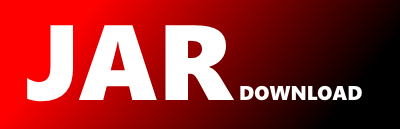
com.plaid.client.model.TransactionsGetRequestOptions Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of plaid-java Show documentation
Show all versions of plaid-java Show documentation
Bindings for the Plaid (plaid.com) API.
/*
* The Plaid API
* The Plaid REST API. Please see https://plaid.com/docs/api for more details.
*
* The version of the OpenAPI document: 2020-09-14_1.565.0
*
*
* NOTE: This class is auto generated by OpenAPI Generator (https://openapi-generator.tech).
* https://openapi-generator.tech
* Do not edit the class manually.
*/
package com.plaid.client.model;
import java.util.Objects;
import java.util.Arrays;
import com.google.gson.TypeAdapter;
import com.google.gson.annotations.JsonAdapter;
import com.google.gson.annotations.SerializedName;
import com.google.gson.stream.JsonReader;
import com.google.gson.stream.JsonWriter;
import io.swagger.annotations.ApiModel;
import io.swagger.annotations.ApiModelProperty;
import java.io.IOException;
import java.util.ArrayList;
import java.util.List;
/**
* An optional object to be used with the request. If specified, `options` must not be `null`.
*/
@ApiModel(description = "An optional object to be used with the request. If specified, `options` must not be `null`.")
@javax.annotation.Generated(value = "org.openapitools.codegen.languages.JavaClientCodegen", date = "2024-09-17T16:42:02.150702Z[Etc/UTC]")
public class TransactionsGetRequestOptions {
public static final String SERIALIZED_NAME_ACCOUNT_IDS = "account_ids";
@SerializedName(SERIALIZED_NAME_ACCOUNT_IDS)
private List accountIds = null;
public static final String SERIALIZED_NAME_COUNT = "count";
@SerializedName(SERIALIZED_NAME_COUNT)
private Integer count = 100;
public static final String SERIALIZED_NAME_OFFSET = "offset";
@SerializedName(SERIALIZED_NAME_OFFSET)
private Integer offset = 0;
public static final String SERIALIZED_NAME_INCLUDE_ORIGINAL_DESCRIPTION = "include_original_description";
@SerializedName(SERIALIZED_NAME_INCLUDE_ORIGINAL_DESCRIPTION)
private Boolean includeOriginalDescription = false;
public static final String SERIALIZED_NAME_INCLUDE_PERSONAL_FINANCE_CATEGORY_BETA = "include_personal_finance_category_beta";
@SerializedName(SERIALIZED_NAME_INCLUDE_PERSONAL_FINANCE_CATEGORY_BETA)
private Boolean includePersonalFinanceCategoryBeta = false;
public static final String SERIALIZED_NAME_INCLUDE_PERSONAL_FINANCE_CATEGORY = "include_personal_finance_category";
@SerializedName(SERIALIZED_NAME_INCLUDE_PERSONAL_FINANCE_CATEGORY)
private Boolean includePersonalFinanceCategory = false;
public static final String SERIALIZED_NAME_INCLUDE_LOGO_AND_COUNTERPARTY_BETA = "include_logo_and_counterparty_beta";
@SerializedName(SERIALIZED_NAME_INCLUDE_LOGO_AND_COUNTERPARTY_BETA)
private Boolean includeLogoAndCounterpartyBeta = false;
public static final String SERIALIZED_NAME_DAYS_REQUESTED = "days_requested";
@SerializedName(SERIALIZED_NAME_DAYS_REQUESTED)
private Integer daysRequested = 90;
public TransactionsGetRequestOptions accountIds(List accountIds) {
this.accountIds = accountIds;
return this;
}
public TransactionsGetRequestOptions addAccountIdsItem(String accountIdsItem) {
if (this.accountIds == null) {
this.accountIds = new ArrayList<>();
}
this.accountIds.add(accountIdsItem);
return this;
}
/**
* A list of `account_ids` to retrieve for the Item Note: An error will be returned if a provided `account_id` is not associated with the Item.
* @return accountIds
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "A list of `account_ids` to retrieve for the Item Note: An error will be returned if a provided `account_id` is not associated with the Item.")
public List getAccountIds() {
return accountIds;
}
public void setAccountIds(List accountIds) {
this.accountIds = accountIds;
}
public TransactionsGetRequestOptions count(Integer count) {
this.count = count;
return this;
}
/**
* The number of transactions to fetch.
* minimum: 1
* maximum: 500
* @return count
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "The number of transactions to fetch.")
public Integer getCount() {
return count;
}
public void setCount(Integer count) {
this.count = count;
}
public TransactionsGetRequestOptions offset(Integer offset) {
this.offset = offset;
return this;
}
/**
* The number of transactions to skip. The default value is 0.
* minimum: 0
* @return offset
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "The number of transactions to skip. The default value is 0.")
public Integer getOffset() {
return offset;
}
public void setOffset(Integer offset) {
this.offset = offset;
}
public TransactionsGetRequestOptions includeOriginalDescription(Boolean includeOriginalDescription) {
this.includeOriginalDescription = includeOriginalDescription;
return this;
}
/**
* Include the raw unparsed transaction description from the financial institution.
* @return includeOriginalDescription
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Include the raw unparsed transaction description from the financial institution.")
public Boolean getIncludeOriginalDescription() {
return includeOriginalDescription;
}
public void setIncludeOriginalDescription(Boolean includeOriginalDescription) {
this.includeOriginalDescription = includeOriginalDescription;
}
public TransactionsGetRequestOptions includePersonalFinanceCategoryBeta(Boolean includePersonalFinanceCategoryBeta) {
this.includePersonalFinanceCategoryBeta = includePersonalFinanceCategoryBeta;
return this;
}
/**
* Personal finance categories are now returned by default.
* @return includePersonalFinanceCategoryBeta
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Personal finance categories are now returned by default.")
public Boolean getIncludePersonalFinanceCategoryBeta() {
return includePersonalFinanceCategoryBeta;
}
public void setIncludePersonalFinanceCategoryBeta(Boolean includePersonalFinanceCategoryBeta) {
this.includePersonalFinanceCategoryBeta = includePersonalFinanceCategoryBeta;
}
public TransactionsGetRequestOptions includePersonalFinanceCategory(Boolean includePersonalFinanceCategory) {
this.includePersonalFinanceCategory = includePersonalFinanceCategory;
return this;
}
/**
* Personal finance categories are now returned by default.
* @return includePersonalFinanceCategory
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Personal finance categories are now returned by default.")
public Boolean getIncludePersonalFinanceCategory() {
return includePersonalFinanceCategory;
}
public void setIncludePersonalFinanceCategory(Boolean includePersonalFinanceCategory) {
this.includePersonalFinanceCategory = includePersonalFinanceCategory;
}
public TransactionsGetRequestOptions includeLogoAndCounterpartyBeta(Boolean includeLogoAndCounterpartyBeta) {
this.includeLogoAndCounterpartyBeta = includeLogoAndCounterpartyBeta;
return this;
}
/**
* Counterparties and extra merchant fields are now returned by default.
* @return includeLogoAndCounterpartyBeta
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Counterparties and extra merchant fields are now returned by default.")
public Boolean getIncludeLogoAndCounterpartyBeta() {
return includeLogoAndCounterpartyBeta;
}
public void setIncludeLogoAndCounterpartyBeta(Boolean includeLogoAndCounterpartyBeta) {
this.includeLogoAndCounterpartyBeta = includeLogoAndCounterpartyBeta;
}
public TransactionsGetRequestOptions daysRequested(Integer daysRequested) {
this.daysRequested = daysRequested;
return this;
}
/**
* This field only applies to calls for Items where the Transactions product has not already been initialized (i.e. by specifying `transactions` in the `products`, `optional_products`, or `required_if_consented_products` array when calling `/link/token/create` or by making a previous call to `/transactions/sync` or `/transactions/get`). In those cases, the field controls the maximum number of days of transaction history that Plaid will request from the financial institution. The more transaction history is requested, the longer the historical update poll will take. If no value is specified, 90 days of history will be requested by default. If a value under 30 is provided, a minimum of 30 days of history will be requested. If you are initializing your Items with transactions during the `/link/token/create` call (e.g. by including `transactions` in the `/link/token/create` `products` array), you must use the [`transactions.days_requested`](https://plaid.com/docs/api/link/#link-token-create-request-transactions-days-requested) field in the `/link/token/create` request instead of in the `/transactions/get` request. If the Item has already been initialized with the Transactions product, this field will have no effect. The maximum amount of transaction history to request on an Item cannot be updated if Transactions has already been added to the Item. To request older transaction history on an Item where Transactions has already been added, you must delete the Item via `/item/remove` and send the user through Link to create a new Item. Customers using [Recurring Transactions](https://plaid.com/docs/api/products/transactions/#transactionsrecurringget) should request at least 180 days of history for optimal results.
* minimum: 1
* maximum: 730
* @return daysRequested
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "This field only applies to calls for Items where the Transactions product has not already been initialized (i.e. by specifying `transactions` in the `products`, `optional_products`, or `required_if_consented_products` array when calling `/link/token/create` or by making a previous call to `/transactions/sync` or `/transactions/get`). In those cases, the field controls the maximum number of days of transaction history that Plaid will request from the financial institution. The more transaction history is requested, the longer the historical update poll will take. If no value is specified, 90 days of history will be requested by default. If a value under 30 is provided, a minimum of 30 days of history will be requested. If you are initializing your Items with transactions during the `/link/token/create` call (e.g. by including `transactions` in the `/link/token/create` `products` array), you must use the [`transactions.days_requested`](https://plaid.com/docs/api/link/#link-token-create-request-transactions-days-requested) field in the `/link/token/create` request instead of in the `/transactions/get` request. If the Item has already been initialized with the Transactions product, this field will have no effect. The maximum amount of transaction history to request on an Item cannot be updated if Transactions has already been added to the Item. To request older transaction history on an Item where Transactions has already been added, you must delete the Item via `/item/remove` and send the user through Link to create a new Item. Customers using [Recurring Transactions](https://plaid.com/docs/api/products/transactions/#transactionsrecurringget) should request at least 180 days of history for optimal results.")
public Integer getDaysRequested() {
return daysRequested;
}
public void setDaysRequested(Integer daysRequested) {
this.daysRequested = daysRequested;
}
@Override
public boolean equals(Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
TransactionsGetRequestOptions transactionsGetRequestOptions = (TransactionsGetRequestOptions) o;
return Objects.equals(this.accountIds, transactionsGetRequestOptions.accountIds) &&
Objects.equals(this.count, transactionsGetRequestOptions.count) &&
Objects.equals(this.offset, transactionsGetRequestOptions.offset) &&
Objects.equals(this.includeOriginalDescription, transactionsGetRequestOptions.includeOriginalDescription) &&
Objects.equals(this.includePersonalFinanceCategoryBeta, transactionsGetRequestOptions.includePersonalFinanceCategoryBeta) &&
Objects.equals(this.includePersonalFinanceCategory, transactionsGetRequestOptions.includePersonalFinanceCategory) &&
Objects.equals(this.includeLogoAndCounterpartyBeta, transactionsGetRequestOptions.includeLogoAndCounterpartyBeta) &&
Objects.equals(this.daysRequested, transactionsGetRequestOptions.daysRequested);
}
@Override
public int hashCode() {
return Objects.hash(accountIds, count, offset, includeOriginalDescription, includePersonalFinanceCategoryBeta, includePersonalFinanceCategory, includeLogoAndCounterpartyBeta, daysRequested);
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class TransactionsGetRequestOptions {\n");
sb.append(" accountIds: ").append(toIndentedString(accountIds)).append("\n");
sb.append(" count: ").append(toIndentedString(count)).append("\n");
sb.append(" offset: ").append(toIndentedString(offset)).append("\n");
sb.append(" includeOriginalDescription: ").append(toIndentedString(includeOriginalDescription)).append("\n");
sb.append(" includePersonalFinanceCategoryBeta: ").append(toIndentedString(includePersonalFinanceCategoryBeta)).append("\n");
sb.append(" includePersonalFinanceCategory: ").append(toIndentedString(includePersonalFinanceCategory)).append("\n");
sb.append(" includeLogoAndCounterpartyBeta: ").append(toIndentedString(includeLogoAndCounterpartyBeta)).append("\n");
sb.append(" daysRequested: ").append(toIndentedString(daysRequested)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces
* (except the first line).
*/
private String toIndentedString(Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy