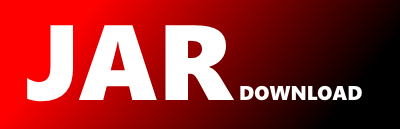
com.plaid.client.model.RecurringTransfer Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of plaid-java Show documentation
Show all versions of plaid-java Show documentation
Bindings for the Plaid (plaid.com) API.
/*
* The Plaid API
* The Plaid REST API. Please see https://plaid.com/docs/api for more details.
*
* The version of the OpenAPI document: 2020-09-14_1.534.3
*
*
* NOTE: This class is auto generated by OpenAPI Generator (https://openapi-generator.tech).
* https://openapi-generator.tech
* Do not edit the class manually.
*/
package com.plaid.client.model;
import java.util.Objects;
import java.util.Arrays;
import com.google.gson.TypeAdapter;
import com.google.gson.annotations.JsonAdapter;
import com.google.gson.annotations.SerializedName;
import com.google.gson.stream.JsonReader;
import com.google.gson.stream.JsonWriter;
import com.plaid.client.model.ACHClass;
import com.plaid.client.model.TransferRecurringNetwork;
import com.plaid.client.model.TransferRecurringSchedule;
import com.plaid.client.model.TransferRecurringStatus;
import com.plaid.client.model.TransferType;
import com.plaid.client.model.TransferUserInResponse;
import io.swagger.annotations.ApiModel;
import io.swagger.annotations.ApiModelProperty;
import java.io.IOException;
import java.time.LocalDate;
import java.time.OffsetDateTime;
import java.util.ArrayList;
import java.util.List;
/**
* Represents a recurring transfer within the Transfers API.
*/
@ApiModel(description = "Represents a recurring transfer within the Transfers API.")
@javax.annotation.Generated(value = "org.openapitools.codegen.languages.JavaClientCodegen", date = "2024-07-05T19:36:13.426392Z[Etc/UTC]")
public class RecurringTransfer {
public static final String SERIALIZED_NAME_RECURRING_TRANSFER_ID = "recurring_transfer_id";
@SerializedName(SERIALIZED_NAME_RECURRING_TRANSFER_ID)
private String recurringTransferId;
public static final String SERIALIZED_NAME_CREATED = "created";
@SerializedName(SERIALIZED_NAME_CREATED)
private OffsetDateTime created;
public static final String SERIALIZED_NAME_NEXT_ORIGINATION_DATE = "next_origination_date";
@SerializedName(SERIALIZED_NAME_NEXT_ORIGINATION_DATE)
private LocalDate nextOriginationDate;
public static final String SERIALIZED_NAME_TEST_CLOCK_ID = "test_clock_id";
@SerializedName(SERIALIZED_NAME_TEST_CLOCK_ID)
private String testClockId;
public static final String SERIALIZED_NAME_TYPE = "type";
@SerializedName(SERIALIZED_NAME_TYPE)
private TransferType type;
public static final String SERIALIZED_NAME_AMOUNT = "amount";
@SerializedName(SERIALIZED_NAME_AMOUNT)
private String amount;
public static final String SERIALIZED_NAME_STATUS = "status";
@SerializedName(SERIALIZED_NAME_STATUS)
private TransferRecurringStatus status;
public static final String SERIALIZED_NAME_ACH_CLASS = "ach_class";
@SerializedName(SERIALIZED_NAME_ACH_CLASS)
private ACHClass achClass;
public static final String SERIALIZED_NAME_NETWORK = "network";
@SerializedName(SERIALIZED_NAME_NETWORK)
private TransferRecurringNetwork network;
public static final String SERIALIZED_NAME_ORIGINATION_ACCOUNT_ID = "origination_account_id";
@SerializedName(SERIALIZED_NAME_ORIGINATION_ACCOUNT_ID)
private String originationAccountId;
public static final String SERIALIZED_NAME_ACCOUNT_ID = "account_id";
@SerializedName(SERIALIZED_NAME_ACCOUNT_ID)
private String accountId;
public static final String SERIALIZED_NAME_FUNDING_ACCOUNT_ID = "funding_account_id";
@SerializedName(SERIALIZED_NAME_FUNDING_ACCOUNT_ID)
private String fundingAccountId;
public static final String SERIALIZED_NAME_ISO_CURRENCY_CODE = "iso_currency_code";
@SerializedName(SERIALIZED_NAME_ISO_CURRENCY_CODE)
private String isoCurrencyCode;
public static final String SERIALIZED_NAME_DESCRIPTION = "description";
@SerializedName(SERIALIZED_NAME_DESCRIPTION)
private String description;
public static final String SERIALIZED_NAME_TRANSFER_IDS = "transfer_ids";
@SerializedName(SERIALIZED_NAME_TRANSFER_IDS)
private List transferIds = new ArrayList<>();
public static final String SERIALIZED_NAME_USER = "user";
@SerializedName(SERIALIZED_NAME_USER)
private TransferUserInResponse user;
public static final String SERIALIZED_NAME_SCHEDULE = "schedule";
@SerializedName(SERIALIZED_NAME_SCHEDULE)
private TransferRecurringSchedule schedule;
public RecurringTransfer recurringTransferId(String recurringTransferId) {
this.recurringTransferId = recurringTransferId;
return this;
}
/**
* Plaid’s unique identifier for a recurring transfer.
* @return recurringTransferId
**/
@ApiModelProperty(required = true, value = "Plaid’s unique identifier for a recurring transfer.")
public String getRecurringTransferId() {
return recurringTransferId;
}
public void setRecurringTransferId(String recurringTransferId) {
this.recurringTransferId = recurringTransferId;
}
public RecurringTransfer created(OffsetDateTime created) {
this.created = created;
return this;
}
/**
* The datetime when this transfer was created. This will be of the form `2006-01-02T15:04:05Z`
* @return created
**/
@ApiModelProperty(required = true, value = "The datetime when this transfer was created. This will be of the form `2006-01-02T15:04:05Z`")
public OffsetDateTime getCreated() {
return created;
}
public void setCreated(OffsetDateTime created) {
this.created = created;
}
public RecurringTransfer nextOriginationDate(LocalDate nextOriginationDate) {
this.nextOriginationDate = nextOriginationDate;
return this;
}
/**
* A date in [ISO 8601](https://wikipedia.org/wiki/ISO_8601) format (YYYY-MM-DD). The next transfer origination date after bank holiday adjustment.
* @return nextOriginationDate
**/
@javax.annotation.Nullable
@ApiModelProperty(required = true, value = "A date in [ISO 8601](https://wikipedia.org/wiki/ISO_8601) format (YYYY-MM-DD). The next transfer origination date after bank holiday adjustment.")
public LocalDate getNextOriginationDate() {
return nextOriginationDate;
}
public void setNextOriginationDate(LocalDate nextOriginationDate) {
this.nextOriginationDate = nextOriginationDate;
}
public RecurringTransfer testClockId(String testClockId) {
this.testClockId = testClockId;
return this;
}
/**
* Plaid’s unique identifier for a test clock.
* @return testClockId
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Plaid’s unique identifier for a test clock.")
public String getTestClockId() {
return testClockId;
}
public void setTestClockId(String testClockId) {
this.testClockId = testClockId;
}
public RecurringTransfer type(TransferType type) {
this.type = type;
return this;
}
/**
* Get type
* @return type
**/
@ApiModelProperty(required = true, value = "")
public TransferType getType() {
return type;
}
public void setType(TransferType type) {
this.type = type;
}
public RecurringTransfer amount(String amount) {
this.amount = amount;
return this;
}
/**
* The amount of the transfer (decimal string with two digits of precision e.g. \"10.00\"). When calling `/transfer/authorization/create`, specify the maximum amount to authorize. When calling `/transfer/create`, specify the exact amount of the transfer, up to a maximum of the amount authorized. If this field is left blank when calling `/transfer/create`, the maximum amount authorized in the `authorization_id` will be sent.
* @return amount
**/
@ApiModelProperty(required = true, value = "The amount of the transfer (decimal string with two digits of precision e.g. \"10.00\"). When calling `/transfer/authorization/create`, specify the maximum amount to authorize. When calling `/transfer/create`, specify the exact amount of the transfer, up to a maximum of the amount authorized. If this field is left blank when calling `/transfer/create`, the maximum amount authorized in the `authorization_id` will be sent.")
public String getAmount() {
return amount;
}
public void setAmount(String amount) {
this.amount = amount;
}
public RecurringTransfer status(TransferRecurringStatus status) {
this.status = status;
return this;
}
/**
* Get status
* @return status
**/
@ApiModelProperty(required = true, value = "")
public TransferRecurringStatus getStatus() {
return status;
}
public void setStatus(TransferRecurringStatus status) {
this.status = status;
}
public RecurringTransfer achClass(ACHClass achClass) {
this.achClass = achClass;
return this;
}
/**
* Get achClass
* @return achClass
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
public ACHClass getAchClass() {
return achClass;
}
public void setAchClass(ACHClass achClass) {
this.achClass = achClass;
}
public RecurringTransfer network(TransferRecurringNetwork network) {
this.network = network;
return this;
}
/**
* Get network
* @return network
**/
@ApiModelProperty(required = true, value = "")
public TransferRecurringNetwork getNetwork() {
return network;
}
public void setNetwork(TransferRecurringNetwork network) {
this.network = network;
}
public RecurringTransfer originationAccountId(String originationAccountId) {
this.originationAccountId = originationAccountId;
return this;
}
/**
* Plaid’s unique identifier for the origination account that was used for this transfer.
* @return originationAccountId
**/
@ApiModelProperty(required = true, value = "Plaid’s unique identifier for the origination account that was used for this transfer.")
public String getOriginationAccountId() {
return originationAccountId;
}
public void setOriginationAccountId(String originationAccountId) {
this.originationAccountId = originationAccountId;
}
public RecurringTransfer accountId(String accountId) {
this.accountId = accountId;
return this;
}
/**
* The Plaid `account_id` corresponding to the end-user account that will be debited or credited.
* @return accountId
**/
@ApiModelProperty(required = true, value = "The Plaid `account_id` corresponding to the end-user account that will be debited or credited.")
public String getAccountId() {
return accountId;
}
public void setAccountId(String accountId) {
this.accountId = accountId;
}
public RecurringTransfer fundingAccountId(String fundingAccountId) {
this.fundingAccountId = fundingAccountId;
return this;
}
/**
* The id of the funding account to use, available in the Plaid Dashboard. This determines which of your business checking accounts will be credited or debited.
* @return fundingAccountId
**/
@ApiModelProperty(required = true, value = "The id of the funding account to use, available in the Plaid Dashboard. This determines which of your business checking accounts will be credited or debited.")
public String getFundingAccountId() {
return fundingAccountId;
}
public void setFundingAccountId(String fundingAccountId) {
this.fundingAccountId = fundingAccountId;
}
public RecurringTransfer isoCurrencyCode(String isoCurrencyCode) {
this.isoCurrencyCode = isoCurrencyCode;
return this;
}
/**
* The currency of the transfer amount, e.g. \"USD\"
* @return isoCurrencyCode
**/
@ApiModelProperty(required = true, value = "The currency of the transfer amount, e.g. \"USD\"")
public String getIsoCurrencyCode() {
return isoCurrencyCode;
}
public void setIsoCurrencyCode(String isoCurrencyCode) {
this.isoCurrencyCode = isoCurrencyCode;
}
public RecurringTransfer description(String description) {
this.description = description;
return this;
}
/**
* The description of the recurring transfer.
* @return description
**/
@ApiModelProperty(required = true, value = "The description of the recurring transfer.")
public String getDescription() {
return description;
}
public void setDescription(String description) {
this.description = description;
}
public RecurringTransfer transferIds(List transferIds) {
this.transferIds = transferIds;
return this;
}
public RecurringTransfer addTransferIdsItem(String transferIdsItem) {
this.transferIds.add(transferIdsItem);
return this;
}
/**
* Get transferIds
* @return transferIds
**/
@ApiModelProperty(required = true, value = "")
public List getTransferIds() {
return transferIds;
}
public void setTransferIds(List transferIds) {
this.transferIds = transferIds;
}
public RecurringTransfer user(TransferUserInResponse user) {
this.user = user;
return this;
}
/**
* Get user
* @return user
**/
@ApiModelProperty(required = true, value = "")
public TransferUserInResponse getUser() {
return user;
}
public void setUser(TransferUserInResponse user) {
this.user = user;
}
public RecurringTransfer schedule(TransferRecurringSchedule schedule) {
this.schedule = schedule;
return this;
}
/**
* Get schedule
* @return schedule
**/
@ApiModelProperty(required = true, value = "")
public TransferRecurringSchedule getSchedule() {
return schedule;
}
public void setSchedule(TransferRecurringSchedule schedule) {
this.schedule = schedule;
}
@Override
public boolean equals(Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
RecurringTransfer recurringTransfer = (RecurringTransfer) o;
return Objects.equals(this.recurringTransferId, recurringTransfer.recurringTransferId) &&
Objects.equals(this.created, recurringTransfer.created) &&
Objects.equals(this.nextOriginationDate, recurringTransfer.nextOriginationDate) &&
Objects.equals(this.testClockId, recurringTransfer.testClockId) &&
Objects.equals(this.type, recurringTransfer.type) &&
Objects.equals(this.amount, recurringTransfer.amount) &&
Objects.equals(this.status, recurringTransfer.status) &&
Objects.equals(this.achClass, recurringTransfer.achClass) &&
Objects.equals(this.network, recurringTransfer.network) &&
Objects.equals(this.originationAccountId, recurringTransfer.originationAccountId) &&
Objects.equals(this.accountId, recurringTransfer.accountId) &&
Objects.equals(this.fundingAccountId, recurringTransfer.fundingAccountId) &&
Objects.equals(this.isoCurrencyCode, recurringTransfer.isoCurrencyCode) &&
Objects.equals(this.description, recurringTransfer.description) &&
Objects.equals(this.transferIds, recurringTransfer.transferIds) &&
Objects.equals(this.user, recurringTransfer.user) &&
Objects.equals(this.schedule, recurringTransfer.schedule);
}
@Override
public int hashCode() {
return Objects.hash(recurringTransferId, created, nextOriginationDate, testClockId, type, amount, status, achClass, network, originationAccountId, accountId, fundingAccountId, isoCurrencyCode, description, transferIds, user, schedule);
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class RecurringTransfer {\n");
sb.append(" recurringTransferId: ").append(toIndentedString(recurringTransferId)).append("\n");
sb.append(" created: ").append(toIndentedString(created)).append("\n");
sb.append(" nextOriginationDate: ").append(toIndentedString(nextOriginationDate)).append("\n");
sb.append(" testClockId: ").append(toIndentedString(testClockId)).append("\n");
sb.append(" type: ").append(toIndentedString(type)).append("\n");
sb.append(" amount: ").append(toIndentedString(amount)).append("\n");
sb.append(" status: ").append(toIndentedString(status)).append("\n");
sb.append(" achClass: ").append(toIndentedString(achClass)).append("\n");
sb.append(" network: ").append(toIndentedString(network)).append("\n");
sb.append(" originationAccountId: ").append(toIndentedString(originationAccountId)).append("\n");
sb.append(" accountId: ").append(toIndentedString(accountId)).append("\n");
sb.append(" fundingAccountId: ").append(toIndentedString(fundingAccountId)).append("\n");
sb.append(" isoCurrencyCode: ").append(toIndentedString(isoCurrencyCode)).append("\n");
sb.append(" description: ").append(toIndentedString(description)).append("\n");
sb.append(" transferIds: ").append(toIndentedString(transferIds)).append("\n");
sb.append(" user: ").append(toIndentedString(user)).append("\n");
sb.append(" schedule: ").append(toIndentedString(schedule)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces
* (except the first line).
*/
private String toIndentedString(Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy