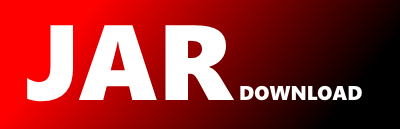
com.pivovarit.collectors.CompletionOrderSpliterator Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of parallel-collectors Show documentation
Show all versions of parallel-collectors Show documentation
Parallel collection processing with customizable thread pools
package com.pivovarit.collectors;
import java.util.AbstractMap;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import java.util.Spliterator;
import java.util.concurrent.CompletableFuture;
import java.util.function.Consumer;
import static java.util.concurrent.CompletableFuture.anyOf;
/**
* @author Grzegorz Piwowarek
*/
final class CompletionOrderSpliterator implements Spliterator {
private final Map>> indexedFutures;
CompletionOrderSpliterator(List> futures) {
indexedFutures = toIndexedFutures(futures);
}
@Override
public boolean tryAdvance(Consumer super T> action) {
if (!indexedFutures.isEmpty()) {
action.accept(nextCompleted());
return true;
} else {
return false;
}
}
private T nextCompleted() {
return anyOf(indexedFutures.values().toArray(new CompletableFuture[0]))
.thenApply(result -> ((Map.Entry) result))
.thenApply(result -> {
indexedFutures.remove(result.getKey());
return result.getValue();
}).join();
}
@Override
public Spliterator trySplit() {
return null;
}
@Override
public long estimateSize() {
return indexedFutures.size();
}
@Override
public int characteristics() {
return SIZED & IMMUTABLE & NONNULL;
}
private static Map>> toIndexedFutures(List> futures) {
Map>> map = new HashMap<>(futures.size(), 1);
int counter = 0;
for (CompletableFuture future : futures) {
int index = counter++;
map.put(index, future.thenApply(value -> new AbstractMap.SimpleEntry<>(index, value)));
}
return map;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy