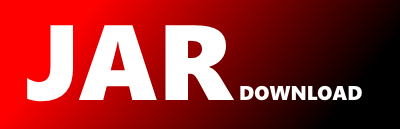
com.plaid.client.model.TransferLedgerDistributeRequest Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of plaid-java Show documentation
Show all versions of plaid-java Show documentation
Bindings for the Plaid (plaid.com) API.
/*
* The Plaid API
* The Plaid REST API. Please see https://plaid.com/docs/api for more details.
*
* The version of the OpenAPI document: 2020-09-14_1.565.0
*
*
* NOTE: This class is auto generated by OpenAPI Generator (https://openapi-generator.tech).
* https://openapi-generator.tech
* Do not edit the class manually.
*/
package com.plaid.client.model;
import java.util.Objects;
import java.util.Arrays;
import com.google.gson.TypeAdapter;
import com.google.gson.annotations.JsonAdapter;
import com.google.gson.annotations.SerializedName;
import com.google.gson.stream.JsonReader;
import com.google.gson.stream.JsonWriter;
import io.swagger.annotations.ApiModel;
import io.swagger.annotations.ApiModelProperty;
import java.io.IOException;
/**
* Defines the request schema for `/transfer/ledger/distribute`
*/
@ApiModel(description = "Defines the request schema for `/transfer/ledger/distribute`")
@javax.annotation.Generated(value = "org.openapitools.codegen.languages.JavaClientCodegen", date = "2024-09-17T16:42:02.150702Z[Etc/UTC]")
public class TransferLedgerDistributeRequest {
public static final String SERIALIZED_NAME_CLIENT_ID = "client_id";
@SerializedName(SERIALIZED_NAME_CLIENT_ID)
private String clientId;
public static final String SERIALIZED_NAME_SECRET = "secret";
@SerializedName(SERIALIZED_NAME_SECRET)
private String secret;
public static final String SERIALIZED_NAME_FROM_LEDGER_ID = "from_ledger_id";
@SerializedName(SERIALIZED_NAME_FROM_LEDGER_ID)
private String fromLedgerId;
public static final String SERIALIZED_NAME_TO_LEDGER_ID = "to_ledger_id";
@SerializedName(SERIALIZED_NAME_TO_LEDGER_ID)
private String toLedgerId;
public static final String SERIALIZED_NAME_AMOUNT = "amount";
@SerializedName(SERIALIZED_NAME_AMOUNT)
private String amount;
public static final String SERIALIZED_NAME_IDEMPOTENCY_KEY = "idempotency_key";
@SerializedName(SERIALIZED_NAME_IDEMPOTENCY_KEY)
private String idempotencyKey;
public static final String SERIALIZED_NAME_DESCRIPTION = "description";
@SerializedName(SERIALIZED_NAME_DESCRIPTION)
private String description;
public TransferLedgerDistributeRequest clientId(String clientId) {
this.clientId = clientId;
return this;
}
/**
* Your Plaid API `client_id`. The `client_id` is required and may be provided either in the `PLAID-CLIENT-ID` header or as part of a request body.
* @return clientId
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Your Plaid API `client_id`. The `client_id` is required and may be provided either in the `PLAID-CLIENT-ID` header or as part of a request body.")
public String getClientId() {
return clientId;
}
public void setClientId(String clientId) {
this.clientId = clientId;
}
public TransferLedgerDistributeRequest secret(String secret) {
this.secret = secret;
return this;
}
/**
* Your Plaid API `secret`. The `secret` is required and may be provided either in the `PLAID-SECRET` header or as part of a request body.
* @return secret
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Your Plaid API `secret`. The `secret` is required and may be provided either in the `PLAID-SECRET` header or as part of a request body.")
public String getSecret() {
return secret;
}
public void setSecret(String secret) {
this.secret = secret;
}
public TransferLedgerDistributeRequest fromLedgerId(String fromLedgerId) {
this.fromLedgerId = fromLedgerId;
return this;
}
/**
* The Ledger to pull money from.
* @return fromLedgerId
**/
@ApiModelProperty(required = true, value = "The Ledger to pull money from.")
public String getFromLedgerId() {
return fromLedgerId;
}
public void setFromLedgerId(String fromLedgerId) {
this.fromLedgerId = fromLedgerId;
}
public TransferLedgerDistributeRequest toLedgerId(String toLedgerId) {
this.toLedgerId = toLedgerId;
return this;
}
/**
* The Ledger to credit money to.
* @return toLedgerId
**/
@ApiModelProperty(required = true, value = "The Ledger to credit money to.")
public String getToLedgerId() {
return toLedgerId;
}
public void setToLedgerId(String toLedgerId) {
this.toLedgerId = toLedgerId;
}
public TransferLedgerDistributeRequest amount(String amount) {
this.amount = amount;
return this;
}
/**
* The amount to move (decimal string with two digits of precision e.g. \"10.00\"). Amount must be positive.
* @return amount
**/
@ApiModelProperty(required = true, value = "The amount to move (decimal string with two digits of precision e.g. \"10.00\"). Amount must be positive.")
public String getAmount() {
return amount;
}
public void setAmount(String amount) {
this.amount = amount;
}
public TransferLedgerDistributeRequest idempotencyKey(String idempotencyKey) {
this.idempotencyKey = idempotencyKey;
return this;
}
/**
* A unique key provided by the client, per unique ledger distribute. Maximum of 50 characters. The API supports idempotency for safely retrying the request without accidentally performing the same operation twice. For example, if a request to create a ledger distribute fails due to a network connection error, you can retry the request with the same idempotency key to guarantee that only a single distribute is created.
* @return idempotencyKey
**/
@ApiModelProperty(required = true, value = "A unique key provided by the client, per unique ledger distribute. Maximum of 50 characters. The API supports idempotency for safely retrying the request without accidentally performing the same operation twice. For example, if a request to create a ledger distribute fails due to a network connection error, you can retry the request with the same idempotency key to guarantee that only a single distribute is created.")
public String getIdempotencyKey() {
return idempotencyKey;
}
public void setIdempotencyKey(String idempotencyKey) {
this.idempotencyKey = idempotencyKey;
}
public TransferLedgerDistributeRequest description(String description) {
this.description = description;
return this;
}
/**
* An optional description for the ledger distribute operation.
* @return description
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "An optional description for the ledger distribute operation.")
public String getDescription() {
return description;
}
public void setDescription(String description) {
this.description = description;
}
@Override
public boolean equals(Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
TransferLedgerDistributeRequest transferLedgerDistributeRequest = (TransferLedgerDistributeRequest) o;
return Objects.equals(this.clientId, transferLedgerDistributeRequest.clientId) &&
Objects.equals(this.secret, transferLedgerDistributeRequest.secret) &&
Objects.equals(this.fromLedgerId, transferLedgerDistributeRequest.fromLedgerId) &&
Objects.equals(this.toLedgerId, transferLedgerDistributeRequest.toLedgerId) &&
Objects.equals(this.amount, transferLedgerDistributeRequest.amount) &&
Objects.equals(this.idempotencyKey, transferLedgerDistributeRequest.idempotencyKey) &&
Objects.equals(this.description, transferLedgerDistributeRequest.description);
}
@Override
public int hashCode() {
return Objects.hash(clientId, secret, fromLedgerId, toLedgerId, amount, idempotencyKey, description);
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class TransferLedgerDistributeRequest {\n");
sb.append(" clientId: ").append(toIndentedString(clientId)).append("\n");
sb.append(" secret: ").append(toIndentedString(secret)).append("\n");
sb.append(" fromLedgerId: ").append(toIndentedString(fromLedgerId)).append("\n");
sb.append(" toLedgerId: ").append(toIndentedString(toLedgerId)).append("\n");
sb.append(" amount: ").append(toIndentedString(amount)).append("\n");
sb.append(" idempotencyKey: ").append(toIndentedString(idempotencyKey)).append("\n");
sb.append(" description: ").append(toIndentedString(description)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces
* (except the first line).
*/
private String toIndentedString(Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy