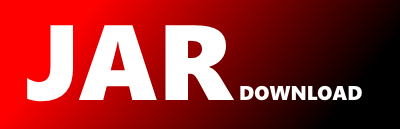
com.plaid.client.model.LinkTokenCreateHostedLink Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of plaid-java Show documentation
Show all versions of plaid-java Show documentation
Bindings for the Plaid (plaid.com) API.
/*
* The Plaid API
* The Plaid REST API. Please see https://plaid.com/docs/api for more details.
*
* The version of the OpenAPI document: 2020-09-14_1.565.0
*
*
* NOTE: This class is auto generated by OpenAPI Generator (https://openapi-generator.tech).
* https://openapi-generator.tech
* Do not edit the class manually.
*/
package com.plaid.client.model;
import java.util.Objects;
import java.util.Arrays;
import com.google.gson.TypeAdapter;
import com.google.gson.annotations.JsonAdapter;
import com.google.gson.annotations.SerializedName;
import com.google.gson.stream.JsonReader;
import com.google.gson.stream.JsonWriter;
import com.plaid.client.model.HostedLinkDeliveryMethod;
import io.swagger.annotations.ApiModel;
import io.swagger.annotations.ApiModelProperty;
import java.io.IOException;
/**
* Configuration parameters for Hosted Link. To request access to Hosted Link, contact your account manager.
*/
@ApiModel(description = "Configuration parameters for Hosted Link. To request access to Hosted Link, contact your account manager.")
@javax.annotation.Generated(value = "org.openapitools.codegen.languages.JavaClientCodegen", date = "2024-09-17T16:42:02.150702Z[Etc/UTC]")
public class LinkTokenCreateHostedLink {
public static final String SERIALIZED_NAME_DELIVERY_METHOD = "delivery_method";
@SerializedName(SERIALIZED_NAME_DELIVERY_METHOD)
private HostedLinkDeliveryMethod deliveryMethod;
public static final String SERIALIZED_NAME_COMPLETION_REDIRECT_URI = "completion_redirect_uri";
@SerializedName(SERIALIZED_NAME_COMPLETION_REDIRECT_URI)
private String completionRedirectUri;
public static final String SERIALIZED_NAME_URL_LIFETIME_SECONDS = "url_lifetime_seconds";
@SerializedName(SERIALIZED_NAME_URL_LIFETIME_SECONDS)
private Integer urlLifetimeSeconds;
public static final String SERIALIZED_NAME_IS_MOBILE_APP = "is_mobile_app";
@SerializedName(SERIALIZED_NAME_IS_MOBILE_APP)
private Boolean isMobileApp = false;
public LinkTokenCreateHostedLink deliveryMethod(HostedLinkDeliveryMethod deliveryMethod) {
this.deliveryMethod = deliveryMethod;
return this;
}
/**
* Get deliveryMethod
* @return deliveryMethod
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
public HostedLinkDeliveryMethod getDeliveryMethod() {
return deliveryMethod;
}
public void setDeliveryMethod(HostedLinkDeliveryMethod deliveryMethod) {
this.deliveryMethod = deliveryMethod;
}
public LinkTokenCreateHostedLink completionRedirectUri(String completionRedirectUri) {
this.completionRedirectUri = completionRedirectUri;
return this;
}
/**
* URI that Hosted Link will redirect to upon completion of the Link flow. This will only occur in Hosted Link sessions, not in other implementation methods.
* @return completionRedirectUri
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "URI that Hosted Link will redirect to upon completion of the Link flow. This will only occur in Hosted Link sessions, not in other implementation methods. ")
public String getCompletionRedirectUri() {
return completionRedirectUri;
}
public void setCompletionRedirectUri(String completionRedirectUri) {
this.completionRedirectUri = completionRedirectUri;
}
public LinkTokenCreateHostedLink urlLifetimeSeconds(Integer urlLifetimeSeconds) {
this.urlLifetimeSeconds = urlLifetimeSeconds;
return this;
}
/**
* How many seconds the link will be valid for. Must be positive. Cannot be longer than 21 days. The default lifetime is 7 days for links delivered by email, 1 day for links delivered via SMS, and 30 minutes for links not sent via Plaid Link delivery. This parameter will override the value of all three link types.
* @return urlLifetimeSeconds
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "How many seconds the link will be valid for. Must be positive. Cannot be longer than 21 days. The default lifetime is 7 days for links delivered by email, 1 day for links delivered via SMS, and 30 minutes for links not sent via Plaid Link delivery. This parameter will override the value of all three link types. ")
public Integer getUrlLifetimeSeconds() {
return urlLifetimeSeconds;
}
public void setUrlLifetimeSeconds(Integer urlLifetimeSeconds) {
this.urlLifetimeSeconds = urlLifetimeSeconds;
}
public LinkTokenCreateHostedLink isMobileApp(Boolean isMobileApp) {
this.isMobileApp = isMobileApp;
return this;
}
/**
* This indicates whether the client is opening hosted Link in a mobile app in an out of process web view (OOPWV) (i.e., an `AsWebAuthenticationSession` / `SFSafariViewController` or Android Custom Tab).
* @return isMobileApp
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "This indicates whether the client is opening hosted Link in a mobile app in an out of process web view (OOPWV) (i.e., an `AsWebAuthenticationSession` / `SFSafariViewController` or Android Custom Tab). ")
public Boolean getIsMobileApp() {
return isMobileApp;
}
public void setIsMobileApp(Boolean isMobileApp) {
this.isMobileApp = isMobileApp;
}
@Override
public boolean equals(Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
LinkTokenCreateHostedLink linkTokenCreateHostedLink = (LinkTokenCreateHostedLink) o;
return Objects.equals(this.deliveryMethod, linkTokenCreateHostedLink.deliveryMethod) &&
Objects.equals(this.completionRedirectUri, linkTokenCreateHostedLink.completionRedirectUri) &&
Objects.equals(this.urlLifetimeSeconds, linkTokenCreateHostedLink.urlLifetimeSeconds) &&
Objects.equals(this.isMobileApp, linkTokenCreateHostedLink.isMobileApp);
}
@Override
public int hashCode() {
return Objects.hash(deliveryMethod, completionRedirectUri, urlLifetimeSeconds, isMobileApp);
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class LinkTokenCreateHostedLink {\n");
sb.append(" deliveryMethod: ").append(toIndentedString(deliveryMethod)).append("\n");
sb.append(" completionRedirectUri: ").append(toIndentedString(completionRedirectUri)).append("\n");
sb.append(" urlLifetimeSeconds: ").append(toIndentedString(urlLifetimeSeconds)).append("\n");
sb.append(" isMobileApp: ").append(toIndentedString(isMobileApp)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces
* (except the first line).
*/
private String toIndentedString(Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy