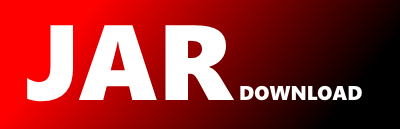
com.plaid.client.model.BeaconUserUpdateRequest Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of plaid-java Show documentation
Show all versions of plaid-java Show documentation
Bindings for the Plaid (plaid.com) API.
/*
* The Plaid API
* The Plaid REST API. Please see https://plaid.com/docs/api for more details.
*
* The version of the OpenAPI document: 2020-09-14_1.565.0
*
*
* NOTE: This class is auto generated by OpenAPI Generator (https://openapi-generator.tech).
* https://openapi-generator.tech
* Do not edit the class manually.
*/
package com.plaid.client.model;
import java.util.Objects;
import java.util.Arrays;
import com.google.gson.TypeAdapter;
import com.google.gson.annotations.JsonAdapter;
import com.google.gson.annotations.SerializedName;
import com.google.gson.stream.JsonReader;
import com.google.gson.stream.JsonWriter;
import com.plaid.client.model.BeaconUserUpdateRequestData;
import io.swagger.annotations.ApiModel;
import io.swagger.annotations.ApiModelProperty;
import java.io.IOException;
import java.util.ArrayList;
import java.util.List;
/**
* Request input for updating the identity data of a Beacon User.
*/
@ApiModel(description = "Request input for updating the identity data of a Beacon User.")
@javax.annotation.Generated(value = "org.openapitools.codegen.languages.JavaClientCodegen", date = "2024-09-17T16:42:02.150702Z[Etc/UTC]")
public class BeaconUserUpdateRequest {
public static final String SERIALIZED_NAME_BEACON_USER_ID = "beacon_user_id";
@SerializedName(SERIALIZED_NAME_BEACON_USER_ID)
private String beaconUserId;
public static final String SERIALIZED_NAME_USER = "user";
@SerializedName(SERIALIZED_NAME_USER)
private BeaconUserUpdateRequestData user;
public static final String SERIALIZED_NAME_ACCESS_TOKENS = "access_tokens";
@SerializedName(SERIALIZED_NAME_ACCESS_TOKENS)
private List accessTokens = null;
public static final String SERIALIZED_NAME_CLIENT_ID = "client_id";
@SerializedName(SERIALIZED_NAME_CLIENT_ID)
private String clientId;
public static final String SERIALIZED_NAME_SECRET = "secret";
@SerializedName(SERIALIZED_NAME_SECRET)
private String secret;
public BeaconUserUpdateRequest beaconUserId(String beaconUserId) {
this.beaconUserId = beaconUserId;
return this;
}
/**
* ID of the associated Beacon User.
* @return beaconUserId
**/
@ApiModelProperty(example = "becusr_42cF1MNo42r9Xj", required = true, value = "ID of the associated Beacon User.")
public String getBeaconUserId() {
return beaconUserId;
}
public void setBeaconUserId(String beaconUserId) {
this.beaconUserId = beaconUserId;
}
public BeaconUserUpdateRequest user(BeaconUserUpdateRequestData user) {
this.user = user;
return this;
}
/**
* Get user
* @return user
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
public BeaconUserUpdateRequestData getUser() {
return user;
}
public void setUser(BeaconUserUpdateRequestData user) {
this.user = user;
}
public BeaconUserUpdateRequest accessTokens(List accessTokens) {
this.accessTokens = accessTokens;
return this;
}
public BeaconUserUpdateRequest addAccessTokensItem(String accessTokensItem) {
if (this.accessTokens == null) {
this.accessTokens = new ArrayList<>();
}
this.accessTokens.add(accessTokensItem);
return this;
}
/**
* Send this array of access tokens to add accounts to this user for evaluation. This will add accounts to this Beacon User. If left null only existing accounts will be returned in response. A maximum of 50 accounts total can be added to a Beacon User.
* @return accessTokens
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Send this array of access tokens to add accounts to this user for evaluation. This will add accounts to this Beacon User. If left null only existing accounts will be returned in response. A maximum of 50 accounts total can be added to a Beacon User.")
public List getAccessTokens() {
return accessTokens;
}
public void setAccessTokens(List accessTokens) {
this.accessTokens = accessTokens;
}
public BeaconUserUpdateRequest clientId(String clientId) {
this.clientId = clientId;
return this;
}
/**
* Your Plaid API `client_id`. The `client_id` is required and may be provided either in the `PLAID-CLIENT-ID` header or as part of a request body.
* @return clientId
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Your Plaid API `client_id`. The `client_id` is required and may be provided either in the `PLAID-CLIENT-ID` header or as part of a request body.")
public String getClientId() {
return clientId;
}
public void setClientId(String clientId) {
this.clientId = clientId;
}
public BeaconUserUpdateRequest secret(String secret) {
this.secret = secret;
return this;
}
/**
* Your Plaid API `secret`. The `secret` is required and may be provided either in the `PLAID-SECRET` header or as part of a request body.
* @return secret
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Your Plaid API `secret`. The `secret` is required and may be provided either in the `PLAID-SECRET` header or as part of a request body.")
public String getSecret() {
return secret;
}
public void setSecret(String secret) {
this.secret = secret;
}
@Override
public boolean equals(Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
BeaconUserUpdateRequest beaconUserUpdateRequest = (BeaconUserUpdateRequest) o;
return Objects.equals(this.beaconUserId, beaconUserUpdateRequest.beaconUserId) &&
Objects.equals(this.user, beaconUserUpdateRequest.user) &&
Objects.equals(this.accessTokens, beaconUserUpdateRequest.accessTokens) &&
Objects.equals(this.clientId, beaconUserUpdateRequest.clientId) &&
Objects.equals(this.secret, beaconUserUpdateRequest.secret);
}
@Override
public int hashCode() {
return Objects.hash(beaconUserId, user, accessTokens, clientId, secret);
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class BeaconUserUpdateRequest {\n");
sb.append(" beaconUserId: ").append(toIndentedString(beaconUserId)).append("\n");
sb.append(" user: ").append(toIndentedString(user)).append("\n");
sb.append(" accessTokens: ").append(toIndentedString(accessTokens)).append("\n");
sb.append(" clientId: ").append(toIndentedString(clientId)).append("\n");
sb.append(" secret: ").append(toIndentedString(secret)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces
* (except the first line).
*/
private String toIndentedString(Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy