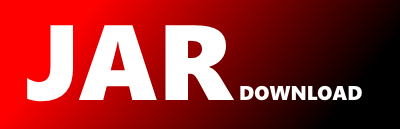
com.plaid.client.model.Item Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of plaid-java Show documentation
Show all versions of plaid-java Show documentation
Bindings for the Plaid (plaid.com) API.
/*
* The Plaid API
* The Plaid REST API. Please see https://plaid.com/docs/api for more details.
*
* The version of the OpenAPI document: 2020-09-14_1.565.0
*
*
* NOTE: This class is auto generated by OpenAPI Generator (https://openapi-generator.tech).
* https://openapi-generator.tech
* Do not edit the class manually.
*/
package com.plaid.client.model;
import java.util.Objects;
import java.util.Arrays;
import com.google.gson.TypeAdapter;
import com.google.gson.annotations.JsonAdapter;
import com.google.gson.annotations.SerializedName;
import com.google.gson.stream.JsonReader;
import com.google.gson.stream.JsonWriter;
import com.plaid.client.model.ItemConsentedDataScope;
import com.plaid.client.model.PlaidError;
import com.plaid.client.model.Products;
import io.swagger.annotations.ApiModel;
import io.swagger.annotations.ApiModelProperty;
import java.io.IOException;
import java.time.OffsetDateTime;
import java.util.ArrayList;
import java.util.List;
/**
* Metadata about the Item.
*/
@ApiModel(description = "Metadata about the Item.")
@javax.annotation.Generated(value = "org.openapitools.codegen.languages.JavaClientCodegen", date = "2024-09-17T16:42:02.150702Z[Etc/UTC]")
public class Item {
public static final String SERIALIZED_NAME_ITEM_ID = "item_id";
@SerializedName(SERIALIZED_NAME_ITEM_ID)
private String itemId;
public static final String SERIALIZED_NAME_INSTITUTION_ID = "institution_id";
@SerializedName(SERIALIZED_NAME_INSTITUTION_ID)
private String institutionId;
public static final String SERIALIZED_NAME_CREATED_AT = "created_at";
@SerializedName(SERIALIZED_NAME_CREATED_AT)
private OffsetDateTime createdAt;
public static final String SERIALIZED_NAME_WEBHOOK = "webhook";
@SerializedName(SERIALIZED_NAME_WEBHOOK)
private String webhook;
public static final String SERIALIZED_NAME_ERROR = "error";
@SerializedName(SERIALIZED_NAME_ERROR)
private PlaidError error;
public static final String SERIALIZED_NAME_AVAILABLE_PRODUCTS = "available_products";
@SerializedName(SERIALIZED_NAME_AVAILABLE_PRODUCTS)
private List availableProducts = new ArrayList<>();
public static final String SERIALIZED_NAME_BILLED_PRODUCTS = "billed_products";
@SerializedName(SERIALIZED_NAME_BILLED_PRODUCTS)
private List billedProducts = new ArrayList<>();
public static final String SERIALIZED_NAME_PRODUCTS = "products";
@SerializedName(SERIALIZED_NAME_PRODUCTS)
private List products = null;
public static final String SERIALIZED_NAME_CONSENTED_PRODUCTS = "consented_products";
@SerializedName(SERIALIZED_NAME_CONSENTED_PRODUCTS)
private List consentedProducts = null;
public static final String SERIALIZED_NAME_CONSENTED_USE_CASES = "consented_use_cases";
@SerializedName(SERIALIZED_NAME_CONSENTED_USE_CASES)
private List consentedUseCases = null;
public static final String SERIALIZED_NAME_CONSENTED_DATA_SCOPES = "consented_data_scopes";
@SerializedName(SERIALIZED_NAME_CONSENTED_DATA_SCOPES)
private List consentedDataScopes = null;
public static final String SERIALIZED_NAME_CONSENT_EXPIRATION_TIME = "consent_expiration_time";
@SerializedName(SERIALIZED_NAME_CONSENT_EXPIRATION_TIME)
private OffsetDateTime consentExpirationTime;
/**
* Indicates whether an Item requires user interaction to be updated, which can be the case for Items with some forms of two-factor authentication. `background` - Item can be updated in the background `user_present_required` - Item requires user interaction to be updated
*/
@JsonAdapter(UpdateTypeEnum.Adapter.class)
public enum UpdateTypeEnum {
BACKGROUND("background"),
USER_PRESENT_REQUIRED("user_present_required");
private String value;
UpdateTypeEnum(String value) {
this.value = value;
}
public String getValue() {
return value;
}
@Override
public String toString() {
return String.valueOf(value);
}
public static UpdateTypeEnum fromValue(String value) {
for (UpdateTypeEnum b : UpdateTypeEnum.values()) {
if (b.value.equals(value)) {
return b;
}
}
throw new IllegalArgumentException("Unexpected value '" + value + "'");
}
public static class Adapter extends TypeAdapter {
@Override
public void write(final JsonWriter jsonWriter, final UpdateTypeEnum enumeration) throws IOException {
jsonWriter.value(enumeration.getValue());
}
@Override
public UpdateTypeEnum read(final JsonReader jsonReader) throws IOException {
String value = jsonReader.nextString();
return UpdateTypeEnum.fromValue(value);
}
}
}
public static final String SERIALIZED_NAME_UPDATE_TYPE = "update_type";
@SerializedName(SERIALIZED_NAME_UPDATE_TYPE)
private UpdateTypeEnum updateType;
public Item itemId(String itemId) {
this.itemId = itemId;
return this;
}
/**
* The Plaid Item ID. The `item_id` is always unique; linking the same account at the same institution twice will result in two Items with different `item_id` values. Like all Plaid identifiers, the `item_id` is case-sensitive.
* @return itemId
**/
@ApiModelProperty(required = true, value = "The Plaid Item ID. The `item_id` is always unique; linking the same account at the same institution twice will result in two Items with different `item_id` values. Like all Plaid identifiers, the `item_id` is case-sensitive.")
public String getItemId() {
return itemId;
}
public void setItemId(String itemId) {
this.itemId = itemId;
}
public Item institutionId(String institutionId) {
this.institutionId = institutionId;
return this;
}
/**
* The Plaid Institution ID associated with the Item. Field is `null` for Items created via Same Day Micro-deposits.
* @return institutionId
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "The Plaid Institution ID associated with the Item. Field is `null` for Items created via Same Day Micro-deposits.")
public String getInstitutionId() {
return institutionId;
}
public void setInstitutionId(String institutionId) {
this.institutionId = institutionId;
}
public Item createdAt(OffsetDateTime createdAt) {
this.createdAt = createdAt;
return this;
}
/**
* The date and time when the Item was created, in [ISO 8601](https://wikipedia.org/wiki/ISO_8601) format.
* @return createdAt
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "The date and time when the Item was created, in [ISO 8601](https://wikipedia.org/wiki/ISO_8601) format.")
public OffsetDateTime getCreatedAt() {
return createdAt;
}
public void setCreatedAt(OffsetDateTime createdAt) {
this.createdAt = createdAt;
}
public Item webhook(String webhook) {
this.webhook = webhook;
return this;
}
/**
* The URL registered to receive webhooks for the Item.
* @return webhook
**/
@javax.annotation.Nullable
@ApiModelProperty(required = true, value = "The URL registered to receive webhooks for the Item.")
public String getWebhook() {
return webhook;
}
public void setWebhook(String webhook) {
this.webhook = webhook;
}
public Item error(PlaidError error) {
this.error = error;
return this;
}
/**
* Get error
* @return error
**/
@javax.annotation.Nullable
@ApiModelProperty(required = true, value = "")
public PlaidError getError() {
return error;
}
public void setError(PlaidError error) {
this.error = error;
}
public Item availableProducts(List availableProducts) {
this.availableProducts = availableProducts;
return this;
}
public Item addAvailableProductsItem(Products availableProductsItem) {
this.availableProducts.add(availableProductsItem);
return this;
}
/**
* A list of products available for the Item that have not yet been accessed. The contents of this array will be mutually exclusive with `billed_products`.
* @return availableProducts
**/
@ApiModelProperty(required = true, value = "A list of products available for the Item that have not yet been accessed. The contents of this array will be mutually exclusive with `billed_products`.")
public List getAvailableProducts() {
return availableProducts;
}
public void setAvailableProducts(List availableProducts) {
this.availableProducts = availableProducts;
}
public Item billedProducts(List billedProducts) {
this.billedProducts = billedProducts;
return this;
}
public Item addBilledProductsItem(Products billedProductsItem) {
this.billedProducts.add(billedProductsItem);
return this;
}
/**
* A list of products that have been billed for the Item. The contents of this array will be mutually exclusive with `available_products`. Note - `billed_products` is populated in all environments but only requests in Production are billed. Also note that products that are billed on a pay-per-call basis rather than a pay-per-Item basis, such as `balance`, will not appear here.
* @return billedProducts
**/
@ApiModelProperty(required = true, value = "A list of products that have been billed for the Item. The contents of this array will be mutually exclusive with `available_products`. Note - `billed_products` is populated in all environments but only requests in Production are billed. Also note that products that are billed on a pay-per-call basis rather than a pay-per-Item basis, such as `balance`, will not appear here. ")
public List getBilledProducts() {
return billedProducts;
}
public void setBilledProducts(List billedProducts) {
this.billedProducts = billedProducts;
}
public Item products(List products) {
this.products = products;
return this;
}
public Item addProductsItem(Products productsItem) {
if (this.products == null) {
this.products = new ArrayList<>();
}
this.products.add(productsItem);
return this;
}
/**
* A list of products added to the Item. In almost all cases, this will be the same as the `billed_products` field. For some products, it is possible for the product to be added to an Item but not yet billed (e.g. Assets, before `/asset_report/create` has been called, or Auth or Identity when added as Optional Products but before their endpoints have been called), in which case the product may appear in `products` but not in `billed_products`.
* @return products
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "A list of products added to the Item. In almost all cases, this will be the same as the `billed_products` field. For some products, it is possible for the product to be added to an Item but not yet billed (e.g. Assets, before `/asset_report/create` has been called, or Auth or Identity when added as Optional Products but before their endpoints have been called), in which case the product may appear in `products` but not in `billed_products`. ")
public List getProducts() {
return products;
}
public void setProducts(List products) {
this.products = products;
}
public Item consentedProducts(List consentedProducts) {
this.consentedProducts = consentedProducts;
return this;
}
public Item addConsentedProductsItem(Products consentedProductsItem) {
if (this.consentedProducts == null) {
this.consentedProducts = new ArrayList<>();
}
this.consentedProducts.add(consentedProductsItem);
return this;
}
/**
* A list of products that the user has consented to for the Item via [Data Transparency Messaging](/docs/link/data-transparency-messaging-migration-guide). This will consist of all products where both of the following are true: the user has consented to the required data scopes for that product and you have Production access for that product.
* @return consentedProducts
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "A list of products that the user has consented to for the Item via [Data Transparency Messaging](/docs/link/data-transparency-messaging-migration-guide). This will consist of all products where both of the following are true: the user has consented to the required data scopes for that product and you have Production access for that product. ")
public List getConsentedProducts() {
return consentedProducts;
}
public void setConsentedProducts(List consentedProducts) {
this.consentedProducts = consentedProducts;
}
public Item consentedUseCases(List consentedUseCases) {
this.consentedUseCases = consentedUseCases;
return this;
}
public Item addConsentedUseCasesItem(String consentedUseCasesItem) {
if (this.consentedUseCases == null) {
this.consentedUseCases = new ArrayList<>();
}
this.consentedUseCases.add(consentedUseCasesItem);
return this;
}
/**
* A list of use cases that the user has consented to for the Item via [Data Transparency Messaging](/docs/link/data-transparency-messaging-migration-guide). You can see the full list of use cases or update the list of use cases to request at any time via the Link Customization section of the [Plaid Dashboard](https://dashboard.plaid.com/link/data-transparency-v5).
* @return consentedUseCases
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "A list of use cases that the user has consented to for the Item via [Data Transparency Messaging](/docs/link/data-transparency-messaging-migration-guide). You can see the full list of use cases or update the list of use cases to request at any time via the Link Customization section of the [Plaid Dashboard](https://dashboard.plaid.com/link/data-transparency-v5).")
public List getConsentedUseCases() {
return consentedUseCases;
}
public void setConsentedUseCases(List consentedUseCases) {
this.consentedUseCases = consentedUseCases;
}
public Item consentedDataScopes(List consentedDataScopes) {
this.consentedDataScopes = consentedDataScopes;
return this;
}
public Item addConsentedDataScopesItem(ItemConsentedDataScope consentedDataScopesItem) {
if (this.consentedDataScopes == null) {
this.consentedDataScopes = new ArrayList<>();
}
this.consentedDataScopes.add(consentedDataScopesItem);
return this;
}
/**
* A list of data scopes that the user has consented to for the Item via [Data Transparency Messaging](/docs/link/data-transparency-messaging-migration-guide). These are based on the `consented_products`; see the [full mapping](/docs/link/data-transparency-messaging-migration-guide/#data-scopes-by-product) of data scopes and products.
* @return consentedDataScopes
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "A list of data scopes that the user has consented to for the Item via [Data Transparency Messaging](/docs/link/data-transparency-messaging-migration-guide). These are based on the `consented_products`; see the [full mapping](/docs/link/data-transparency-messaging-migration-guide/#data-scopes-by-product) of data scopes and products.")
public List getConsentedDataScopes() {
return consentedDataScopes;
}
public void setConsentedDataScopes(List consentedDataScopes) {
this.consentedDataScopes = consentedDataScopes;
}
public Item consentExpirationTime(OffsetDateTime consentExpirationTime) {
this.consentExpirationTime = consentExpirationTime;
return this;
}
/**
* The date and time at which the Item's access consent will expire, in [ISO 8601](https://wikipedia.org/wiki/ISO_8601) format
* @return consentExpirationTime
**/
@javax.annotation.Nullable
@ApiModelProperty(required = true, value = "The date and time at which the Item's access consent will expire, in [ISO 8601](https://wikipedia.org/wiki/ISO_8601) format")
public OffsetDateTime getConsentExpirationTime() {
return consentExpirationTime;
}
public void setConsentExpirationTime(OffsetDateTime consentExpirationTime) {
this.consentExpirationTime = consentExpirationTime;
}
public Item updateType(UpdateTypeEnum updateType) {
this.updateType = updateType;
return this;
}
/**
* Indicates whether an Item requires user interaction to be updated, which can be the case for Items with some forms of two-factor authentication. `background` - Item can be updated in the background `user_present_required` - Item requires user interaction to be updated
* @return updateType
**/
@ApiModelProperty(required = true, value = "Indicates whether an Item requires user interaction to be updated, which can be the case for Items with some forms of two-factor authentication. `background` - Item can be updated in the background `user_present_required` - Item requires user interaction to be updated")
public UpdateTypeEnum getUpdateType() {
return updateType;
}
public void setUpdateType(UpdateTypeEnum updateType) {
this.updateType = updateType;
}
@Override
public boolean equals(Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
Item item = (Item) o;
return Objects.equals(this.itemId, item.itemId) &&
Objects.equals(this.institutionId, item.institutionId) &&
Objects.equals(this.createdAt, item.createdAt) &&
Objects.equals(this.webhook, item.webhook) &&
Objects.equals(this.error, item.error) &&
Objects.equals(this.availableProducts, item.availableProducts) &&
Objects.equals(this.billedProducts, item.billedProducts) &&
Objects.equals(this.products, item.products) &&
Objects.equals(this.consentedProducts, item.consentedProducts) &&
Objects.equals(this.consentedUseCases, item.consentedUseCases) &&
Objects.equals(this.consentedDataScopes, item.consentedDataScopes) &&
Objects.equals(this.consentExpirationTime, item.consentExpirationTime) &&
Objects.equals(this.updateType, item.updateType);
}
@Override
public int hashCode() {
return Objects.hash(itemId, institutionId, createdAt, webhook, error, availableProducts, billedProducts, products, consentedProducts, consentedUseCases, consentedDataScopes, consentExpirationTime, updateType);
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class Item {\n");
sb.append(" itemId: ").append(toIndentedString(itemId)).append("\n");
sb.append(" institutionId: ").append(toIndentedString(institutionId)).append("\n");
sb.append(" createdAt: ").append(toIndentedString(createdAt)).append("\n");
sb.append(" webhook: ").append(toIndentedString(webhook)).append("\n");
sb.append(" error: ").append(toIndentedString(error)).append("\n");
sb.append(" availableProducts: ").append(toIndentedString(availableProducts)).append("\n");
sb.append(" billedProducts: ").append(toIndentedString(billedProducts)).append("\n");
sb.append(" products: ").append(toIndentedString(products)).append("\n");
sb.append(" consentedProducts: ").append(toIndentedString(consentedProducts)).append("\n");
sb.append(" consentedUseCases: ").append(toIndentedString(consentedUseCases)).append("\n");
sb.append(" consentedDataScopes: ").append(toIndentedString(consentedDataScopes)).append("\n");
sb.append(" consentExpirationTime: ").append(toIndentedString(consentExpirationTime)).append("\n");
sb.append(" updateType: ").append(toIndentedString(updateType)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces
* (except the first line).
*/
private String toIndentedString(Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy