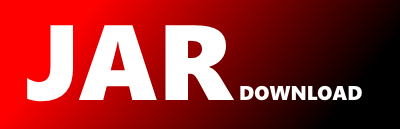
com.plaid.client.model.AssetReportCreateRequest Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of plaid-java Show documentation
Show all versions of plaid-java Show documentation
Bindings for the Plaid (plaid.com) API.
/*
* The Plaid API
* The Plaid REST API. Please see https://plaid.com/docs/api for more details.
*
* The version of the OpenAPI document: 2020-09-14_1.503.5
*
*
* NOTE: This class is auto generated by OpenAPI Generator (https://openapi-generator.tech).
* https://openapi-generator.tech
* Do not edit the class manually.
*/
package com.plaid.client.model;
import java.util.Objects;
import java.util.Arrays;
import com.google.gson.TypeAdapter;
import com.google.gson.annotations.JsonAdapter;
import com.google.gson.annotations.SerializedName;
import com.google.gson.stream.JsonReader;
import com.google.gson.stream.JsonWriter;
import com.plaid.client.model.AssetReportCreateRequestOptions;
import io.swagger.annotations.ApiModel;
import io.swagger.annotations.ApiModelProperty;
import java.io.IOException;
import java.util.ArrayList;
import java.util.List;
/**
* AssetReportCreateRequest defines the request schema for `/asset_report/create`
*/
@ApiModel(description = "AssetReportCreateRequest defines the request schema for `/asset_report/create`")
@javax.annotation.Generated(value = "org.openapitools.codegen.languages.JavaClientCodegen", date = "2024-03-28T21:00:55.745394Z[Etc/UTC]")
public class AssetReportCreateRequest {
public static final String SERIALIZED_NAME_CLIENT_ID = "client_id";
@SerializedName(SERIALIZED_NAME_CLIENT_ID)
private String clientId;
public static final String SERIALIZED_NAME_SECRET = "secret";
@SerializedName(SERIALIZED_NAME_SECRET)
private String secret;
public static final String SERIALIZED_NAME_ACCESS_TOKENS = "access_tokens";
@SerializedName(SERIALIZED_NAME_ACCESS_TOKENS)
private List accessTokens = null;
public static final String SERIALIZED_NAME_DAYS_REQUESTED = "days_requested";
@SerializedName(SERIALIZED_NAME_DAYS_REQUESTED)
private Integer daysRequested;
public static final String SERIALIZED_NAME_OPTIONS = "options";
@SerializedName(SERIALIZED_NAME_OPTIONS)
private AssetReportCreateRequestOptions options;
public AssetReportCreateRequest clientId(String clientId) {
this.clientId = clientId;
return this;
}
/**
* Your Plaid API `client_id`. The `client_id` is required and may be provided either in the `PLAID-CLIENT-ID` header or as part of a request body.
* @return clientId
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Your Plaid API `client_id`. The `client_id` is required and may be provided either in the `PLAID-CLIENT-ID` header or as part of a request body.")
public String getClientId() {
return clientId;
}
public void setClientId(String clientId) {
this.clientId = clientId;
}
public AssetReportCreateRequest secret(String secret) {
this.secret = secret;
return this;
}
/**
* Your Plaid API `secret`. The `secret` is required and may be provided either in the `PLAID-SECRET` header or as part of a request body.
* @return secret
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Your Plaid API `secret`. The `secret` is required and may be provided either in the `PLAID-SECRET` header or as part of a request body.")
public String getSecret() {
return secret;
}
public void setSecret(String secret) {
this.secret = secret;
}
public AssetReportCreateRequest accessTokens(List accessTokens) {
this.accessTokens = accessTokens;
return this;
}
public AssetReportCreateRequest addAccessTokensItem(String accessTokensItem) {
if (this.accessTokens == null) {
this.accessTokens = new ArrayList<>();
}
this.accessTokens.add(accessTokensItem);
return this;
}
/**
* An array of access tokens corresponding to the Items that will be included in the report. The `assets` product must have been initialized for the Items during link; the Assets product cannot be added after initialization.
* @return accessTokens
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "An array of access tokens corresponding to the Items that will be included in the report. The `assets` product must have been initialized for the Items during link; the Assets product cannot be added after initialization.")
public List getAccessTokens() {
return accessTokens;
}
public void setAccessTokens(List accessTokens) {
this.accessTokens = accessTokens;
}
public AssetReportCreateRequest daysRequested(Integer daysRequested) {
this.daysRequested = daysRequested;
return this;
}
/**
* The maximum integer number of days of history to include in the Asset Report. If using Fannie Mae Day 1 Certainty, `days_requested` must be at least 61 for new originations or at least 31 for refinancings. An Asset Report requested with \"Additional History\" (that is, with more than 61 days of transaction history) will incur an Additional History fee.
* minimum: 0
* maximum: 731
* @return daysRequested
**/
@ApiModelProperty(required = true, value = "The maximum integer number of days of history to include in the Asset Report. If using Fannie Mae Day 1 Certainty, `days_requested` must be at least 61 for new originations or at least 31 for refinancings. An Asset Report requested with \"Additional History\" (that is, with more than 61 days of transaction history) will incur an Additional History fee.")
public Integer getDaysRequested() {
return daysRequested;
}
public void setDaysRequested(Integer daysRequested) {
this.daysRequested = daysRequested;
}
public AssetReportCreateRequest options(AssetReportCreateRequestOptions options) {
this.options = options;
return this;
}
/**
* Get options
* @return options
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
public AssetReportCreateRequestOptions getOptions() {
return options;
}
public void setOptions(AssetReportCreateRequestOptions options) {
this.options = options;
}
@Override
public boolean equals(Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
AssetReportCreateRequest assetReportCreateRequest = (AssetReportCreateRequest) o;
return Objects.equals(this.clientId, assetReportCreateRequest.clientId) &&
Objects.equals(this.secret, assetReportCreateRequest.secret) &&
Objects.equals(this.accessTokens, assetReportCreateRequest.accessTokens) &&
Objects.equals(this.daysRequested, assetReportCreateRequest.daysRequested) &&
Objects.equals(this.options, assetReportCreateRequest.options);
}
@Override
public int hashCode() {
return Objects.hash(clientId, secret, accessTokens, daysRequested, options);
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class AssetReportCreateRequest {\n");
sb.append(" clientId: ").append(toIndentedString(clientId)).append("\n");
sb.append(" secret: ").append(toIndentedString(secret)).append("\n");
sb.append(" accessTokens: ").append(toIndentedString(accessTokens)).append("\n");
sb.append(" daysRequested: ").append(toIndentedString(daysRequested)).append("\n");
sb.append(" options: ").append(toIndentedString(options)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces
* (except the first line).
*/
private String toIndentedString(Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy