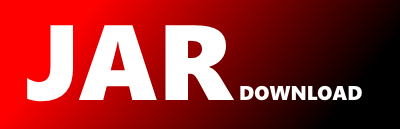
com.plaid.client.model.InvestmentsAuthOwner Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of plaid-java Show documentation
Show all versions of plaid-java Show documentation
Bindings for the Plaid (plaid.com) API.
/*
* The Plaid API
* The Plaid REST API. Please see https://plaid.com/docs/api for more details.
*
* The version of the OpenAPI document: 2020-09-14_1.534.3
*
*
* NOTE: This class is auto generated by OpenAPI Generator (https://openapi-generator.tech).
* https://openapi-generator.tech
* Do not edit the class manually.
*/
package com.plaid.client.model;
import java.util.Objects;
import java.util.Arrays;
import com.google.gson.TypeAdapter;
import com.google.gson.annotations.JsonAdapter;
import com.google.gson.annotations.SerializedName;
import com.google.gson.stream.JsonReader;
import com.google.gson.stream.JsonWriter;
import io.swagger.annotations.ApiModel;
import io.swagger.annotations.ApiModelProperty;
import java.io.IOException;
import java.util.ArrayList;
import java.util.List;
/**
* Information on the ownership of an investments account
*/
@ApiModel(description = "Information on the ownership of an investments account")
@javax.annotation.Generated(value = "org.openapitools.codegen.languages.JavaClientCodegen", date = "2024-07-05T19:36:13.426392Z[Etc/UTC]")
public class InvestmentsAuthOwner {
public static final String SERIALIZED_NAME_ACCOUNT_ID = "account_id";
@SerializedName(SERIALIZED_NAME_ACCOUNT_ID)
private String accountId;
public static final String SERIALIZED_NAME_NAMES = "names";
@SerializedName(SERIALIZED_NAME_NAMES)
private List names = null;
public InvestmentsAuthOwner accountId(String accountId) {
this.accountId = accountId;
return this;
}
/**
* The ID of the account that this identity information pertains to
* @return accountId
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "The ID of the account that this identity information pertains to")
public String getAccountId() {
return accountId;
}
public void setAccountId(String accountId) {
this.accountId = accountId;
}
public InvestmentsAuthOwner names(List names) {
this.names = names;
return this;
}
public InvestmentsAuthOwner addNamesItem(String namesItem) {
if (this.names == null) {
this.names = new ArrayList<>();
}
this.names.add(namesItem);
return this;
}
/**
* A list of names associated with the account by the financial institution. In the case of a joint account, Plaid will make a best effort to report the names of all account holders. If an Item contains multiple accounts with different owner names, some institutions will report all names associated with the Item in each account's `names` array.
* @return names
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "A list of names associated with the account by the financial institution. In the case of a joint account, Plaid will make a best effort to report the names of all account holders. If an Item contains multiple accounts with different owner names, some institutions will report all names associated with the Item in each account's `names` array.")
public List getNames() {
return names;
}
public void setNames(List names) {
this.names = names;
}
@Override
public boolean equals(Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
InvestmentsAuthOwner investmentsAuthOwner = (InvestmentsAuthOwner) o;
return Objects.equals(this.accountId, investmentsAuthOwner.accountId) &&
Objects.equals(this.names, investmentsAuthOwner.names);
}
@Override
public int hashCode() {
return Objects.hash(accountId, names);
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class InvestmentsAuthOwner {\n");
sb.append(" accountId: ").append(toIndentedString(accountId)).append("\n");
sb.append(" names: ").append(toIndentedString(names)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces
* (except the first line).
*/
private String toIndentedString(Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy