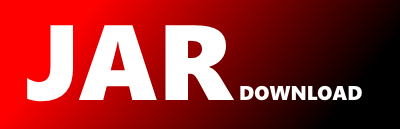
com.plaid.client.model.TransactionsSyncRequest Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of plaid-java Show documentation
Show all versions of plaid-java Show documentation
Bindings for the Plaid (plaid.com) API.
/*
* The Plaid API
* The Plaid REST API. Please see https://plaid.com/docs/api for more details.
*
* The version of the OpenAPI document: 2020-09-14_1.565.0
*
*
* NOTE: This class is auto generated by OpenAPI Generator (https://openapi-generator.tech).
* https://openapi-generator.tech
* Do not edit the class manually.
*/
package com.plaid.client.model;
import java.util.Objects;
import java.util.Arrays;
import com.google.gson.TypeAdapter;
import com.google.gson.annotations.JsonAdapter;
import com.google.gson.annotations.SerializedName;
import com.google.gson.stream.JsonReader;
import com.google.gson.stream.JsonWriter;
import com.plaid.client.model.TransactionsSyncRequestOptions;
import io.swagger.annotations.ApiModel;
import io.swagger.annotations.ApiModelProperty;
import java.io.IOException;
/**
* TransactionsSyncRequest defines the request schema for `/transactions/sync`
*/
@ApiModel(description = "TransactionsSyncRequest defines the request schema for `/transactions/sync`")
@javax.annotation.Generated(value = "org.openapitools.codegen.languages.JavaClientCodegen", date = "2024-09-17T16:42:02.150702Z[Etc/UTC]")
public class TransactionsSyncRequest {
public static final String SERIALIZED_NAME_CLIENT_ID = "client_id";
@SerializedName(SERIALIZED_NAME_CLIENT_ID)
private String clientId;
public static final String SERIALIZED_NAME_ACCESS_TOKEN = "access_token";
@SerializedName(SERIALIZED_NAME_ACCESS_TOKEN)
private String accessToken;
public static final String SERIALIZED_NAME_SECRET = "secret";
@SerializedName(SERIALIZED_NAME_SECRET)
private String secret;
public static final String SERIALIZED_NAME_CURSOR = "cursor";
@SerializedName(SERIALIZED_NAME_CURSOR)
private String cursor;
public static final String SERIALIZED_NAME_COUNT = "count";
@SerializedName(SERIALIZED_NAME_COUNT)
private Integer count = 100;
public static final String SERIALIZED_NAME_OPTIONS = "options";
@SerializedName(SERIALIZED_NAME_OPTIONS)
private TransactionsSyncRequestOptions options;
public TransactionsSyncRequest clientId(String clientId) {
this.clientId = clientId;
return this;
}
/**
* Your Plaid API `client_id`. The `client_id` is required and may be provided either in the `PLAID-CLIENT-ID` header or as part of a request body.
* @return clientId
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Your Plaid API `client_id`. The `client_id` is required and may be provided either in the `PLAID-CLIENT-ID` header or as part of a request body.")
public String getClientId() {
return clientId;
}
public void setClientId(String clientId) {
this.clientId = clientId;
}
public TransactionsSyncRequest accessToken(String accessToken) {
this.accessToken = accessToken;
return this;
}
/**
* The access token associated with the Item data is being requested for.
* @return accessToken
**/
@ApiModelProperty(required = true, value = "The access token associated with the Item data is being requested for.")
public String getAccessToken() {
return accessToken;
}
public void setAccessToken(String accessToken) {
this.accessToken = accessToken;
}
public TransactionsSyncRequest secret(String secret) {
this.secret = secret;
return this;
}
/**
* Your Plaid API `secret`. The `secret` is required and may be provided either in the `PLAID-SECRET` header or as part of a request body.
* @return secret
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Your Plaid API `secret`. The `secret` is required and may be provided either in the `PLAID-SECRET` header or as part of a request body.")
public String getSecret() {
return secret;
}
public void setSecret(String secret) {
this.secret = secret;
}
public TransactionsSyncRequest cursor(String cursor) {
this.cursor = cursor;
return this;
}
/**
* The cursor value represents the last update requested. Providing it will cause the response to only return changes after this update. If omitted, the entire history of updates will be returned, starting with the first-added transactions on the Item. The cursor also accepts the special value of `\"now\"`, which can be used to fast-forward the cursor as part of migrating an existing Item from `/transactions/get` to `/transactions/sync`. For more information, see the [Transactions sync migration guide](https://plaid.com/docs/transactions/sync-migration/). Note that using the `\"now\"` value is not supported for any use case other than migrating existing Items from `/transactions/get`. The upper-bound length of this cursor is 256 characters of base64.
* @return cursor
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "The cursor value represents the last update requested. Providing it will cause the response to only return changes after this update. If omitted, the entire history of updates will be returned, starting with the first-added transactions on the Item. The cursor also accepts the special value of `\"now\"`, which can be used to fast-forward the cursor as part of migrating an existing Item from `/transactions/get` to `/transactions/sync`. For more information, see the [Transactions sync migration guide](https://plaid.com/docs/transactions/sync-migration/). Note that using the `\"now\"` value is not supported for any use case other than migrating existing Items from `/transactions/get`. The upper-bound length of this cursor is 256 characters of base64.")
public String getCursor() {
return cursor;
}
public void setCursor(String cursor) {
this.cursor = cursor;
}
public TransactionsSyncRequest count(Integer count) {
this.count = count;
return this;
}
/**
* The number of transaction updates to fetch.
* minimum: 1
* maximum: 500
* @return count
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "The number of transaction updates to fetch.")
public Integer getCount() {
return count;
}
public void setCount(Integer count) {
this.count = count;
}
public TransactionsSyncRequest options(TransactionsSyncRequestOptions options) {
this.options = options;
return this;
}
/**
* Get options
* @return options
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
public TransactionsSyncRequestOptions getOptions() {
return options;
}
public void setOptions(TransactionsSyncRequestOptions options) {
this.options = options;
}
@Override
public boolean equals(Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
TransactionsSyncRequest transactionsSyncRequest = (TransactionsSyncRequest) o;
return Objects.equals(this.clientId, transactionsSyncRequest.clientId) &&
Objects.equals(this.accessToken, transactionsSyncRequest.accessToken) &&
Objects.equals(this.secret, transactionsSyncRequest.secret) &&
Objects.equals(this.cursor, transactionsSyncRequest.cursor) &&
Objects.equals(this.count, transactionsSyncRequest.count) &&
Objects.equals(this.options, transactionsSyncRequest.options);
}
@Override
public int hashCode() {
return Objects.hash(clientId, accessToken, secret, cursor, count, options);
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class TransactionsSyncRequest {\n");
sb.append(" clientId: ").append(toIndentedString(clientId)).append("\n");
sb.append(" accessToken: ").append(toIndentedString(accessToken)).append("\n");
sb.append(" secret: ").append(toIndentedString(secret)).append("\n");
sb.append(" cursor: ").append(toIndentedString(cursor)).append("\n");
sb.append(" count: ").append(toIndentedString(count)).append("\n");
sb.append(" options: ").append(toIndentedString(options)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces
* (except the first line).
*/
private String toIndentedString(Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy