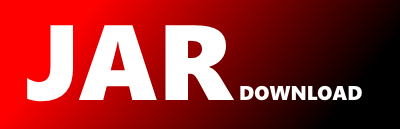
com.plaid.client.model.TransactionBase Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of plaid-java Show documentation
Show all versions of plaid-java Show documentation
Bindings for the Plaid (plaid.com) API.
/*
* The Plaid API
* The Plaid REST API. Please see https://plaid.com/docs/api for more details.
*
* The version of the OpenAPI document: 2020-09-14_1.565.0
*
*
* NOTE: This class is auto generated by OpenAPI Generator (https://openapi-generator.tech).
* https://openapi-generator.tech
* Do not edit the class manually.
*/
package com.plaid.client.model;
import java.util.Objects;
import java.util.Arrays;
import com.google.gson.TypeAdapter;
import com.google.gson.annotations.JsonAdapter;
import com.google.gson.annotations.SerializedName;
import com.google.gson.stream.JsonReader;
import com.google.gson.stream.JsonWriter;
import com.plaid.client.model.Location;
import com.plaid.client.model.PaymentMeta;
import io.swagger.annotations.ApiModel;
import io.swagger.annotations.ApiModelProperty;
import java.io.IOException;
import java.time.LocalDate;
import java.util.ArrayList;
import java.util.List;
/**
* A representation of a transaction
*/
@ApiModel(description = "A representation of a transaction")
@javax.annotation.Generated(value = "org.openapitools.codegen.languages.JavaClientCodegen", date = "2024-09-17T16:42:02.150702Z[Etc/UTC]")
public class TransactionBase {
public static final String SERIALIZED_NAME_ACCOUNT_ID = "account_id";
@SerializedName(SERIALIZED_NAME_ACCOUNT_ID)
private String accountId;
public static final String SERIALIZED_NAME_AMOUNT = "amount";
@SerializedName(SERIALIZED_NAME_AMOUNT)
private Double amount;
public static final String SERIALIZED_NAME_ISO_CURRENCY_CODE = "iso_currency_code";
@SerializedName(SERIALIZED_NAME_ISO_CURRENCY_CODE)
private String isoCurrencyCode;
public static final String SERIALIZED_NAME_UNOFFICIAL_CURRENCY_CODE = "unofficial_currency_code";
@SerializedName(SERIALIZED_NAME_UNOFFICIAL_CURRENCY_CODE)
private String unofficialCurrencyCode;
public static final String SERIALIZED_NAME_CATEGORY = "category";
@SerializedName(SERIALIZED_NAME_CATEGORY)
private List category = null;
public static final String SERIALIZED_NAME_CATEGORY_ID = "category_id";
@SerializedName(SERIALIZED_NAME_CATEGORY_ID)
private String categoryId;
public static final String SERIALIZED_NAME_CHECK_NUMBER = "check_number";
@SerializedName(SERIALIZED_NAME_CHECK_NUMBER)
private String checkNumber;
public static final String SERIALIZED_NAME_DATE = "date";
@SerializedName(SERIALIZED_NAME_DATE)
private LocalDate date;
public static final String SERIALIZED_NAME_LOCATION = "location";
@SerializedName(SERIALIZED_NAME_LOCATION)
private Location location;
public static final String SERIALIZED_NAME_NAME = "name";
@SerializedName(SERIALIZED_NAME_NAME)
private String name;
public static final String SERIALIZED_NAME_MERCHANT_NAME = "merchant_name";
@SerializedName(SERIALIZED_NAME_MERCHANT_NAME)
private String merchantName;
public static final String SERIALIZED_NAME_ORIGINAL_DESCRIPTION = "original_description";
@SerializedName(SERIALIZED_NAME_ORIGINAL_DESCRIPTION)
private String originalDescription;
public static final String SERIALIZED_NAME_PAYMENT_META = "payment_meta";
@SerializedName(SERIALIZED_NAME_PAYMENT_META)
private PaymentMeta paymentMeta;
public static final String SERIALIZED_NAME_PENDING = "pending";
@SerializedName(SERIALIZED_NAME_PENDING)
private Boolean pending;
public static final String SERIALIZED_NAME_PENDING_TRANSACTION_ID = "pending_transaction_id";
@SerializedName(SERIALIZED_NAME_PENDING_TRANSACTION_ID)
private String pendingTransactionId;
public static final String SERIALIZED_NAME_ACCOUNT_OWNER = "account_owner";
@SerializedName(SERIALIZED_NAME_ACCOUNT_OWNER)
private String accountOwner;
public static final String SERIALIZED_NAME_TRANSACTION_ID = "transaction_id";
@SerializedName(SERIALIZED_NAME_TRANSACTION_ID)
private String transactionId;
/**
* Please use the `payment_channel` field, `transaction_type` will be deprecated in the future. `digital:` transactions that took place online. `place:` transactions that were made at a physical location. `special:` transactions that relate to banks, e.g. fees or deposits. `unresolved:` transactions that do not fit into the other three types.
*/
@JsonAdapter(TransactionTypeEnum.Adapter.class)
public enum TransactionTypeEnum {
DIGITAL("digital"),
PLACE("place"),
SPECIAL("special"),
UNRESOLVED("unresolved");
private String value;
TransactionTypeEnum(String value) {
this.value = value;
}
public String getValue() {
return value;
}
@Override
public String toString() {
return String.valueOf(value);
}
public static TransactionTypeEnum fromValue(String value) {
for (TransactionTypeEnum b : TransactionTypeEnum.values()) {
if (b.value.equals(value)) {
return b;
}
}
throw new IllegalArgumentException("Unexpected value '" + value + "'");
}
public static class Adapter extends TypeAdapter {
@Override
public void write(final JsonWriter jsonWriter, final TransactionTypeEnum enumeration) throws IOException {
jsonWriter.value(enumeration.getValue());
}
@Override
public TransactionTypeEnum read(final JsonReader jsonReader) throws IOException {
String value = jsonReader.nextString();
return TransactionTypeEnum.fromValue(value);
}
}
}
public static final String SERIALIZED_NAME_TRANSACTION_TYPE = "transaction_type";
@SerializedName(SERIALIZED_NAME_TRANSACTION_TYPE)
private TransactionTypeEnum transactionType;
public static final String SERIALIZED_NAME_LOGO_URL = "logo_url";
@SerializedName(SERIALIZED_NAME_LOGO_URL)
private String logoUrl;
public static final String SERIALIZED_NAME_WEBSITE = "website";
@SerializedName(SERIALIZED_NAME_WEBSITE)
private String website;
public TransactionBase accountId(String accountId) {
this.accountId = accountId;
return this;
}
/**
* The ID of the account in which this transaction occurred.
* @return accountId
**/
@ApiModelProperty(required = true, value = "The ID of the account in which this transaction occurred.")
public String getAccountId() {
return accountId;
}
public void setAccountId(String accountId) {
this.accountId = accountId;
}
public TransactionBase amount(Double amount) {
this.amount = amount;
return this;
}
/**
* The settled value of the transaction, denominated in the transactions's currency, as stated in `iso_currency_code` or `unofficial_currency_code`. For all products except Income: Positive values when money moves out of the account; negative values when money moves in. For example, debit card purchases are positive; credit card payments, direct deposits, and refunds are negative. For Income endpoints, values are positive when representing income.
* @return amount
**/
@ApiModelProperty(required = true, value = "The settled value of the transaction, denominated in the transactions's currency, as stated in `iso_currency_code` or `unofficial_currency_code`. For all products except Income: Positive values when money moves out of the account; negative values when money moves in. For example, debit card purchases are positive; credit card payments, direct deposits, and refunds are negative. For Income endpoints, values are positive when representing income.")
public Double getAmount() {
return amount;
}
public void setAmount(Double amount) {
this.amount = amount;
}
public TransactionBase isoCurrencyCode(String isoCurrencyCode) {
this.isoCurrencyCode = isoCurrencyCode;
return this;
}
/**
* The ISO-4217 currency code of the transaction. Always `null` if `unofficial_currency_code` is non-null.
* @return isoCurrencyCode
**/
@javax.annotation.Nullable
@ApiModelProperty(required = true, value = "The ISO-4217 currency code of the transaction. Always `null` if `unofficial_currency_code` is non-null.")
public String getIsoCurrencyCode() {
return isoCurrencyCode;
}
public void setIsoCurrencyCode(String isoCurrencyCode) {
this.isoCurrencyCode = isoCurrencyCode;
}
public TransactionBase unofficialCurrencyCode(String unofficialCurrencyCode) {
this.unofficialCurrencyCode = unofficialCurrencyCode;
return this;
}
/**
* The unofficial currency code associated with the transaction. Always `null` if `iso_currency_code` is non-`null`. Unofficial currency codes are used for currencies that do not have official ISO currency codes, such as cryptocurrencies and the currencies of certain countries. See the [currency code schema](https://plaid.com/docs/api/accounts#currency-code-schema) for a full listing of supported `iso_currency_code`s.
* @return unofficialCurrencyCode
**/
@javax.annotation.Nullable
@ApiModelProperty(required = true, value = "The unofficial currency code associated with the transaction. Always `null` if `iso_currency_code` is non-`null`. Unofficial currency codes are used for currencies that do not have official ISO currency codes, such as cryptocurrencies and the currencies of certain countries. See the [currency code schema](https://plaid.com/docs/api/accounts#currency-code-schema) for a full listing of supported `iso_currency_code`s.")
public String getUnofficialCurrencyCode() {
return unofficialCurrencyCode;
}
public void setUnofficialCurrencyCode(String unofficialCurrencyCode) {
this.unofficialCurrencyCode = unofficialCurrencyCode;
}
public TransactionBase category(List category) {
this.category = category;
return this;
}
public TransactionBase addCategoryItem(String categoryItem) {
if (this.category == null) {
this.category = new ArrayList<>();
}
this.category.add(categoryItem);
return this;
}
/**
* A hierarchical array of the categories to which this transaction belongs. For a full list of categories, see [`/categories/get`](https://plaid.com/docs/api/products/transactions/#categoriesget). All Transactions implementations are recommended to use the new `personal_finance_category` instead of `category`, as it provides greater accuracy and more meaningful categorization. If the `transactions` object was returned by an Assets endpoint such as `/asset_report/get/` or `/asset_report/pdf/get`, this field will only appear in an Asset Report with Insights.
* @return category
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "A hierarchical array of the categories to which this transaction belongs. For a full list of categories, see [`/categories/get`](https://plaid.com/docs/api/products/transactions/#categoriesget). All Transactions implementations are recommended to use the new `personal_finance_category` instead of `category`, as it provides greater accuracy and more meaningful categorization. If the `transactions` object was returned by an Assets endpoint such as `/asset_report/get/` or `/asset_report/pdf/get`, this field will only appear in an Asset Report with Insights.")
public List getCategory() {
return category;
}
public void setCategory(List category) {
this.category = category;
}
public TransactionBase categoryId(String categoryId) {
this.categoryId = categoryId;
return this;
}
/**
* The ID of the category to which this transaction belongs. For a full list of categories, see [`/categories/get`](https://plaid.com/docs/api/products/transactions/#categoriesget). All Transactions implementations are recommended to use the new `personal_finance_category` instead of `category`, as it provides greater accuracy and more meaningful categorization. If the `transactions` object was returned by an Assets endpoint such as `/asset_report/get/` or `/asset_report/pdf/get`, this field will only appear in an Asset Report with Insights.
* @return categoryId
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "The ID of the category to which this transaction belongs. For a full list of categories, see [`/categories/get`](https://plaid.com/docs/api/products/transactions/#categoriesget). All Transactions implementations are recommended to use the new `personal_finance_category` instead of `category`, as it provides greater accuracy and more meaningful categorization. If the `transactions` object was returned by an Assets endpoint such as `/asset_report/get/` or `/asset_report/pdf/get`, this field will only appear in an Asset Report with Insights.")
public String getCategoryId() {
return categoryId;
}
public void setCategoryId(String categoryId) {
this.categoryId = categoryId;
}
public TransactionBase checkNumber(String checkNumber) {
this.checkNumber = checkNumber;
return this;
}
/**
* The check number of the transaction. This field is only populated for check transactions.
* @return checkNumber
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "The check number of the transaction. This field is only populated for check transactions.")
public String getCheckNumber() {
return checkNumber;
}
public void setCheckNumber(String checkNumber) {
this.checkNumber = checkNumber;
}
public TransactionBase date(LocalDate date) {
this.date = date;
return this;
}
/**
* For pending transactions, the date that the transaction occurred; for posted transactions, the date that the transaction posted. Both dates are returned in an [ISO 8601](https://wikipedia.org/wiki/ISO_8601) format ( `YYYY-MM-DD` ). To receive information about the date that a posted transaction was initiated, see the `authorized_date` field.
* @return date
**/
@ApiModelProperty(required = true, value = "For pending transactions, the date that the transaction occurred; for posted transactions, the date that the transaction posted. Both dates are returned in an [ISO 8601](https://wikipedia.org/wiki/ISO_8601) format ( `YYYY-MM-DD` ). To receive information about the date that a posted transaction was initiated, see the `authorized_date` field.")
public LocalDate getDate() {
return date;
}
public void setDate(LocalDate date) {
this.date = date;
}
public TransactionBase location(Location location) {
this.location = location;
return this;
}
/**
* Get location
* @return location
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
public Location getLocation() {
return location;
}
public void setLocation(Location location) {
this.location = location;
}
public TransactionBase name(String name) {
this.name = name;
return this;
}
/**
* The merchant name or transaction description. Note: This is a legacy field that is not actively maintained. Use `merchant_name` instead for the merchant name. If the `transactions` object was returned by a Transactions endpoint such as `/transactions/sync` or `/transactions/get`, this field will always appear. If the `transactions` object was returned by an Assets endpoint such as `/asset_report/get/` or `/asset_report/pdf/get`, this field will only appear in an Asset Report with Insights.
* @return name
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "The merchant name or transaction description. Note: This is a legacy field that is not actively maintained. Use `merchant_name` instead for the merchant name. If the `transactions` object was returned by a Transactions endpoint such as `/transactions/sync` or `/transactions/get`, this field will always appear. If the `transactions` object was returned by an Assets endpoint such as `/asset_report/get/` or `/asset_report/pdf/get`, this field will only appear in an Asset Report with Insights.")
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public TransactionBase merchantName(String merchantName) {
this.merchantName = merchantName;
return this;
}
/**
* The merchant name, as enriched by Plaid from the `name` field. This is typically a more human-readable version of the merchant counterparty in the transaction. For some bank transactions (such as checks or account transfers) where there is no meaningful merchant name, this value will be `null`.
* @return merchantName
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "The merchant name, as enriched by Plaid from the `name` field. This is typically a more human-readable version of the merchant counterparty in the transaction. For some bank transactions (such as checks or account transfers) where there is no meaningful merchant name, this value will be `null`.")
public String getMerchantName() {
return merchantName;
}
public void setMerchantName(String merchantName) {
this.merchantName = merchantName;
}
public TransactionBase originalDescription(String originalDescription) {
this.originalDescription = originalDescription;
return this;
}
/**
* The string returned by the financial institution to describe the transaction. For transactions returned by `/transactions/sync` or `/transactions/get`, this field will be omitted unless the client has set `options.include_original_description` to `true`.
* @return originalDescription
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "The string returned by the financial institution to describe the transaction. For transactions returned by `/transactions/sync` or `/transactions/get`, this field will be omitted unless the client has set `options.include_original_description` to `true`.")
public String getOriginalDescription() {
return originalDescription;
}
public void setOriginalDescription(String originalDescription) {
this.originalDescription = originalDescription;
}
public TransactionBase paymentMeta(PaymentMeta paymentMeta) {
this.paymentMeta = paymentMeta;
return this;
}
/**
* Get paymentMeta
* @return paymentMeta
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
public PaymentMeta getPaymentMeta() {
return paymentMeta;
}
public void setPaymentMeta(PaymentMeta paymentMeta) {
this.paymentMeta = paymentMeta;
}
public TransactionBase pending(Boolean pending) {
this.pending = pending;
return this;
}
/**
* When `true`, identifies the transaction as pending or unsettled. Pending transaction details (name, type, amount, category ID) may change before they are settled. Not all institutions provide pending transactions.
* @return pending
**/
@ApiModelProperty(required = true, value = "When `true`, identifies the transaction as pending or unsettled. Pending transaction details (name, type, amount, category ID) may change before they are settled. Not all institutions provide pending transactions.")
public Boolean getPending() {
return pending;
}
public void setPending(Boolean pending) {
this.pending = pending;
}
public TransactionBase pendingTransactionId(String pendingTransactionId) {
this.pendingTransactionId = pendingTransactionId;
return this;
}
/**
* The ID of a posted transaction's associated pending transaction, where applicable. Not all institutions provide pending transactions.
* @return pendingTransactionId
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "The ID of a posted transaction's associated pending transaction, where applicable. Not all institutions provide pending transactions.")
public String getPendingTransactionId() {
return pendingTransactionId;
}
public void setPendingTransactionId(String pendingTransactionId) {
this.pendingTransactionId = pendingTransactionId;
}
public TransactionBase accountOwner(String accountOwner) {
this.accountOwner = accountOwner;
return this;
}
/**
* The name of the account owner. This field is not typically populated and only relevant when dealing with sub-accounts.
* @return accountOwner
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "The name of the account owner. This field is not typically populated and only relevant when dealing with sub-accounts.")
public String getAccountOwner() {
return accountOwner;
}
public void setAccountOwner(String accountOwner) {
this.accountOwner = accountOwner;
}
public TransactionBase transactionId(String transactionId) {
this.transactionId = transactionId;
return this;
}
/**
* The unique ID of the transaction. Like all Plaid identifiers, the `transaction_id` is case sensitive.
* @return transactionId
**/
@ApiModelProperty(required = true, value = "The unique ID of the transaction. Like all Plaid identifiers, the `transaction_id` is case sensitive.")
public String getTransactionId() {
return transactionId;
}
public void setTransactionId(String transactionId) {
this.transactionId = transactionId;
}
public TransactionBase transactionType(TransactionTypeEnum transactionType) {
this.transactionType = transactionType;
return this;
}
/**
* Please use the `payment_channel` field, `transaction_type` will be deprecated in the future. `digital:` transactions that took place online. `place:` transactions that were made at a physical location. `special:` transactions that relate to banks, e.g. fees or deposits. `unresolved:` transactions that do not fit into the other three types.
* @return transactionType
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Please use the `payment_channel` field, `transaction_type` will be deprecated in the future. `digital:` transactions that took place online. `place:` transactions that were made at a physical location. `special:` transactions that relate to banks, e.g. fees or deposits. `unresolved:` transactions that do not fit into the other three types. ")
public TransactionTypeEnum getTransactionType() {
return transactionType;
}
public void setTransactionType(TransactionTypeEnum transactionType) {
this.transactionType = transactionType;
}
public TransactionBase logoUrl(String logoUrl) {
this.logoUrl = logoUrl;
return this;
}
/**
* The URL of a logo associated with this transaction, if available. The logo will always be 100×100 pixel PNG file.
* @return logoUrl
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "The URL of a logo associated with this transaction, if available. The logo will always be 100×100 pixel PNG file.")
public String getLogoUrl() {
return logoUrl;
}
public void setLogoUrl(String logoUrl) {
this.logoUrl = logoUrl;
}
public TransactionBase website(String website) {
this.website = website;
return this;
}
/**
* The website associated with this transaction, if available.
* @return website
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "The website associated with this transaction, if available.")
public String getWebsite() {
return website;
}
public void setWebsite(String website) {
this.website = website;
}
@Override
public boolean equals(Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
TransactionBase transactionBase = (TransactionBase) o;
return Objects.equals(this.accountId, transactionBase.accountId) &&
Objects.equals(this.amount, transactionBase.amount) &&
Objects.equals(this.isoCurrencyCode, transactionBase.isoCurrencyCode) &&
Objects.equals(this.unofficialCurrencyCode, transactionBase.unofficialCurrencyCode) &&
Objects.equals(this.category, transactionBase.category) &&
Objects.equals(this.categoryId, transactionBase.categoryId) &&
Objects.equals(this.checkNumber, transactionBase.checkNumber) &&
Objects.equals(this.date, transactionBase.date) &&
Objects.equals(this.location, transactionBase.location) &&
Objects.equals(this.name, transactionBase.name) &&
Objects.equals(this.merchantName, transactionBase.merchantName) &&
Objects.equals(this.originalDescription, transactionBase.originalDescription) &&
Objects.equals(this.paymentMeta, transactionBase.paymentMeta) &&
Objects.equals(this.pending, transactionBase.pending) &&
Objects.equals(this.pendingTransactionId, transactionBase.pendingTransactionId) &&
Objects.equals(this.accountOwner, transactionBase.accountOwner) &&
Objects.equals(this.transactionId, transactionBase.transactionId) &&
Objects.equals(this.transactionType, transactionBase.transactionType) &&
Objects.equals(this.logoUrl, transactionBase.logoUrl) &&
Objects.equals(this.website, transactionBase.website);
}
@Override
public int hashCode() {
return Objects.hash(accountId, amount, isoCurrencyCode, unofficialCurrencyCode, category, categoryId, checkNumber, date, location, name, merchantName, originalDescription, paymentMeta, pending, pendingTransactionId, accountOwner, transactionId, transactionType, logoUrl, website);
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class TransactionBase {\n");
sb.append(" accountId: ").append(toIndentedString(accountId)).append("\n");
sb.append(" amount: ").append(toIndentedString(amount)).append("\n");
sb.append(" isoCurrencyCode: ").append(toIndentedString(isoCurrencyCode)).append("\n");
sb.append(" unofficialCurrencyCode: ").append(toIndentedString(unofficialCurrencyCode)).append("\n");
sb.append(" category: ").append(toIndentedString(category)).append("\n");
sb.append(" categoryId: ").append(toIndentedString(categoryId)).append("\n");
sb.append(" checkNumber: ").append(toIndentedString(checkNumber)).append("\n");
sb.append(" date: ").append(toIndentedString(date)).append("\n");
sb.append(" location: ").append(toIndentedString(location)).append("\n");
sb.append(" name: ").append(toIndentedString(name)).append("\n");
sb.append(" merchantName: ").append(toIndentedString(merchantName)).append("\n");
sb.append(" originalDescription: ").append(toIndentedString(originalDescription)).append("\n");
sb.append(" paymentMeta: ").append(toIndentedString(paymentMeta)).append("\n");
sb.append(" pending: ").append(toIndentedString(pending)).append("\n");
sb.append(" pendingTransactionId: ").append(toIndentedString(pendingTransactionId)).append("\n");
sb.append(" accountOwner: ").append(toIndentedString(accountOwner)).append("\n");
sb.append(" transactionId: ").append(toIndentedString(transactionId)).append("\n");
sb.append(" transactionType: ").append(toIndentedString(transactionType)).append("\n");
sb.append(" logoUrl: ").append(toIndentedString(logoUrl)).append("\n");
sb.append(" website: ").append(toIndentedString(website)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces
* (except the first line).
*/
private String toIndentedString(Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy