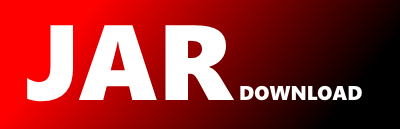
com.plaid.client.model.Credit1099 Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of plaid-java Show documentation
Show all versions of plaid-java Show documentation
Bindings for the Plaid (plaid.com) API.
/*
* The Plaid API
* The Plaid REST API. Please see https://plaid.com/docs/api for more details.
*
* The version of the OpenAPI document: 2020-09-14_1.503.5
*
*
* NOTE: This class is auto generated by OpenAPI Generator (https://openapi-generator.tech).
* https://openapi-generator.tech
* Do not edit the class manually.
*/
package com.plaid.client.model;
import java.util.Objects;
import java.util.Arrays;
import com.google.gson.TypeAdapter;
import com.google.gson.annotations.JsonAdapter;
import com.google.gson.annotations.SerializedName;
import com.google.gson.stream.JsonReader;
import com.google.gson.stream.JsonWriter;
import com.plaid.client.model.Credit1099Filer;
import com.plaid.client.model.Credit1099Payer;
import com.plaid.client.model.Credit1099Recipient;
import com.plaid.client.model.CreditDocumentMetadata;
import com.plaid.client.model.Form1099Type;
import io.swagger.annotations.ApiModel;
import io.swagger.annotations.ApiModelProperty;
import java.io.IOException;
/**
* An object representing an end user's 1099 tax form
*/
@ApiModel(description = "An object representing an end user's 1099 tax form")
@javax.annotation.Generated(value = "org.openapitools.codegen.languages.JavaClientCodegen", date = "2024-03-28T21:00:55.745394Z[Etc/UTC]")
public class Credit1099 {
public static final String SERIALIZED_NAME_DOCUMENT_ID = "document_id";
@SerializedName(SERIALIZED_NAME_DOCUMENT_ID)
private String documentId;
public static final String SERIALIZED_NAME_DOCUMENT_METADATA = "document_metadata";
@SerializedName(SERIALIZED_NAME_DOCUMENT_METADATA)
private CreditDocumentMetadata documentMetadata;
public static final String SERIALIZED_NAME_FORM1099_TYPE = "form_1099_type";
@SerializedName(SERIALIZED_NAME_FORM1099_TYPE)
private Form1099Type form1099Type;
public static final String SERIALIZED_NAME_RECIPIENT = "recipient";
@SerializedName(SERIALIZED_NAME_RECIPIENT)
private Credit1099Recipient recipient;
public static final String SERIALIZED_NAME_PAYER = "payer";
@SerializedName(SERIALIZED_NAME_PAYER)
private Credit1099Payer payer;
public static final String SERIALIZED_NAME_FILER = "filer";
@SerializedName(SERIALIZED_NAME_FILER)
private Credit1099Filer filer;
public static final String SERIALIZED_NAME_TAX_YEAR = "tax_year";
@SerializedName(SERIALIZED_NAME_TAX_YEAR)
private String taxYear;
public static final String SERIALIZED_NAME_RENTS = "rents";
@SerializedName(SERIALIZED_NAME_RENTS)
private Double rents;
public static final String SERIALIZED_NAME_ROYALTIES = "royalties";
@SerializedName(SERIALIZED_NAME_ROYALTIES)
private Double royalties;
public static final String SERIALIZED_NAME_OTHER_INCOME = "other_income";
@SerializedName(SERIALIZED_NAME_OTHER_INCOME)
private Double otherIncome;
public static final String SERIALIZED_NAME_FEDERAL_INCOME_TAX_WITHHELD = "federal_income_tax_withheld";
@SerializedName(SERIALIZED_NAME_FEDERAL_INCOME_TAX_WITHHELD)
private Double federalIncomeTaxWithheld;
public static final String SERIALIZED_NAME_FISHING_BOAT_PROCEEDS = "fishing_boat_proceeds";
@SerializedName(SERIALIZED_NAME_FISHING_BOAT_PROCEEDS)
private Double fishingBoatProceeds;
public static final String SERIALIZED_NAME_MEDICAL_AND_HEALTHCARE_PAYMENTS = "medical_and_healthcare_payments";
@SerializedName(SERIALIZED_NAME_MEDICAL_AND_HEALTHCARE_PAYMENTS)
private Double medicalAndHealthcarePayments;
public static final String SERIALIZED_NAME_NONEMPLOYEE_COMPENSATION = "nonemployee_compensation";
@SerializedName(SERIALIZED_NAME_NONEMPLOYEE_COMPENSATION)
private Double nonemployeeCompensation;
public static final String SERIALIZED_NAME_SUBSTITUTE_PAYMENTS_IN_LIEU_OF_DIVIDENDS_OR_INTEREST = "substitute_payments_in_lieu_of_dividends_or_interest";
@SerializedName(SERIALIZED_NAME_SUBSTITUTE_PAYMENTS_IN_LIEU_OF_DIVIDENDS_OR_INTEREST)
private Double substitutePaymentsInLieuOfDividendsOrInterest;
public static final String SERIALIZED_NAME_PAYER_MADE_DIRECT_SALES_OF5000_OR_MORE_OF_CONSUMER_PRODUCTS_TO_BUYER = "payer_made_direct_sales_of_5000_or_more_of_consumer_products_to_buyer";
@SerializedName(SERIALIZED_NAME_PAYER_MADE_DIRECT_SALES_OF5000_OR_MORE_OF_CONSUMER_PRODUCTS_TO_BUYER)
private String payerMadeDirectSalesOf5000OrMoreOfConsumerProductsToBuyer;
public static final String SERIALIZED_NAME_CROP_INSURANCE_PROCEEDS = "crop_insurance_proceeds";
@SerializedName(SERIALIZED_NAME_CROP_INSURANCE_PROCEEDS)
private Double cropInsuranceProceeds;
public static final String SERIALIZED_NAME_EXCESS_GOLDEN_PARACHUTE_PAYMENTS = "excess_golden_parachute_payments";
@SerializedName(SERIALIZED_NAME_EXCESS_GOLDEN_PARACHUTE_PAYMENTS)
private Double excessGoldenParachutePayments;
public static final String SERIALIZED_NAME_GROSS_PROCEEDS_PAID_TO_AN_ATTORNEY = "gross_proceeds_paid_to_an_attorney";
@SerializedName(SERIALIZED_NAME_GROSS_PROCEEDS_PAID_TO_AN_ATTORNEY)
private Double grossProceedsPaidToAnAttorney;
public static final String SERIALIZED_NAME_SECTION409A_DEFERRALS = "section_409a_deferrals";
@SerializedName(SERIALIZED_NAME_SECTION409A_DEFERRALS)
private Double section409aDeferrals;
public static final String SERIALIZED_NAME_SECTION409A_INCOME = "section_409a_income";
@SerializedName(SERIALIZED_NAME_SECTION409A_INCOME)
private Double section409aIncome;
public static final String SERIALIZED_NAME_STATE_TAX_WITHHELD = "state_tax_withheld";
@SerializedName(SERIALIZED_NAME_STATE_TAX_WITHHELD)
private Double stateTaxWithheld;
public static final String SERIALIZED_NAME_STATE_TAX_WITHHELD_LOWER = "state_tax_withheld_lower";
@SerializedName(SERIALIZED_NAME_STATE_TAX_WITHHELD_LOWER)
private Double stateTaxWithheldLower;
public static final String SERIALIZED_NAME_PAYER_STATE_NUMBER = "payer_state_number";
@SerializedName(SERIALIZED_NAME_PAYER_STATE_NUMBER)
private String payerStateNumber;
public static final String SERIALIZED_NAME_PAYER_STATE_NUMBER_LOWER = "payer_state_number_lower";
@SerializedName(SERIALIZED_NAME_PAYER_STATE_NUMBER_LOWER)
private String payerStateNumberLower;
public static final String SERIALIZED_NAME_STATE_INCOME = "state_income";
@SerializedName(SERIALIZED_NAME_STATE_INCOME)
private Double stateIncome;
public static final String SERIALIZED_NAME_STATE_INCOME_LOWER = "state_income_lower";
@SerializedName(SERIALIZED_NAME_STATE_INCOME_LOWER)
private Double stateIncomeLower;
public static final String SERIALIZED_NAME_TRANSACTIONS_REPORTED = "transactions_reported";
@SerializedName(SERIALIZED_NAME_TRANSACTIONS_REPORTED)
private String transactionsReported;
public static final String SERIALIZED_NAME_PSE_NAME = "pse_name";
@SerializedName(SERIALIZED_NAME_PSE_NAME)
private String pseName;
public static final String SERIALIZED_NAME_PSE_TELEPHONE_NUMBER = "pse_telephone_number";
@SerializedName(SERIALIZED_NAME_PSE_TELEPHONE_NUMBER)
private String pseTelephoneNumber;
public static final String SERIALIZED_NAME_GROSS_AMOUNT = "gross_amount";
@SerializedName(SERIALIZED_NAME_GROSS_AMOUNT)
private Double grossAmount;
public static final String SERIALIZED_NAME_CARD_NOT_PRESENT_TRANSACTION = "card_not_present_transaction";
@SerializedName(SERIALIZED_NAME_CARD_NOT_PRESENT_TRANSACTION)
private Double cardNotPresentTransaction;
public static final String SERIALIZED_NAME_MERCHANT_CATEGORY_CODE = "merchant_category_code";
@SerializedName(SERIALIZED_NAME_MERCHANT_CATEGORY_CODE)
private String merchantCategoryCode;
public static final String SERIALIZED_NAME_NUMBER_OF_PAYMENT_TRANSACTIONS = "number_of_payment_transactions";
@SerializedName(SERIALIZED_NAME_NUMBER_OF_PAYMENT_TRANSACTIONS)
private String numberOfPaymentTransactions;
public static final String SERIALIZED_NAME_JANUARY_AMOUNT = "january_amount";
@SerializedName(SERIALIZED_NAME_JANUARY_AMOUNT)
private Double januaryAmount;
public static final String SERIALIZED_NAME_FEBRUARY_AMOUNT = "february_amount";
@SerializedName(SERIALIZED_NAME_FEBRUARY_AMOUNT)
private Double februaryAmount;
public static final String SERIALIZED_NAME_MARCH_AMOUNT = "march_amount";
@SerializedName(SERIALIZED_NAME_MARCH_AMOUNT)
private Double marchAmount;
public static final String SERIALIZED_NAME_APRIL_AMOUNT = "april_amount";
@SerializedName(SERIALIZED_NAME_APRIL_AMOUNT)
private Double aprilAmount;
public static final String SERIALIZED_NAME_MAY_AMOUNT = "may_amount";
@SerializedName(SERIALIZED_NAME_MAY_AMOUNT)
private Double mayAmount;
public static final String SERIALIZED_NAME_JUNE_AMOUNT = "june_amount";
@SerializedName(SERIALIZED_NAME_JUNE_AMOUNT)
private Double juneAmount;
public static final String SERIALIZED_NAME_JULY_AMOUNT = "july_amount";
@SerializedName(SERIALIZED_NAME_JULY_AMOUNT)
private Double julyAmount;
public static final String SERIALIZED_NAME_AUGUST_AMOUNT = "august_amount";
@SerializedName(SERIALIZED_NAME_AUGUST_AMOUNT)
private Double augustAmount;
public static final String SERIALIZED_NAME_SEPTEMBER_AMOUNT = "september_amount";
@SerializedName(SERIALIZED_NAME_SEPTEMBER_AMOUNT)
private Double septemberAmount;
public static final String SERIALIZED_NAME_OCTOBER_AMOUNT = "october_amount";
@SerializedName(SERIALIZED_NAME_OCTOBER_AMOUNT)
private Double octoberAmount;
public static final String SERIALIZED_NAME_NOVEMBER_AMOUNT = "november_amount";
@SerializedName(SERIALIZED_NAME_NOVEMBER_AMOUNT)
private Double novemberAmount;
public static final String SERIALIZED_NAME_DECEMBER_AMOUNT = "december_amount";
@SerializedName(SERIALIZED_NAME_DECEMBER_AMOUNT)
private Double decemberAmount;
public static final String SERIALIZED_NAME_PRIMARY_STATE = "primary_state";
@SerializedName(SERIALIZED_NAME_PRIMARY_STATE)
private String primaryState;
public static final String SERIALIZED_NAME_SECONDARY_STATE = "secondary_state";
@SerializedName(SERIALIZED_NAME_SECONDARY_STATE)
private String secondaryState;
public static final String SERIALIZED_NAME_PRIMARY_STATE_ID = "primary_state_id";
@SerializedName(SERIALIZED_NAME_PRIMARY_STATE_ID)
private String primaryStateId;
public static final String SERIALIZED_NAME_SECONDARY_STATE_ID = "secondary_state_id";
@SerializedName(SERIALIZED_NAME_SECONDARY_STATE_ID)
private String secondaryStateId;
public static final String SERIALIZED_NAME_PRIMARY_STATE_INCOME_TAX = "primary_state_income_tax";
@SerializedName(SERIALIZED_NAME_PRIMARY_STATE_INCOME_TAX)
private Double primaryStateIncomeTax;
public static final String SERIALIZED_NAME_SECONDARY_STATE_INCOME_TAX = "secondary_state_income_tax";
@SerializedName(SERIALIZED_NAME_SECONDARY_STATE_INCOME_TAX)
private Double secondaryStateIncomeTax;
public Credit1099 documentId(String documentId) {
this.documentId = documentId;
return this;
}
/**
* An identifier of the document referenced by the document metadata.
* @return documentId
**/
@javax.annotation.Nullable
@ApiModelProperty(required = true, value = "An identifier of the document referenced by the document metadata.")
public String getDocumentId() {
return documentId;
}
public void setDocumentId(String documentId) {
this.documentId = documentId;
}
public Credit1099 documentMetadata(CreditDocumentMetadata documentMetadata) {
this.documentMetadata = documentMetadata;
return this;
}
/**
* Get documentMetadata
* @return documentMetadata
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
public CreditDocumentMetadata getDocumentMetadata() {
return documentMetadata;
}
public void setDocumentMetadata(CreditDocumentMetadata documentMetadata) {
this.documentMetadata = documentMetadata;
}
public Credit1099 form1099Type(Form1099Type form1099Type) {
this.form1099Type = form1099Type;
return this;
}
/**
* Get form1099Type
* @return form1099Type
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
public Form1099Type getForm1099Type() {
return form1099Type;
}
public void setForm1099Type(Form1099Type form1099Type) {
this.form1099Type = form1099Type;
}
public Credit1099 recipient(Credit1099Recipient recipient) {
this.recipient = recipient;
return this;
}
/**
* Get recipient
* @return recipient
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
public Credit1099Recipient getRecipient() {
return recipient;
}
public void setRecipient(Credit1099Recipient recipient) {
this.recipient = recipient;
}
public Credit1099 payer(Credit1099Payer payer) {
this.payer = payer;
return this;
}
/**
* Get payer
* @return payer
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
public Credit1099Payer getPayer() {
return payer;
}
public void setPayer(Credit1099Payer payer) {
this.payer = payer;
}
public Credit1099 filer(Credit1099Filer filer) {
this.filer = filer;
return this;
}
/**
* Get filer
* @return filer
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
public Credit1099Filer getFiler() {
return filer;
}
public void setFiler(Credit1099Filer filer) {
this.filer = filer;
}
public Credit1099 taxYear(String taxYear) {
this.taxYear = taxYear;
return this;
}
/**
* Tax year of the tax form.
* @return taxYear
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Tax year of the tax form.")
public String getTaxYear() {
return taxYear;
}
public void setTaxYear(String taxYear) {
this.taxYear = taxYear;
}
public Credit1099 rents(Double rents) {
this.rents = rents;
return this;
}
/**
* Amount in rent by payer.
* @return rents
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Amount in rent by payer.")
public Double getRents() {
return rents;
}
public void setRents(Double rents) {
this.rents = rents;
}
public Credit1099 royalties(Double royalties) {
this.royalties = royalties;
return this;
}
/**
* Amount in royalties by payer.
* @return royalties
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Amount in royalties by payer.")
public Double getRoyalties() {
return royalties;
}
public void setRoyalties(Double royalties) {
this.royalties = royalties;
}
public Credit1099 otherIncome(Double otherIncome) {
this.otherIncome = otherIncome;
return this;
}
/**
* Amount in other income by payer.
* @return otherIncome
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Amount in other income by payer.")
public Double getOtherIncome() {
return otherIncome;
}
public void setOtherIncome(Double otherIncome) {
this.otherIncome = otherIncome;
}
public Credit1099 federalIncomeTaxWithheld(Double federalIncomeTaxWithheld) {
this.federalIncomeTaxWithheld = federalIncomeTaxWithheld;
return this;
}
/**
* Amount of federal income tax withheld from payer.
* @return federalIncomeTaxWithheld
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Amount of federal income tax withheld from payer.")
public Double getFederalIncomeTaxWithheld() {
return federalIncomeTaxWithheld;
}
public void setFederalIncomeTaxWithheld(Double federalIncomeTaxWithheld) {
this.federalIncomeTaxWithheld = federalIncomeTaxWithheld;
}
public Credit1099 fishingBoatProceeds(Double fishingBoatProceeds) {
this.fishingBoatProceeds = fishingBoatProceeds;
return this;
}
/**
* Amount of fishing boat proceeds from payer.
* @return fishingBoatProceeds
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Amount of fishing boat proceeds from payer.")
public Double getFishingBoatProceeds() {
return fishingBoatProceeds;
}
public void setFishingBoatProceeds(Double fishingBoatProceeds) {
this.fishingBoatProceeds = fishingBoatProceeds;
}
public Credit1099 medicalAndHealthcarePayments(Double medicalAndHealthcarePayments) {
this.medicalAndHealthcarePayments = medicalAndHealthcarePayments;
return this;
}
/**
* Amount of medical and healthcare payments from payer.
* @return medicalAndHealthcarePayments
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Amount of medical and healthcare payments from payer.")
public Double getMedicalAndHealthcarePayments() {
return medicalAndHealthcarePayments;
}
public void setMedicalAndHealthcarePayments(Double medicalAndHealthcarePayments) {
this.medicalAndHealthcarePayments = medicalAndHealthcarePayments;
}
public Credit1099 nonemployeeCompensation(Double nonemployeeCompensation) {
this.nonemployeeCompensation = nonemployeeCompensation;
return this;
}
/**
* Amount of nonemployee compensation from payer.
* @return nonemployeeCompensation
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Amount of nonemployee compensation from payer.")
public Double getNonemployeeCompensation() {
return nonemployeeCompensation;
}
public void setNonemployeeCompensation(Double nonemployeeCompensation) {
this.nonemployeeCompensation = nonemployeeCompensation;
}
public Credit1099 substitutePaymentsInLieuOfDividendsOrInterest(Double substitutePaymentsInLieuOfDividendsOrInterest) {
this.substitutePaymentsInLieuOfDividendsOrInterest = substitutePaymentsInLieuOfDividendsOrInterest;
return this;
}
/**
* Amount of substitute payments made by payer.
* @return substitutePaymentsInLieuOfDividendsOrInterest
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Amount of substitute payments made by payer.")
public Double getSubstitutePaymentsInLieuOfDividendsOrInterest() {
return substitutePaymentsInLieuOfDividendsOrInterest;
}
public void setSubstitutePaymentsInLieuOfDividendsOrInterest(Double substitutePaymentsInLieuOfDividendsOrInterest) {
this.substitutePaymentsInLieuOfDividendsOrInterest = substitutePaymentsInLieuOfDividendsOrInterest;
}
public Credit1099 payerMadeDirectSalesOf5000OrMoreOfConsumerProductsToBuyer(String payerMadeDirectSalesOf5000OrMoreOfConsumerProductsToBuyer) {
this.payerMadeDirectSalesOf5000OrMoreOfConsumerProductsToBuyer = payerMadeDirectSalesOf5000OrMoreOfConsumerProductsToBuyer;
return this;
}
/**
* Whether or not payer made direct sales over $5000 of consumer products.
* @return payerMadeDirectSalesOf5000OrMoreOfConsumerProductsToBuyer
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Whether or not payer made direct sales over $5000 of consumer products.")
public String getPayerMadeDirectSalesOf5000OrMoreOfConsumerProductsToBuyer() {
return payerMadeDirectSalesOf5000OrMoreOfConsumerProductsToBuyer;
}
public void setPayerMadeDirectSalesOf5000OrMoreOfConsumerProductsToBuyer(String payerMadeDirectSalesOf5000OrMoreOfConsumerProductsToBuyer) {
this.payerMadeDirectSalesOf5000OrMoreOfConsumerProductsToBuyer = payerMadeDirectSalesOf5000OrMoreOfConsumerProductsToBuyer;
}
public Credit1099 cropInsuranceProceeds(Double cropInsuranceProceeds) {
this.cropInsuranceProceeds = cropInsuranceProceeds;
return this;
}
/**
* Amount of crop insurance proceeds.
* @return cropInsuranceProceeds
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Amount of crop insurance proceeds.")
public Double getCropInsuranceProceeds() {
return cropInsuranceProceeds;
}
public void setCropInsuranceProceeds(Double cropInsuranceProceeds) {
this.cropInsuranceProceeds = cropInsuranceProceeds;
}
public Credit1099 excessGoldenParachutePayments(Double excessGoldenParachutePayments) {
this.excessGoldenParachutePayments = excessGoldenParachutePayments;
return this;
}
/**
* Amount of golden parachute payments made by payer.
* @return excessGoldenParachutePayments
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Amount of golden parachute payments made by payer.")
public Double getExcessGoldenParachutePayments() {
return excessGoldenParachutePayments;
}
public void setExcessGoldenParachutePayments(Double excessGoldenParachutePayments) {
this.excessGoldenParachutePayments = excessGoldenParachutePayments;
}
public Credit1099 grossProceedsPaidToAnAttorney(Double grossProceedsPaidToAnAttorney) {
this.grossProceedsPaidToAnAttorney = grossProceedsPaidToAnAttorney;
return this;
}
/**
* Amount of gross proceeds paid to an attorney by payer.
* @return grossProceedsPaidToAnAttorney
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Amount of gross proceeds paid to an attorney by payer.")
public Double getGrossProceedsPaidToAnAttorney() {
return grossProceedsPaidToAnAttorney;
}
public void setGrossProceedsPaidToAnAttorney(Double grossProceedsPaidToAnAttorney) {
this.grossProceedsPaidToAnAttorney = grossProceedsPaidToAnAttorney;
}
public Credit1099 section409aDeferrals(Double section409aDeferrals) {
this.section409aDeferrals = section409aDeferrals;
return this;
}
/**
* Amount of 409A deferrals earned by payer.
* @return section409aDeferrals
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Amount of 409A deferrals earned by payer.")
public Double getSection409aDeferrals() {
return section409aDeferrals;
}
public void setSection409aDeferrals(Double section409aDeferrals) {
this.section409aDeferrals = section409aDeferrals;
}
public Credit1099 section409aIncome(Double section409aIncome) {
this.section409aIncome = section409aIncome;
return this;
}
/**
* Amount of 409A income earned by payer.
* @return section409aIncome
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Amount of 409A income earned by payer.")
public Double getSection409aIncome() {
return section409aIncome;
}
public void setSection409aIncome(Double section409aIncome) {
this.section409aIncome = section409aIncome;
}
public Credit1099 stateTaxWithheld(Double stateTaxWithheld) {
this.stateTaxWithheld = stateTaxWithheld;
return this;
}
/**
* Amount of state tax withheld of payer for primary state.
* @return stateTaxWithheld
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Amount of state tax withheld of payer for primary state.")
public Double getStateTaxWithheld() {
return stateTaxWithheld;
}
public void setStateTaxWithheld(Double stateTaxWithheld) {
this.stateTaxWithheld = stateTaxWithheld;
}
public Credit1099 stateTaxWithheldLower(Double stateTaxWithheldLower) {
this.stateTaxWithheldLower = stateTaxWithheldLower;
return this;
}
/**
* Amount of state tax withheld of payer for secondary state.
* @return stateTaxWithheldLower
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Amount of state tax withheld of payer for secondary state.")
public Double getStateTaxWithheldLower() {
return stateTaxWithheldLower;
}
public void setStateTaxWithheldLower(Double stateTaxWithheldLower) {
this.stateTaxWithheldLower = stateTaxWithheldLower;
}
public Credit1099 payerStateNumber(String payerStateNumber) {
this.payerStateNumber = payerStateNumber;
return this;
}
/**
* Primary state ID.
* @return payerStateNumber
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Primary state ID.")
public String getPayerStateNumber() {
return payerStateNumber;
}
public void setPayerStateNumber(String payerStateNumber) {
this.payerStateNumber = payerStateNumber;
}
public Credit1099 payerStateNumberLower(String payerStateNumberLower) {
this.payerStateNumberLower = payerStateNumberLower;
return this;
}
/**
* Secondary state ID.
* @return payerStateNumberLower
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Secondary state ID.")
public String getPayerStateNumberLower() {
return payerStateNumberLower;
}
public void setPayerStateNumberLower(String payerStateNumberLower) {
this.payerStateNumberLower = payerStateNumberLower;
}
public Credit1099 stateIncome(Double stateIncome) {
this.stateIncome = stateIncome;
return this;
}
/**
* State income reported for primary state.
* @return stateIncome
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "State income reported for primary state.")
public Double getStateIncome() {
return stateIncome;
}
public void setStateIncome(Double stateIncome) {
this.stateIncome = stateIncome;
}
public Credit1099 stateIncomeLower(Double stateIncomeLower) {
this.stateIncomeLower = stateIncomeLower;
return this;
}
/**
* State income reported for secondary state.
* @return stateIncomeLower
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "State income reported for secondary state.")
public Double getStateIncomeLower() {
return stateIncomeLower;
}
public void setStateIncomeLower(Double stateIncomeLower) {
this.stateIncomeLower = stateIncomeLower;
}
public Credit1099 transactionsReported(String transactionsReported) {
this.transactionsReported = transactionsReported;
return this;
}
/**
* One of the values will be provided Payment card Third party network
* @return transactionsReported
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "One of the values will be provided Payment card Third party network")
public String getTransactionsReported() {
return transactionsReported;
}
public void setTransactionsReported(String transactionsReported) {
this.transactionsReported = transactionsReported;
}
public Credit1099 pseName(String pseName) {
this.pseName = pseName;
return this;
}
/**
* Name of the PSE (Payment Settlement Entity).
* @return pseName
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Name of the PSE (Payment Settlement Entity).")
public String getPseName() {
return pseName;
}
public void setPseName(String pseName) {
this.pseName = pseName;
}
public Credit1099 pseTelephoneNumber(String pseTelephoneNumber) {
this.pseTelephoneNumber = pseTelephoneNumber;
return this;
}
/**
* Formatted (XXX) XXX-XXXX. Phone number of the PSE (Payment Settlement Entity).
* @return pseTelephoneNumber
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Formatted (XXX) XXX-XXXX. Phone number of the PSE (Payment Settlement Entity).")
public String getPseTelephoneNumber() {
return pseTelephoneNumber;
}
public void setPseTelephoneNumber(String pseTelephoneNumber) {
this.pseTelephoneNumber = pseTelephoneNumber;
}
public Credit1099 grossAmount(Double grossAmount) {
this.grossAmount = grossAmount;
return this;
}
/**
* Gross amount reported.
* @return grossAmount
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Gross amount reported.")
public Double getGrossAmount() {
return grossAmount;
}
public void setGrossAmount(Double grossAmount) {
this.grossAmount = grossAmount;
}
public Credit1099 cardNotPresentTransaction(Double cardNotPresentTransaction) {
this.cardNotPresentTransaction = cardNotPresentTransaction;
return this;
}
/**
* Amount in card not present transactions.
* @return cardNotPresentTransaction
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Amount in card not present transactions.")
public Double getCardNotPresentTransaction() {
return cardNotPresentTransaction;
}
public void setCardNotPresentTransaction(Double cardNotPresentTransaction) {
this.cardNotPresentTransaction = cardNotPresentTransaction;
}
public Credit1099 merchantCategoryCode(String merchantCategoryCode) {
this.merchantCategoryCode = merchantCategoryCode;
return this;
}
/**
* Merchant category of filer.
* @return merchantCategoryCode
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Merchant category of filer.")
public String getMerchantCategoryCode() {
return merchantCategoryCode;
}
public void setMerchantCategoryCode(String merchantCategoryCode) {
this.merchantCategoryCode = merchantCategoryCode;
}
public Credit1099 numberOfPaymentTransactions(String numberOfPaymentTransactions) {
this.numberOfPaymentTransactions = numberOfPaymentTransactions;
return this;
}
/**
* Number of payment transactions made.
* @return numberOfPaymentTransactions
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Number of payment transactions made.")
public String getNumberOfPaymentTransactions() {
return numberOfPaymentTransactions;
}
public void setNumberOfPaymentTransactions(String numberOfPaymentTransactions) {
this.numberOfPaymentTransactions = numberOfPaymentTransactions;
}
public Credit1099 januaryAmount(Double januaryAmount) {
this.januaryAmount = januaryAmount;
return this;
}
/**
* Amount reported for January.
* @return januaryAmount
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Amount reported for January.")
public Double getJanuaryAmount() {
return januaryAmount;
}
public void setJanuaryAmount(Double januaryAmount) {
this.januaryAmount = januaryAmount;
}
public Credit1099 februaryAmount(Double februaryAmount) {
this.februaryAmount = februaryAmount;
return this;
}
/**
* Amount reported for February.
* @return februaryAmount
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Amount reported for February.")
public Double getFebruaryAmount() {
return februaryAmount;
}
public void setFebruaryAmount(Double februaryAmount) {
this.februaryAmount = februaryAmount;
}
public Credit1099 marchAmount(Double marchAmount) {
this.marchAmount = marchAmount;
return this;
}
/**
* Amount reported for March.
* @return marchAmount
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Amount reported for March.")
public Double getMarchAmount() {
return marchAmount;
}
public void setMarchAmount(Double marchAmount) {
this.marchAmount = marchAmount;
}
public Credit1099 aprilAmount(Double aprilAmount) {
this.aprilAmount = aprilAmount;
return this;
}
/**
* Amount reported for April.
* @return aprilAmount
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Amount reported for April.")
public Double getAprilAmount() {
return aprilAmount;
}
public void setAprilAmount(Double aprilAmount) {
this.aprilAmount = aprilAmount;
}
public Credit1099 mayAmount(Double mayAmount) {
this.mayAmount = mayAmount;
return this;
}
/**
* Amount reported for May.
* @return mayAmount
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Amount reported for May.")
public Double getMayAmount() {
return mayAmount;
}
public void setMayAmount(Double mayAmount) {
this.mayAmount = mayAmount;
}
public Credit1099 juneAmount(Double juneAmount) {
this.juneAmount = juneAmount;
return this;
}
/**
* Amount reported for June.
* @return juneAmount
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Amount reported for June.")
public Double getJuneAmount() {
return juneAmount;
}
public void setJuneAmount(Double juneAmount) {
this.juneAmount = juneAmount;
}
public Credit1099 julyAmount(Double julyAmount) {
this.julyAmount = julyAmount;
return this;
}
/**
* Amount reported for July.
* @return julyAmount
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Amount reported for July.")
public Double getJulyAmount() {
return julyAmount;
}
public void setJulyAmount(Double julyAmount) {
this.julyAmount = julyAmount;
}
public Credit1099 augustAmount(Double augustAmount) {
this.augustAmount = augustAmount;
return this;
}
/**
* Amount reported for August.
* @return augustAmount
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Amount reported for August.")
public Double getAugustAmount() {
return augustAmount;
}
public void setAugustAmount(Double augustAmount) {
this.augustAmount = augustAmount;
}
public Credit1099 septemberAmount(Double septemberAmount) {
this.septemberAmount = septemberAmount;
return this;
}
/**
* Amount reported for September.
* @return septemberAmount
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Amount reported for September.")
public Double getSeptemberAmount() {
return septemberAmount;
}
public void setSeptemberAmount(Double septemberAmount) {
this.septemberAmount = septemberAmount;
}
public Credit1099 octoberAmount(Double octoberAmount) {
this.octoberAmount = octoberAmount;
return this;
}
/**
* Amount reported for October.
* @return octoberAmount
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Amount reported for October.")
public Double getOctoberAmount() {
return octoberAmount;
}
public void setOctoberAmount(Double octoberAmount) {
this.octoberAmount = octoberAmount;
}
public Credit1099 novemberAmount(Double novemberAmount) {
this.novemberAmount = novemberAmount;
return this;
}
/**
* Amount reported for November.
* @return novemberAmount
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Amount reported for November.")
public Double getNovemberAmount() {
return novemberAmount;
}
public void setNovemberAmount(Double novemberAmount) {
this.novemberAmount = novemberAmount;
}
public Credit1099 decemberAmount(Double decemberAmount) {
this.decemberAmount = decemberAmount;
return this;
}
/**
* Amount reported for December.
* @return decemberAmount
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Amount reported for December.")
public Double getDecemberAmount() {
return decemberAmount;
}
public void setDecemberAmount(Double decemberAmount) {
this.decemberAmount = decemberAmount;
}
public Credit1099 primaryState(String primaryState) {
this.primaryState = primaryState;
return this;
}
/**
* Primary state of business.
* @return primaryState
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Primary state of business.")
public String getPrimaryState() {
return primaryState;
}
public void setPrimaryState(String primaryState) {
this.primaryState = primaryState;
}
public Credit1099 secondaryState(String secondaryState) {
this.secondaryState = secondaryState;
return this;
}
/**
* Secondary state of business.
* @return secondaryState
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Secondary state of business.")
public String getSecondaryState() {
return secondaryState;
}
public void setSecondaryState(String secondaryState) {
this.secondaryState = secondaryState;
}
public Credit1099 primaryStateId(String primaryStateId) {
this.primaryStateId = primaryStateId;
return this;
}
/**
* Primary state ID.
* @return primaryStateId
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Primary state ID.")
public String getPrimaryStateId() {
return primaryStateId;
}
public void setPrimaryStateId(String primaryStateId) {
this.primaryStateId = primaryStateId;
}
public Credit1099 secondaryStateId(String secondaryStateId) {
this.secondaryStateId = secondaryStateId;
return this;
}
/**
* Secondary state ID.
* @return secondaryStateId
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Secondary state ID.")
public String getSecondaryStateId() {
return secondaryStateId;
}
public void setSecondaryStateId(String secondaryStateId) {
this.secondaryStateId = secondaryStateId;
}
public Credit1099 primaryStateIncomeTax(Double primaryStateIncomeTax) {
this.primaryStateIncomeTax = primaryStateIncomeTax;
return this;
}
/**
* State income tax reported for primary state.
* @return primaryStateIncomeTax
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "State income tax reported for primary state.")
public Double getPrimaryStateIncomeTax() {
return primaryStateIncomeTax;
}
public void setPrimaryStateIncomeTax(Double primaryStateIncomeTax) {
this.primaryStateIncomeTax = primaryStateIncomeTax;
}
public Credit1099 secondaryStateIncomeTax(Double secondaryStateIncomeTax) {
this.secondaryStateIncomeTax = secondaryStateIncomeTax;
return this;
}
/**
* State income tax reported for secondary state.
* @return secondaryStateIncomeTax
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "State income tax reported for secondary state.")
public Double getSecondaryStateIncomeTax() {
return secondaryStateIncomeTax;
}
public void setSecondaryStateIncomeTax(Double secondaryStateIncomeTax) {
this.secondaryStateIncomeTax = secondaryStateIncomeTax;
}
@Override
public boolean equals(Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
Credit1099 credit1099 = (Credit1099) o;
return Objects.equals(this.documentId, credit1099.documentId) &&
Objects.equals(this.documentMetadata, credit1099.documentMetadata) &&
Objects.equals(this.form1099Type, credit1099.form1099Type) &&
Objects.equals(this.recipient, credit1099.recipient) &&
Objects.equals(this.payer, credit1099.payer) &&
Objects.equals(this.filer, credit1099.filer) &&
Objects.equals(this.taxYear, credit1099.taxYear) &&
Objects.equals(this.rents, credit1099.rents) &&
Objects.equals(this.royalties, credit1099.royalties) &&
Objects.equals(this.otherIncome, credit1099.otherIncome) &&
Objects.equals(this.federalIncomeTaxWithheld, credit1099.federalIncomeTaxWithheld) &&
Objects.equals(this.fishingBoatProceeds, credit1099.fishingBoatProceeds) &&
Objects.equals(this.medicalAndHealthcarePayments, credit1099.medicalAndHealthcarePayments) &&
Objects.equals(this.nonemployeeCompensation, credit1099.nonemployeeCompensation) &&
Objects.equals(this.substitutePaymentsInLieuOfDividendsOrInterest, credit1099.substitutePaymentsInLieuOfDividendsOrInterest) &&
Objects.equals(this.payerMadeDirectSalesOf5000OrMoreOfConsumerProductsToBuyer, credit1099.payerMadeDirectSalesOf5000OrMoreOfConsumerProductsToBuyer) &&
Objects.equals(this.cropInsuranceProceeds, credit1099.cropInsuranceProceeds) &&
Objects.equals(this.excessGoldenParachutePayments, credit1099.excessGoldenParachutePayments) &&
Objects.equals(this.grossProceedsPaidToAnAttorney, credit1099.grossProceedsPaidToAnAttorney) &&
Objects.equals(this.section409aDeferrals, credit1099.section409aDeferrals) &&
Objects.equals(this.section409aIncome, credit1099.section409aIncome) &&
Objects.equals(this.stateTaxWithheld, credit1099.stateTaxWithheld) &&
Objects.equals(this.stateTaxWithheldLower, credit1099.stateTaxWithheldLower) &&
Objects.equals(this.payerStateNumber, credit1099.payerStateNumber) &&
Objects.equals(this.payerStateNumberLower, credit1099.payerStateNumberLower) &&
Objects.equals(this.stateIncome, credit1099.stateIncome) &&
Objects.equals(this.stateIncomeLower, credit1099.stateIncomeLower) &&
Objects.equals(this.transactionsReported, credit1099.transactionsReported) &&
Objects.equals(this.pseName, credit1099.pseName) &&
Objects.equals(this.pseTelephoneNumber, credit1099.pseTelephoneNumber) &&
Objects.equals(this.grossAmount, credit1099.grossAmount) &&
Objects.equals(this.cardNotPresentTransaction, credit1099.cardNotPresentTransaction) &&
Objects.equals(this.merchantCategoryCode, credit1099.merchantCategoryCode) &&
Objects.equals(this.numberOfPaymentTransactions, credit1099.numberOfPaymentTransactions) &&
Objects.equals(this.januaryAmount, credit1099.januaryAmount) &&
Objects.equals(this.februaryAmount, credit1099.februaryAmount) &&
Objects.equals(this.marchAmount, credit1099.marchAmount) &&
Objects.equals(this.aprilAmount, credit1099.aprilAmount) &&
Objects.equals(this.mayAmount, credit1099.mayAmount) &&
Objects.equals(this.juneAmount, credit1099.juneAmount) &&
Objects.equals(this.julyAmount, credit1099.julyAmount) &&
Objects.equals(this.augustAmount, credit1099.augustAmount) &&
Objects.equals(this.septemberAmount, credit1099.septemberAmount) &&
Objects.equals(this.octoberAmount, credit1099.octoberAmount) &&
Objects.equals(this.novemberAmount, credit1099.novemberAmount) &&
Objects.equals(this.decemberAmount, credit1099.decemberAmount) &&
Objects.equals(this.primaryState, credit1099.primaryState) &&
Objects.equals(this.secondaryState, credit1099.secondaryState) &&
Objects.equals(this.primaryStateId, credit1099.primaryStateId) &&
Objects.equals(this.secondaryStateId, credit1099.secondaryStateId) &&
Objects.equals(this.primaryStateIncomeTax, credit1099.primaryStateIncomeTax) &&
Objects.equals(this.secondaryStateIncomeTax, credit1099.secondaryStateIncomeTax);
}
@Override
public int hashCode() {
return Objects.hash(documentId, documentMetadata, form1099Type, recipient, payer, filer, taxYear, rents, royalties, otherIncome, federalIncomeTaxWithheld, fishingBoatProceeds, medicalAndHealthcarePayments, nonemployeeCompensation, substitutePaymentsInLieuOfDividendsOrInterest, payerMadeDirectSalesOf5000OrMoreOfConsumerProductsToBuyer, cropInsuranceProceeds, excessGoldenParachutePayments, grossProceedsPaidToAnAttorney, section409aDeferrals, section409aIncome, stateTaxWithheld, stateTaxWithheldLower, payerStateNumber, payerStateNumberLower, stateIncome, stateIncomeLower, transactionsReported, pseName, pseTelephoneNumber, grossAmount, cardNotPresentTransaction, merchantCategoryCode, numberOfPaymentTransactions, januaryAmount, februaryAmount, marchAmount, aprilAmount, mayAmount, juneAmount, julyAmount, augustAmount, septemberAmount, octoberAmount, novemberAmount, decemberAmount, primaryState, secondaryState, primaryStateId, secondaryStateId, primaryStateIncomeTax, secondaryStateIncomeTax);
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class Credit1099 {\n");
sb.append(" documentId: ").append(toIndentedString(documentId)).append("\n");
sb.append(" documentMetadata: ").append(toIndentedString(documentMetadata)).append("\n");
sb.append(" form1099Type: ").append(toIndentedString(form1099Type)).append("\n");
sb.append(" recipient: ").append(toIndentedString(recipient)).append("\n");
sb.append(" payer: ").append(toIndentedString(payer)).append("\n");
sb.append(" filer: ").append(toIndentedString(filer)).append("\n");
sb.append(" taxYear: ").append(toIndentedString(taxYear)).append("\n");
sb.append(" rents: ").append(toIndentedString(rents)).append("\n");
sb.append(" royalties: ").append(toIndentedString(royalties)).append("\n");
sb.append(" otherIncome: ").append(toIndentedString(otherIncome)).append("\n");
sb.append(" federalIncomeTaxWithheld: ").append(toIndentedString(federalIncomeTaxWithheld)).append("\n");
sb.append(" fishingBoatProceeds: ").append(toIndentedString(fishingBoatProceeds)).append("\n");
sb.append(" medicalAndHealthcarePayments: ").append(toIndentedString(medicalAndHealthcarePayments)).append("\n");
sb.append(" nonemployeeCompensation: ").append(toIndentedString(nonemployeeCompensation)).append("\n");
sb.append(" substitutePaymentsInLieuOfDividendsOrInterest: ").append(toIndentedString(substitutePaymentsInLieuOfDividendsOrInterest)).append("\n");
sb.append(" payerMadeDirectSalesOf5000OrMoreOfConsumerProductsToBuyer: ").append(toIndentedString(payerMadeDirectSalesOf5000OrMoreOfConsumerProductsToBuyer)).append("\n");
sb.append(" cropInsuranceProceeds: ").append(toIndentedString(cropInsuranceProceeds)).append("\n");
sb.append(" excessGoldenParachutePayments: ").append(toIndentedString(excessGoldenParachutePayments)).append("\n");
sb.append(" grossProceedsPaidToAnAttorney: ").append(toIndentedString(grossProceedsPaidToAnAttorney)).append("\n");
sb.append(" section409aDeferrals: ").append(toIndentedString(section409aDeferrals)).append("\n");
sb.append(" section409aIncome: ").append(toIndentedString(section409aIncome)).append("\n");
sb.append(" stateTaxWithheld: ").append(toIndentedString(stateTaxWithheld)).append("\n");
sb.append(" stateTaxWithheldLower: ").append(toIndentedString(stateTaxWithheldLower)).append("\n");
sb.append(" payerStateNumber: ").append(toIndentedString(payerStateNumber)).append("\n");
sb.append(" payerStateNumberLower: ").append(toIndentedString(payerStateNumberLower)).append("\n");
sb.append(" stateIncome: ").append(toIndentedString(stateIncome)).append("\n");
sb.append(" stateIncomeLower: ").append(toIndentedString(stateIncomeLower)).append("\n");
sb.append(" transactionsReported: ").append(toIndentedString(transactionsReported)).append("\n");
sb.append(" pseName: ").append(toIndentedString(pseName)).append("\n");
sb.append(" pseTelephoneNumber: ").append(toIndentedString(pseTelephoneNumber)).append("\n");
sb.append(" grossAmount: ").append(toIndentedString(grossAmount)).append("\n");
sb.append(" cardNotPresentTransaction: ").append(toIndentedString(cardNotPresentTransaction)).append("\n");
sb.append(" merchantCategoryCode: ").append(toIndentedString(merchantCategoryCode)).append("\n");
sb.append(" numberOfPaymentTransactions: ").append(toIndentedString(numberOfPaymentTransactions)).append("\n");
sb.append(" januaryAmount: ").append(toIndentedString(januaryAmount)).append("\n");
sb.append(" februaryAmount: ").append(toIndentedString(februaryAmount)).append("\n");
sb.append(" marchAmount: ").append(toIndentedString(marchAmount)).append("\n");
sb.append(" aprilAmount: ").append(toIndentedString(aprilAmount)).append("\n");
sb.append(" mayAmount: ").append(toIndentedString(mayAmount)).append("\n");
sb.append(" juneAmount: ").append(toIndentedString(juneAmount)).append("\n");
sb.append(" julyAmount: ").append(toIndentedString(julyAmount)).append("\n");
sb.append(" augustAmount: ").append(toIndentedString(augustAmount)).append("\n");
sb.append(" septemberAmount: ").append(toIndentedString(septemberAmount)).append("\n");
sb.append(" octoberAmount: ").append(toIndentedString(octoberAmount)).append("\n");
sb.append(" novemberAmount: ").append(toIndentedString(novemberAmount)).append("\n");
sb.append(" decemberAmount: ").append(toIndentedString(decemberAmount)).append("\n");
sb.append(" primaryState: ").append(toIndentedString(primaryState)).append("\n");
sb.append(" secondaryState: ").append(toIndentedString(secondaryState)).append("\n");
sb.append(" primaryStateId: ").append(toIndentedString(primaryStateId)).append("\n");
sb.append(" secondaryStateId: ").append(toIndentedString(secondaryStateId)).append("\n");
sb.append(" primaryStateIncomeTax: ").append(toIndentedString(primaryStateIncomeTax)).append("\n");
sb.append(" secondaryStateIncomeTax: ").append(toIndentedString(secondaryStateIncomeTax)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces
* (except the first line).
*/
private String toIndentedString(Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy