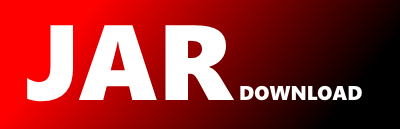
com.plaid.client.model.ConsentEvent Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of plaid-java Show documentation
Show all versions of plaid-java Show documentation
Bindings for the Plaid (plaid.com) API.
/*
* The Plaid API
* The Plaid REST API. Please see https://plaid.com/docs/api for more details.
*
* The version of the OpenAPI document: 2020-09-14_1.575.0
*
*
* NOTE: This class is auto generated by OpenAPI Generator (https://openapi-generator.tech).
* https://openapi-generator.tech
* Do not edit the class manually.
*/
package com.plaid.client.model;
import java.util.Objects;
import java.util.Arrays;
import com.google.gson.TypeAdapter;
import com.google.gson.annotations.JsonAdapter;
import com.google.gson.annotations.SerializedName;
import com.google.gson.stream.JsonReader;
import com.google.gson.stream.JsonWriter;
import com.plaid.client.model.ConsentEventCode;
import com.plaid.client.model.ConsentEventInitiator;
import com.plaid.client.model.ConsentEventType;
import com.plaid.client.model.ConsentedAccount;
import io.swagger.annotations.ApiModel;
import io.swagger.annotations.ApiModelProperty;
import java.io.IOException;
import java.time.OffsetDateTime;
import java.util.ArrayList;
import java.util.List;
/**
* Describes a consent event.
*/
@ApiModel(description = "Describes a consent event.")
@javax.annotation.Generated(value = "org.openapitools.codegen.languages.JavaClientCodegen", date = "2024-10-09T17:51:17.896278Z[Etc/UTC]")
public class ConsentEvent {
public static final String SERIALIZED_NAME_ITEM_ID = "item_id";
@SerializedName(SERIALIZED_NAME_ITEM_ID)
private String itemId;
public static final String SERIALIZED_NAME_CREATED_AT = "created_at";
@SerializedName(SERIALIZED_NAME_CREATED_AT)
private OffsetDateTime createdAt;
public static final String SERIALIZED_NAME_EVENT_TYPE = "event_type";
@SerializedName(SERIALIZED_NAME_EVENT_TYPE)
private ConsentEventType eventType;
public static final String SERIALIZED_NAME_EVENT_CODE = "event_code";
@SerializedName(SERIALIZED_NAME_EVENT_CODE)
private ConsentEventCode eventCode;
public static final String SERIALIZED_NAME_INSTITUTION_ID = "institution_id";
@SerializedName(SERIALIZED_NAME_INSTITUTION_ID)
private String institutionId;
public static final String SERIALIZED_NAME_INSTITUTION_NAME = "institution_name";
@SerializedName(SERIALIZED_NAME_INSTITUTION_NAME)
private String institutionName;
public static final String SERIALIZED_NAME_INITIATOR = "initiator";
@SerializedName(SERIALIZED_NAME_INITIATOR)
private ConsentEventInitiator initiator;
public static final String SERIALIZED_NAME_CONSENTED_USE_CASES = "consented_use_cases";
@SerializedName(SERIALIZED_NAME_CONSENTED_USE_CASES)
private List consentedUseCases = null;
public static final String SERIALIZED_NAME_CONSENTED_DATA_SCOPES = "consented_data_scopes";
@SerializedName(SERIALIZED_NAME_CONSENTED_DATA_SCOPES)
private List consentedDataScopes = null;
public static final String SERIALIZED_NAME_CONSENTED_ACCOUNTS = "consented_accounts";
@SerializedName(SERIALIZED_NAME_CONSENTED_ACCOUNTS)
private List consentedAccounts = null;
public ConsentEvent itemId(String itemId) {
this.itemId = itemId;
return this;
}
/**
* The Plaid Item ID. The `item_id` is always unique; linking the same account at the same institution twice will result in two Items with different `item_id` values. Like all Plaid identifiers, the `item_id` is case-sensitive.
* @return itemId
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "The Plaid Item ID. The `item_id` is always unique; linking the same account at the same institution twice will result in two Items with different `item_id` values. Like all Plaid identifiers, the `item_id` is case-sensitive.")
public String getItemId() {
return itemId;
}
public void setItemId(String itemId) {
this.itemId = itemId;
}
public ConsentEvent createdAt(OffsetDateTime createdAt) {
this.createdAt = createdAt;
return this;
}
/**
* The date and time when the consent event occurred, in [ISO 8601](https://en.wikipedia.org/wiki/ISO_8601) format.
* @return createdAt
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "The date and time when the consent event occurred, in [ISO 8601](https://en.wikipedia.org/wiki/ISO_8601) format.")
public OffsetDateTime getCreatedAt() {
return createdAt;
}
public void setCreatedAt(OffsetDateTime createdAt) {
this.createdAt = createdAt;
}
public ConsentEvent eventType(ConsentEventType eventType) {
this.eventType = eventType;
return this;
}
/**
* Get eventType
* @return eventType
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
public ConsentEventType getEventType() {
return eventType;
}
public void setEventType(ConsentEventType eventType) {
this.eventType = eventType;
}
public ConsentEvent eventCode(ConsentEventCode eventCode) {
this.eventCode = eventCode;
return this;
}
/**
* Get eventCode
* @return eventCode
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
public ConsentEventCode getEventCode() {
return eventCode;
}
public void setEventCode(ConsentEventCode eventCode) {
this.eventCode = eventCode;
}
public ConsentEvent institutionId(String institutionId) {
this.institutionId = institutionId;
return this;
}
/**
* Unique identifier for the institution associated with the Item. Field is `null` for Items created via Same Day Micro-deposits.
* @return institutionId
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Unique identifier for the institution associated with the Item. Field is `null` for Items created via Same Day Micro-deposits.")
public String getInstitutionId() {
return institutionId;
}
public void setInstitutionId(String institutionId) {
this.institutionId = institutionId;
}
public ConsentEvent institutionName(String institutionName) {
this.institutionName = institutionName;
return this;
}
/**
* The full name of the institution associated with the Item. Field is `null` for Items created via Same Day Micro-deposits.
* @return institutionName
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "The full name of the institution associated with the Item. Field is `null` for Items created via Same Day Micro-deposits.")
public String getInstitutionName() {
return institutionName;
}
public void setInstitutionName(String institutionName) {
this.institutionName = institutionName;
}
public ConsentEvent initiator(ConsentEventInitiator initiator) {
this.initiator = initiator;
return this;
}
/**
* Get initiator
* @return initiator
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
public ConsentEventInitiator getInitiator() {
return initiator;
}
public void setInitiator(ConsentEventInitiator initiator) {
this.initiator = initiator;
}
public ConsentEvent consentedUseCases(List consentedUseCases) {
this.consentedUseCases = consentedUseCases;
return this;
}
public ConsentEvent addConsentedUseCasesItem(String consentedUseCasesItem) {
if (this.consentedUseCases == null) {
this.consentedUseCases = new ArrayList<>();
}
this.consentedUseCases.add(consentedUseCasesItem);
return this;
}
/**
* A list of strings containing the full list of use cases the end user has consented to for the Item. See the [full list](/docs/link/data-transparency-messaging-migration-guide/#updating-link-customizations) of use cases.
* @return consentedUseCases
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "A list of strings containing the full list of use cases the end user has consented to for the Item. See the [full list](/docs/link/data-transparency-messaging-migration-guide/#updating-link-customizations) of use cases.")
public List getConsentedUseCases() {
return consentedUseCases;
}
public void setConsentedUseCases(List consentedUseCases) {
this.consentedUseCases = consentedUseCases;
}
public ConsentEvent consentedDataScopes(List consentedDataScopes) {
this.consentedDataScopes = consentedDataScopes;
return this;
}
public ConsentEvent addConsentedDataScopesItem(String consentedDataScopesItem) {
if (this.consentedDataScopes == null) {
this.consentedDataScopes = new ArrayList<>();
}
this.consentedDataScopes.add(consentedDataScopesItem);
return this;
}
/**
* A list of strings containing the full list of data scopes the end user has consented to for the Item. These correspond to consented products; see the [full mapping](/docs/link/data-transparency-messaging-migration-guide/#data-scopes-by-product) of data scopes and products.
* @return consentedDataScopes
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "A list of strings containing the full list of data scopes the end user has consented to for the Item. These correspond to consented products; see the [full mapping](/docs/link/data-transparency-messaging-migration-guide/#data-scopes-by-product) of data scopes and products.")
public List getConsentedDataScopes() {
return consentedDataScopes;
}
public void setConsentedDataScopes(List consentedDataScopes) {
this.consentedDataScopes = consentedDataScopes;
}
public ConsentEvent consentedAccounts(List consentedAccounts) {
this.consentedAccounts = consentedAccounts;
return this;
}
public ConsentEvent addConsentedAccountsItem(ConsentedAccount consentedAccountsItem) {
if (this.consentedAccounts == null) {
this.consentedAccounts = new ArrayList<>();
}
this.consentedAccounts.add(consentedAccountsItem);
return this;
}
/**
* An array containing the accounts associated with the Item for which authorizations are granted.
* @return consentedAccounts
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "An array containing the accounts associated with the Item for which authorizations are granted.")
public List getConsentedAccounts() {
return consentedAccounts;
}
public void setConsentedAccounts(List consentedAccounts) {
this.consentedAccounts = consentedAccounts;
}
@Override
public boolean equals(Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
ConsentEvent consentEvent = (ConsentEvent) o;
return Objects.equals(this.itemId, consentEvent.itemId) &&
Objects.equals(this.createdAt, consentEvent.createdAt) &&
Objects.equals(this.eventType, consentEvent.eventType) &&
Objects.equals(this.eventCode, consentEvent.eventCode) &&
Objects.equals(this.institutionId, consentEvent.institutionId) &&
Objects.equals(this.institutionName, consentEvent.institutionName) &&
Objects.equals(this.initiator, consentEvent.initiator) &&
Objects.equals(this.consentedUseCases, consentEvent.consentedUseCases) &&
Objects.equals(this.consentedDataScopes, consentEvent.consentedDataScopes) &&
Objects.equals(this.consentedAccounts, consentEvent.consentedAccounts);
}
@Override
public int hashCode() {
return Objects.hash(itemId, createdAt, eventType, eventCode, institutionId, institutionName, initiator, consentedUseCases, consentedDataScopes, consentedAccounts);
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class ConsentEvent {\n");
sb.append(" itemId: ").append(toIndentedString(itemId)).append("\n");
sb.append(" createdAt: ").append(toIndentedString(createdAt)).append("\n");
sb.append(" eventType: ").append(toIndentedString(eventType)).append("\n");
sb.append(" eventCode: ").append(toIndentedString(eventCode)).append("\n");
sb.append(" institutionId: ").append(toIndentedString(institutionId)).append("\n");
sb.append(" institutionName: ").append(toIndentedString(institutionName)).append("\n");
sb.append(" initiator: ").append(toIndentedString(initiator)).append("\n");
sb.append(" consentedUseCases: ").append(toIndentedString(consentedUseCases)).append("\n");
sb.append(" consentedDataScopes: ").append(toIndentedString(consentedDataScopes)).append("\n");
sb.append(" consentedAccounts: ").append(toIndentedString(consentedAccounts)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces
* (except the first line).
*/
private String toIndentedString(Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy