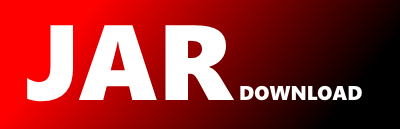
com.plaid.client.model.ForecastedMonthlyIncome Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of plaid-java Show documentation
Show all versions of plaid-java Show documentation
Bindings for the Plaid (plaid.com) API.
/*
* The Plaid API
* The Plaid REST API. Please see https://plaid.com/docs/api for more details.
*
* The version of the OpenAPI document: 2020-09-14_1.565.0
*
*
* NOTE: This class is auto generated by OpenAPI Generator (https://openapi-generator.tech).
* https://openapi-generator.tech
* Do not edit the class manually.
*/
package com.plaid.client.model;
import java.util.Objects;
import java.util.Arrays;
import com.google.gson.TypeAdapter;
import com.google.gson.annotations.JsonAdapter;
import com.google.gson.annotations.SerializedName;
import com.google.gson.stream.JsonReader;
import com.google.gson.stream.JsonWriter;
import io.swagger.annotations.ApiModel;
import io.swagger.annotations.ApiModelProperty;
import java.io.IOException;
import java.math.BigDecimal;
/**
* An object representing the predicted average monthly net income amount. This amount reflects the funds deposited into the account and may not include any withheld income such as taxes or other payroll deductions
*/
@ApiModel(description = "An object representing the predicted average monthly net income amount. This amount reflects the funds deposited into the account and may not include any withheld income such as taxes or other payroll deductions")
@javax.annotation.Generated(value = "org.openapitools.codegen.languages.JavaClientCodegen", date = "2024-09-17T16:42:02.150702Z[Etc/UTC]")
public class ForecastedMonthlyIncome {
public static final String SERIALIZED_NAME_BASELINE_AMOUNT = "baseline_amount";
@SerializedName(SERIALIZED_NAME_BASELINE_AMOUNT)
private Double baselineAmount;
public static final String SERIALIZED_NAME_CURRENT_AMOUNT = "current_amount";
@SerializedName(SERIALIZED_NAME_CURRENT_AMOUNT)
private Double currentAmount;
public ForecastedMonthlyIncome baselineAmount(Double baselineAmount) {
this.baselineAmount = baselineAmount;
return this;
}
/**
* The forecasted monthly income at the time of subscription
* @return baselineAmount
**/
@ApiModelProperty(required = true, value = "The forecasted monthly income at the time of subscription")
public Double getBaselineAmount() {
return baselineAmount;
}
public void setBaselineAmount(Double baselineAmount) {
this.baselineAmount = baselineAmount;
}
public ForecastedMonthlyIncome currentAmount(Double currentAmount) {
this.currentAmount = currentAmount;
return this;
}
/**
* The current forecasted monthly income
* @return currentAmount
**/
@ApiModelProperty(required = true, value = "The current forecasted monthly income")
public Double getCurrentAmount() {
return currentAmount;
}
public void setCurrentAmount(Double currentAmount) {
this.currentAmount = currentAmount;
}
@Override
public boolean equals(Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
ForecastedMonthlyIncome forecastedMonthlyIncome = (ForecastedMonthlyIncome) o;
return Objects.equals(this.baselineAmount, forecastedMonthlyIncome.baselineAmount) &&
Objects.equals(this.currentAmount, forecastedMonthlyIncome.currentAmount);
}
@Override
public int hashCode() {
return Objects.hash(baselineAmount, currentAmount);
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class ForecastedMonthlyIncome {\n");
sb.append(" baselineAmount: ").append(toIndentedString(baselineAmount)).append("\n");
sb.append(" currentAmount: ").append(toIndentedString(currentAmount)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces
* (except the first line).
*/
private String toIndentedString(Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy