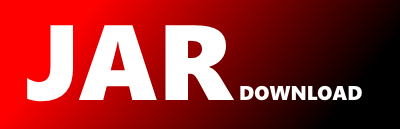
com.podio.item.ItemUpdate Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of api Show documentation
Show all versions of api Show documentation
The official Java wrapper for the Podio API
package com.podio.item;
import java.util.List;
import org.codehaus.jackson.annotate.JsonProperty;
public class ItemUpdate {
/**
* The external id of the item. This can be used to hold a reference to the
* item in an external system.
*/
private String externalId;
/**
* The internal revision from podio.
*/
private int revision;
/**
* The values for each field
*/
private List fields;
public ItemUpdate() {
super();
}
public ItemUpdate(String externalId, List fields) {
super();
this.externalId = externalId;
this.fields = fields;
}
@JsonProperty("external_id")
public String getExternalId() {
return externalId;
}
@JsonProperty("external_id")
public void setExternalId(String externalId) {
this.externalId = externalId;
}
@JsonProperty("revision")
public int getRevision() {
return revision;
}
@JsonProperty("revision")
public void setRevision(int revision) {
this.revision = revision;
}
public List getFields() {
return fields;
}
public void setFields(List fields) {
this.fields = fields;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy