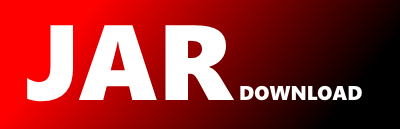
org.tikv.kvproto.Encryptionpb Maven / Gradle / Ivy
// Generated by the protocol buffer compiler. DO NOT EDIT!
// source: encryptionpb.proto
package org.tikv.kvproto;
public final class Encryptionpb {
private Encryptionpb() {}
public static void registerAllExtensions(
com.google.protobuf.ExtensionRegistryLite registry) {
}
public static void registerAllExtensions(
com.google.protobuf.ExtensionRegistry registry) {
registerAllExtensions(
(com.google.protobuf.ExtensionRegistryLite) registry);
}
/**
* Protobuf enum {@code encryptionpb.EncryptionMethod}
*/
public enum EncryptionMethod
implements com.google.protobuf.ProtocolMessageEnum {
/**
* UNKNOWN = 0;
*/
UNKNOWN(0),
/**
* PLAINTEXT = 1;
*/
PLAINTEXT(1),
/**
* AES128_CTR = 2;
*/
AES128_CTR(2),
/**
* AES192_CTR = 3;
*/
AES192_CTR(3),
/**
* AES256_CTR = 4;
*/
AES256_CTR(4),
UNRECOGNIZED(-1),
;
/**
* UNKNOWN = 0;
*/
public static final int UNKNOWN_VALUE = 0;
/**
* PLAINTEXT = 1;
*/
public static final int PLAINTEXT_VALUE = 1;
/**
* AES128_CTR = 2;
*/
public static final int AES128_CTR_VALUE = 2;
/**
* AES192_CTR = 3;
*/
public static final int AES192_CTR_VALUE = 3;
/**
* AES256_CTR = 4;
*/
public static final int AES256_CTR_VALUE = 4;
public final int getNumber() {
if (this == UNRECOGNIZED) {
throw new java.lang.IllegalArgumentException(
"Can't get the number of an unknown enum value.");
}
return value;
}
/**
* @deprecated Use {@link #forNumber(int)} instead.
*/
@java.lang.Deprecated
public static EncryptionMethod valueOf(int value) {
return forNumber(value);
}
public static EncryptionMethod forNumber(int value) {
switch (value) {
case 0: return UNKNOWN;
case 1: return PLAINTEXT;
case 2: return AES128_CTR;
case 3: return AES192_CTR;
case 4: return AES256_CTR;
default: return null;
}
}
public static com.google.protobuf.Internal.EnumLiteMap
internalGetValueMap() {
return internalValueMap;
}
private static final com.google.protobuf.Internal.EnumLiteMap<
EncryptionMethod> internalValueMap =
new com.google.protobuf.Internal.EnumLiteMap() {
public EncryptionMethod findValueByNumber(int number) {
return EncryptionMethod.forNumber(number);
}
};
public final com.google.protobuf.Descriptors.EnumValueDescriptor
getValueDescriptor() {
return getDescriptor().getValues().get(ordinal());
}
public final com.google.protobuf.Descriptors.EnumDescriptor
getDescriptorForType() {
return getDescriptor();
}
public static final com.google.protobuf.Descriptors.EnumDescriptor
getDescriptor() {
return org.tikv.kvproto.Encryptionpb.getDescriptor().getEnumTypes().get(0);
}
private static final EncryptionMethod[] VALUES = values();
public static EncryptionMethod valueOf(
com.google.protobuf.Descriptors.EnumValueDescriptor desc) {
if (desc.getType() != getDescriptor()) {
throw new java.lang.IllegalArgumentException(
"EnumValueDescriptor is not for this type.");
}
if (desc.getIndex() == -1) {
return UNRECOGNIZED;
}
return VALUES[desc.getIndex()];
}
private final int value;
private EncryptionMethod(int value) {
this.value = value;
}
// @@protoc_insertion_point(enum_scope:encryptionpb.EncryptionMethod)
}
public interface EncryptionMetaOrBuilder extends
// @@protoc_insertion_point(interface_extends:encryptionpb.EncryptionMeta)
com.google.protobuf.MessageOrBuilder {
/**
*
* ID of the key used to encrypt the data.
*
*
* uint64 key_id = 1;
*/
long getKeyId();
/**
*
* Initialization vector (IV) of the data.
*
*
* bytes iv = 2;
*/
com.google.protobuf.ByteString getIv();
}
/**
*
* General encryption metadata for any data type.
*
*
* Protobuf type {@code encryptionpb.EncryptionMeta}
*/
public static final class EncryptionMeta extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:encryptionpb.EncryptionMeta)
EncryptionMetaOrBuilder {
private static final long serialVersionUID = 0L;
// Use EncryptionMeta.newBuilder() to construct.
private EncryptionMeta(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private EncryptionMeta() {
keyId_ = 0L;
iv_ = com.google.protobuf.ByteString.EMPTY;
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private EncryptionMeta(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
default: {
if (!parseUnknownFieldProto3(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
case 8: {
keyId_ = input.readUInt64();
break;
}
case 18: {
iv_ = input.readBytes();
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.tikv.kvproto.Encryptionpb.internal_static_encryptionpb_EncryptionMeta_descriptor;
}
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.tikv.kvproto.Encryptionpb.internal_static_encryptionpb_EncryptionMeta_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.tikv.kvproto.Encryptionpb.EncryptionMeta.class, org.tikv.kvproto.Encryptionpb.EncryptionMeta.Builder.class);
}
public static final int KEY_ID_FIELD_NUMBER = 1;
private long keyId_;
/**
*
* ID of the key used to encrypt the data.
*
*
* uint64 key_id = 1;
*/
public long getKeyId() {
return keyId_;
}
public static final int IV_FIELD_NUMBER = 2;
private com.google.protobuf.ByteString iv_;
/**
*
* Initialization vector (IV) of the data.
*
*
* bytes iv = 2;
*/
public com.google.protobuf.ByteString getIv() {
return iv_;
}
private byte memoizedIsInitialized = -1;
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (keyId_ != 0L) {
output.writeUInt64(1, keyId_);
}
if (!iv_.isEmpty()) {
output.writeBytes(2, iv_);
}
unknownFields.writeTo(output);
}
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (keyId_ != 0L) {
size += com.google.protobuf.CodedOutputStream
.computeUInt64Size(1, keyId_);
}
if (!iv_.isEmpty()) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(2, iv_);
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof org.tikv.kvproto.Encryptionpb.EncryptionMeta)) {
return super.equals(obj);
}
org.tikv.kvproto.Encryptionpb.EncryptionMeta other = (org.tikv.kvproto.Encryptionpb.EncryptionMeta) obj;
boolean result = true;
result = result && (getKeyId()
== other.getKeyId());
result = result && getIv()
.equals(other.getIv());
result = result && unknownFields.equals(other.unknownFields);
return result;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
hash = (37 * hash) + KEY_ID_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
getKeyId());
hash = (37 * hash) + IV_FIELD_NUMBER;
hash = (53 * hash) + getIv().hashCode();
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static org.tikv.kvproto.Encryptionpb.EncryptionMeta parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.tikv.kvproto.Encryptionpb.EncryptionMeta parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.tikv.kvproto.Encryptionpb.EncryptionMeta parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.tikv.kvproto.Encryptionpb.EncryptionMeta parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.tikv.kvproto.Encryptionpb.EncryptionMeta parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.tikv.kvproto.Encryptionpb.EncryptionMeta parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.tikv.kvproto.Encryptionpb.EncryptionMeta parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static org.tikv.kvproto.Encryptionpb.EncryptionMeta parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static org.tikv.kvproto.Encryptionpb.EncryptionMeta parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static org.tikv.kvproto.Encryptionpb.EncryptionMeta parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static org.tikv.kvproto.Encryptionpb.EncryptionMeta parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static org.tikv.kvproto.Encryptionpb.EncryptionMeta parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(org.tikv.kvproto.Encryptionpb.EncryptionMeta prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
* General encryption metadata for any data type.
*
*
* Protobuf type {@code encryptionpb.EncryptionMeta}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:encryptionpb.EncryptionMeta)
org.tikv.kvproto.Encryptionpb.EncryptionMetaOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.tikv.kvproto.Encryptionpb.internal_static_encryptionpb_EncryptionMeta_descriptor;
}
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.tikv.kvproto.Encryptionpb.internal_static_encryptionpb_EncryptionMeta_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.tikv.kvproto.Encryptionpb.EncryptionMeta.class, org.tikv.kvproto.Encryptionpb.EncryptionMeta.Builder.class);
}
// Construct using org.tikv.kvproto.Encryptionpb.EncryptionMeta.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
public Builder clear() {
super.clear();
keyId_ = 0L;
iv_ = com.google.protobuf.ByteString.EMPTY;
return this;
}
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return org.tikv.kvproto.Encryptionpb.internal_static_encryptionpb_EncryptionMeta_descriptor;
}
public org.tikv.kvproto.Encryptionpb.EncryptionMeta getDefaultInstanceForType() {
return org.tikv.kvproto.Encryptionpb.EncryptionMeta.getDefaultInstance();
}
public org.tikv.kvproto.Encryptionpb.EncryptionMeta build() {
org.tikv.kvproto.Encryptionpb.EncryptionMeta result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
public org.tikv.kvproto.Encryptionpb.EncryptionMeta buildPartial() {
org.tikv.kvproto.Encryptionpb.EncryptionMeta result = new org.tikv.kvproto.Encryptionpb.EncryptionMeta(this);
result.keyId_ = keyId_;
result.iv_ = iv_;
onBuilt();
return result;
}
public Builder clone() {
return (Builder) super.clone();
}
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.setField(field, value);
}
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return (Builder) super.clearField(field);
}
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return (Builder) super.clearOneof(oneof);
}
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return (Builder) super.setRepeatedField(field, index, value);
}
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.addRepeatedField(field, value);
}
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof org.tikv.kvproto.Encryptionpb.EncryptionMeta) {
return mergeFrom((org.tikv.kvproto.Encryptionpb.EncryptionMeta)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(org.tikv.kvproto.Encryptionpb.EncryptionMeta other) {
if (other == org.tikv.kvproto.Encryptionpb.EncryptionMeta.getDefaultInstance()) return this;
if (other.getKeyId() != 0L) {
setKeyId(other.getKeyId());
}
if (other.getIv() != com.google.protobuf.ByteString.EMPTY) {
setIv(other.getIv());
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
public final boolean isInitialized() {
return true;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
org.tikv.kvproto.Encryptionpb.EncryptionMeta parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (org.tikv.kvproto.Encryptionpb.EncryptionMeta) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private long keyId_ ;
/**
*
* ID of the key used to encrypt the data.
*
*
* uint64 key_id = 1;
*/
public long getKeyId() {
return keyId_;
}
/**
*
* ID of the key used to encrypt the data.
*
*
* uint64 key_id = 1;
*/
public Builder setKeyId(long value) {
keyId_ = value;
onChanged();
return this;
}
/**
*
* ID of the key used to encrypt the data.
*
*
* uint64 key_id = 1;
*/
public Builder clearKeyId() {
keyId_ = 0L;
onChanged();
return this;
}
private com.google.protobuf.ByteString iv_ = com.google.protobuf.ByteString.EMPTY;
/**
*
* Initialization vector (IV) of the data.
*
*
* bytes iv = 2;
*/
public com.google.protobuf.ByteString getIv() {
return iv_;
}
/**
*
* Initialization vector (IV) of the data.
*
*
* bytes iv = 2;
*/
public Builder setIv(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
iv_ = value;
onChanged();
return this;
}
/**
*
* Initialization vector (IV) of the data.
*
*
* bytes iv = 2;
*/
public Builder clearIv() {
iv_ = getDefaultInstance().getIv();
onChanged();
return this;
}
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFieldsProto3(unknownFields);
}
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:encryptionpb.EncryptionMeta)
}
// @@protoc_insertion_point(class_scope:encryptionpb.EncryptionMeta)
private static final org.tikv.kvproto.Encryptionpb.EncryptionMeta DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new org.tikv.kvproto.Encryptionpb.EncryptionMeta();
}
public static org.tikv.kvproto.Encryptionpb.EncryptionMeta getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
public EncryptionMeta parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new EncryptionMeta(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
public org.tikv.kvproto.Encryptionpb.EncryptionMeta getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface FileInfoOrBuilder extends
// @@protoc_insertion_point(interface_extends:encryptionpb.FileInfo)
com.google.protobuf.MessageOrBuilder {
/**
*
* ID of the key used to encrypt the file.
*
*
* uint64 key_id = 1;
*/
long getKeyId();
/**
*
* Initialization vector (IV) of the file.
*
*
* bytes iv = 2;
*/
com.google.protobuf.ByteString getIv();
/**
*
* Method of encryption algorithm used to encrypted the file.
*
*
* .encryptionpb.EncryptionMethod method = 3;
*/
int getMethodValue();
/**
*
* Method of encryption algorithm used to encrypted the file.
*
*
* .encryptionpb.EncryptionMethod method = 3;
*/
org.tikv.kvproto.Encryptionpb.EncryptionMethod getMethod();
}
/**
*
* Information about an encrypted file.
*
*
* Protobuf type {@code encryptionpb.FileInfo}
*/
public static final class FileInfo extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:encryptionpb.FileInfo)
FileInfoOrBuilder {
private static final long serialVersionUID = 0L;
// Use FileInfo.newBuilder() to construct.
private FileInfo(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private FileInfo() {
keyId_ = 0L;
iv_ = com.google.protobuf.ByteString.EMPTY;
method_ = 0;
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private FileInfo(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
default: {
if (!parseUnknownFieldProto3(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
case 8: {
keyId_ = input.readUInt64();
break;
}
case 18: {
iv_ = input.readBytes();
break;
}
case 24: {
int rawValue = input.readEnum();
method_ = rawValue;
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.tikv.kvproto.Encryptionpb.internal_static_encryptionpb_FileInfo_descriptor;
}
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.tikv.kvproto.Encryptionpb.internal_static_encryptionpb_FileInfo_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.tikv.kvproto.Encryptionpb.FileInfo.class, org.tikv.kvproto.Encryptionpb.FileInfo.Builder.class);
}
public static final int KEY_ID_FIELD_NUMBER = 1;
private long keyId_;
/**
*
* ID of the key used to encrypt the file.
*
*
* uint64 key_id = 1;
*/
public long getKeyId() {
return keyId_;
}
public static final int IV_FIELD_NUMBER = 2;
private com.google.protobuf.ByteString iv_;
/**
*
* Initialization vector (IV) of the file.
*
*
* bytes iv = 2;
*/
public com.google.protobuf.ByteString getIv() {
return iv_;
}
public static final int METHOD_FIELD_NUMBER = 3;
private int method_;
/**
*
* Method of encryption algorithm used to encrypted the file.
*
*
* .encryptionpb.EncryptionMethod method = 3;
*/
public int getMethodValue() {
return method_;
}
/**
*
* Method of encryption algorithm used to encrypted the file.
*
*
* .encryptionpb.EncryptionMethod method = 3;
*/
public org.tikv.kvproto.Encryptionpb.EncryptionMethod getMethod() {
org.tikv.kvproto.Encryptionpb.EncryptionMethod result = org.tikv.kvproto.Encryptionpb.EncryptionMethod.valueOf(method_);
return result == null ? org.tikv.kvproto.Encryptionpb.EncryptionMethod.UNRECOGNIZED : result;
}
private byte memoizedIsInitialized = -1;
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (keyId_ != 0L) {
output.writeUInt64(1, keyId_);
}
if (!iv_.isEmpty()) {
output.writeBytes(2, iv_);
}
if (method_ != org.tikv.kvproto.Encryptionpb.EncryptionMethod.UNKNOWN.getNumber()) {
output.writeEnum(3, method_);
}
unknownFields.writeTo(output);
}
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (keyId_ != 0L) {
size += com.google.protobuf.CodedOutputStream
.computeUInt64Size(1, keyId_);
}
if (!iv_.isEmpty()) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(2, iv_);
}
if (method_ != org.tikv.kvproto.Encryptionpb.EncryptionMethod.UNKNOWN.getNumber()) {
size += com.google.protobuf.CodedOutputStream
.computeEnumSize(3, method_);
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof org.tikv.kvproto.Encryptionpb.FileInfo)) {
return super.equals(obj);
}
org.tikv.kvproto.Encryptionpb.FileInfo other = (org.tikv.kvproto.Encryptionpb.FileInfo) obj;
boolean result = true;
result = result && (getKeyId()
== other.getKeyId());
result = result && getIv()
.equals(other.getIv());
result = result && method_ == other.method_;
result = result && unknownFields.equals(other.unknownFields);
return result;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
hash = (37 * hash) + KEY_ID_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
getKeyId());
hash = (37 * hash) + IV_FIELD_NUMBER;
hash = (53 * hash) + getIv().hashCode();
hash = (37 * hash) + METHOD_FIELD_NUMBER;
hash = (53 * hash) + method_;
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static org.tikv.kvproto.Encryptionpb.FileInfo parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.tikv.kvproto.Encryptionpb.FileInfo parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.tikv.kvproto.Encryptionpb.FileInfo parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.tikv.kvproto.Encryptionpb.FileInfo parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.tikv.kvproto.Encryptionpb.FileInfo parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.tikv.kvproto.Encryptionpb.FileInfo parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.tikv.kvproto.Encryptionpb.FileInfo parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static org.tikv.kvproto.Encryptionpb.FileInfo parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static org.tikv.kvproto.Encryptionpb.FileInfo parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static org.tikv.kvproto.Encryptionpb.FileInfo parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static org.tikv.kvproto.Encryptionpb.FileInfo parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static org.tikv.kvproto.Encryptionpb.FileInfo parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(org.tikv.kvproto.Encryptionpb.FileInfo prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
* Information about an encrypted file.
*
*
* Protobuf type {@code encryptionpb.FileInfo}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:encryptionpb.FileInfo)
org.tikv.kvproto.Encryptionpb.FileInfoOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.tikv.kvproto.Encryptionpb.internal_static_encryptionpb_FileInfo_descriptor;
}
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.tikv.kvproto.Encryptionpb.internal_static_encryptionpb_FileInfo_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.tikv.kvproto.Encryptionpb.FileInfo.class, org.tikv.kvproto.Encryptionpb.FileInfo.Builder.class);
}
// Construct using org.tikv.kvproto.Encryptionpb.FileInfo.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
public Builder clear() {
super.clear();
keyId_ = 0L;
iv_ = com.google.protobuf.ByteString.EMPTY;
method_ = 0;
return this;
}
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return org.tikv.kvproto.Encryptionpb.internal_static_encryptionpb_FileInfo_descriptor;
}
public org.tikv.kvproto.Encryptionpb.FileInfo getDefaultInstanceForType() {
return org.tikv.kvproto.Encryptionpb.FileInfo.getDefaultInstance();
}
public org.tikv.kvproto.Encryptionpb.FileInfo build() {
org.tikv.kvproto.Encryptionpb.FileInfo result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
public org.tikv.kvproto.Encryptionpb.FileInfo buildPartial() {
org.tikv.kvproto.Encryptionpb.FileInfo result = new org.tikv.kvproto.Encryptionpb.FileInfo(this);
result.keyId_ = keyId_;
result.iv_ = iv_;
result.method_ = method_;
onBuilt();
return result;
}
public Builder clone() {
return (Builder) super.clone();
}
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.setField(field, value);
}
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return (Builder) super.clearField(field);
}
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return (Builder) super.clearOneof(oneof);
}
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return (Builder) super.setRepeatedField(field, index, value);
}
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.addRepeatedField(field, value);
}
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof org.tikv.kvproto.Encryptionpb.FileInfo) {
return mergeFrom((org.tikv.kvproto.Encryptionpb.FileInfo)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(org.tikv.kvproto.Encryptionpb.FileInfo other) {
if (other == org.tikv.kvproto.Encryptionpb.FileInfo.getDefaultInstance()) return this;
if (other.getKeyId() != 0L) {
setKeyId(other.getKeyId());
}
if (other.getIv() != com.google.protobuf.ByteString.EMPTY) {
setIv(other.getIv());
}
if (other.method_ != 0) {
setMethodValue(other.getMethodValue());
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
public final boolean isInitialized() {
return true;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
org.tikv.kvproto.Encryptionpb.FileInfo parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (org.tikv.kvproto.Encryptionpb.FileInfo) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private long keyId_ ;
/**
*
* ID of the key used to encrypt the file.
*
*
* uint64 key_id = 1;
*/
public long getKeyId() {
return keyId_;
}
/**
*
* ID of the key used to encrypt the file.
*
*
* uint64 key_id = 1;
*/
public Builder setKeyId(long value) {
keyId_ = value;
onChanged();
return this;
}
/**
*
* ID of the key used to encrypt the file.
*
*
* uint64 key_id = 1;
*/
public Builder clearKeyId() {
keyId_ = 0L;
onChanged();
return this;
}
private com.google.protobuf.ByteString iv_ = com.google.protobuf.ByteString.EMPTY;
/**
*
* Initialization vector (IV) of the file.
*
*
* bytes iv = 2;
*/
public com.google.protobuf.ByteString getIv() {
return iv_;
}
/**
*
* Initialization vector (IV) of the file.
*
*
* bytes iv = 2;
*/
public Builder setIv(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
iv_ = value;
onChanged();
return this;
}
/**
*
* Initialization vector (IV) of the file.
*
*
* bytes iv = 2;
*/
public Builder clearIv() {
iv_ = getDefaultInstance().getIv();
onChanged();
return this;
}
private int method_ = 0;
/**
*
* Method of encryption algorithm used to encrypted the file.
*
*
* .encryptionpb.EncryptionMethod method = 3;
*/
public int getMethodValue() {
return method_;
}
/**
*
* Method of encryption algorithm used to encrypted the file.
*
*
* .encryptionpb.EncryptionMethod method = 3;
*/
public Builder setMethodValue(int value) {
method_ = value;
onChanged();
return this;
}
/**
*
* Method of encryption algorithm used to encrypted the file.
*
*
* .encryptionpb.EncryptionMethod method = 3;
*/
public org.tikv.kvproto.Encryptionpb.EncryptionMethod getMethod() {
org.tikv.kvproto.Encryptionpb.EncryptionMethod result = org.tikv.kvproto.Encryptionpb.EncryptionMethod.valueOf(method_);
return result == null ? org.tikv.kvproto.Encryptionpb.EncryptionMethod.UNRECOGNIZED : result;
}
/**
*
* Method of encryption algorithm used to encrypted the file.
*
*
* .encryptionpb.EncryptionMethod method = 3;
*/
public Builder setMethod(org.tikv.kvproto.Encryptionpb.EncryptionMethod value) {
if (value == null) {
throw new NullPointerException();
}
method_ = value.getNumber();
onChanged();
return this;
}
/**
*
* Method of encryption algorithm used to encrypted the file.
*
*
* .encryptionpb.EncryptionMethod method = 3;
*/
public Builder clearMethod() {
method_ = 0;
onChanged();
return this;
}
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFieldsProto3(unknownFields);
}
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:encryptionpb.FileInfo)
}
// @@protoc_insertion_point(class_scope:encryptionpb.FileInfo)
private static final org.tikv.kvproto.Encryptionpb.FileInfo DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new org.tikv.kvproto.Encryptionpb.FileInfo();
}
public static org.tikv.kvproto.Encryptionpb.FileInfo getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
public FileInfo parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new FileInfo(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
public org.tikv.kvproto.Encryptionpb.FileInfo getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface FileDictionaryOrBuilder extends
// @@protoc_insertion_point(interface_extends:encryptionpb.FileDictionary)
com.google.protobuf.MessageOrBuilder {
/**
*
* A map of file name to file info.
*
*
* map<string, .encryptionpb.FileInfo> files = 1;
*/
int getFilesCount();
/**
*
* A map of file name to file info.
*
*
* map<string, .encryptionpb.FileInfo> files = 1;
*/
boolean containsFiles(
java.lang.String key);
/**
* Use {@link #getFilesMap()} instead.
*/
@java.lang.Deprecated
java.util.Map
getFiles();
/**
*
* A map of file name to file info.
*
*
* map<string, .encryptionpb.FileInfo> files = 1;
*/
java.util.Map
getFilesMap();
/**
*
* A map of file name to file info.
*
*
* map<string, .encryptionpb.FileInfo> files = 1;
*/
org.tikv.kvproto.Encryptionpb.FileInfo getFilesOrDefault(
java.lang.String key,
org.tikv.kvproto.Encryptionpb.FileInfo defaultValue);
/**
*
* A map of file name to file info.
*
*
* map<string, .encryptionpb.FileInfo> files = 1;
*/
org.tikv.kvproto.Encryptionpb.FileInfo getFilesOrThrow(
java.lang.String key);
}
/**
* Protobuf type {@code encryptionpb.FileDictionary}
*/
public static final class FileDictionary extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:encryptionpb.FileDictionary)
FileDictionaryOrBuilder {
private static final long serialVersionUID = 0L;
// Use FileDictionary.newBuilder() to construct.
private FileDictionary(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private FileDictionary() {
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private FileDictionary(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
default: {
if (!parseUnknownFieldProto3(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
case 10: {
if (!((mutable_bitField0_ & 0x00000001) == 0x00000001)) {
files_ = com.google.protobuf.MapField.newMapField(
FilesDefaultEntryHolder.defaultEntry);
mutable_bitField0_ |= 0x00000001;
}
com.google.protobuf.MapEntry
files__ = input.readMessage(
FilesDefaultEntryHolder.defaultEntry.getParserForType(), extensionRegistry);
files_.getMutableMap().put(
files__.getKey(), files__.getValue());
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.tikv.kvproto.Encryptionpb.internal_static_encryptionpb_FileDictionary_descriptor;
}
@SuppressWarnings({"rawtypes"})
protected com.google.protobuf.MapField internalGetMapField(
int number) {
switch (number) {
case 1:
return internalGetFiles();
default:
throw new RuntimeException(
"Invalid map field number: " + number);
}
}
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.tikv.kvproto.Encryptionpb.internal_static_encryptionpb_FileDictionary_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.tikv.kvproto.Encryptionpb.FileDictionary.class, org.tikv.kvproto.Encryptionpb.FileDictionary.Builder.class);
}
public static final int FILES_FIELD_NUMBER = 1;
private static final class FilesDefaultEntryHolder {
static final com.google.protobuf.MapEntry<
java.lang.String, org.tikv.kvproto.Encryptionpb.FileInfo> defaultEntry =
com.google.protobuf.MapEntry
.newDefaultInstance(
org.tikv.kvproto.Encryptionpb.internal_static_encryptionpb_FileDictionary_FilesEntry_descriptor,
com.google.protobuf.WireFormat.FieldType.STRING,
"",
com.google.protobuf.WireFormat.FieldType.MESSAGE,
org.tikv.kvproto.Encryptionpb.FileInfo.getDefaultInstance());
}
private com.google.protobuf.MapField<
java.lang.String, org.tikv.kvproto.Encryptionpb.FileInfo> files_;
private com.google.protobuf.MapField
internalGetFiles() {
if (files_ == null) {
return com.google.protobuf.MapField.emptyMapField(
FilesDefaultEntryHolder.defaultEntry);
}
return files_;
}
public int getFilesCount() {
return internalGetFiles().getMap().size();
}
/**
*
* A map of file name to file info.
*
*
* map<string, .encryptionpb.FileInfo> files = 1;
*/
public boolean containsFiles(
java.lang.String key) {
if (key == null) { throw new java.lang.NullPointerException(); }
return internalGetFiles().getMap().containsKey(key);
}
/**
* Use {@link #getFilesMap()} instead.
*/
@java.lang.Deprecated
public java.util.Map getFiles() {
return getFilesMap();
}
/**
*
* A map of file name to file info.
*
*
* map<string, .encryptionpb.FileInfo> files = 1;
*/
public java.util.Map getFilesMap() {
return internalGetFiles().getMap();
}
/**
*
* A map of file name to file info.
*
*
* map<string, .encryptionpb.FileInfo> files = 1;
*/
public org.tikv.kvproto.Encryptionpb.FileInfo getFilesOrDefault(
java.lang.String key,
org.tikv.kvproto.Encryptionpb.FileInfo defaultValue) {
if (key == null) { throw new java.lang.NullPointerException(); }
java.util.Map map =
internalGetFiles().getMap();
return map.containsKey(key) ? map.get(key) : defaultValue;
}
/**
*
* A map of file name to file info.
*
*
* map<string, .encryptionpb.FileInfo> files = 1;
*/
public org.tikv.kvproto.Encryptionpb.FileInfo getFilesOrThrow(
java.lang.String key) {
if (key == null) { throw new java.lang.NullPointerException(); }
java.util.Map map =
internalGetFiles().getMap();
if (!map.containsKey(key)) {
throw new java.lang.IllegalArgumentException();
}
return map.get(key);
}
private byte memoizedIsInitialized = -1;
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
com.google.protobuf.GeneratedMessageV3
.serializeStringMapTo(
output,
internalGetFiles(),
FilesDefaultEntryHolder.defaultEntry,
1);
unknownFields.writeTo(output);
}
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
for (java.util.Map.Entry entry
: internalGetFiles().getMap().entrySet()) {
com.google.protobuf.MapEntry
files__ = FilesDefaultEntryHolder.defaultEntry.newBuilderForType()
.setKey(entry.getKey())
.setValue(entry.getValue())
.build();
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(1, files__);
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof org.tikv.kvproto.Encryptionpb.FileDictionary)) {
return super.equals(obj);
}
org.tikv.kvproto.Encryptionpb.FileDictionary other = (org.tikv.kvproto.Encryptionpb.FileDictionary) obj;
boolean result = true;
result = result && internalGetFiles().equals(
other.internalGetFiles());
result = result && unknownFields.equals(other.unknownFields);
return result;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (!internalGetFiles().getMap().isEmpty()) {
hash = (37 * hash) + FILES_FIELD_NUMBER;
hash = (53 * hash) + internalGetFiles().hashCode();
}
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static org.tikv.kvproto.Encryptionpb.FileDictionary parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.tikv.kvproto.Encryptionpb.FileDictionary parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.tikv.kvproto.Encryptionpb.FileDictionary parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.tikv.kvproto.Encryptionpb.FileDictionary parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.tikv.kvproto.Encryptionpb.FileDictionary parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.tikv.kvproto.Encryptionpb.FileDictionary parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.tikv.kvproto.Encryptionpb.FileDictionary parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static org.tikv.kvproto.Encryptionpb.FileDictionary parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static org.tikv.kvproto.Encryptionpb.FileDictionary parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static org.tikv.kvproto.Encryptionpb.FileDictionary parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static org.tikv.kvproto.Encryptionpb.FileDictionary parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static org.tikv.kvproto.Encryptionpb.FileDictionary parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(org.tikv.kvproto.Encryptionpb.FileDictionary prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code encryptionpb.FileDictionary}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:encryptionpb.FileDictionary)
org.tikv.kvproto.Encryptionpb.FileDictionaryOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.tikv.kvproto.Encryptionpb.internal_static_encryptionpb_FileDictionary_descriptor;
}
@SuppressWarnings({"rawtypes"})
protected com.google.protobuf.MapField internalGetMapField(
int number) {
switch (number) {
case 1:
return internalGetFiles();
default:
throw new RuntimeException(
"Invalid map field number: " + number);
}
}
@SuppressWarnings({"rawtypes"})
protected com.google.protobuf.MapField internalGetMutableMapField(
int number) {
switch (number) {
case 1:
return internalGetMutableFiles();
default:
throw new RuntimeException(
"Invalid map field number: " + number);
}
}
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.tikv.kvproto.Encryptionpb.internal_static_encryptionpb_FileDictionary_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.tikv.kvproto.Encryptionpb.FileDictionary.class, org.tikv.kvproto.Encryptionpb.FileDictionary.Builder.class);
}
// Construct using org.tikv.kvproto.Encryptionpb.FileDictionary.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
public Builder clear() {
super.clear();
internalGetMutableFiles().clear();
return this;
}
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return org.tikv.kvproto.Encryptionpb.internal_static_encryptionpb_FileDictionary_descriptor;
}
public org.tikv.kvproto.Encryptionpb.FileDictionary getDefaultInstanceForType() {
return org.tikv.kvproto.Encryptionpb.FileDictionary.getDefaultInstance();
}
public org.tikv.kvproto.Encryptionpb.FileDictionary build() {
org.tikv.kvproto.Encryptionpb.FileDictionary result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
public org.tikv.kvproto.Encryptionpb.FileDictionary buildPartial() {
org.tikv.kvproto.Encryptionpb.FileDictionary result = new org.tikv.kvproto.Encryptionpb.FileDictionary(this);
int from_bitField0_ = bitField0_;
result.files_ = internalGetFiles();
result.files_.makeImmutable();
onBuilt();
return result;
}
public Builder clone() {
return (Builder) super.clone();
}
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.setField(field, value);
}
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return (Builder) super.clearField(field);
}
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return (Builder) super.clearOneof(oneof);
}
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return (Builder) super.setRepeatedField(field, index, value);
}
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.addRepeatedField(field, value);
}
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof org.tikv.kvproto.Encryptionpb.FileDictionary) {
return mergeFrom((org.tikv.kvproto.Encryptionpb.FileDictionary)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(org.tikv.kvproto.Encryptionpb.FileDictionary other) {
if (other == org.tikv.kvproto.Encryptionpb.FileDictionary.getDefaultInstance()) return this;
internalGetMutableFiles().mergeFrom(
other.internalGetFiles());
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
public final boolean isInitialized() {
return true;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
org.tikv.kvproto.Encryptionpb.FileDictionary parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (org.tikv.kvproto.Encryptionpb.FileDictionary) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
private com.google.protobuf.MapField<
java.lang.String, org.tikv.kvproto.Encryptionpb.FileInfo> files_;
private com.google.protobuf.MapField
internalGetFiles() {
if (files_ == null) {
return com.google.protobuf.MapField.emptyMapField(
FilesDefaultEntryHolder.defaultEntry);
}
return files_;
}
private com.google.protobuf.MapField
internalGetMutableFiles() {
onChanged();;
if (files_ == null) {
files_ = com.google.protobuf.MapField.newMapField(
FilesDefaultEntryHolder.defaultEntry);
}
if (!files_.isMutable()) {
files_ = files_.copy();
}
return files_;
}
public int getFilesCount() {
return internalGetFiles().getMap().size();
}
/**
*
* A map of file name to file info.
*
*
* map<string, .encryptionpb.FileInfo> files = 1;
*/
public boolean containsFiles(
java.lang.String key) {
if (key == null) { throw new java.lang.NullPointerException(); }
return internalGetFiles().getMap().containsKey(key);
}
/**
* Use {@link #getFilesMap()} instead.
*/
@java.lang.Deprecated
public java.util.Map getFiles() {
return getFilesMap();
}
/**
*
* A map of file name to file info.
*
*
* map<string, .encryptionpb.FileInfo> files = 1;
*/
public java.util.Map getFilesMap() {
return internalGetFiles().getMap();
}
/**
*
* A map of file name to file info.
*
*
* map<string, .encryptionpb.FileInfo> files = 1;
*/
public org.tikv.kvproto.Encryptionpb.FileInfo getFilesOrDefault(
java.lang.String key,
org.tikv.kvproto.Encryptionpb.FileInfo defaultValue) {
if (key == null) { throw new java.lang.NullPointerException(); }
java.util.Map map =
internalGetFiles().getMap();
return map.containsKey(key) ? map.get(key) : defaultValue;
}
/**
*
* A map of file name to file info.
*
*
* map<string, .encryptionpb.FileInfo> files = 1;
*/
public org.tikv.kvproto.Encryptionpb.FileInfo getFilesOrThrow(
java.lang.String key) {
if (key == null) { throw new java.lang.NullPointerException(); }
java.util.Map map =
internalGetFiles().getMap();
if (!map.containsKey(key)) {
throw new java.lang.IllegalArgumentException();
}
return map.get(key);
}
public Builder clearFiles() {
internalGetMutableFiles().getMutableMap()
.clear();
return this;
}
/**
*
* A map of file name to file info.
*
*
* map<string, .encryptionpb.FileInfo> files = 1;
*/
public Builder removeFiles(
java.lang.String key) {
if (key == null) { throw new java.lang.NullPointerException(); }
internalGetMutableFiles().getMutableMap()
.remove(key);
return this;
}
/**
* Use alternate mutation accessors instead.
*/
@java.lang.Deprecated
public java.util.Map
getMutableFiles() {
return internalGetMutableFiles().getMutableMap();
}
/**
*
* A map of file name to file info.
*
*
* map<string, .encryptionpb.FileInfo> files = 1;
*/
public Builder putFiles(
java.lang.String key,
org.tikv.kvproto.Encryptionpb.FileInfo value) {
if (key == null) { throw new java.lang.NullPointerException(); }
if (value == null) { throw new java.lang.NullPointerException(); }
internalGetMutableFiles().getMutableMap()
.put(key, value);
return this;
}
/**
*
* A map of file name to file info.
*
*
* map<string, .encryptionpb.FileInfo> files = 1;
*/
public Builder putAllFiles(
java.util.Map values) {
internalGetMutableFiles().getMutableMap()
.putAll(values);
return this;
}
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFieldsProto3(unknownFields);
}
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:encryptionpb.FileDictionary)
}
// @@protoc_insertion_point(class_scope:encryptionpb.FileDictionary)
private static final org.tikv.kvproto.Encryptionpb.FileDictionary DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new org.tikv.kvproto.Encryptionpb.FileDictionary();
}
public static org.tikv.kvproto.Encryptionpb.FileDictionary getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
public FileDictionary parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new FileDictionary(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
public org.tikv.kvproto.Encryptionpb.FileDictionary getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface DataKeyOrBuilder extends
// @@protoc_insertion_point(interface_extends:encryptionpb.DataKey)
com.google.protobuf.MessageOrBuilder {
/**
*
* A sequence of secret bytes used to encrypt data.
*
*
* bytes key = 1;
*/
com.google.protobuf.ByteString getKey();
/**
*
* Method of encryption algorithm used to encrypted data.
*
*
* .encryptionpb.EncryptionMethod method = 2;
*/
int getMethodValue();
/**
*
* Method of encryption algorithm used to encrypted data.
*
*
* .encryptionpb.EncryptionMethod method = 2;
*/
org.tikv.kvproto.Encryptionpb.EncryptionMethod getMethod();
/**
*
* Creation time of the key.
*
*
* uint64 creation_time = 3;
*/
long getCreationTime();
/**
*
* A flag for the key have ever been exposed.
*
*
* bool was_exposed = 4;
*/
boolean getWasExposed();
}
/**
*
* The key used to encrypt the user data.
*
*
* Protobuf type {@code encryptionpb.DataKey}
*/
public static final class DataKey extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:encryptionpb.DataKey)
DataKeyOrBuilder {
private static final long serialVersionUID = 0L;
// Use DataKey.newBuilder() to construct.
private DataKey(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private DataKey() {
key_ = com.google.protobuf.ByteString.EMPTY;
method_ = 0;
creationTime_ = 0L;
wasExposed_ = false;
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private DataKey(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
default: {
if (!parseUnknownFieldProto3(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
case 10: {
key_ = input.readBytes();
break;
}
case 16: {
int rawValue = input.readEnum();
method_ = rawValue;
break;
}
case 24: {
creationTime_ = input.readUInt64();
break;
}
case 32: {
wasExposed_ = input.readBool();
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.tikv.kvproto.Encryptionpb.internal_static_encryptionpb_DataKey_descriptor;
}
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.tikv.kvproto.Encryptionpb.internal_static_encryptionpb_DataKey_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.tikv.kvproto.Encryptionpb.DataKey.class, org.tikv.kvproto.Encryptionpb.DataKey.Builder.class);
}
public static final int KEY_FIELD_NUMBER = 1;
private com.google.protobuf.ByteString key_;
/**
*
* A sequence of secret bytes used to encrypt data.
*
*
* bytes key = 1;
*/
public com.google.protobuf.ByteString getKey() {
return key_;
}
public static final int METHOD_FIELD_NUMBER = 2;
private int method_;
/**
*
* Method of encryption algorithm used to encrypted data.
*
*
* .encryptionpb.EncryptionMethod method = 2;
*/
public int getMethodValue() {
return method_;
}
/**
*
* Method of encryption algorithm used to encrypted data.
*
*
* .encryptionpb.EncryptionMethod method = 2;
*/
public org.tikv.kvproto.Encryptionpb.EncryptionMethod getMethod() {
org.tikv.kvproto.Encryptionpb.EncryptionMethod result = org.tikv.kvproto.Encryptionpb.EncryptionMethod.valueOf(method_);
return result == null ? org.tikv.kvproto.Encryptionpb.EncryptionMethod.UNRECOGNIZED : result;
}
public static final int CREATION_TIME_FIELD_NUMBER = 3;
private long creationTime_;
/**
*
* Creation time of the key.
*
*
* uint64 creation_time = 3;
*/
public long getCreationTime() {
return creationTime_;
}
public static final int WAS_EXPOSED_FIELD_NUMBER = 4;
private boolean wasExposed_;
/**
*
* A flag for the key have ever been exposed.
*
*
* bool was_exposed = 4;
*/
public boolean getWasExposed() {
return wasExposed_;
}
private byte memoizedIsInitialized = -1;
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (!key_.isEmpty()) {
output.writeBytes(1, key_);
}
if (method_ != org.tikv.kvproto.Encryptionpb.EncryptionMethod.UNKNOWN.getNumber()) {
output.writeEnum(2, method_);
}
if (creationTime_ != 0L) {
output.writeUInt64(3, creationTime_);
}
if (wasExposed_ != false) {
output.writeBool(4, wasExposed_);
}
unknownFields.writeTo(output);
}
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (!key_.isEmpty()) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(1, key_);
}
if (method_ != org.tikv.kvproto.Encryptionpb.EncryptionMethod.UNKNOWN.getNumber()) {
size += com.google.protobuf.CodedOutputStream
.computeEnumSize(2, method_);
}
if (creationTime_ != 0L) {
size += com.google.protobuf.CodedOutputStream
.computeUInt64Size(3, creationTime_);
}
if (wasExposed_ != false) {
size += com.google.protobuf.CodedOutputStream
.computeBoolSize(4, wasExposed_);
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof org.tikv.kvproto.Encryptionpb.DataKey)) {
return super.equals(obj);
}
org.tikv.kvproto.Encryptionpb.DataKey other = (org.tikv.kvproto.Encryptionpb.DataKey) obj;
boolean result = true;
result = result && getKey()
.equals(other.getKey());
result = result && method_ == other.method_;
result = result && (getCreationTime()
== other.getCreationTime());
result = result && (getWasExposed()
== other.getWasExposed());
result = result && unknownFields.equals(other.unknownFields);
return result;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
hash = (37 * hash) + KEY_FIELD_NUMBER;
hash = (53 * hash) + getKey().hashCode();
hash = (37 * hash) + METHOD_FIELD_NUMBER;
hash = (53 * hash) + method_;
hash = (37 * hash) + CREATION_TIME_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
getCreationTime());
hash = (37 * hash) + WAS_EXPOSED_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashBoolean(
getWasExposed());
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static org.tikv.kvproto.Encryptionpb.DataKey parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.tikv.kvproto.Encryptionpb.DataKey parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.tikv.kvproto.Encryptionpb.DataKey parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.tikv.kvproto.Encryptionpb.DataKey parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.tikv.kvproto.Encryptionpb.DataKey parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.tikv.kvproto.Encryptionpb.DataKey parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.tikv.kvproto.Encryptionpb.DataKey parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static org.tikv.kvproto.Encryptionpb.DataKey parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static org.tikv.kvproto.Encryptionpb.DataKey parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static org.tikv.kvproto.Encryptionpb.DataKey parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static org.tikv.kvproto.Encryptionpb.DataKey parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static org.tikv.kvproto.Encryptionpb.DataKey parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(org.tikv.kvproto.Encryptionpb.DataKey prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
* The key used to encrypt the user data.
*
*
* Protobuf type {@code encryptionpb.DataKey}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:encryptionpb.DataKey)
org.tikv.kvproto.Encryptionpb.DataKeyOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.tikv.kvproto.Encryptionpb.internal_static_encryptionpb_DataKey_descriptor;
}
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.tikv.kvproto.Encryptionpb.internal_static_encryptionpb_DataKey_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.tikv.kvproto.Encryptionpb.DataKey.class, org.tikv.kvproto.Encryptionpb.DataKey.Builder.class);
}
// Construct using org.tikv.kvproto.Encryptionpb.DataKey.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
public Builder clear() {
super.clear();
key_ = com.google.protobuf.ByteString.EMPTY;
method_ = 0;
creationTime_ = 0L;
wasExposed_ = false;
return this;
}
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return org.tikv.kvproto.Encryptionpb.internal_static_encryptionpb_DataKey_descriptor;
}
public org.tikv.kvproto.Encryptionpb.DataKey getDefaultInstanceForType() {
return org.tikv.kvproto.Encryptionpb.DataKey.getDefaultInstance();
}
public org.tikv.kvproto.Encryptionpb.DataKey build() {
org.tikv.kvproto.Encryptionpb.DataKey result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
public org.tikv.kvproto.Encryptionpb.DataKey buildPartial() {
org.tikv.kvproto.Encryptionpb.DataKey result = new org.tikv.kvproto.Encryptionpb.DataKey(this);
result.key_ = key_;
result.method_ = method_;
result.creationTime_ = creationTime_;
result.wasExposed_ = wasExposed_;
onBuilt();
return result;
}
public Builder clone() {
return (Builder) super.clone();
}
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.setField(field, value);
}
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return (Builder) super.clearField(field);
}
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return (Builder) super.clearOneof(oneof);
}
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return (Builder) super.setRepeatedField(field, index, value);
}
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.addRepeatedField(field, value);
}
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof org.tikv.kvproto.Encryptionpb.DataKey) {
return mergeFrom((org.tikv.kvproto.Encryptionpb.DataKey)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(org.tikv.kvproto.Encryptionpb.DataKey other) {
if (other == org.tikv.kvproto.Encryptionpb.DataKey.getDefaultInstance()) return this;
if (other.getKey() != com.google.protobuf.ByteString.EMPTY) {
setKey(other.getKey());
}
if (other.method_ != 0) {
setMethodValue(other.getMethodValue());
}
if (other.getCreationTime() != 0L) {
setCreationTime(other.getCreationTime());
}
if (other.getWasExposed() != false) {
setWasExposed(other.getWasExposed());
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
public final boolean isInitialized() {
return true;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
org.tikv.kvproto.Encryptionpb.DataKey parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (org.tikv.kvproto.Encryptionpb.DataKey) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private com.google.protobuf.ByteString key_ = com.google.protobuf.ByteString.EMPTY;
/**
*
* A sequence of secret bytes used to encrypt data.
*
*
* bytes key = 1;
*/
public com.google.protobuf.ByteString getKey() {
return key_;
}
/**
*
* A sequence of secret bytes used to encrypt data.
*
*
* bytes key = 1;
*/
public Builder setKey(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
key_ = value;
onChanged();
return this;
}
/**
*
* A sequence of secret bytes used to encrypt data.
*
*
* bytes key = 1;
*/
public Builder clearKey() {
key_ = getDefaultInstance().getKey();
onChanged();
return this;
}
private int method_ = 0;
/**
*
* Method of encryption algorithm used to encrypted data.
*
*
* .encryptionpb.EncryptionMethod method = 2;
*/
public int getMethodValue() {
return method_;
}
/**
*
* Method of encryption algorithm used to encrypted data.
*
*
* .encryptionpb.EncryptionMethod method = 2;
*/
public Builder setMethodValue(int value) {
method_ = value;
onChanged();
return this;
}
/**
*
* Method of encryption algorithm used to encrypted data.
*
*
* .encryptionpb.EncryptionMethod method = 2;
*/
public org.tikv.kvproto.Encryptionpb.EncryptionMethod getMethod() {
org.tikv.kvproto.Encryptionpb.EncryptionMethod result = org.tikv.kvproto.Encryptionpb.EncryptionMethod.valueOf(method_);
return result == null ? org.tikv.kvproto.Encryptionpb.EncryptionMethod.UNRECOGNIZED : result;
}
/**
*
* Method of encryption algorithm used to encrypted data.
*
*
* .encryptionpb.EncryptionMethod method = 2;
*/
public Builder setMethod(org.tikv.kvproto.Encryptionpb.EncryptionMethod value) {
if (value == null) {
throw new NullPointerException();
}
method_ = value.getNumber();
onChanged();
return this;
}
/**
*
* Method of encryption algorithm used to encrypted data.
*
*
* .encryptionpb.EncryptionMethod method = 2;
*/
public Builder clearMethod() {
method_ = 0;
onChanged();
return this;
}
private long creationTime_ ;
/**
*
* Creation time of the key.
*
*
* uint64 creation_time = 3;
*/
public long getCreationTime() {
return creationTime_;
}
/**
*
* Creation time of the key.
*
*
* uint64 creation_time = 3;
*/
public Builder setCreationTime(long value) {
creationTime_ = value;
onChanged();
return this;
}
/**
*
* Creation time of the key.
*
*
* uint64 creation_time = 3;
*/
public Builder clearCreationTime() {
creationTime_ = 0L;
onChanged();
return this;
}
private boolean wasExposed_ ;
/**
*
* A flag for the key have ever been exposed.
*
*
* bool was_exposed = 4;
*/
public boolean getWasExposed() {
return wasExposed_;
}
/**
*
* A flag for the key have ever been exposed.
*
*
* bool was_exposed = 4;
*/
public Builder setWasExposed(boolean value) {
wasExposed_ = value;
onChanged();
return this;
}
/**
*
* A flag for the key have ever been exposed.
*
*
* bool was_exposed = 4;
*/
public Builder clearWasExposed() {
wasExposed_ = false;
onChanged();
return this;
}
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFieldsProto3(unknownFields);
}
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:encryptionpb.DataKey)
}
// @@protoc_insertion_point(class_scope:encryptionpb.DataKey)
private static final org.tikv.kvproto.Encryptionpb.DataKey DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new org.tikv.kvproto.Encryptionpb.DataKey();
}
public static org.tikv.kvproto.Encryptionpb.DataKey getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
public DataKey parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new DataKey(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
public org.tikv.kvproto.Encryptionpb.DataKey getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface KeyDictionaryOrBuilder extends
// @@protoc_insertion_point(interface_extends:encryptionpb.KeyDictionary)
com.google.protobuf.MessageOrBuilder {
/**
*
* A map of key ID to dat key.
*
*
* map<uint64, .encryptionpb.DataKey> keys = 1;
*/
int getKeysCount();
/**
*
* A map of key ID to dat key.
*
*
* map<uint64, .encryptionpb.DataKey> keys = 1;
*/
boolean containsKeys(
long key);
/**
* Use {@link #getKeysMap()} instead.
*/
@java.lang.Deprecated
java.util.Map
getKeys();
/**
*
* A map of key ID to dat key.
*
*
* map<uint64, .encryptionpb.DataKey> keys = 1;
*/
java.util.Map
getKeysMap();
/**
*
* A map of key ID to dat key.
*
*
* map<uint64, .encryptionpb.DataKey> keys = 1;
*/
org.tikv.kvproto.Encryptionpb.DataKey getKeysOrDefault(
long key,
org.tikv.kvproto.Encryptionpb.DataKey defaultValue);
/**
*
* A map of key ID to dat key.
*
*
* map<uint64, .encryptionpb.DataKey> keys = 1;
*/
org.tikv.kvproto.Encryptionpb.DataKey getKeysOrThrow(
long key);
/**
*
* ID of a key currently in use.
*
*
* uint64 current_key_id = 2;
*/
long getCurrentKeyId();
}
/**
* Protobuf type {@code encryptionpb.KeyDictionary}
*/
public static final class KeyDictionary extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:encryptionpb.KeyDictionary)
KeyDictionaryOrBuilder {
private static final long serialVersionUID = 0L;
// Use KeyDictionary.newBuilder() to construct.
private KeyDictionary(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private KeyDictionary() {
currentKeyId_ = 0L;
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private KeyDictionary(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
default: {
if (!parseUnknownFieldProto3(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
case 10: {
if (!((mutable_bitField0_ & 0x00000001) == 0x00000001)) {
keys_ = com.google.protobuf.MapField.newMapField(
KeysDefaultEntryHolder.defaultEntry);
mutable_bitField0_ |= 0x00000001;
}
com.google.protobuf.MapEntry
keys__ = input.readMessage(
KeysDefaultEntryHolder.defaultEntry.getParserForType(), extensionRegistry);
keys_.getMutableMap().put(
keys__.getKey(), keys__.getValue());
break;
}
case 16: {
currentKeyId_ = input.readUInt64();
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.tikv.kvproto.Encryptionpb.internal_static_encryptionpb_KeyDictionary_descriptor;
}
@SuppressWarnings({"rawtypes"})
protected com.google.protobuf.MapField internalGetMapField(
int number) {
switch (number) {
case 1:
return internalGetKeys();
default:
throw new RuntimeException(
"Invalid map field number: " + number);
}
}
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.tikv.kvproto.Encryptionpb.internal_static_encryptionpb_KeyDictionary_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.tikv.kvproto.Encryptionpb.KeyDictionary.class, org.tikv.kvproto.Encryptionpb.KeyDictionary.Builder.class);
}
private int bitField0_;
public static final int KEYS_FIELD_NUMBER = 1;
private static final class KeysDefaultEntryHolder {
static final com.google.protobuf.MapEntry<
java.lang.Long, org.tikv.kvproto.Encryptionpb.DataKey> defaultEntry =
com.google.protobuf.MapEntry
.newDefaultInstance(
org.tikv.kvproto.Encryptionpb.internal_static_encryptionpb_KeyDictionary_KeysEntry_descriptor,
com.google.protobuf.WireFormat.FieldType.UINT64,
0L,
com.google.protobuf.WireFormat.FieldType.MESSAGE,
org.tikv.kvproto.Encryptionpb.DataKey.getDefaultInstance());
}
private com.google.protobuf.MapField<
java.lang.Long, org.tikv.kvproto.Encryptionpb.DataKey> keys_;
private com.google.protobuf.MapField
internalGetKeys() {
if (keys_ == null) {
return com.google.protobuf.MapField.emptyMapField(
KeysDefaultEntryHolder.defaultEntry);
}
return keys_;
}
public int getKeysCount() {
return internalGetKeys().getMap().size();
}
/**
*
* A map of key ID to dat key.
*
*
* map<uint64, .encryptionpb.DataKey> keys = 1;
*/
public boolean containsKeys(
long key) {
return internalGetKeys().getMap().containsKey(key);
}
/**
* Use {@link #getKeysMap()} instead.
*/
@java.lang.Deprecated
public java.util.Map getKeys() {
return getKeysMap();
}
/**
*
* A map of key ID to dat key.
*
*
* map<uint64, .encryptionpb.DataKey> keys = 1;
*/
public java.util.Map getKeysMap() {
return internalGetKeys().getMap();
}
/**
*
* A map of key ID to dat key.
*
*
* map<uint64, .encryptionpb.DataKey> keys = 1;
*/
public org.tikv.kvproto.Encryptionpb.DataKey getKeysOrDefault(
long key,
org.tikv.kvproto.Encryptionpb.DataKey defaultValue) {
java.util.Map map =
internalGetKeys().getMap();
return map.containsKey(key) ? map.get(key) : defaultValue;
}
/**
*
* A map of key ID to dat key.
*
*
* map<uint64, .encryptionpb.DataKey> keys = 1;
*/
public org.tikv.kvproto.Encryptionpb.DataKey getKeysOrThrow(
long key) {
java.util.Map map =
internalGetKeys().getMap();
if (!map.containsKey(key)) {
throw new java.lang.IllegalArgumentException();
}
return map.get(key);
}
public static final int CURRENT_KEY_ID_FIELD_NUMBER = 2;
private long currentKeyId_;
/**
*
* ID of a key currently in use.
*
*
* uint64 current_key_id = 2;
*/
public long getCurrentKeyId() {
return currentKeyId_;
}
private byte memoizedIsInitialized = -1;
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
com.google.protobuf.GeneratedMessageV3
.serializeLongMapTo(
output,
internalGetKeys(),
KeysDefaultEntryHolder.defaultEntry,
1);
if (currentKeyId_ != 0L) {
output.writeUInt64(2, currentKeyId_);
}
unknownFields.writeTo(output);
}
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
for (java.util.Map.Entry entry
: internalGetKeys().getMap().entrySet()) {
com.google.protobuf.MapEntry
keys__ = KeysDefaultEntryHolder.defaultEntry.newBuilderForType()
.setKey(entry.getKey())
.setValue(entry.getValue())
.build();
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(1, keys__);
}
if (currentKeyId_ != 0L) {
size += com.google.protobuf.CodedOutputStream
.computeUInt64Size(2, currentKeyId_);
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof org.tikv.kvproto.Encryptionpb.KeyDictionary)) {
return super.equals(obj);
}
org.tikv.kvproto.Encryptionpb.KeyDictionary other = (org.tikv.kvproto.Encryptionpb.KeyDictionary) obj;
boolean result = true;
result = result && internalGetKeys().equals(
other.internalGetKeys());
result = result && (getCurrentKeyId()
== other.getCurrentKeyId());
result = result && unknownFields.equals(other.unknownFields);
return result;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (!internalGetKeys().getMap().isEmpty()) {
hash = (37 * hash) + KEYS_FIELD_NUMBER;
hash = (53 * hash) + internalGetKeys().hashCode();
}
hash = (37 * hash) + CURRENT_KEY_ID_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
getCurrentKeyId());
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static org.tikv.kvproto.Encryptionpb.KeyDictionary parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.tikv.kvproto.Encryptionpb.KeyDictionary parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.tikv.kvproto.Encryptionpb.KeyDictionary parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.tikv.kvproto.Encryptionpb.KeyDictionary parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.tikv.kvproto.Encryptionpb.KeyDictionary parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.tikv.kvproto.Encryptionpb.KeyDictionary parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.tikv.kvproto.Encryptionpb.KeyDictionary parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static org.tikv.kvproto.Encryptionpb.KeyDictionary parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static org.tikv.kvproto.Encryptionpb.KeyDictionary parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static org.tikv.kvproto.Encryptionpb.KeyDictionary parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static org.tikv.kvproto.Encryptionpb.KeyDictionary parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static org.tikv.kvproto.Encryptionpb.KeyDictionary parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(org.tikv.kvproto.Encryptionpb.KeyDictionary prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code encryptionpb.KeyDictionary}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:encryptionpb.KeyDictionary)
org.tikv.kvproto.Encryptionpb.KeyDictionaryOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.tikv.kvproto.Encryptionpb.internal_static_encryptionpb_KeyDictionary_descriptor;
}
@SuppressWarnings({"rawtypes"})
protected com.google.protobuf.MapField internalGetMapField(
int number) {
switch (number) {
case 1:
return internalGetKeys();
default:
throw new RuntimeException(
"Invalid map field number: " + number);
}
}
@SuppressWarnings({"rawtypes"})
protected com.google.protobuf.MapField internalGetMutableMapField(
int number) {
switch (number) {
case 1:
return internalGetMutableKeys();
default:
throw new RuntimeException(
"Invalid map field number: " + number);
}
}
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.tikv.kvproto.Encryptionpb.internal_static_encryptionpb_KeyDictionary_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.tikv.kvproto.Encryptionpb.KeyDictionary.class, org.tikv.kvproto.Encryptionpb.KeyDictionary.Builder.class);
}
// Construct using org.tikv.kvproto.Encryptionpb.KeyDictionary.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
public Builder clear() {
super.clear();
internalGetMutableKeys().clear();
currentKeyId_ = 0L;
return this;
}
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return org.tikv.kvproto.Encryptionpb.internal_static_encryptionpb_KeyDictionary_descriptor;
}
public org.tikv.kvproto.Encryptionpb.KeyDictionary getDefaultInstanceForType() {
return org.tikv.kvproto.Encryptionpb.KeyDictionary.getDefaultInstance();
}
public org.tikv.kvproto.Encryptionpb.KeyDictionary build() {
org.tikv.kvproto.Encryptionpb.KeyDictionary result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
public org.tikv.kvproto.Encryptionpb.KeyDictionary buildPartial() {
org.tikv.kvproto.Encryptionpb.KeyDictionary result = new org.tikv.kvproto.Encryptionpb.KeyDictionary(this);
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
result.keys_ = internalGetKeys();
result.keys_.makeImmutable();
result.currentKeyId_ = currentKeyId_;
result.bitField0_ = to_bitField0_;
onBuilt();
return result;
}
public Builder clone() {
return (Builder) super.clone();
}
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.setField(field, value);
}
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return (Builder) super.clearField(field);
}
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return (Builder) super.clearOneof(oneof);
}
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return (Builder) super.setRepeatedField(field, index, value);
}
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.addRepeatedField(field, value);
}
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof org.tikv.kvproto.Encryptionpb.KeyDictionary) {
return mergeFrom((org.tikv.kvproto.Encryptionpb.KeyDictionary)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(org.tikv.kvproto.Encryptionpb.KeyDictionary other) {
if (other == org.tikv.kvproto.Encryptionpb.KeyDictionary.getDefaultInstance()) return this;
internalGetMutableKeys().mergeFrom(
other.internalGetKeys());
if (other.getCurrentKeyId() != 0L) {
setCurrentKeyId(other.getCurrentKeyId());
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
public final boolean isInitialized() {
return true;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
org.tikv.kvproto.Encryptionpb.KeyDictionary parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (org.tikv.kvproto.Encryptionpb.KeyDictionary) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
private com.google.protobuf.MapField<
java.lang.Long, org.tikv.kvproto.Encryptionpb.DataKey> keys_;
private com.google.protobuf.MapField
internalGetKeys() {
if (keys_ == null) {
return com.google.protobuf.MapField.emptyMapField(
KeysDefaultEntryHolder.defaultEntry);
}
return keys_;
}
private com.google.protobuf.MapField
internalGetMutableKeys() {
onChanged();;
if (keys_ == null) {
keys_ = com.google.protobuf.MapField.newMapField(
KeysDefaultEntryHolder.defaultEntry);
}
if (!keys_.isMutable()) {
keys_ = keys_.copy();
}
return keys_;
}
public int getKeysCount() {
return internalGetKeys().getMap().size();
}
/**
*
* A map of key ID to dat key.
*
*
* map<uint64, .encryptionpb.DataKey> keys = 1;
*/
public boolean containsKeys(
long key) {
return internalGetKeys().getMap().containsKey(key);
}
/**
* Use {@link #getKeysMap()} instead.
*/
@java.lang.Deprecated
public java.util.Map getKeys() {
return getKeysMap();
}
/**
*
* A map of key ID to dat key.
*
*
* map<uint64, .encryptionpb.DataKey> keys = 1;
*/
public java.util.Map getKeysMap() {
return internalGetKeys().getMap();
}
/**
*
* A map of key ID to dat key.
*
*
* map<uint64, .encryptionpb.DataKey> keys = 1;
*/
public org.tikv.kvproto.Encryptionpb.DataKey getKeysOrDefault(
long key,
org.tikv.kvproto.Encryptionpb.DataKey defaultValue) {
java.util.Map map =
internalGetKeys().getMap();
return map.containsKey(key) ? map.get(key) : defaultValue;
}
/**
*
* A map of key ID to dat key.
*
*
* map<uint64, .encryptionpb.DataKey> keys = 1;
*/
public org.tikv.kvproto.Encryptionpb.DataKey getKeysOrThrow(
long key) {
java.util.Map map =
internalGetKeys().getMap();
if (!map.containsKey(key)) {
throw new java.lang.IllegalArgumentException();
}
return map.get(key);
}
public Builder clearKeys() {
internalGetMutableKeys().getMutableMap()
.clear();
return this;
}
/**
*
* A map of key ID to dat key.
*
*
* map<uint64, .encryptionpb.DataKey> keys = 1;
*/
public Builder removeKeys(
long key) {
internalGetMutableKeys().getMutableMap()
.remove(key);
return this;
}
/**
* Use alternate mutation accessors instead.
*/
@java.lang.Deprecated
public java.util.Map
getMutableKeys() {
return internalGetMutableKeys().getMutableMap();
}
/**
*
* A map of key ID to dat key.
*
*
* map<uint64, .encryptionpb.DataKey> keys = 1;
*/
public Builder putKeys(
long key,
org.tikv.kvproto.Encryptionpb.DataKey value) {
if (value == null) { throw new java.lang.NullPointerException(); }
internalGetMutableKeys().getMutableMap()
.put(key, value);
return this;
}
/**
*
* A map of key ID to dat key.
*
*
* map<uint64, .encryptionpb.DataKey> keys = 1;
*/
public Builder putAllKeys(
java.util.Map values) {
internalGetMutableKeys().getMutableMap()
.putAll(values);
return this;
}
private long currentKeyId_ ;
/**
*
* ID of a key currently in use.
*
*
* uint64 current_key_id = 2;
*/
public long getCurrentKeyId() {
return currentKeyId_;
}
/**
*
* ID of a key currently in use.
*
*
* uint64 current_key_id = 2;
*/
public Builder setCurrentKeyId(long value) {
currentKeyId_ = value;
onChanged();
return this;
}
/**
*
* ID of a key currently in use.
*
*
* uint64 current_key_id = 2;
*/
public Builder clearCurrentKeyId() {
currentKeyId_ = 0L;
onChanged();
return this;
}
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFieldsProto3(unknownFields);
}
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:encryptionpb.KeyDictionary)
}
// @@protoc_insertion_point(class_scope:encryptionpb.KeyDictionary)
private static final org.tikv.kvproto.Encryptionpb.KeyDictionary DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new org.tikv.kvproto.Encryptionpb.KeyDictionary();
}
public static org.tikv.kvproto.Encryptionpb.KeyDictionary getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
public KeyDictionary parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new KeyDictionary(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
public org.tikv.kvproto.Encryptionpb.KeyDictionary getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface MasterKeyOrBuilder extends
// @@protoc_insertion_point(interface_extends:encryptionpb.MasterKey)
com.google.protobuf.MessageOrBuilder {
/**
* .encryptionpb.MasterKeyPlaintext plaintext = 1;
*/
boolean hasPlaintext();
/**
* .encryptionpb.MasterKeyPlaintext plaintext = 1;
*/
org.tikv.kvproto.Encryptionpb.MasterKeyPlaintext getPlaintext();
/**
* .encryptionpb.MasterKeyPlaintext plaintext = 1;
*/
org.tikv.kvproto.Encryptionpb.MasterKeyPlaintextOrBuilder getPlaintextOrBuilder();
/**
* .encryptionpb.MasterKeyFile file = 2;
*/
boolean hasFile();
/**
* .encryptionpb.MasterKeyFile file = 2;
*/
org.tikv.kvproto.Encryptionpb.MasterKeyFile getFile();
/**
* .encryptionpb.MasterKeyFile file = 2;
*/
org.tikv.kvproto.Encryptionpb.MasterKeyFileOrBuilder getFileOrBuilder();
/**
* .encryptionpb.MasterKeyKms kms = 3;
*/
boolean hasKms();
/**
* .encryptionpb.MasterKeyKms kms = 3;
*/
org.tikv.kvproto.Encryptionpb.MasterKeyKms getKms();
/**
* .encryptionpb.MasterKeyKms kms = 3;
*/
org.tikv.kvproto.Encryptionpb.MasterKeyKmsOrBuilder getKmsOrBuilder();
public org.tikv.kvproto.Encryptionpb.MasterKey.BackendCase getBackendCase();
}
/**
*
* Master key config.
*
*
* Protobuf type {@code encryptionpb.MasterKey}
*/
public static final class MasterKey extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:encryptionpb.MasterKey)
MasterKeyOrBuilder {
private static final long serialVersionUID = 0L;
// Use MasterKey.newBuilder() to construct.
private MasterKey(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private MasterKey() {
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private MasterKey(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
default: {
if (!parseUnknownFieldProto3(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
case 10: {
org.tikv.kvproto.Encryptionpb.MasterKeyPlaintext.Builder subBuilder = null;
if (backendCase_ == 1) {
subBuilder = ((org.tikv.kvproto.Encryptionpb.MasterKeyPlaintext) backend_).toBuilder();
}
backend_ =
input.readMessage(org.tikv.kvproto.Encryptionpb.MasterKeyPlaintext.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom((org.tikv.kvproto.Encryptionpb.MasterKeyPlaintext) backend_);
backend_ = subBuilder.buildPartial();
}
backendCase_ = 1;
break;
}
case 18: {
org.tikv.kvproto.Encryptionpb.MasterKeyFile.Builder subBuilder = null;
if (backendCase_ == 2) {
subBuilder = ((org.tikv.kvproto.Encryptionpb.MasterKeyFile) backend_).toBuilder();
}
backend_ =
input.readMessage(org.tikv.kvproto.Encryptionpb.MasterKeyFile.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom((org.tikv.kvproto.Encryptionpb.MasterKeyFile) backend_);
backend_ = subBuilder.buildPartial();
}
backendCase_ = 2;
break;
}
case 26: {
org.tikv.kvproto.Encryptionpb.MasterKeyKms.Builder subBuilder = null;
if (backendCase_ == 3) {
subBuilder = ((org.tikv.kvproto.Encryptionpb.MasterKeyKms) backend_).toBuilder();
}
backend_ =
input.readMessage(org.tikv.kvproto.Encryptionpb.MasterKeyKms.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom((org.tikv.kvproto.Encryptionpb.MasterKeyKms) backend_);
backend_ = subBuilder.buildPartial();
}
backendCase_ = 3;
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.tikv.kvproto.Encryptionpb.internal_static_encryptionpb_MasterKey_descriptor;
}
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.tikv.kvproto.Encryptionpb.internal_static_encryptionpb_MasterKey_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.tikv.kvproto.Encryptionpb.MasterKey.class, org.tikv.kvproto.Encryptionpb.MasterKey.Builder.class);
}
private int backendCase_ = 0;
private java.lang.Object backend_;
public enum BackendCase
implements com.google.protobuf.Internal.EnumLite {
PLAINTEXT(1),
FILE(2),
KMS(3),
BACKEND_NOT_SET(0);
private final int value;
private BackendCase(int value) {
this.value = value;
}
/**
* @deprecated Use {@link #forNumber(int)} instead.
*/
@java.lang.Deprecated
public static BackendCase valueOf(int value) {
return forNumber(value);
}
public static BackendCase forNumber(int value) {
switch (value) {
case 1: return PLAINTEXT;
case 2: return FILE;
case 3: return KMS;
case 0: return BACKEND_NOT_SET;
default: return null;
}
}
public int getNumber() {
return this.value;
}
};
public BackendCase
getBackendCase() {
return BackendCase.forNumber(
backendCase_);
}
public static final int PLAINTEXT_FIELD_NUMBER = 1;
/**
* .encryptionpb.MasterKeyPlaintext plaintext = 1;
*/
public boolean hasPlaintext() {
return backendCase_ == 1;
}
/**
* .encryptionpb.MasterKeyPlaintext plaintext = 1;
*/
public org.tikv.kvproto.Encryptionpb.MasterKeyPlaintext getPlaintext() {
if (backendCase_ == 1) {
return (org.tikv.kvproto.Encryptionpb.MasterKeyPlaintext) backend_;
}
return org.tikv.kvproto.Encryptionpb.MasterKeyPlaintext.getDefaultInstance();
}
/**
* .encryptionpb.MasterKeyPlaintext plaintext = 1;
*/
public org.tikv.kvproto.Encryptionpb.MasterKeyPlaintextOrBuilder getPlaintextOrBuilder() {
if (backendCase_ == 1) {
return (org.tikv.kvproto.Encryptionpb.MasterKeyPlaintext) backend_;
}
return org.tikv.kvproto.Encryptionpb.MasterKeyPlaintext.getDefaultInstance();
}
public static final int FILE_FIELD_NUMBER = 2;
/**
* .encryptionpb.MasterKeyFile file = 2;
*/
public boolean hasFile() {
return backendCase_ == 2;
}
/**
* .encryptionpb.MasterKeyFile file = 2;
*/
public org.tikv.kvproto.Encryptionpb.MasterKeyFile getFile() {
if (backendCase_ == 2) {
return (org.tikv.kvproto.Encryptionpb.MasterKeyFile) backend_;
}
return org.tikv.kvproto.Encryptionpb.MasterKeyFile.getDefaultInstance();
}
/**
* .encryptionpb.MasterKeyFile file = 2;
*/
public org.tikv.kvproto.Encryptionpb.MasterKeyFileOrBuilder getFileOrBuilder() {
if (backendCase_ == 2) {
return (org.tikv.kvproto.Encryptionpb.MasterKeyFile) backend_;
}
return org.tikv.kvproto.Encryptionpb.MasterKeyFile.getDefaultInstance();
}
public static final int KMS_FIELD_NUMBER = 3;
/**
* .encryptionpb.MasterKeyKms kms = 3;
*/
public boolean hasKms() {
return backendCase_ == 3;
}
/**
* .encryptionpb.MasterKeyKms kms = 3;
*/
public org.tikv.kvproto.Encryptionpb.MasterKeyKms getKms() {
if (backendCase_ == 3) {
return (org.tikv.kvproto.Encryptionpb.MasterKeyKms) backend_;
}
return org.tikv.kvproto.Encryptionpb.MasterKeyKms.getDefaultInstance();
}
/**
* .encryptionpb.MasterKeyKms kms = 3;
*/
public org.tikv.kvproto.Encryptionpb.MasterKeyKmsOrBuilder getKmsOrBuilder() {
if (backendCase_ == 3) {
return (org.tikv.kvproto.Encryptionpb.MasterKeyKms) backend_;
}
return org.tikv.kvproto.Encryptionpb.MasterKeyKms.getDefaultInstance();
}
private byte memoizedIsInitialized = -1;
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (backendCase_ == 1) {
output.writeMessage(1, (org.tikv.kvproto.Encryptionpb.MasterKeyPlaintext) backend_);
}
if (backendCase_ == 2) {
output.writeMessage(2, (org.tikv.kvproto.Encryptionpb.MasterKeyFile) backend_);
}
if (backendCase_ == 3) {
output.writeMessage(3, (org.tikv.kvproto.Encryptionpb.MasterKeyKms) backend_);
}
unknownFields.writeTo(output);
}
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (backendCase_ == 1) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(1, (org.tikv.kvproto.Encryptionpb.MasterKeyPlaintext) backend_);
}
if (backendCase_ == 2) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(2, (org.tikv.kvproto.Encryptionpb.MasterKeyFile) backend_);
}
if (backendCase_ == 3) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(3, (org.tikv.kvproto.Encryptionpb.MasterKeyKms) backend_);
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof org.tikv.kvproto.Encryptionpb.MasterKey)) {
return super.equals(obj);
}
org.tikv.kvproto.Encryptionpb.MasterKey other = (org.tikv.kvproto.Encryptionpb.MasterKey) obj;
boolean result = true;
result = result && getBackendCase().equals(
other.getBackendCase());
if (!result) return false;
switch (backendCase_) {
case 1:
result = result && getPlaintext()
.equals(other.getPlaintext());
break;
case 2:
result = result && getFile()
.equals(other.getFile());
break;
case 3:
result = result && getKms()
.equals(other.getKms());
break;
case 0:
default:
}
result = result && unknownFields.equals(other.unknownFields);
return result;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
switch (backendCase_) {
case 1:
hash = (37 * hash) + PLAINTEXT_FIELD_NUMBER;
hash = (53 * hash) + getPlaintext().hashCode();
break;
case 2:
hash = (37 * hash) + FILE_FIELD_NUMBER;
hash = (53 * hash) + getFile().hashCode();
break;
case 3:
hash = (37 * hash) + KMS_FIELD_NUMBER;
hash = (53 * hash) + getKms().hashCode();
break;
case 0:
default:
}
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static org.tikv.kvproto.Encryptionpb.MasterKey parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.tikv.kvproto.Encryptionpb.MasterKey parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.tikv.kvproto.Encryptionpb.MasterKey parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.tikv.kvproto.Encryptionpb.MasterKey parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.tikv.kvproto.Encryptionpb.MasterKey parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.tikv.kvproto.Encryptionpb.MasterKey parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.tikv.kvproto.Encryptionpb.MasterKey parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static org.tikv.kvproto.Encryptionpb.MasterKey parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static org.tikv.kvproto.Encryptionpb.MasterKey parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static org.tikv.kvproto.Encryptionpb.MasterKey parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static org.tikv.kvproto.Encryptionpb.MasterKey parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static org.tikv.kvproto.Encryptionpb.MasterKey parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(org.tikv.kvproto.Encryptionpb.MasterKey prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
* Master key config.
*
*
* Protobuf type {@code encryptionpb.MasterKey}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:encryptionpb.MasterKey)
org.tikv.kvproto.Encryptionpb.MasterKeyOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.tikv.kvproto.Encryptionpb.internal_static_encryptionpb_MasterKey_descriptor;
}
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.tikv.kvproto.Encryptionpb.internal_static_encryptionpb_MasterKey_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.tikv.kvproto.Encryptionpb.MasterKey.class, org.tikv.kvproto.Encryptionpb.MasterKey.Builder.class);
}
// Construct using org.tikv.kvproto.Encryptionpb.MasterKey.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
public Builder clear() {
super.clear();
backendCase_ = 0;
backend_ = null;
return this;
}
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return org.tikv.kvproto.Encryptionpb.internal_static_encryptionpb_MasterKey_descriptor;
}
public org.tikv.kvproto.Encryptionpb.MasterKey getDefaultInstanceForType() {
return org.tikv.kvproto.Encryptionpb.MasterKey.getDefaultInstance();
}
public org.tikv.kvproto.Encryptionpb.MasterKey build() {
org.tikv.kvproto.Encryptionpb.MasterKey result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
public org.tikv.kvproto.Encryptionpb.MasterKey buildPartial() {
org.tikv.kvproto.Encryptionpb.MasterKey result = new org.tikv.kvproto.Encryptionpb.MasterKey(this);
if (backendCase_ == 1) {
if (plaintextBuilder_ == null) {
result.backend_ = backend_;
} else {
result.backend_ = plaintextBuilder_.build();
}
}
if (backendCase_ == 2) {
if (fileBuilder_ == null) {
result.backend_ = backend_;
} else {
result.backend_ = fileBuilder_.build();
}
}
if (backendCase_ == 3) {
if (kmsBuilder_ == null) {
result.backend_ = backend_;
} else {
result.backend_ = kmsBuilder_.build();
}
}
result.backendCase_ = backendCase_;
onBuilt();
return result;
}
public Builder clone() {
return (Builder) super.clone();
}
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.setField(field, value);
}
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return (Builder) super.clearField(field);
}
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return (Builder) super.clearOneof(oneof);
}
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return (Builder) super.setRepeatedField(field, index, value);
}
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.addRepeatedField(field, value);
}
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof org.tikv.kvproto.Encryptionpb.MasterKey) {
return mergeFrom((org.tikv.kvproto.Encryptionpb.MasterKey)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(org.tikv.kvproto.Encryptionpb.MasterKey other) {
if (other == org.tikv.kvproto.Encryptionpb.MasterKey.getDefaultInstance()) return this;
switch (other.getBackendCase()) {
case PLAINTEXT: {
mergePlaintext(other.getPlaintext());
break;
}
case FILE: {
mergeFile(other.getFile());
break;
}
case KMS: {
mergeKms(other.getKms());
break;
}
case BACKEND_NOT_SET: {
break;
}
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
public final boolean isInitialized() {
return true;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
org.tikv.kvproto.Encryptionpb.MasterKey parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (org.tikv.kvproto.Encryptionpb.MasterKey) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int backendCase_ = 0;
private java.lang.Object backend_;
public BackendCase
getBackendCase() {
return BackendCase.forNumber(
backendCase_);
}
public Builder clearBackend() {
backendCase_ = 0;
backend_ = null;
onChanged();
return this;
}
private com.google.protobuf.SingleFieldBuilderV3<
org.tikv.kvproto.Encryptionpb.MasterKeyPlaintext, org.tikv.kvproto.Encryptionpb.MasterKeyPlaintext.Builder, org.tikv.kvproto.Encryptionpb.MasterKeyPlaintextOrBuilder> plaintextBuilder_;
/**
* .encryptionpb.MasterKeyPlaintext plaintext = 1;
*/
public boolean hasPlaintext() {
return backendCase_ == 1;
}
/**
* .encryptionpb.MasterKeyPlaintext plaintext = 1;
*/
public org.tikv.kvproto.Encryptionpb.MasterKeyPlaintext getPlaintext() {
if (plaintextBuilder_ == null) {
if (backendCase_ == 1) {
return (org.tikv.kvproto.Encryptionpb.MasterKeyPlaintext) backend_;
}
return org.tikv.kvproto.Encryptionpb.MasterKeyPlaintext.getDefaultInstance();
} else {
if (backendCase_ == 1) {
return plaintextBuilder_.getMessage();
}
return org.tikv.kvproto.Encryptionpb.MasterKeyPlaintext.getDefaultInstance();
}
}
/**
* .encryptionpb.MasterKeyPlaintext plaintext = 1;
*/
public Builder setPlaintext(org.tikv.kvproto.Encryptionpb.MasterKeyPlaintext value) {
if (plaintextBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
backend_ = value;
onChanged();
} else {
plaintextBuilder_.setMessage(value);
}
backendCase_ = 1;
return this;
}
/**
* .encryptionpb.MasterKeyPlaintext plaintext = 1;
*/
public Builder setPlaintext(
org.tikv.kvproto.Encryptionpb.MasterKeyPlaintext.Builder builderForValue) {
if (plaintextBuilder_ == null) {
backend_ = builderForValue.build();
onChanged();
} else {
plaintextBuilder_.setMessage(builderForValue.build());
}
backendCase_ = 1;
return this;
}
/**
* .encryptionpb.MasterKeyPlaintext plaintext = 1;
*/
public Builder mergePlaintext(org.tikv.kvproto.Encryptionpb.MasterKeyPlaintext value) {
if (plaintextBuilder_ == null) {
if (backendCase_ == 1 &&
backend_ != org.tikv.kvproto.Encryptionpb.MasterKeyPlaintext.getDefaultInstance()) {
backend_ = org.tikv.kvproto.Encryptionpb.MasterKeyPlaintext.newBuilder((org.tikv.kvproto.Encryptionpb.MasterKeyPlaintext) backend_)
.mergeFrom(value).buildPartial();
} else {
backend_ = value;
}
onChanged();
} else {
if (backendCase_ == 1) {
plaintextBuilder_.mergeFrom(value);
}
plaintextBuilder_.setMessage(value);
}
backendCase_ = 1;
return this;
}
/**
* .encryptionpb.MasterKeyPlaintext plaintext = 1;
*/
public Builder clearPlaintext() {
if (plaintextBuilder_ == null) {
if (backendCase_ == 1) {
backendCase_ = 0;
backend_ = null;
onChanged();
}
} else {
if (backendCase_ == 1) {
backendCase_ = 0;
backend_ = null;
}
plaintextBuilder_.clear();
}
return this;
}
/**
* .encryptionpb.MasterKeyPlaintext plaintext = 1;
*/
public org.tikv.kvproto.Encryptionpb.MasterKeyPlaintext.Builder getPlaintextBuilder() {
return getPlaintextFieldBuilder().getBuilder();
}
/**
* .encryptionpb.MasterKeyPlaintext plaintext = 1;
*/
public org.tikv.kvproto.Encryptionpb.MasterKeyPlaintextOrBuilder getPlaintextOrBuilder() {
if ((backendCase_ == 1) && (plaintextBuilder_ != null)) {
return plaintextBuilder_.getMessageOrBuilder();
} else {
if (backendCase_ == 1) {
return (org.tikv.kvproto.Encryptionpb.MasterKeyPlaintext) backend_;
}
return org.tikv.kvproto.Encryptionpb.MasterKeyPlaintext.getDefaultInstance();
}
}
/**
* .encryptionpb.MasterKeyPlaintext plaintext = 1;
*/
private com.google.protobuf.SingleFieldBuilderV3<
org.tikv.kvproto.Encryptionpb.MasterKeyPlaintext, org.tikv.kvproto.Encryptionpb.MasterKeyPlaintext.Builder, org.tikv.kvproto.Encryptionpb.MasterKeyPlaintextOrBuilder>
getPlaintextFieldBuilder() {
if (plaintextBuilder_ == null) {
if (!(backendCase_ == 1)) {
backend_ = org.tikv.kvproto.Encryptionpb.MasterKeyPlaintext.getDefaultInstance();
}
plaintextBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
org.tikv.kvproto.Encryptionpb.MasterKeyPlaintext, org.tikv.kvproto.Encryptionpb.MasterKeyPlaintext.Builder, org.tikv.kvproto.Encryptionpb.MasterKeyPlaintextOrBuilder>(
(org.tikv.kvproto.Encryptionpb.MasterKeyPlaintext) backend_,
getParentForChildren(),
isClean());
backend_ = null;
}
backendCase_ = 1;
onChanged();;
return plaintextBuilder_;
}
private com.google.protobuf.SingleFieldBuilderV3<
org.tikv.kvproto.Encryptionpb.MasterKeyFile, org.tikv.kvproto.Encryptionpb.MasterKeyFile.Builder, org.tikv.kvproto.Encryptionpb.MasterKeyFileOrBuilder> fileBuilder_;
/**
* .encryptionpb.MasterKeyFile file = 2;
*/
public boolean hasFile() {
return backendCase_ == 2;
}
/**
* .encryptionpb.MasterKeyFile file = 2;
*/
public org.tikv.kvproto.Encryptionpb.MasterKeyFile getFile() {
if (fileBuilder_ == null) {
if (backendCase_ == 2) {
return (org.tikv.kvproto.Encryptionpb.MasterKeyFile) backend_;
}
return org.tikv.kvproto.Encryptionpb.MasterKeyFile.getDefaultInstance();
} else {
if (backendCase_ == 2) {
return fileBuilder_.getMessage();
}
return org.tikv.kvproto.Encryptionpb.MasterKeyFile.getDefaultInstance();
}
}
/**
* .encryptionpb.MasterKeyFile file = 2;
*/
public Builder setFile(org.tikv.kvproto.Encryptionpb.MasterKeyFile value) {
if (fileBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
backend_ = value;
onChanged();
} else {
fileBuilder_.setMessage(value);
}
backendCase_ = 2;
return this;
}
/**
* .encryptionpb.MasterKeyFile file = 2;
*/
public Builder setFile(
org.tikv.kvproto.Encryptionpb.MasterKeyFile.Builder builderForValue) {
if (fileBuilder_ == null) {
backend_ = builderForValue.build();
onChanged();
} else {
fileBuilder_.setMessage(builderForValue.build());
}
backendCase_ = 2;
return this;
}
/**
* .encryptionpb.MasterKeyFile file = 2;
*/
public Builder mergeFile(org.tikv.kvproto.Encryptionpb.MasterKeyFile value) {
if (fileBuilder_ == null) {
if (backendCase_ == 2 &&
backend_ != org.tikv.kvproto.Encryptionpb.MasterKeyFile.getDefaultInstance()) {
backend_ = org.tikv.kvproto.Encryptionpb.MasterKeyFile.newBuilder((org.tikv.kvproto.Encryptionpb.MasterKeyFile) backend_)
.mergeFrom(value).buildPartial();
} else {
backend_ = value;
}
onChanged();
} else {
if (backendCase_ == 2) {
fileBuilder_.mergeFrom(value);
}
fileBuilder_.setMessage(value);
}
backendCase_ = 2;
return this;
}
/**
* .encryptionpb.MasterKeyFile file = 2;
*/
public Builder clearFile() {
if (fileBuilder_ == null) {
if (backendCase_ == 2) {
backendCase_ = 0;
backend_ = null;
onChanged();
}
} else {
if (backendCase_ == 2) {
backendCase_ = 0;
backend_ = null;
}
fileBuilder_.clear();
}
return this;
}
/**
* .encryptionpb.MasterKeyFile file = 2;
*/
public org.tikv.kvproto.Encryptionpb.MasterKeyFile.Builder getFileBuilder() {
return getFileFieldBuilder().getBuilder();
}
/**
* .encryptionpb.MasterKeyFile file = 2;
*/
public org.tikv.kvproto.Encryptionpb.MasterKeyFileOrBuilder getFileOrBuilder() {
if ((backendCase_ == 2) && (fileBuilder_ != null)) {
return fileBuilder_.getMessageOrBuilder();
} else {
if (backendCase_ == 2) {
return (org.tikv.kvproto.Encryptionpb.MasterKeyFile) backend_;
}
return org.tikv.kvproto.Encryptionpb.MasterKeyFile.getDefaultInstance();
}
}
/**
* .encryptionpb.MasterKeyFile file = 2;
*/
private com.google.protobuf.SingleFieldBuilderV3<
org.tikv.kvproto.Encryptionpb.MasterKeyFile, org.tikv.kvproto.Encryptionpb.MasterKeyFile.Builder, org.tikv.kvproto.Encryptionpb.MasterKeyFileOrBuilder>
getFileFieldBuilder() {
if (fileBuilder_ == null) {
if (!(backendCase_ == 2)) {
backend_ = org.tikv.kvproto.Encryptionpb.MasterKeyFile.getDefaultInstance();
}
fileBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
org.tikv.kvproto.Encryptionpb.MasterKeyFile, org.tikv.kvproto.Encryptionpb.MasterKeyFile.Builder, org.tikv.kvproto.Encryptionpb.MasterKeyFileOrBuilder>(
(org.tikv.kvproto.Encryptionpb.MasterKeyFile) backend_,
getParentForChildren(),
isClean());
backend_ = null;
}
backendCase_ = 2;
onChanged();;
return fileBuilder_;
}
private com.google.protobuf.SingleFieldBuilderV3<
org.tikv.kvproto.Encryptionpb.MasterKeyKms, org.tikv.kvproto.Encryptionpb.MasterKeyKms.Builder, org.tikv.kvproto.Encryptionpb.MasterKeyKmsOrBuilder> kmsBuilder_;
/**
* .encryptionpb.MasterKeyKms kms = 3;
*/
public boolean hasKms() {
return backendCase_ == 3;
}
/**
* .encryptionpb.MasterKeyKms kms = 3;
*/
public org.tikv.kvproto.Encryptionpb.MasterKeyKms getKms() {
if (kmsBuilder_ == null) {
if (backendCase_ == 3) {
return (org.tikv.kvproto.Encryptionpb.MasterKeyKms) backend_;
}
return org.tikv.kvproto.Encryptionpb.MasterKeyKms.getDefaultInstance();
} else {
if (backendCase_ == 3) {
return kmsBuilder_.getMessage();
}
return org.tikv.kvproto.Encryptionpb.MasterKeyKms.getDefaultInstance();
}
}
/**
* .encryptionpb.MasterKeyKms kms = 3;
*/
public Builder setKms(org.tikv.kvproto.Encryptionpb.MasterKeyKms value) {
if (kmsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
backend_ = value;
onChanged();
} else {
kmsBuilder_.setMessage(value);
}
backendCase_ = 3;
return this;
}
/**
* .encryptionpb.MasterKeyKms kms = 3;
*/
public Builder setKms(
org.tikv.kvproto.Encryptionpb.MasterKeyKms.Builder builderForValue) {
if (kmsBuilder_ == null) {
backend_ = builderForValue.build();
onChanged();
} else {
kmsBuilder_.setMessage(builderForValue.build());
}
backendCase_ = 3;
return this;
}
/**
* .encryptionpb.MasterKeyKms kms = 3;
*/
public Builder mergeKms(org.tikv.kvproto.Encryptionpb.MasterKeyKms value) {
if (kmsBuilder_ == null) {
if (backendCase_ == 3 &&
backend_ != org.tikv.kvproto.Encryptionpb.MasterKeyKms.getDefaultInstance()) {
backend_ = org.tikv.kvproto.Encryptionpb.MasterKeyKms.newBuilder((org.tikv.kvproto.Encryptionpb.MasterKeyKms) backend_)
.mergeFrom(value).buildPartial();
} else {
backend_ = value;
}
onChanged();
} else {
if (backendCase_ == 3) {
kmsBuilder_.mergeFrom(value);
}
kmsBuilder_.setMessage(value);
}
backendCase_ = 3;
return this;
}
/**
* .encryptionpb.MasterKeyKms kms = 3;
*/
public Builder clearKms() {
if (kmsBuilder_ == null) {
if (backendCase_ == 3) {
backendCase_ = 0;
backend_ = null;
onChanged();
}
} else {
if (backendCase_ == 3) {
backendCase_ = 0;
backend_ = null;
}
kmsBuilder_.clear();
}
return this;
}
/**
* .encryptionpb.MasterKeyKms kms = 3;
*/
public org.tikv.kvproto.Encryptionpb.MasterKeyKms.Builder getKmsBuilder() {
return getKmsFieldBuilder().getBuilder();
}
/**
* .encryptionpb.MasterKeyKms kms = 3;
*/
public org.tikv.kvproto.Encryptionpb.MasterKeyKmsOrBuilder getKmsOrBuilder() {
if ((backendCase_ == 3) && (kmsBuilder_ != null)) {
return kmsBuilder_.getMessageOrBuilder();
} else {
if (backendCase_ == 3) {
return (org.tikv.kvproto.Encryptionpb.MasterKeyKms) backend_;
}
return org.tikv.kvproto.Encryptionpb.MasterKeyKms.getDefaultInstance();
}
}
/**
* .encryptionpb.MasterKeyKms kms = 3;
*/
private com.google.protobuf.SingleFieldBuilderV3<
org.tikv.kvproto.Encryptionpb.MasterKeyKms, org.tikv.kvproto.Encryptionpb.MasterKeyKms.Builder, org.tikv.kvproto.Encryptionpb.MasterKeyKmsOrBuilder>
getKmsFieldBuilder() {
if (kmsBuilder_ == null) {
if (!(backendCase_ == 3)) {
backend_ = org.tikv.kvproto.Encryptionpb.MasterKeyKms.getDefaultInstance();
}
kmsBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
org.tikv.kvproto.Encryptionpb.MasterKeyKms, org.tikv.kvproto.Encryptionpb.MasterKeyKms.Builder, org.tikv.kvproto.Encryptionpb.MasterKeyKmsOrBuilder>(
(org.tikv.kvproto.Encryptionpb.MasterKeyKms) backend_,
getParentForChildren(),
isClean());
backend_ = null;
}
backendCase_ = 3;
onChanged();;
return kmsBuilder_;
}
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFieldsProto3(unknownFields);
}
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:encryptionpb.MasterKey)
}
// @@protoc_insertion_point(class_scope:encryptionpb.MasterKey)
private static final org.tikv.kvproto.Encryptionpb.MasterKey DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new org.tikv.kvproto.Encryptionpb.MasterKey();
}
public static org.tikv.kvproto.Encryptionpb.MasterKey getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
public MasterKey parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new MasterKey(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
public org.tikv.kvproto.Encryptionpb.MasterKey getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface MasterKeyPlaintextOrBuilder extends
// @@protoc_insertion_point(interface_extends:encryptionpb.MasterKeyPlaintext)
com.google.protobuf.MessageOrBuilder {
}
/**
*
* MasterKeyPlaintext indicates content is stored as plaintext.
*
*
* Protobuf type {@code encryptionpb.MasterKeyPlaintext}
*/
public static final class MasterKeyPlaintext extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:encryptionpb.MasterKeyPlaintext)
MasterKeyPlaintextOrBuilder {
private static final long serialVersionUID = 0L;
// Use MasterKeyPlaintext.newBuilder() to construct.
private MasterKeyPlaintext(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private MasterKeyPlaintext() {
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private MasterKeyPlaintext(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
default: {
if (!parseUnknownFieldProto3(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.tikv.kvproto.Encryptionpb.internal_static_encryptionpb_MasterKeyPlaintext_descriptor;
}
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.tikv.kvproto.Encryptionpb.internal_static_encryptionpb_MasterKeyPlaintext_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.tikv.kvproto.Encryptionpb.MasterKeyPlaintext.class, org.tikv.kvproto.Encryptionpb.MasterKeyPlaintext.Builder.class);
}
private byte memoizedIsInitialized = -1;
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
unknownFields.writeTo(output);
}
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof org.tikv.kvproto.Encryptionpb.MasterKeyPlaintext)) {
return super.equals(obj);
}
org.tikv.kvproto.Encryptionpb.MasterKeyPlaintext other = (org.tikv.kvproto.Encryptionpb.MasterKeyPlaintext) obj;
boolean result = true;
result = result && unknownFields.equals(other.unknownFields);
return result;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static org.tikv.kvproto.Encryptionpb.MasterKeyPlaintext parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.tikv.kvproto.Encryptionpb.MasterKeyPlaintext parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.tikv.kvproto.Encryptionpb.MasterKeyPlaintext parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.tikv.kvproto.Encryptionpb.MasterKeyPlaintext parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.tikv.kvproto.Encryptionpb.MasterKeyPlaintext parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.tikv.kvproto.Encryptionpb.MasterKeyPlaintext parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.tikv.kvproto.Encryptionpb.MasterKeyPlaintext parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static org.tikv.kvproto.Encryptionpb.MasterKeyPlaintext parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static org.tikv.kvproto.Encryptionpb.MasterKeyPlaintext parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static org.tikv.kvproto.Encryptionpb.MasterKeyPlaintext parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static org.tikv.kvproto.Encryptionpb.MasterKeyPlaintext parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static org.tikv.kvproto.Encryptionpb.MasterKeyPlaintext parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(org.tikv.kvproto.Encryptionpb.MasterKeyPlaintext prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
* MasterKeyPlaintext indicates content is stored as plaintext.
*
*
* Protobuf type {@code encryptionpb.MasterKeyPlaintext}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:encryptionpb.MasterKeyPlaintext)
org.tikv.kvproto.Encryptionpb.MasterKeyPlaintextOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.tikv.kvproto.Encryptionpb.internal_static_encryptionpb_MasterKeyPlaintext_descriptor;
}
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.tikv.kvproto.Encryptionpb.internal_static_encryptionpb_MasterKeyPlaintext_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.tikv.kvproto.Encryptionpb.MasterKeyPlaintext.class, org.tikv.kvproto.Encryptionpb.MasterKeyPlaintext.Builder.class);
}
// Construct using org.tikv.kvproto.Encryptionpb.MasterKeyPlaintext.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
public Builder clear() {
super.clear();
return this;
}
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return org.tikv.kvproto.Encryptionpb.internal_static_encryptionpb_MasterKeyPlaintext_descriptor;
}
public org.tikv.kvproto.Encryptionpb.MasterKeyPlaintext getDefaultInstanceForType() {
return org.tikv.kvproto.Encryptionpb.MasterKeyPlaintext.getDefaultInstance();
}
public org.tikv.kvproto.Encryptionpb.MasterKeyPlaintext build() {
org.tikv.kvproto.Encryptionpb.MasterKeyPlaintext result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
public org.tikv.kvproto.Encryptionpb.MasterKeyPlaintext buildPartial() {
org.tikv.kvproto.Encryptionpb.MasterKeyPlaintext result = new org.tikv.kvproto.Encryptionpb.MasterKeyPlaintext(this);
onBuilt();
return result;
}
public Builder clone() {
return (Builder) super.clone();
}
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.setField(field, value);
}
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return (Builder) super.clearField(field);
}
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return (Builder) super.clearOneof(oneof);
}
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return (Builder) super.setRepeatedField(field, index, value);
}
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.addRepeatedField(field, value);
}
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof org.tikv.kvproto.Encryptionpb.MasterKeyPlaintext) {
return mergeFrom((org.tikv.kvproto.Encryptionpb.MasterKeyPlaintext)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(org.tikv.kvproto.Encryptionpb.MasterKeyPlaintext other) {
if (other == org.tikv.kvproto.Encryptionpb.MasterKeyPlaintext.getDefaultInstance()) return this;
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
public final boolean isInitialized() {
return true;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
org.tikv.kvproto.Encryptionpb.MasterKeyPlaintext parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (org.tikv.kvproto.Encryptionpb.MasterKeyPlaintext) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFieldsProto3(unknownFields);
}
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:encryptionpb.MasterKeyPlaintext)
}
// @@protoc_insertion_point(class_scope:encryptionpb.MasterKeyPlaintext)
private static final org.tikv.kvproto.Encryptionpb.MasterKeyPlaintext DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new org.tikv.kvproto.Encryptionpb.MasterKeyPlaintext();
}
public static org.tikv.kvproto.Encryptionpb.MasterKeyPlaintext getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
public MasterKeyPlaintext parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new MasterKeyPlaintext(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
public org.tikv.kvproto.Encryptionpb.MasterKeyPlaintext getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface MasterKeyFileOrBuilder extends
// @@protoc_insertion_point(interface_extends:encryptionpb.MasterKeyFile)
com.google.protobuf.MessageOrBuilder {
/**
*
* Local file path.
*
*
* string path = 1;
*/
java.lang.String getPath();
/**
*
* Local file path.
*
*
* string path = 1;
*/
com.google.protobuf.ByteString
getPathBytes();
}
/**
*
* MasterKeyFile is a master key backed by a file containing encryption key in human-readable
* hex format.
*
*
* Protobuf type {@code encryptionpb.MasterKeyFile}
*/
public static final class MasterKeyFile extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:encryptionpb.MasterKeyFile)
MasterKeyFileOrBuilder {
private static final long serialVersionUID = 0L;
// Use MasterKeyFile.newBuilder() to construct.
private MasterKeyFile(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private MasterKeyFile() {
path_ = "";
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private MasterKeyFile(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
default: {
if (!parseUnknownFieldProto3(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
case 10: {
java.lang.String s = input.readStringRequireUtf8();
path_ = s;
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.tikv.kvproto.Encryptionpb.internal_static_encryptionpb_MasterKeyFile_descriptor;
}
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.tikv.kvproto.Encryptionpb.internal_static_encryptionpb_MasterKeyFile_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.tikv.kvproto.Encryptionpb.MasterKeyFile.class, org.tikv.kvproto.Encryptionpb.MasterKeyFile.Builder.class);
}
public static final int PATH_FIELD_NUMBER = 1;
private volatile java.lang.Object path_;
/**
*
* Local file path.
*
*
* string path = 1;
*/
public java.lang.String getPath() {
java.lang.Object ref = path_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
path_ = s;
return s;
}
}
/**
*
* Local file path.
*
*
* string path = 1;
*/
public com.google.protobuf.ByteString
getPathBytes() {
java.lang.Object ref = path_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
path_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
private byte memoizedIsInitialized = -1;
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (!getPathBytes().isEmpty()) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 1, path_);
}
unknownFields.writeTo(output);
}
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (!getPathBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(1, path_);
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof org.tikv.kvproto.Encryptionpb.MasterKeyFile)) {
return super.equals(obj);
}
org.tikv.kvproto.Encryptionpb.MasterKeyFile other = (org.tikv.kvproto.Encryptionpb.MasterKeyFile) obj;
boolean result = true;
result = result && getPath()
.equals(other.getPath());
result = result && unknownFields.equals(other.unknownFields);
return result;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
hash = (37 * hash) + PATH_FIELD_NUMBER;
hash = (53 * hash) + getPath().hashCode();
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static org.tikv.kvproto.Encryptionpb.MasterKeyFile parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.tikv.kvproto.Encryptionpb.MasterKeyFile parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.tikv.kvproto.Encryptionpb.MasterKeyFile parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.tikv.kvproto.Encryptionpb.MasterKeyFile parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.tikv.kvproto.Encryptionpb.MasterKeyFile parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.tikv.kvproto.Encryptionpb.MasterKeyFile parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.tikv.kvproto.Encryptionpb.MasterKeyFile parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static org.tikv.kvproto.Encryptionpb.MasterKeyFile parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static org.tikv.kvproto.Encryptionpb.MasterKeyFile parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static org.tikv.kvproto.Encryptionpb.MasterKeyFile parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static org.tikv.kvproto.Encryptionpb.MasterKeyFile parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static org.tikv.kvproto.Encryptionpb.MasterKeyFile parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(org.tikv.kvproto.Encryptionpb.MasterKeyFile prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
* MasterKeyFile is a master key backed by a file containing encryption key in human-readable
* hex format.
*
*
* Protobuf type {@code encryptionpb.MasterKeyFile}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:encryptionpb.MasterKeyFile)
org.tikv.kvproto.Encryptionpb.MasterKeyFileOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.tikv.kvproto.Encryptionpb.internal_static_encryptionpb_MasterKeyFile_descriptor;
}
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.tikv.kvproto.Encryptionpb.internal_static_encryptionpb_MasterKeyFile_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.tikv.kvproto.Encryptionpb.MasterKeyFile.class, org.tikv.kvproto.Encryptionpb.MasterKeyFile.Builder.class);
}
// Construct using org.tikv.kvproto.Encryptionpb.MasterKeyFile.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
public Builder clear() {
super.clear();
path_ = "";
return this;
}
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return org.tikv.kvproto.Encryptionpb.internal_static_encryptionpb_MasterKeyFile_descriptor;
}
public org.tikv.kvproto.Encryptionpb.MasterKeyFile getDefaultInstanceForType() {
return org.tikv.kvproto.Encryptionpb.MasterKeyFile.getDefaultInstance();
}
public org.tikv.kvproto.Encryptionpb.MasterKeyFile build() {
org.tikv.kvproto.Encryptionpb.MasterKeyFile result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
public org.tikv.kvproto.Encryptionpb.MasterKeyFile buildPartial() {
org.tikv.kvproto.Encryptionpb.MasterKeyFile result = new org.tikv.kvproto.Encryptionpb.MasterKeyFile(this);
result.path_ = path_;
onBuilt();
return result;
}
public Builder clone() {
return (Builder) super.clone();
}
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.setField(field, value);
}
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return (Builder) super.clearField(field);
}
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return (Builder) super.clearOneof(oneof);
}
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return (Builder) super.setRepeatedField(field, index, value);
}
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.addRepeatedField(field, value);
}
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof org.tikv.kvproto.Encryptionpb.MasterKeyFile) {
return mergeFrom((org.tikv.kvproto.Encryptionpb.MasterKeyFile)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(org.tikv.kvproto.Encryptionpb.MasterKeyFile other) {
if (other == org.tikv.kvproto.Encryptionpb.MasterKeyFile.getDefaultInstance()) return this;
if (!other.getPath().isEmpty()) {
path_ = other.path_;
onChanged();
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
public final boolean isInitialized() {
return true;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
org.tikv.kvproto.Encryptionpb.MasterKeyFile parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (org.tikv.kvproto.Encryptionpb.MasterKeyFile) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private java.lang.Object path_ = "";
/**
*
* Local file path.
*
*
* string path = 1;
*/
public java.lang.String getPath() {
java.lang.Object ref = path_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
path_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* Local file path.
*
*
* string path = 1;
*/
public com.google.protobuf.ByteString
getPathBytes() {
java.lang.Object ref = path_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
path_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* Local file path.
*
*
* string path = 1;
*/
public Builder setPath(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
path_ = value;
onChanged();
return this;
}
/**
*
* Local file path.
*
*
* string path = 1;
*/
public Builder clearPath() {
path_ = getDefaultInstance().getPath();
onChanged();
return this;
}
/**
*
* Local file path.
*
*
* string path = 1;
*/
public Builder setPathBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
path_ = value;
onChanged();
return this;
}
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFieldsProto3(unknownFields);
}
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:encryptionpb.MasterKeyFile)
}
// @@protoc_insertion_point(class_scope:encryptionpb.MasterKeyFile)
private static final org.tikv.kvproto.Encryptionpb.MasterKeyFile DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new org.tikv.kvproto.Encryptionpb.MasterKeyFile();
}
public static org.tikv.kvproto.Encryptionpb.MasterKeyFile getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
public MasterKeyFile parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new MasterKeyFile(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
public org.tikv.kvproto.Encryptionpb.MasterKeyFile getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface MasterKeyKmsOrBuilder extends
// @@protoc_insertion_point(interface_extends:encryptionpb.MasterKeyKms)
com.google.protobuf.MessageOrBuilder {
/**
*
* KMS vendor.
*
*
* string vendor = 1;
*/
java.lang.String getVendor();
/**
*
* KMS vendor.
*
*
* string vendor = 1;
*/
com.google.protobuf.ByteString
getVendorBytes();
/**
*
* KMS key id.
*
*
* string key_id = 2;
*/
java.lang.String getKeyId();
/**
*
* KMS key id.
*
*
* string key_id = 2;
*/
com.google.protobuf.ByteString
getKeyIdBytes();
/**
*
* KMS region.
*
*
* string region = 3;
*/
java.lang.String getRegion();
/**
*
* KMS region.
*
*
* string region = 3;
*/
com.google.protobuf.ByteString
getRegionBytes();
/**
*
* KMS endpoint. Normally not needed.
*
*
* string endpoint = 4;
*/
java.lang.String getEndpoint();
/**
*
* KMS endpoint. Normally not needed.
*
*
* string endpoint = 4;
*/
com.google.protobuf.ByteString
getEndpointBytes();
}
/**
*
* MasterKeyKms is a master key backed by KMS service that manages the encryption key,
* and provide API to encrypt and decrypt a data key, which is used to encrypt the content.
*
*
* Protobuf type {@code encryptionpb.MasterKeyKms}
*/
public static final class MasterKeyKms extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:encryptionpb.MasterKeyKms)
MasterKeyKmsOrBuilder {
private static final long serialVersionUID = 0L;
// Use MasterKeyKms.newBuilder() to construct.
private MasterKeyKms(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private MasterKeyKms() {
vendor_ = "";
keyId_ = "";
region_ = "";
endpoint_ = "";
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private MasterKeyKms(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
default: {
if (!parseUnknownFieldProto3(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
case 10: {
java.lang.String s = input.readStringRequireUtf8();
vendor_ = s;
break;
}
case 18: {
java.lang.String s = input.readStringRequireUtf8();
keyId_ = s;
break;
}
case 26: {
java.lang.String s = input.readStringRequireUtf8();
region_ = s;
break;
}
case 34: {
java.lang.String s = input.readStringRequireUtf8();
endpoint_ = s;
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.tikv.kvproto.Encryptionpb.internal_static_encryptionpb_MasterKeyKms_descriptor;
}
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.tikv.kvproto.Encryptionpb.internal_static_encryptionpb_MasterKeyKms_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.tikv.kvproto.Encryptionpb.MasterKeyKms.class, org.tikv.kvproto.Encryptionpb.MasterKeyKms.Builder.class);
}
public static final int VENDOR_FIELD_NUMBER = 1;
private volatile java.lang.Object vendor_;
/**
*
* KMS vendor.
*
*
* string vendor = 1;
*/
public java.lang.String getVendor() {
java.lang.Object ref = vendor_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
vendor_ = s;
return s;
}
}
/**
*
* KMS vendor.
*
*
* string vendor = 1;
*/
public com.google.protobuf.ByteString
getVendorBytes() {
java.lang.Object ref = vendor_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
vendor_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int KEY_ID_FIELD_NUMBER = 2;
private volatile java.lang.Object keyId_;
/**
*
* KMS key id.
*
*
* string key_id = 2;
*/
public java.lang.String getKeyId() {
java.lang.Object ref = keyId_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
keyId_ = s;
return s;
}
}
/**
*
* KMS key id.
*
*
* string key_id = 2;
*/
public com.google.protobuf.ByteString
getKeyIdBytes() {
java.lang.Object ref = keyId_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
keyId_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int REGION_FIELD_NUMBER = 3;
private volatile java.lang.Object region_;
/**
*
* KMS region.
*
*
* string region = 3;
*/
public java.lang.String getRegion() {
java.lang.Object ref = region_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
region_ = s;
return s;
}
}
/**
*
* KMS region.
*
*
* string region = 3;
*/
public com.google.protobuf.ByteString
getRegionBytes() {
java.lang.Object ref = region_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
region_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int ENDPOINT_FIELD_NUMBER = 4;
private volatile java.lang.Object endpoint_;
/**
*
* KMS endpoint. Normally not needed.
*
*
* string endpoint = 4;
*/
public java.lang.String getEndpoint() {
java.lang.Object ref = endpoint_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
endpoint_ = s;
return s;
}
}
/**
*
* KMS endpoint. Normally not needed.
*
*
* string endpoint = 4;
*/
public com.google.protobuf.ByteString
getEndpointBytes() {
java.lang.Object ref = endpoint_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
endpoint_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
private byte memoizedIsInitialized = -1;
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (!getVendorBytes().isEmpty()) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 1, vendor_);
}
if (!getKeyIdBytes().isEmpty()) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 2, keyId_);
}
if (!getRegionBytes().isEmpty()) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 3, region_);
}
if (!getEndpointBytes().isEmpty()) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 4, endpoint_);
}
unknownFields.writeTo(output);
}
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (!getVendorBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(1, vendor_);
}
if (!getKeyIdBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(2, keyId_);
}
if (!getRegionBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(3, region_);
}
if (!getEndpointBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(4, endpoint_);
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof org.tikv.kvproto.Encryptionpb.MasterKeyKms)) {
return super.equals(obj);
}
org.tikv.kvproto.Encryptionpb.MasterKeyKms other = (org.tikv.kvproto.Encryptionpb.MasterKeyKms) obj;
boolean result = true;
result = result && getVendor()
.equals(other.getVendor());
result = result && getKeyId()
.equals(other.getKeyId());
result = result && getRegion()
.equals(other.getRegion());
result = result && getEndpoint()
.equals(other.getEndpoint());
result = result && unknownFields.equals(other.unknownFields);
return result;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
hash = (37 * hash) + VENDOR_FIELD_NUMBER;
hash = (53 * hash) + getVendor().hashCode();
hash = (37 * hash) + KEY_ID_FIELD_NUMBER;
hash = (53 * hash) + getKeyId().hashCode();
hash = (37 * hash) + REGION_FIELD_NUMBER;
hash = (53 * hash) + getRegion().hashCode();
hash = (37 * hash) + ENDPOINT_FIELD_NUMBER;
hash = (53 * hash) + getEndpoint().hashCode();
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static org.tikv.kvproto.Encryptionpb.MasterKeyKms parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.tikv.kvproto.Encryptionpb.MasterKeyKms parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.tikv.kvproto.Encryptionpb.MasterKeyKms parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.tikv.kvproto.Encryptionpb.MasterKeyKms parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.tikv.kvproto.Encryptionpb.MasterKeyKms parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.tikv.kvproto.Encryptionpb.MasterKeyKms parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.tikv.kvproto.Encryptionpb.MasterKeyKms parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static org.tikv.kvproto.Encryptionpb.MasterKeyKms parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static org.tikv.kvproto.Encryptionpb.MasterKeyKms parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static org.tikv.kvproto.Encryptionpb.MasterKeyKms parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static org.tikv.kvproto.Encryptionpb.MasterKeyKms parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static org.tikv.kvproto.Encryptionpb.MasterKeyKms parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(org.tikv.kvproto.Encryptionpb.MasterKeyKms prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
* MasterKeyKms is a master key backed by KMS service that manages the encryption key,
* and provide API to encrypt and decrypt a data key, which is used to encrypt the content.
*
*
* Protobuf type {@code encryptionpb.MasterKeyKms}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:encryptionpb.MasterKeyKms)
org.tikv.kvproto.Encryptionpb.MasterKeyKmsOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.tikv.kvproto.Encryptionpb.internal_static_encryptionpb_MasterKeyKms_descriptor;
}
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.tikv.kvproto.Encryptionpb.internal_static_encryptionpb_MasterKeyKms_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.tikv.kvproto.Encryptionpb.MasterKeyKms.class, org.tikv.kvproto.Encryptionpb.MasterKeyKms.Builder.class);
}
// Construct using org.tikv.kvproto.Encryptionpb.MasterKeyKms.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
public Builder clear() {
super.clear();
vendor_ = "";
keyId_ = "";
region_ = "";
endpoint_ = "";
return this;
}
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return org.tikv.kvproto.Encryptionpb.internal_static_encryptionpb_MasterKeyKms_descriptor;
}
public org.tikv.kvproto.Encryptionpb.MasterKeyKms getDefaultInstanceForType() {
return org.tikv.kvproto.Encryptionpb.MasterKeyKms.getDefaultInstance();
}
public org.tikv.kvproto.Encryptionpb.MasterKeyKms build() {
org.tikv.kvproto.Encryptionpb.MasterKeyKms result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
public org.tikv.kvproto.Encryptionpb.MasterKeyKms buildPartial() {
org.tikv.kvproto.Encryptionpb.MasterKeyKms result = new org.tikv.kvproto.Encryptionpb.MasterKeyKms(this);
result.vendor_ = vendor_;
result.keyId_ = keyId_;
result.region_ = region_;
result.endpoint_ = endpoint_;
onBuilt();
return result;
}
public Builder clone() {
return (Builder) super.clone();
}
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.setField(field, value);
}
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return (Builder) super.clearField(field);
}
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return (Builder) super.clearOneof(oneof);
}
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return (Builder) super.setRepeatedField(field, index, value);
}
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.addRepeatedField(field, value);
}
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof org.tikv.kvproto.Encryptionpb.MasterKeyKms) {
return mergeFrom((org.tikv.kvproto.Encryptionpb.MasterKeyKms)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(org.tikv.kvproto.Encryptionpb.MasterKeyKms other) {
if (other == org.tikv.kvproto.Encryptionpb.MasterKeyKms.getDefaultInstance()) return this;
if (!other.getVendor().isEmpty()) {
vendor_ = other.vendor_;
onChanged();
}
if (!other.getKeyId().isEmpty()) {
keyId_ = other.keyId_;
onChanged();
}
if (!other.getRegion().isEmpty()) {
region_ = other.region_;
onChanged();
}
if (!other.getEndpoint().isEmpty()) {
endpoint_ = other.endpoint_;
onChanged();
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
public final boolean isInitialized() {
return true;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
org.tikv.kvproto.Encryptionpb.MasterKeyKms parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (org.tikv.kvproto.Encryptionpb.MasterKeyKms) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private java.lang.Object vendor_ = "";
/**
*
* KMS vendor.
*
*
* string vendor = 1;
*/
public java.lang.String getVendor() {
java.lang.Object ref = vendor_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
vendor_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* KMS vendor.
*
*
* string vendor = 1;
*/
public com.google.protobuf.ByteString
getVendorBytes() {
java.lang.Object ref = vendor_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
vendor_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* KMS vendor.
*
*
* string vendor = 1;
*/
public Builder setVendor(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
vendor_ = value;
onChanged();
return this;
}
/**
*
* KMS vendor.
*
*
* string vendor = 1;
*/
public Builder clearVendor() {
vendor_ = getDefaultInstance().getVendor();
onChanged();
return this;
}
/**
*
* KMS vendor.
*
*
* string vendor = 1;
*/
public Builder setVendorBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
vendor_ = value;
onChanged();
return this;
}
private java.lang.Object keyId_ = "";
/**
*
* KMS key id.
*
*
* string key_id = 2;
*/
public java.lang.String getKeyId() {
java.lang.Object ref = keyId_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
keyId_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* KMS key id.
*
*
* string key_id = 2;
*/
public com.google.protobuf.ByteString
getKeyIdBytes() {
java.lang.Object ref = keyId_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
keyId_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* KMS key id.
*
*
* string key_id = 2;
*/
public Builder setKeyId(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
keyId_ = value;
onChanged();
return this;
}
/**
*
* KMS key id.
*
*
* string key_id = 2;
*/
public Builder clearKeyId() {
keyId_ = getDefaultInstance().getKeyId();
onChanged();
return this;
}
/**
*
* KMS key id.
*
*
* string key_id = 2;
*/
public Builder setKeyIdBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
keyId_ = value;
onChanged();
return this;
}
private java.lang.Object region_ = "";
/**
*
* KMS region.
*
*
* string region = 3;
*/
public java.lang.String getRegion() {
java.lang.Object ref = region_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
region_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* KMS region.
*
*
* string region = 3;
*/
public com.google.protobuf.ByteString
getRegionBytes() {
java.lang.Object ref = region_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
region_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* KMS region.
*
*
* string region = 3;
*/
public Builder setRegion(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
region_ = value;
onChanged();
return this;
}
/**
*
* KMS region.
*
*
* string region = 3;
*/
public Builder clearRegion() {
region_ = getDefaultInstance().getRegion();
onChanged();
return this;
}
/**
*
* KMS region.
*
*
* string region = 3;
*/
public Builder setRegionBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
region_ = value;
onChanged();
return this;
}
private java.lang.Object endpoint_ = "";
/**
*
* KMS endpoint. Normally not needed.
*
*
* string endpoint = 4;
*/
public java.lang.String getEndpoint() {
java.lang.Object ref = endpoint_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
endpoint_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* KMS endpoint. Normally not needed.
*
*
* string endpoint = 4;
*/
public com.google.protobuf.ByteString
getEndpointBytes() {
java.lang.Object ref = endpoint_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
endpoint_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* KMS endpoint. Normally not needed.
*
*
* string endpoint = 4;
*/
public Builder setEndpoint(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
endpoint_ = value;
onChanged();
return this;
}
/**
*
* KMS endpoint. Normally not needed.
*
*
* string endpoint = 4;
*/
public Builder clearEndpoint() {
endpoint_ = getDefaultInstance().getEndpoint();
onChanged();
return this;
}
/**
*
* KMS endpoint. Normally not needed.
*
*
* string endpoint = 4;
*/
public Builder setEndpointBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
endpoint_ = value;
onChanged();
return this;
}
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFieldsProto3(unknownFields);
}
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:encryptionpb.MasterKeyKms)
}
// @@protoc_insertion_point(class_scope:encryptionpb.MasterKeyKms)
private static final org.tikv.kvproto.Encryptionpb.MasterKeyKms DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new org.tikv.kvproto.Encryptionpb.MasterKeyKms();
}
public static org.tikv.kvproto.Encryptionpb.MasterKeyKms getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
public MasterKeyKms parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new MasterKeyKms(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
public org.tikv.kvproto.Encryptionpb.MasterKeyKms getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface EncryptedContentOrBuilder extends
// @@protoc_insertion_point(interface_extends:encryptionpb.EncryptedContent)
com.google.protobuf.MessageOrBuilder {
/**
*
* Metadata of the encrypted content.
* Eg. IV, method and KMS key ID
* It is preferred to define new fields for extra metadata than using this metadata map.
*
*
* map<string, bytes> metadata = 1;
*/
int getMetadataCount();
/**
*
* Metadata of the encrypted content.
* Eg. IV, method and KMS key ID
* It is preferred to define new fields for extra metadata than using this metadata map.
*
*
* map<string, bytes> metadata = 1;
*/
boolean containsMetadata(
java.lang.String key);
/**
* Use {@link #getMetadataMap()} instead.
*/
@java.lang.Deprecated
java.util.Map
getMetadata();
/**
*
* Metadata of the encrypted content.
* Eg. IV, method and KMS key ID
* It is preferred to define new fields for extra metadata than using this metadata map.
*
*
* map<string, bytes> metadata = 1;
*/
java.util.Map
getMetadataMap();
/**
*
* Metadata of the encrypted content.
* Eg. IV, method and KMS key ID
* It is preferred to define new fields for extra metadata than using this metadata map.
*
*
* map<string, bytes> metadata = 1;
*/
com.google.protobuf.ByteString getMetadataOrDefault(
java.lang.String key,
com.google.protobuf.ByteString defaultValue);
/**
*
* Metadata of the encrypted content.
* Eg. IV, method and KMS key ID
* It is preferred to define new fields for extra metadata than using this metadata map.
*
*
* map<string, bytes> metadata = 1;
*/
com.google.protobuf.ByteString getMetadataOrThrow(
java.lang.String key);
/**
*
* Encrypted content.
*
*
* bytes content = 2;
*/
com.google.protobuf.ByteString getContent();
/**
*
* Master key used to encrypt the content.
*
*
* .encryptionpb.MasterKey master_key = 3;
*/
boolean hasMasterKey();
/**
*
* Master key used to encrypt the content.
*
*
* .encryptionpb.MasterKey master_key = 3;
*/
org.tikv.kvproto.Encryptionpb.MasterKey getMasterKey();
/**
*
* Master key used to encrypt the content.
*
*
* .encryptionpb.MasterKey master_key = 3;
*/
org.tikv.kvproto.Encryptionpb.MasterKeyOrBuilder getMasterKeyOrBuilder();
/**
*
* Initilization vector (IV) used.
*
*
* bytes iv = 4;
*/
com.google.protobuf.ByteString getIv();
/**
*
* Encrypted data key generated by KMS and used to actually encrypt data.
* Valid only when KMS is used.
*
*
* bytes ciphertext_key = 5;
*/
com.google.protobuf.ByteString getCiphertextKey();
}
/**
* Protobuf type {@code encryptionpb.EncryptedContent}
*/
public static final class EncryptedContent extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:encryptionpb.EncryptedContent)
EncryptedContentOrBuilder {
private static final long serialVersionUID = 0L;
// Use EncryptedContent.newBuilder() to construct.
private EncryptedContent(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private EncryptedContent() {
content_ = com.google.protobuf.ByteString.EMPTY;
iv_ = com.google.protobuf.ByteString.EMPTY;
ciphertextKey_ = com.google.protobuf.ByteString.EMPTY;
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private EncryptedContent(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
default: {
if (!parseUnknownFieldProto3(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
case 10: {
if (!((mutable_bitField0_ & 0x00000001) == 0x00000001)) {
metadata_ = com.google.protobuf.MapField.newMapField(
MetadataDefaultEntryHolder.defaultEntry);
mutable_bitField0_ |= 0x00000001;
}
com.google.protobuf.MapEntry
metadata__ = input.readMessage(
MetadataDefaultEntryHolder.defaultEntry.getParserForType(), extensionRegistry);
metadata_.getMutableMap().put(
metadata__.getKey(), metadata__.getValue());
break;
}
case 18: {
content_ = input.readBytes();
break;
}
case 26: {
org.tikv.kvproto.Encryptionpb.MasterKey.Builder subBuilder = null;
if (masterKey_ != null) {
subBuilder = masterKey_.toBuilder();
}
masterKey_ = input.readMessage(org.tikv.kvproto.Encryptionpb.MasterKey.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(masterKey_);
masterKey_ = subBuilder.buildPartial();
}
break;
}
case 34: {
iv_ = input.readBytes();
break;
}
case 42: {
ciphertextKey_ = input.readBytes();
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.tikv.kvproto.Encryptionpb.internal_static_encryptionpb_EncryptedContent_descriptor;
}
@SuppressWarnings({"rawtypes"})
protected com.google.protobuf.MapField internalGetMapField(
int number) {
switch (number) {
case 1:
return internalGetMetadata();
default:
throw new RuntimeException(
"Invalid map field number: " + number);
}
}
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.tikv.kvproto.Encryptionpb.internal_static_encryptionpb_EncryptedContent_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.tikv.kvproto.Encryptionpb.EncryptedContent.class, org.tikv.kvproto.Encryptionpb.EncryptedContent.Builder.class);
}
private int bitField0_;
public static final int METADATA_FIELD_NUMBER = 1;
private static final class MetadataDefaultEntryHolder {
static final com.google.protobuf.MapEntry<
java.lang.String, com.google.protobuf.ByteString> defaultEntry =
com.google.protobuf.MapEntry
.newDefaultInstance(
org.tikv.kvproto.Encryptionpb.internal_static_encryptionpb_EncryptedContent_MetadataEntry_descriptor,
com.google.protobuf.WireFormat.FieldType.STRING,
"",
com.google.protobuf.WireFormat.FieldType.BYTES,
com.google.protobuf.ByteString.EMPTY);
}
private com.google.protobuf.MapField<
java.lang.String, com.google.protobuf.ByteString> metadata_;
private com.google.protobuf.MapField
internalGetMetadata() {
if (metadata_ == null) {
return com.google.protobuf.MapField.emptyMapField(
MetadataDefaultEntryHolder.defaultEntry);
}
return metadata_;
}
public int getMetadataCount() {
return internalGetMetadata().getMap().size();
}
/**
*
* Metadata of the encrypted content.
* Eg. IV, method and KMS key ID
* It is preferred to define new fields for extra metadata than using this metadata map.
*
*
* map<string, bytes> metadata = 1;
*/
public boolean containsMetadata(
java.lang.String key) {
if (key == null) { throw new java.lang.NullPointerException(); }
return internalGetMetadata().getMap().containsKey(key);
}
/**
* Use {@link #getMetadataMap()} instead.
*/
@java.lang.Deprecated
public java.util.Map getMetadata() {
return getMetadataMap();
}
/**
*
* Metadata of the encrypted content.
* Eg. IV, method and KMS key ID
* It is preferred to define new fields for extra metadata than using this metadata map.
*
*
* map<string, bytes> metadata = 1;
*/
public java.util.Map getMetadataMap() {
return internalGetMetadata().getMap();
}
/**
*
* Metadata of the encrypted content.
* Eg. IV, method and KMS key ID
* It is preferred to define new fields for extra metadata than using this metadata map.
*
*
* map<string, bytes> metadata = 1;
*/
public com.google.protobuf.ByteString getMetadataOrDefault(
java.lang.String key,
com.google.protobuf.ByteString defaultValue) {
if (key == null) { throw new java.lang.NullPointerException(); }
java.util.Map map =
internalGetMetadata().getMap();
return map.containsKey(key) ? map.get(key) : defaultValue;
}
/**
*
* Metadata of the encrypted content.
* Eg. IV, method and KMS key ID
* It is preferred to define new fields for extra metadata than using this metadata map.
*
*
* map<string, bytes> metadata = 1;
*/
public com.google.protobuf.ByteString getMetadataOrThrow(
java.lang.String key) {
if (key == null) { throw new java.lang.NullPointerException(); }
java.util.Map map =
internalGetMetadata().getMap();
if (!map.containsKey(key)) {
throw new java.lang.IllegalArgumentException();
}
return map.get(key);
}
public static final int CONTENT_FIELD_NUMBER = 2;
private com.google.protobuf.ByteString content_;
/**
*
* Encrypted content.
*
*
* bytes content = 2;
*/
public com.google.protobuf.ByteString getContent() {
return content_;
}
public static final int MASTER_KEY_FIELD_NUMBER = 3;
private org.tikv.kvproto.Encryptionpb.MasterKey masterKey_;
/**
*
* Master key used to encrypt the content.
*
*
* .encryptionpb.MasterKey master_key = 3;
*/
public boolean hasMasterKey() {
return masterKey_ != null;
}
/**
*
* Master key used to encrypt the content.
*
*
* .encryptionpb.MasterKey master_key = 3;
*/
public org.tikv.kvproto.Encryptionpb.MasterKey getMasterKey() {
return masterKey_ == null ? org.tikv.kvproto.Encryptionpb.MasterKey.getDefaultInstance() : masterKey_;
}
/**
*
* Master key used to encrypt the content.
*
*
* .encryptionpb.MasterKey master_key = 3;
*/
public org.tikv.kvproto.Encryptionpb.MasterKeyOrBuilder getMasterKeyOrBuilder() {
return getMasterKey();
}
public static final int IV_FIELD_NUMBER = 4;
private com.google.protobuf.ByteString iv_;
/**
*
* Initilization vector (IV) used.
*
*
* bytes iv = 4;
*/
public com.google.protobuf.ByteString getIv() {
return iv_;
}
public static final int CIPHERTEXT_KEY_FIELD_NUMBER = 5;
private com.google.protobuf.ByteString ciphertextKey_;
/**
*
* Encrypted data key generated by KMS and used to actually encrypt data.
* Valid only when KMS is used.
*
*
* bytes ciphertext_key = 5;
*/
public com.google.protobuf.ByteString getCiphertextKey() {
return ciphertextKey_;
}
private byte memoizedIsInitialized = -1;
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
com.google.protobuf.GeneratedMessageV3
.serializeStringMapTo(
output,
internalGetMetadata(),
MetadataDefaultEntryHolder.defaultEntry,
1);
if (!content_.isEmpty()) {
output.writeBytes(2, content_);
}
if (masterKey_ != null) {
output.writeMessage(3, getMasterKey());
}
if (!iv_.isEmpty()) {
output.writeBytes(4, iv_);
}
if (!ciphertextKey_.isEmpty()) {
output.writeBytes(5, ciphertextKey_);
}
unknownFields.writeTo(output);
}
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
for (java.util.Map.Entry entry
: internalGetMetadata().getMap().entrySet()) {
com.google.protobuf.MapEntry
metadata__ = MetadataDefaultEntryHolder.defaultEntry.newBuilderForType()
.setKey(entry.getKey())
.setValue(entry.getValue())
.build();
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(1, metadata__);
}
if (!content_.isEmpty()) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(2, content_);
}
if (masterKey_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(3, getMasterKey());
}
if (!iv_.isEmpty()) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(4, iv_);
}
if (!ciphertextKey_.isEmpty()) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(5, ciphertextKey_);
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof org.tikv.kvproto.Encryptionpb.EncryptedContent)) {
return super.equals(obj);
}
org.tikv.kvproto.Encryptionpb.EncryptedContent other = (org.tikv.kvproto.Encryptionpb.EncryptedContent) obj;
boolean result = true;
result = result && internalGetMetadata().equals(
other.internalGetMetadata());
result = result && getContent()
.equals(other.getContent());
result = result && (hasMasterKey() == other.hasMasterKey());
if (hasMasterKey()) {
result = result && getMasterKey()
.equals(other.getMasterKey());
}
result = result && getIv()
.equals(other.getIv());
result = result && getCiphertextKey()
.equals(other.getCiphertextKey());
result = result && unknownFields.equals(other.unknownFields);
return result;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (!internalGetMetadata().getMap().isEmpty()) {
hash = (37 * hash) + METADATA_FIELD_NUMBER;
hash = (53 * hash) + internalGetMetadata().hashCode();
}
hash = (37 * hash) + CONTENT_FIELD_NUMBER;
hash = (53 * hash) + getContent().hashCode();
if (hasMasterKey()) {
hash = (37 * hash) + MASTER_KEY_FIELD_NUMBER;
hash = (53 * hash) + getMasterKey().hashCode();
}
hash = (37 * hash) + IV_FIELD_NUMBER;
hash = (53 * hash) + getIv().hashCode();
hash = (37 * hash) + CIPHERTEXT_KEY_FIELD_NUMBER;
hash = (53 * hash) + getCiphertextKey().hashCode();
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static org.tikv.kvproto.Encryptionpb.EncryptedContent parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.tikv.kvproto.Encryptionpb.EncryptedContent parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.tikv.kvproto.Encryptionpb.EncryptedContent parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.tikv.kvproto.Encryptionpb.EncryptedContent parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.tikv.kvproto.Encryptionpb.EncryptedContent parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.tikv.kvproto.Encryptionpb.EncryptedContent parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.tikv.kvproto.Encryptionpb.EncryptedContent parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static org.tikv.kvproto.Encryptionpb.EncryptedContent parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static org.tikv.kvproto.Encryptionpb.EncryptedContent parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static org.tikv.kvproto.Encryptionpb.EncryptedContent parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static org.tikv.kvproto.Encryptionpb.EncryptedContent parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static org.tikv.kvproto.Encryptionpb.EncryptedContent parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(org.tikv.kvproto.Encryptionpb.EncryptedContent prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code encryptionpb.EncryptedContent}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:encryptionpb.EncryptedContent)
org.tikv.kvproto.Encryptionpb.EncryptedContentOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.tikv.kvproto.Encryptionpb.internal_static_encryptionpb_EncryptedContent_descriptor;
}
@SuppressWarnings({"rawtypes"})
protected com.google.protobuf.MapField internalGetMapField(
int number) {
switch (number) {
case 1:
return internalGetMetadata();
default:
throw new RuntimeException(
"Invalid map field number: " + number);
}
}
@SuppressWarnings({"rawtypes"})
protected com.google.protobuf.MapField internalGetMutableMapField(
int number) {
switch (number) {
case 1:
return internalGetMutableMetadata();
default:
throw new RuntimeException(
"Invalid map field number: " + number);
}
}
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.tikv.kvproto.Encryptionpb.internal_static_encryptionpb_EncryptedContent_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.tikv.kvproto.Encryptionpb.EncryptedContent.class, org.tikv.kvproto.Encryptionpb.EncryptedContent.Builder.class);
}
// Construct using org.tikv.kvproto.Encryptionpb.EncryptedContent.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
public Builder clear() {
super.clear();
internalGetMutableMetadata().clear();
content_ = com.google.protobuf.ByteString.EMPTY;
if (masterKeyBuilder_ == null) {
masterKey_ = null;
} else {
masterKey_ = null;
masterKeyBuilder_ = null;
}
iv_ = com.google.protobuf.ByteString.EMPTY;
ciphertextKey_ = com.google.protobuf.ByteString.EMPTY;
return this;
}
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return org.tikv.kvproto.Encryptionpb.internal_static_encryptionpb_EncryptedContent_descriptor;
}
public org.tikv.kvproto.Encryptionpb.EncryptedContent getDefaultInstanceForType() {
return org.tikv.kvproto.Encryptionpb.EncryptedContent.getDefaultInstance();
}
public org.tikv.kvproto.Encryptionpb.EncryptedContent build() {
org.tikv.kvproto.Encryptionpb.EncryptedContent result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
public org.tikv.kvproto.Encryptionpb.EncryptedContent buildPartial() {
org.tikv.kvproto.Encryptionpb.EncryptedContent result = new org.tikv.kvproto.Encryptionpb.EncryptedContent(this);
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
result.metadata_ = internalGetMetadata();
result.metadata_.makeImmutable();
result.content_ = content_;
if (masterKeyBuilder_ == null) {
result.masterKey_ = masterKey_;
} else {
result.masterKey_ = masterKeyBuilder_.build();
}
result.iv_ = iv_;
result.ciphertextKey_ = ciphertextKey_;
result.bitField0_ = to_bitField0_;
onBuilt();
return result;
}
public Builder clone() {
return (Builder) super.clone();
}
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.setField(field, value);
}
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return (Builder) super.clearField(field);
}
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return (Builder) super.clearOneof(oneof);
}
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return (Builder) super.setRepeatedField(field, index, value);
}
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.addRepeatedField(field, value);
}
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof org.tikv.kvproto.Encryptionpb.EncryptedContent) {
return mergeFrom((org.tikv.kvproto.Encryptionpb.EncryptedContent)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(org.tikv.kvproto.Encryptionpb.EncryptedContent other) {
if (other == org.tikv.kvproto.Encryptionpb.EncryptedContent.getDefaultInstance()) return this;
internalGetMutableMetadata().mergeFrom(
other.internalGetMetadata());
if (other.getContent() != com.google.protobuf.ByteString.EMPTY) {
setContent(other.getContent());
}
if (other.hasMasterKey()) {
mergeMasterKey(other.getMasterKey());
}
if (other.getIv() != com.google.protobuf.ByteString.EMPTY) {
setIv(other.getIv());
}
if (other.getCiphertextKey() != com.google.protobuf.ByteString.EMPTY) {
setCiphertextKey(other.getCiphertextKey());
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
public final boolean isInitialized() {
return true;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
org.tikv.kvproto.Encryptionpb.EncryptedContent parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (org.tikv.kvproto.Encryptionpb.EncryptedContent) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
private com.google.protobuf.MapField<
java.lang.String, com.google.protobuf.ByteString> metadata_;
private com.google.protobuf.MapField
internalGetMetadata() {
if (metadata_ == null) {
return com.google.protobuf.MapField.emptyMapField(
MetadataDefaultEntryHolder.defaultEntry);
}
return metadata_;
}
private com.google.protobuf.MapField
internalGetMutableMetadata() {
onChanged();;
if (metadata_ == null) {
metadata_ = com.google.protobuf.MapField.newMapField(
MetadataDefaultEntryHolder.defaultEntry);
}
if (!metadata_.isMutable()) {
metadata_ = metadata_.copy();
}
return metadata_;
}
public int getMetadataCount() {
return internalGetMetadata().getMap().size();
}
/**
*
* Metadata of the encrypted content.
* Eg. IV, method and KMS key ID
* It is preferred to define new fields for extra metadata than using this metadata map.
*
*
* map<string, bytes> metadata = 1;
*/
public boolean containsMetadata(
java.lang.String key) {
if (key == null) { throw new java.lang.NullPointerException(); }
return internalGetMetadata().getMap().containsKey(key);
}
/**
* Use {@link #getMetadataMap()} instead.
*/
@java.lang.Deprecated
public java.util.Map getMetadata() {
return getMetadataMap();
}
/**
*
* Metadata of the encrypted content.
* Eg. IV, method and KMS key ID
* It is preferred to define new fields for extra metadata than using this metadata map.
*
*
* map<string, bytes> metadata = 1;
*/
public java.util.Map getMetadataMap() {
return internalGetMetadata().getMap();
}
/**
*
* Metadata of the encrypted content.
* Eg. IV, method and KMS key ID
* It is preferred to define new fields for extra metadata than using this metadata map.
*
*
* map<string, bytes> metadata = 1;
*/
public com.google.protobuf.ByteString getMetadataOrDefault(
java.lang.String key,
com.google.protobuf.ByteString defaultValue) {
if (key == null) { throw new java.lang.NullPointerException(); }
java.util.Map map =
internalGetMetadata().getMap();
return map.containsKey(key) ? map.get(key) : defaultValue;
}
/**
*
* Metadata of the encrypted content.
* Eg. IV, method and KMS key ID
* It is preferred to define new fields for extra metadata than using this metadata map.
*
*
* map<string, bytes> metadata = 1;
*/
public com.google.protobuf.ByteString getMetadataOrThrow(
java.lang.String key) {
if (key == null) { throw new java.lang.NullPointerException(); }
java.util.Map map =
internalGetMetadata().getMap();
if (!map.containsKey(key)) {
throw new java.lang.IllegalArgumentException();
}
return map.get(key);
}
public Builder clearMetadata() {
internalGetMutableMetadata().getMutableMap()
.clear();
return this;
}
/**
*
* Metadata of the encrypted content.
* Eg. IV, method and KMS key ID
* It is preferred to define new fields for extra metadata than using this metadata map.
*
*
* map<string, bytes> metadata = 1;
*/
public Builder removeMetadata(
java.lang.String key) {
if (key == null) { throw new java.lang.NullPointerException(); }
internalGetMutableMetadata().getMutableMap()
.remove(key);
return this;
}
/**
* Use alternate mutation accessors instead.
*/
@java.lang.Deprecated
public java.util.Map
getMutableMetadata() {
return internalGetMutableMetadata().getMutableMap();
}
/**
*
* Metadata of the encrypted content.
* Eg. IV, method and KMS key ID
* It is preferred to define new fields for extra metadata than using this metadata map.
*
*
* map<string, bytes> metadata = 1;
*/
public Builder putMetadata(
java.lang.String key,
com.google.protobuf.ByteString value) {
if (key == null) { throw new java.lang.NullPointerException(); }
if (value == null) { throw new java.lang.NullPointerException(); }
internalGetMutableMetadata().getMutableMap()
.put(key, value);
return this;
}
/**
*
* Metadata of the encrypted content.
* Eg. IV, method and KMS key ID
* It is preferred to define new fields for extra metadata than using this metadata map.
*
*
* map<string, bytes> metadata = 1;
*/
public Builder putAllMetadata(
java.util.Map values) {
internalGetMutableMetadata().getMutableMap()
.putAll(values);
return this;
}
private com.google.protobuf.ByteString content_ = com.google.protobuf.ByteString.EMPTY;
/**
*
* Encrypted content.
*
*
* bytes content = 2;
*/
public com.google.protobuf.ByteString getContent() {
return content_;
}
/**
*
* Encrypted content.
*
*
* bytes content = 2;
*/
public Builder setContent(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
content_ = value;
onChanged();
return this;
}
/**
*
* Encrypted content.
*
*
* bytes content = 2;
*/
public Builder clearContent() {
content_ = getDefaultInstance().getContent();
onChanged();
return this;
}
private org.tikv.kvproto.Encryptionpb.MasterKey masterKey_ = null;
private com.google.protobuf.SingleFieldBuilderV3<
org.tikv.kvproto.Encryptionpb.MasterKey, org.tikv.kvproto.Encryptionpb.MasterKey.Builder, org.tikv.kvproto.Encryptionpb.MasterKeyOrBuilder> masterKeyBuilder_;
/**
*
* Master key used to encrypt the content.
*
*
* .encryptionpb.MasterKey master_key = 3;
*/
public boolean hasMasterKey() {
return masterKeyBuilder_ != null || masterKey_ != null;
}
/**
*
* Master key used to encrypt the content.
*
*
* .encryptionpb.MasterKey master_key = 3;
*/
public org.tikv.kvproto.Encryptionpb.MasterKey getMasterKey() {
if (masterKeyBuilder_ == null) {
return masterKey_ == null ? org.tikv.kvproto.Encryptionpb.MasterKey.getDefaultInstance() : masterKey_;
} else {
return masterKeyBuilder_.getMessage();
}
}
/**
*
* Master key used to encrypt the content.
*
*
* .encryptionpb.MasterKey master_key = 3;
*/
public Builder setMasterKey(org.tikv.kvproto.Encryptionpb.MasterKey value) {
if (masterKeyBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
masterKey_ = value;
onChanged();
} else {
masterKeyBuilder_.setMessage(value);
}
return this;
}
/**
*
* Master key used to encrypt the content.
*
*
* .encryptionpb.MasterKey master_key = 3;
*/
public Builder setMasterKey(
org.tikv.kvproto.Encryptionpb.MasterKey.Builder builderForValue) {
if (masterKeyBuilder_ == null) {
masterKey_ = builderForValue.build();
onChanged();
} else {
masterKeyBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
*
* Master key used to encrypt the content.
*
*
* .encryptionpb.MasterKey master_key = 3;
*/
public Builder mergeMasterKey(org.tikv.kvproto.Encryptionpb.MasterKey value) {
if (masterKeyBuilder_ == null) {
if (masterKey_ != null) {
masterKey_ =
org.tikv.kvproto.Encryptionpb.MasterKey.newBuilder(masterKey_).mergeFrom(value).buildPartial();
} else {
masterKey_ = value;
}
onChanged();
} else {
masterKeyBuilder_.mergeFrom(value);
}
return this;
}
/**
*
* Master key used to encrypt the content.
*
*
* .encryptionpb.MasterKey master_key = 3;
*/
public Builder clearMasterKey() {
if (masterKeyBuilder_ == null) {
masterKey_ = null;
onChanged();
} else {
masterKey_ = null;
masterKeyBuilder_ = null;
}
return this;
}
/**
*
* Master key used to encrypt the content.
*
*
* .encryptionpb.MasterKey master_key = 3;
*/
public org.tikv.kvproto.Encryptionpb.MasterKey.Builder getMasterKeyBuilder() {
onChanged();
return getMasterKeyFieldBuilder().getBuilder();
}
/**
*
* Master key used to encrypt the content.
*
*
* .encryptionpb.MasterKey master_key = 3;
*/
public org.tikv.kvproto.Encryptionpb.MasterKeyOrBuilder getMasterKeyOrBuilder() {
if (masterKeyBuilder_ != null) {
return masterKeyBuilder_.getMessageOrBuilder();
} else {
return masterKey_ == null ?
org.tikv.kvproto.Encryptionpb.MasterKey.getDefaultInstance() : masterKey_;
}
}
/**
*
* Master key used to encrypt the content.
*
*
* .encryptionpb.MasterKey master_key = 3;
*/
private com.google.protobuf.SingleFieldBuilderV3<
org.tikv.kvproto.Encryptionpb.MasterKey, org.tikv.kvproto.Encryptionpb.MasterKey.Builder, org.tikv.kvproto.Encryptionpb.MasterKeyOrBuilder>
getMasterKeyFieldBuilder() {
if (masterKeyBuilder_ == null) {
masterKeyBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
org.tikv.kvproto.Encryptionpb.MasterKey, org.tikv.kvproto.Encryptionpb.MasterKey.Builder, org.tikv.kvproto.Encryptionpb.MasterKeyOrBuilder>(
getMasterKey(),
getParentForChildren(),
isClean());
masterKey_ = null;
}
return masterKeyBuilder_;
}
private com.google.protobuf.ByteString iv_ = com.google.protobuf.ByteString.EMPTY;
/**
*
* Initilization vector (IV) used.
*
*
* bytes iv = 4;
*/
public com.google.protobuf.ByteString getIv() {
return iv_;
}
/**
*
* Initilization vector (IV) used.
*
*
* bytes iv = 4;
*/
public Builder setIv(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
iv_ = value;
onChanged();
return this;
}
/**
*
* Initilization vector (IV) used.
*
*
* bytes iv = 4;
*/
public Builder clearIv() {
iv_ = getDefaultInstance().getIv();
onChanged();
return this;
}
private com.google.protobuf.ByteString ciphertextKey_ = com.google.protobuf.ByteString.EMPTY;
/**
*
* Encrypted data key generated by KMS and used to actually encrypt data.
* Valid only when KMS is used.
*
*
* bytes ciphertext_key = 5;
*/
public com.google.protobuf.ByteString getCiphertextKey() {
return ciphertextKey_;
}
/**
*
* Encrypted data key generated by KMS and used to actually encrypt data.
* Valid only when KMS is used.
*
*
* bytes ciphertext_key = 5;
*/
public Builder setCiphertextKey(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
ciphertextKey_ = value;
onChanged();
return this;
}
/**
*
* Encrypted data key generated by KMS and used to actually encrypt data.
* Valid only when KMS is used.
*
*
* bytes ciphertext_key = 5;
*/
public Builder clearCiphertextKey() {
ciphertextKey_ = getDefaultInstance().getCiphertextKey();
onChanged();
return this;
}
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFieldsProto3(unknownFields);
}
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:encryptionpb.EncryptedContent)
}
// @@protoc_insertion_point(class_scope:encryptionpb.EncryptedContent)
private static final org.tikv.kvproto.Encryptionpb.EncryptedContent DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new org.tikv.kvproto.Encryptionpb.EncryptedContent();
}
public static org.tikv.kvproto.Encryptionpb.EncryptedContent getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
public EncryptedContent parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new EncryptedContent(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
public org.tikv.kvproto.Encryptionpb.EncryptedContent getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_encryptionpb_EncryptionMeta_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_encryptionpb_EncryptionMeta_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_encryptionpb_FileInfo_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_encryptionpb_FileInfo_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_encryptionpb_FileDictionary_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_encryptionpb_FileDictionary_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_encryptionpb_FileDictionary_FilesEntry_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_encryptionpb_FileDictionary_FilesEntry_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_encryptionpb_DataKey_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_encryptionpb_DataKey_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_encryptionpb_KeyDictionary_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_encryptionpb_KeyDictionary_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_encryptionpb_KeyDictionary_KeysEntry_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_encryptionpb_KeyDictionary_KeysEntry_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_encryptionpb_MasterKey_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_encryptionpb_MasterKey_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_encryptionpb_MasterKeyPlaintext_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_encryptionpb_MasterKeyPlaintext_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_encryptionpb_MasterKeyFile_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_encryptionpb_MasterKeyFile_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_encryptionpb_MasterKeyKms_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_encryptionpb_MasterKeyKms_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_encryptionpb_EncryptedContent_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_encryptionpb_EncryptedContent_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_encryptionpb_EncryptedContent_MetadataEntry_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_encryptionpb_EncryptedContent_MetadataEntry_fieldAccessorTable;
public static com.google.protobuf.Descriptors.FileDescriptor
getDescriptor() {
return descriptor;
}
private static com.google.protobuf.Descriptors.FileDescriptor
descriptor;
static {
java.lang.String[] descriptorData = {
"\n\022encryptionpb.proto\022\014encryptionpb\032\024gogo" +
"proto/gogo.proto\032\017rustproto.proto\",\n\016Enc" +
"ryptionMeta\022\016\n\006key_id\030\001 \001(\004\022\n\n\002iv\030\002 \001(\014\"" +
"V\n\010FileInfo\022\016\n\006key_id\030\001 \001(\004\022\n\n\002iv\030\002 \001(\014\022" +
".\n\006method\030\003 \001(\0162\036.encryptionpb.Encryptio" +
"nMethod\"\216\001\n\016FileDictionary\0226\n\005files\030\001 \003(" +
"\0132\'.encryptionpb.FileDictionary.FilesEnt" +
"ry\032D\n\nFilesEntry\022\013\n\003key\030\001 \001(\t\022%\n\005value\030\002" +
" \001(\0132\026.encryptionpb.FileInfo:\0028\001\"r\n\007Data" +
"Key\022\013\n\003key\030\001 \001(\014\022.\n\006method\030\002 \001(\0162\036.encry" +
"ptionpb.EncryptionMethod\022\025\n\rcreation_tim" +
"e\030\003 \001(\004\022\023\n\013was_exposed\030\004 \001(\010\"\240\001\n\rKeyDict" +
"ionary\0223\n\004keys\030\001 \003(\0132%.encryptionpb.KeyD" +
"ictionary.KeysEntry\022\026\n\016current_key_id\030\002 " +
"\001(\004\032B\n\tKeysEntry\022\013\n\003key\030\001 \001(\004\022$\n\005value\030\002" +
" \001(\0132\025.encryptionpb.DataKey:\0028\001\"\245\001\n\tMast" +
"erKey\0225\n\tplaintext\030\001 \001(\0132 .encryptionpb." +
"MasterKeyPlaintextH\000\022+\n\004file\030\002 \001(\0132\033.enc" +
"ryptionpb.MasterKeyFileH\000\022)\n\003kms\030\003 \001(\0132\032" +
".encryptionpb.MasterKeyKmsH\000B\t\n\007backend\"" +
"\024\n\022MasterKeyPlaintext\"\035\n\rMasterKeyFile\022\014" +
"\n\004path\030\001 \001(\t\"P\n\014MasterKeyKms\022\016\n\006vendor\030\001" +
" \001(\t\022\016\n\006key_id\030\002 \001(\t\022\016\n\006region\030\003 \001(\t\022\020\n\010" +
"endpoint\030\004 \001(\t\"\345\001\n\020EncryptedContent\022>\n\010m" +
"etadata\030\001 \003(\0132,.encryptionpb.EncryptedCo" +
"ntent.MetadataEntry\022\017\n\007content\030\002 \001(\014\022+\n\n" +
"master_key\030\003 \001(\0132\027.encryptionpb.MasterKe" +
"y\022\n\n\002iv\030\004 \001(\014\022\026\n\016ciphertext_key\030\005 \001(\014\032/\n" +
"\rMetadataEntry\022\013\n\003key\030\001 \001(\t\022\r\n\005value\030\002 \001" +
"(\014:\0028\001*^\n\020EncryptionMethod\022\013\n\007UNKNOWN\020\000\022" +
"\r\n\tPLAINTEXT\020\001\022\016\n\nAES128_CTR\020\002\022\016\n\nAES192" +
"_CTR\020\003\022\016\n\nAES256_CTR\020\004B\"\n\020org.tikv.kvpro" +
"to\340\342\036\001\310\342\036\001\320\342\036\001\330\250\010\001b\006proto3"
};
com.google.protobuf.Descriptors.FileDescriptor.InternalDescriptorAssigner assigner =
new com.google.protobuf.Descriptors.FileDescriptor. InternalDescriptorAssigner() {
public com.google.protobuf.ExtensionRegistry assignDescriptors(
com.google.protobuf.Descriptors.FileDescriptor root) {
descriptor = root;
return null;
}
};
com.google.protobuf.Descriptors.FileDescriptor
.internalBuildGeneratedFileFrom(descriptorData,
new com.google.protobuf.Descriptors.FileDescriptor[] {
com.google.protobuf.GoGoProtos.getDescriptor(),
rustproto.Rustproto.getDescriptor(),
}, assigner);
internal_static_encryptionpb_EncryptionMeta_descriptor =
getDescriptor().getMessageTypes().get(0);
internal_static_encryptionpb_EncryptionMeta_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_encryptionpb_EncryptionMeta_descriptor,
new java.lang.String[] { "KeyId", "Iv", });
internal_static_encryptionpb_FileInfo_descriptor =
getDescriptor().getMessageTypes().get(1);
internal_static_encryptionpb_FileInfo_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_encryptionpb_FileInfo_descriptor,
new java.lang.String[] { "KeyId", "Iv", "Method", });
internal_static_encryptionpb_FileDictionary_descriptor =
getDescriptor().getMessageTypes().get(2);
internal_static_encryptionpb_FileDictionary_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_encryptionpb_FileDictionary_descriptor,
new java.lang.String[] { "Files", });
internal_static_encryptionpb_FileDictionary_FilesEntry_descriptor =
internal_static_encryptionpb_FileDictionary_descriptor.getNestedTypes().get(0);
internal_static_encryptionpb_FileDictionary_FilesEntry_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_encryptionpb_FileDictionary_FilesEntry_descriptor,
new java.lang.String[] { "Key", "Value", });
internal_static_encryptionpb_DataKey_descriptor =
getDescriptor().getMessageTypes().get(3);
internal_static_encryptionpb_DataKey_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_encryptionpb_DataKey_descriptor,
new java.lang.String[] { "Key", "Method", "CreationTime", "WasExposed", });
internal_static_encryptionpb_KeyDictionary_descriptor =
getDescriptor().getMessageTypes().get(4);
internal_static_encryptionpb_KeyDictionary_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_encryptionpb_KeyDictionary_descriptor,
new java.lang.String[] { "Keys", "CurrentKeyId", });
internal_static_encryptionpb_KeyDictionary_KeysEntry_descriptor =
internal_static_encryptionpb_KeyDictionary_descriptor.getNestedTypes().get(0);
internal_static_encryptionpb_KeyDictionary_KeysEntry_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_encryptionpb_KeyDictionary_KeysEntry_descriptor,
new java.lang.String[] { "Key", "Value", });
internal_static_encryptionpb_MasterKey_descriptor =
getDescriptor().getMessageTypes().get(5);
internal_static_encryptionpb_MasterKey_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_encryptionpb_MasterKey_descriptor,
new java.lang.String[] { "Plaintext", "File", "Kms", "Backend", });
internal_static_encryptionpb_MasterKeyPlaintext_descriptor =
getDescriptor().getMessageTypes().get(6);
internal_static_encryptionpb_MasterKeyPlaintext_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_encryptionpb_MasterKeyPlaintext_descriptor,
new java.lang.String[] { });
internal_static_encryptionpb_MasterKeyFile_descriptor =
getDescriptor().getMessageTypes().get(7);
internal_static_encryptionpb_MasterKeyFile_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_encryptionpb_MasterKeyFile_descriptor,
new java.lang.String[] { "Path", });
internal_static_encryptionpb_MasterKeyKms_descriptor =
getDescriptor().getMessageTypes().get(8);
internal_static_encryptionpb_MasterKeyKms_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_encryptionpb_MasterKeyKms_descriptor,
new java.lang.String[] { "Vendor", "KeyId", "Region", "Endpoint", });
internal_static_encryptionpb_EncryptedContent_descriptor =
getDescriptor().getMessageTypes().get(9);
internal_static_encryptionpb_EncryptedContent_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_encryptionpb_EncryptedContent_descriptor,
new java.lang.String[] { "Metadata", "Content", "MasterKey", "Iv", "CiphertextKey", });
internal_static_encryptionpb_EncryptedContent_MetadataEntry_descriptor =
internal_static_encryptionpb_EncryptedContent_descriptor.getNestedTypes().get(0);
internal_static_encryptionpb_EncryptedContent_MetadataEntry_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_encryptionpb_EncryptedContent_MetadataEntry_descriptor,
new java.lang.String[] { "Key", "Value", });
com.google.protobuf.ExtensionRegistry registry =
com.google.protobuf.ExtensionRegistry.newInstance();
registry.add(com.google.protobuf.GoGoProtos.marshalerAll);
registry.add(com.google.protobuf.GoGoProtos.sizerAll);
registry.add(com.google.protobuf.GoGoProtos.unmarshalerAll);
registry.add(rustproto.Rustproto.liteRuntimeAll);
com.google.protobuf.Descriptors.FileDescriptor
.internalUpdateFileDescriptor(descriptor, registry);
com.google.protobuf.GoGoProtos.getDescriptor();
rustproto.Rustproto.getDescriptor();
}
// @@protoc_insertion_point(outer_class_scope)
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy