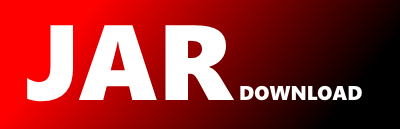
com.plaid.client.model.AssetReportCreateRequestOptions Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of plaid-java Show documentation
Show all versions of plaid-java Show documentation
Bindings for the Plaid (plaid.com) API.
/*
* The Plaid API
* The Plaid REST API. Please see https://plaid.com/docs/api for more details.
*
* The version of the OpenAPI document: 2020-09-14_1.565.0
*
*
* NOTE: This class is auto generated by OpenAPI Generator (https://openapi-generator.tech).
* https://openapi-generator.tech
* Do not edit the class manually.
*/
package com.plaid.client.model;
import java.util.Objects;
import java.util.Arrays;
import com.google.gson.TypeAdapter;
import com.google.gson.annotations.JsonAdapter;
import com.google.gson.annotations.SerializedName;
import com.google.gson.stream.JsonReader;
import com.google.gson.stream.JsonWriter;
import com.plaid.client.model.AssetReportAddOns;
import com.plaid.client.model.AssetReportUser;
import io.swagger.annotations.ApiModel;
import io.swagger.annotations.ApiModelProperty;
import java.io.IOException;
import java.util.ArrayList;
import java.util.List;
/**
* An optional object to filter `/asset_report/create` results. If provided, must be non-`null`. The optional `user` object is required for the report to be eligible for Fannie Mae's Day 1 Certainty program.
*/
@ApiModel(description = "An optional object to filter `/asset_report/create` results. If provided, must be non-`null`. The optional `user` object is required for the report to be eligible for Fannie Mae's Day 1 Certainty program.")
@javax.annotation.Generated(value = "org.openapitools.codegen.languages.JavaClientCodegen", date = "2024-09-17T16:42:02.150702Z[Etc/UTC]")
public class AssetReportCreateRequestOptions {
public static final String SERIALIZED_NAME_CLIENT_REPORT_ID = "client_report_id";
@SerializedName(SERIALIZED_NAME_CLIENT_REPORT_ID)
private String clientReportId;
public static final String SERIALIZED_NAME_WEBHOOK = "webhook";
@SerializedName(SERIALIZED_NAME_WEBHOOK)
private String webhook;
public static final String SERIALIZED_NAME_INCLUDE_FAST_REPORT = "include_fast_report";
@SerializedName(SERIALIZED_NAME_INCLUDE_FAST_REPORT)
private Boolean includeFastReport;
public static final String SERIALIZED_NAME_PRODUCTS = "products";
@SerializedName(SERIALIZED_NAME_PRODUCTS)
private List products = null;
public static final String SERIALIZED_NAME_ADD_ONS = "add_ons";
@SerializedName(SERIALIZED_NAME_ADD_ONS)
private List addOns = null;
public static final String SERIALIZED_NAME_USER = "user";
@SerializedName(SERIALIZED_NAME_USER)
private AssetReportUser user;
public static final String SERIALIZED_NAME_REQUIRE_ALL_ITEMS = "require_all_items";
@SerializedName(SERIALIZED_NAME_REQUIRE_ALL_ITEMS)
private Boolean requireAllItems = true;
public AssetReportCreateRequestOptions clientReportId(String clientReportId) {
this.clientReportId = clientReportId;
return this;
}
/**
* Client-generated identifier, which can be used by lenders to track loan applications.
* @return clientReportId
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Client-generated identifier, which can be used by lenders to track loan applications.")
public String getClientReportId() {
return clientReportId;
}
public void setClientReportId(String clientReportId) {
this.clientReportId = clientReportId;
}
public AssetReportCreateRequestOptions webhook(String webhook) {
this.webhook = webhook;
return this;
}
/**
* URL to which Plaid will send Assets webhooks, for example when the requested Asset Report is ready.
* @return webhook
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "URL to which Plaid will send Assets webhooks, for example when the requested Asset Report is ready.")
public String getWebhook() {
return webhook;
}
public void setWebhook(String webhook) {
this.webhook = webhook;
}
public AssetReportCreateRequestOptions includeFastReport(Boolean includeFastReport) {
this.includeFastReport = includeFastReport;
return this;
}
/**
* true to return balance and identity earlier as a fast report. Defaults to false if omitted.
* @return includeFastReport
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "true to return balance and identity earlier as a fast report. Defaults to false if omitted.")
public Boolean getIncludeFastReport() {
return includeFastReport;
}
public void setIncludeFastReport(Boolean includeFastReport) {
this.includeFastReport = includeFastReport;
}
public AssetReportCreateRequestOptions products(List products) {
this.products = products;
return this;
}
public AssetReportCreateRequestOptions addProductsItem(String productsItem) {
if (this.products == null) {
this.products = new ArrayList<>();
}
this.products.add(productsItem);
return this;
}
/**
* Additional information that can be included in the asset report. Possible values: `\"investments\"`
* @return products
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Additional information that can be included in the asset report. Possible values: `\"investments\"`")
public List getProducts() {
return products;
}
public void setProducts(List products) {
this.products = products;
}
public AssetReportCreateRequestOptions addOns(List addOns) {
this.addOns = addOns;
return this;
}
public AssetReportCreateRequestOptions addAddOnsItem(AssetReportAddOns addOnsItem) {
if (this.addOns == null) {
this.addOns = new ArrayList<>();
}
this.addOns.add(addOnsItem);
return this;
}
/**
* Use this field to request a `fast_asset` report. When Fast Assets is requested, Plaid will create two versions of the Asset Report: first, the Fast Asset Report, which will contain only current identity and balance information, and later, the Full Asset Report, which will also contain historical balance information and transaction data. A `PRODUCT_READY` webhook will be fired for each Asset Report when it is ready, and the `report_type` field will indicate whether the webhook is firing for the `full` or `fast` Asset Report. To retrieve the Fast Asset Report, call `/asset_report/get` with `fast_report` set to `true`. There is no additional charge for using Fast Assets.
* @return addOns
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Use this field to request a `fast_asset` report. When Fast Assets is requested, Plaid will create two versions of the Asset Report: first, the Fast Asset Report, which will contain only current identity and balance information, and later, the Full Asset Report, which will also contain historical balance information and transaction data. A `PRODUCT_READY` webhook will be fired for each Asset Report when it is ready, and the `report_type` field will indicate whether the webhook is firing for the `full` or `fast` Asset Report. To retrieve the Fast Asset Report, call `/asset_report/get` with `fast_report` set to `true`. There is no additional charge for using Fast Assets.")
public List getAddOns() {
return addOns;
}
public void setAddOns(List addOns) {
this.addOns = addOns;
}
public AssetReportCreateRequestOptions user(AssetReportUser user) {
this.user = user;
return this;
}
/**
* Get user
* @return user
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
public AssetReportUser getUser() {
return user;
}
public void setUser(AssetReportUser user) {
this.user = user;
}
public AssetReportCreateRequestOptions requireAllItems(Boolean requireAllItems) {
this.requireAllItems = requireAllItems;
return this;
}
/**
* If set to false, only 1 item must be healthy at the time of report creation. The default value is true, which would require all items to be healthy at the time of report creation.
* @return requireAllItems
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "If set to false, only 1 item must be healthy at the time of report creation. The default value is true, which would require all items to be healthy at the time of report creation.")
public Boolean getRequireAllItems() {
return requireAllItems;
}
public void setRequireAllItems(Boolean requireAllItems) {
this.requireAllItems = requireAllItems;
}
@Override
public boolean equals(Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
AssetReportCreateRequestOptions assetReportCreateRequestOptions = (AssetReportCreateRequestOptions) o;
return Objects.equals(this.clientReportId, assetReportCreateRequestOptions.clientReportId) &&
Objects.equals(this.webhook, assetReportCreateRequestOptions.webhook) &&
Objects.equals(this.includeFastReport, assetReportCreateRequestOptions.includeFastReport) &&
Objects.equals(this.products, assetReportCreateRequestOptions.products) &&
Objects.equals(this.addOns, assetReportCreateRequestOptions.addOns) &&
Objects.equals(this.user, assetReportCreateRequestOptions.user) &&
Objects.equals(this.requireAllItems, assetReportCreateRequestOptions.requireAllItems);
}
@Override
public int hashCode() {
return Objects.hash(clientReportId, webhook, includeFastReport, products, addOns, user, requireAllItems);
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class AssetReportCreateRequestOptions {\n");
sb.append(" clientReportId: ").append(toIndentedString(clientReportId)).append("\n");
sb.append(" webhook: ").append(toIndentedString(webhook)).append("\n");
sb.append(" includeFastReport: ").append(toIndentedString(includeFastReport)).append("\n");
sb.append(" products: ").append(toIndentedString(products)).append("\n");
sb.append(" addOns: ").append(toIndentedString(addOns)).append("\n");
sb.append(" user: ").append(toIndentedString(user)).append("\n");
sb.append(" requireAllItems: ").append(toIndentedString(requireAllItems)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces
* (except the first line).
*/
private String toIndentedString(Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy