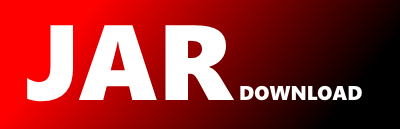
com.plaid.client.model.SandboxTransferLedgerSimulateAvailableRequest Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of plaid-java Show documentation
Show all versions of plaid-java Show documentation
Bindings for the Plaid (plaid.com) API.
/*
* The Plaid API
* The Plaid REST API. Please see https://plaid.com/docs/api for more details.
*
* The version of the OpenAPI document: 2020-09-14_1.565.0
*
*
* NOTE: This class is auto generated by OpenAPI Generator (https://openapi-generator.tech).
* https://openapi-generator.tech
* Do not edit the class manually.
*/
package com.plaid.client.model;
import java.util.Objects;
import java.util.Arrays;
import com.google.gson.TypeAdapter;
import com.google.gson.annotations.JsonAdapter;
import com.google.gson.annotations.SerializedName;
import com.google.gson.stream.JsonReader;
import com.google.gson.stream.JsonWriter;
import io.swagger.annotations.ApiModel;
import io.swagger.annotations.ApiModelProperty;
import java.io.IOException;
/**
* Defines the request schema for `/sandbox/transfer/ledger/simulate_available`
*/
@ApiModel(description = "Defines the request schema for `/sandbox/transfer/ledger/simulate_available`")
@javax.annotation.Generated(value = "org.openapitools.codegen.languages.JavaClientCodegen", date = "2024-09-17T16:42:02.150702Z[Etc/UTC]")
public class SandboxTransferLedgerSimulateAvailableRequest {
public static final String SERIALIZED_NAME_CLIENT_ID = "client_id";
@SerializedName(SERIALIZED_NAME_CLIENT_ID)
private String clientId;
public static final String SERIALIZED_NAME_SECRET = "secret";
@SerializedName(SERIALIZED_NAME_SECRET)
private String secret;
public static final String SERIALIZED_NAME_LEDGER_ID = "ledger_id";
@SerializedName(SERIALIZED_NAME_LEDGER_ID)
private String ledgerId;
public static final String SERIALIZED_NAME_TEST_CLOCK_ID = "test_clock_id";
@SerializedName(SERIALIZED_NAME_TEST_CLOCK_ID)
private String testClockId;
public SandboxTransferLedgerSimulateAvailableRequest clientId(String clientId) {
this.clientId = clientId;
return this;
}
/**
* Your Plaid API `client_id`. The `client_id` is required and may be provided either in the `PLAID-CLIENT-ID` header or as part of a request body.
* @return clientId
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Your Plaid API `client_id`. The `client_id` is required and may be provided either in the `PLAID-CLIENT-ID` header or as part of a request body.")
public String getClientId() {
return clientId;
}
public void setClientId(String clientId) {
this.clientId = clientId;
}
public SandboxTransferLedgerSimulateAvailableRequest secret(String secret) {
this.secret = secret;
return this;
}
/**
* Your Plaid API `secret`. The `secret` is required and may be provided either in the `PLAID-SECRET` header or as part of a request body.
* @return secret
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Your Plaid API `secret`. The `secret` is required and may be provided either in the `PLAID-SECRET` header or as part of a request body.")
public String getSecret() {
return secret;
}
public void setSecret(String secret) {
this.secret = secret;
}
public SandboxTransferLedgerSimulateAvailableRequest ledgerId(String ledgerId) {
this.ledgerId = ledgerId;
return this;
}
/**
* Specify which ledger balance to simulate converting pending balance to available balance. If this field is left blank, this will default to id of the default ledger balance.
* @return ledgerId
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Specify which ledger balance to simulate converting pending balance to available balance. If this field is left blank, this will default to id of the default ledger balance.")
public String getLedgerId() {
return ledgerId;
}
public void setLedgerId(String ledgerId) {
this.ledgerId = ledgerId;
}
public SandboxTransferLedgerSimulateAvailableRequest testClockId(String testClockId) {
this.testClockId = testClockId;
return this;
}
/**
* Plaid’s unique identifier for a test clock. If provided, only the pending balance that is due before the `virtual_timestamp` on the test clock will be converted.
* @return testClockId
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Plaid’s unique identifier for a test clock. If provided, only the pending balance that is due before the `virtual_timestamp` on the test clock will be converted.")
public String getTestClockId() {
return testClockId;
}
public void setTestClockId(String testClockId) {
this.testClockId = testClockId;
}
@Override
public boolean equals(Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
SandboxTransferLedgerSimulateAvailableRequest sandboxTransferLedgerSimulateAvailableRequest = (SandboxTransferLedgerSimulateAvailableRequest) o;
return Objects.equals(this.clientId, sandboxTransferLedgerSimulateAvailableRequest.clientId) &&
Objects.equals(this.secret, sandboxTransferLedgerSimulateAvailableRequest.secret) &&
Objects.equals(this.ledgerId, sandboxTransferLedgerSimulateAvailableRequest.ledgerId) &&
Objects.equals(this.testClockId, sandboxTransferLedgerSimulateAvailableRequest.testClockId);
}
@Override
public int hashCode() {
return Objects.hash(clientId, secret, ledgerId, testClockId);
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class SandboxTransferLedgerSimulateAvailableRequest {\n");
sb.append(" clientId: ").append(toIndentedString(clientId)).append("\n");
sb.append(" secret: ").append(toIndentedString(secret)).append("\n");
sb.append(" ledgerId: ").append(toIndentedString(ledgerId)).append("\n");
sb.append(" testClockId: ").append(toIndentedString(testClockId)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces
* (except the first line).
*/
private String toIndentedString(Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy