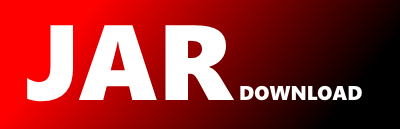
com.podio.contact.ProfileField Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of api Show documentation
Show all versions of api Show documentation
The official Java wrapper for the Podio API
package com.podio.contact;
import java.util.HashMap;
import java.util.Map;
import org.codehaus.jackson.annotate.JsonCreator;
import org.codehaus.jackson.annotate.JsonValue;
import org.joda.time.LocalDate;
import com.podio.serialize.DateTimeUtil;
public abstract class ProfileField {
private static final Map> MAP = new HashMap>();
/**
* The full name
*/
public static final ProfileField NAME = new StringProfileField(
"name", true);
/**
* The file id of the avatar
*/
public static final ProfileField AVATAR = new IntegerProfileField(
"avatar", true);
/**
* The birthdate
*/
public static final ProfileField BIRTHDATE = new DateProfileField(
"birthdate", true);
/**
* The organization or company the person is associated with
*/
public static final ProfileField ORGANIZATION = new StringProfileField(
"organization", true);
/**
* The username for Skype
*/
public static final ProfileField SKYPE = new StringProfileField(
"skype", true);
/**
* Short text about the person
*/
public static final ProfileField ABOUT = new StringProfileField(
"about", true);
/**
* Email address
*/
public static final ProfileField MAIL = new StringProfileField(
"mail", false);
/**
* The address where the person lives or work
*/
public static final ProfileField ADDRESS = new StringProfileField(
"address", false);
/**
* Any instant messaging address
*/
public static final ProfileField IM = new StringProfileField(
"im", false);
/**
* The location of the person
*/
public static final ProfileField LOCATION = new StringProfileField(
"location", false);
/**
* The phone number
*/
public static final ProfileField PHONE = new StringProfileField(
"phone", false);
/**
* The persons title, usually the work title
*/
public static final ProfileField TITLE = new StringProfileField(
"title", false);
/**
* An URL to the persons homepage or the homepage of the company
*/
public static final ProfileField URL = new StringProfileField(
"url", false);
private final String name;
private final boolean single;
public ProfileField(String name, boolean single) {
super();
this.name = name;
this.single = single;
MAP.put(name, this);
}
@JsonValue
public String getName() {
return name;
}
public boolean isSingle() {
return single;
}
@Override
public String toString() {
return getName();
}
public abstract F parse(R object);
public abstract R format(F object);
private static class StringProfileField extends
ProfileField {
public StringProfileField(String name, boolean single) {
super(name, single);
}
@Override
public String parse(String object) {
return object;
}
@Override
public String format(String object) {
return object;
}
}
private static class IntegerProfileField extends
ProfileField {
public IntegerProfileField(String name, boolean single) {
super(name, single);
}
@Override
public Integer parse(Integer object) {
return object;
}
@Override
public Integer format(Integer object) {
return object;
}
}
private static class DateProfileField extends
ProfileField {
public DateProfileField(String name, boolean single) {
super(name, single);
}
@Override
public LocalDate parse(String object) {
return DateTimeUtil.parseDate(object);
}
@Override
public String format(LocalDate object) {
return DateTimeUtil.formatDate(object);
}
}
@JsonCreator
public static ProfileField, ?> getByName(String value) {
return MAP.get(value);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy