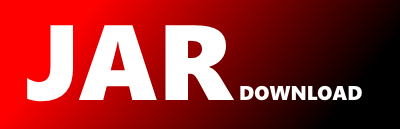
com.plaid.client.model.ProductStatus Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of plaid-java Show documentation
Show all versions of plaid-java Show documentation
Bindings for the Plaid (plaid.com) API.
/*
* The Plaid API
* The Plaid REST API. Please see https://plaid.com/docs/api for more details.
*
* The version of the OpenAPI document: 2020-09-14_1.565.0
*
*
* NOTE: This class is auto generated by OpenAPI Generator (https://openapi-generator.tech).
* https://openapi-generator.tech
* Do not edit the class manually.
*/
package com.plaid.client.model;
import java.util.Objects;
import java.util.Arrays;
import com.google.gson.TypeAdapter;
import com.google.gson.annotations.JsonAdapter;
import com.google.gson.annotations.SerializedName;
import com.google.gson.stream.JsonReader;
import com.google.gson.stream.JsonWriter;
import com.plaid.client.model.ProductStatusBreakdown;
import io.swagger.annotations.ApiModel;
import io.swagger.annotations.ApiModelProperty;
import java.io.IOException;
import java.time.OffsetDateTime;
/**
* A representation of the status health of a request type. Auth requests, Balance requests, Identity requests, Investments requests, Liabilities requests, Transactions updates, Investments updates, Liabilities updates, and Item logins each have their own status object.
*/
@ApiModel(description = "A representation of the status health of a request type. Auth requests, Balance requests, Identity requests, Investments requests, Liabilities requests, Transactions updates, Investments updates, Liabilities updates, and Item logins each have their own status object.")
@javax.annotation.Generated(value = "org.openapitools.codegen.languages.JavaClientCodegen", date = "2024-09-17T16:42:02.150702Z[Etc/UTC]")
public class ProductStatus {
/**
* This field is deprecated in favor of the `breakdown` object, which provides more granular institution health data. `HEALTHY`: the majority of requests are successful `DEGRADED`: only some requests are successful `DOWN`: all requests are failing
*/
@JsonAdapter(StatusEnum.Adapter.class)
public enum StatusEnum {
HEALTHY("HEALTHY"),
DEGRADED("DEGRADED"),
DOWN("DOWN");
private String value;
StatusEnum(String value) {
this.value = value;
}
public String getValue() {
return value;
}
@Override
public String toString() {
return String.valueOf(value);
}
public static StatusEnum fromValue(String value) {
for (StatusEnum b : StatusEnum.values()) {
if (b.value.equals(value)) {
return b;
}
}
throw new IllegalArgumentException("Unexpected value '" + value + "'");
}
public static class Adapter extends TypeAdapter {
@Override
public void write(final JsonWriter jsonWriter, final StatusEnum enumeration) throws IOException {
jsonWriter.value(enumeration.getValue());
}
@Override
public StatusEnum read(final JsonReader jsonReader) throws IOException {
String value = jsonReader.nextString();
return StatusEnum.fromValue(value);
}
}
}
public static final String SERIALIZED_NAME_STATUS = "status";
@SerializedName(SERIALIZED_NAME_STATUS)
private StatusEnum status;
public static final String SERIALIZED_NAME_LAST_STATUS_CHANGE = "last_status_change";
@SerializedName(SERIALIZED_NAME_LAST_STATUS_CHANGE)
private OffsetDateTime lastStatusChange;
public static final String SERIALIZED_NAME_BREAKDOWN = "breakdown";
@SerializedName(SERIALIZED_NAME_BREAKDOWN)
private ProductStatusBreakdown breakdown;
public ProductStatus status(StatusEnum status) {
this.status = status;
return this;
}
/**
* This field is deprecated in favor of the `breakdown` object, which provides more granular institution health data. `HEALTHY`: the majority of requests are successful `DEGRADED`: only some requests are successful `DOWN`: all requests are failing
* @return status
**/
@ApiModelProperty(required = true, value = "This field is deprecated in favor of the `breakdown` object, which provides more granular institution health data. `HEALTHY`: the majority of requests are successful `DEGRADED`: only some requests are successful `DOWN`: all requests are failing")
public StatusEnum getStatus() {
return status;
}
public void setStatus(StatusEnum status) {
this.status = status;
}
public ProductStatus lastStatusChange(OffsetDateTime lastStatusChange) {
this.lastStatusChange = lastStatusChange;
return this;
}
/**
* [ISO 8601](https://wikipedia.org/wiki/ISO_8601) formatted timestamp of the last status change for the institution.
* @return lastStatusChange
**/
@ApiModelProperty(required = true, value = "[ISO 8601](https://wikipedia.org/wiki/ISO_8601) formatted timestamp of the last status change for the institution. ")
public OffsetDateTime getLastStatusChange() {
return lastStatusChange;
}
public void setLastStatusChange(OffsetDateTime lastStatusChange) {
this.lastStatusChange = lastStatusChange;
}
public ProductStatus breakdown(ProductStatusBreakdown breakdown) {
this.breakdown = breakdown;
return this;
}
/**
* Get breakdown
* @return breakdown
**/
@ApiModelProperty(required = true, value = "")
public ProductStatusBreakdown getBreakdown() {
return breakdown;
}
public void setBreakdown(ProductStatusBreakdown breakdown) {
this.breakdown = breakdown;
}
@Override
public boolean equals(Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
ProductStatus productStatus = (ProductStatus) o;
return Objects.equals(this.status, productStatus.status) &&
Objects.equals(this.lastStatusChange, productStatus.lastStatusChange) &&
Objects.equals(this.breakdown, productStatus.breakdown);
}
@Override
public int hashCode() {
return Objects.hash(status, lastStatusChange, breakdown);
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class ProductStatus {\n");
sb.append(" status: ").append(toIndentedString(status)).append("\n");
sb.append(" lastStatusChange: ").append(toIndentedString(lastStatusChange)).append("\n");
sb.append(" breakdown: ").append(toIndentedString(breakdown)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces
* (except the first line).
*/
private String toIndentedString(Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy