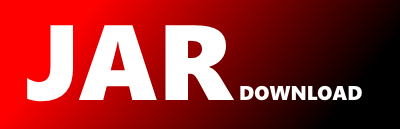
com.plaid.client.model.TransferAuthorizationDecision Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of plaid-java Show documentation
Show all versions of plaid-java Show documentation
Bindings for the Plaid (plaid.com) API.
/*
* The Plaid API
* The Plaid REST API. Please see https://plaid.com/docs/api for more details.
*
* The version of the OpenAPI document: 2020-09-14_1.534.3
*
*
* NOTE: This class is auto generated by OpenAPI Generator (https://openapi-generator.tech).
* https://openapi-generator.tech
* Do not edit the class manually.
*/
package com.plaid.client.model;
import java.util.Objects;
import java.util.Arrays;
import io.swagger.annotations.ApiModel;
import com.google.gson.annotations.SerializedName;
import java.io.IOException;
import com.google.gson.TypeAdapter;
import com.google.gson.annotations.JsonAdapter;
import com.google.gson.stream.JsonReader;
import com.google.gson.stream.JsonWriter;
/**
* A decision regarding the proposed transfer. `approved` – The proposed transfer has received the end user's consent and has been approved for processing by Plaid. The `decision_rationale` field is set if Plaid was unable to fetch the account information. You may proceed with the transfer, but further review is recommended (i.e., use Link in update mode to re-authenticate your user when `decision_rationale.code` is `ITEM_LOGIN_REQUIRED`). Refer to the `code` field in the `decision_rationale` object for details. `declined` – Plaid reviewed the proposed transfer and declined processing. Refer to the `code` field in the `decision_rationale` object for details. `user_action_required` – An action is required before Plaid can assess the transfer risk and make a decision. The most common scenario is to update authentication for an Item. To complete the required action, initialize Link by setting `transfer.authorization_id` in the request of `/link/token/create`.
*/
@JsonAdapter(TransferAuthorizationDecision.Adapter.class)
public enum TransferAuthorizationDecision {
APPROVED("approved"),
DECLINED("declined"),
USER_ACTION_REQUIRED("user_action_required"),
// This is returned when an enum is returned from the API that doesn't exist in the OpenAPI file.
// Try upgrading your client-library version.
ENUM_UNKNOWN("ENUM_UNKNOWN");
private String value;
TransferAuthorizationDecision(String value) {
this.value = value;
}
public String getValue() {
return value;
}
@Override
public String toString() {
return String.valueOf(value);
}
public static TransferAuthorizationDecision fromValue(String value) {
for (TransferAuthorizationDecision b : TransferAuthorizationDecision.values()) {
if (b.value.equals(value)) {
return b;
}
}
return TransferAuthorizationDecision.ENUM_UNKNOWN;
}
public static class Adapter extends TypeAdapter {
@Override
public void write(final JsonWriter jsonWriter, final TransferAuthorizationDecision enumeration) throws IOException {
jsonWriter.value(enumeration.getValue());
}
@Override
public TransferAuthorizationDecision read(final JsonReader jsonReader) throws IOException {
String value = jsonReader.nextString();
return TransferAuthorizationDecision.fromValue(value);
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy