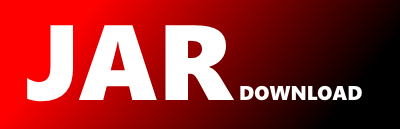
com.plaid.client.model.UserPermissionRevokedWebhook Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of plaid-java Show documentation
Show all versions of plaid-java Show documentation
Bindings for the Plaid (plaid.com) API.
/*
* The Plaid API
* The Plaid REST API. Please see https://plaid.com/docs/api for more details.
*
* The version of the OpenAPI document: 2020-09-14_1.534.3
*
*
* NOTE: This class is auto generated by OpenAPI Generator (https://openapi-generator.tech).
* https://openapi-generator.tech
* Do not edit the class manually.
*/
package com.plaid.client.model;
import java.util.Objects;
import java.util.Arrays;
import com.google.gson.TypeAdapter;
import com.google.gson.annotations.JsonAdapter;
import com.google.gson.annotations.SerializedName;
import com.google.gson.stream.JsonReader;
import com.google.gson.stream.JsonWriter;
import com.plaid.client.model.PlaidError;
import com.plaid.client.model.WebhookEnvironmentValues;
import io.swagger.annotations.ApiModel;
import io.swagger.annotations.ApiModelProperty;
import java.io.IOException;
/**
* The `USER_PERMISSION_REVOKED` webhook may be fired when an end user has used either the [my.plaid.com portal](https://my.plaid.com) or the financial institution’s OAuth consent portal to revoke the permission that they previously granted to access an Item. This webhook is not guaranteed to always be fired upon consent revocation, since some institutions’ consent portals do not trigger this webhook. Once access to an Item has been revoked, it cannot be restored. If the user subsequently returns to your application, a new Item must be created for the user. Upon receiving this webhook, it is recommended to call `/item/remove` to delete the underlying Item and to delete any stored data from Plaid associated with the Item. Note that when using ACH flows with Chase Items specifically, the account number provided by Plaid will no longer work for creating transfers once user permission has been revoked. If you receive this webhook for a Chase Item, you should not create any new ACH transfers for that Item, as they will be returned.
*/
@ApiModel(description = "The `USER_PERMISSION_REVOKED` webhook may be fired when an end user has used either the [my.plaid.com portal](https://my.plaid.com) or the financial institution’s OAuth consent portal to revoke the permission that they previously granted to access an Item. This webhook is not guaranteed to always be fired upon consent revocation, since some institutions’ consent portals do not trigger this webhook. Once access to an Item has been revoked, it cannot be restored. If the user subsequently returns to your application, a new Item must be created for the user. Upon receiving this webhook, it is recommended to call `/item/remove` to delete the underlying Item and to delete any stored data from Plaid associated with the Item. Note that when using ACH flows with Chase Items specifically, the account number provided by Plaid will no longer work for creating transfers once user permission has been revoked. If you receive this webhook for a Chase Item, you should not create any new ACH transfers for that Item, as they will be returned.")
@javax.annotation.Generated(value = "org.openapitools.codegen.languages.JavaClientCodegen", date = "2024-07-05T19:36:13.426392Z[Etc/UTC]")
public class UserPermissionRevokedWebhook {
public static final String SERIALIZED_NAME_WEBHOOK_TYPE = "webhook_type";
@SerializedName(SERIALIZED_NAME_WEBHOOK_TYPE)
private String webhookType;
public static final String SERIALIZED_NAME_WEBHOOK_CODE = "webhook_code";
@SerializedName(SERIALIZED_NAME_WEBHOOK_CODE)
private String webhookCode;
public static final String SERIALIZED_NAME_ITEM_ID = "item_id";
@SerializedName(SERIALIZED_NAME_ITEM_ID)
private String itemId;
public static final String SERIALIZED_NAME_ERROR = "error";
@SerializedName(SERIALIZED_NAME_ERROR)
private PlaidError error;
public static final String SERIALIZED_NAME_ENVIRONMENT = "environment";
@SerializedName(SERIALIZED_NAME_ENVIRONMENT)
private WebhookEnvironmentValues environment;
public UserPermissionRevokedWebhook webhookType(String webhookType) {
this.webhookType = webhookType;
return this;
}
/**
* `ITEM`
* @return webhookType
**/
@ApiModelProperty(required = true, value = "`ITEM`")
public String getWebhookType() {
return webhookType;
}
public void setWebhookType(String webhookType) {
this.webhookType = webhookType;
}
public UserPermissionRevokedWebhook webhookCode(String webhookCode) {
this.webhookCode = webhookCode;
return this;
}
/**
* `USER_PERMISSION_REVOKED`
* @return webhookCode
**/
@ApiModelProperty(required = true, value = "`USER_PERMISSION_REVOKED`")
public String getWebhookCode() {
return webhookCode;
}
public void setWebhookCode(String webhookCode) {
this.webhookCode = webhookCode;
}
public UserPermissionRevokedWebhook itemId(String itemId) {
this.itemId = itemId;
return this;
}
/**
* The `item_id` of the Item associated with this webhook, warning, or error
* @return itemId
**/
@ApiModelProperty(required = true, value = "The `item_id` of the Item associated with this webhook, warning, or error")
public String getItemId() {
return itemId;
}
public void setItemId(String itemId) {
this.itemId = itemId;
}
public UserPermissionRevokedWebhook error(PlaidError error) {
this.error = error;
return this;
}
/**
* Get error
* @return error
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
public PlaidError getError() {
return error;
}
public void setError(PlaidError error) {
this.error = error;
}
public UserPermissionRevokedWebhook environment(WebhookEnvironmentValues environment) {
this.environment = environment;
return this;
}
/**
* Get environment
* @return environment
**/
@ApiModelProperty(required = true, value = "")
public WebhookEnvironmentValues getEnvironment() {
return environment;
}
public void setEnvironment(WebhookEnvironmentValues environment) {
this.environment = environment;
}
@Override
public boolean equals(Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
UserPermissionRevokedWebhook userPermissionRevokedWebhook = (UserPermissionRevokedWebhook) o;
return Objects.equals(this.webhookType, userPermissionRevokedWebhook.webhookType) &&
Objects.equals(this.webhookCode, userPermissionRevokedWebhook.webhookCode) &&
Objects.equals(this.itemId, userPermissionRevokedWebhook.itemId) &&
Objects.equals(this.error, userPermissionRevokedWebhook.error) &&
Objects.equals(this.environment, userPermissionRevokedWebhook.environment);
}
@Override
public int hashCode() {
return Objects.hash(webhookType, webhookCode, itemId, error, environment);
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class UserPermissionRevokedWebhook {\n");
sb.append(" webhookType: ").append(toIndentedString(webhookType)).append("\n");
sb.append(" webhookCode: ").append(toIndentedString(webhookCode)).append("\n");
sb.append(" itemId: ").append(toIndentedString(itemId)).append("\n");
sb.append(" error: ").append(toIndentedString(error)).append("\n");
sb.append(" environment: ").append(toIndentedString(environment)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces
* (except the first line).
*/
private String toIndentedString(Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy