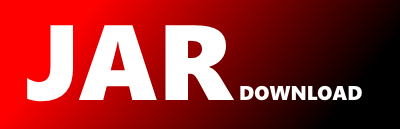
com.plaid.client.model.LinkTokenCreateRequest Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of plaid-java Show documentation
Show all versions of plaid-java Show documentation
Bindings for the Plaid (plaid.com) API.
/*
* The Plaid API
* The Plaid REST API. Please see https://plaid.com/docs/api for more details.
*
* The version of the OpenAPI document: 2020-09-14_1.565.0
*
*
* NOTE: This class is auto generated by OpenAPI Generator (https://openapi-generator.tech).
* https://openapi-generator.tech
* Do not edit the class manually.
*/
package com.plaid.client.model;
import java.util.Objects;
import java.util.Arrays;
import com.google.gson.TypeAdapter;
import com.google.gson.annotations.JsonAdapter;
import com.google.gson.annotations.SerializedName;
import com.google.gson.stream.JsonReader;
import com.google.gson.stream.JsonWriter;
import com.plaid.client.model.ConsumerReportPermissiblePurpose;
import com.plaid.client.model.CountryCode;
import com.plaid.client.model.LinkTokenAccountFilters;
import com.plaid.client.model.LinkTokenCreateCardSwitch;
import com.plaid.client.model.LinkTokenCreateHostedLink;
import com.plaid.client.model.LinkTokenCreateIdentity;
import com.plaid.client.model.LinkTokenCreateInstitutionData;
import com.plaid.client.model.LinkTokenCreateRequestAuth;
import com.plaid.client.model.LinkTokenCreateRequestBaseReport;
import com.plaid.client.model.LinkTokenCreateRequestCraOptions;
import com.plaid.client.model.LinkTokenCreateRequestCreditPartnerInsights;
import com.plaid.client.model.LinkTokenCreateRequestDepositSwitch;
import com.plaid.client.model.LinkTokenCreateRequestEmployment;
import com.plaid.client.model.LinkTokenCreateRequestIdentityVerification;
import com.plaid.client.model.LinkTokenCreateRequestIncomeVerification;
import com.plaid.client.model.LinkTokenCreateRequestPaymentInitiation;
import com.plaid.client.model.LinkTokenCreateRequestStatements;
import com.plaid.client.model.LinkTokenCreateRequestTransfer;
import com.plaid.client.model.LinkTokenCreateRequestUpdate;
import com.plaid.client.model.LinkTokenCreateRequestUser;
import com.plaid.client.model.LinkTokenEUConfig;
import com.plaid.client.model.LinkTokenInvestments;
import com.plaid.client.model.LinkTokenInvestmentsAuth;
import com.plaid.client.model.LinkTokenTransactions;
import com.plaid.client.model.Products;
import io.swagger.annotations.ApiModel;
import io.swagger.annotations.ApiModelProperty;
import java.io.IOException;
import java.util.ArrayList;
import java.util.List;
/**
* LinkTokenCreateRequest defines the request schema for `/link/token/create`
*/
@ApiModel(description = "LinkTokenCreateRequest defines the request schema for `/link/token/create`")
@javax.annotation.Generated(value = "org.openapitools.codegen.languages.JavaClientCodegen", date = "2024-09-17T16:42:02.150702Z[Etc/UTC]")
public class LinkTokenCreateRequest {
public static final String SERIALIZED_NAME_CLIENT_ID = "client_id";
@SerializedName(SERIALIZED_NAME_CLIENT_ID)
private String clientId;
public static final String SERIALIZED_NAME_SECRET = "secret";
@SerializedName(SERIALIZED_NAME_SECRET)
private String secret;
public static final String SERIALIZED_NAME_CLIENT_NAME = "client_name";
@SerializedName(SERIALIZED_NAME_CLIENT_NAME)
private String clientName;
public static final String SERIALIZED_NAME_LANGUAGE = "language";
@SerializedName(SERIALIZED_NAME_LANGUAGE)
private String language;
public static final String SERIALIZED_NAME_COUNTRY_CODES = "country_codes";
@SerializedName(SERIALIZED_NAME_COUNTRY_CODES)
private List countryCodes = new ArrayList<>();
public static final String SERIALIZED_NAME_USER = "user";
@SerializedName(SERIALIZED_NAME_USER)
private LinkTokenCreateRequestUser user;
public static final String SERIALIZED_NAME_PRODUCTS = "products";
@SerializedName(SERIALIZED_NAME_PRODUCTS)
private List products = null;
public static final String SERIALIZED_NAME_REQUIRED_IF_SUPPORTED_PRODUCTS = "required_if_supported_products";
@SerializedName(SERIALIZED_NAME_REQUIRED_IF_SUPPORTED_PRODUCTS)
private List requiredIfSupportedProducts = null;
public static final String SERIALIZED_NAME_OPTIONAL_PRODUCTS = "optional_products";
@SerializedName(SERIALIZED_NAME_OPTIONAL_PRODUCTS)
private List optionalProducts = null;
public static final String SERIALIZED_NAME_ADDITIONAL_CONSENTED_PRODUCTS = "additional_consented_products";
@SerializedName(SERIALIZED_NAME_ADDITIONAL_CONSENTED_PRODUCTS)
private List additionalConsentedProducts = null;
public static final String SERIALIZED_NAME_WEBHOOK = "webhook";
@SerializedName(SERIALIZED_NAME_WEBHOOK)
private String webhook;
public static final String SERIALIZED_NAME_ACCESS_TOKEN = "access_token";
@SerializedName(SERIALIZED_NAME_ACCESS_TOKEN)
private String accessToken;
public static final String SERIALIZED_NAME_ACCESS_TOKENS = "access_tokens";
@SerializedName(SERIALIZED_NAME_ACCESS_TOKENS)
private List accessTokens = null;
public static final String SERIALIZED_NAME_LINK_CUSTOMIZATION_NAME = "link_customization_name";
@SerializedName(SERIALIZED_NAME_LINK_CUSTOMIZATION_NAME)
private String linkCustomizationName;
public static final String SERIALIZED_NAME_REDIRECT_URI = "redirect_uri";
@SerializedName(SERIALIZED_NAME_REDIRECT_URI)
private String redirectUri;
public static final String SERIALIZED_NAME_ANDROID_PACKAGE_NAME = "android_package_name";
@SerializedName(SERIALIZED_NAME_ANDROID_PACKAGE_NAME)
private String androidPackageName;
public static final String SERIALIZED_NAME_INSTITUTION_DATA = "institution_data";
@SerializedName(SERIALIZED_NAME_INSTITUTION_DATA)
private LinkTokenCreateInstitutionData institutionData;
public static final String SERIALIZED_NAME_CARD_SWITCH = "card_switch";
@SerializedName(SERIALIZED_NAME_CARD_SWITCH)
private LinkTokenCreateCardSwitch cardSwitch;
public static final String SERIALIZED_NAME_ACCOUNT_FILTERS = "account_filters";
@SerializedName(SERIALIZED_NAME_ACCOUNT_FILTERS)
private LinkTokenAccountFilters accountFilters;
public static final String SERIALIZED_NAME_EU_CONFIG = "eu_config";
@SerializedName(SERIALIZED_NAME_EU_CONFIG)
private LinkTokenEUConfig euConfig;
public static final String SERIALIZED_NAME_INSTITUTION_ID = "institution_id";
@SerializedName(SERIALIZED_NAME_INSTITUTION_ID)
private String institutionId;
public static final String SERIALIZED_NAME_PAYMENT_INITIATION = "payment_initiation";
@SerializedName(SERIALIZED_NAME_PAYMENT_INITIATION)
private LinkTokenCreateRequestPaymentInitiation paymentInitiation;
public static final String SERIALIZED_NAME_DEPOSIT_SWITCH = "deposit_switch";
@SerializedName(SERIALIZED_NAME_DEPOSIT_SWITCH)
private LinkTokenCreateRequestDepositSwitch depositSwitch;
public static final String SERIALIZED_NAME_EMPLOYMENT = "employment";
@SerializedName(SERIALIZED_NAME_EMPLOYMENT)
private LinkTokenCreateRequestEmployment employment;
public static final String SERIALIZED_NAME_INCOME_VERIFICATION = "income_verification";
@SerializedName(SERIALIZED_NAME_INCOME_VERIFICATION)
private LinkTokenCreateRequestIncomeVerification incomeVerification;
public static final String SERIALIZED_NAME_BASE_REPORT = "base_report";
@SerializedName(SERIALIZED_NAME_BASE_REPORT)
private LinkTokenCreateRequestBaseReport baseReport;
public static final String SERIALIZED_NAME_CREDIT_PARTNER_INSIGHTS = "credit_partner_insights";
@SerializedName(SERIALIZED_NAME_CREDIT_PARTNER_INSIGHTS)
private LinkTokenCreateRequestCreditPartnerInsights creditPartnerInsights;
public static final String SERIALIZED_NAME_CRA_OPTIONS = "cra_options";
@SerializedName(SERIALIZED_NAME_CRA_OPTIONS)
private LinkTokenCreateRequestCraOptions craOptions;
public static final String SERIALIZED_NAME_CONSUMER_REPORT_PERMISSIBLE_PURPOSE = "consumer_report_permissible_purpose";
@SerializedName(SERIALIZED_NAME_CONSUMER_REPORT_PERMISSIBLE_PURPOSE)
private ConsumerReportPermissiblePurpose consumerReportPermissiblePurpose;
public static final String SERIALIZED_NAME_AUTH = "auth";
@SerializedName(SERIALIZED_NAME_AUTH)
private LinkTokenCreateRequestAuth auth;
public static final String SERIALIZED_NAME_TRANSFER = "transfer";
@SerializedName(SERIALIZED_NAME_TRANSFER)
private LinkTokenCreateRequestTransfer transfer;
public static final String SERIALIZED_NAME_UPDATE = "update";
@SerializedName(SERIALIZED_NAME_UPDATE)
private LinkTokenCreateRequestUpdate update;
public static final String SERIALIZED_NAME_IDENTITY_VERIFICATION = "identity_verification";
@SerializedName(SERIALIZED_NAME_IDENTITY_VERIFICATION)
private LinkTokenCreateRequestIdentityVerification identityVerification;
public static final String SERIALIZED_NAME_STATEMENTS = "statements";
@SerializedName(SERIALIZED_NAME_STATEMENTS)
private LinkTokenCreateRequestStatements statements;
public static final String SERIALIZED_NAME_USER_TOKEN = "user_token";
@SerializedName(SERIALIZED_NAME_USER_TOKEN)
private String userToken;
public static final String SERIALIZED_NAME_INVESTMENTS = "investments";
@SerializedName(SERIALIZED_NAME_INVESTMENTS)
private LinkTokenInvestments investments;
public static final String SERIALIZED_NAME_INVESTMENTS_AUTH = "investments_auth";
@SerializedName(SERIALIZED_NAME_INVESTMENTS_AUTH)
private LinkTokenInvestmentsAuth investmentsAuth;
public static final String SERIALIZED_NAME_HOSTED_LINK = "hosted_link";
@SerializedName(SERIALIZED_NAME_HOSTED_LINK)
private LinkTokenCreateHostedLink hostedLink;
public static final String SERIALIZED_NAME_TRANSACTIONS = "transactions";
@SerializedName(SERIALIZED_NAME_TRANSACTIONS)
private LinkTokenTransactions transactions;
public static final String SERIALIZED_NAME_CRA_ENABLED = "cra_enabled";
@SerializedName(SERIALIZED_NAME_CRA_ENABLED)
private Boolean craEnabled;
public static final String SERIALIZED_NAME_IDENTITY = "identity";
@SerializedName(SERIALIZED_NAME_IDENTITY)
private LinkTokenCreateIdentity identity;
public static final String SERIALIZED_NAME_FINANCEKIT_SUPPORTED = "financekit_supported";
@SerializedName(SERIALIZED_NAME_FINANCEKIT_SUPPORTED)
private Boolean financekitSupported;
public static final String SERIALIZED_NAME_ENABLE_MULTI_ITEM_LINK = "enable_multi_item_link";
@SerializedName(SERIALIZED_NAME_ENABLE_MULTI_ITEM_LINK)
private Boolean enableMultiItemLink;
public LinkTokenCreateRequest clientId(String clientId) {
this.clientId = clientId;
return this;
}
/**
* Your Plaid API `client_id`. The `client_id` is required and may be provided either in the `PLAID-CLIENT-ID` header or as part of a request body.
* @return clientId
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Your Plaid API `client_id`. The `client_id` is required and may be provided either in the `PLAID-CLIENT-ID` header or as part of a request body.")
public String getClientId() {
return clientId;
}
public void setClientId(String clientId) {
this.clientId = clientId;
}
public LinkTokenCreateRequest secret(String secret) {
this.secret = secret;
return this;
}
/**
* Your Plaid API `secret`. The `secret` is required and may be provided either in the `PLAID-SECRET` header or as part of a request body.
* @return secret
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Your Plaid API `secret`. The `secret` is required and may be provided either in the `PLAID-SECRET` header or as part of a request body.")
public String getSecret() {
return secret;
}
public void setSecret(String secret) {
this.secret = secret;
}
public LinkTokenCreateRequest clientName(String clientName) {
this.clientName = clientName;
return this;
}
/**
* The name of your application, as it should be displayed in Link. Maximum length of 30 characters. If a value longer than 30 characters is provided, Link will display \"This Application\" instead.
* @return clientName
**/
@ApiModelProperty(required = true, value = "The name of your application, as it should be displayed in Link. Maximum length of 30 characters. If a value longer than 30 characters is provided, Link will display \"This Application\" instead.")
public String getClientName() {
return clientName;
}
public void setClientName(String clientName) {
this.clientName = clientName;
}
public LinkTokenCreateRequest language(String language) {
this.language = language;
return this;
}
/**
* The language that Link should be displayed in. When initializing with Identity Verification, this field is not used; for more details, see [Identity Verification supported languages](https://www.plaid.com/docs/identity-verification/#supported-languages). Supported languages are: - Danish (`'da'`) - Dutch (`'nl'`) - English (`'en'`) - Estonian (`'et'`) - French (`'fr'`) - German (`'de'`) - Italian (`'it'`) - Latvian (`'lv'`) - Lithuanian (`'lt'`) - Norwegian (`'no'`) - Polish (`'pl'`) - Portuguese (`'pt'`) - Romanian (`'ro'`) - Spanish (`'es'`) - Swedish (`'sv'`) When using a Link customization, the language configured here must match the setting in the customization, or the customization will not be applied.
* @return language
**/
@ApiModelProperty(required = true, value = "The language that Link should be displayed in. When initializing with Identity Verification, this field is not used; for more details, see [Identity Verification supported languages](https://www.plaid.com/docs/identity-verification/#supported-languages). Supported languages are: - Danish (`'da'`) - Dutch (`'nl'`) - English (`'en'`) - Estonian (`'et'`) - French (`'fr'`) - German (`'de'`) - Italian (`'it'`) - Latvian (`'lv'`) - Lithuanian (`'lt'`) - Norwegian (`'no'`) - Polish (`'pl'`) - Portuguese (`'pt'`) - Romanian (`'ro'`) - Spanish (`'es'`) - Swedish (`'sv'`) When using a Link customization, the language configured here must match the setting in the customization, or the customization will not be applied.")
public String getLanguage() {
return language;
}
public void setLanguage(String language) {
this.language = language;
}
public LinkTokenCreateRequest countryCodes(List countryCodes) {
this.countryCodes = countryCodes;
return this;
}
public LinkTokenCreateRequest addCountryCodesItem(CountryCode countryCodesItem) {
this.countryCodes.add(countryCodesItem);
return this;
}
/**
* Specify an array of Plaid-supported country codes using the ISO-3166-1 alpha-2 country code standard. Institutions from all listed countries will be shown. For a complete mapping of supported products by country, see https://plaid.com/global/. If using Identity Verification, `country_codes` should be set to the country where your company is based, not the country where your user is located. For all other products, `country_codes` represents the location of your user's financial institution. If Link is launched with multiple country codes, only products that you are enabled for in all countries will be used by Link. Note that while all countries are enabled by default in Sandbox, in Production only US and Canada are enabled by default. To request access to European institutions, [file a product access Support ticket](https://dashboard.plaid.com/support/new/product-and-development/product-troubleshooting/request-product-access) via the Plaid dashboard. If using a Link customization, make sure the country codes in the customization match those specified in `country_codes`, or the customization may not be applied. If using the Auth features Instant Match, Instant Micro-deposits, Same-day Micro-deposits, Automated Micro-deposits, or Database Insights, `country_codes` must be set to `['US']`.
* @return countryCodes
**/
@ApiModelProperty(required = true, value = "Specify an array of Plaid-supported country codes using the ISO-3166-1 alpha-2 country code standard. Institutions from all listed countries will be shown. For a complete mapping of supported products by country, see https://plaid.com/global/. If using Identity Verification, `country_codes` should be set to the country where your company is based, not the country where your user is located. For all other products, `country_codes` represents the location of your user's financial institution. If Link is launched with multiple country codes, only products that you are enabled for in all countries will be used by Link. Note that while all countries are enabled by default in Sandbox, in Production only US and Canada are enabled by default. To request access to European institutions, [file a product access Support ticket](https://dashboard.plaid.com/support/new/product-and-development/product-troubleshooting/request-product-access) via the Plaid dashboard. If using a Link customization, make sure the country codes in the customization match those specified in `country_codes`, or the customization may not be applied. If using the Auth features Instant Match, Instant Micro-deposits, Same-day Micro-deposits, Automated Micro-deposits, or Database Insights, `country_codes` must be set to `['US']`.")
public List getCountryCodes() {
return countryCodes;
}
public void setCountryCodes(List countryCodes) {
this.countryCodes = countryCodes;
}
public LinkTokenCreateRequest user(LinkTokenCreateRequestUser user) {
this.user = user;
return this;
}
/**
* Get user
* @return user
**/
@ApiModelProperty(required = true, value = "")
public LinkTokenCreateRequestUser getUser() {
return user;
}
public void setUser(LinkTokenCreateRequestUser user) {
this.user = user;
}
public LinkTokenCreateRequest products(List products) {
this.products = products;
return this;
}
public LinkTokenCreateRequest addProductsItem(Products productsItem) {
if (this.products == null) {
this.products = new ArrayList<>();
}
this.products.add(productsItem);
return this;
}
/**
* List of Plaid product(s) you wish to use. If launching Link in update mode, should be omitted (unless you are using update mode to add Income or Assets to an Item); required otherwise. `balance` is *not* a valid value, the Balance product does not require explicit initialization and will automatically be initialized when any other product is initialized. The products specified here will determine which institutions will be available to your users in Link. Only institutions that support *all* requested products can be selected; a if a user attempts to select an institution that does not support a listed product, a \"Connectivity not supported\" error message will appear in Link. To maximize the number of institutions available, initialize Link with the minimal product set required for your use case. Additional products can be included via the [`optional_products`](https://plaid.com/docs/api/link/#link-token-create-request-optional-products) or [`required_if_supported_products`](https://plaid.com/docs/api/link/#link-token-create-request-required-if-supported-products) fields. Products can also be be initialized by calling the endpoint after obtaining an access token; this may require the product to be listed in the [`additional_consented_products`](https://plaid.com/docs/api/link/#link-token-create-request-additional-consented-products) array. For details, see [Choosing when to initialize products](https://plaid.com/docs/link/initializing-products/). Note that, unless you have opted to disable Instant Match support, institutions that support Instant Match will also be shown in Link if `auth` is specified as a product, even though these institutions do not contain `auth` in their product array. In Production, you will be billed for each product that you specify when initializing Link. Note that a product cannot be removed from an Item once the Item has been initialized with that product. To stop billing on an Item for subscription-based products, such as Liabilities, Investments, and Transactions, remove the Item via `/item/remove`.
* @return products
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "List of Plaid product(s) you wish to use. If launching Link in update mode, should be omitted (unless you are using update mode to add Income or Assets to an Item); required otherwise. `balance` is *not* a valid value, the Balance product does not require explicit initialization and will automatically be initialized when any other product is initialized. The products specified here will determine which institutions will be available to your users in Link. Only institutions that support *all* requested products can be selected; a if a user attempts to select an institution that does not support a listed product, a \"Connectivity not supported\" error message will appear in Link. To maximize the number of institutions available, initialize Link with the minimal product set required for your use case. Additional products can be included via the [`optional_products`](https://plaid.com/docs/api/link/#link-token-create-request-optional-products) or [`required_if_supported_products`](https://plaid.com/docs/api/link/#link-token-create-request-required-if-supported-products) fields. Products can also be be initialized by calling the endpoint after obtaining an access token; this may require the product to be listed in the [`additional_consented_products`](https://plaid.com/docs/api/link/#link-token-create-request-additional-consented-products) array. For details, see [Choosing when to initialize products](https://plaid.com/docs/link/initializing-products/). Note that, unless you have opted to disable Instant Match support, institutions that support Instant Match will also be shown in Link if `auth` is specified as a product, even though these institutions do not contain `auth` in their product array. In Production, you will be billed for each product that you specify when initializing Link. Note that a product cannot be removed from an Item once the Item has been initialized with that product. To stop billing on an Item for subscription-based products, such as Liabilities, Investments, and Transactions, remove the Item via `/item/remove`.")
public List getProducts() {
return products;
}
public void setProducts(List products) {
this.products = products;
}
public LinkTokenCreateRequest requiredIfSupportedProducts(List requiredIfSupportedProducts) {
this.requiredIfSupportedProducts = requiredIfSupportedProducts;
return this;
}
public LinkTokenCreateRequest addRequiredIfSupportedProductsItem(Products requiredIfSupportedProductsItem) {
if (this.requiredIfSupportedProducts == null) {
this.requiredIfSupportedProducts = new ArrayList<>();
}
this.requiredIfSupportedProducts.add(requiredIfSupportedProductsItem);
return this;
}
/**
* List of Plaid product(s) you wish to use only if the institution and account(s) selected by the user support the product. Institutions that do not support these products will still be shown in Link. The products will only be extracted and billed if the user selects an institution and account type that supports them. There should be no overlap between this array and the `products`, `optional_products`, or `additional_consented_products` arrays. The `products` array must have at least one product. For more details on using this feature, see [Required if Supported Products](https://www.plaid.com/docs/link/initializing-products/#required-if-supported-products).
* @return requiredIfSupportedProducts
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "List of Plaid product(s) you wish to use only if the institution and account(s) selected by the user support the product. Institutions that do not support these products will still be shown in Link. The products will only be extracted and billed if the user selects an institution and account type that supports them. There should be no overlap between this array and the `products`, `optional_products`, or `additional_consented_products` arrays. The `products` array must have at least one product. For more details on using this feature, see [Required if Supported Products](https://www.plaid.com/docs/link/initializing-products/#required-if-supported-products).")
public List getRequiredIfSupportedProducts() {
return requiredIfSupportedProducts;
}
public void setRequiredIfSupportedProducts(List requiredIfSupportedProducts) {
this.requiredIfSupportedProducts = requiredIfSupportedProducts;
}
public LinkTokenCreateRequest optionalProducts(List optionalProducts) {
this.optionalProducts = optionalProducts;
return this;
}
public LinkTokenCreateRequest addOptionalProductsItem(Products optionalProductsItem) {
if (this.optionalProducts == null) {
this.optionalProducts = new ArrayList<>();
}
this.optionalProducts.add(optionalProductsItem);
return this;
}
/**
* List of Plaid product(s) that will enhance the consumer's use case, but that your app can function without. Plaid will attempt to fetch data for these products on a best-effort basis, and failure to support these products will not affect Item creation. There should be no overlap between this array and the `products`, `required_if_supported_products`, or `additional_consented_products` arrays. The `products` array must have at least one product. For more details on using this feature, see [Optional Products](https://www.plaid.com/docs/link/initializing-products/#optional-products).
* @return optionalProducts
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "List of Plaid product(s) that will enhance the consumer's use case, but that your app can function without. Plaid will attempt to fetch data for these products on a best-effort basis, and failure to support these products will not affect Item creation. There should be no overlap between this array and the `products`, `required_if_supported_products`, or `additional_consented_products` arrays. The `products` array must have at least one product. For more details on using this feature, see [Optional Products](https://www.plaid.com/docs/link/initializing-products/#optional-products).")
public List getOptionalProducts() {
return optionalProducts;
}
public void setOptionalProducts(List optionalProducts) {
this.optionalProducts = optionalProducts;
}
public LinkTokenCreateRequest additionalConsentedProducts(List additionalConsentedProducts) {
this.additionalConsentedProducts = additionalConsentedProducts;
return this;
}
public LinkTokenCreateRequest addAdditionalConsentedProductsItem(Products additionalConsentedProductsItem) {
if (this.additionalConsentedProducts == null) {
this.additionalConsentedProducts = new ArrayList<>();
}
this.additionalConsentedProducts.add(additionalConsentedProductsItem);
return this;
}
/**
* List of additional Plaid product(s) you wish to collect consent for to support your use case. These products will not be billed until you start using them by calling the relevant endpoints. `balance` is *not* a valid value, the Balance product does not require explicit initialization and will automatically have consent collected. Institutions that do not support these products will still be shown in Link. There should be no overlap between this array and the `products` or `required_if_supported_products` arrays.
* @return additionalConsentedProducts
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "List of additional Plaid product(s) you wish to collect consent for to support your use case. These products will not be billed until you start using them by calling the relevant endpoints. `balance` is *not* a valid value, the Balance product does not require explicit initialization and will automatically have consent collected. Institutions that do not support these products will still be shown in Link. There should be no overlap between this array and the `products` or `required_if_supported_products` arrays.")
public List getAdditionalConsentedProducts() {
return additionalConsentedProducts;
}
public void setAdditionalConsentedProducts(List additionalConsentedProducts) {
this.additionalConsentedProducts = additionalConsentedProducts;
}
public LinkTokenCreateRequest webhook(String webhook) {
this.webhook = webhook;
return this;
}
/**
* The destination URL to which any webhooks should be sent. Note that webhooks for Payment Initiation (e-wallet transactions only), Transfer, Bank Transfer (including Auth micro-deposit notification webhooks) and Identity Verification are configured via the Dashboard instead.
* @return webhook
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "The destination URL to which any webhooks should be sent. Note that webhooks for Payment Initiation (e-wallet transactions only), Transfer, Bank Transfer (including Auth micro-deposit notification webhooks) and Identity Verification are configured via the Dashboard instead.")
public String getWebhook() {
return webhook;
}
public void setWebhook(String webhook) {
this.webhook = webhook;
}
public LinkTokenCreateRequest accessToken(String accessToken) {
this.accessToken = accessToken;
return this;
}
/**
* The `access_token` associated with the Item to update or reference, used when updating, modifying, or accessing an existing `access_token`. Used when launching Link in update mode, when completing the Same-day (manual) Micro-deposit flow, or (optionally) when initializing Link for a returning user as part of the Transfer UI flow.
* @return accessToken
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "The `access_token` associated with the Item to update or reference, used when updating, modifying, or accessing an existing `access_token`. Used when launching Link in update mode, when completing the Same-day (manual) Micro-deposit flow, or (optionally) when initializing Link for a returning user as part of the Transfer UI flow.")
public String getAccessToken() {
return accessToken;
}
public void setAccessToken(String accessToken) {
this.accessToken = accessToken;
}
public LinkTokenCreateRequest accessTokens(List accessTokens) {
this.accessTokens = accessTokens;
return this;
}
public LinkTokenCreateRequest addAccessTokensItem(String accessTokensItem) {
if (this.accessTokens == null) {
this.accessTokens = new ArrayList<>();
}
this.accessTokens.add(accessTokensItem);
return this;
}
/**
* A list of access tokens associated with the items to update in Link update mode for the Assets product. Using this instead of the `access_token` field allows the updating of multiple items at once. This feature is in closed beta, please contact your account manager for more info.
* @return accessTokens
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "A list of access tokens associated with the items to update in Link update mode for the Assets product. Using this instead of the `access_token` field allows the updating of multiple items at once. This feature is in closed beta, please contact your account manager for more info.")
public List getAccessTokens() {
return accessTokens;
}
public void setAccessTokens(List accessTokens) {
this.accessTokens = accessTokens;
}
public LinkTokenCreateRequest linkCustomizationName(String linkCustomizationName) {
this.linkCustomizationName = linkCustomizationName;
return this;
}
/**
* The name of the Link customization from the Plaid Dashboard to be applied to Link. If not specified, the `default` customization will be used. When using a Link customization, the language in the customization must match the language selected via the `language` parameter, and the countries in the customization should match the country codes selected via `country_codes`.
* @return linkCustomizationName
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "The name of the Link customization from the Plaid Dashboard to be applied to Link. If not specified, the `default` customization will be used. When using a Link customization, the language in the customization must match the language selected via the `language` parameter, and the countries in the customization should match the country codes selected via `country_codes`.")
public String getLinkCustomizationName() {
return linkCustomizationName;
}
public void setLinkCustomizationName(String linkCustomizationName) {
this.linkCustomizationName = linkCustomizationName;
}
public LinkTokenCreateRequest redirectUri(String redirectUri) {
this.redirectUri = redirectUri;
return this;
}
/**
* A URI indicating the destination where a user should be forwarded after completing the Link flow; used to support OAuth authentication flows when launching Link in the browser or another app. The `redirect_uri` should not contain any query parameters. When used in Production, must be an https URI. To specify any subdomain, use `*` as a wildcard character, e.g. `https://_*.example.com/oauth.html`. Note that any redirect URI must also be added to the Allowed redirect URIs list in the [developer dashboard](https://dashboard.plaid.com/team/api). If initializing on Android, `android_package_name` must be specified instead and `redirect_uri` should be left blank.
* @return redirectUri
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "A URI indicating the destination where a user should be forwarded after completing the Link flow; used to support OAuth authentication flows when launching Link in the browser or another app. The `redirect_uri` should not contain any query parameters. When used in Production, must be an https URI. To specify any subdomain, use `*` as a wildcard character, e.g. `https://_*.example.com/oauth.html`. Note that any redirect URI must also be added to the Allowed redirect URIs list in the [developer dashboard](https://dashboard.plaid.com/team/api). If initializing on Android, `android_package_name` must be specified instead and `redirect_uri` should be left blank.")
public String getRedirectUri() {
return redirectUri;
}
public void setRedirectUri(String redirectUri) {
this.redirectUri = redirectUri;
}
public LinkTokenCreateRequest androidPackageName(String androidPackageName) {
this.androidPackageName = androidPackageName;
return this;
}
/**
* The name of your app's Android package. Required if using the `link_token` to initialize Link on Android. Any package name specified here must also be added to the Allowed Android package names setting on the [developer dashboard](https://dashboard.plaid.com/team/api). When creating a `link_token` for initializing Link on other platforms, `android_package_name` must be left blank and `redirect_uri` should be used instead.
* @return androidPackageName
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "The name of your app's Android package. Required if using the `link_token` to initialize Link on Android. Any package name specified here must also be added to the Allowed Android package names setting on the [developer dashboard](https://dashboard.plaid.com/team/api). When creating a `link_token` for initializing Link on other platforms, `android_package_name` must be left blank and `redirect_uri` should be used instead.")
public String getAndroidPackageName() {
return androidPackageName;
}
public void setAndroidPackageName(String androidPackageName) {
this.androidPackageName = androidPackageName;
}
public LinkTokenCreateRequest institutionData(LinkTokenCreateInstitutionData institutionData) {
this.institutionData = institutionData;
return this;
}
/**
* Get institutionData
* @return institutionData
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
public LinkTokenCreateInstitutionData getInstitutionData() {
return institutionData;
}
public void setInstitutionData(LinkTokenCreateInstitutionData institutionData) {
this.institutionData = institutionData;
}
public LinkTokenCreateRequest cardSwitch(LinkTokenCreateCardSwitch cardSwitch) {
this.cardSwitch = cardSwitch;
return this;
}
/**
* Get cardSwitch
* @return cardSwitch
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
public LinkTokenCreateCardSwitch getCardSwitch() {
return cardSwitch;
}
public void setCardSwitch(LinkTokenCreateCardSwitch cardSwitch) {
this.cardSwitch = cardSwitch;
}
public LinkTokenCreateRequest accountFilters(LinkTokenAccountFilters accountFilters) {
this.accountFilters = accountFilters;
return this;
}
/**
* Get accountFilters
* @return accountFilters
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
public LinkTokenAccountFilters getAccountFilters() {
return accountFilters;
}
public void setAccountFilters(LinkTokenAccountFilters accountFilters) {
this.accountFilters = accountFilters;
}
public LinkTokenCreateRequest euConfig(LinkTokenEUConfig euConfig) {
this.euConfig = euConfig;
return this;
}
/**
* Get euConfig
* @return euConfig
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
public LinkTokenEUConfig getEuConfig() {
return euConfig;
}
public void setEuConfig(LinkTokenEUConfig euConfig) {
this.euConfig = euConfig;
}
public LinkTokenCreateRequest institutionId(String institutionId) {
this.institutionId = institutionId;
return this;
}
/**
* Used for certain Europe-only configurations, as well as certain legacy use cases in other regions.
* @return institutionId
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Used for certain Europe-only configurations, as well as certain legacy use cases in other regions.")
public String getInstitutionId() {
return institutionId;
}
public void setInstitutionId(String institutionId) {
this.institutionId = institutionId;
}
public LinkTokenCreateRequest paymentInitiation(LinkTokenCreateRequestPaymentInitiation paymentInitiation) {
this.paymentInitiation = paymentInitiation;
return this;
}
/**
* Get paymentInitiation
* @return paymentInitiation
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
public LinkTokenCreateRequestPaymentInitiation getPaymentInitiation() {
return paymentInitiation;
}
public void setPaymentInitiation(LinkTokenCreateRequestPaymentInitiation paymentInitiation) {
this.paymentInitiation = paymentInitiation;
}
public LinkTokenCreateRequest depositSwitch(LinkTokenCreateRequestDepositSwitch depositSwitch) {
this.depositSwitch = depositSwitch;
return this;
}
/**
* Get depositSwitch
* @return depositSwitch
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
public LinkTokenCreateRequestDepositSwitch getDepositSwitch() {
return depositSwitch;
}
public void setDepositSwitch(LinkTokenCreateRequestDepositSwitch depositSwitch) {
this.depositSwitch = depositSwitch;
}
public LinkTokenCreateRequest employment(LinkTokenCreateRequestEmployment employment) {
this.employment = employment;
return this;
}
/**
* Get employment
* @return employment
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
public LinkTokenCreateRequestEmployment getEmployment() {
return employment;
}
public void setEmployment(LinkTokenCreateRequestEmployment employment) {
this.employment = employment;
}
public LinkTokenCreateRequest incomeVerification(LinkTokenCreateRequestIncomeVerification incomeVerification) {
this.incomeVerification = incomeVerification;
return this;
}
/**
* Get incomeVerification
* @return incomeVerification
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
public LinkTokenCreateRequestIncomeVerification getIncomeVerification() {
return incomeVerification;
}
public void setIncomeVerification(LinkTokenCreateRequestIncomeVerification incomeVerification) {
this.incomeVerification = incomeVerification;
}
public LinkTokenCreateRequest baseReport(LinkTokenCreateRequestBaseReport baseReport) {
this.baseReport = baseReport;
return this;
}
/**
* Get baseReport
* @return baseReport
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
public LinkTokenCreateRequestBaseReport getBaseReport() {
return baseReport;
}
public void setBaseReport(LinkTokenCreateRequestBaseReport baseReport) {
this.baseReport = baseReport;
}
public LinkTokenCreateRequest creditPartnerInsights(LinkTokenCreateRequestCreditPartnerInsights creditPartnerInsights) {
this.creditPartnerInsights = creditPartnerInsights;
return this;
}
/**
* Get creditPartnerInsights
* @return creditPartnerInsights
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
public LinkTokenCreateRequestCreditPartnerInsights getCreditPartnerInsights() {
return creditPartnerInsights;
}
public void setCreditPartnerInsights(LinkTokenCreateRequestCreditPartnerInsights creditPartnerInsights) {
this.creditPartnerInsights = creditPartnerInsights;
}
public LinkTokenCreateRequest craOptions(LinkTokenCreateRequestCraOptions craOptions) {
this.craOptions = craOptions;
return this;
}
/**
* Get craOptions
* @return craOptions
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
public LinkTokenCreateRequestCraOptions getCraOptions() {
return craOptions;
}
public void setCraOptions(LinkTokenCreateRequestCraOptions craOptions) {
this.craOptions = craOptions;
}
public LinkTokenCreateRequest consumerReportPermissiblePurpose(ConsumerReportPermissiblePurpose consumerReportPermissiblePurpose) {
this.consumerReportPermissiblePurpose = consumerReportPermissiblePurpose;
return this;
}
/**
* Get consumerReportPermissiblePurpose
* @return consumerReportPermissiblePurpose
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
public ConsumerReportPermissiblePurpose getConsumerReportPermissiblePurpose() {
return consumerReportPermissiblePurpose;
}
public void setConsumerReportPermissiblePurpose(ConsumerReportPermissiblePurpose consumerReportPermissiblePurpose) {
this.consumerReportPermissiblePurpose = consumerReportPermissiblePurpose;
}
public LinkTokenCreateRequest auth(LinkTokenCreateRequestAuth auth) {
this.auth = auth;
return this;
}
/**
* Get auth
* @return auth
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
public LinkTokenCreateRequestAuth getAuth() {
return auth;
}
public void setAuth(LinkTokenCreateRequestAuth auth) {
this.auth = auth;
}
public LinkTokenCreateRequest transfer(LinkTokenCreateRequestTransfer transfer) {
this.transfer = transfer;
return this;
}
/**
* Get transfer
* @return transfer
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
public LinkTokenCreateRequestTransfer getTransfer() {
return transfer;
}
public void setTransfer(LinkTokenCreateRequestTransfer transfer) {
this.transfer = transfer;
}
public LinkTokenCreateRequest update(LinkTokenCreateRequestUpdate update) {
this.update = update;
return this;
}
/**
* Get update
* @return update
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
public LinkTokenCreateRequestUpdate getUpdate() {
return update;
}
public void setUpdate(LinkTokenCreateRequestUpdate update) {
this.update = update;
}
public LinkTokenCreateRequest identityVerification(LinkTokenCreateRequestIdentityVerification identityVerification) {
this.identityVerification = identityVerification;
return this;
}
/**
* Get identityVerification
* @return identityVerification
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
public LinkTokenCreateRequestIdentityVerification getIdentityVerification() {
return identityVerification;
}
public void setIdentityVerification(LinkTokenCreateRequestIdentityVerification identityVerification) {
this.identityVerification = identityVerification;
}
public LinkTokenCreateRequest statements(LinkTokenCreateRequestStatements statements) {
this.statements = statements;
return this;
}
/**
* Get statements
* @return statements
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
public LinkTokenCreateRequestStatements getStatements() {
return statements;
}
public void setStatements(LinkTokenCreateRequestStatements statements) {
this.statements = statements;
}
public LinkTokenCreateRequest userToken(String userToken) {
this.userToken = userToken;
return this;
}
/**
* A user token generated using `/user/create`. Any Item created during the Link session will be associated with the user.
* @return userToken
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "A user token generated using `/user/create`. Any Item created during the Link session will be associated with the user.")
public String getUserToken() {
return userToken;
}
public void setUserToken(String userToken) {
this.userToken = userToken;
}
public LinkTokenCreateRequest investments(LinkTokenInvestments investments) {
this.investments = investments;
return this;
}
/**
* Get investments
* @return investments
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
public LinkTokenInvestments getInvestments() {
return investments;
}
public void setInvestments(LinkTokenInvestments investments) {
this.investments = investments;
}
public LinkTokenCreateRequest investmentsAuth(LinkTokenInvestmentsAuth investmentsAuth) {
this.investmentsAuth = investmentsAuth;
return this;
}
/**
* Get investmentsAuth
* @return investmentsAuth
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
public LinkTokenInvestmentsAuth getInvestmentsAuth() {
return investmentsAuth;
}
public void setInvestmentsAuth(LinkTokenInvestmentsAuth investmentsAuth) {
this.investmentsAuth = investmentsAuth;
}
public LinkTokenCreateRequest hostedLink(LinkTokenCreateHostedLink hostedLink) {
this.hostedLink = hostedLink;
return this;
}
/**
* Get hostedLink
* @return hostedLink
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
public LinkTokenCreateHostedLink getHostedLink() {
return hostedLink;
}
public void setHostedLink(LinkTokenCreateHostedLink hostedLink) {
this.hostedLink = hostedLink;
}
public LinkTokenCreateRequest transactions(LinkTokenTransactions transactions) {
this.transactions = transactions;
return this;
}
/**
* Get transactions
* @return transactions
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
public LinkTokenTransactions getTransactions() {
return transactions;
}
public void setTransactions(LinkTokenTransactions transactions) {
this.transactions = transactions;
}
public LinkTokenCreateRequest craEnabled(Boolean craEnabled) {
this.craEnabled = craEnabled;
return this;
}
/**
* If `true`, request a CRA connection. Defaults to `false`.
* @return craEnabled
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "If `true`, request a CRA connection. Defaults to `false`.")
public Boolean getCraEnabled() {
return craEnabled;
}
public void setCraEnabled(Boolean craEnabled) {
this.craEnabled = craEnabled;
}
public LinkTokenCreateRequest identity(LinkTokenCreateIdentity identity) {
this.identity = identity;
return this;
}
/**
* Get identity
* @return identity
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
public LinkTokenCreateIdentity getIdentity() {
return identity;
}
public void setIdentity(LinkTokenCreateIdentity identity) {
this.identity = identity;
}
public LinkTokenCreateRequest financekitSupported(Boolean financekitSupported) {
this.financekitSupported = financekitSupported;
return this;
}
/**
* If `true`, indicates that client supports linking FinanceKit / AppleCard items. Defaults to `false`.
* @return financekitSupported
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "If `true`, indicates that client supports linking FinanceKit / AppleCard items. Defaults to `false`.")
public Boolean getFinancekitSupported() {
return financekitSupported;
}
public void setFinancekitSupported(Boolean financekitSupported) {
this.financekitSupported = financekitSupported;
}
public LinkTokenCreateRequest enableMultiItemLink(Boolean enableMultiItemLink) {
this.enableMultiItemLink = enableMultiItemLink;
return this;
}
/**
* If `true`, enable linking multiple items in the same Link session. Defaults to `false`.
* @return enableMultiItemLink
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "If `true`, enable linking multiple items in the same Link session. Defaults to `false`.")
public Boolean getEnableMultiItemLink() {
return enableMultiItemLink;
}
public void setEnableMultiItemLink(Boolean enableMultiItemLink) {
this.enableMultiItemLink = enableMultiItemLink;
}
@Override
public boolean equals(Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
LinkTokenCreateRequest linkTokenCreateRequest = (LinkTokenCreateRequest) o;
return Objects.equals(this.clientId, linkTokenCreateRequest.clientId) &&
Objects.equals(this.secret, linkTokenCreateRequest.secret) &&
Objects.equals(this.clientName, linkTokenCreateRequest.clientName) &&
Objects.equals(this.language, linkTokenCreateRequest.language) &&
Objects.equals(this.countryCodes, linkTokenCreateRequest.countryCodes) &&
Objects.equals(this.user, linkTokenCreateRequest.user) &&
Objects.equals(this.products, linkTokenCreateRequest.products) &&
Objects.equals(this.requiredIfSupportedProducts, linkTokenCreateRequest.requiredIfSupportedProducts) &&
Objects.equals(this.optionalProducts, linkTokenCreateRequest.optionalProducts) &&
Objects.equals(this.additionalConsentedProducts, linkTokenCreateRequest.additionalConsentedProducts) &&
Objects.equals(this.webhook, linkTokenCreateRequest.webhook) &&
Objects.equals(this.accessToken, linkTokenCreateRequest.accessToken) &&
Objects.equals(this.accessTokens, linkTokenCreateRequest.accessTokens) &&
Objects.equals(this.linkCustomizationName, linkTokenCreateRequest.linkCustomizationName) &&
Objects.equals(this.redirectUri, linkTokenCreateRequest.redirectUri) &&
Objects.equals(this.androidPackageName, linkTokenCreateRequest.androidPackageName) &&
Objects.equals(this.institutionData, linkTokenCreateRequest.institutionData) &&
Objects.equals(this.cardSwitch, linkTokenCreateRequest.cardSwitch) &&
Objects.equals(this.accountFilters, linkTokenCreateRequest.accountFilters) &&
Objects.equals(this.euConfig, linkTokenCreateRequest.euConfig) &&
Objects.equals(this.institutionId, linkTokenCreateRequest.institutionId) &&
Objects.equals(this.paymentInitiation, linkTokenCreateRequest.paymentInitiation) &&
Objects.equals(this.depositSwitch, linkTokenCreateRequest.depositSwitch) &&
Objects.equals(this.employment, linkTokenCreateRequest.employment) &&
Objects.equals(this.incomeVerification, linkTokenCreateRequest.incomeVerification) &&
Objects.equals(this.baseReport, linkTokenCreateRequest.baseReport) &&
Objects.equals(this.creditPartnerInsights, linkTokenCreateRequest.creditPartnerInsights) &&
Objects.equals(this.craOptions, linkTokenCreateRequest.craOptions) &&
Objects.equals(this.consumerReportPermissiblePurpose, linkTokenCreateRequest.consumerReportPermissiblePurpose) &&
Objects.equals(this.auth, linkTokenCreateRequest.auth) &&
Objects.equals(this.transfer, linkTokenCreateRequest.transfer) &&
Objects.equals(this.update, linkTokenCreateRequest.update) &&
Objects.equals(this.identityVerification, linkTokenCreateRequest.identityVerification) &&
Objects.equals(this.statements, linkTokenCreateRequest.statements) &&
Objects.equals(this.userToken, linkTokenCreateRequest.userToken) &&
Objects.equals(this.investments, linkTokenCreateRequest.investments) &&
Objects.equals(this.investmentsAuth, linkTokenCreateRequest.investmentsAuth) &&
Objects.equals(this.hostedLink, linkTokenCreateRequest.hostedLink) &&
Objects.equals(this.transactions, linkTokenCreateRequest.transactions) &&
Objects.equals(this.craEnabled, linkTokenCreateRequest.craEnabled) &&
Objects.equals(this.identity, linkTokenCreateRequest.identity) &&
Objects.equals(this.financekitSupported, linkTokenCreateRequest.financekitSupported) &&
Objects.equals(this.enableMultiItemLink, linkTokenCreateRequest.enableMultiItemLink);
}
@Override
public int hashCode() {
return Objects.hash(clientId, secret, clientName, language, countryCodes, user, products, requiredIfSupportedProducts, optionalProducts, additionalConsentedProducts, webhook, accessToken, accessTokens, linkCustomizationName, redirectUri, androidPackageName, institutionData, cardSwitch, accountFilters, euConfig, institutionId, paymentInitiation, depositSwitch, employment, incomeVerification, baseReport, creditPartnerInsights, craOptions, consumerReportPermissiblePurpose, auth, transfer, update, identityVerification, statements, userToken, investments, investmentsAuth, hostedLink, transactions, craEnabled, identity, financekitSupported, enableMultiItemLink);
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class LinkTokenCreateRequest {\n");
sb.append(" clientId: ").append(toIndentedString(clientId)).append("\n");
sb.append(" secret: ").append(toIndentedString(secret)).append("\n");
sb.append(" clientName: ").append(toIndentedString(clientName)).append("\n");
sb.append(" language: ").append(toIndentedString(language)).append("\n");
sb.append(" countryCodes: ").append(toIndentedString(countryCodes)).append("\n");
sb.append(" user: ").append(toIndentedString(user)).append("\n");
sb.append(" products: ").append(toIndentedString(products)).append("\n");
sb.append(" requiredIfSupportedProducts: ").append(toIndentedString(requiredIfSupportedProducts)).append("\n");
sb.append(" optionalProducts: ").append(toIndentedString(optionalProducts)).append("\n");
sb.append(" additionalConsentedProducts: ").append(toIndentedString(additionalConsentedProducts)).append("\n");
sb.append(" webhook: ").append(toIndentedString(webhook)).append("\n");
sb.append(" accessToken: ").append(toIndentedString(accessToken)).append("\n");
sb.append(" accessTokens: ").append(toIndentedString(accessTokens)).append("\n");
sb.append(" linkCustomizationName: ").append(toIndentedString(linkCustomizationName)).append("\n");
sb.append(" redirectUri: ").append(toIndentedString(redirectUri)).append("\n");
sb.append(" androidPackageName: ").append(toIndentedString(androidPackageName)).append("\n");
sb.append(" institutionData: ").append(toIndentedString(institutionData)).append("\n");
sb.append(" cardSwitch: ").append(toIndentedString(cardSwitch)).append("\n");
sb.append(" accountFilters: ").append(toIndentedString(accountFilters)).append("\n");
sb.append(" euConfig: ").append(toIndentedString(euConfig)).append("\n");
sb.append(" institutionId: ").append(toIndentedString(institutionId)).append("\n");
sb.append(" paymentInitiation: ").append(toIndentedString(paymentInitiation)).append("\n");
sb.append(" depositSwitch: ").append(toIndentedString(depositSwitch)).append("\n");
sb.append(" employment: ").append(toIndentedString(employment)).append("\n");
sb.append(" incomeVerification: ").append(toIndentedString(incomeVerification)).append("\n");
sb.append(" baseReport: ").append(toIndentedString(baseReport)).append("\n");
sb.append(" creditPartnerInsights: ").append(toIndentedString(creditPartnerInsights)).append("\n");
sb.append(" craOptions: ").append(toIndentedString(craOptions)).append("\n");
sb.append(" consumerReportPermissiblePurpose: ").append(toIndentedString(consumerReportPermissiblePurpose)).append("\n");
sb.append(" auth: ").append(toIndentedString(auth)).append("\n");
sb.append(" transfer: ").append(toIndentedString(transfer)).append("\n");
sb.append(" update: ").append(toIndentedString(update)).append("\n");
sb.append(" identityVerification: ").append(toIndentedString(identityVerification)).append("\n");
sb.append(" statements: ").append(toIndentedString(statements)).append("\n");
sb.append(" userToken: ").append(toIndentedString(userToken)).append("\n");
sb.append(" investments: ").append(toIndentedString(investments)).append("\n");
sb.append(" investmentsAuth: ").append(toIndentedString(investmentsAuth)).append("\n");
sb.append(" hostedLink: ").append(toIndentedString(hostedLink)).append("\n");
sb.append(" transactions: ").append(toIndentedString(transactions)).append("\n");
sb.append(" craEnabled: ").append(toIndentedString(craEnabled)).append("\n");
sb.append(" identity: ").append(toIndentedString(identity)).append("\n");
sb.append(" financekitSupported: ").append(toIndentedString(financekitSupported)).append("\n");
sb.append(" enableMultiItemLink: ").append(toIndentedString(enableMultiItemLink)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces
* (except the first line).
*/
private String toIndentedString(Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy