ListFailPointsRequest parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.tikv.kvproto.Debugpb.ListFailPointsRequest parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.tikv.kvproto.Debugpb.ListFailPointsRequest parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.tikv.kvproto.Debugpb.ListFailPointsRequest parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static org.tikv.kvproto.Debugpb.ListFailPointsRequest parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static org.tikv.kvproto.Debugpb.ListFailPointsRequest parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static org.tikv.kvproto.Debugpb.ListFailPointsRequest parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static org.tikv.kvproto.Debugpb.ListFailPointsRequest parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static org.tikv.kvproto.Debugpb.ListFailPointsRequest parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(org.tikv.kvproto.Debugpb.ListFailPointsRequest prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code debugpb.ListFailPointsRequest}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:debugpb.ListFailPointsRequest)
org.tikv.kvproto.Debugpb.ListFailPointsRequestOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.tikv.kvproto.Debugpb.internal_static_debugpb_ListFailPointsRequest_descriptor;
}
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.tikv.kvproto.Debugpb.internal_static_debugpb_ListFailPointsRequest_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.tikv.kvproto.Debugpb.ListFailPointsRequest.class, org.tikv.kvproto.Debugpb.ListFailPointsRequest.Builder.class);
}
// Construct using org.tikv.kvproto.Debugpb.ListFailPointsRequest.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
public Builder clear() {
super.clear();
return this;
}
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return org.tikv.kvproto.Debugpb.internal_static_debugpb_ListFailPointsRequest_descriptor;
}
public org.tikv.kvproto.Debugpb.ListFailPointsRequest getDefaultInstanceForType() {
return org.tikv.kvproto.Debugpb.ListFailPointsRequest.getDefaultInstance();
}
public org.tikv.kvproto.Debugpb.ListFailPointsRequest build() {
org.tikv.kvproto.Debugpb.ListFailPointsRequest result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
public org.tikv.kvproto.Debugpb.ListFailPointsRequest buildPartial() {
org.tikv.kvproto.Debugpb.ListFailPointsRequest result = new org.tikv.kvproto.Debugpb.ListFailPointsRequest(this);
onBuilt();
return result;
}
public Builder clone() {
return (Builder) super.clone();
}
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.setField(field, value);
}
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return (Builder) super.clearField(field);
}
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return (Builder) super.clearOneof(oneof);
}
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return (Builder) super.setRepeatedField(field, index, value);
}
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.addRepeatedField(field, value);
}
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof org.tikv.kvproto.Debugpb.ListFailPointsRequest) {
return mergeFrom((org.tikv.kvproto.Debugpb.ListFailPointsRequest)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(org.tikv.kvproto.Debugpb.ListFailPointsRequest other) {
if (other == org.tikv.kvproto.Debugpb.ListFailPointsRequest.getDefaultInstance()) return this;
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
public final boolean isInitialized() {
return true;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
org.tikv.kvproto.Debugpb.ListFailPointsRequest parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (org.tikv.kvproto.Debugpb.ListFailPointsRequest) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFieldsProto3(unknownFields);
}
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:debugpb.ListFailPointsRequest)
}
// @@protoc_insertion_point(class_scope:debugpb.ListFailPointsRequest)
private static final org.tikv.kvproto.Debugpb.ListFailPointsRequest DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new org.tikv.kvproto.Debugpb.ListFailPointsRequest();
}
public static org.tikv.kvproto.Debugpb.ListFailPointsRequest getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
public ListFailPointsRequest parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new ListFailPointsRequest(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
public org.tikv.kvproto.Debugpb.ListFailPointsRequest getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface ListFailPointsResponseOrBuilder extends
// @@protoc_insertion_point(interface_extends:debugpb.ListFailPointsResponse)
com.google.protobuf.MessageOrBuilder {
/**
* repeated .debugpb.ListFailPointsResponse.Entry entries = 1;
*/
java.util.List
getEntriesList();
/**
* repeated .debugpb.ListFailPointsResponse.Entry entries = 1;
*/
org.tikv.kvproto.Debugpb.ListFailPointsResponse.Entry getEntries(int index);
/**
* repeated .debugpb.ListFailPointsResponse.Entry entries = 1;
*/
int getEntriesCount();
/**
* repeated .debugpb.ListFailPointsResponse.Entry entries = 1;
*/
java.util.List extends org.tikv.kvproto.Debugpb.ListFailPointsResponse.EntryOrBuilder>
getEntriesOrBuilderList();
/**
* repeated .debugpb.ListFailPointsResponse.Entry entries = 1;
*/
org.tikv.kvproto.Debugpb.ListFailPointsResponse.EntryOrBuilder getEntriesOrBuilder(
int index);
}
/**
* Protobuf type {@code debugpb.ListFailPointsResponse}
*/
public static final class ListFailPointsResponse extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:debugpb.ListFailPointsResponse)
ListFailPointsResponseOrBuilder {
private static final long serialVersionUID = 0L;
// Use ListFailPointsResponse.newBuilder() to construct.
private ListFailPointsResponse(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private ListFailPointsResponse() {
entries_ = java.util.Collections.emptyList();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private ListFailPointsResponse(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
default: {
if (!parseUnknownFieldProto3(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
case 10: {
if (!((mutable_bitField0_ & 0x00000001) == 0x00000001)) {
entries_ = new java.util.ArrayList();
mutable_bitField0_ |= 0x00000001;
}
entries_.add(
input.readMessage(org.tikv.kvproto.Debugpb.ListFailPointsResponse.Entry.parser(), extensionRegistry));
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
if (((mutable_bitField0_ & 0x00000001) == 0x00000001)) {
entries_ = java.util.Collections.unmodifiableList(entries_);
}
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.tikv.kvproto.Debugpb.internal_static_debugpb_ListFailPointsResponse_descriptor;
}
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.tikv.kvproto.Debugpb.internal_static_debugpb_ListFailPointsResponse_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.tikv.kvproto.Debugpb.ListFailPointsResponse.class, org.tikv.kvproto.Debugpb.ListFailPointsResponse.Builder.class);
}
public interface EntryOrBuilder extends
// @@protoc_insertion_point(interface_extends:debugpb.ListFailPointsResponse.Entry)
com.google.protobuf.MessageOrBuilder {
/**
* string name = 1;
*/
java.lang.String getName();
/**
* string name = 1;
*/
com.google.protobuf.ByteString
getNameBytes();
/**
* string actions = 2;
*/
java.lang.String getActions();
/**
* string actions = 2;
*/
com.google.protobuf.ByteString
getActionsBytes();
}
/**
* Protobuf type {@code debugpb.ListFailPointsResponse.Entry}
*/
public static final class Entry extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:debugpb.ListFailPointsResponse.Entry)
EntryOrBuilder {
private static final long serialVersionUID = 0L;
// Use Entry.newBuilder() to construct.
private Entry(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private Entry() {
name_ = "";
actions_ = "";
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private Entry(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
default: {
if (!parseUnknownFieldProto3(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
case 10: {
java.lang.String s = input.readStringRequireUtf8();
name_ = s;
break;
}
case 18: {
java.lang.String s = input.readStringRequireUtf8();
actions_ = s;
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.tikv.kvproto.Debugpb.internal_static_debugpb_ListFailPointsResponse_Entry_descriptor;
}
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.tikv.kvproto.Debugpb.internal_static_debugpb_ListFailPointsResponse_Entry_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.tikv.kvproto.Debugpb.ListFailPointsResponse.Entry.class, org.tikv.kvproto.Debugpb.ListFailPointsResponse.Entry.Builder.class);
}
public static final int NAME_FIELD_NUMBER = 1;
private volatile java.lang.Object name_;
/**
* string name = 1;
*/
public java.lang.String getName() {
java.lang.Object ref = name_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
name_ = s;
return s;
}
}
/**
* string name = 1;
*/
public com.google.protobuf.ByteString
getNameBytes() {
java.lang.Object ref = name_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
name_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int ACTIONS_FIELD_NUMBER = 2;
private volatile java.lang.Object actions_;
/**
* string actions = 2;
*/
public java.lang.String getActions() {
java.lang.Object ref = actions_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
actions_ = s;
return s;
}
}
/**
* string actions = 2;
*/
public com.google.protobuf.ByteString
getActionsBytes() {
java.lang.Object ref = actions_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
actions_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
private byte memoizedIsInitialized = -1;
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (!getNameBytes().isEmpty()) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 1, name_);
}
if (!getActionsBytes().isEmpty()) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 2, actions_);
}
unknownFields.writeTo(output);
}
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (!getNameBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(1, name_);
}
if (!getActionsBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(2, actions_);
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof org.tikv.kvproto.Debugpb.ListFailPointsResponse.Entry)) {
return super.equals(obj);
}
org.tikv.kvproto.Debugpb.ListFailPointsResponse.Entry other = (org.tikv.kvproto.Debugpb.ListFailPointsResponse.Entry) obj;
boolean result = true;
result = result && getName()
.equals(other.getName());
result = result && getActions()
.equals(other.getActions());
result = result && unknownFields.equals(other.unknownFields);
return result;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
hash = (37 * hash) + NAME_FIELD_NUMBER;
hash = (53 * hash) + getName().hashCode();
hash = (37 * hash) + ACTIONS_FIELD_NUMBER;
hash = (53 * hash) + getActions().hashCode();
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static org.tikv.kvproto.Debugpb.ListFailPointsResponse.Entry parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.tikv.kvproto.Debugpb.ListFailPointsResponse.Entry parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.tikv.kvproto.Debugpb.ListFailPointsResponse.Entry parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.tikv.kvproto.Debugpb.ListFailPointsResponse.Entry parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.tikv.kvproto.Debugpb.ListFailPointsResponse.Entry parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.tikv.kvproto.Debugpb.ListFailPointsResponse.Entry parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.tikv.kvproto.Debugpb.ListFailPointsResponse.Entry parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static org.tikv.kvproto.Debugpb.ListFailPointsResponse.Entry parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static org.tikv.kvproto.Debugpb.ListFailPointsResponse.Entry parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static org.tikv.kvproto.Debugpb.ListFailPointsResponse.Entry parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static org.tikv.kvproto.Debugpb.ListFailPointsResponse.Entry parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static org.tikv.kvproto.Debugpb.ListFailPointsResponse.Entry parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(org.tikv.kvproto.Debugpb.ListFailPointsResponse.Entry prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code debugpb.ListFailPointsResponse.Entry}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:debugpb.ListFailPointsResponse.Entry)
org.tikv.kvproto.Debugpb.ListFailPointsResponse.EntryOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.tikv.kvproto.Debugpb.internal_static_debugpb_ListFailPointsResponse_Entry_descriptor;
}
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.tikv.kvproto.Debugpb.internal_static_debugpb_ListFailPointsResponse_Entry_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.tikv.kvproto.Debugpb.ListFailPointsResponse.Entry.class, org.tikv.kvproto.Debugpb.ListFailPointsResponse.Entry.Builder.class);
}
// Construct using org.tikv.kvproto.Debugpb.ListFailPointsResponse.Entry.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
public Builder clear() {
super.clear();
name_ = "";
actions_ = "";
return this;
}
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return org.tikv.kvproto.Debugpb.internal_static_debugpb_ListFailPointsResponse_Entry_descriptor;
}
public org.tikv.kvproto.Debugpb.ListFailPointsResponse.Entry getDefaultInstanceForType() {
return org.tikv.kvproto.Debugpb.ListFailPointsResponse.Entry.getDefaultInstance();
}
public org.tikv.kvproto.Debugpb.ListFailPointsResponse.Entry build() {
org.tikv.kvproto.Debugpb.ListFailPointsResponse.Entry result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
public org.tikv.kvproto.Debugpb.ListFailPointsResponse.Entry buildPartial() {
org.tikv.kvproto.Debugpb.ListFailPointsResponse.Entry result = new org.tikv.kvproto.Debugpb.ListFailPointsResponse.Entry(this);
result.name_ = name_;
result.actions_ = actions_;
onBuilt();
return result;
}
public Builder clone() {
return (Builder) super.clone();
}
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.setField(field, value);
}
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return (Builder) super.clearField(field);
}
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return (Builder) super.clearOneof(oneof);
}
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return (Builder) super.setRepeatedField(field, index, value);
}
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.addRepeatedField(field, value);
}
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof org.tikv.kvproto.Debugpb.ListFailPointsResponse.Entry) {
return mergeFrom((org.tikv.kvproto.Debugpb.ListFailPointsResponse.Entry)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(org.tikv.kvproto.Debugpb.ListFailPointsResponse.Entry other) {
if (other == org.tikv.kvproto.Debugpb.ListFailPointsResponse.Entry.getDefaultInstance()) return this;
if (!other.getName().isEmpty()) {
name_ = other.name_;
onChanged();
}
if (!other.getActions().isEmpty()) {
actions_ = other.actions_;
onChanged();
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
public final boolean isInitialized() {
return true;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
org.tikv.kvproto.Debugpb.ListFailPointsResponse.Entry parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (org.tikv.kvproto.Debugpb.ListFailPointsResponse.Entry) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private java.lang.Object name_ = "";
/**
* string name = 1;
*/
public java.lang.String getName() {
java.lang.Object ref = name_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
name_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* string name = 1;
*/
public com.google.protobuf.ByteString
getNameBytes() {
java.lang.Object ref = name_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
name_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* string name = 1;
*/
public Builder setName(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
name_ = value;
onChanged();
return this;
}
/**
* string name = 1;
*/
public Builder clearName() {
name_ = getDefaultInstance().getName();
onChanged();
return this;
}
/**
* string name = 1;
*/
public Builder setNameBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
name_ = value;
onChanged();
return this;
}
private java.lang.Object actions_ = "";
/**
* string actions = 2;
*/
public java.lang.String getActions() {
java.lang.Object ref = actions_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
actions_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* string actions = 2;
*/
public com.google.protobuf.ByteString
getActionsBytes() {
java.lang.Object ref = actions_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
actions_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* string actions = 2;
*/
public Builder setActions(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
actions_ = value;
onChanged();
return this;
}
/**
* string actions = 2;
*/
public Builder clearActions() {
actions_ = getDefaultInstance().getActions();
onChanged();
return this;
}
/**
* string actions = 2;
*/
public Builder setActionsBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
actions_ = value;
onChanged();
return this;
}
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFieldsProto3(unknownFields);
}
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:debugpb.ListFailPointsResponse.Entry)
}
// @@protoc_insertion_point(class_scope:debugpb.ListFailPointsResponse.Entry)
private static final org.tikv.kvproto.Debugpb.ListFailPointsResponse.Entry DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new org.tikv.kvproto.Debugpb.ListFailPointsResponse.Entry();
}
public static org.tikv.kvproto.Debugpb.ListFailPointsResponse.Entry getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
public Entry parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new Entry(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
public org.tikv.kvproto.Debugpb.ListFailPointsResponse.Entry getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public static final int ENTRIES_FIELD_NUMBER = 1;
private java.util.List entries_;
/**
* repeated .debugpb.ListFailPointsResponse.Entry entries = 1;
*/
public java.util.List getEntriesList() {
return entries_;
}
/**
* repeated .debugpb.ListFailPointsResponse.Entry entries = 1;
*/
public java.util.List extends org.tikv.kvproto.Debugpb.ListFailPointsResponse.EntryOrBuilder>
getEntriesOrBuilderList() {
return entries_;
}
/**
* repeated .debugpb.ListFailPointsResponse.Entry entries = 1;
*/
public int getEntriesCount() {
return entries_.size();
}
/**
* repeated .debugpb.ListFailPointsResponse.Entry entries = 1;
*/
public org.tikv.kvproto.Debugpb.ListFailPointsResponse.Entry getEntries(int index) {
return entries_.get(index);
}
/**
* repeated .debugpb.ListFailPointsResponse.Entry entries = 1;
*/
public org.tikv.kvproto.Debugpb.ListFailPointsResponse.EntryOrBuilder getEntriesOrBuilder(
int index) {
return entries_.get(index);
}
private byte memoizedIsInitialized = -1;
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
for (int i = 0; i < entries_.size(); i++) {
output.writeMessage(1, entries_.get(i));
}
unknownFields.writeTo(output);
}
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
for (int i = 0; i < entries_.size(); i++) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(1, entries_.get(i));
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof org.tikv.kvproto.Debugpb.ListFailPointsResponse)) {
return super.equals(obj);
}
org.tikv.kvproto.Debugpb.ListFailPointsResponse other = (org.tikv.kvproto.Debugpb.ListFailPointsResponse) obj;
boolean result = true;
result = result && getEntriesList()
.equals(other.getEntriesList());
result = result && unknownFields.equals(other.unknownFields);
return result;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (getEntriesCount() > 0) {
hash = (37 * hash) + ENTRIES_FIELD_NUMBER;
hash = (53 * hash) + getEntriesList().hashCode();
}
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static org.tikv.kvproto.Debugpb.ListFailPointsResponse parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.tikv.kvproto.Debugpb.ListFailPointsResponse parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.tikv.kvproto.Debugpb.ListFailPointsResponse parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.tikv.kvproto.Debugpb.ListFailPointsResponse parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.tikv.kvproto.Debugpb.ListFailPointsResponse parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.tikv.kvproto.Debugpb.ListFailPointsResponse parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.tikv.kvproto.Debugpb.ListFailPointsResponse parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static org.tikv.kvproto.Debugpb.ListFailPointsResponse parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static org.tikv.kvproto.Debugpb.ListFailPointsResponse parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static org.tikv.kvproto.Debugpb.ListFailPointsResponse parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static org.tikv.kvproto.Debugpb.ListFailPointsResponse parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static org.tikv.kvproto.Debugpb.ListFailPointsResponse parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(org.tikv.kvproto.Debugpb.ListFailPointsResponse prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code debugpb.ListFailPointsResponse}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:debugpb.ListFailPointsResponse)
org.tikv.kvproto.Debugpb.ListFailPointsResponseOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.tikv.kvproto.Debugpb.internal_static_debugpb_ListFailPointsResponse_descriptor;
}
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.tikv.kvproto.Debugpb.internal_static_debugpb_ListFailPointsResponse_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.tikv.kvproto.Debugpb.ListFailPointsResponse.class, org.tikv.kvproto.Debugpb.ListFailPointsResponse.Builder.class);
}
// Construct using org.tikv.kvproto.Debugpb.ListFailPointsResponse.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
getEntriesFieldBuilder();
}
}
public Builder clear() {
super.clear();
if (entriesBuilder_ == null) {
entries_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000001);
} else {
entriesBuilder_.clear();
}
return this;
}
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return org.tikv.kvproto.Debugpb.internal_static_debugpb_ListFailPointsResponse_descriptor;
}
public org.tikv.kvproto.Debugpb.ListFailPointsResponse getDefaultInstanceForType() {
return org.tikv.kvproto.Debugpb.ListFailPointsResponse.getDefaultInstance();
}
public org.tikv.kvproto.Debugpb.ListFailPointsResponse build() {
org.tikv.kvproto.Debugpb.ListFailPointsResponse result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
public org.tikv.kvproto.Debugpb.ListFailPointsResponse buildPartial() {
org.tikv.kvproto.Debugpb.ListFailPointsResponse result = new org.tikv.kvproto.Debugpb.ListFailPointsResponse(this);
int from_bitField0_ = bitField0_;
if (entriesBuilder_ == null) {
if (((bitField0_ & 0x00000001) == 0x00000001)) {
entries_ = java.util.Collections.unmodifiableList(entries_);
bitField0_ = (bitField0_ & ~0x00000001);
}
result.entries_ = entries_;
} else {
result.entries_ = entriesBuilder_.build();
}
onBuilt();
return result;
}
public Builder clone() {
return (Builder) super.clone();
}
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.setField(field, value);
}
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return (Builder) super.clearField(field);
}
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return (Builder) super.clearOneof(oneof);
}
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return (Builder) super.setRepeatedField(field, index, value);
}
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.addRepeatedField(field, value);
}
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof org.tikv.kvproto.Debugpb.ListFailPointsResponse) {
return mergeFrom((org.tikv.kvproto.Debugpb.ListFailPointsResponse)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(org.tikv.kvproto.Debugpb.ListFailPointsResponse other) {
if (other == org.tikv.kvproto.Debugpb.ListFailPointsResponse.getDefaultInstance()) return this;
if (entriesBuilder_ == null) {
if (!other.entries_.isEmpty()) {
if (entries_.isEmpty()) {
entries_ = other.entries_;
bitField0_ = (bitField0_ & ~0x00000001);
} else {
ensureEntriesIsMutable();
entries_.addAll(other.entries_);
}
onChanged();
}
} else {
if (!other.entries_.isEmpty()) {
if (entriesBuilder_.isEmpty()) {
entriesBuilder_.dispose();
entriesBuilder_ = null;
entries_ = other.entries_;
bitField0_ = (bitField0_ & ~0x00000001);
entriesBuilder_ =
com.google.protobuf.GeneratedMessageV3.alwaysUseFieldBuilders ?
getEntriesFieldBuilder() : null;
} else {
entriesBuilder_.addAllMessages(other.entries_);
}
}
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
public final boolean isInitialized() {
return true;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
org.tikv.kvproto.Debugpb.ListFailPointsResponse parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (org.tikv.kvproto.Debugpb.ListFailPointsResponse) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
private java.util.List entries_ =
java.util.Collections.emptyList();
private void ensureEntriesIsMutable() {
if (!((bitField0_ & 0x00000001) == 0x00000001)) {
entries_ = new java.util.ArrayList(entries_);
bitField0_ |= 0x00000001;
}
}
private com.google.protobuf.RepeatedFieldBuilderV3<
org.tikv.kvproto.Debugpb.ListFailPointsResponse.Entry, org.tikv.kvproto.Debugpb.ListFailPointsResponse.Entry.Builder, org.tikv.kvproto.Debugpb.ListFailPointsResponse.EntryOrBuilder> entriesBuilder_;
/**
* repeated .debugpb.ListFailPointsResponse.Entry entries = 1;
*/
public java.util.List getEntriesList() {
if (entriesBuilder_ == null) {
return java.util.Collections.unmodifiableList(entries_);
} else {
return entriesBuilder_.getMessageList();
}
}
/**
* repeated .debugpb.ListFailPointsResponse.Entry entries = 1;
*/
public int getEntriesCount() {
if (entriesBuilder_ == null) {
return entries_.size();
} else {
return entriesBuilder_.getCount();
}
}
/**
* repeated .debugpb.ListFailPointsResponse.Entry entries = 1;
*/
public org.tikv.kvproto.Debugpb.ListFailPointsResponse.Entry getEntries(int index) {
if (entriesBuilder_ == null) {
return entries_.get(index);
} else {
return entriesBuilder_.getMessage(index);
}
}
/**
* repeated .debugpb.ListFailPointsResponse.Entry entries = 1;
*/
public Builder setEntries(
int index, org.tikv.kvproto.Debugpb.ListFailPointsResponse.Entry value) {
if (entriesBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureEntriesIsMutable();
entries_.set(index, value);
onChanged();
} else {
entriesBuilder_.setMessage(index, value);
}
return this;
}
/**
* repeated .debugpb.ListFailPointsResponse.Entry entries = 1;
*/
public Builder setEntries(
int index, org.tikv.kvproto.Debugpb.ListFailPointsResponse.Entry.Builder builderForValue) {
if (entriesBuilder_ == null) {
ensureEntriesIsMutable();
entries_.set(index, builderForValue.build());
onChanged();
} else {
entriesBuilder_.setMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .debugpb.ListFailPointsResponse.Entry entries = 1;
*/
public Builder addEntries(org.tikv.kvproto.Debugpb.ListFailPointsResponse.Entry value) {
if (entriesBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureEntriesIsMutable();
entries_.add(value);
onChanged();
} else {
entriesBuilder_.addMessage(value);
}
return this;
}
/**
* repeated .debugpb.ListFailPointsResponse.Entry entries = 1;
*/
public Builder addEntries(
int index, org.tikv.kvproto.Debugpb.ListFailPointsResponse.Entry value) {
if (entriesBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureEntriesIsMutable();
entries_.add(index, value);
onChanged();
} else {
entriesBuilder_.addMessage(index, value);
}
return this;
}
/**
* repeated .debugpb.ListFailPointsResponse.Entry entries = 1;
*/
public Builder addEntries(
org.tikv.kvproto.Debugpb.ListFailPointsResponse.Entry.Builder builderForValue) {
if (entriesBuilder_ == null) {
ensureEntriesIsMutable();
entries_.add(builderForValue.build());
onChanged();
} else {
entriesBuilder_.addMessage(builderForValue.build());
}
return this;
}
/**
* repeated .debugpb.ListFailPointsResponse.Entry entries = 1;
*/
public Builder addEntries(
int index, org.tikv.kvproto.Debugpb.ListFailPointsResponse.Entry.Builder builderForValue) {
if (entriesBuilder_ == null) {
ensureEntriesIsMutable();
entries_.add(index, builderForValue.build());
onChanged();
} else {
entriesBuilder_.addMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .debugpb.ListFailPointsResponse.Entry entries = 1;
*/
public Builder addAllEntries(
java.lang.Iterable extends org.tikv.kvproto.Debugpb.ListFailPointsResponse.Entry> values) {
if (entriesBuilder_ == null) {
ensureEntriesIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, entries_);
onChanged();
} else {
entriesBuilder_.addAllMessages(values);
}
return this;
}
/**
* repeated .debugpb.ListFailPointsResponse.Entry entries = 1;
*/
public Builder clearEntries() {
if (entriesBuilder_ == null) {
entries_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000001);
onChanged();
} else {
entriesBuilder_.clear();
}
return this;
}
/**
* repeated .debugpb.ListFailPointsResponse.Entry entries = 1;
*/
public Builder removeEntries(int index) {
if (entriesBuilder_ == null) {
ensureEntriesIsMutable();
entries_.remove(index);
onChanged();
} else {
entriesBuilder_.remove(index);
}
return this;
}
/**
* repeated .debugpb.ListFailPointsResponse.Entry entries = 1;
*/
public org.tikv.kvproto.Debugpb.ListFailPointsResponse.Entry.Builder getEntriesBuilder(
int index) {
return getEntriesFieldBuilder().getBuilder(index);
}
/**
* repeated .debugpb.ListFailPointsResponse.Entry entries = 1;
*/
public org.tikv.kvproto.Debugpb.ListFailPointsResponse.EntryOrBuilder getEntriesOrBuilder(
int index) {
if (entriesBuilder_ == null) {
return entries_.get(index); } else {
return entriesBuilder_.getMessageOrBuilder(index);
}
}
/**
* repeated .debugpb.ListFailPointsResponse.Entry entries = 1;
*/
public java.util.List extends org.tikv.kvproto.Debugpb.ListFailPointsResponse.EntryOrBuilder>
getEntriesOrBuilderList() {
if (entriesBuilder_ != null) {
return entriesBuilder_.getMessageOrBuilderList();
} else {
return java.util.Collections.unmodifiableList(entries_);
}
}
/**
* repeated .debugpb.ListFailPointsResponse.Entry entries = 1;
*/
public org.tikv.kvproto.Debugpb.ListFailPointsResponse.Entry.Builder addEntriesBuilder() {
return getEntriesFieldBuilder().addBuilder(
org.tikv.kvproto.Debugpb.ListFailPointsResponse.Entry.getDefaultInstance());
}
/**
* repeated .debugpb.ListFailPointsResponse.Entry entries = 1;
*/
public org.tikv.kvproto.Debugpb.ListFailPointsResponse.Entry.Builder addEntriesBuilder(
int index) {
return getEntriesFieldBuilder().addBuilder(
index, org.tikv.kvproto.Debugpb.ListFailPointsResponse.Entry.getDefaultInstance());
}
/**
* repeated .debugpb.ListFailPointsResponse.Entry entries = 1;
*/
public java.util.List
getEntriesBuilderList() {
return getEntriesFieldBuilder().getBuilderList();
}
private com.google.protobuf.RepeatedFieldBuilderV3<
org.tikv.kvproto.Debugpb.ListFailPointsResponse.Entry, org.tikv.kvproto.Debugpb.ListFailPointsResponse.Entry.Builder, org.tikv.kvproto.Debugpb.ListFailPointsResponse.EntryOrBuilder>
getEntriesFieldBuilder() {
if (entriesBuilder_ == null) {
entriesBuilder_ = new com.google.protobuf.RepeatedFieldBuilderV3<
org.tikv.kvproto.Debugpb.ListFailPointsResponse.Entry, org.tikv.kvproto.Debugpb.ListFailPointsResponse.Entry.Builder, org.tikv.kvproto.Debugpb.ListFailPointsResponse.EntryOrBuilder>(
entries_,
((bitField0_ & 0x00000001) == 0x00000001),
getParentForChildren(),
isClean());
entries_ = null;
}
return entriesBuilder_;
}
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFieldsProto3(unknownFields);
}
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:debugpb.ListFailPointsResponse)
}
// @@protoc_insertion_point(class_scope:debugpb.ListFailPointsResponse)
private static final org.tikv.kvproto.Debugpb.ListFailPointsResponse DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new org.tikv.kvproto.Debugpb.ListFailPointsResponse();
}
public static org.tikv.kvproto.Debugpb.ListFailPointsResponse getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
public ListFailPointsResponse parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new ListFailPointsResponse(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
public org.tikv.kvproto.Debugpb.ListFailPointsResponse getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface GetMetricsRequestOrBuilder extends
// @@protoc_insertion_point(interface_extends:debugpb.GetMetricsRequest)
com.google.protobuf.MessageOrBuilder {
/**
* bool all = 1;
*/
boolean getAll();
}
/**
* Protobuf type {@code debugpb.GetMetricsRequest}
*/
public static final class GetMetricsRequest extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:debugpb.GetMetricsRequest)
GetMetricsRequestOrBuilder {
private static final long serialVersionUID = 0L;
// Use GetMetricsRequest.newBuilder() to construct.
private GetMetricsRequest(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private GetMetricsRequest() {
all_ = false;
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private GetMetricsRequest(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
default: {
if (!parseUnknownFieldProto3(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
case 8: {
all_ = input.readBool();
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.tikv.kvproto.Debugpb.internal_static_debugpb_GetMetricsRequest_descriptor;
}
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.tikv.kvproto.Debugpb.internal_static_debugpb_GetMetricsRequest_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.tikv.kvproto.Debugpb.GetMetricsRequest.class, org.tikv.kvproto.Debugpb.GetMetricsRequest.Builder.class);
}
public static final int ALL_FIELD_NUMBER = 1;
private boolean all_;
/**
* bool all = 1;
*/
public boolean getAll() {
return all_;
}
private byte memoizedIsInitialized = -1;
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (all_ != false) {
output.writeBool(1, all_);
}
unknownFields.writeTo(output);
}
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (all_ != false) {
size += com.google.protobuf.CodedOutputStream
.computeBoolSize(1, all_);
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof org.tikv.kvproto.Debugpb.GetMetricsRequest)) {
return super.equals(obj);
}
org.tikv.kvproto.Debugpb.GetMetricsRequest other = (org.tikv.kvproto.Debugpb.GetMetricsRequest) obj;
boolean result = true;
result = result && (getAll()
== other.getAll());
result = result && unknownFields.equals(other.unknownFields);
return result;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
hash = (37 * hash) + ALL_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashBoolean(
getAll());
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static org.tikv.kvproto.Debugpb.GetMetricsRequest parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.tikv.kvproto.Debugpb.GetMetricsRequest parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.tikv.kvproto.Debugpb.GetMetricsRequest parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.tikv.kvproto.Debugpb.GetMetricsRequest parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.tikv.kvproto.Debugpb.GetMetricsRequest parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.tikv.kvproto.Debugpb.GetMetricsRequest parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.tikv.kvproto.Debugpb.GetMetricsRequest parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static org.tikv.kvproto.Debugpb.GetMetricsRequest parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static org.tikv.kvproto.Debugpb.GetMetricsRequest parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static org.tikv.kvproto.Debugpb.GetMetricsRequest parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static org.tikv.kvproto.Debugpb.GetMetricsRequest parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static org.tikv.kvproto.Debugpb.GetMetricsRequest parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(org.tikv.kvproto.Debugpb.GetMetricsRequest prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code debugpb.GetMetricsRequest}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:debugpb.GetMetricsRequest)
org.tikv.kvproto.Debugpb.GetMetricsRequestOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.tikv.kvproto.Debugpb.internal_static_debugpb_GetMetricsRequest_descriptor;
}
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.tikv.kvproto.Debugpb.internal_static_debugpb_GetMetricsRequest_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.tikv.kvproto.Debugpb.GetMetricsRequest.class, org.tikv.kvproto.Debugpb.GetMetricsRequest.Builder.class);
}
// Construct using org.tikv.kvproto.Debugpb.GetMetricsRequest.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
public Builder clear() {
super.clear();
all_ = false;
return this;
}
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return org.tikv.kvproto.Debugpb.internal_static_debugpb_GetMetricsRequest_descriptor;
}
public org.tikv.kvproto.Debugpb.GetMetricsRequest getDefaultInstanceForType() {
return org.tikv.kvproto.Debugpb.GetMetricsRequest.getDefaultInstance();
}
public org.tikv.kvproto.Debugpb.GetMetricsRequest build() {
org.tikv.kvproto.Debugpb.GetMetricsRequest result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
public org.tikv.kvproto.Debugpb.GetMetricsRequest buildPartial() {
org.tikv.kvproto.Debugpb.GetMetricsRequest result = new org.tikv.kvproto.Debugpb.GetMetricsRequest(this);
result.all_ = all_;
onBuilt();
return result;
}
public Builder clone() {
return (Builder) super.clone();
}
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.setField(field, value);
}
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return (Builder) super.clearField(field);
}
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return (Builder) super.clearOneof(oneof);
}
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return (Builder) super.setRepeatedField(field, index, value);
}
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.addRepeatedField(field, value);
}
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof org.tikv.kvproto.Debugpb.GetMetricsRequest) {
return mergeFrom((org.tikv.kvproto.Debugpb.GetMetricsRequest)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(org.tikv.kvproto.Debugpb.GetMetricsRequest other) {
if (other == org.tikv.kvproto.Debugpb.GetMetricsRequest.getDefaultInstance()) return this;
if (other.getAll() != false) {
setAll(other.getAll());
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
public final boolean isInitialized() {
return true;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
org.tikv.kvproto.Debugpb.GetMetricsRequest parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (org.tikv.kvproto.Debugpb.GetMetricsRequest) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private boolean all_ ;
/**
* bool all = 1;
*/
public boolean getAll() {
return all_;
}
/**
* bool all = 1;
*/
public Builder setAll(boolean value) {
all_ = value;
onChanged();
return this;
}
/**
* bool all = 1;
*/
public Builder clearAll() {
all_ = false;
onChanged();
return this;
}
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFieldsProto3(unknownFields);
}
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:debugpb.GetMetricsRequest)
}
// @@protoc_insertion_point(class_scope:debugpb.GetMetricsRequest)
private static final org.tikv.kvproto.Debugpb.GetMetricsRequest DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new org.tikv.kvproto.Debugpb.GetMetricsRequest();
}
public static org.tikv.kvproto.Debugpb.GetMetricsRequest getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
public GetMetricsRequest parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new GetMetricsRequest(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
public org.tikv.kvproto.Debugpb.GetMetricsRequest getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface GetMetricsResponseOrBuilder extends
// @@protoc_insertion_point(interface_extends:debugpb.GetMetricsResponse)
com.google.protobuf.MessageOrBuilder {
/**
* string prometheus = 1;
*/
java.lang.String getPrometheus();
/**
* string prometheus = 1;
*/
com.google.protobuf.ByteString
getPrometheusBytes();
/**
* string rocksdb_kv = 2;
*/
java.lang.String getRocksdbKv();
/**
* string rocksdb_kv = 2;
*/
com.google.protobuf.ByteString
getRocksdbKvBytes();
/**
* string rocksdb_raft = 3;
*/
java.lang.String getRocksdbRaft();
/**
* string rocksdb_raft = 3;
*/
com.google.protobuf.ByteString
getRocksdbRaftBytes();
/**
* string jemalloc = 4;
*/
java.lang.String getJemalloc();
/**
* string jemalloc = 4;
*/
com.google.protobuf.ByteString
getJemallocBytes();
/**
* uint64 store_id = 5;
*/
long getStoreId();
}
/**
* Protobuf type {@code debugpb.GetMetricsResponse}
*/
public static final class GetMetricsResponse extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:debugpb.GetMetricsResponse)
GetMetricsResponseOrBuilder {
private static final long serialVersionUID = 0L;
// Use GetMetricsResponse.newBuilder() to construct.
private GetMetricsResponse(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private GetMetricsResponse() {
prometheus_ = "";
rocksdbKv_ = "";
rocksdbRaft_ = "";
jemalloc_ = "";
storeId_ = 0L;
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private GetMetricsResponse(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
default: {
if (!parseUnknownFieldProto3(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
case 10: {
java.lang.String s = input.readStringRequireUtf8();
prometheus_ = s;
break;
}
case 18: {
java.lang.String s = input.readStringRequireUtf8();
rocksdbKv_ = s;
break;
}
case 26: {
java.lang.String s = input.readStringRequireUtf8();
rocksdbRaft_ = s;
break;
}
case 34: {
java.lang.String s = input.readStringRequireUtf8();
jemalloc_ = s;
break;
}
case 40: {
storeId_ = input.readUInt64();
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.tikv.kvproto.Debugpb.internal_static_debugpb_GetMetricsResponse_descriptor;
}
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.tikv.kvproto.Debugpb.internal_static_debugpb_GetMetricsResponse_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.tikv.kvproto.Debugpb.GetMetricsResponse.class, org.tikv.kvproto.Debugpb.GetMetricsResponse.Builder.class);
}
public static final int PROMETHEUS_FIELD_NUMBER = 1;
private volatile java.lang.Object prometheus_;
/**
* string prometheus = 1;
*/
public java.lang.String getPrometheus() {
java.lang.Object ref = prometheus_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
prometheus_ = s;
return s;
}
}
/**
* string prometheus = 1;
*/
public com.google.protobuf.ByteString
getPrometheusBytes() {
java.lang.Object ref = prometheus_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
prometheus_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int ROCKSDB_KV_FIELD_NUMBER = 2;
private volatile java.lang.Object rocksdbKv_;
/**
* string rocksdb_kv = 2;
*/
public java.lang.String getRocksdbKv() {
java.lang.Object ref = rocksdbKv_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
rocksdbKv_ = s;
return s;
}
}
/**
* string rocksdb_kv = 2;
*/
public com.google.protobuf.ByteString
getRocksdbKvBytes() {
java.lang.Object ref = rocksdbKv_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
rocksdbKv_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int ROCKSDB_RAFT_FIELD_NUMBER = 3;
private volatile java.lang.Object rocksdbRaft_;
/**
* string rocksdb_raft = 3;
*/
public java.lang.String getRocksdbRaft() {
java.lang.Object ref = rocksdbRaft_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
rocksdbRaft_ = s;
return s;
}
}
/**
* string rocksdb_raft = 3;
*/
public com.google.protobuf.ByteString
getRocksdbRaftBytes() {
java.lang.Object ref = rocksdbRaft_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
rocksdbRaft_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int JEMALLOC_FIELD_NUMBER = 4;
private volatile java.lang.Object jemalloc_;
/**
* string jemalloc = 4;
*/
public java.lang.String getJemalloc() {
java.lang.Object ref = jemalloc_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
jemalloc_ = s;
return s;
}
}
/**
* string jemalloc = 4;
*/
public com.google.protobuf.ByteString
getJemallocBytes() {
java.lang.Object ref = jemalloc_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
jemalloc_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int STORE_ID_FIELD_NUMBER = 5;
private long storeId_;
/**
* uint64 store_id = 5;
*/
public long getStoreId() {
return storeId_;
}
private byte memoizedIsInitialized = -1;
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (!getPrometheusBytes().isEmpty()) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 1, prometheus_);
}
if (!getRocksdbKvBytes().isEmpty()) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 2, rocksdbKv_);
}
if (!getRocksdbRaftBytes().isEmpty()) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 3, rocksdbRaft_);
}
if (!getJemallocBytes().isEmpty()) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 4, jemalloc_);
}
if (storeId_ != 0L) {
output.writeUInt64(5, storeId_);
}
unknownFields.writeTo(output);
}
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (!getPrometheusBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(1, prometheus_);
}
if (!getRocksdbKvBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(2, rocksdbKv_);
}
if (!getRocksdbRaftBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(3, rocksdbRaft_);
}
if (!getJemallocBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(4, jemalloc_);
}
if (storeId_ != 0L) {
size += com.google.protobuf.CodedOutputStream
.computeUInt64Size(5, storeId_);
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof org.tikv.kvproto.Debugpb.GetMetricsResponse)) {
return super.equals(obj);
}
org.tikv.kvproto.Debugpb.GetMetricsResponse other = (org.tikv.kvproto.Debugpb.GetMetricsResponse) obj;
boolean result = true;
result = result && getPrometheus()
.equals(other.getPrometheus());
result = result && getRocksdbKv()
.equals(other.getRocksdbKv());
result = result && getRocksdbRaft()
.equals(other.getRocksdbRaft());
result = result && getJemalloc()
.equals(other.getJemalloc());
result = result && (getStoreId()
== other.getStoreId());
result = result && unknownFields.equals(other.unknownFields);
return result;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
hash = (37 * hash) + PROMETHEUS_FIELD_NUMBER;
hash = (53 * hash) + getPrometheus().hashCode();
hash = (37 * hash) + ROCKSDB_KV_FIELD_NUMBER;
hash = (53 * hash) + getRocksdbKv().hashCode();
hash = (37 * hash) + ROCKSDB_RAFT_FIELD_NUMBER;
hash = (53 * hash) + getRocksdbRaft().hashCode();
hash = (37 * hash) + JEMALLOC_FIELD_NUMBER;
hash = (53 * hash) + getJemalloc().hashCode();
hash = (37 * hash) + STORE_ID_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
getStoreId());
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static org.tikv.kvproto.Debugpb.GetMetricsResponse parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.tikv.kvproto.Debugpb.GetMetricsResponse parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.tikv.kvproto.Debugpb.GetMetricsResponse parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.tikv.kvproto.Debugpb.GetMetricsResponse parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.tikv.kvproto.Debugpb.GetMetricsResponse parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.tikv.kvproto.Debugpb.GetMetricsResponse parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.tikv.kvproto.Debugpb.GetMetricsResponse parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static org.tikv.kvproto.Debugpb.GetMetricsResponse parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static org.tikv.kvproto.Debugpb.GetMetricsResponse parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static org.tikv.kvproto.Debugpb.GetMetricsResponse parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static org.tikv.kvproto.Debugpb.GetMetricsResponse parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static org.tikv.kvproto.Debugpb.GetMetricsResponse parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(org.tikv.kvproto.Debugpb.GetMetricsResponse prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code debugpb.GetMetricsResponse}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:debugpb.GetMetricsResponse)
org.tikv.kvproto.Debugpb.GetMetricsResponseOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.tikv.kvproto.Debugpb.internal_static_debugpb_GetMetricsResponse_descriptor;
}
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.tikv.kvproto.Debugpb.internal_static_debugpb_GetMetricsResponse_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.tikv.kvproto.Debugpb.GetMetricsResponse.class, org.tikv.kvproto.Debugpb.GetMetricsResponse.Builder.class);
}
// Construct using org.tikv.kvproto.Debugpb.GetMetricsResponse.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
public Builder clear() {
super.clear();
prometheus_ = "";
rocksdbKv_ = "";
rocksdbRaft_ = "";
jemalloc_ = "";
storeId_ = 0L;
return this;
}
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return org.tikv.kvproto.Debugpb.internal_static_debugpb_GetMetricsResponse_descriptor;
}
public org.tikv.kvproto.Debugpb.GetMetricsResponse getDefaultInstanceForType() {
return org.tikv.kvproto.Debugpb.GetMetricsResponse.getDefaultInstance();
}
public org.tikv.kvproto.Debugpb.GetMetricsResponse build() {
org.tikv.kvproto.Debugpb.GetMetricsResponse result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
public org.tikv.kvproto.Debugpb.GetMetricsResponse buildPartial() {
org.tikv.kvproto.Debugpb.GetMetricsResponse result = new org.tikv.kvproto.Debugpb.GetMetricsResponse(this);
result.prometheus_ = prometheus_;
result.rocksdbKv_ = rocksdbKv_;
result.rocksdbRaft_ = rocksdbRaft_;
result.jemalloc_ = jemalloc_;
result.storeId_ = storeId_;
onBuilt();
return result;
}
public Builder clone() {
return (Builder) super.clone();
}
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.setField(field, value);
}
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return (Builder) super.clearField(field);
}
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return (Builder) super.clearOneof(oneof);
}
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return (Builder) super.setRepeatedField(field, index, value);
}
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.addRepeatedField(field, value);
}
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof org.tikv.kvproto.Debugpb.GetMetricsResponse) {
return mergeFrom((org.tikv.kvproto.Debugpb.GetMetricsResponse)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(org.tikv.kvproto.Debugpb.GetMetricsResponse other) {
if (other == org.tikv.kvproto.Debugpb.GetMetricsResponse.getDefaultInstance()) return this;
if (!other.getPrometheus().isEmpty()) {
prometheus_ = other.prometheus_;
onChanged();
}
if (!other.getRocksdbKv().isEmpty()) {
rocksdbKv_ = other.rocksdbKv_;
onChanged();
}
if (!other.getRocksdbRaft().isEmpty()) {
rocksdbRaft_ = other.rocksdbRaft_;
onChanged();
}
if (!other.getJemalloc().isEmpty()) {
jemalloc_ = other.jemalloc_;
onChanged();
}
if (other.getStoreId() != 0L) {
setStoreId(other.getStoreId());
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
public final boolean isInitialized() {
return true;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
org.tikv.kvproto.Debugpb.GetMetricsResponse parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (org.tikv.kvproto.Debugpb.GetMetricsResponse) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private java.lang.Object prometheus_ = "";
/**
* string prometheus = 1;
*/
public java.lang.String getPrometheus() {
java.lang.Object ref = prometheus_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
prometheus_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* string prometheus = 1;
*/
public com.google.protobuf.ByteString
getPrometheusBytes() {
java.lang.Object ref = prometheus_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
prometheus_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* string prometheus = 1;
*/
public Builder setPrometheus(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
prometheus_ = value;
onChanged();
return this;
}
/**
* string prometheus = 1;
*/
public Builder clearPrometheus() {
prometheus_ = getDefaultInstance().getPrometheus();
onChanged();
return this;
}
/**
* string prometheus = 1;
*/
public Builder setPrometheusBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
prometheus_ = value;
onChanged();
return this;
}
private java.lang.Object rocksdbKv_ = "";
/**
* string rocksdb_kv = 2;
*/
public java.lang.String getRocksdbKv() {
java.lang.Object ref = rocksdbKv_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
rocksdbKv_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* string rocksdb_kv = 2;
*/
public com.google.protobuf.ByteString
getRocksdbKvBytes() {
java.lang.Object ref = rocksdbKv_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
rocksdbKv_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* string rocksdb_kv = 2;
*/
public Builder setRocksdbKv(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
rocksdbKv_ = value;
onChanged();
return this;
}
/**
* string rocksdb_kv = 2;
*/
public Builder clearRocksdbKv() {
rocksdbKv_ = getDefaultInstance().getRocksdbKv();
onChanged();
return this;
}
/**
* string rocksdb_kv = 2;
*/
public Builder setRocksdbKvBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
rocksdbKv_ = value;
onChanged();
return this;
}
private java.lang.Object rocksdbRaft_ = "";
/**
* string rocksdb_raft = 3;
*/
public java.lang.String getRocksdbRaft() {
java.lang.Object ref = rocksdbRaft_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
rocksdbRaft_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* string rocksdb_raft = 3;
*/
public com.google.protobuf.ByteString
getRocksdbRaftBytes() {
java.lang.Object ref = rocksdbRaft_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
rocksdbRaft_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* string rocksdb_raft = 3;
*/
public Builder setRocksdbRaft(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
rocksdbRaft_ = value;
onChanged();
return this;
}
/**
* string rocksdb_raft = 3;
*/
public Builder clearRocksdbRaft() {
rocksdbRaft_ = getDefaultInstance().getRocksdbRaft();
onChanged();
return this;
}
/**
* string rocksdb_raft = 3;
*/
public Builder setRocksdbRaftBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
rocksdbRaft_ = value;
onChanged();
return this;
}
private java.lang.Object jemalloc_ = "";
/**
* string jemalloc = 4;
*/
public java.lang.String getJemalloc() {
java.lang.Object ref = jemalloc_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
jemalloc_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* string jemalloc = 4;
*/
public com.google.protobuf.ByteString
getJemallocBytes() {
java.lang.Object ref = jemalloc_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
jemalloc_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* string jemalloc = 4;
*/
public Builder setJemalloc(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
jemalloc_ = value;
onChanged();
return this;
}
/**
* string jemalloc = 4;
*/
public Builder clearJemalloc() {
jemalloc_ = getDefaultInstance().getJemalloc();
onChanged();
return this;
}
/**
* string jemalloc = 4;
*/
public Builder setJemallocBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
jemalloc_ = value;
onChanged();
return this;
}
private long storeId_ ;
/**
* uint64 store_id = 5;
*/
public long getStoreId() {
return storeId_;
}
/**
* uint64 store_id = 5;
*/
public Builder setStoreId(long value) {
storeId_ = value;
onChanged();
return this;
}
/**
* uint64 store_id = 5;
*/
public Builder clearStoreId() {
storeId_ = 0L;
onChanged();
return this;
}
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFieldsProto3(unknownFields);
}
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:debugpb.GetMetricsResponse)
}
// @@protoc_insertion_point(class_scope:debugpb.GetMetricsResponse)
private static final org.tikv.kvproto.Debugpb.GetMetricsResponse DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new org.tikv.kvproto.Debugpb.GetMetricsResponse();
}
public static org.tikv.kvproto.Debugpb.GetMetricsResponse getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
public GetMetricsResponse parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new GetMetricsResponse(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
public org.tikv.kvproto.Debugpb.GetMetricsResponse getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface RegionConsistencyCheckRequestOrBuilder extends
// @@protoc_insertion_point(interface_extends:debugpb.RegionConsistencyCheckRequest)
com.google.protobuf.MessageOrBuilder {
/**
* uint64 region_id = 1;
*/
long getRegionId();
}
/**
* Protobuf type {@code debugpb.RegionConsistencyCheckRequest}
*/
public static final class RegionConsistencyCheckRequest extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:debugpb.RegionConsistencyCheckRequest)
RegionConsistencyCheckRequestOrBuilder {
private static final long serialVersionUID = 0L;
// Use RegionConsistencyCheckRequest.newBuilder() to construct.
private RegionConsistencyCheckRequest(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private RegionConsistencyCheckRequest() {
regionId_ = 0L;
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private RegionConsistencyCheckRequest(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
default: {
if (!parseUnknownFieldProto3(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
case 8: {
regionId_ = input.readUInt64();
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.tikv.kvproto.Debugpb.internal_static_debugpb_RegionConsistencyCheckRequest_descriptor;
}
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.tikv.kvproto.Debugpb.internal_static_debugpb_RegionConsistencyCheckRequest_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.tikv.kvproto.Debugpb.RegionConsistencyCheckRequest.class, org.tikv.kvproto.Debugpb.RegionConsistencyCheckRequest.Builder.class);
}
public static final int REGION_ID_FIELD_NUMBER = 1;
private long regionId_;
/**
* uint64 region_id = 1;
*/
public long getRegionId() {
return regionId_;
}
private byte memoizedIsInitialized = -1;
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (regionId_ != 0L) {
output.writeUInt64(1, regionId_);
}
unknownFields.writeTo(output);
}
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (regionId_ != 0L) {
size += com.google.protobuf.CodedOutputStream
.computeUInt64Size(1, regionId_);
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof org.tikv.kvproto.Debugpb.RegionConsistencyCheckRequest)) {
return super.equals(obj);
}
org.tikv.kvproto.Debugpb.RegionConsistencyCheckRequest other = (org.tikv.kvproto.Debugpb.RegionConsistencyCheckRequest) obj;
boolean result = true;
result = result && (getRegionId()
== other.getRegionId());
result = result && unknownFields.equals(other.unknownFields);
return result;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
hash = (37 * hash) + REGION_ID_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
getRegionId());
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static org.tikv.kvproto.Debugpb.RegionConsistencyCheckRequest parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.tikv.kvproto.Debugpb.RegionConsistencyCheckRequest parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.tikv.kvproto.Debugpb.RegionConsistencyCheckRequest parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.tikv.kvproto.Debugpb.RegionConsistencyCheckRequest parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.tikv.kvproto.Debugpb.RegionConsistencyCheckRequest parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.tikv.kvproto.Debugpb.RegionConsistencyCheckRequest parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.tikv.kvproto.Debugpb.RegionConsistencyCheckRequest parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static org.tikv.kvproto.Debugpb.RegionConsistencyCheckRequest parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static org.tikv.kvproto.Debugpb.RegionConsistencyCheckRequest parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static org.tikv.kvproto.Debugpb.RegionConsistencyCheckRequest parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static org.tikv.kvproto.Debugpb.RegionConsistencyCheckRequest parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static org.tikv.kvproto.Debugpb.RegionConsistencyCheckRequest parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(org.tikv.kvproto.Debugpb.RegionConsistencyCheckRequest prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code debugpb.RegionConsistencyCheckRequest}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:debugpb.RegionConsistencyCheckRequest)
org.tikv.kvproto.Debugpb.RegionConsistencyCheckRequestOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.tikv.kvproto.Debugpb.internal_static_debugpb_RegionConsistencyCheckRequest_descriptor;
}
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.tikv.kvproto.Debugpb.internal_static_debugpb_RegionConsistencyCheckRequest_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.tikv.kvproto.Debugpb.RegionConsistencyCheckRequest.class, org.tikv.kvproto.Debugpb.RegionConsistencyCheckRequest.Builder.class);
}
// Construct using org.tikv.kvproto.Debugpb.RegionConsistencyCheckRequest.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
public Builder clear() {
super.clear();
regionId_ = 0L;
return this;
}
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return org.tikv.kvproto.Debugpb.internal_static_debugpb_RegionConsistencyCheckRequest_descriptor;
}
public org.tikv.kvproto.Debugpb.RegionConsistencyCheckRequest getDefaultInstanceForType() {
return org.tikv.kvproto.Debugpb.RegionConsistencyCheckRequest.getDefaultInstance();
}
public org.tikv.kvproto.Debugpb.RegionConsistencyCheckRequest build() {
org.tikv.kvproto.Debugpb.RegionConsistencyCheckRequest result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
public org.tikv.kvproto.Debugpb.RegionConsistencyCheckRequest buildPartial() {
org.tikv.kvproto.Debugpb.RegionConsistencyCheckRequest result = new org.tikv.kvproto.Debugpb.RegionConsistencyCheckRequest(this);
result.regionId_ = regionId_;
onBuilt();
return result;
}
public Builder clone() {
return (Builder) super.clone();
}
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.setField(field, value);
}
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return (Builder) super.clearField(field);
}
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return (Builder) super.clearOneof(oneof);
}
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return (Builder) super.setRepeatedField(field, index, value);
}
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.addRepeatedField(field, value);
}
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof org.tikv.kvproto.Debugpb.RegionConsistencyCheckRequest) {
return mergeFrom((org.tikv.kvproto.Debugpb.RegionConsistencyCheckRequest)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(org.tikv.kvproto.Debugpb.RegionConsistencyCheckRequest other) {
if (other == org.tikv.kvproto.Debugpb.RegionConsistencyCheckRequest.getDefaultInstance()) return this;
if (other.getRegionId() != 0L) {
setRegionId(other.getRegionId());
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
public final boolean isInitialized() {
return true;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
org.tikv.kvproto.Debugpb.RegionConsistencyCheckRequest parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (org.tikv.kvproto.Debugpb.RegionConsistencyCheckRequest) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private long regionId_ ;
/**
* uint64 region_id = 1;
*/
public long getRegionId() {
return regionId_;
}
/**
* uint64 region_id = 1;
*/
public Builder setRegionId(long value) {
regionId_ = value;
onChanged();
return this;
}
/**
* uint64 region_id = 1;
*/
public Builder clearRegionId() {
regionId_ = 0L;
onChanged();
return this;
}
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFieldsProto3(unknownFields);
}
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:debugpb.RegionConsistencyCheckRequest)
}
// @@protoc_insertion_point(class_scope:debugpb.RegionConsistencyCheckRequest)
private static final org.tikv.kvproto.Debugpb.RegionConsistencyCheckRequest DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new org.tikv.kvproto.Debugpb.RegionConsistencyCheckRequest();
}
public static org.tikv.kvproto.Debugpb.RegionConsistencyCheckRequest getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
public RegionConsistencyCheckRequest parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new RegionConsistencyCheckRequest(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
public org.tikv.kvproto.Debugpb.RegionConsistencyCheckRequest getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface RegionConsistencyCheckResponseOrBuilder extends
// @@protoc_insertion_point(interface_extends:debugpb.RegionConsistencyCheckResponse)
com.google.protobuf.MessageOrBuilder {
}
/**
* Protobuf type {@code debugpb.RegionConsistencyCheckResponse}
*/
public static final class RegionConsistencyCheckResponse extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:debugpb.RegionConsistencyCheckResponse)
RegionConsistencyCheckResponseOrBuilder {
private static final long serialVersionUID = 0L;
// Use RegionConsistencyCheckResponse.newBuilder() to construct.
private RegionConsistencyCheckResponse(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private RegionConsistencyCheckResponse() {
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private RegionConsistencyCheckResponse(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
default: {
if (!parseUnknownFieldProto3(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.tikv.kvproto.Debugpb.internal_static_debugpb_RegionConsistencyCheckResponse_descriptor;
}
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.tikv.kvproto.Debugpb.internal_static_debugpb_RegionConsistencyCheckResponse_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.tikv.kvproto.Debugpb.RegionConsistencyCheckResponse.class, org.tikv.kvproto.Debugpb.RegionConsistencyCheckResponse.Builder.class);
}
private byte memoizedIsInitialized = -1;
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
unknownFields.writeTo(output);
}
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof org.tikv.kvproto.Debugpb.RegionConsistencyCheckResponse)) {
return super.equals(obj);
}
org.tikv.kvproto.Debugpb.RegionConsistencyCheckResponse other = (org.tikv.kvproto.Debugpb.RegionConsistencyCheckResponse) obj;
boolean result = true;
result = result && unknownFields.equals(other.unknownFields);
return result;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static org.tikv.kvproto.Debugpb.RegionConsistencyCheckResponse parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.tikv.kvproto.Debugpb.RegionConsistencyCheckResponse parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.tikv.kvproto.Debugpb.RegionConsistencyCheckResponse parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.tikv.kvproto.Debugpb.RegionConsistencyCheckResponse parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.tikv.kvproto.Debugpb.RegionConsistencyCheckResponse parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.tikv.kvproto.Debugpb.RegionConsistencyCheckResponse parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.tikv.kvproto.Debugpb.RegionConsistencyCheckResponse parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static org.tikv.kvproto.Debugpb.RegionConsistencyCheckResponse parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static org.tikv.kvproto.Debugpb.RegionConsistencyCheckResponse parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static org.tikv.kvproto.Debugpb.RegionConsistencyCheckResponse parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static org.tikv.kvproto.Debugpb.RegionConsistencyCheckResponse parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static org.tikv.kvproto.Debugpb.RegionConsistencyCheckResponse parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(org.tikv.kvproto.Debugpb.RegionConsistencyCheckResponse prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code debugpb.RegionConsistencyCheckResponse}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:debugpb.RegionConsistencyCheckResponse)
org.tikv.kvproto.Debugpb.RegionConsistencyCheckResponseOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.tikv.kvproto.Debugpb.internal_static_debugpb_RegionConsistencyCheckResponse_descriptor;
}
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.tikv.kvproto.Debugpb.internal_static_debugpb_RegionConsistencyCheckResponse_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.tikv.kvproto.Debugpb.RegionConsistencyCheckResponse.class, org.tikv.kvproto.Debugpb.RegionConsistencyCheckResponse.Builder.class);
}
// Construct using org.tikv.kvproto.Debugpb.RegionConsistencyCheckResponse.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
public Builder clear() {
super.clear();
return this;
}
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return org.tikv.kvproto.Debugpb.internal_static_debugpb_RegionConsistencyCheckResponse_descriptor;
}
public org.tikv.kvproto.Debugpb.RegionConsistencyCheckResponse getDefaultInstanceForType() {
return org.tikv.kvproto.Debugpb.RegionConsistencyCheckResponse.getDefaultInstance();
}
public org.tikv.kvproto.Debugpb.RegionConsistencyCheckResponse build() {
org.tikv.kvproto.Debugpb.RegionConsistencyCheckResponse result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
public org.tikv.kvproto.Debugpb.RegionConsistencyCheckResponse buildPartial() {
org.tikv.kvproto.Debugpb.RegionConsistencyCheckResponse result = new org.tikv.kvproto.Debugpb.RegionConsistencyCheckResponse(this);
onBuilt();
return result;
}
public Builder clone() {
return (Builder) super.clone();
}
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.setField(field, value);
}
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return (Builder) super.clearField(field);
}
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return (Builder) super.clearOneof(oneof);
}
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return (Builder) super.setRepeatedField(field, index, value);
}
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.addRepeatedField(field, value);
}
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof org.tikv.kvproto.Debugpb.RegionConsistencyCheckResponse) {
return mergeFrom((org.tikv.kvproto.Debugpb.RegionConsistencyCheckResponse)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(org.tikv.kvproto.Debugpb.RegionConsistencyCheckResponse other) {
if (other == org.tikv.kvproto.Debugpb.RegionConsistencyCheckResponse.getDefaultInstance()) return this;
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
public final boolean isInitialized() {
return true;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
org.tikv.kvproto.Debugpb.RegionConsistencyCheckResponse parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (org.tikv.kvproto.Debugpb.RegionConsistencyCheckResponse) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFieldsProto3(unknownFields);
}
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:debugpb.RegionConsistencyCheckResponse)
}
// @@protoc_insertion_point(class_scope:debugpb.RegionConsistencyCheckResponse)
private static final org.tikv.kvproto.Debugpb.RegionConsistencyCheckResponse DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new org.tikv.kvproto.Debugpb.RegionConsistencyCheckResponse();
}
public static org.tikv.kvproto.Debugpb.RegionConsistencyCheckResponse getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
public RegionConsistencyCheckResponse parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new RegionConsistencyCheckResponse(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
public org.tikv.kvproto.Debugpb.RegionConsistencyCheckResponse getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface ModifyTikvConfigRequestOrBuilder extends
// @@protoc_insertion_point(interface_extends:debugpb.ModifyTikvConfigRequest)
com.google.protobuf.MessageOrBuilder {
/**
* .debugpb.MODULE module = 1;
*/
int getModuleValue();
/**
* .debugpb.MODULE module = 1;
*/
org.tikv.kvproto.Debugpb.MODULE getModule();
/**
* string config_name = 2;
*/
java.lang.String getConfigName();
/**
* string config_name = 2;
*/
com.google.protobuf.ByteString
getConfigNameBytes();
/**
* string config_value = 3;
*/
java.lang.String getConfigValue();
/**
* string config_value = 3;
*/
com.google.protobuf.ByteString
getConfigValueBytes();
}
/**
* Protobuf type {@code debugpb.ModifyTikvConfigRequest}
*/
public static final class ModifyTikvConfigRequest extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:debugpb.ModifyTikvConfigRequest)
ModifyTikvConfigRequestOrBuilder {
private static final long serialVersionUID = 0L;
// Use ModifyTikvConfigRequest.newBuilder() to construct.
private ModifyTikvConfigRequest(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private ModifyTikvConfigRequest() {
module_ = 0;
configName_ = "";
configValue_ = "";
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private ModifyTikvConfigRequest(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
default: {
if (!parseUnknownFieldProto3(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
case 8: {
int rawValue = input.readEnum();
module_ = rawValue;
break;
}
case 18: {
java.lang.String s = input.readStringRequireUtf8();
configName_ = s;
break;
}
case 26: {
java.lang.String s = input.readStringRequireUtf8();
configValue_ = s;
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.tikv.kvproto.Debugpb.internal_static_debugpb_ModifyTikvConfigRequest_descriptor;
}
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.tikv.kvproto.Debugpb.internal_static_debugpb_ModifyTikvConfigRequest_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.tikv.kvproto.Debugpb.ModifyTikvConfigRequest.class, org.tikv.kvproto.Debugpb.ModifyTikvConfigRequest.Builder.class);
}
public static final int MODULE_FIELD_NUMBER = 1;
private int module_;
/**
* .debugpb.MODULE module = 1;
*/
public int getModuleValue() {
return module_;
}
/**
* .debugpb.MODULE module = 1;
*/
public org.tikv.kvproto.Debugpb.MODULE getModule() {
org.tikv.kvproto.Debugpb.MODULE result = org.tikv.kvproto.Debugpb.MODULE.valueOf(module_);
return result == null ? org.tikv.kvproto.Debugpb.MODULE.UNRECOGNIZED : result;
}
public static final int CONFIG_NAME_FIELD_NUMBER = 2;
private volatile java.lang.Object configName_;
/**
* string config_name = 2;
*/
public java.lang.String getConfigName() {
java.lang.Object ref = configName_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
configName_ = s;
return s;
}
}
/**
* string config_name = 2;
*/
public com.google.protobuf.ByteString
getConfigNameBytes() {
java.lang.Object ref = configName_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
configName_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int CONFIG_VALUE_FIELD_NUMBER = 3;
private volatile java.lang.Object configValue_;
/**
* string config_value = 3;
*/
public java.lang.String getConfigValue() {
java.lang.Object ref = configValue_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
configValue_ = s;
return s;
}
}
/**
* string config_value = 3;
*/
public com.google.protobuf.ByteString
getConfigValueBytes() {
java.lang.Object ref = configValue_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
configValue_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
private byte memoizedIsInitialized = -1;
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (module_ != org.tikv.kvproto.Debugpb.MODULE.UNUSED.getNumber()) {
output.writeEnum(1, module_);
}
if (!getConfigNameBytes().isEmpty()) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 2, configName_);
}
if (!getConfigValueBytes().isEmpty()) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 3, configValue_);
}
unknownFields.writeTo(output);
}
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (module_ != org.tikv.kvproto.Debugpb.MODULE.UNUSED.getNumber()) {
size += com.google.protobuf.CodedOutputStream
.computeEnumSize(1, module_);
}
if (!getConfigNameBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(2, configName_);
}
if (!getConfigValueBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(3, configValue_);
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof org.tikv.kvproto.Debugpb.ModifyTikvConfigRequest)) {
return super.equals(obj);
}
org.tikv.kvproto.Debugpb.ModifyTikvConfigRequest other = (org.tikv.kvproto.Debugpb.ModifyTikvConfigRequest) obj;
boolean result = true;
result = result && module_ == other.module_;
result = result && getConfigName()
.equals(other.getConfigName());
result = result && getConfigValue()
.equals(other.getConfigValue());
result = result && unknownFields.equals(other.unknownFields);
return result;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
hash = (37 * hash) + MODULE_FIELD_NUMBER;
hash = (53 * hash) + module_;
hash = (37 * hash) + CONFIG_NAME_FIELD_NUMBER;
hash = (53 * hash) + getConfigName().hashCode();
hash = (37 * hash) + CONFIG_VALUE_FIELD_NUMBER;
hash = (53 * hash) + getConfigValue().hashCode();
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static org.tikv.kvproto.Debugpb.ModifyTikvConfigRequest parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.tikv.kvproto.Debugpb.ModifyTikvConfigRequest parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.tikv.kvproto.Debugpb.ModifyTikvConfigRequest parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.tikv.kvproto.Debugpb.ModifyTikvConfigRequest parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.tikv.kvproto.Debugpb.ModifyTikvConfigRequest parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.tikv.kvproto.Debugpb.ModifyTikvConfigRequest parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.tikv.kvproto.Debugpb.ModifyTikvConfigRequest parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static org.tikv.kvproto.Debugpb.ModifyTikvConfigRequest parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static org.tikv.kvproto.Debugpb.ModifyTikvConfigRequest parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static org.tikv.kvproto.Debugpb.ModifyTikvConfigRequest parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static org.tikv.kvproto.Debugpb.ModifyTikvConfigRequest parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static org.tikv.kvproto.Debugpb.ModifyTikvConfigRequest parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(org.tikv.kvproto.Debugpb.ModifyTikvConfigRequest prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code debugpb.ModifyTikvConfigRequest}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:debugpb.ModifyTikvConfigRequest)
org.tikv.kvproto.Debugpb.ModifyTikvConfigRequestOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.tikv.kvproto.Debugpb.internal_static_debugpb_ModifyTikvConfigRequest_descriptor;
}
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.tikv.kvproto.Debugpb.internal_static_debugpb_ModifyTikvConfigRequest_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.tikv.kvproto.Debugpb.ModifyTikvConfigRequest.class, org.tikv.kvproto.Debugpb.ModifyTikvConfigRequest.Builder.class);
}
// Construct using org.tikv.kvproto.Debugpb.ModifyTikvConfigRequest.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
public Builder clear() {
super.clear();
module_ = 0;
configName_ = "";
configValue_ = "";
return this;
}
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return org.tikv.kvproto.Debugpb.internal_static_debugpb_ModifyTikvConfigRequest_descriptor;
}
public org.tikv.kvproto.Debugpb.ModifyTikvConfigRequest getDefaultInstanceForType() {
return org.tikv.kvproto.Debugpb.ModifyTikvConfigRequest.getDefaultInstance();
}
public org.tikv.kvproto.Debugpb.ModifyTikvConfigRequest build() {
org.tikv.kvproto.Debugpb.ModifyTikvConfigRequest result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
public org.tikv.kvproto.Debugpb.ModifyTikvConfigRequest buildPartial() {
org.tikv.kvproto.Debugpb.ModifyTikvConfigRequest result = new org.tikv.kvproto.Debugpb.ModifyTikvConfigRequest(this);
result.module_ = module_;
result.configName_ = configName_;
result.configValue_ = configValue_;
onBuilt();
return result;
}
public Builder clone() {
return (Builder) super.clone();
}
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.setField(field, value);
}
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return (Builder) super.clearField(field);
}
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return (Builder) super.clearOneof(oneof);
}
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return (Builder) super.setRepeatedField(field, index, value);
}
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.addRepeatedField(field, value);
}
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof org.tikv.kvproto.Debugpb.ModifyTikvConfigRequest) {
return mergeFrom((org.tikv.kvproto.Debugpb.ModifyTikvConfigRequest)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(org.tikv.kvproto.Debugpb.ModifyTikvConfigRequest other) {
if (other == org.tikv.kvproto.Debugpb.ModifyTikvConfigRequest.getDefaultInstance()) return this;
if (other.module_ != 0) {
setModuleValue(other.getModuleValue());
}
if (!other.getConfigName().isEmpty()) {
configName_ = other.configName_;
onChanged();
}
if (!other.getConfigValue().isEmpty()) {
configValue_ = other.configValue_;
onChanged();
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
public final boolean isInitialized() {
return true;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
org.tikv.kvproto.Debugpb.ModifyTikvConfigRequest parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (org.tikv.kvproto.Debugpb.ModifyTikvConfigRequest) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int module_ = 0;
/**
* .debugpb.MODULE module = 1;
*/
public int getModuleValue() {
return module_;
}
/**
* .debugpb.MODULE module = 1;
*/
public Builder setModuleValue(int value) {
module_ = value;
onChanged();
return this;
}
/**
* .debugpb.MODULE module = 1;
*/
public org.tikv.kvproto.Debugpb.MODULE getModule() {
org.tikv.kvproto.Debugpb.MODULE result = org.tikv.kvproto.Debugpb.MODULE.valueOf(module_);
return result == null ? org.tikv.kvproto.Debugpb.MODULE.UNRECOGNIZED : result;
}
/**
* .debugpb.MODULE module = 1;
*/
public Builder setModule(org.tikv.kvproto.Debugpb.MODULE value) {
if (value == null) {
throw new NullPointerException();
}
module_ = value.getNumber();
onChanged();
return this;
}
/**
* .debugpb.MODULE module = 1;
*/
public Builder clearModule() {
module_ = 0;
onChanged();
return this;
}
private java.lang.Object configName_ = "";
/**
* string config_name = 2;
*/
public java.lang.String getConfigName() {
java.lang.Object ref = configName_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
configName_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* string config_name = 2;
*/
public com.google.protobuf.ByteString
getConfigNameBytes() {
java.lang.Object ref = configName_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
configName_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* string config_name = 2;
*/
public Builder setConfigName(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
configName_ = value;
onChanged();
return this;
}
/**
* string config_name = 2;
*/
public Builder clearConfigName() {
configName_ = getDefaultInstance().getConfigName();
onChanged();
return this;
}
/**
* string config_name = 2;
*/
public Builder setConfigNameBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
configName_ = value;
onChanged();
return this;
}
private java.lang.Object configValue_ = "";
/**
* string config_value = 3;
*/
public java.lang.String getConfigValue() {
java.lang.Object ref = configValue_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
configValue_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* string config_value = 3;
*/
public com.google.protobuf.ByteString
getConfigValueBytes() {
java.lang.Object ref = configValue_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
configValue_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* string config_value = 3;
*/
public Builder setConfigValue(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
configValue_ = value;
onChanged();
return this;
}
/**
* string config_value = 3;
*/
public Builder clearConfigValue() {
configValue_ = getDefaultInstance().getConfigValue();
onChanged();
return this;
}
/**
* string config_value = 3;
*/
public Builder setConfigValueBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
configValue_ = value;
onChanged();
return this;
}
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFieldsProto3(unknownFields);
}
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:debugpb.ModifyTikvConfigRequest)
}
// @@protoc_insertion_point(class_scope:debugpb.ModifyTikvConfigRequest)
private static final org.tikv.kvproto.Debugpb.ModifyTikvConfigRequest DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new org.tikv.kvproto.Debugpb.ModifyTikvConfigRequest();
}
public static org.tikv.kvproto.Debugpb.ModifyTikvConfigRequest getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
public ModifyTikvConfigRequest parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new ModifyTikvConfigRequest(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
public org.tikv.kvproto.Debugpb.ModifyTikvConfigRequest getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface ModifyTikvConfigResponseOrBuilder extends
// @@protoc_insertion_point(interface_extends:debugpb.ModifyTikvConfigResponse)
com.google.protobuf.MessageOrBuilder {
}
/**
* Protobuf type {@code debugpb.ModifyTikvConfigResponse}
*/
public static final class ModifyTikvConfigResponse extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:debugpb.ModifyTikvConfigResponse)
ModifyTikvConfigResponseOrBuilder {
private static final long serialVersionUID = 0L;
// Use ModifyTikvConfigResponse.newBuilder() to construct.
private ModifyTikvConfigResponse(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private ModifyTikvConfigResponse() {
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private ModifyTikvConfigResponse(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
default: {
if (!parseUnknownFieldProto3(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.tikv.kvproto.Debugpb.internal_static_debugpb_ModifyTikvConfigResponse_descriptor;
}
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.tikv.kvproto.Debugpb.internal_static_debugpb_ModifyTikvConfigResponse_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.tikv.kvproto.Debugpb.ModifyTikvConfigResponse.class, org.tikv.kvproto.Debugpb.ModifyTikvConfigResponse.Builder.class);
}
private byte memoizedIsInitialized = -1;
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
unknownFields.writeTo(output);
}
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof org.tikv.kvproto.Debugpb.ModifyTikvConfigResponse)) {
return super.equals(obj);
}
org.tikv.kvproto.Debugpb.ModifyTikvConfigResponse other = (org.tikv.kvproto.Debugpb.ModifyTikvConfigResponse) obj;
boolean result = true;
result = result && unknownFields.equals(other.unknownFields);
return result;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static org.tikv.kvproto.Debugpb.ModifyTikvConfigResponse parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.tikv.kvproto.Debugpb.ModifyTikvConfigResponse parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.tikv.kvproto.Debugpb.ModifyTikvConfigResponse parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.tikv.kvproto.Debugpb.ModifyTikvConfigResponse parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.tikv.kvproto.Debugpb.ModifyTikvConfigResponse parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.tikv.kvproto.Debugpb.ModifyTikvConfigResponse parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.tikv.kvproto.Debugpb.ModifyTikvConfigResponse parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static org.tikv.kvproto.Debugpb.ModifyTikvConfigResponse parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static org.tikv.kvproto.Debugpb.ModifyTikvConfigResponse parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static org.tikv.kvproto.Debugpb.ModifyTikvConfigResponse parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static org.tikv.kvproto.Debugpb.ModifyTikvConfigResponse parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static org.tikv.kvproto.Debugpb.ModifyTikvConfigResponse parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(org.tikv.kvproto.Debugpb.ModifyTikvConfigResponse prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code debugpb.ModifyTikvConfigResponse}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:debugpb.ModifyTikvConfigResponse)
org.tikv.kvproto.Debugpb.ModifyTikvConfigResponseOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.tikv.kvproto.Debugpb.internal_static_debugpb_ModifyTikvConfigResponse_descriptor;
}
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.tikv.kvproto.Debugpb.internal_static_debugpb_ModifyTikvConfigResponse_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.tikv.kvproto.Debugpb.ModifyTikvConfigResponse.class, org.tikv.kvproto.Debugpb.ModifyTikvConfigResponse.Builder.class);
}
// Construct using org.tikv.kvproto.Debugpb.ModifyTikvConfigResponse.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
public Builder clear() {
super.clear();
return this;
}
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return org.tikv.kvproto.Debugpb.internal_static_debugpb_ModifyTikvConfigResponse_descriptor;
}
public org.tikv.kvproto.Debugpb.ModifyTikvConfigResponse getDefaultInstanceForType() {
return org.tikv.kvproto.Debugpb.ModifyTikvConfigResponse.getDefaultInstance();
}
public org.tikv.kvproto.Debugpb.ModifyTikvConfigResponse build() {
org.tikv.kvproto.Debugpb.ModifyTikvConfigResponse result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
public org.tikv.kvproto.Debugpb.ModifyTikvConfigResponse buildPartial() {
org.tikv.kvproto.Debugpb.ModifyTikvConfigResponse result = new org.tikv.kvproto.Debugpb.ModifyTikvConfigResponse(this);
onBuilt();
return result;
}
public Builder clone() {
return (Builder) super.clone();
}
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.setField(field, value);
}
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return (Builder) super.clearField(field);
}
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return (Builder) super.clearOneof(oneof);
}
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return (Builder) super.setRepeatedField(field, index, value);
}
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.addRepeatedField(field, value);
}
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof org.tikv.kvproto.Debugpb.ModifyTikvConfigResponse) {
return mergeFrom((org.tikv.kvproto.Debugpb.ModifyTikvConfigResponse)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(org.tikv.kvproto.Debugpb.ModifyTikvConfigResponse other) {
if (other == org.tikv.kvproto.Debugpb.ModifyTikvConfigResponse.getDefaultInstance()) return this;
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
public final boolean isInitialized() {
return true;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
org.tikv.kvproto.Debugpb.ModifyTikvConfigResponse parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (org.tikv.kvproto.Debugpb.ModifyTikvConfigResponse) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFieldsProto3(unknownFields);
}
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:debugpb.ModifyTikvConfigResponse)
}
// @@protoc_insertion_point(class_scope:debugpb.ModifyTikvConfigResponse)
private static final org.tikv.kvproto.Debugpb.ModifyTikvConfigResponse DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new org.tikv.kvproto.Debugpb.ModifyTikvConfigResponse();
}
public static org.tikv.kvproto.Debugpb.ModifyTikvConfigResponse getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
public ModifyTikvConfigResponse parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new ModifyTikvConfigResponse(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
public org.tikv.kvproto.Debugpb.ModifyTikvConfigResponse getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface PropertyOrBuilder extends
// @@protoc_insertion_point(interface_extends:debugpb.Property)
com.google.protobuf.MessageOrBuilder {
/**
* string name = 1;
*/
java.lang.String getName();
/**
* string name = 1;
*/
com.google.protobuf.ByteString
getNameBytes();
/**
* string value = 2;
*/
java.lang.String getValue();
/**
* string value = 2;
*/
com.google.protobuf.ByteString
getValueBytes();
}
/**
* Protobuf type {@code debugpb.Property}
*/
public static final class Property extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:debugpb.Property)
PropertyOrBuilder {
private static final long serialVersionUID = 0L;
// Use Property.newBuilder() to construct.
private Property(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private Property() {
name_ = "";
value_ = "";
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private Property(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
default: {
if (!parseUnknownFieldProto3(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
case 10: {
java.lang.String s = input.readStringRequireUtf8();
name_ = s;
break;
}
case 18: {
java.lang.String s = input.readStringRequireUtf8();
value_ = s;
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.tikv.kvproto.Debugpb.internal_static_debugpb_Property_descriptor;
}
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.tikv.kvproto.Debugpb.internal_static_debugpb_Property_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.tikv.kvproto.Debugpb.Property.class, org.tikv.kvproto.Debugpb.Property.Builder.class);
}
public static final int NAME_FIELD_NUMBER = 1;
private volatile java.lang.Object name_;
/**
* string name = 1;
*/
public java.lang.String getName() {
java.lang.Object ref = name_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
name_ = s;
return s;
}
}
/**
* string name = 1;
*/
public com.google.protobuf.ByteString
getNameBytes() {
java.lang.Object ref = name_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
name_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int VALUE_FIELD_NUMBER = 2;
private volatile java.lang.Object value_;
/**
* string value = 2;
*/
public java.lang.String getValue() {
java.lang.Object ref = value_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
value_ = s;
return s;
}
}
/**
* string value = 2;
*/
public com.google.protobuf.ByteString
getValueBytes() {
java.lang.Object ref = value_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
value_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
private byte memoizedIsInitialized = -1;
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (!getNameBytes().isEmpty()) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 1, name_);
}
if (!getValueBytes().isEmpty()) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 2, value_);
}
unknownFields.writeTo(output);
}
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (!getNameBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(1, name_);
}
if (!getValueBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(2, value_);
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof org.tikv.kvproto.Debugpb.Property)) {
return super.equals(obj);
}
org.tikv.kvproto.Debugpb.Property other = (org.tikv.kvproto.Debugpb.Property) obj;
boolean result = true;
result = result && getName()
.equals(other.getName());
result = result && getValue()
.equals(other.getValue());
result = result && unknownFields.equals(other.unknownFields);
return result;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
hash = (37 * hash) + NAME_FIELD_NUMBER;
hash = (53 * hash) + getName().hashCode();
hash = (37 * hash) + VALUE_FIELD_NUMBER;
hash = (53 * hash) + getValue().hashCode();
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static org.tikv.kvproto.Debugpb.Property parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.tikv.kvproto.Debugpb.Property parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.tikv.kvproto.Debugpb.Property parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.tikv.kvproto.Debugpb.Property parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.tikv.kvproto.Debugpb.Property parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.tikv.kvproto.Debugpb.Property parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.tikv.kvproto.Debugpb.Property parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static org.tikv.kvproto.Debugpb.Property parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static org.tikv.kvproto.Debugpb.Property parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static org.tikv.kvproto.Debugpb.Property parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static org.tikv.kvproto.Debugpb.Property parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static org.tikv.kvproto.Debugpb.Property parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(org.tikv.kvproto.Debugpb.Property prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code debugpb.Property}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:debugpb.Property)
org.tikv.kvproto.Debugpb.PropertyOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.tikv.kvproto.Debugpb.internal_static_debugpb_Property_descriptor;
}
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.tikv.kvproto.Debugpb.internal_static_debugpb_Property_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.tikv.kvproto.Debugpb.Property.class, org.tikv.kvproto.Debugpb.Property.Builder.class);
}
// Construct using org.tikv.kvproto.Debugpb.Property.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
public Builder clear() {
super.clear();
name_ = "";
value_ = "";
return this;
}
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return org.tikv.kvproto.Debugpb.internal_static_debugpb_Property_descriptor;
}
public org.tikv.kvproto.Debugpb.Property getDefaultInstanceForType() {
return org.tikv.kvproto.Debugpb.Property.getDefaultInstance();
}
public org.tikv.kvproto.Debugpb.Property build() {
org.tikv.kvproto.Debugpb.Property result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
public org.tikv.kvproto.Debugpb.Property buildPartial() {
org.tikv.kvproto.Debugpb.Property result = new org.tikv.kvproto.Debugpb.Property(this);
result.name_ = name_;
result.value_ = value_;
onBuilt();
return result;
}
public Builder clone() {
return (Builder) super.clone();
}
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.setField(field, value);
}
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return (Builder) super.clearField(field);
}
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return (Builder) super.clearOneof(oneof);
}
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return (Builder) super.setRepeatedField(field, index, value);
}
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.addRepeatedField(field, value);
}
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof org.tikv.kvproto.Debugpb.Property) {
return mergeFrom((org.tikv.kvproto.Debugpb.Property)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(org.tikv.kvproto.Debugpb.Property other) {
if (other == org.tikv.kvproto.Debugpb.Property.getDefaultInstance()) return this;
if (!other.getName().isEmpty()) {
name_ = other.name_;
onChanged();
}
if (!other.getValue().isEmpty()) {
value_ = other.value_;
onChanged();
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
public final boolean isInitialized() {
return true;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
org.tikv.kvproto.Debugpb.Property parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (org.tikv.kvproto.Debugpb.Property) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private java.lang.Object name_ = "";
/**
* string name = 1;
*/
public java.lang.String getName() {
java.lang.Object ref = name_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
name_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* string name = 1;
*/
public com.google.protobuf.ByteString
getNameBytes() {
java.lang.Object ref = name_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
name_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* string name = 1;
*/
public Builder setName(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
name_ = value;
onChanged();
return this;
}
/**
* string name = 1;
*/
public Builder clearName() {
name_ = getDefaultInstance().getName();
onChanged();
return this;
}
/**
* string name = 1;
*/
public Builder setNameBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
name_ = value;
onChanged();
return this;
}
private java.lang.Object value_ = "";
/**
* string value = 2;
*/
public java.lang.String getValue() {
java.lang.Object ref = value_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
value_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* string value = 2;
*/
public com.google.protobuf.ByteString
getValueBytes() {
java.lang.Object ref = value_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
value_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* string value = 2;
*/
public Builder setValue(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
value_ = value;
onChanged();
return this;
}
/**
* string value = 2;
*/
public Builder clearValue() {
value_ = getDefaultInstance().getValue();
onChanged();
return this;
}
/**
* string value = 2;
*/
public Builder setValueBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
value_ = value;
onChanged();
return this;
}
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFieldsProto3(unknownFields);
}
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:debugpb.Property)
}
// @@protoc_insertion_point(class_scope:debugpb.Property)
private static final org.tikv.kvproto.Debugpb.Property DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new org.tikv.kvproto.Debugpb.Property();
}
public static org.tikv.kvproto.Debugpb.Property getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
public Property parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new Property(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
public org.tikv.kvproto.Debugpb.Property getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface GetRegionPropertiesRequestOrBuilder extends
// @@protoc_insertion_point(interface_extends:debugpb.GetRegionPropertiesRequest)
com.google.protobuf.MessageOrBuilder {
/**
* uint64 region_id = 1;
*/
long getRegionId();
}
/**
* Protobuf type {@code debugpb.GetRegionPropertiesRequest}
*/
public static final class GetRegionPropertiesRequest extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:debugpb.GetRegionPropertiesRequest)
GetRegionPropertiesRequestOrBuilder {
private static final long serialVersionUID = 0L;
// Use GetRegionPropertiesRequest.newBuilder() to construct.
private GetRegionPropertiesRequest(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private GetRegionPropertiesRequest() {
regionId_ = 0L;
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private GetRegionPropertiesRequest(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
default: {
if (!parseUnknownFieldProto3(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
case 8: {
regionId_ = input.readUInt64();
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.tikv.kvproto.Debugpb.internal_static_debugpb_GetRegionPropertiesRequest_descriptor;
}
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.tikv.kvproto.Debugpb.internal_static_debugpb_GetRegionPropertiesRequest_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.tikv.kvproto.Debugpb.GetRegionPropertiesRequest.class, org.tikv.kvproto.Debugpb.GetRegionPropertiesRequest.Builder.class);
}
public static final int REGION_ID_FIELD_NUMBER = 1;
private long regionId_;
/**
* uint64 region_id = 1;
*/
public long getRegionId() {
return regionId_;
}
private byte memoizedIsInitialized = -1;
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (regionId_ != 0L) {
output.writeUInt64(1, regionId_);
}
unknownFields.writeTo(output);
}
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (regionId_ != 0L) {
size += com.google.protobuf.CodedOutputStream
.computeUInt64Size(1, regionId_);
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof org.tikv.kvproto.Debugpb.GetRegionPropertiesRequest)) {
return super.equals(obj);
}
org.tikv.kvproto.Debugpb.GetRegionPropertiesRequest other = (org.tikv.kvproto.Debugpb.GetRegionPropertiesRequest) obj;
boolean result = true;
result = result && (getRegionId()
== other.getRegionId());
result = result && unknownFields.equals(other.unknownFields);
return result;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
hash = (37 * hash) + REGION_ID_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
getRegionId());
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static org.tikv.kvproto.Debugpb.GetRegionPropertiesRequest parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.tikv.kvproto.Debugpb.GetRegionPropertiesRequest parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.tikv.kvproto.Debugpb.GetRegionPropertiesRequest parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.tikv.kvproto.Debugpb.GetRegionPropertiesRequest parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.tikv.kvproto.Debugpb.GetRegionPropertiesRequest parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.tikv.kvproto.Debugpb.GetRegionPropertiesRequest parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.tikv.kvproto.Debugpb.GetRegionPropertiesRequest parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static org.tikv.kvproto.Debugpb.GetRegionPropertiesRequest parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static org.tikv.kvproto.Debugpb.GetRegionPropertiesRequest parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static org.tikv.kvproto.Debugpb.GetRegionPropertiesRequest parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static org.tikv.kvproto.Debugpb.GetRegionPropertiesRequest parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static org.tikv.kvproto.Debugpb.GetRegionPropertiesRequest parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(org.tikv.kvproto.Debugpb.GetRegionPropertiesRequest prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code debugpb.GetRegionPropertiesRequest}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:debugpb.GetRegionPropertiesRequest)
org.tikv.kvproto.Debugpb.GetRegionPropertiesRequestOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.tikv.kvproto.Debugpb.internal_static_debugpb_GetRegionPropertiesRequest_descriptor;
}
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.tikv.kvproto.Debugpb.internal_static_debugpb_GetRegionPropertiesRequest_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.tikv.kvproto.Debugpb.GetRegionPropertiesRequest.class, org.tikv.kvproto.Debugpb.GetRegionPropertiesRequest.Builder.class);
}
// Construct using org.tikv.kvproto.Debugpb.GetRegionPropertiesRequest.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
public Builder clear() {
super.clear();
regionId_ = 0L;
return this;
}
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return org.tikv.kvproto.Debugpb.internal_static_debugpb_GetRegionPropertiesRequest_descriptor;
}
public org.tikv.kvproto.Debugpb.GetRegionPropertiesRequest getDefaultInstanceForType() {
return org.tikv.kvproto.Debugpb.GetRegionPropertiesRequest.getDefaultInstance();
}
public org.tikv.kvproto.Debugpb.GetRegionPropertiesRequest build() {
org.tikv.kvproto.Debugpb.GetRegionPropertiesRequest result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
public org.tikv.kvproto.Debugpb.GetRegionPropertiesRequest buildPartial() {
org.tikv.kvproto.Debugpb.GetRegionPropertiesRequest result = new org.tikv.kvproto.Debugpb.GetRegionPropertiesRequest(this);
result.regionId_ = regionId_;
onBuilt();
return result;
}
public Builder clone() {
return (Builder) super.clone();
}
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.setField(field, value);
}
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return (Builder) super.clearField(field);
}
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return (Builder) super.clearOneof(oneof);
}
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return (Builder) super.setRepeatedField(field, index, value);
}
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.addRepeatedField(field, value);
}
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof org.tikv.kvproto.Debugpb.GetRegionPropertiesRequest) {
return mergeFrom((org.tikv.kvproto.Debugpb.GetRegionPropertiesRequest)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(org.tikv.kvproto.Debugpb.GetRegionPropertiesRequest other) {
if (other == org.tikv.kvproto.Debugpb.GetRegionPropertiesRequest.getDefaultInstance()) return this;
if (other.getRegionId() != 0L) {
setRegionId(other.getRegionId());
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
public final boolean isInitialized() {
return true;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
org.tikv.kvproto.Debugpb.GetRegionPropertiesRequest parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (org.tikv.kvproto.Debugpb.GetRegionPropertiesRequest) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private long regionId_ ;
/**
* uint64 region_id = 1;
*/
public long getRegionId() {
return regionId_;
}
/**
* uint64 region_id = 1;
*/
public Builder setRegionId(long value) {
regionId_ = value;
onChanged();
return this;
}
/**
* uint64 region_id = 1;
*/
public Builder clearRegionId() {
regionId_ = 0L;
onChanged();
return this;
}
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFieldsProto3(unknownFields);
}
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:debugpb.GetRegionPropertiesRequest)
}
// @@protoc_insertion_point(class_scope:debugpb.GetRegionPropertiesRequest)
private static final org.tikv.kvproto.Debugpb.GetRegionPropertiesRequest DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new org.tikv.kvproto.Debugpb.GetRegionPropertiesRequest();
}
public static org.tikv.kvproto.Debugpb.GetRegionPropertiesRequest getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
public GetRegionPropertiesRequest parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new GetRegionPropertiesRequest(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
public org.tikv.kvproto.Debugpb.GetRegionPropertiesRequest getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface GetRegionPropertiesResponseOrBuilder extends
// @@protoc_insertion_point(interface_extends:debugpb.GetRegionPropertiesResponse)
com.google.protobuf.MessageOrBuilder {
/**
* repeated .debugpb.Property props = 1;
*/
java.util.List
getPropsList();
/**
* repeated .debugpb.Property props = 1;
*/
org.tikv.kvproto.Debugpb.Property getProps(int index);
/**
* repeated .debugpb.Property props = 1;
*/
int getPropsCount();
/**
* repeated .debugpb.Property props = 1;
*/
java.util.List extends org.tikv.kvproto.Debugpb.PropertyOrBuilder>
getPropsOrBuilderList();
/**
* repeated .debugpb.Property props = 1;
*/
org.tikv.kvproto.Debugpb.PropertyOrBuilder getPropsOrBuilder(
int index);
}
/**
* Protobuf type {@code debugpb.GetRegionPropertiesResponse}
*/
public static final class GetRegionPropertiesResponse extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:debugpb.GetRegionPropertiesResponse)
GetRegionPropertiesResponseOrBuilder {
private static final long serialVersionUID = 0L;
// Use GetRegionPropertiesResponse.newBuilder() to construct.
private GetRegionPropertiesResponse(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private GetRegionPropertiesResponse() {
props_ = java.util.Collections.emptyList();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private GetRegionPropertiesResponse(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
default: {
if (!parseUnknownFieldProto3(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
case 10: {
if (!((mutable_bitField0_ & 0x00000001) == 0x00000001)) {
props_ = new java.util.ArrayList();
mutable_bitField0_ |= 0x00000001;
}
props_.add(
input.readMessage(org.tikv.kvproto.Debugpb.Property.parser(), extensionRegistry));
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
if (((mutable_bitField0_ & 0x00000001) == 0x00000001)) {
props_ = java.util.Collections.unmodifiableList(props_);
}
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.tikv.kvproto.Debugpb.internal_static_debugpb_GetRegionPropertiesResponse_descriptor;
}
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.tikv.kvproto.Debugpb.internal_static_debugpb_GetRegionPropertiesResponse_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.tikv.kvproto.Debugpb.GetRegionPropertiesResponse.class, org.tikv.kvproto.Debugpb.GetRegionPropertiesResponse.Builder.class);
}
public static final int PROPS_FIELD_NUMBER = 1;
private java.util.List props_;
/**
* repeated .debugpb.Property props = 1;
*/
public java.util.List getPropsList() {
return props_;
}
/**
* repeated .debugpb.Property props = 1;
*/
public java.util.List extends org.tikv.kvproto.Debugpb.PropertyOrBuilder>
getPropsOrBuilderList() {
return props_;
}
/**
* repeated .debugpb.Property props = 1;
*/
public int getPropsCount() {
return props_.size();
}
/**
* repeated .debugpb.Property props = 1;
*/
public org.tikv.kvproto.Debugpb.Property getProps(int index) {
return props_.get(index);
}
/**
* repeated .debugpb.Property props = 1;
*/
public org.tikv.kvproto.Debugpb.PropertyOrBuilder getPropsOrBuilder(
int index) {
return props_.get(index);
}
private byte memoizedIsInitialized = -1;
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
for (int i = 0; i < props_.size(); i++) {
output.writeMessage(1, props_.get(i));
}
unknownFields.writeTo(output);
}
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
for (int i = 0; i < props_.size(); i++) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(1, props_.get(i));
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof org.tikv.kvproto.Debugpb.GetRegionPropertiesResponse)) {
return super.equals(obj);
}
org.tikv.kvproto.Debugpb.GetRegionPropertiesResponse other = (org.tikv.kvproto.Debugpb.GetRegionPropertiesResponse) obj;
boolean result = true;
result = result && getPropsList()
.equals(other.getPropsList());
result = result && unknownFields.equals(other.unknownFields);
return result;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (getPropsCount() > 0) {
hash = (37 * hash) + PROPS_FIELD_NUMBER;
hash = (53 * hash) + getPropsList().hashCode();
}
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static org.tikv.kvproto.Debugpb.GetRegionPropertiesResponse parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.tikv.kvproto.Debugpb.GetRegionPropertiesResponse parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.tikv.kvproto.Debugpb.GetRegionPropertiesResponse parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.tikv.kvproto.Debugpb.GetRegionPropertiesResponse parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.tikv.kvproto.Debugpb.GetRegionPropertiesResponse parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.tikv.kvproto.Debugpb.GetRegionPropertiesResponse parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.tikv.kvproto.Debugpb.GetRegionPropertiesResponse parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static org.tikv.kvproto.Debugpb.GetRegionPropertiesResponse parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static org.tikv.kvproto.Debugpb.GetRegionPropertiesResponse parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static org.tikv.kvproto.Debugpb.GetRegionPropertiesResponse parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static org.tikv.kvproto.Debugpb.GetRegionPropertiesResponse parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static org.tikv.kvproto.Debugpb.GetRegionPropertiesResponse parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(org.tikv.kvproto.Debugpb.GetRegionPropertiesResponse prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code debugpb.GetRegionPropertiesResponse}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:debugpb.GetRegionPropertiesResponse)
org.tikv.kvproto.Debugpb.GetRegionPropertiesResponseOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.tikv.kvproto.Debugpb.internal_static_debugpb_GetRegionPropertiesResponse_descriptor;
}
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.tikv.kvproto.Debugpb.internal_static_debugpb_GetRegionPropertiesResponse_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.tikv.kvproto.Debugpb.GetRegionPropertiesResponse.class, org.tikv.kvproto.Debugpb.GetRegionPropertiesResponse.Builder.class);
}
// Construct using org.tikv.kvproto.Debugpb.GetRegionPropertiesResponse.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
getPropsFieldBuilder();
}
}
public Builder clear() {
super.clear();
if (propsBuilder_ == null) {
props_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000001);
} else {
propsBuilder_.clear();
}
return this;
}
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return org.tikv.kvproto.Debugpb.internal_static_debugpb_GetRegionPropertiesResponse_descriptor;
}
public org.tikv.kvproto.Debugpb.GetRegionPropertiesResponse getDefaultInstanceForType() {
return org.tikv.kvproto.Debugpb.GetRegionPropertiesResponse.getDefaultInstance();
}
public org.tikv.kvproto.Debugpb.GetRegionPropertiesResponse build() {
org.tikv.kvproto.Debugpb.GetRegionPropertiesResponse result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
public org.tikv.kvproto.Debugpb.GetRegionPropertiesResponse buildPartial() {
org.tikv.kvproto.Debugpb.GetRegionPropertiesResponse result = new org.tikv.kvproto.Debugpb.GetRegionPropertiesResponse(this);
int from_bitField0_ = bitField0_;
if (propsBuilder_ == null) {
if (((bitField0_ & 0x00000001) == 0x00000001)) {
props_ = java.util.Collections.unmodifiableList(props_);
bitField0_ = (bitField0_ & ~0x00000001);
}
result.props_ = props_;
} else {
result.props_ = propsBuilder_.build();
}
onBuilt();
return result;
}
public Builder clone() {
return (Builder) super.clone();
}
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.setField(field, value);
}
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return (Builder) super.clearField(field);
}
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return (Builder) super.clearOneof(oneof);
}
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return (Builder) super.setRepeatedField(field, index, value);
}
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.addRepeatedField(field, value);
}
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof org.tikv.kvproto.Debugpb.GetRegionPropertiesResponse) {
return mergeFrom((org.tikv.kvproto.Debugpb.GetRegionPropertiesResponse)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(org.tikv.kvproto.Debugpb.GetRegionPropertiesResponse other) {
if (other == org.tikv.kvproto.Debugpb.GetRegionPropertiesResponse.getDefaultInstance()) return this;
if (propsBuilder_ == null) {
if (!other.props_.isEmpty()) {
if (props_.isEmpty()) {
props_ = other.props_;
bitField0_ = (bitField0_ & ~0x00000001);
} else {
ensurePropsIsMutable();
props_.addAll(other.props_);
}
onChanged();
}
} else {
if (!other.props_.isEmpty()) {
if (propsBuilder_.isEmpty()) {
propsBuilder_.dispose();
propsBuilder_ = null;
props_ = other.props_;
bitField0_ = (bitField0_ & ~0x00000001);
propsBuilder_ =
com.google.protobuf.GeneratedMessageV3.alwaysUseFieldBuilders ?
getPropsFieldBuilder() : null;
} else {
propsBuilder_.addAllMessages(other.props_);
}
}
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
public final boolean isInitialized() {
return true;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
org.tikv.kvproto.Debugpb.GetRegionPropertiesResponse parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (org.tikv.kvproto.Debugpb.GetRegionPropertiesResponse) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
private java.util.List props_ =
java.util.Collections.emptyList();
private void ensurePropsIsMutable() {
if (!((bitField0_ & 0x00000001) == 0x00000001)) {
props_ = new java.util.ArrayList(props_);
bitField0_ |= 0x00000001;
}
}
private com.google.protobuf.RepeatedFieldBuilderV3<
org.tikv.kvproto.Debugpb.Property, org.tikv.kvproto.Debugpb.Property.Builder, org.tikv.kvproto.Debugpb.PropertyOrBuilder> propsBuilder_;
/**
* repeated .debugpb.Property props = 1;
*/
public java.util.List getPropsList() {
if (propsBuilder_ == null) {
return java.util.Collections.unmodifiableList(props_);
} else {
return propsBuilder_.getMessageList();
}
}
/**
* repeated .debugpb.Property props = 1;
*/
public int getPropsCount() {
if (propsBuilder_ == null) {
return props_.size();
} else {
return propsBuilder_.getCount();
}
}
/**
* repeated .debugpb.Property props = 1;
*/
public org.tikv.kvproto.Debugpb.Property getProps(int index) {
if (propsBuilder_ == null) {
return props_.get(index);
} else {
return propsBuilder_.getMessage(index);
}
}
/**
* repeated .debugpb.Property props = 1;
*/
public Builder setProps(
int index, org.tikv.kvproto.Debugpb.Property value) {
if (propsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensurePropsIsMutable();
props_.set(index, value);
onChanged();
} else {
propsBuilder_.setMessage(index, value);
}
return this;
}
/**
* repeated .debugpb.Property props = 1;
*/
public Builder setProps(
int index, org.tikv.kvproto.Debugpb.Property.Builder builderForValue) {
if (propsBuilder_ == null) {
ensurePropsIsMutable();
props_.set(index, builderForValue.build());
onChanged();
} else {
propsBuilder_.setMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .debugpb.Property props = 1;
*/
public Builder addProps(org.tikv.kvproto.Debugpb.Property value) {
if (propsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensurePropsIsMutable();
props_.add(value);
onChanged();
} else {
propsBuilder_.addMessage(value);
}
return this;
}
/**
* repeated .debugpb.Property props = 1;
*/
public Builder addProps(
int index, org.tikv.kvproto.Debugpb.Property value) {
if (propsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensurePropsIsMutable();
props_.add(index, value);
onChanged();
} else {
propsBuilder_.addMessage(index, value);
}
return this;
}
/**
* repeated .debugpb.Property props = 1;
*/
public Builder addProps(
org.tikv.kvproto.Debugpb.Property.Builder builderForValue) {
if (propsBuilder_ == null) {
ensurePropsIsMutable();
props_.add(builderForValue.build());
onChanged();
} else {
propsBuilder_.addMessage(builderForValue.build());
}
return this;
}
/**
* repeated .debugpb.Property props = 1;
*/
public Builder addProps(
int index, org.tikv.kvproto.Debugpb.Property.Builder builderForValue) {
if (propsBuilder_ == null) {
ensurePropsIsMutable();
props_.add(index, builderForValue.build());
onChanged();
} else {
propsBuilder_.addMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .debugpb.Property props = 1;
*/
public Builder addAllProps(
java.lang.Iterable extends org.tikv.kvproto.Debugpb.Property> values) {
if (propsBuilder_ == null) {
ensurePropsIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, props_);
onChanged();
} else {
propsBuilder_.addAllMessages(values);
}
return this;
}
/**
* repeated .debugpb.Property props = 1;
*/
public Builder clearProps() {
if (propsBuilder_ == null) {
props_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000001);
onChanged();
} else {
propsBuilder_.clear();
}
return this;
}
/**
* repeated .debugpb.Property props = 1;
*/
public Builder removeProps(int index) {
if (propsBuilder_ == null) {
ensurePropsIsMutable();
props_.remove(index);
onChanged();
} else {
propsBuilder_.remove(index);
}
return this;
}
/**
* repeated .debugpb.Property props = 1;
*/
public org.tikv.kvproto.Debugpb.Property.Builder getPropsBuilder(
int index) {
return getPropsFieldBuilder().getBuilder(index);
}
/**
* repeated .debugpb.Property props = 1;
*/
public org.tikv.kvproto.Debugpb.PropertyOrBuilder getPropsOrBuilder(
int index) {
if (propsBuilder_ == null) {
return props_.get(index); } else {
return propsBuilder_.getMessageOrBuilder(index);
}
}
/**
* repeated .debugpb.Property props = 1;
*/
public java.util.List extends org.tikv.kvproto.Debugpb.PropertyOrBuilder>
getPropsOrBuilderList() {
if (propsBuilder_ != null) {
return propsBuilder_.getMessageOrBuilderList();
} else {
return java.util.Collections.unmodifiableList(props_);
}
}
/**
* repeated .debugpb.Property props = 1;
*/
public org.tikv.kvproto.Debugpb.Property.Builder addPropsBuilder() {
return getPropsFieldBuilder().addBuilder(
org.tikv.kvproto.Debugpb.Property.getDefaultInstance());
}
/**
* repeated .debugpb.Property props = 1;
*/
public org.tikv.kvproto.Debugpb.Property.Builder addPropsBuilder(
int index) {
return getPropsFieldBuilder().addBuilder(
index, org.tikv.kvproto.Debugpb.Property.getDefaultInstance());
}
/**
* repeated .debugpb.Property props = 1;
*/
public java.util.List
getPropsBuilderList() {
return getPropsFieldBuilder().getBuilderList();
}
private com.google.protobuf.RepeatedFieldBuilderV3<
org.tikv.kvproto.Debugpb.Property, org.tikv.kvproto.Debugpb.Property.Builder, org.tikv.kvproto.Debugpb.PropertyOrBuilder>
getPropsFieldBuilder() {
if (propsBuilder_ == null) {
propsBuilder_ = new com.google.protobuf.RepeatedFieldBuilderV3<
org.tikv.kvproto.Debugpb.Property, org.tikv.kvproto.Debugpb.Property.Builder, org.tikv.kvproto.Debugpb.PropertyOrBuilder>(
props_,
((bitField0_ & 0x00000001) == 0x00000001),
getParentForChildren(),
isClean());
props_ = null;
}
return propsBuilder_;
}
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFieldsProto3(unknownFields);
}
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:debugpb.GetRegionPropertiesResponse)
}
// @@protoc_insertion_point(class_scope:debugpb.GetRegionPropertiesResponse)
private static final org.tikv.kvproto.Debugpb.GetRegionPropertiesResponse DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new org.tikv.kvproto.Debugpb.GetRegionPropertiesResponse();
}
public static org.tikv.kvproto.Debugpb.GetRegionPropertiesResponse getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
public GetRegionPropertiesResponse parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new GetRegionPropertiesResponse(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
public org.tikv.kvproto.Debugpb.GetRegionPropertiesResponse getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface GetStoreInfoRequestOrBuilder extends
// @@protoc_insertion_point(interface_extends:debugpb.GetStoreInfoRequest)
com.google.protobuf.MessageOrBuilder {
}
/**
* Protobuf type {@code debugpb.GetStoreInfoRequest}
*/
public static final class GetStoreInfoRequest extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:debugpb.GetStoreInfoRequest)
GetStoreInfoRequestOrBuilder {
private static final long serialVersionUID = 0L;
// Use GetStoreInfoRequest.newBuilder() to construct.
private GetStoreInfoRequest(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private GetStoreInfoRequest() {
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private GetStoreInfoRequest(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
default: {
if (!parseUnknownFieldProto3(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.tikv.kvproto.Debugpb.internal_static_debugpb_GetStoreInfoRequest_descriptor;
}
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.tikv.kvproto.Debugpb.internal_static_debugpb_GetStoreInfoRequest_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.tikv.kvproto.Debugpb.GetStoreInfoRequest.class, org.tikv.kvproto.Debugpb.GetStoreInfoRequest.Builder.class);
}
private byte memoizedIsInitialized = -1;
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
unknownFields.writeTo(output);
}
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof org.tikv.kvproto.Debugpb.GetStoreInfoRequest)) {
return super.equals(obj);
}
org.tikv.kvproto.Debugpb.GetStoreInfoRequest other = (org.tikv.kvproto.Debugpb.GetStoreInfoRequest) obj;
boolean result = true;
result = result && unknownFields.equals(other.unknownFields);
return result;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static org.tikv.kvproto.Debugpb.GetStoreInfoRequest parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.tikv.kvproto.Debugpb.GetStoreInfoRequest parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.tikv.kvproto.Debugpb.GetStoreInfoRequest parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.tikv.kvproto.Debugpb.GetStoreInfoRequest parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.tikv.kvproto.Debugpb.GetStoreInfoRequest parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.tikv.kvproto.Debugpb.GetStoreInfoRequest parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.tikv.kvproto.Debugpb.GetStoreInfoRequest parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static org.tikv.kvproto.Debugpb.GetStoreInfoRequest parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static org.tikv.kvproto.Debugpb.GetStoreInfoRequest parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static org.tikv.kvproto.Debugpb.GetStoreInfoRequest parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static org.tikv.kvproto.Debugpb.GetStoreInfoRequest parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static org.tikv.kvproto.Debugpb.GetStoreInfoRequest parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(org.tikv.kvproto.Debugpb.GetStoreInfoRequest prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code debugpb.GetStoreInfoRequest}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:debugpb.GetStoreInfoRequest)
org.tikv.kvproto.Debugpb.GetStoreInfoRequestOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.tikv.kvproto.Debugpb.internal_static_debugpb_GetStoreInfoRequest_descriptor;
}
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.tikv.kvproto.Debugpb.internal_static_debugpb_GetStoreInfoRequest_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.tikv.kvproto.Debugpb.GetStoreInfoRequest.class, org.tikv.kvproto.Debugpb.GetStoreInfoRequest.Builder.class);
}
// Construct using org.tikv.kvproto.Debugpb.GetStoreInfoRequest.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
public Builder clear() {
super.clear();
return this;
}
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return org.tikv.kvproto.Debugpb.internal_static_debugpb_GetStoreInfoRequest_descriptor;
}
public org.tikv.kvproto.Debugpb.GetStoreInfoRequest getDefaultInstanceForType() {
return org.tikv.kvproto.Debugpb.GetStoreInfoRequest.getDefaultInstance();
}
public org.tikv.kvproto.Debugpb.GetStoreInfoRequest build() {
org.tikv.kvproto.Debugpb.GetStoreInfoRequest result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
public org.tikv.kvproto.Debugpb.GetStoreInfoRequest buildPartial() {
org.tikv.kvproto.Debugpb.GetStoreInfoRequest result = new org.tikv.kvproto.Debugpb.GetStoreInfoRequest(this);
onBuilt();
return result;
}
public Builder clone() {
return (Builder) super.clone();
}
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.setField(field, value);
}
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return (Builder) super.clearField(field);
}
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return (Builder) super.clearOneof(oneof);
}
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return (Builder) super.setRepeatedField(field, index, value);
}
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.addRepeatedField(field, value);
}
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof org.tikv.kvproto.Debugpb.GetStoreInfoRequest) {
return mergeFrom((org.tikv.kvproto.Debugpb.GetStoreInfoRequest)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(org.tikv.kvproto.Debugpb.GetStoreInfoRequest other) {
if (other == org.tikv.kvproto.Debugpb.GetStoreInfoRequest.getDefaultInstance()) return this;
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
public final boolean isInitialized() {
return true;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
org.tikv.kvproto.Debugpb.GetStoreInfoRequest parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (org.tikv.kvproto.Debugpb.GetStoreInfoRequest) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFieldsProto3(unknownFields);
}
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:debugpb.GetStoreInfoRequest)
}
// @@protoc_insertion_point(class_scope:debugpb.GetStoreInfoRequest)
private static final org.tikv.kvproto.Debugpb.GetStoreInfoRequest DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new org.tikv.kvproto.Debugpb.GetStoreInfoRequest();
}
public static org.tikv.kvproto.Debugpb.GetStoreInfoRequest getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
public GetStoreInfoRequest parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new GetStoreInfoRequest(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
public org.tikv.kvproto.Debugpb.GetStoreInfoRequest getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface GetStoreInfoResponseOrBuilder extends
// @@protoc_insertion_point(interface_extends:debugpb.GetStoreInfoResponse)
com.google.protobuf.MessageOrBuilder {
/**
* uint64 store_id = 1;
*/
long getStoreId();
}
/**
* Protobuf type {@code debugpb.GetStoreInfoResponse}
*/
public static final class GetStoreInfoResponse extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:debugpb.GetStoreInfoResponse)
GetStoreInfoResponseOrBuilder {
private static final long serialVersionUID = 0L;
// Use GetStoreInfoResponse.newBuilder() to construct.
private GetStoreInfoResponse(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private GetStoreInfoResponse() {
storeId_ = 0L;
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private GetStoreInfoResponse(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
default: {
if (!parseUnknownFieldProto3(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
case 8: {
storeId_ = input.readUInt64();
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.tikv.kvproto.Debugpb.internal_static_debugpb_GetStoreInfoResponse_descriptor;
}
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.tikv.kvproto.Debugpb.internal_static_debugpb_GetStoreInfoResponse_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.tikv.kvproto.Debugpb.GetStoreInfoResponse.class, org.tikv.kvproto.Debugpb.GetStoreInfoResponse.Builder.class);
}
public static final int STORE_ID_FIELD_NUMBER = 1;
private long storeId_;
/**
* uint64 store_id = 1;
*/
public long getStoreId() {
return storeId_;
}
private byte memoizedIsInitialized = -1;
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (storeId_ != 0L) {
output.writeUInt64(1, storeId_);
}
unknownFields.writeTo(output);
}
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (storeId_ != 0L) {
size += com.google.protobuf.CodedOutputStream
.computeUInt64Size(1, storeId_);
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof org.tikv.kvproto.Debugpb.GetStoreInfoResponse)) {
return super.equals(obj);
}
org.tikv.kvproto.Debugpb.GetStoreInfoResponse other = (org.tikv.kvproto.Debugpb.GetStoreInfoResponse) obj;
boolean result = true;
result = result && (getStoreId()
== other.getStoreId());
result = result && unknownFields.equals(other.unknownFields);
return result;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
hash = (37 * hash) + STORE_ID_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
getStoreId());
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static org.tikv.kvproto.Debugpb.GetStoreInfoResponse parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.tikv.kvproto.Debugpb.GetStoreInfoResponse parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.tikv.kvproto.Debugpb.GetStoreInfoResponse parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.tikv.kvproto.Debugpb.GetStoreInfoResponse parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.tikv.kvproto.Debugpb.GetStoreInfoResponse parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.tikv.kvproto.Debugpb.GetStoreInfoResponse parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.tikv.kvproto.Debugpb.GetStoreInfoResponse parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static org.tikv.kvproto.Debugpb.GetStoreInfoResponse parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static org.tikv.kvproto.Debugpb.GetStoreInfoResponse parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static org.tikv.kvproto.Debugpb.GetStoreInfoResponse parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static org.tikv.kvproto.Debugpb.GetStoreInfoResponse parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static org.tikv.kvproto.Debugpb.GetStoreInfoResponse parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(org.tikv.kvproto.Debugpb.GetStoreInfoResponse prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code debugpb.GetStoreInfoResponse}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:debugpb.GetStoreInfoResponse)
org.tikv.kvproto.Debugpb.GetStoreInfoResponseOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.tikv.kvproto.Debugpb.internal_static_debugpb_GetStoreInfoResponse_descriptor;
}
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.tikv.kvproto.Debugpb.internal_static_debugpb_GetStoreInfoResponse_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.tikv.kvproto.Debugpb.GetStoreInfoResponse.class, org.tikv.kvproto.Debugpb.GetStoreInfoResponse.Builder.class);
}
// Construct using org.tikv.kvproto.Debugpb.GetStoreInfoResponse.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
public Builder clear() {
super.clear();
storeId_ = 0L;
return this;
}
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return org.tikv.kvproto.Debugpb.internal_static_debugpb_GetStoreInfoResponse_descriptor;
}
public org.tikv.kvproto.Debugpb.GetStoreInfoResponse getDefaultInstanceForType() {
return org.tikv.kvproto.Debugpb.GetStoreInfoResponse.getDefaultInstance();
}
public org.tikv.kvproto.Debugpb.GetStoreInfoResponse build() {
org.tikv.kvproto.Debugpb.GetStoreInfoResponse result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
public org.tikv.kvproto.Debugpb.GetStoreInfoResponse buildPartial() {
org.tikv.kvproto.Debugpb.GetStoreInfoResponse result = new org.tikv.kvproto.Debugpb.GetStoreInfoResponse(this);
result.storeId_ = storeId_;
onBuilt();
return result;
}
public Builder clone() {
return (Builder) super.clone();
}
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.setField(field, value);
}
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return (Builder) super.clearField(field);
}
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return (Builder) super.clearOneof(oneof);
}
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return (Builder) super.setRepeatedField(field, index, value);
}
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.addRepeatedField(field, value);
}
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof org.tikv.kvproto.Debugpb.GetStoreInfoResponse) {
return mergeFrom((org.tikv.kvproto.Debugpb.GetStoreInfoResponse)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(org.tikv.kvproto.Debugpb.GetStoreInfoResponse other) {
if (other == org.tikv.kvproto.Debugpb.GetStoreInfoResponse.getDefaultInstance()) return this;
if (other.getStoreId() != 0L) {
setStoreId(other.getStoreId());
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
public final boolean isInitialized() {
return true;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
org.tikv.kvproto.Debugpb.GetStoreInfoResponse parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (org.tikv.kvproto.Debugpb.GetStoreInfoResponse) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private long storeId_ ;
/**
* uint64 store_id = 1;
*/
public long getStoreId() {
return storeId_;
}
/**
* uint64 store_id = 1;
*/
public Builder setStoreId(long value) {
storeId_ = value;
onChanged();
return this;
}
/**
* uint64 store_id = 1;
*/
public Builder clearStoreId() {
storeId_ = 0L;
onChanged();
return this;
}
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFieldsProto3(unknownFields);
}
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:debugpb.GetStoreInfoResponse)
}
// @@protoc_insertion_point(class_scope:debugpb.GetStoreInfoResponse)
private static final org.tikv.kvproto.Debugpb.GetStoreInfoResponse DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new org.tikv.kvproto.Debugpb.GetStoreInfoResponse();
}
public static org.tikv.kvproto.Debugpb.GetStoreInfoResponse getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
public GetStoreInfoResponse parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new GetStoreInfoResponse(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
public org.tikv.kvproto.Debugpb.GetStoreInfoResponse getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface GetClusterInfoRequestOrBuilder extends
// @@protoc_insertion_point(interface_extends:debugpb.GetClusterInfoRequest)
com.google.protobuf.MessageOrBuilder {
}
/**
* Protobuf type {@code debugpb.GetClusterInfoRequest}
*/
public static final class GetClusterInfoRequest extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:debugpb.GetClusterInfoRequest)
GetClusterInfoRequestOrBuilder {
private static final long serialVersionUID = 0L;
// Use GetClusterInfoRequest.newBuilder() to construct.
private GetClusterInfoRequest(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private GetClusterInfoRequest() {
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private GetClusterInfoRequest(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
default: {
if (!parseUnknownFieldProto3(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.tikv.kvproto.Debugpb.internal_static_debugpb_GetClusterInfoRequest_descriptor;
}
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.tikv.kvproto.Debugpb.internal_static_debugpb_GetClusterInfoRequest_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.tikv.kvproto.Debugpb.GetClusterInfoRequest.class, org.tikv.kvproto.Debugpb.GetClusterInfoRequest.Builder.class);
}
private byte memoizedIsInitialized = -1;
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
unknownFields.writeTo(output);
}
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof org.tikv.kvproto.Debugpb.GetClusterInfoRequest)) {
return super.equals(obj);
}
org.tikv.kvproto.Debugpb.GetClusterInfoRequest other = (org.tikv.kvproto.Debugpb.GetClusterInfoRequest) obj;
boolean result = true;
result = result && unknownFields.equals(other.unknownFields);
return result;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static org.tikv.kvproto.Debugpb.GetClusterInfoRequest parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.tikv.kvproto.Debugpb.GetClusterInfoRequest parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.tikv.kvproto.Debugpb.GetClusterInfoRequest parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.tikv.kvproto.Debugpb.GetClusterInfoRequest parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.tikv.kvproto.Debugpb.GetClusterInfoRequest parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.tikv.kvproto.Debugpb.GetClusterInfoRequest parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.tikv.kvproto.Debugpb.GetClusterInfoRequest parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static org.tikv.kvproto.Debugpb.GetClusterInfoRequest parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static org.tikv.kvproto.Debugpb.GetClusterInfoRequest parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static org.tikv.kvproto.Debugpb.GetClusterInfoRequest parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static org.tikv.kvproto.Debugpb.GetClusterInfoRequest parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static org.tikv.kvproto.Debugpb.GetClusterInfoRequest parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(org.tikv.kvproto.Debugpb.GetClusterInfoRequest prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code debugpb.GetClusterInfoRequest}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:debugpb.GetClusterInfoRequest)
org.tikv.kvproto.Debugpb.GetClusterInfoRequestOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.tikv.kvproto.Debugpb.internal_static_debugpb_GetClusterInfoRequest_descriptor;
}
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.tikv.kvproto.Debugpb.internal_static_debugpb_GetClusterInfoRequest_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.tikv.kvproto.Debugpb.GetClusterInfoRequest.class, org.tikv.kvproto.Debugpb.GetClusterInfoRequest.Builder.class);
}
// Construct using org.tikv.kvproto.Debugpb.GetClusterInfoRequest.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
public Builder clear() {
super.clear();
return this;
}
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return org.tikv.kvproto.Debugpb.internal_static_debugpb_GetClusterInfoRequest_descriptor;
}
public org.tikv.kvproto.Debugpb.GetClusterInfoRequest getDefaultInstanceForType() {
return org.tikv.kvproto.Debugpb.GetClusterInfoRequest.getDefaultInstance();
}
public org.tikv.kvproto.Debugpb.GetClusterInfoRequest build() {
org.tikv.kvproto.Debugpb.GetClusterInfoRequest result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
public org.tikv.kvproto.Debugpb.GetClusterInfoRequest buildPartial() {
org.tikv.kvproto.Debugpb.GetClusterInfoRequest result = new org.tikv.kvproto.Debugpb.GetClusterInfoRequest(this);
onBuilt();
return result;
}
public Builder clone() {
return (Builder) super.clone();
}
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.setField(field, value);
}
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return (Builder) super.clearField(field);
}
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return (Builder) super.clearOneof(oneof);
}
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return (Builder) super.setRepeatedField(field, index, value);
}
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.addRepeatedField(field, value);
}
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof org.tikv.kvproto.Debugpb.GetClusterInfoRequest) {
return mergeFrom((org.tikv.kvproto.Debugpb.GetClusterInfoRequest)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(org.tikv.kvproto.Debugpb.GetClusterInfoRequest other) {
if (other == org.tikv.kvproto.Debugpb.GetClusterInfoRequest.getDefaultInstance()) return this;
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
public final boolean isInitialized() {
return true;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
org.tikv.kvproto.Debugpb.GetClusterInfoRequest parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (org.tikv.kvproto.Debugpb.GetClusterInfoRequest) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFieldsProto3(unknownFields);
}
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:debugpb.GetClusterInfoRequest)
}
// @@protoc_insertion_point(class_scope:debugpb.GetClusterInfoRequest)
private static final org.tikv.kvproto.Debugpb.GetClusterInfoRequest DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new org.tikv.kvproto.Debugpb.GetClusterInfoRequest();
}
public static org.tikv.kvproto.Debugpb.GetClusterInfoRequest getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
public GetClusterInfoRequest parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new GetClusterInfoRequest(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
public org.tikv.kvproto.Debugpb.GetClusterInfoRequest getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface GetClusterInfoResponseOrBuilder extends
// @@protoc_insertion_point(interface_extends:debugpb.GetClusterInfoResponse)
com.google.protobuf.MessageOrBuilder {
/**
* uint64 cluster_id = 1;
*/
long getClusterId();
}
/**
* Protobuf type {@code debugpb.GetClusterInfoResponse}
*/
public static final class GetClusterInfoResponse extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:debugpb.GetClusterInfoResponse)
GetClusterInfoResponseOrBuilder {
private static final long serialVersionUID = 0L;
// Use GetClusterInfoResponse.newBuilder() to construct.
private GetClusterInfoResponse(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private GetClusterInfoResponse() {
clusterId_ = 0L;
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private GetClusterInfoResponse(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
default: {
if (!parseUnknownFieldProto3(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
case 8: {
clusterId_ = input.readUInt64();
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.tikv.kvproto.Debugpb.internal_static_debugpb_GetClusterInfoResponse_descriptor;
}
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.tikv.kvproto.Debugpb.internal_static_debugpb_GetClusterInfoResponse_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.tikv.kvproto.Debugpb.GetClusterInfoResponse.class, org.tikv.kvproto.Debugpb.GetClusterInfoResponse.Builder.class);
}
public static final int CLUSTER_ID_FIELD_NUMBER = 1;
private long clusterId_;
/**
* uint64 cluster_id = 1;
*/
public long getClusterId() {
return clusterId_;
}
private byte memoizedIsInitialized = -1;
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (clusterId_ != 0L) {
output.writeUInt64(1, clusterId_);
}
unknownFields.writeTo(output);
}
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (clusterId_ != 0L) {
size += com.google.protobuf.CodedOutputStream
.computeUInt64Size(1, clusterId_);
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof org.tikv.kvproto.Debugpb.GetClusterInfoResponse)) {
return super.equals(obj);
}
org.tikv.kvproto.Debugpb.GetClusterInfoResponse other = (org.tikv.kvproto.Debugpb.GetClusterInfoResponse) obj;
boolean result = true;
result = result && (getClusterId()
== other.getClusterId());
result = result && unknownFields.equals(other.unknownFields);
return result;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
hash = (37 * hash) + CLUSTER_ID_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
getClusterId());
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static org.tikv.kvproto.Debugpb.GetClusterInfoResponse parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.tikv.kvproto.Debugpb.GetClusterInfoResponse parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.tikv.kvproto.Debugpb.GetClusterInfoResponse parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.tikv.kvproto.Debugpb.GetClusterInfoResponse parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.tikv.kvproto.Debugpb.GetClusterInfoResponse parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.tikv.kvproto.Debugpb.GetClusterInfoResponse parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.tikv.kvproto.Debugpb.GetClusterInfoResponse parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static org.tikv.kvproto.Debugpb.GetClusterInfoResponse parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static org.tikv.kvproto.Debugpb.GetClusterInfoResponse parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static org.tikv.kvproto.Debugpb.GetClusterInfoResponse parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static org.tikv.kvproto.Debugpb.GetClusterInfoResponse parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static org.tikv.kvproto.Debugpb.GetClusterInfoResponse parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(org.tikv.kvproto.Debugpb.GetClusterInfoResponse prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code debugpb.GetClusterInfoResponse}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:debugpb.GetClusterInfoResponse)
org.tikv.kvproto.Debugpb.GetClusterInfoResponseOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.tikv.kvproto.Debugpb.internal_static_debugpb_GetClusterInfoResponse_descriptor;
}
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.tikv.kvproto.Debugpb.internal_static_debugpb_GetClusterInfoResponse_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.tikv.kvproto.Debugpb.GetClusterInfoResponse.class, org.tikv.kvproto.Debugpb.GetClusterInfoResponse.Builder.class);
}
// Construct using org.tikv.kvproto.Debugpb.GetClusterInfoResponse.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
public Builder clear() {
super.clear();
clusterId_ = 0L;
return this;
}
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return org.tikv.kvproto.Debugpb.internal_static_debugpb_GetClusterInfoResponse_descriptor;
}
public org.tikv.kvproto.Debugpb.GetClusterInfoResponse getDefaultInstanceForType() {
return org.tikv.kvproto.Debugpb.GetClusterInfoResponse.getDefaultInstance();
}
public org.tikv.kvproto.Debugpb.GetClusterInfoResponse build() {
org.tikv.kvproto.Debugpb.GetClusterInfoResponse result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
public org.tikv.kvproto.Debugpb.GetClusterInfoResponse buildPartial() {
org.tikv.kvproto.Debugpb.GetClusterInfoResponse result = new org.tikv.kvproto.Debugpb.GetClusterInfoResponse(this);
result.clusterId_ = clusterId_;
onBuilt();
return result;
}
public Builder clone() {
return (Builder) super.clone();
}
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.setField(field, value);
}
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return (Builder) super.clearField(field);
}
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return (Builder) super.clearOneof(oneof);
}
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return (Builder) super.setRepeatedField(field, index, value);
}
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.addRepeatedField(field, value);
}
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof org.tikv.kvproto.Debugpb.GetClusterInfoResponse) {
return mergeFrom((org.tikv.kvproto.Debugpb.GetClusterInfoResponse)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(org.tikv.kvproto.Debugpb.GetClusterInfoResponse other) {
if (other == org.tikv.kvproto.Debugpb.GetClusterInfoResponse.getDefaultInstance()) return this;
if (other.getClusterId() != 0L) {
setClusterId(other.getClusterId());
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
public final boolean isInitialized() {
return true;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
org.tikv.kvproto.Debugpb.GetClusterInfoResponse parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (org.tikv.kvproto.Debugpb.GetClusterInfoResponse) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private long clusterId_ ;
/**
* uint64 cluster_id = 1;
*/
public long getClusterId() {
return clusterId_;
}
/**
* uint64 cluster_id = 1;
*/
public Builder setClusterId(long value) {
clusterId_ = value;
onChanged();
return this;
}
/**
* uint64 cluster_id = 1;
*/
public Builder clearClusterId() {
clusterId_ = 0L;
onChanged();
return this;
}
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFieldsProto3(unknownFields);
}
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:debugpb.GetClusterInfoResponse)
}
// @@protoc_insertion_point(class_scope:debugpb.GetClusterInfoResponse)
private static final org.tikv.kvproto.Debugpb.GetClusterInfoResponse DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new org.tikv.kvproto.Debugpb.GetClusterInfoResponse();
}
public static org.tikv.kvproto.Debugpb.GetClusterInfoResponse getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
public GetClusterInfoResponse parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new GetClusterInfoResponse(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
public org.tikv.kvproto.Debugpb.GetClusterInfoResponse getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_debugpb_GetRequest_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_debugpb_GetRequest_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_debugpb_GetResponse_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_debugpb_GetResponse_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_debugpb_RaftLogRequest_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_debugpb_RaftLogRequest_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_debugpb_RaftLogResponse_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_debugpb_RaftLogResponse_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_debugpb_RegionInfoRequest_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_debugpb_RegionInfoRequest_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_debugpb_RegionInfoResponse_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_debugpb_RegionInfoResponse_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_debugpb_RegionSizeRequest_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_debugpb_RegionSizeRequest_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_debugpb_RegionSizeResponse_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_debugpb_RegionSizeResponse_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_debugpb_RegionSizeResponse_Entry_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_debugpb_RegionSizeResponse_Entry_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_debugpb_ScanMvccRequest_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_debugpb_ScanMvccRequest_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_debugpb_ScanMvccResponse_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_debugpb_ScanMvccResponse_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_debugpb_CompactRequest_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_debugpb_CompactRequest_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_debugpb_CompactResponse_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_debugpb_CompactResponse_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_debugpb_InjectFailPointRequest_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_debugpb_InjectFailPointRequest_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_debugpb_InjectFailPointResponse_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_debugpb_InjectFailPointResponse_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_debugpb_RecoverFailPointRequest_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_debugpb_RecoverFailPointRequest_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_debugpb_RecoverFailPointResponse_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_debugpb_RecoverFailPointResponse_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_debugpb_ListFailPointsRequest_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_debugpb_ListFailPointsRequest_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_debugpb_ListFailPointsResponse_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_debugpb_ListFailPointsResponse_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_debugpb_ListFailPointsResponse_Entry_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_debugpb_ListFailPointsResponse_Entry_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_debugpb_GetMetricsRequest_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_debugpb_GetMetricsRequest_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_debugpb_GetMetricsResponse_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_debugpb_GetMetricsResponse_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_debugpb_RegionConsistencyCheckRequest_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_debugpb_RegionConsistencyCheckRequest_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_debugpb_RegionConsistencyCheckResponse_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_debugpb_RegionConsistencyCheckResponse_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_debugpb_ModifyTikvConfigRequest_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_debugpb_ModifyTikvConfigRequest_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_debugpb_ModifyTikvConfigResponse_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_debugpb_ModifyTikvConfigResponse_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_debugpb_Property_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_debugpb_Property_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_debugpb_GetRegionPropertiesRequest_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_debugpb_GetRegionPropertiesRequest_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_debugpb_GetRegionPropertiesResponse_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_debugpb_GetRegionPropertiesResponse_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_debugpb_GetStoreInfoRequest_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_debugpb_GetStoreInfoRequest_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_debugpb_GetStoreInfoResponse_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_debugpb_GetStoreInfoResponse_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_debugpb_GetClusterInfoRequest_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_debugpb_GetClusterInfoRequest_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_debugpb_GetClusterInfoResponse_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_debugpb_GetClusterInfoResponse_fieldAccessorTable;
public static com.google.protobuf.Descriptors.FileDescriptor
getDescriptor() {
return descriptor;
}
private static com.google.protobuf.Descriptors.FileDescriptor
descriptor;
static {
java.lang.String[] descriptorData = {
"\n\rdebugpb.proto\022\007debugpb\032\reraftpb.proto\032" +
"\rkvrpcpb.proto\032\023raft_serverpb.proto\032\024gog" +
"oproto/gogo.proto\032\017rustproto.proto\">\n\nGe" +
"tRequest\022\027\n\002db\030\001 \001(\0162\013.debugpb.DB\022\n\n\002cf\030" +
"\002 \001(\t\022\013\n\003key\030\003 \001(\014\"\034\n\013GetResponse\022\r\n\005val" +
"ue\030\001 \001(\014\"6\n\016RaftLogRequest\022\021\n\tregion_id\030" +
"\001 \001(\004\022\021\n\tlog_index\030\002 \001(\004\"0\n\017RaftLogRespo" +
"nse\022\035\n\005entry\030\001 \001(\0132\016.eraftpb.Entry\"&\n\021Re" +
"gionInfoRequest\022\021\n\tregion_id\030\001 \001(\004\"\303\001\n\022R" +
"egionInfoResponse\0227\n\020raft_local_state\030\001 " +
"\001(\0132\035.raft_serverpb.RaftLocalState\0227\n\020ra" +
"ft_apply_state\030\002 \001(\0132\035.raft_serverpb.Raf" +
"tApplyState\022;\n\022region_local_state\030\003 \001(\0132" +
"\037.raft_serverpb.RegionLocalState\"3\n\021Regi" +
"onSizeRequest\022\021\n\tregion_id\030\001 \001(\004\022\013\n\003cfs\030" +
"\002 \003(\t\"k\n\022RegionSizeResponse\0222\n\007entries\030\001" +
" \003(\0132!.debugpb.RegionSizeResponse.Entry\032" +
"!\n\005Entry\022\n\n\002cf\030\001 \001(\t\022\014\n\004size\030\002 \001(\004\"B\n\017Sc" +
"anMvccRequest\022\020\n\010from_key\030\001 \001(\014\022\016\n\006to_ke" +
"y\030\002 \001(\014\022\r\n\005limit\030\003 \001(\004\"@\n\020ScanMvccRespon" +
"se\022\013\n\003key\030\001 \001(\014\022\037\n\004info\030\002 \001(\0132\021.kvrpcpb." +
"MvccInfo\"\261\001\n\016CompactRequest\022\027\n\002db\030\001 \001(\0162" +
"\013.debugpb.DB\022\n\n\002cf\030\002 \001(\t\022\020\n\010from_key\030\003 \001" +
"(\014\022\016\n\006to_key\030\004 \001(\014\022\017\n\007threads\030\005 \001(\r\022G\n\033b" +
"ottommost_level_compaction\030\006 \001(\0162\".debug" +
"pb.BottommostLevelCompaction\"\021\n\017CompactR" +
"esponse\"7\n\026InjectFailPointRequest\022\014\n\004nam" +
"e\030\001 \001(\t\022\017\n\007actions\030\002 \001(\t\"\031\n\027InjectFailPo" +
"intResponse\"\'\n\027RecoverFailPointRequest\022\014" +
"\n\004name\030\001 \001(\t\"\032\n\030RecoverFailPointResponse" +
"\"\027\n\025ListFailPointsRequest\"x\n\026ListFailPoi" +
"ntsResponse\0226\n\007entries\030\001 \003(\0132%.debugpb.L" +
"istFailPointsResponse.Entry\032&\n\005Entry\022\014\n\004" +
"name\030\001 \001(\t\022\017\n\007actions\030\002 \001(\t\" \n\021GetMetric" +
"sRequest\022\013\n\003all\030\001 \001(\010\"v\n\022GetMetricsRespo" +
"nse\022\022\n\nprometheus\030\001 \001(\t\022\022\n\nrocksdb_kv\030\002 " +
"\001(\t\022\024\n\014rocksdb_raft\030\003 \001(\t\022\020\n\010jemalloc\030\004 " +
"\001(\t\022\020\n\010store_id\030\005 \001(\004\"2\n\035RegionConsisten" +
"cyCheckRequest\022\021\n\tregion_id\030\001 \001(\004\" \n\036Reg" +
"ionConsistencyCheckResponse\"e\n\027ModifyTik" +
"vConfigRequest\022\037\n\006module\030\001 \001(\0162\017.debugpb" +
".MODULE\022\023\n\013config_name\030\002 \001(\t\022\024\n\014config_v" +
"alue\030\003 \001(\t\"\032\n\030ModifyTikvConfigResponse\"\'" +
"\n\010Property\022\014\n\004name\030\001 \001(\t\022\r\n\005value\030\002 \001(\t\"" +
"/\n\032GetRegionPropertiesRequest\022\021\n\tregion_" +
"id\030\001 \001(\004\"?\n\033GetRegionPropertiesResponse\022" +
" \n\005props\030\001 \003(\0132\021.debugpb.Property\"\025\n\023Get" +
"StoreInfoRequest\"(\n\024GetStoreInfoResponse" +
"\022\020\n\010store_id\030\001 \001(\004\"\027\n\025GetClusterInfoRequ" +
"est\",\n\026GetClusterInfoResponse\022\022\n\ncluster" +
"_id\030\001 \001(\004*#\n\002DB\022\013\n\007INVALID\020\000\022\006\n\002KV\020\001\022\010\n\004" +
"RAFT\020\002*\220\001\n\006MODULE\022\n\n\006UNUSED\020\000\022\010\n\004KVDB\020\001\022" +
"\n\n\006RAFTDB\020\002\022\014\n\010READPOOL\020\003\022\n\n\006SERVER\020\004\022\013\n" +
"\007STORAGE\020\005\022\006\n\002PD\020\006\022\n\n\006METRIC\020\007\022\017\n\013COPROC" +
"ESSOR\020\010\022\014\n\010SECURITY\020\t\022\n\n\006IMPORT\020\n*L\n\031Bot" +
"tommostLevelCompaction\022\010\n\004Skip\020\000\022\t\n\005Forc" +
"e\020\001\022\032\n\026IfHaveCompactionFilter\020\0022\263\t\n\005Debu" +
"g\0222\n\003Get\022\023.debugpb.GetRequest\032\024.debugpb." +
"GetResponse\"\000\022>\n\007RaftLog\022\027.debugpb.RaftL" +
"ogRequest\032\030.debugpb.RaftLogResponse\"\000\022G\n" +
"\nRegionInfo\022\032.debugpb.RegionInfoRequest\032" +
"\033.debugpb.RegionInfoResponse\"\000\022G\n\nRegion" +
"Size\022\032.debugpb.RegionSizeRequest\032\033.debug" +
"pb.RegionSizeResponse\"\000\022C\n\010ScanMvcc\022\030.de" +
"bugpb.ScanMvccRequest\032\031.debugpb.ScanMvcc" +
"Response\"\0000\001\022>\n\007Compact\022\027.debugpb.Compac" +
"tRequest\032\030.debugpb.CompactResponse\"\000\022V\n\017" +
"InjectFailPoint\022\037.debugpb.InjectFailPoin" +
"tRequest\032 .debugpb.InjectFailPointRespon" +
"se\"\000\022Y\n\020RecoverFailPoint\022 .debugpb.Recov" +
"erFailPointRequest\032!.debugpb.RecoverFail" +
"PointResponse\"\000\022S\n\016ListFailPoints\022\036.debu" +
"gpb.ListFailPointsRequest\032\037.debugpb.List" +
"FailPointsResponse\"\000\022G\n\nGetMetrics\022\032.deb" +
"ugpb.GetMetricsRequest\032\033.debugpb.GetMetr" +
"icsResponse\"\000\022k\n\026CheckRegionConsistency\022" +
"&.debugpb.RegionConsistencyCheckRequest\032" +
"\'.debugpb.RegionConsistencyCheckResponse" +
"\"\000\022Y\n\020ModifyTikvConfig\022 .debugpb.ModifyT" +
"ikvConfigRequest\032!.debugpb.ModifyTikvCon" +
"figResponse\"\000\022b\n\023GetRegionProperties\022#.d" +
"ebugpb.GetRegionPropertiesRequest\032$.debu" +
"gpb.GetRegionPropertiesResponse\"\000\022M\n\014Get" +
"StoreInfo\022\034.debugpb.GetStoreInfoRequest\032" +
"\035.debugpb.GetStoreInfoResponse\"\000\022S\n\016GetC" +
"lusterInfo\022\036.debugpb.GetClusterInfoReque" +
"st\032\037.debugpb.GetClusterInfoResponse\"\000B\"\n" +
"\020org.tikv.kvproto\340\342\036\001\310\342\036\001\320\342\036\001\330\250\010\001b\006proto" +
"3"
};
com.google.protobuf.Descriptors.FileDescriptor.InternalDescriptorAssigner assigner =
new com.google.protobuf.Descriptors.FileDescriptor. InternalDescriptorAssigner() {
public com.google.protobuf.ExtensionRegistry assignDescriptors(
com.google.protobuf.Descriptors.FileDescriptor root) {
descriptor = root;
return null;
}
};
com.google.protobuf.Descriptors.FileDescriptor
.internalBuildGeneratedFileFrom(descriptorData,
new com.google.protobuf.Descriptors.FileDescriptor[] {
eraftpb.Eraftpb.getDescriptor(),
org.tikv.kvproto.Kvrpcpb.getDescriptor(),
org.tikv.kvproto.RaftServerpb.getDescriptor(),
com.google.protobuf.GoGoProtos.getDescriptor(),
rustproto.Rustproto.getDescriptor(),
}, assigner);
internal_static_debugpb_GetRequest_descriptor =
getDescriptor().getMessageTypes().get(0);
internal_static_debugpb_GetRequest_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_debugpb_GetRequest_descriptor,
new java.lang.String[] { "Db", "Cf", "Key", });
internal_static_debugpb_GetResponse_descriptor =
getDescriptor().getMessageTypes().get(1);
internal_static_debugpb_GetResponse_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_debugpb_GetResponse_descriptor,
new java.lang.String[] { "Value", });
internal_static_debugpb_RaftLogRequest_descriptor =
getDescriptor().getMessageTypes().get(2);
internal_static_debugpb_RaftLogRequest_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_debugpb_RaftLogRequest_descriptor,
new java.lang.String[] { "RegionId", "LogIndex", });
internal_static_debugpb_RaftLogResponse_descriptor =
getDescriptor().getMessageTypes().get(3);
internal_static_debugpb_RaftLogResponse_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_debugpb_RaftLogResponse_descriptor,
new java.lang.String[] { "Entry", });
internal_static_debugpb_RegionInfoRequest_descriptor =
getDescriptor().getMessageTypes().get(4);
internal_static_debugpb_RegionInfoRequest_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_debugpb_RegionInfoRequest_descriptor,
new java.lang.String[] { "RegionId", });
internal_static_debugpb_RegionInfoResponse_descriptor =
getDescriptor().getMessageTypes().get(5);
internal_static_debugpb_RegionInfoResponse_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_debugpb_RegionInfoResponse_descriptor,
new java.lang.String[] { "RaftLocalState", "RaftApplyState", "RegionLocalState", });
internal_static_debugpb_RegionSizeRequest_descriptor =
getDescriptor().getMessageTypes().get(6);
internal_static_debugpb_RegionSizeRequest_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_debugpb_RegionSizeRequest_descriptor,
new java.lang.String[] { "RegionId", "Cfs", });
internal_static_debugpb_RegionSizeResponse_descriptor =
getDescriptor().getMessageTypes().get(7);
internal_static_debugpb_RegionSizeResponse_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_debugpb_RegionSizeResponse_descriptor,
new java.lang.String[] { "Entries", });
internal_static_debugpb_RegionSizeResponse_Entry_descriptor =
internal_static_debugpb_RegionSizeResponse_descriptor.getNestedTypes().get(0);
internal_static_debugpb_RegionSizeResponse_Entry_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_debugpb_RegionSizeResponse_Entry_descriptor,
new java.lang.String[] { "Cf", "Size", });
internal_static_debugpb_ScanMvccRequest_descriptor =
getDescriptor().getMessageTypes().get(8);
internal_static_debugpb_ScanMvccRequest_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_debugpb_ScanMvccRequest_descriptor,
new java.lang.String[] { "FromKey", "ToKey", "Limit", });
internal_static_debugpb_ScanMvccResponse_descriptor =
getDescriptor().getMessageTypes().get(9);
internal_static_debugpb_ScanMvccResponse_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_debugpb_ScanMvccResponse_descriptor,
new java.lang.String[] { "Key", "Info", });
internal_static_debugpb_CompactRequest_descriptor =
getDescriptor().getMessageTypes().get(10);
internal_static_debugpb_CompactRequest_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_debugpb_CompactRequest_descriptor,
new java.lang.String[] { "Db", "Cf", "FromKey", "ToKey", "Threads", "BottommostLevelCompaction", });
internal_static_debugpb_CompactResponse_descriptor =
getDescriptor().getMessageTypes().get(11);
internal_static_debugpb_CompactResponse_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_debugpb_CompactResponse_descriptor,
new java.lang.String[] { });
internal_static_debugpb_InjectFailPointRequest_descriptor =
getDescriptor().getMessageTypes().get(12);
internal_static_debugpb_InjectFailPointRequest_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_debugpb_InjectFailPointRequest_descriptor,
new java.lang.String[] { "Name", "Actions", });
internal_static_debugpb_InjectFailPointResponse_descriptor =
getDescriptor().getMessageTypes().get(13);
internal_static_debugpb_InjectFailPointResponse_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_debugpb_InjectFailPointResponse_descriptor,
new java.lang.String[] { });
internal_static_debugpb_RecoverFailPointRequest_descriptor =
getDescriptor().getMessageTypes().get(14);
internal_static_debugpb_RecoverFailPointRequest_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_debugpb_RecoverFailPointRequest_descriptor,
new java.lang.String[] { "Name", });
internal_static_debugpb_RecoverFailPointResponse_descriptor =
getDescriptor().getMessageTypes().get(15);
internal_static_debugpb_RecoverFailPointResponse_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_debugpb_RecoverFailPointResponse_descriptor,
new java.lang.String[] { });
internal_static_debugpb_ListFailPointsRequest_descriptor =
getDescriptor().getMessageTypes().get(16);
internal_static_debugpb_ListFailPointsRequest_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_debugpb_ListFailPointsRequest_descriptor,
new java.lang.String[] { });
internal_static_debugpb_ListFailPointsResponse_descriptor =
getDescriptor().getMessageTypes().get(17);
internal_static_debugpb_ListFailPointsResponse_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_debugpb_ListFailPointsResponse_descriptor,
new java.lang.String[] { "Entries", });
internal_static_debugpb_ListFailPointsResponse_Entry_descriptor =
internal_static_debugpb_ListFailPointsResponse_descriptor.getNestedTypes().get(0);
internal_static_debugpb_ListFailPointsResponse_Entry_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_debugpb_ListFailPointsResponse_Entry_descriptor,
new java.lang.String[] { "Name", "Actions", });
internal_static_debugpb_GetMetricsRequest_descriptor =
getDescriptor().getMessageTypes().get(18);
internal_static_debugpb_GetMetricsRequest_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_debugpb_GetMetricsRequest_descriptor,
new java.lang.String[] { "All", });
internal_static_debugpb_GetMetricsResponse_descriptor =
getDescriptor().getMessageTypes().get(19);
internal_static_debugpb_GetMetricsResponse_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_debugpb_GetMetricsResponse_descriptor,
new java.lang.String[] { "Prometheus", "RocksdbKv", "RocksdbRaft", "Jemalloc", "StoreId", });
internal_static_debugpb_RegionConsistencyCheckRequest_descriptor =
getDescriptor().getMessageTypes().get(20);
internal_static_debugpb_RegionConsistencyCheckRequest_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_debugpb_RegionConsistencyCheckRequest_descriptor,
new java.lang.String[] { "RegionId", });
internal_static_debugpb_RegionConsistencyCheckResponse_descriptor =
getDescriptor().getMessageTypes().get(21);
internal_static_debugpb_RegionConsistencyCheckResponse_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_debugpb_RegionConsistencyCheckResponse_descriptor,
new java.lang.String[] { });
internal_static_debugpb_ModifyTikvConfigRequest_descriptor =
getDescriptor().getMessageTypes().get(22);
internal_static_debugpb_ModifyTikvConfigRequest_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_debugpb_ModifyTikvConfigRequest_descriptor,
new java.lang.String[] { "Module", "ConfigName", "ConfigValue", });
internal_static_debugpb_ModifyTikvConfigResponse_descriptor =
getDescriptor().getMessageTypes().get(23);
internal_static_debugpb_ModifyTikvConfigResponse_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_debugpb_ModifyTikvConfigResponse_descriptor,
new java.lang.String[] { });
internal_static_debugpb_Property_descriptor =
getDescriptor().getMessageTypes().get(24);
internal_static_debugpb_Property_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_debugpb_Property_descriptor,
new java.lang.String[] { "Name", "Value", });
internal_static_debugpb_GetRegionPropertiesRequest_descriptor =
getDescriptor().getMessageTypes().get(25);
internal_static_debugpb_GetRegionPropertiesRequest_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_debugpb_GetRegionPropertiesRequest_descriptor,
new java.lang.String[] { "RegionId", });
internal_static_debugpb_GetRegionPropertiesResponse_descriptor =
getDescriptor().getMessageTypes().get(26);
internal_static_debugpb_GetRegionPropertiesResponse_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_debugpb_GetRegionPropertiesResponse_descriptor,
new java.lang.String[] { "Props", });
internal_static_debugpb_GetStoreInfoRequest_descriptor =
getDescriptor().getMessageTypes().get(27);
internal_static_debugpb_GetStoreInfoRequest_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_debugpb_GetStoreInfoRequest_descriptor,
new java.lang.String[] { });
internal_static_debugpb_GetStoreInfoResponse_descriptor =
getDescriptor().getMessageTypes().get(28);
internal_static_debugpb_GetStoreInfoResponse_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_debugpb_GetStoreInfoResponse_descriptor,
new java.lang.String[] { "StoreId", });
internal_static_debugpb_GetClusterInfoRequest_descriptor =
getDescriptor().getMessageTypes().get(29);
internal_static_debugpb_GetClusterInfoRequest_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_debugpb_GetClusterInfoRequest_descriptor,
new java.lang.String[] { });
internal_static_debugpb_GetClusterInfoResponse_descriptor =
getDescriptor().getMessageTypes().get(30);
internal_static_debugpb_GetClusterInfoResponse_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_debugpb_GetClusterInfoResponse_descriptor,
new java.lang.String[] { "ClusterId", });
com.google.protobuf.ExtensionRegistry registry =
com.google.protobuf.ExtensionRegistry.newInstance();
registry.add(com.google.protobuf.GoGoProtos.marshalerAll);
registry.add(com.google.protobuf.GoGoProtos.sizerAll);
registry.add(com.google.protobuf.GoGoProtos.unmarshalerAll);
registry.add(rustproto.Rustproto.liteRuntimeAll);
com.google.protobuf.Descriptors.FileDescriptor
.internalUpdateFileDescriptor(descriptor, registry);
eraftpb.Eraftpb.getDescriptor();
org.tikv.kvproto.Kvrpcpb.getDescriptor();
org.tikv.kvproto.RaftServerpb.getDescriptor();
com.google.protobuf.GoGoProtos.getDescriptor();
rustproto.Rustproto.getDescriptor();
}
// @@protoc_insertion_point(outer_class_scope)
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy