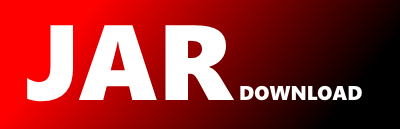
com.plaid.client.model.WalletTransactionExecuteRequest Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of plaid-java Show documentation
Show all versions of plaid-java Show documentation
Bindings for the Plaid (plaid.com) API.
/*
* The Plaid API
* The Plaid REST API. Please see https://plaid.com/docs/api for more details.
*
* The version of the OpenAPI document: 2020-09-14_1.565.0
*
*
* NOTE: This class is auto generated by OpenAPI Generator (https://openapi-generator.tech).
* https://openapi-generator.tech
* Do not edit the class manually.
*/
package com.plaid.client.model;
import java.util.Objects;
import java.util.Arrays;
import com.google.gson.TypeAdapter;
import com.google.gson.annotations.JsonAdapter;
import com.google.gson.annotations.SerializedName;
import com.google.gson.stream.JsonReader;
import com.google.gson.stream.JsonWriter;
import com.plaid.client.model.WalletTransactionAmount;
import com.plaid.client.model.WalletTransactionCounterparty;
import io.swagger.annotations.ApiModel;
import io.swagger.annotations.ApiModelProperty;
import java.io.IOException;
/**
* WalletTransactionExecuteRequest defines the request schema for `/wallet/transaction/execute`
*/
@ApiModel(description = "WalletTransactionExecuteRequest defines the request schema for `/wallet/transaction/execute`")
@javax.annotation.Generated(value = "org.openapitools.codegen.languages.JavaClientCodegen", date = "2024-09-17T16:42:02.150702Z[Etc/UTC]")
public class WalletTransactionExecuteRequest {
public static final String SERIALIZED_NAME_CLIENT_ID = "client_id";
@SerializedName(SERIALIZED_NAME_CLIENT_ID)
private String clientId;
public static final String SERIALIZED_NAME_SECRET = "secret";
@SerializedName(SERIALIZED_NAME_SECRET)
private String secret;
public static final String SERIALIZED_NAME_IDEMPOTENCY_KEY = "idempotency_key";
@SerializedName(SERIALIZED_NAME_IDEMPOTENCY_KEY)
private String idempotencyKey;
public static final String SERIALIZED_NAME_WALLET_ID = "wallet_id";
@SerializedName(SERIALIZED_NAME_WALLET_ID)
private String walletId;
public static final String SERIALIZED_NAME_COUNTERPARTY = "counterparty";
@SerializedName(SERIALIZED_NAME_COUNTERPARTY)
private WalletTransactionCounterparty counterparty;
public static final String SERIALIZED_NAME_AMOUNT = "amount";
@SerializedName(SERIALIZED_NAME_AMOUNT)
private WalletTransactionAmount amount;
public static final String SERIALIZED_NAME_REFERENCE = "reference";
@SerializedName(SERIALIZED_NAME_REFERENCE)
private String reference;
public WalletTransactionExecuteRequest clientId(String clientId) {
this.clientId = clientId;
return this;
}
/**
* Your Plaid API `client_id`. The `client_id` is required and may be provided either in the `PLAID-CLIENT-ID` header or as part of a request body.
* @return clientId
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Your Plaid API `client_id`. The `client_id` is required and may be provided either in the `PLAID-CLIENT-ID` header or as part of a request body.")
public String getClientId() {
return clientId;
}
public void setClientId(String clientId) {
this.clientId = clientId;
}
public WalletTransactionExecuteRequest secret(String secret) {
this.secret = secret;
return this;
}
/**
* Your Plaid API `secret`. The `secret` is required and may be provided either in the `PLAID-SECRET` header or as part of a request body.
* @return secret
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Your Plaid API `secret`. The `secret` is required and may be provided either in the `PLAID-SECRET` header or as part of a request body.")
public String getSecret() {
return secret;
}
public void setSecret(String secret) {
this.secret = secret;
}
public WalletTransactionExecuteRequest idempotencyKey(String idempotencyKey) {
this.idempotencyKey = idempotencyKey;
return this;
}
/**
* A random key provided by the client, per unique wallet transaction. Maximum of 128 characters. The API supports idempotency for safely retrying requests without accidentally performing the same operation twice. If a request to execute a wallet transaction fails due to a network connection error, then after a minimum delay of one minute, you can retry the request with the same idempotency key to guarantee that only a single wallet transaction is created. If the request was successfully processed, it will prevent any transaction that uses the same idempotency key, and was received within 24 hours of the first request, from being processed.
* @return idempotencyKey
**/
@ApiModelProperty(required = true, value = "A random key provided by the client, per unique wallet transaction. Maximum of 128 characters. The API supports idempotency for safely retrying requests without accidentally performing the same operation twice. If a request to execute a wallet transaction fails due to a network connection error, then after a minimum delay of one minute, you can retry the request with the same idempotency key to guarantee that only a single wallet transaction is created. If the request was successfully processed, it will prevent any transaction that uses the same idempotency key, and was received within 24 hours of the first request, from being processed.")
public String getIdempotencyKey() {
return idempotencyKey;
}
public void setIdempotencyKey(String idempotencyKey) {
this.idempotencyKey = idempotencyKey;
}
public WalletTransactionExecuteRequest walletId(String walletId) {
this.walletId = walletId;
return this;
}
/**
* The ID of the e-wallet to debit from
* @return walletId
**/
@ApiModelProperty(required = true, value = "The ID of the e-wallet to debit from")
public String getWalletId() {
return walletId;
}
public void setWalletId(String walletId) {
this.walletId = walletId;
}
public WalletTransactionExecuteRequest counterparty(WalletTransactionCounterparty counterparty) {
this.counterparty = counterparty;
return this;
}
/**
* Get counterparty
* @return counterparty
**/
@ApiModelProperty(required = true, value = "")
public WalletTransactionCounterparty getCounterparty() {
return counterparty;
}
public void setCounterparty(WalletTransactionCounterparty counterparty) {
this.counterparty = counterparty;
}
public WalletTransactionExecuteRequest amount(WalletTransactionAmount amount) {
this.amount = amount;
return this;
}
/**
* Get amount
* @return amount
**/
@ApiModelProperty(required = true, value = "")
public WalletTransactionAmount getAmount() {
return amount;
}
public void setAmount(WalletTransactionAmount amount) {
this.amount = amount;
}
public WalletTransactionExecuteRequest reference(String reference) {
this.reference = reference;
return this;
}
/**
* A reference for the transaction. This must be an alphanumeric string with 6 to 18 characters and must not contain any special characters or spaces. Ensure that the `reference` field is unique for each transaction.
* @return reference
**/
@ApiModelProperty(required = true, value = "A reference for the transaction. This must be an alphanumeric string with 6 to 18 characters and must not contain any special characters or spaces. Ensure that the `reference` field is unique for each transaction.")
public String getReference() {
return reference;
}
public void setReference(String reference) {
this.reference = reference;
}
@Override
public boolean equals(Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
WalletTransactionExecuteRequest walletTransactionExecuteRequest = (WalletTransactionExecuteRequest) o;
return Objects.equals(this.clientId, walletTransactionExecuteRequest.clientId) &&
Objects.equals(this.secret, walletTransactionExecuteRequest.secret) &&
Objects.equals(this.idempotencyKey, walletTransactionExecuteRequest.idempotencyKey) &&
Objects.equals(this.walletId, walletTransactionExecuteRequest.walletId) &&
Objects.equals(this.counterparty, walletTransactionExecuteRequest.counterparty) &&
Objects.equals(this.amount, walletTransactionExecuteRequest.amount) &&
Objects.equals(this.reference, walletTransactionExecuteRequest.reference);
}
@Override
public int hashCode() {
return Objects.hash(clientId, secret, idempotencyKey, walletId, counterparty, amount, reference);
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class WalletTransactionExecuteRequest {\n");
sb.append(" clientId: ").append(toIndentedString(clientId)).append("\n");
sb.append(" secret: ").append(toIndentedString(secret)).append("\n");
sb.append(" idempotencyKey: ").append(toIndentedString(idempotencyKey)).append("\n");
sb.append(" walletId: ").append(toIndentedString(walletId)).append("\n");
sb.append(" counterparty: ").append(toIndentedString(counterparty)).append("\n");
sb.append(" amount: ").append(toIndentedString(amount)).append("\n");
sb.append(" reference: ").append(toIndentedString(reference)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces
* (except the first line).
*/
private String toIndentedString(Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy