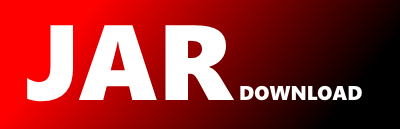
com.plaid.client.model.Institution Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of plaid-java Show documentation
Show all versions of plaid-java Show documentation
Bindings for the Plaid (plaid.com) API.
/*
* The Plaid API
* The Plaid REST API. Please see https://plaid.com/docs/api for more details.
*
* The version of the OpenAPI document: 2020-09-14_1.565.0
*
*
* NOTE: This class is auto generated by OpenAPI Generator (https://openapi-generator.tech).
* https://openapi-generator.tech
* Do not edit the class manually.
*/
package com.plaid.client.model;
import java.util.Objects;
import java.util.Arrays;
import com.google.gson.TypeAdapter;
import com.google.gson.annotations.JsonAdapter;
import com.google.gson.annotations.SerializedName;
import com.google.gson.stream.JsonReader;
import com.google.gson.stream.JsonWriter;
import com.plaid.client.model.AuthMetadata;
import com.plaid.client.model.CountryCode;
import com.plaid.client.model.InstitutionStatus;
import com.plaid.client.model.PaymentInitiationMetadata;
import com.plaid.client.model.Products;
import io.swagger.annotations.ApiModel;
import io.swagger.annotations.ApiModelProperty;
import java.io.IOException;
import java.util.ArrayList;
import java.util.List;
/**
* Details relating to a specific financial institution
*/
@ApiModel(description = "Details relating to a specific financial institution")
@javax.annotation.Generated(value = "org.openapitools.codegen.languages.JavaClientCodegen", date = "2024-09-17T16:42:02.150702Z[Etc/UTC]")
public class Institution {
public static final String SERIALIZED_NAME_INSTITUTION_ID = "institution_id";
@SerializedName(SERIALIZED_NAME_INSTITUTION_ID)
private String institutionId;
public static final String SERIALIZED_NAME_NAME = "name";
@SerializedName(SERIALIZED_NAME_NAME)
private String name;
public static final String SERIALIZED_NAME_PRODUCTS = "products";
@SerializedName(SERIALIZED_NAME_PRODUCTS)
private List products = new ArrayList<>();
public static final String SERIALIZED_NAME_COUNTRY_CODES = "country_codes";
@SerializedName(SERIALIZED_NAME_COUNTRY_CODES)
private List countryCodes = new ArrayList<>();
public static final String SERIALIZED_NAME_URL = "url";
@SerializedName(SERIALIZED_NAME_URL)
private String url;
public static final String SERIALIZED_NAME_PRIMARY_COLOR = "primary_color";
@SerializedName(SERIALIZED_NAME_PRIMARY_COLOR)
private String primaryColor;
public static final String SERIALIZED_NAME_LOGO = "logo";
@SerializedName(SERIALIZED_NAME_LOGO)
private String logo;
public static final String SERIALIZED_NAME_ROUTING_NUMBERS = "routing_numbers";
@SerializedName(SERIALIZED_NAME_ROUTING_NUMBERS)
private List routingNumbers = new ArrayList<>();
public static final String SERIALIZED_NAME_DTC_NUMBERS = "dtc_numbers";
@SerializedName(SERIALIZED_NAME_DTC_NUMBERS)
private List dtcNumbers = null;
public static final String SERIALIZED_NAME_OAUTH = "oauth";
@SerializedName(SERIALIZED_NAME_OAUTH)
private Boolean oauth;
public static final String SERIALIZED_NAME_STATUS = "status";
@SerializedName(SERIALIZED_NAME_STATUS)
private InstitutionStatus status;
public static final String SERIALIZED_NAME_PAYMENT_INITIATION_METADATA = "payment_initiation_metadata";
@SerializedName(SERIALIZED_NAME_PAYMENT_INITIATION_METADATA)
private PaymentInitiationMetadata paymentInitiationMetadata;
public static final String SERIALIZED_NAME_AUTH_METADATA = "auth_metadata";
@SerializedName(SERIALIZED_NAME_AUTH_METADATA)
private AuthMetadata authMetadata;
public Institution institutionId(String institutionId) {
this.institutionId = institutionId;
return this;
}
/**
* Unique identifier for the institution. Note that the same institution may have multiple records, each with different institution IDs; for example, if the institution has migrated to OAuth, there may be separate `institution_id`s for the OAuth and non-OAuth versions of the institution. Institutions that operate in different countries or with multiple login portals may also have separate `institution_id`s for each country or portal.
* @return institutionId
**/
@ApiModelProperty(required = true, value = "Unique identifier for the institution. Note that the same institution may have multiple records, each with different institution IDs; for example, if the institution has migrated to OAuth, there may be separate `institution_id`s for the OAuth and non-OAuth versions of the institution. Institutions that operate in different countries or with multiple login portals may also have separate `institution_id`s for each country or portal.")
public String getInstitutionId() {
return institutionId;
}
public void setInstitutionId(String institutionId) {
this.institutionId = institutionId;
}
public Institution name(String name) {
this.name = name;
return this;
}
/**
* The official name of the institution.
* @return name
**/
@ApiModelProperty(required = true, value = "The official name of the institution.")
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public Institution products(List products) {
this.products = products;
return this;
}
public Institution addProductsItem(Products productsItem) {
this.products.add(productsItem);
return this;
}
/**
* A list of the Plaid products supported by the institution. Note that only institutions that support Instant Auth will return `auth` in the product array; institutions that do not list `auth` may still support other Auth methods such as Instant Match or Automated Micro-deposit Verification. To identify institutions that support those methods, use the `auth_metadata` object. For more details, see [Full Auth coverage](https://plaid.com/docs/auth/coverage/). The `income_verification` product here indicates support for Bank Income.
* @return products
**/
@ApiModelProperty(required = true, value = "A list of the Plaid products supported by the institution. Note that only institutions that support Instant Auth will return `auth` in the product array; institutions that do not list `auth` may still support other Auth methods such as Instant Match or Automated Micro-deposit Verification. To identify institutions that support those methods, use the `auth_metadata` object. For more details, see [Full Auth coverage](https://plaid.com/docs/auth/coverage/). The `income_verification` product here indicates support for Bank Income.")
public List getProducts() {
return products;
}
public void setProducts(List products) {
this.products = products;
}
public Institution countryCodes(List countryCodes) {
this.countryCodes = countryCodes;
return this;
}
public Institution addCountryCodesItem(CountryCode countryCodesItem) {
this.countryCodes.add(countryCodesItem);
return this;
}
/**
* A list of the country codes supported by the institution.
* @return countryCodes
**/
@ApiModelProperty(required = true, value = "A list of the country codes supported by the institution.")
public List getCountryCodes() {
return countryCodes;
}
public void setCountryCodes(List countryCodes) {
this.countryCodes = countryCodes;
}
public Institution url(String url) {
this.url = url;
return this;
}
/**
* The URL for the institution's website
* @return url
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "The URL for the institution's website")
public String getUrl() {
return url;
}
public void setUrl(String url) {
this.url = url;
}
public Institution primaryColor(String primaryColor) {
this.primaryColor = primaryColor;
return this;
}
/**
* Hexadecimal representation of the primary color used by the institution
* @return primaryColor
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Hexadecimal representation of the primary color used by the institution")
public String getPrimaryColor() {
return primaryColor;
}
public void setPrimaryColor(String primaryColor) {
this.primaryColor = primaryColor;
}
public Institution logo(String logo) {
this.logo = logo;
return this;
}
/**
* Base64 encoded representation of the institution's logo, returned as a base64 encoded 152x152 PNG. Not all institutions' logos are available.
* @return logo
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Base64 encoded representation of the institution's logo, returned as a base64 encoded 152x152 PNG. Not all institutions' logos are available.")
public String getLogo() {
return logo;
}
public void setLogo(String logo) {
this.logo = logo;
}
public Institution routingNumbers(List routingNumbers) {
this.routingNumbers = routingNumbers;
return this;
}
public Institution addRoutingNumbersItem(String routingNumbersItem) {
this.routingNumbers.add(routingNumbersItem);
return this;
}
/**
* A list of routing numbers known to be associated with the institution. This list is provided for the purpose of looking up institutions by routing number. It is generally comprehensive but is not guaranteed to be a complete list of routing numbers for an institution.
* @return routingNumbers
**/
@ApiModelProperty(required = true, value = "A list of routing numbers known to be associated with the institution. This list is provided for the purpose of looking up institutions by routing number. It is generally comprehensive but is not guaranteed to be a complete list of routing numbers for an institution.")
public List getRoutingNumbers() {
return routingNumbers;
}
public void setRoutingNumbers(List routingNumbers) {
this.routingNumbers = routingNumbers;
}
public Institution dtcNumbers(List dtcNumbers) {
this.dtcNumbers = dtcNumbers;
return this;
}
public Institution addDtcNumbersItem(String dtcNumbersItem) {
if (this.dtcNumbers == null) {
this.dtcNumbers = new ArrayList<>();
}
this.dtcNumbers.add(dtcNumbersItem);
return this;
}
/**
* A partial list of DTC numbers associated with the institution.
* @return dtcNumbers
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "A partial list of DTC numbers associated with the institution.")
public List getDtcNumbers() {
return dtcNumbers;
}
public void setDtcNumbers(List dtcNumbers) {
this.dtcNumbers = dtcNumbers;
}
public Institution oauth(Boolean oauth) {
this.oauth = oauth;
return this;
}
/**
* Indicates that the institution has an OAuth login flow. This will be `true` if OAuth is supported for any Items associated with the institution, even if the institution also supports non-OAuth connections.
* @return oauth
**/
@ApiModelProperty(required = true, value = "Indicates that the institution has an OAuth login flow. This will be `true` if OAuth is supported for any Items associated with the institution, even if the institution also supports non-OAuth connections.")
public Boolean getOauth() {
return oauth;
}
public void setOauth(Boolean oauth) {
this.oauth = oauth;
}
public Institution status(InstitutionStatus status) {
this.status = status;
return this;
}
/**
* Get status
* @return status
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
public InstitutionStatus getStatus() {
return status;
}
public void setStatus(InstitutionStatus status) {
this.status = status;
}
public Institution paymentInitiationMetadata(PaymentInitiationMetadata paymentInitiationMetadata) {
this.paymentInitiationMetadata = paymentInitiationMetadata;
return this;
}
/**
* Get paymentInitiationMetadata
* @return paymentInitiationMetadata
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
public PaymentInitiationMetadata getPaymentInitiationMetadata() {
return paymentInitiationMetadata;
}
public void setPaymentInitiationMetadata(PaymentInitiationMetadata paymentInitiationMetadata) {
this.paymentInitiationMetadata = paymentInitiationMetadata;
}
public Institution authMetadata(AuthMetadata authMetadata) {
this.authMetadata = authMetadata;
return this;
}
/**
* Get authMetadata
* @return authMetadata
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
public AuthMetadata getAuthMetadata() {
return authMetadata;
}
public void setAuthMetadata(AuthMetadata authMetadata) {
this.authMetadata = authMetadata;
}
@Override
public boolean equals(Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
Institution institution = (Institution) o;
return Objects.equals(this.institutionId, institution.institutionId) &&
Objects.equals(this.name, institution.name) &&
Objects.equals(this.products, institution.products) &&
Objects.equals(this.countryCodes, institution.countryCodes) &&
Objects.equals(this.url, institution.url) &&
Objects.equals(this.primaryColor, institution.primaryColor) &&
Objects.equals(this.logo, institution.logo) &&
Objects.equals(this.routingNumbers, institution.routingNumbers) &&
Objects.equals(this.dtcNumbers, institution.dtcNumbers) &&
Objects.equals(this.oauth, institution.oauth) &&
Objects.equals(this.status, institution.status) &&
Objects.equals(this.paymentInitiationMetadata, institution.paymentInitiationMetadata) &&
Objects.equals(this.authMetadata, institution.authMetadata);
}
@Override
public int hashCode() {
return Objects.hash(institutionId, name, products, countryCodes, url, primaryColor, logo, routingNumbers, dtcNumbers, oauth, status, paymentInitiationMetadata, authMetadata);
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class Institution {\n");
sb.append(" institutionId: ").append(toIndentedString(institutionId)).append("\n");
sb.append(" name: ").append(toIndentedString(name)).append("\n");
sb.append(" products: ").append(toIndentedString(products)).append("\n");
sb.append(" countryCodes: ").append(toIndentedString(countryCodes)).append("\n");
sb.append(" url: ").append(toIndentedString(url)).append("\n");
sb.append(" primaryColor: ").append(toIndentedString(primaryColor)).append("\n");
sb.append(" logo: ").append(toIndentedString(logo)).append("\n");
sb.append(" routingNumbers: ").append(toIndentedString(routingNumbers)).append("\n");
sb.append(" dtcNumbers: ").append(toIndentedString(dtcNumbers)).append("\n");
sb.append(" oauth: ").append(toIndentedString(oauth)).append("\n");
sb.append(" status: ").append(toIndentedString(status)).append("\n");
sb.append(" paymentInitiationMetadata: ").append(toIndentedString(paymentInitiationMetadata)).append("\n");
sb.append(" authMetadata: ").append(toIndentedString(authMetadata)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces
* (except the first line).
*/
private String toIndentedString(Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy