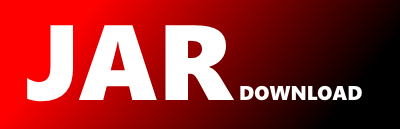
com.pivovarit.collectors.ParallelCollectors Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of parallel-collectors Show documentation
Show all versions of parallel-collectors Show documentation
Parallel collection processing with customizable thread pools
package com.pivovarit.collectors;
import java.util.Collection;
import java.util.List;
import java.util.Map;
import java.util.Set;
import java.util.concurrent.CompletableFuture;
import java.util.concurrent.Executor;
import java.util.function.BinaryOperator;
import java.util.function.Function;
import java.util.function.Supplier;
import java.util.stream.Collector;
import java.util.stream.Stream;
import static com.pivovarit.collectors.AsyncParallelCollector.defaultListSupplier;
import static com.pivovarit.collectors.AsyncParallelCollector.defaultSetSupplier;
import static java.util.Objects.requireNonNull;
/**
* An umbrella class exposing static factory methods for instantiating parallel {@link Collector}s
*
* @author Grzegorz Piwowarek
*/
public final class ParallelCollectors {
private ParallelCollectors() {
}
/**
* A convenience {@link Collector} used for executing parallel computations on a custom {@link Executor}
* and returning them as a {@link CompletableFuture} containing a user-provided {@link Collection} of these elements.
*
*
* The parallelism level defaults to {@code Runtime.availableProcessors() - 1}
*
*
* The collector maintains the order of processed {@link Stream}. Instances should not be reused.
*
*
* Example:
* {@code
* CompletableFuture> result = Stream.of(1, 2, 3)
* .collect(parallelToCollection(i -> foo(i), TreeSet::new, executor));
* }
*
* @param mapper a transformation to be performed in parallel
* @param collectionSupplier a {@code Supplier} which returns a mutable {@code Collection} of the appropriate type
* @param executor the {@code Executor} to use for asynchronous execution
* @param the type of the collected elements
* @param the result returned by {@code mapper}
* @param the collection supplier
*
* @return a {@code Collector} which collects all processed elements into a user-provided mutable {@code Collection} in parallel
*
* @since 0.0.1
*/
public static > Collector> parallelToCollection(Function mapper, Supplier collectionSupplier, Executor executor) {
return AsyncParallelCollector.collectingToCollection(mapper, collectionSupplier, executor);
}
/**
* A convenience {@link Collector} used for executing parallel computations on a custom {@link Executor}
* and returning them as {@link CompletableFuture} containing a user-provided {@link Collection} {@link R} of these elements
*
*
* Encounter order is preserved. Instances should not be reused.
*
*
* Example:
* {@code
* CompletableFuture> result = Stream.of(1, 2, 3)
* .collect(parallelToCollection(i -> foo(i), TreeSet::new, executor, 2));
* }
*
* @param mapper a transformation to be performed in parallel
* @param collectionSupplier a {@code Supplier} which returns a mutable {@code Collection} of the appropriate type
* @param executor the {@code Executor} to use for asynchronous execution
* @param parallelism the parallelism level
* @param the type of the collected elements
* @param the result returned by {@code mapper}
* @param the collection supplier
*
* @return a {@code Collector} which collects all processed elements into a user-provided mutable {@code Collection} in parallel
*
* @since 0.0.1
*/
public static > Collector> parallelToCollection(Function mapper, Supplier collectionSupplier, Executor executor, int parallelism) {
return AsyncParallelCollector.collectingToCollection(mapper, collectionSupplier, executor, parallelism);
}
/**
* A convenience {@link Collector} used for executing parallel computations on a custom {@link Executor}
* and returning them as {@link CompletableFuture} containing a {@link List} of these elements
*
*
* The parallelism level defaults to {@code Runtime.availableProcessors() - 1}
*
*
* The collector maintains the order of processed {@link Stream}. Instances should not be reused.
*
*
* Example:
* {@code
* CompletableFuture> result = Stream.of(1, 2, 3)
* .collect(parallelToList(i -> foo(), executor));
* }
*
* @param mapper a transformation to be performed in parallel
* @param executor the {@code Executor} to use for asynchronous execution
* @param the type of the collected elements
* @param the result returned by {@code mapper}
*
* @return a {@code Collector} which collects all processed elements into a user-provided mutable {@code List} in parallel
*
* @since 0.0.1
*/
public static Collector>> parallelToList(Function mapper, Executor executor) {
return AsyncParallelCollector.collectingToCollection(mapper, defaultListSupplier(), executor);
}
/**
* A convenience {@link Collector} used for executing parallel computations on a custom {@link Executor}
* and returning them as {@link CompletableFuture} containing a {@link List} of these elements
*
*
* Encounter order is preserved. Instances should not be reused.
*
*
* Example:
* {@code
* CompletableFuture> result = Stream.of(1, 2, 3)
* .collect(parallelToList(i -> foo(), executor, 2));
* }
*
* @param mapper a transformation to be performed in parallel
* @param executor the {@code Executor} to use for asynchronous execution
* @param parallelism the parallelism level
* @param the type of the collected elements
* @param the result returned by {@code mapper}
*
* @return a {@code Collector} which collects all processed elements into a user-provided mutable {@code List} in parallel
*
* @since 0.0.1
*/
public static Collector>> parallelToList(Function mapper, Executor executor, int parallelism) {
return AsyncParallelCollector.collectingToCollection(mapper, defaultListSupplier(), executor, parallelism);
}
/**
* A convenience {@link Collector} used for executing parallel computations on a custom {@link Executor}
* and returning them as {@link CompletableFuture} containing a {@link Set} of these elements
*
*
* The parallelism level defaults to {@code Runtime.availableProcessors() - 1}
*
*
* The collector maintains the order of processed {@link Stream}. Instances should not be reused.
*
*
* Example:
* {@code
* CompletableFuture> result = Stream.of(1, 2, 3)
* .collect(parallelToSet(i -> foo(), executor));
* }
*
* @param mapper a transformation to be performed in parallel
* @param executor the {@code Executor} to use for asynchronous execution
* @param the type of the collected elements
* @param the result returned by {@code mapper}
*
* @return a {@code Collector} which collects all processed elements into a user-provided mutable {@code Set} in parallel
*
* @since 0.0.1
*/
public static Collector>> parallelToSet(Function mapper, Executor executor) {
return AsyncParallelCollector.collectingToCollection(mapper, defaultSetSupplier(), executor);
}
/**
* A convenience {@link Collector} used for executing parallel computations on a custom {@link Executor}
* and returning them as {@link CompletableFuture} containing a {@link Set} of these elements
*
*
* Encounter order is preserved. Instances should not be reused.
*
*
* Warning: this implementation can't be used with infinite {@link java.util.stream.Stream} instances.
* It will try to submit {@code N} tasks to a provided {@link Executor}
* where {@code N} is a size of a collected {@link java.util.stream.Stream}
*
*
* Example:
* {@code
* CompletableFuture> result = Stream.of(1, 2, 3)
* .collect(parallelToSet(i -> foo(), executor, 2));
* }
*
* @param mapper a transformation to be performed in parallel
* @param executor the {@code Executor} to use for asynchronous execution
* @param parallelism the parallelism level
* @param the type of the collected elements
* @param the result returned by {@code mapper}
*
* @return a {@code Collector} which collects all processed elements into a user-provided mutable {@code Set} in parallel
*
* @since 0.0.1
*/
public static Collector>> parallelToSet(Function mapper, Executor executor, int parallelism) {
return AsyncParallelCollector.collectingToCollection(mapper, defaultSetSupplier(), executor, parallelism);
}
/**
* A convenience {@link Collector} used for executing parallel computations on a custom {@link Executor}
* and returning them as {@link CompletableFuture} containing a {@link Map} of these elements
*
*
* The parallelism level defaults to {@code Runtime.availableProcessors() - 1}
*
*
* On duplicate key, completes exceptionally with {@link IllegalStateException}
*
*
* Example:
* {@code
* CompletableFuture
*
* @param keyMapper the key deriving operation to be performed in parallel
* @param valueMapper the value deriving operation to be performed in parallel
* @param executor the {@code Executor} to use for asynchronous execution
* @param the type of the collected elements
* @param the target {@code Map} key type
* @param the target {@code Map} value type
*
* @return a {@code Collector} which collects all input elements into a user-provided mutable {@code Map} in parallel
*
* @since 0.2.0
*/
public static Collector>> parallelToMap(Function keyMapper, Function valueMapper, Executor executor) {
return AsyncParallelCollector.collectingToMap(keyMapper, valueMapper, executor);
}
/**
* A convenience {@link Collector} used for executing parallel computations on a custom {@link Executor}
* and returning them as {@link CompletableFuture} containing a {@link Map} of these elements
*
*
* Encounter order is preserved. Instances should not be reused.
*
* On duplicate key, completes exceptionally with {@link IllegalStateException}
*
*
* Example:
* {@code
* CompletableFuture
*
* @param keyMapper the key deriving operation to be performed in parallel
* @param valueMapper the value deriving operation to be performed in parallel
* @param executor the {@code Executor} to use for asynchronous execution
* @param parallelism the parallelism level
* @param the type of the collected elements
* @param the target {@code Map} key type
* @param the target {@code Map} value type
*
* @return a {@code Collector} which collects all input elements into a user-provided mutable {@code Map} in parallel
*
* @since 0.2.0
*/
public static Collector>> parallelToMap(Function keyMapper, Function valueMapper, Executor executor, int parallelism) {
return AsyncParallelCollector.collectingToMap(keyMapper, valueMapper, executor, parallelism);
}
/**
* A convenience {@link Collector} used for executing parallel computations on a custom {@link Executor}
* and returning them as {@link CompletableFuture} containing a {@link Map} of these elements
*
*
* The parallelism level defaults to {@code Runtime.availableProcessors() - 1}
*
*
* Example:
* {@code
* CompletableFuture
*
* @param keyMapper the key deriving operation to be performed in parallel
* @param valueMapper the value deriving operation to be performed in parallel
* @param merger the duplicate key value resolution strategy
* @param executor the {@code Executor} to use for asynchronous execution
* @param the type of the collected elements
* @param the target {@code Map} key type
* @param the target {@code Map} value type
*
* @return a {@code Collector} which collects all input elements into a user-provided mutable {@code Map} in parallel
*
* @since 0.2.0
*/
public static Collector>> parallelToMap(Function keyMapper, Function valueMapper, BinaryOperator merger, Executor executor) {
return AsyncParallelCollector.collectingToMap(keyMapper, valueMapper, merger, executor);
}
/**
* A convenience {@link Collector} used for executing parallel computations on a custom {@link Executor}
* and returning them as {@link CompletableFuture} containing a {@link Map} of these elements
*
*
* Encounter order is preserved. Instances should not be reused.
*
*
* Example:
* {@code
* CompletableFuture
*
* @param keyMapper the key deriving operation to be performed in parallel
* @param valueMapper the value deriving operation to be performed in parallel
* @param merger the duplicate key value resolution strategy
* @param executor the {@code Executor} to use for asynchronous execution
* @param parallelism the parallelism level
* @param the type of the collected elements
* @param the target {@code Map} key type
* @param the target {@code Map} value type
*
* @return a {@code Collector} which collects all input elements into a user-provided mutable {@code Map} in parallel
*
* @since 0.2.0
*/
public static Collector>> parallelToMap(Function keyMapper, Function valueMapper, BinaryOperator merger, Executor executor, int parallelism) {
return AsyncParallelCollector.collectingToMap(keyMapper, valueMapper, merger, executor, parallelism);
}
/**
* A convenience {@link Collector} used for executing parallel computations on a custom {@link Executor}
* and returning them as {@link CompletableFuture} containing a {@link Map} of these elements
*
*
* The parallelism level defaults to {@code Runtime.availableProcessors() - 1}
*
*
* The collector maintains the order of processed {@link Stream}. Instances should not be reused.
*
* On duplicate key, completes exceptionally with {@link IllegalStateException}
*
*
* Example:
* {@code
* CompletableFuture
*
* @param keyMapper the key deriving operation to be performed in parallel
* @param valueMapper the value deriving operation to be performed in parallel
* @param mapSupplier the factory returning a target {@code Map} instance
* @param executor the {@code Executor} to use for asynchronous execution
* @param the type of the collected elements
* @param the target {@code Map} key type
* @param the target {@code Map} value type
*
* @return a {@code Collector} which collects all input elements into a user-provided mutable {@code Map} in parallel
*
* @since 0.2.0
*/
public static Collector>> parallelToMap(Function keyMapper, Function valueMapper, Supplier
© 2015 - 2025 Weber Informatics LLC | Privacy Policy