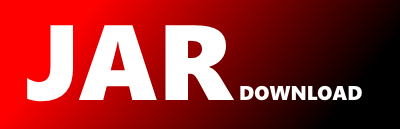
com.plaid.client.model.PaymentInitiationPaymentCreateRequest Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of plaid-java Show documentation
Show all versions of plaid-java Show documentation
Bindings for the Plaid (plaid.com) API.
/*
* The Plaid API
* The Plaid REST API. Please see https://plaid.com/docs/api for more details.
*
* The version of the OpenAPI document: 2020-09-14_1.565.0
*
*
* NOTE: This class is auto generated by OpenAPI Generator (https://openapi-generator.tech).
* https://openapi-generator.tech
* Do not edit the class manually.
*/
package com.plaid.client.model;
import java.util.Objects;
import java.util.Arrays;
import com.google.gson.TypeAdapter;
import com.google.gson.annotations.JsonAdapter;
import com.google.gson.annotations.SerializedName;
import com.google.gson.stream.JsonReader;
import com.google.gson.stream.JsonWriter;
import com.plaid.client.model.ExternalPaymentOptions;
import com.plaid.client.model.ExternalPaymentScheduleRequest;
import com.plaid.client.model.PaymentAmount;
import io.swagger.annotations.ApiModel;
import io.swagger.annotations.ApiModelProperty;
import java.io.IOException;
/**
* PaymentInitiationPaymentCreateRequest defines the request schema for `/payment_initiation/payment/create`
*/
@ApiModel(description = "PaymentInitiationPaymentCreateRequest defines the request schema for `/payment_initiation/payment/create`")
@javax.annotation.Generated(value = "org.openapitools.codegen.languages.JavaClientCodegen", date = "2024-09-17T16:42:02.150702Z[Etc/UTC]")
public class PaymentInitiationPaymentCreateRequest {
public static final String SERIALIZED_NAME_CLIENT_ID = "client_id";
@SerializedName(SERIALIZED_NAME_CLIENT_ID)
private String clientId;
public static final String SERIALIZED_NAME_SECRET = "secret";
@SerializedName(SERIALIZED_NAME_SECRET)
private String secret;
public static final String SERIALIZED_NAME_RECIPIENT_ID = "recipient_id";
@SerializedName(SERIALIZED_NAME_RECIPIENT_ID)
private String recipientId;
public static final String SERIALIZED_NAME_REFERENCE = "reference";
@SerializedName(SERIALIZED_NAME_REFERENCE)
private String reference;
public static final String SERIALIZED_NAME_AMOUNT = "amount";
@SerializedName(SERIALIZED_NAME_AMOUNT)
private PaymentAmount amount;
public static final String SERIALIZED_NAME_SCHEDULE = "schedule";
@SerializedName(SERIALIZED_NAME_SCHEDULE)
private ExternalPaymentScheduleRequest schedule;
public static final String SERIALIZED_NAME_OPTIONS = "options";
@SerializedName(SERIALIZED_NAME_OPTIONS)
private ExternalPaymentOptions options;
public PaymentInitiationPaymentCreateRequest clientId(String clientId) {
this.clientId = clientId;
return this;
}
/**
* Your Plaid API `client_id`. The `client_id` is required and may be provided either in the `PLAID-CLIENT-ID` header or as part of a request body.
* @return clientId
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Your Plaid API `client_id`. The `client_id` is required and may be provided either in the `PLAID-CLIENT-ID` header or as part of a request body.")
public String getClientId() {
return clientId;
}
public void setClientId(String clientId) {
this.clientId = clientId;
}
public PaymentInitiationPaymentCreateRequest secret(String secret) {
this.secret = secret;
return this;
}
/**
* Your Plaid API `secret`. The `secret` is required and may be provided either in the `PLAID-SECRET` header or as part of a request body.
* @return secret
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Your Plaid API `secret`. The `secret` is required and may be provided either in the `PLAID-SECRET` header or as part of a request body.")
public String getSecret() {
return secret;
}
public void setSecret(String secret) {
this.secret = secret;
}
public PaymentInitiationPaymentCreateRequest recipientId(String recipientId) {
this.recipientId = recipientId;
return this;
}
/**
* The ID of the recipient the payment is for.
* @return recipientId
**/
@ApiModelProperty(required = true, value = "The ID of the recipient the payment is for.")
public String getRecipientId() {
return recipientId;
}
public void setRecipientId(String recipientId) {
this.recipientId = recipientId;
}
public PaymentInitiationPaymentCreateRequest reference(String reference) {
this.reference = reference;
return this;
}
/**
* A reference for the payment. This must be an alphanumeric string with at most 18 characters and must not contain any special characters (since not all institutions support them). In order to track settlement via Payment Confirmation, each payment must have a unique reference. If the reference provided through the API is not unique, Plaid will adjust it. Some institutions may limit the reference to less than 18 characters. If necessary, Plaid will adjust the reference by truncating it to fit the institution's requirements. Both the originally provided and automatically adjusted references (if any) can be found in the `reference` and `adjusted_reference` fields, respectively.
* @return reference
**/
@ApiModelProperty(required = true, value = "A reference for the payment. This must be an alphanumeric string with at most 18 characters and must not contain any special characters (since not all institutions support them). In order to track settlement via Payment Confirmation, each payment must have a unique reference. If the reference provided through the API is not unique, Plaid will adjust it. Some institutions may limit the reference to less than 18 characters. If necessary, Plaid will adjust the reference by truncating it to fit the institution's requirements. Both the originally provided and automatically adjusted references (if any) can be found in the `reference` and `adjusted_reference` fields, respectively.")
public String getReference() {
return reference;
}
public void setReference(String reference) {
this.reference = reference;
}
public PaymentInitiationPaymentCreateRequest amount(PaymentAmount amount) {
this.amount = amount;
return this;
}
/**
* Get amount
* @return amount
**/
@ApiModelProperty(required = true, value = "")
public PaymentAmount getAmount() {
return amount;
}
public void setAmount(PaymentAmount amount) {
this.amount = amount;
}
public PaymentInitiationPaymentCreateRequest schedule(ExternalPaymentScheduleRequest schedule) {
this.schedule = schedule;
return this;
}
/**
* Get schedule
* @return schedule
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
public ExternalPaymentScheduleRequest getSchedule() {
return schedule;
}
public void setSchedule(ExternalPaymentScheduleRequest schedule) {
this.schedule = schedule;
}
public PaymentInitiationPaymentCreateRequest options(ExternalPaymentOptions options) {
this.options = options;
return this;
}
/**
* Get options
* @return options
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
public ExternalPaymentOptions getOptions() {
return options;
}
public void setOptions(ExternalPaymentOptions options) {
this.options = options;
}
@Override
public boolean equals(Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
PaymentInitiationPaymentCreateRequest paymentInitiationPaymentCreateRequest = (PaymentInitiationPaymentCreateRequest) o;
return Objects.equals(this.clientId, paymentInitiationPaymentCreateRequest.clientId) &&
Objects.equals(this.secret, paymentInitiationPaymentCreateRequest.secret) &&
Objects.equals(this.recipientId, paymentInitiationPaymentCreateRequest.recipientId) &&
Objects.equals(this.reference, paymentInitiationPaymentCreateRequest.reference) &&
Objects.equals(this.amount, paymentInitiationPaymentCreateRequest.amount) &&
Objects.equals(this.schedule, paymentInitiationPaymentCreateRequest.schedule) &&
Objects.equals(this.options, paymentInitiationPaymentCreateRequest.options);
}
@Override
public int hashCode() {
return Objects.hash(clientId, secret, recipientId, reference, amount, schedule, options);
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class PaymentInitiationPaymentCreateRequest {\n");
sb.append(" clientId: ").append(toIndentedString(clientId)).append("\n");
sb.append(" secret: ").append(toIndentedString(secret)).append("\n");
sb.append(" recipientId: ").append(toIndentedString(recipientId)).append("\n");
sb.append(" reference: ").append(toIndentedString(reference)).append("\n");
sb.append(" amount: ").append(toIndentedString(amount)).append("\n");
sb.append(" schedule: ").append(toIndentedString(schedule)).append("\n");
sb.append(" options: ").append(toIndentedString(options)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces
* (except the first line).
*/
private String toIndentedString(Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy