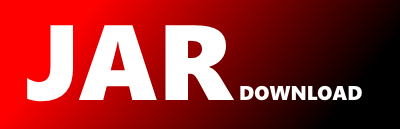
com.plaid.client.model.Transaction Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of plaid-java Show documentation
Show all versions of plaid-java Show documentation
Bindings for the Plaid (plaid.com) API.
/*
* The Plaid API
* The Plaid REST API. Please see https://plaid.com/docs/api for more details.
*
* The version of the OpenAPI document: 2020-09-14_1.503.5
*
*
* NOTE: This class is auto generated by OpenAPI Generator (https://openapi-generator.tech).
* https://openapi-generator.tech
* Do not edit the class manually.
*/
package com.plaid.client.model;
import java.util.Objects;
import java.util.Arrays;
import com.google.gson.TypeAdapter;
import com.google.gson.annotations.JsonAdapter;
import com.google.gson.annotations.SerializedName;
import com.google.gson.stream.JsonReader;
import com.google.gson.stream.JsonWriter;
import com.plaid.client.model.Location;
import com.plaid.client.model.PaymentMeta;
import com.plaid.client.model.PersonalFinanceCategory;
import com.plaid.client.model.TransactionAllOf;
import com.plaid.client.model.TransactionBase;
import com.plaid.client.model.TransactionCode;
import com.plaid.client.model.TransactionCounterparty;
import io.swagger.annotations.ApiModel;
import io.swagger.annotations.ApiModelProperty;
import java.io.IOException;
import java.time.LocalDate;
import java.time.OffsetDateTime;
import java.util.ArrayList;
import java.util.List;
/**
* A representation of a transaction
*/
@ApiModel(description = "A representation of a transaction")
@javax.annotation.Generated(value = "org.openapitools.codegen.languages.JavaClientCodegen", date = "2024-03-28T21:00:55.745394Z[Etc/UTC]")
public class Transaction {
public static final String SERIALIZED_NAME_ACCOUNT_ID = "account_id";
@SerializedName(SERIALIZED_NAME_ACCOUNT_ID)
private String accountId;
public static final String SERIALIZED_NAME_AMOUNT = "amount";
@SerializedName(SERIALIZED_NAME_AMOUNT)
private Double amount;
public static final String SERIALIZED_NAME_ISO_CURRENCY_CODE = "iso_currency_code";
@SerializedName(SERIALIZED_NAME_ISO_CURRENCY_CODE)
private String isoCurrencyCode;
public static final String SERIALIZED_NAME_UNOFFICIAL_CURRENCY_CODE = "unofficial_currency_code";
@SerializedName(SERIALIZED_NAME_UNOFFICIAL_CURRENCY_CODE)
private String unofficialCurrencyCode;
public static final String SERIALIZED_NAME_CATEGORY = "category";
@SerializedName(SERIALIZED_NAME_CATEGORY)
private List category = new ArrayList<>();
public static final String SERIALIZED_NAME_CATEGORY_ID = "category_id";
@SerializedName(SERIALIZED_NAME_CATEGORY_ID)
private String categoryId;
public static final String SERIALIZED_NAME_CHECK_NUMBER = "check_number";
@SerializedName(SERIALIZED_NAME_CHECK_NUMBER)
private String checkNumber;
public static final String SERIALIZED_NAME_DATE = "date";
@SerializedName(SERIALIZED_NAME_DATE)
private LocalDate date;
public static final String SERIALIZED_NAME_LOCATION = "location";
@SerializedName(SERIALIZED_NAME_LOCATION)
private Location location;
public static final String SERIALIZED_NAME_NAME = "name";
@SerializedName(SERIALIZED_NAME_NAME)
private String name;
public static final String SERIALIZED_NAME_MERCHANT_NAME = "merchant_name";
@SerializedName(SERIALIZED_NAME_MERCHANT_NAME)
private String merchantName;
public static final String SERIALIZED_NAME_ORIGINAL_DESCRIPTION = "original_description";
@SerializedName(SERIALIZED_NAME_ORIGINAL_DESCRIPTION)
private String originalDescription;
public static final String SERIALIZED_NAME_PAYMENT_META = "payment_meta";
@SerializedName(SERIALIZED_NAME_PAYMENT_META)
private PaymentMeta paymentMeta;
public static final String SERIALIZED_NAME_PENDING = "pending";
@SerializedName(SERIALIZED_NAME_PENDING)
private Boolean pending;
public static final String SERIALIZED_NAME_PENDING_TRANSACTION_ID = "pending_transaction_id";
@SerializedName(SERIALIZED_NAME_PENDING_TRANSACTION_ID)
private String pendingTransactionId;
public static final String SERIALIZED_NAME_ACCOUNT_OWNER = "account_owner";
@SerializedName(SERIALIZED_NAME_ACCOUNT_OWNER)
private String accountOwner;
public static final String SERIALIZED_NAME_TRANSACTION_ID = "transaction_id";
@SerializedName(SERIALIZED_NAME_TRANSACTION_ID)
private String transactionId;
/**
* Please use the `payment_channel` field, `transaction_type` will be deprecated in the future. `digital:` transactions that took place online. `place:` transactions that were made at a physical location. `special:` transactions that relate to banks, e.g. fees or deposits. `unresolved:` transactions that do not fit into the other three types.
*/
@JsonAdapter(TransactionTypeEnum.Adapter.class)
public enum TransactionTypeEnum {
DIGITAL("digital"),
PLACE("place"),
SPECIAL("special"),
UNRESOLVED("unresolved");
private String value;
TransactionTypeEnum(String value) {
this.value = value;
}
public String getValue() {
return value;
}
@Override
public String toString() {
return String.valueOf(value);
}
public static TransactionTypeEnum fromValue(String value) {
for (TransactionTypeEnum b : TransactionTypeEnum.values()) {
if (b.value.equals(value)) {
return b;
}
}
throw new IllegalArgumentException("Unexpected value '" + value + "'");
}
public static class Adapter extends TypeAdapter {
@Override
public void write(final JsonWriter jsonWriter, final TransactionTypeEnum enumeration) throws IOException {
jsonWriter.value(enumeration.getValue());
}
@Override
public TransactionTypeEnum read(final JsonReader jsonReader) throws IOException {
String value = jsonReader.nextString();
return TransactionTypeEnum.fromValue(value);
}
}
}
public static final String SERIALIZED_NAME_TRANSACTION_TYPE = "transaction_type";
@SerializedName(SERIALIZED_NAME_TRANSACTION_TYPE)
private TransactionTypeEnum transactionType;
public static final String SERIALIZED_NAME_LOGO_URL = "logo_url";
@SerializedName(SERIALIZED_NAME_LOGO_URL)
private String logoUrl;
public static final String SERIALIZED_NAME_WEBSITE = "website";
@SerializedName(SERIALIZED_NAME_WEBSITE)
private String website;
public static final String SERIALIZED_NAME_AUTHORIZED_DATE = "authorized_date";
@SerializedName(SERIALIZED_NAME_AUTHORIZED_DATE)
private LocalDate authorizedDate;
public static final String SERIALIZED_NAME_AUTHORIZED_DATETIME = "authorized_datetime";
@SerializedName(SERIALIZED_NAME_AUTHORIZED_DATETIME)
private OffsetDateTime authorizedDatetime;
public static final String SERIALIZED_NAME_DATETIME = "datetime";
@SerializedName(SERIALIZED_NAME_DATETIME)
private OffsetDateTime datetime;
/**
* The channel used to make a payment. `online:` transactions that took place online. `in store:` transactions that were made at a physical location. `other:` transactions that relate to banks, e.g. fees or deposits. This field replaces the `transaction_type` field.
*/
@JsonAdapter(PaymentChannelEnum.Adapter.class)
public enum PaymentChannelEnum {
ONLINE("online"),
IN_STORE("in store"),
OTHER("other");
private String value;
PaymentChannelEnum(String value) {
this.value = value;
}
public String getValue() {
return value;
}
@Override
public String toString() {
return String.valueOf(value);
}
public static PaymentChannelEnum fromValue(String value) {
for (PaymentChannelEnum b : PaymentChannelEnum.values()) {
if (b.value.equals(value)) {
return b;
}
}
throw new IllegalArgumentException("Unexpected value '" + value + "'");
}
public static class Adapter extends TypeAdapter {
@Override
public void write(final JsonWriter jsonWriter, final PaymentChannelEnum enumeration) throws IOException {
jsonWriter.value(enumeration.getValue());
}
@Override
public PaymentChannelEnum read(final JsonReader jsonReader) throws IOException {
String value = jsonReader.nextString();
return PaymentChannelEnum.fromValue(value);
}
}
}
public static final String SERIALIZED_NAME_PAYMENT_CHANNEL = "payment_channel";
@SerializedName(SERIALIZED_NAME_PAYMENT_CHANNEL)
private PaymentChannelEnum paymentChannel;
public static final String SERIALIZED_NAME_PERSONAL_FINANCE_CATEGORY = "personal_finance_category";
@SerializedName(SERIALIZED_NAME_PERSONAL_FINANCE_CATEGORY)
private PersonalFinanceCategory personalFinanceCategory;
public static final String SERIALIZED_NAME_TRANSACTION_CODE = "transaction_code";
@SerializedName(SERIALIZED_NAME_TRANSACTION_CODE)
private TransactionCode transactionCode;
public static final String SERIALIZED_NAME_PERSONAL_FINANCE_CATEGORY_ICON_URL = "personal_finance_category_icon_url";
@SerializedName(SERIALIZED_NAME_PERSONAL_FINANCE_CATEGORY_ICON_URL)
private String personalFinanceCategoryIconUrl;
public static final String SERIALIZED_NAME_COUNTERPARTIES = "counterparties";
@SerializedName(SERIALIZED_NAME_COUNTERPARTIES)
private List counterparties = null;
public static final String SERIALIZED_NAME_MERCHANT_ENTITY_ID = "merchant_entity_id";
@SerializedName(SERIALIZED_NAME_MERCHANT_ENTITY_ID)
private String merchantEntityId;
public Transaction accountId(String accountId) {
this.accountId = accountId;
return this;
}
/**
* The ID of the account in which this transaction occurred.
* @return accountId
**/
@ApiModelProperty(required = true, value = "The ID of the account in which this transaction occurred.")
public String getAccountId() {
return accountId;
}
public void setAccountId(String accountId) {
this.accountId = accountId;
}
public Transaction amount(Double amount) {
this.amount = amount;
return this;
}
/**
* The settled value of the transaction, denominated in the transactions's currency, as stated in `iso_currency_code` or `unofficial_currency_code`. Positive values when money moves out of the account; negative values when money moves in. For example, debit card purchases are positive; credit card payments, direct deposits, and refunds are negative.
* @return amount
**/
@ApiModelProperty(required = true, value = "The settled value of the transaction, denominated in the transactions's currency, as stated in `iso_currency_code` or `unofficial_currency_code`. Positive values when money moves out of the account; negative values when money moves in. For example, debit card purchases are positive; credit card payments, direct deposits, and refunds are negative.")
public Double getAmount() {
return amount;
}
public void setAmount(Double amount) {
this.amount = amount;
}
public Transaction isoCurrencyCode(String isoCurrencyCode) {
this.isoCurrencyCode = isoCurrencyCode;
return this;
}
/**
* The ISO-4217 currency code of the transaction. Always `null` if `unofficial_currency_code` is non-null.
* @return isoCurrencyCode
**/
@javax.annotation.Nullable
@ApiModelProperty(required = true, value = "The ISO-4217 currency code of the transaction. Always `null` if `unofficial_currency_code` is non-null.")
public String getIsoCurrencyCode() {
return isoCurrencyCode;
}
public void setIsoCurrencyCode(String isoCurrencyCode) {
this.isoCurrencyCode = isoCurrencyCode;
}
public Transaction unofficialCurrencyCode(String unofficialCurrencyCode) {
this.unofficialCurrencyCode = unofficialCurrencyCode;
return this;
}
/**
* The unofficial currency code associated with the transaction. Always `null` if `iso_currency_code` is non-`null`. Unofficial currency codes are used for currencies that do not have official ISO currency codes, such as cryptocurrencies and the currencies of certain countries. See the [currency code schema](https://plaid.com/docs/api/accounts#currency-code-schema) for a full listing of supported `iso_currency_code`s.
* @return unofficialCurrencyCode
**/
@javax.annotation.Nullable
@ApiModelProperty(required = true, value = "The unofficial currency code associated with the transaction. Always `null` if `iso_currency_code` is non-`null`. Unofficial currency codes are used for currencies that do not have official ISO currency codes, such as cryptocurrencies and the currencies of certain countries. See the [currency code schema](https://plaid.com/docs/api/accounts#currency-code-schema) for a full listing of supported `iso_currency_code`s.")
public String getUnofficialCurrencyCode() {
return unofficialCurrencyCode;
}
public void setUnofficialCurrencyCode(String unofficialCurrencyCode) {
this.unofficialCurrencyCode = unofficialCurrencyCode;
}
public Transaction category(List category) {
this.category = category;
return this;
}
public Transaction addCategoryItem(String categoryItem) {
this.category.add(categoryItem);
return this;
}
/**
* A hierarchical array of the categories to which this transaction belongs. For a full list of categories, see [`/categories/get`](https://plaid.com/docs/api/products/transactions/#categoriesget). All Transactions implementations are recommended to use the new `personal_finance_category` instead of `category`, as it provides greater accuracy and more meaningful categorization. If the `transactions` object was returned by an Assets endpoint such as `/asset_report/get/` or `/asset_report/pdf/get`, this field will only appear in an Asset Report with Insights.
* @return category
**/
@javax.annotation.Nullable
@ApiModelProperty(required = true, value = "A hierarchical array of the categories to which this transaction belongs. For a full list of categories, see [`/categories/get`](https://plaid.com/docs/api/products/transactions/#categoriesget). All Transactions implementations are recommended to use the new `personal_finance_category` instead of `category`, as it provides greater accuracy and more meaningful categorization. If the `transactions` object was returned by an Assets endpoint such as `/asset_report/get/` or `/asset_report/pdf/get`, this field will only appear in an Asset Report with Insights.")
public List getCategory() {
return category;
}
public void setCategory(List category) {
this.category = category;
}
public Transaction categoryId(String categoryId) {
this.categoryId = categoryId;
return this;
}
/**
* The ID of the category to which this transaction belongs. For a full list of categories, see [`/categories/get`](https://plaid.com/docs/api/products/transactions/#categoriesget). All Transactions implementations are recommended to use the new `personal_finance_category` instead of `category`, as it provides greater accuracy and more meaningful categorization. If the `transactions` object was returned by an Assets endpoint such as `/asset_report/get/` or `/asset_report/pdf/get`, this field will only appear in an Asset Report with Insights.
* @return categoryId
**/
@javax.annotation.Nullable
@ApiModelProperty(required = true, value = "The ID of the category to which this transaction belongs. For a full list of categories, see [`/categories/get`](https://plaid.com/docs/api/products/transactions/#categoriesget). All Transactions implementations are recommended to use the new `personal_finance_category` instead of `category`, as it provides greater accuracy and more meaningful categorization. If the `transactions` object was returned by an Assets endpoint such as `/asset_report/get/` or `/asset_report/pdf/get`, this field will only appear in an Asset Report with Insights.")
public String getCategoryId() {
return categoryId;
}
public void setCategoryId(String categoryId) {
this.categoryId = categoryId;
}
public Transaction checkNumber(String checkNumber) {
this.checkNumber = checkNumber;
return this;
}
/**
* The check number of the transaction. This field is only populated for check transactions.
* @return checkNumber
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "The check number of the transaction. This field is only populated for check transactions.")
public String getCheckNumber() {
return checkNumber;
}
public void setCheckNumber(String checkNumber) {
this.checkNumber = checkNumber;
}
public Transaction date(LocalDate date) {
this.date = date;
return this;
}
/**
* For pending transactions, the date that the transaction occurred; for posted transactions, the date that the transaction posted. Both dates are returned in an [ISO 8601](https://wikipedia.org/wiki/ISO_8601) format ( `YYYY-MM-DD` ). To receive information about the date that a posted transaction was initiated, see the `authorized_date` field.
* @return date
**/
@ApiModelProperty(required = true, value = "For pending transactions, the date that the transaction occurred; for posted transactions, the date that the transaction posted. Both dates are returned in an [ISO 8601](https://wikipedia.org/wiki/ISO_8601) format ( `YYYY-MM-DD` ). To receive information about the date that a posted transaction was initiated, see the `authorized_date` field.")
public LocalDate getDate() {
return date;
}
public void setDate(LocalDate date) {
this.date = date;
}
public Transaction location(Location location) {
this.location = location;
return this;
}
/**
* Get location
* @return location
**/
@ApiModelProperty(required = true, value = "")
public Location getLocation() {
return location;
}
public void setLocation(Location location) {
this.location = location;
}
public Transaction name(String name) {
this.name = name;
return this;
}
/**
* The merchant name or transaction description. If the `transactions` object was returned by a Transactions endpoint such as `/transactions/sync` or `/transactions/get`, this field will always appear. If the `transactions` object was returned by an Assets endpoint such as `/asset_report/get/` or `/asset_report/pdf/get`, this field will only appear in an Asset Report with Insights.
* @return name
**/
@ApiModelProperty(required = true, value = "The merchant name or transaction description. If the `transactions` object was returned by a Transactions endpoint such as `/transactions/sync` or `/transactions/get`, this field will always appear. If the `transactions` object was returned by an Assets endpoint such as `/asset_report/get/` or `/asset_report/pdf/get`, this field will only appear in an Asset Report with Insights.")
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public Transaction merchantName(String merchantName) {
this.merchantName = merchantName;
return this;
}
/**
* The merchant name, as enriched by Plaid from the `name` field. This is typically a more human-readable version of the merchant counterparty in the transaction. For some bank transactions (such as checks or account transfers) where there is no meaningful merchant name, this value will be `null`.
* @return merchantName
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "The merchant name, as enriched by Plaid from the `name` field. This is typically a more human-readable version of the merchant counterparty in the transaction. For some bank transactions (such as checks or account transfers) where there is no meaningful merchant name, this value will be `null`.")
public String getMerchantName() {
return merchantName;
}
public void setMerchantName(String merchantName) {
this.merchantName = merchantName;
}
public Transaction originalDescription(String originalDescription) {
this.originalDescription = originalDescription;
return this;
}
/**
* The string returned by the financial institution to describe the transaction. For transactions returned by `/transactions/sync` or `/transactions/get`, this field will be omitted unless the client has set `options.include_original_description` to `true`.
* @return originalDescription
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "The string returned by the financial institution to describe the transaction. For transactions returned by `/transactions/sync` or `/transactions/get`, this field will be omitted unless the client has set `options.include_original_description` to `true`.")
public String getOriginalDescription() {
return originalDescription;
}
public void setOriginalDescription(String originalDescription) {
this.originalDescription = originalDescription;
}
public Transaction paymentMeta(PaymentMeta paymentMeta) {
this.paymentMeta = paymentMeta;
return this;
}
/**
* Get paymentMeta
* @return paymentMeta
**/
@ApiModelProperty(required = true, value = "")
public PaymentMeta getPaymentMeta() {
return paymentMeta;
}
public void setPaymentMeta(PaymentMeta paymentMeta) {
this.paymentMeta = paymentMeta;
}
public Transaction pending(Boolean pending) {
this.pending = pending;
return this;
}
/**
* When `true`, identifies the transaction as pending or unsettled. Pending transaction details (name, type, amount, category ID) may change before they are settled.
* @return pending
**/
@ApiModelProperty(required = true, value = "When `true`, identifies the transaction as pending or unsettled. Pending transaction details (name, type, amount, category ID) may change before they are settled.")
public Boolean getPending() {
return pending;
}
public void setPending(Boolean pending) {
this.pending = pending;
}
public Transaction pendingTransactionId(String pendingTransactionId) {
this.pendingTransactionId = pendingTransactionId;
return this;
}
/**
* The ID of a posted transaction's associated pending transaction, where applicable.
* @return pendingTransactionId
**/
@javax.annotation.Nullable
@ApiModelProperty(required = true, value = "The ID of a posted transaction's associated pending transaction, where applicable.")
public String getPendingTransactionId() {
return pendingTransactionId;
}
public void setPendingTransactionId(String pendingTransactionId) {
this.pendingTransactionId = pendingTransactionId;
}
public Transaction accountOwner(String accountOwner) {
this.accountOwner = accountOwner;
return this;
}
/**
* The name of the account owner. This field is not typically populated and only relevant when dealing with sub-accounts.
* @return accountOwner
**/
@javax.annotation.Nullable
@ApiModelProperty(required = true, value = "The name of the account owner. This field is not typically populated and only relevant when dealing with sub-accounts.")
public String getAccountOwner() {
return accountOwner;
}
public void setAccountOwner(String accountOwner) {
this.accountOwner = accountOwner;
}
public Transaction transactionId(String transactionId) {
this.transactionId = transactionId;
return this;
}
/**
* The unique ID of the transaction. Like all Plaid identifiers, the `transaction_id` is case sensitive.
* @return transactionId
**/
@ApiModelProperty(required = true, value = "The unique ID of the transaction. Like all Plaid identifiers, the `transaction_id` is case sensitive.")
public String getTransactionId() {
return transactionId;
}
public void setTransactionId(String transactionId) {
this.transactionId = transactionId;
}
public Transaction transactionType(TransactionTypeEnum transactionType) {
this.transactionType = transactionType;
return this;
}
/**
* Please use the `payment_channel` field, `transaction_type` will be deprecated in the future. `digital:` transactions that took place online. `place:` transactions that were made at a physical location. `special:` transactions that relate to banks, e.g. fees or deposits. `unresolved:` transactions that do not fit into the other three types.
* @return transactionType
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Please use the `payment_channel` field, `transaction_type` will be deprecated in the future. `digital:` transactions that took place online. `place:` transactions that were made at a physical location. `special:` transactions that relate to banks, e.g. fees or deposits. `unresolved:` transactions that do not fit into the other three types. ")
public TransactionTypeEnum getTransactionType() {
return transactionType;
}
public void setTransactionType(TransactionTypeEnum transactionType) {
this.transactionType = transactionType;
}
public Transaction logoUrl(String logoUrl) {
this.logoUrl = logoUrl;
return this;
}
/**
* The URL of a logo associated with this transaction, if available. The logo will always be 100×100 pixel PNG file.
* @return logoUrl
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "The URL of a logo associated with this transaction, if available. The logo will always be 100×100 pixel PNG file.")
public String getLogoUrl() {
return logoUrl;
}
public void setLogoUrl(String logoUrl) {
this.logoUrl = logoUrl;
}
public Transaction website(String website) {
this.website = website;
return this;
}
/**
* The website associated with this transaction, if available.
* @return website
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "The website associated with this transaction, if available.")
public String getWebsite() {
return website;
}
public void setWebsite(String website) {
this.website = website;
}
public Transaction authorizedDate(LocalDate authorizedDate) {
this.authorizedDate = authorizedDate;
return this;
}
/**
* The date that the transaction was authorized. For posted transactions, the `date` field will indicate the posted date, but `authorized_date` will indicate the day the transaction was authorized by the financial institution. If presenting transactions to the user in a UI, the `authorized_date`, when available, is generally preferable to use over the `date` field for posted transactions, as it will generally represent the date the user actually made the transaction. Dates are returned in an [ISO 8601](https://wikipedia.org/wiki/ISO_8601) format ( `YYYY-MM-DD` ).
* @return authorizedDate
**/
@javax.annotation.Nullable
@ApiModelProperty(required = true, value = "The date that the transaction was authorized. For posted transactions, the `date` field will indicate the posted date, but `authorized_date` will indicate the day the transaction was authorized by the financial institution. If presenting transactions to the user in a UI, the `authorized_date`, when available, is generally preferable to use over the `date` field for posted transactions, as it will generally represent the date the user actually made the transaction. Dates are returned in an [ISO 8601](https://wikipedia.org/wiki/ISO_8601) format ( `YYYY-MM-DD` ).")
public LocalDate getAuthorizedDate() {
return authorizedDate;
}
public void setAuthorizedDate(LocalDate authorizedDate) {
this.authorizedDate = authorizedDate;
}
public Transaction authorizedDatetime(OffsetDateTime authorizedDatetime) {
this.authorizedDatetime = authorizedDatetime;
return this;
}
/**
* Date and time when a transaction was authorized in [ISO 8601](https://wikipedia.org/wiki/ISO_8601) format ( `YYYY-MM-DDTHH:mm:ssZ` ). For posted transactions, the `datetime` field will indicate the posted date, but `authorized_datetime` will indicate the day the transaction was authorized by the financial institution. If presenting transactions to the user in a UI, the `authorized_datetime`, when available, is generally preferable to use over the `datetime` field for posted transactions, as it will generally represent the date the user actually made the transaction. This field is returned for select financial institutions and comes as provided by the institution. It may contain default time values (such as 00:00:00). This field is only populated in API version 2019-05-29 and later.
* @return authorizedDatetime
**/
@javax.annotation.Nullable
@ApiModelProperty(required = true, value = "Date and time when a transaction was authorized in [ISO 8601](https://wikipedia.org/wiki/ISO_8601) format ( `YYYY-MM-DDTHH:mm:ssZ` ). For posted transactions, the `datetime` field will indicate the posted date, but `authorized_datetime` will indicate the day the transaction was authorized by the financial institution. If presenting transactions to the user in a UI, the `authorized_datetime`, when available, is generally preferable to use over the `datetime` field for posted transactions, as it will generally represent the date the user actually made the transaction. This field is returned for select financial institutions and comes as provided by the institution. It may contain default time values (such as 00:00:00). This field is only populated in API version 2019-05-29 and later.")
public OffsetDateTime getAuthorizedDatetime() {
return authorizedDatetime;
}
public void setAuthorizedDatetime(OffsetDateTime authorizedDatetime) {
this.authorizedDatetime = authorizedDatetime;
}
public Transaction datetime(OffsetDateTime datetime) {
this.datetime = datetime;
return this;
}
/**
* Date and time when a transaction was posted in [ISO 8601](https://wikipedia.org/wiki/ISO_8601) format ( `YYYY-MM-DDTHH:mm:ssZ` ). For the date that the transaction was initiated, rather than posted, see the `authorized_datetime` field. This field is returned for select financial institutions and comes as provided by the institution. It may contain default time values (such as 00:00:00). This field is only populated in API version 2019-05-29 and later.
* @return datetime
**/
@javax.annotation.Nullable
@ApiModelProperty(required = true, value = "Date and time when a transaction was posted in [ISO 8601](https://wikipedia.org/wiki/ISO_8601) format ( `YYYY-MM-DDTHH:mm:ssZ` ). For the date that the transaction was initiated, rather than posted, see the `authorized_datetime` field. This field is returned for select financial institutions and comes as provided by the institution. It may contain default time values (such as 00:00:00). This field is only populated in API version 2019-05-29 and later.")
public OffsetDateTime getDatetime() {
return datetime;
}
public void setDatetime(OffsetDateTime datetime) {
this.datetime = datetime;
}
public Transaction paymentChannel(PaymentChannelEnum paymentChannel) {
this.paymentChannel = paymentChannel;
return this;
}
/**
* The channel used to make a payment. `online:` transactions that took place online. `in store:` transactions that were made at a physical location. `other:` transactions that relate to banks, e.g. fees or deposits. This field replaces the `transaction_type` field.
* @return paymentChannel
**/
@ApiModelProperty(required = true, value = "The channel used to make a payment. `online:` transactions that took place online. `in store:` transactions that were made at a physical location. `other:` transactions that relate to banks, e.g. fees or deposits. This field replaces the `transaction_type` field. ")
public PaymentChannelEnum getPaymentChannel() {
return paymentChannel;
}
public void setPaymentChannel(PaymentChannelEnum paymentChannel) {
this.paymentChannel = paymentChannel;
}
public Transaction personalFinanceCategory(PersonalFinanceCategory personalFinanceCategory) {
this.personalFinanceCategory = personalFinanceCategory;
return this;
}
/**
* Get personalFinanceCategory
* @return personalFinanceCategory
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
public PersonalFinanceCategory getPersonalFinanceCategory() {
return personalFinanceCategory;
}
public void setPersonalFinanceCategory(PersonalFinanceCategory personalFinanceCategory) {
this.personalFinanceCategory = personalFinanceCategory;
}
public Transaction transactionCode(TransactionCode transactionCode) {
this.transactionCode = transactionCode;
return this;
}
/**
* Get transactionCode
* @return transactionCode
**/
@javax.annotation.Nullable
@ApiModelProperty(required = true, value = "")
public TransactionCode getTransactionCode() {
return transactionCode;
}
public void setTransactionCode(TransactionCode transactionCode) {
this.transactionCode = transactionCode;
}
public Transaction personalFinanceCategoryIconUrl(String personalFinanceCategoryIconUrl) {
this.personalFinanceCategoryIconUrl = personalFinanceCategoryIconUrl;
return this;
}
/**
* The URL of an icon associated with the primary personal finance category. The icon will always be 100×100 pixel PNG file.
* @return personalFinanceCategoryIconUrl
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "The URL of an icon associated with the primary personal finance category. The icon will always be 100×100 pixel PNG file.")
public String getPersonalFinanceCategoryIconUrl() {
return personalFinanceCategoryIconUrl;
}
public void setPersonalFinanceCategoryIconUrl(String personalFinanceCategoryIconUrl) {
this.personalFinanceCategoryIconUrl = personalFinanceCategoryIconUrl;
}
public Transaction counterparties(List counterparties) {
this.counterparties = counterparties;
return this;
}
public Transaction addCounterpartiesItem(TransactionCounterparty counterpartiesItem) {
if (this.counterparties == null) {
this.counterparties = new ArrayList<>();
}
this.counterparties.add(counterpartiesItem);
return this;
}
/**
* The counterparties present in the transaction. Counterparties, such as the merchant or the financial institution, are extracted by Plaid from the raw description.
* @return counterparties
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "The counterparties present in the transaction. Counterparties, such as the merchant or the financial institution, are extracted by Plaid from the raw description.")
public List getCounterparties() {
return counterparties;
}
public void setCounterparties(List counterparties) {
this.counterparties = counterparties;
}
public Transaction merchantEntityId(String merchantEntityId) {
this.merchantEntityId = merchantEntityId;
return this;
}
/**
* A unique, stable, Plaid-generated ID that maps to the merchant. In the case of a merchant with multiple retail locations, this field will map to the broader merchant, not a specific location or store.
* @return merchantEntityId
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "A unique, stable, Plaid-generated ID that maps to the merchant. In the case of a merchant with multiple retail locations, this field will map to the broader merchant, not a specific location or store.")
public String getMerchantEntityId() {
return merchantEntityId;
}
public void setMerchantEntityId(String merchantEntityId) {
this.merchantEntityId = merchantEntityId;
}
@Override
public boolean equals(Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
Transaction transaction = (Transaction) o;
return Objects.equals(this.accountId, transaction.accountId) &&
Objects.equals(this.amount, transaction.amount) &&
Objects.equals(this.isoCurrencyCode, transaction.isoCurrencyCode) &&
Objects.equals(this.unofficialCurrencyCode, transaction.unofficialCurrencyCode) &&
Objects.equals(this.category, transaction.category) &&
Objects.equals(this.categoryId, transaction.categoryId) &&
Objects.equals(this.checkNumber, transaction.checkNumber) &&
Objects.equals(this.date, transaction.date) &&
Objects.equals(this.location, transaction.location) &&
Objects.equals(this.name, transaction.name) &&
Objects.equals(this.merchantName, transaction.merchantName) &&
Objects.equals(this.originalDescription, transaction.originalDescription) &&
Objects.equals(this.paymentMeta, transaction.paymentMeta) &&
Objects.equals(this.pending, transaction.pending) &&
Objects.equals(this.pendingTransactionId, transaction.pendingTransactionId) &&
Objects.equals(this.accountOwner, transaction.accountOwner) &&
Objects.equals(this.transactionId, transaction.transactionId) &&
Objects.equals(this.transactionType, transaction.transactionType) &&
Objects.equals(this.logoUrl, transaction.logoUrl) &&
Objects.equals(this.website, transaction.website) &&
Objects.equals(this.authorizedDate, transaction.authorizedDate) &&
Objects.equals(this.authorizedDatetime, transaction.authorizedDatetime) &&
Objects.equals(this.datetime, transaction.datetime) &&
Objects.equals(this.paymentChannel, transaction.paymentChannel) &&
Objects.equals(this.personalFinanceCategory, transaction.personalFinanceCategory) &&
Objects.equals(this.transactionCode, transaction.transactionCode) &&
Objects.equals(this.personalFinanceCategoryIconUrl, transaction.personalFinanceCategoryIconUrl) &&
Objects.equals(this.counterparties, transaction.counterparties) &&
Objects.equals(this.merchantEntityId, transaction.merchantEntityId);
}
@Override
public int hashCode() {
return Objects.hash(accountId, amount, isoCurrencyCode, unofficialCurrencyCode, category, categoryId, checkNumber, date, location, name, merchantName, originalDescription, paymentMeta, pending, pendingTransactionId, accountOwner, transactionId, transactionType, logoUrl, website, authorizedDate, authorizedDatetime, datetime, paymentChannel, personalFinanceCategory, transactionCode, personalFinanceCategoryIconUrl, counterparties, merchantEntityId);
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class Transaction {\n");
sb.append(" accountId: ").append(toIndentedString(accountId)).append("\n");
sb.append(" amount: ").append(toIndentedString(amount)).append("\n");
sb.append(" isoCurrencyCode: ").append(toIndentedString(isoCurrencyCode)).append("\n");
sb.append(" unofficialCurrencyCode: ").append(toIndentedString(unofficialCurrencyCode)).append("\n");
sb.append(" category: ").append(toIndentedString(category)).append("\n");
sb.append(" categoryId: ").append(toIndentedString(categoryId)).append("\n");
sb.append(" checkNumber: ").append(toIndentedString(checkNumber)).append("\n");
sb.append(" date: ").append(toIndentedString(date)).append("\n");
sb.append(" location: ").append(toIndentedString(location)).append("\n");
sb.append(" name: ").append(toIndentedString(name)).append("\n");
sb.append(" merchantName: ").append(toIndentedString(merchantName)).append("\n");
sb.append(" originalDescription: ").append(toIndentedString(originalDescription)).append("\n");
sb.append(" paymentMeta: ").append(toIndentedString(paymentMeta)).append("\n");
sb.append(" pending: ").append(toIndentedString(pending)).append("\n");
sb.append(" pendingTransactionId: ").append(toIndentedString(pendingTransactionId)).append("\n");
sb.append(" accountOwner: ").append(toIndentedString(accountOwner)).append("\n");
sb.append(" transactionId: ").append(toIndentedString(transactionId)).append("\n");
sb.append(" transactionType: ").append(toIndentedString(transactionType)).append("\n");
sb.append(" logoUrl: ").append(toIndentedString(logoUrl)).append("\n");
sb.append(" website: ").append(toIndentedString(website)).append("\n");
sb.append(" authorizedDate: ").append(toIndentedString(authorizedDate)).append("\n");
sb.append(" authorizedDatetime: ").append(toIndentedString(authorizedDatetime)).append("\n");
sb.append(" datetime: ").append(toIndentedString(datetime)).append("\n");
sb.append(" paymentChannel: ").append(toIndentedString(paymentChannel)).append("\n");
sb.append(" personalFinanceCategory: ").append(toIndentedString(personalFinanceCategory)).append("\n");
sb.append(" transactionCode: ").append(toIndentedString(transactionCode)).append("\n");
sb.append(" personalFinanceCategoryIconUrl: ").append(toIndentedString(personalFinanceCategoryIconUrl)).append("\n");
sb.append(" counterparties: ").append(toIndentedString(counterparties)).append("\n");
sb.append(" merchantEntityId: ").append(toIndentedString(merchantEntityId)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces
* (except the first line).
*/
private String toIndentedString(Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy