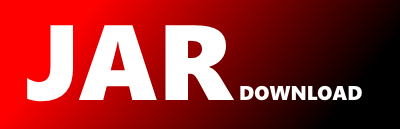
com.plaid.client.model.IndividualWatchlistCode Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of plaid-java Show documentation
Show all versions of plaid-java Show documentation
Bindings for the Plaid (plaid.com) API.
/*
* The Plaid API
* The Plaid REST API. Please see https://plaid.com/docs/api for more details.
*
* The version of the OpenAPI document: 2020-09-14_1.503.5
*
*
* NOTE: This class is auto generated by OpenAPI Generator (https://openapi-generator.tech).
* https://openapi-generator.tech
* Do not edit the class manually.
*/
package com.plaid.client.model;
import java.util.Objects;
import java.util.Arrays;
import io.swagger.annotations.ApiModel;
import com.google.gson.annotations.SerializedName;
import java.io.IOException;
import com.google.gson.TypeAdapter;
import com.google.gson.annotations.JsonAdapter;
import com.google.gson.stream.JsonReader;
import com.google.gson.stream.JsonWriter;
/**
* Shorthand identifier for a specific screening list for individuals. `AU_CON`: Australia Department of Foreign Affairs and Trade Consolidated List `CA_CON`: Government of Canada Consolidated List of Sanctions `EU_CON`: European External Action Service Consolidated List `IZ_CIA`: CIA List of Chiefs of State and Cabinet Members `IZ_IPL`: Interpol Red Notices for Wanted Persons List `IZ_PEP`: Politically Exposed Persons List `IZ_UNC`: United Nations Consolidated Sanctions `IZ_WBK`: World Bank Listing of Ineligible Firms and Individuals `UK_HMC`: UK HM Treasury Consolidated List `US_DPL`: Bureau of Industry and Security Denied Persons List `US_DTC`: US Department of State AECA Debarred `US_FBI`: US Department of Justice FBI Wanted List `US_FSE`: US OFAC Foreign Sanctions Evaders `US_ISN`: US Department of State Nonproliferation Sanctions `US_PLC`: US OFAC Palestinian Legislative Council `US_SDN`: US OFAC Specially Designated Nationals List `US_SSI`: US OFAC Sectoral Sanctions Identifications `SG_SOF`: Government of Singapore Terrorists and Terrorist Entities `TR_TWL`: Government of Turkey Terrorist Wanted List `TR_DFD`: Government of Turkey Domestic Freezing Decisions `TR_FOR`: Government of Turkey Foreign Freezing Requests `TR_WMD`: Government of Turkey Weapons of Mass Destruction `TR_CMB`: Government of Turkey Capital Markets Board
*/
@JsonAdapter(IndividualWatchlistCode.Adapter.class)
public enum IndividualWatchlistCode {
AU_CON("AU_CON"),
CA_CON("CA_CON"),
EU_CON("EU_CON"),
IZ_CIA("IZ_CIA"),
IZ_IPL("IZ_IPL"),
IZ_PEP("IZ_PEP"),
IZ_UNC("IZ_UNC"),
IZ_WBK("IZ_WBK"),
UK_HMC("UK_HMC"),
US_DPL("US_DPL"),
US_DTC("US_DTC"),
US_FBI("US_FBI"),
US_FSE("US_FSE"),
US_ISN("US_ISN"),
US_MBS("US_MBS"),
US_PLC("US_PLC"),
US_SDN("US_SDN"),
US_SSI("US_SSI"),
SG_SOF("SG_SOF"),
TR_TWL("TR_TWL"),
TR_DFD("TR_DFD"),
TR_FOR("TR_FOR"),
TR_WMD("TR_WMD"),
TR_CMB("TR_CMB"),
// This is returned when an enum is returned from the API that doesn't exist in the OpenAPI file.
// Try upgrading your client-library version.
ENUM_UNKNOWN("ENUM_UNKNOWN");
private String value;
IndividualWatchlistCode(String value) {
this.value = value;
}
public String getValue() {
return value;
}
@Override
public String toString() {
return String.valueOf(value);
}
public static IndividualWatchlistCode fromValue(String value) {
for (IndividualWatchlistCode b : IndividualWatchlistCode.values()) {
if (b.value.equals(value)) {
return b;
}
}
return IndividualWatchlistCode.ENUM_UNKNOWN;
}
public static class Adapter extends TypeAdapter {
@Override
public void write(final JsonWriter jsonWriter, final IndividualWatchlistCode enumeration) throws IOException {
jsonWriter.value(enumeration.getValue());
}
@Override
public IndividualWatchlistCode read(final JsonReader jsonReader) throws IOException {
String value = jsonReader.nextString();
return IndividualWatchlistCode.fromValue(value);
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy