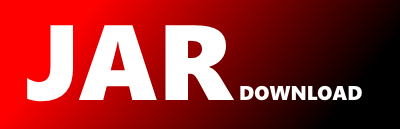
com.plaid.client.model.ConsumerReportUserIdentity Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of plaid-java Show documentation
Show all versions of plaid-java Show documentation
Bindings for the Plaid (plaid.com) API.
/*
* The Plaid API
* The Plaid REST API. Please see https://plaid.com/docs/api for more details.
*
* The version of the OpenAPI document: 2020-09-14_1.575.0
*
*
* NOTE: This class is auto generated by OpenAPI Generator (https://openapi-generator.tech).
* https://openapi-generator.tech
* Do not edit the class manually.
*/
package com.plaid.client.model;
import java.util.Objects;
import java.util.Arrays;
import com.google.gson.TypeAdapter;
import com.google.gson.annotations.JsonAdapter;
import com.google.gson.annotations.SerializedName;
import com.google.gson.stream.JsonReader;
import com.google.gson.stream.JsonWriter;
import com.plaid.client.model.AddressData;
import io.swagger.annotations.ApiModel;
import io.swagger.annotations.ApiModelProperty;
import java.io.IOException;
import java.time.LocalDate;
import java.util.ArrayList;
import java.util.List;
/**
* To create a Plaid Check Consumer Report for a user, this field must be present on the user token. If this field is not provided during user token creation, you can add it to the user later by calling `/user/update`. Once the field has been added to the user, you will be able to call `/link/token/create` with a non-empty `consumer_report_permissible_purpose` (which will automatically create a Plaid Check Consumer Report), or call `/cra/check_report/create` for that user.
*/
@ApiModel(description = "To create a Plaid Check Consumer Report for a user, this field must be present on the user token. If this field is not provided during user token creation, you can add it to the user later by calling `/user/update`. Once the field has been added to the user, you will be able to call `/link/token/create` with a non-empty `consumer_report_permissible_purpose` (which will automatically create a Plaid Check Consumer Report), or call `/cra/check_report/create` for that user.")
@javax.annotation.Generated(value = "org.openapitools.codegen.languages.JavaClientCodegen", date = "2024-10-09T17:51:17.896278Z[Etc/UTC]")
public class ConsumerReportUserIdentity {
public static final String SERIALIZED_NAME_FIRST_NAME = "first_name";
@SerializedName(SERIALIZED_NAME_FIRST_NAME)
private String firstName;
public static final String SERIALIZED_NAME_LAST_NAME = "last_name";
@SerializedName(SERIALIZED_NAME_LAST_NAME)
private String lastName;
public static final String SERIALIZED_NAME_PHONE_NUMBERS = "phone_numbers";
@SerializedName(SERIALIZED_NAME_PHONE_NUMBERS)
private List phoneNumbers = new ArrayList<>();
public static final String SERIALIZED_NAME_EMAILS = "emails";
@SerializedName(SERIALIZED_NAME_EMAILS)
private List emails = new ArrayList<>();
public static final String SERIALIZED_NAME_SSN_LAST4 = "ssn_last_4";
@SerializedName(SERIALIZED_NAME_SSN_LAST4)
private String ssnLast4;
public static final String SERIALIZED_NAME_DATE_OF_BIRTH = "date_of_birth";
@SerializedName(SERIALIZED_NAME_DATE_OF_BIRTH)
private LocalDate dateOfBirth;
public static final String SERIALIZED_NAME_PRIMARY_ADDRESS = "primary_address";
@SerializedName(SERIALIZED_NAME_PRIMARY_ADDRESS)
private AddressData primaryAddress;
public ConsumerReportUserIdentity firstName(String firstName) {
this.firstName = firstName;
return this;
}
/**
* The user's first name
* @return firstName
**/
@ApiModelProperty(required = true, value = "The user's first name")
public String getFirstName() {
return firstName;
}
public void setFirstName(String firstName) {
this.firstName = firstName;
}
public ConsumerReportUserIdentity lastName(String lastName) {
this.lastName = lastName;
return this;
}
/**
* The user's last name
* @return lastName
**/
@ApiModelProperty(required = true, value = "The user's last name")
public String getLastName() {
return lastName;
}
public void setLastName(String lastName) {
this.lastName = lastName;
}
public ConsumerReportUserIdentity phoneNumbers(List phoneNumbers) {
this.phoneNumbers = phoneNumbers;
return this;
}
public ConsumerReportUserIdentity addPhoneNumbersItem(String phoneNumbersItem) {
this.phoneNumbers.add(phoneNumbersItem);
return this;
}
/**
* The user's phone number, in E.164 format: +{countrycode}{number}. For example: \"+14157452130\". Phone numbers provided in other formats will be parsed on a best-effort basis. Phone number input is validated against valid number ranges; number strings that do not match a real-world phone numbering scheme may cause the request to fail, even in the Sandbox test environment.
* @return phoneNumbers
**/
@ApiModelProperty(required = true, value = "The user's phone number, in E.164 format: +{countrycode}{number}. For example: \"+14157452130\". Phone numbers provided in other formats will be parsed on a best-effort basis. Phone number input is validated against valid number ranges; number strings that do not match a real-world phone numbering scheme may cause the request to fail, even in the Sandbox test environment.")
public List getPhoneNumbers() {
return phoneNumbers;
}
public void setPhoneNumbers(List phoneNumbers) {
this.phoneNumbers = phoneNumbers;
}
public ConsumerReportUserIdentity emails(List emails) {
this.emails = emails;
return this;
}
public ConsumerReportUserIdentity addEmailsItem(String emailsItem) {
this.emails.add(emailsItem);
return this;
}
/**
* The user's emails
* @return emails
**/
@ApiModelProperty(required = true, value = "The user's emails")
public List getEmails() {
return emails;
}
public void setEmails(List emails) {
this.emails = emails;
}
public ConsumerReportUserIdentity ssnLast4(String ssnLast4) {
this.ssnLast4 = ssnLast4;
return this;
}
/**
* The last 4 digits of the user's social security number.
* @return ssnLast4
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "The last 4 digits of the user's social security number.")
public String getSsnLast4() {
return ssnLast4;
}
public void setSsnLast4(String ssnLast4) {
this.ssnLast4 = ssnLast4;
}
public ConsumerReportUserIdentity dateOfBirth(LocalDate dateOfBirth) {
this.dateOfBirth = dateOfBirth;
return this;
}
/**
* To be provided in the format \"yyyy-mm-dd\". This field is required as of Oct 21, 2024 for any clients who became Plaid Check customers on or after Oct 1, 2024. This field will be required for all Plaid Check customers as of Feb 1, 2025.
* @return dateOfBirth
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "To be provided in the format \"yyyy-mm-dd\". This field is required as of Oct 21, 2024 for any clients who became Plaid Check customers on or after Oct 1, 2024. This field will be required for all Plaid Check customers as of Feb 1, 2025.")
public LocalDate getDateOfBirth() {
return dateOfBirth;
}
public void setDateOfBirth(LocalDate dateOfBirth) {
this.dateOfBirth = dateOfBirth;
}
public ConsumerReportUserIdentity primaryAddress(AddressData primaryAddress) {
this.primaryAddress = primaryAddress;
return this;
}
/**
* Get primaryAddress
* @return primaryAddress
**/
@ApiModelProperty(required = true, value = "")
public AddressData getPrimaryAddress() {
return primaryAddress;
}
public void setPrimaryAddress(AddressData primaryAddress) {
this.primaryAddress = primaryAddress;
}
@Override
public boolean equals(Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
ConsumerReportUserIdentity consumerReportUserIdentity = (ConsumerReportUserIdentity) o;
return Objects.equals(this.firstName, consumerReportUserIdentity.firstName) &&
Objects.equals(this.lastName, consumerReportUserIdentity.lastName) &&
Objects.equals(this.phoneNumbers, consumerReportUserIdentity.phoneNumbers) &&
Objects.equals(this.emails, consumerReportUserIdentity.emails) &&
Objects.equals(this.ssnLast4, consumerReportUserIdentity.ssnLast4) &&
Objects.equals(this.dateOfBirth, consumerReportUserIdentity.dateOfBirth) &&
Objects.equals(this.primaryAddress, consumerReportUserIdentity.primaryAddress);
}
@Override
public int hashCode() {
return Objects.hash(firstName, lastName, phoneNumbers, emails, ssnLast4, dateOfBirth, primaryAddress);
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class ConsumerReportUserIdentity {\n");
sb.append(" firstName: ").append(toIndentedString(firstName)).append("\n");
sb.append(" lastName: ").append(toIndentedString(lastName)).append("\n");
sb.append(" phoneNumbers: ").append(toIndentedString(phoneNumbers)).append("\n");
sb.append(" emails: ").append(toIndentedString(emails)).append("\n");
sb.append(" ssnLast4: ").append(toIndentedString(ssnLast4)).append("\n");
sb.append(" dateOfBirth: ").append(toIndentedString(dateOfBirth)).append("\n");
sb.append(" primaryAddress: ").append(toIndentedString(primaryAddress)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces
* (except the first line).
*/
private String toIndentedString(Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy