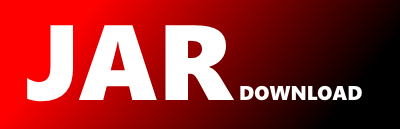
com.plaid.client.model.ItemApplicationScopesUpdateRequest Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of plaid-java Show documentation
Show all versions of plaid-java Show documentation
Bindings for the Plaid (plaid.com) API.
/*
* The Plaid API
* The Plaid REST API. Please see https://plaid.com/docs/api for more details.
*
* The version of the OpenAPI document: 2020-09-14_1.503.5
*
*
* NOTE: This class is auto generated by OpenAPI Generator (https://openapi-generator.tech).
* https://openapi-generator.tech
* Do not edit the class manually.
*/
package com.plaid.client.model;
import java.util.Objects;
import java.util.Arrays;
import com.google.gson.TypeAdapter;
import com.google.gson.annotations.JsonAdapter;
import com.google.gson.annotations.SerializedName;
import com.google.gson.stream.JsonReader;
import com.google.gson.stream.JsonWriter;
import com.plaid.client.model.Scopes;
import com.plaid.client.model.ScopesContext;
import io.swagger.annotations.ApiModel;
import io.swagger.annotations.ApiModelProperty;
import java.io.IOException;
/**
* ItemApplicationScopesUpdateRequest defines the request schema for `/item/application/scopes/update`
*/
@ApiModel(description = "ItemApplicationScopesUpdateRequest defines the request schema for `/item/application/scopes/update`")
@javax.annotation.Generated(value = "org.openapitools.codegen.languages.JavaClientCodegen", date = "2024-03-28T21:00:55.745394Z[Etc/UTC]")
public class ItemApplicationScopesUpdateRequest {
public static final String SERIALIZED_NAME_CLIENT_ID = "client_id";
@SerializedName(SERIALIZED_NAME_CLIENT_ID)
private String clientId;
public static final String SERIALIZED_NAME_SECRET = "secret";
@SerializedName(SERIALIZED_NAME_SECRET)
private String secret;
public static final String SERIALIZED_NAME_ACCESS_TOKEN = "access_token";
@SerializedName(SERIALIZED_NAME_ACCESS_TOKEN)
private String accessToken;
public static final String SERIALIZED_NAME_APPLICATION_ID = "application_id";
@SerializedName(SERIALIZED_NAME_APPLICATION_ID)
private String applicationId;
public static final String SERIALIZED_NAME_SCOPES = "scopes";
@SerializedName(SERIALIZED_NAME_SCOPES)
private Scopes scopes;
public static final String SERIALIZED_NAME_STATE = "state";
@SerializedName(SERIALIZED_NAME_STATE)
private String state;
public static final String SERIALIZED_NAME_CONTEXT = "context";
@SerializedName(SERIALIZED_NAME_CONTEXT)
private ScopesContext context;
public ItemApplicationScopesUpdateRequest clientId(String clientId) {
this.clientId = clientId;
return this;
}
/**
* Your Plaid API `client_id`. The `client_id` is required and may be provided either in the `PLAID-CLIENT-ID` header or as part of a request body.
* @return clientId
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Your Plaid API `client_id`. The `client_id` is required and may be provided either in the `PLAID-CLIENT-ID` header or as part of a request body.")
public String getClientId() {
return clientId;
}
public void setClientId(String clientId) {
this.clientId = clientId;
}
public ItemApplicationScopesUpdateRequest secret(String secret) {
this.secret = secret;
return this;
}
/**
* Your Plaid API `secret`. The `secret` is required and may be provided either in the `PLAID-SECRET` header or as part of a request body.
* @return secret
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Your Plaid API `secret`. The `secret` is required and may be provided either in the `PLAID-SECRET` header or as part of a request body.")
public String getSecret() {
return secret;
}
public void setSecret(String secret) {
this.secret = secret;
}
public ItemApplicationScopesUpdateRequest accessToken(String accessToken) {
this.accessToken = accessToken;
return this;
}
/**
* The access token associated with the Item data is being requested for.
* @return accessToken
**/
@ApiModelProperty(required = true, value = "The access token associated with the Item data is being requested for.")
public String getAccessToken() {
return accessToken;
}
public void setAccessToken(String accessToken) {
this.accessToken = accessToken;
}
public ItemApplicationScopesUpdateRequest applicationId(String applicationId) {
this.applicationId = applicationId;
return this;
}
/**
* This field will map to the application ID that is returned from /item/application/list, or provided to the institution in an oauth redirect.
* @return applicationId
**/
@ApiModelProperty(required = true, value = "This field will map to the application ID that is returned from /item/application/list, or provided to the institution in an oauth redirect.")
public String getApplicationId() {
return applicationId;
}
public void setApplicationId(String applicationId) {
this.applicationId = applicationId;
}
public ItemApplicationScopesUpdateRequest scopes(Scopes scopes) {
this.scopes = scopes;
return this;
}
/**
* Get scopes
* @return scopes
**/
@ApiModelProperty(required = true, value = "")
public Scopes getScopes() {
return scopes;
}
public void setScopes(Scopes scopes) {
this.scopes = scopes;
}
public ItemApplicationScopesUpdateRequest state(String state) {
this.state = state;
return this;
}
/**
* When scopes are updated during enrollment, this field must be populated with the state sent to the partner in the OAuth Login URI. This field is required when the context is `ENROLLMENT`.
* @return state
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "When scopes are updated during enrollment, this field must be populated with the state sent to the partner in the OAuth Login URI. This field is required when the context is `ENROLLMENT`.")
public String getState() {
return state;
}
public void setState(String state) {
this.state = state;
}
public ItemApplicationScopesUpdateRequest context(ScopesContext context) {
this.context = context;
return this;
}
/**
* Get context
* @return context
**/
@ApiModelProperty(required = true, value = "")
public ScopesContext getContext() {
return context;
}
public void setContext(ScopesContext context) {
this.context = context;
}
@Override
public boolean equals(Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
ItemApplicationScopesUpdateRequest itemApplicationScopesUpdateRequest = (ItemApplicationScopesUpdateRequest) o;
return Objects.equals(this.clientId, itemApplicationScopesUpdateRequest.clientId) &&
Objects.equals(this.secret, itemApplicationScopesUpdateRequest.secret) &&
Objects.equals(this.accessToken, itemApplicationScopesUpdateRequest.accessToken) &&
Objects.equals(this.applicationId, itemApplicationScopesUpdateRequest.applicationId) &&
Objects.equals(this.scopes, itemApplicationScopesUpdateRequest.scopes) &&
Objects.equals(this.state, itemApplicationScopesUpdateRequest.state) &&
Objects.equals(this.context, itemApplicationScopesUpdateRequest.context);
}
@Override
public int hashCode() {
return Objects.hash(clientId, secret, accessToken, applicationId, scopes, state, context);
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class ItemApplicationScopesUpdateRequest {\n");
sb.append(" clientId: ").append(toIndentedString(clientId)).append("\n");
sb.append(" secret: ").append(toIndentedString(secret)).append("\n");
sb.append(" accessToken: ").append(toIndentedString(accessToken)).append("\n");
sb.append(" applicationId: ").append(toIndentedString(applicationId)).append("\n");
sb.append(" scopes: ").append(toIndentedString(scopes)).append("\n");
sb.append(" state: ").append(toIndentedString(state)).append("\n");
sb.append(" context: ").append(toIndentedString(context)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces
* (except the first line).
*/
private String toIndentedString(Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy