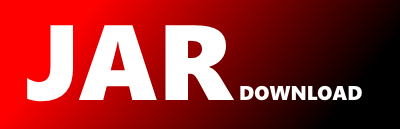
com.plaid.client.model.UserCustomPassword Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of plaid-java Show documentation
Show all versions of plaid-java Show documentation
Bindings for the Plaid (plaid.com) API.
/*
* The Plaid API
* The Plaid REST API. Please see https://plaid.com/docs/api for more details.
*
* The version of the OpenAPI document: 2020-09-14_1.503.5
*
*
* NOTE: This class is auto generated by OpenAPI Generator (https://openapi-generator.tech).
* https://openapi-generator.tech
* Do not edit the class manually.
*/
package com.plaid.client.model;
import java.util.Objects;
import java.util.Arrays;
import com.google.gson.TypeAdapter;
import com.google.gson.annotations.JsonAdapter;
import com.google.gson.annotations.SerializedName;
import com.google.gson.stream.JsonReader;
import com.google.gson.stream.JsonWriter;
import com.plaid.client.model.MFA;
import com.plaid.client.model.OverrideAccounts;
import io.swagger.annotations.ApiModel;
import io.swagger.annotations.ApiModelProperty;
import java.io.IOException;
import java.util.ArrayList;
import java.util.List;
/**
* Custom test accounts are configured with a JSON configuration object formulated according to the schema below. All top level fields are optional. Sending an empty object as a configuration will result in an account configured with random balances and transaction history.
*/
@ApiModel(description = "Custom test accounts are configured with a JSON configuration object formulated according to the schema below. All top level fields are optional. Sending an empty object as a configuration will result in an account configured with random balances and transaction history.")
@javax.annotation.Generated(value = "org.openapitools.codegen.languages.JavaClientCodegen", date = "2024-03-28T21:00:55.745394Z[Etc/UTC]")
public class UserCustomPassword {
public static final String SERIALIZED_NAME_VERSION = "version";
@SerializedName(SERIALIZED_NAME_VERSION)
private String version;
public static final String SERIALIZED_NAME_SEED = "seed";
@SerializedName(SERIALIZED_NAME_SEED)
private String seed;
public static final String SERIALIZED_NAME_OVERRIDE_ACCOUNTS = "override_accounts";
@SerializedName(SERIALIZED_NAME_OVERRIDE_ACCOUNTS)
private List overrideAccounts = new ArrayList<>();
public static final String SERIALIZED_NAME_MFA = "mfa";
@SerializedName(SERIALIZED_NAME_MFA)
private MFA mfa;
public static final String SERIALIZED_NAME_RECAPTCHA = "recaptcha";
@SerializedName(SERIALIZED_NAME_RECAPTCHA)
private String recaptcha;
public static final String SERIALIZED_NAME_FORCE_ERROR = "force_error";
@SerializedName(SERIALIZED_NAME_FORCE_ERROR)
private String forceError;
public UserCustomPassword version(String version) {
this.version = version;
return this;
}
/**
* The version of the password schema to use, possible values are 1 or 2. The default value is 2. You should only specify 1 if you know it is necessary for your test suite.
* @return version
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "The version of the password schema to use, possible values are 1 or 2. The default value is 2. You should only specify 1 if you know it is necessary for your test suite.")
public String getVersion() {
return version;
}
public void setVersion(String version) {
this.version = version;
}
public UserCustomPassword seed(String seed) {
this.seed = seed;
return this;
}
/**
* A seed, in the form of a string, that will be used to randomly generate account and transaction data, if this data is not specified using the `override_accounts` argument. If no seed is specified, the randomly generated data will be different each time. Note that transactions data is generated relative to the Item's creation date. Different Items created on different dates with the same seed for transactions data will have different dates for the transactions. The number of days between each transaction and the Item creation will remain constant. For example, an Item created on December 15 might show a transaction on December 14. An Item created on December 20, using the same seed, would show that same transaction occurring on December 19.
* @return seed
**/
@ApiModelProperty(required = true, value = "A seed, in the form of a string, that will be used to randomly generate account and transaction data, if this data is not specified using the `override_accounts` argument. If no seed is specified, the randomly generated data will be different each time. Note that transactions data is generated relative to the Item's creation date. Different Items created on different dates with the same seed for transactions data will have different dates for the transactions. The number of days between each transaction and the Item creation will remain constant. For example, an Item created on December 15 might show a transaction on December 14. An Item created on December 20, using the same seed, would show that same transaction occurring on December 19.")
public String getSeed() {
return seed;
}
public void setSeed(String seed) {
this.seed = seed;
}
public UserCustomPassword overrideAccounts(List overrideAccounts) {
this.overrideAccounts = overrideAccounts;
return this;
}
public UserCustomPassword addOverrideAccountsItem(OverrideAccounts overrideAccountsItem) {
this.overrideAccounts.add(overrideAccountsItem);
return this;
}
/**
* An array of account overrides to configure the accounts for the Item. By default, if no override is specified, transactions and account data will be randomly generated based on the account type and subtype, and other products will have fixed or empty data.
* @return overrideAccounts
**/
@ApiModelProperty(required = true, value = "An array of account overrides to configure the accounts for the Item. By default, if no override is specified, transactions and account data will be randomly generated based on the account type and subtype, and other products will have fixed or empty data.")
public List getOverrideAccounts() {
return overrideAccounts;
}
public void setOverrideAccounts(List overrideAccounts) {
this.overrideAccounts = overrideAccounts;
}
public UserCustomPassword mfa(MFA mfa) {
this.mfa = mfa;
return this;
}
/**
* Get mfa
* @return mfa
**/
@ApiModelProperty(required = true, value = "")
public MFA getMfa() {
return mfa;
}
public void setMfa(MFA mfa) {
this.mfa = mfa;
}
public UserCustomPassword recaptcha(String recaptcha) {
this.recaptcha = recaptcha;
return this;
}
/**
* You may trigger a reCAPTCHA in Plaid Link in the Sandbox environment by using the recaptcha field. Possible values are `good` or `bad`. A value of `good` will result in successful Item creation and `bad` will result in a `RECAPTCHA_BAD` error to simulate a failed reCAPTCHA. Both values require the reCAPTCHA to be manually solved within Plaid Link.
* @return recaptcha
**/
@ApiModelProperty(required = true, value = "You may trigger a reCAPTCHA in Plaid Link in the Sandbox environment by using the recaptcha field. Possible values are `good` or `bad`. A value of `good` will result in successful Item creation and `bad` will result in a `RECAPTCHA_BAD` error to simulate a failed reCAPTCHA. Both values require the reCAPTCHA to be manually solved within Plaid Link.")
public String getRecaptcha() {
return recaptcha;
}
public void setRecaptcha(String recaptcha) {
this.recaptcha = recaptcha;
}
public UserCustomPassword forceError(String forceError) {
this.forceError = forceError;
return this;
}
/**
* An error code to force on Item creation. Possible values are: `\"INSTITUTION_NOT_RESPONDING\"` `\"INSTITUTION_NO_LONGER_SUPPORTED\"` `\"INVALID_CREDENTIALS\"` `\"INVALID_MFA\"` `\"ITEM_LOCKED\"` `\"ITEM_LOGIN_REQUIRED\"` `\"ITEM_NOT_SUPPORTED\"` `\"INVALID_LINK_TOKEN\"` `\"MFA_NOT_SUPPORTED\"` `\"NO_ACCOUNTS\"` `\"PLAID_ERROR\"` `\"USER_INPUT_TIMEOUT\"` `\"USER_SETUP_REQUIRED\"`
* @return forceError
**/
@ApiModelProperty(required = true, value = "An error code to force on Item creation. Possible values are: `\"INSTITUTION_NOT_RESPONDING\"` `\"INSTITUTION_NO_LONGER_SUPPORTED\"` `\"INVALID_CREDENTIALS\"` `\"INVALID_MFA\"` `\"ITEM_LOCKED\"` `\"ITEM_LOGIN_REQUIRED\"` `\"ITEM_NOT_SUPPORTED\"` `\"INVALID_LINK_TOKEN\"` `\"MFA_NOT_SUPPORTED\"` `\"NO_ACCOUNTS\"` `\"PLAID_ERROR\"` `\"USER_INPUT_TIMEOUT\"` `\"USER_SETUP_REQUIRED\"`")
public String getForceError() {
return forceError;
}
public void setForceError(String forceError) {
this.forceError = forceError;
}
@Override
public boolean equals(Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
UserCustomPassword userCustomPassword = (UserCustomPassword) o;
return Objects.equals(this.version, userCustomPassword.version) &&
Objects.equals(this.seed, userCustomPassword.seed) &&
Objects.equals(this.overrideAccounts, userCustomPassword.overrideAccounts) &&
Objects.equals(this.mfa, userCustomPassword.mfa) &&
Objects.equals(this.recaptcha, userCustomPassword.recaptcha) &&
Objects.equals(this.forceError, userCustomPassword.forceError);
}
@Override
public int hashCode() {
return Objects.hash(version, seed, overrideAccounts, mfa, recaptcha, forceError);
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class UserCustomPassword {\n");
sb.append(" version: ").append(toIndentedString(version)).append("\n");
sb.append(" seed: ").append(toIndentedString(seed)).append("\n");
sb.append(" overrideAccounts: ").append(toIndentedString(overrideAccounts)).append("\n");
sb.append(" mfa: ").append(toIndentedString(mfa)).append("\n");
sb.append(" recaptcha: ").append(toIndentedString(recaptcha)).append("\n");
sb.append(" forceError: ").append(toIndentedString(forceError)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces
* (except the first line).
*/
private String toIndentedString(Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy