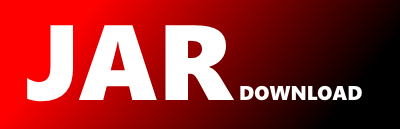
org.monte.media.anim.AmigaDisplayInfo Maven / Gradle / Ivy
The newest version!
package org.monte.media.anim;
import java.awt.Dimension;
import java.util.Collections;
import java.util.HashMap;
import java.util.Map;
import java.util.TreeMap;
public class AmigaDisplayInfo {
public final int camg;
public final String name;
public final int textOverscanWidth,textOverscanHeight;
public final int maxOverscanWidth,maxOverscanHeight;
public final int minimalSizeWidth,minimalSizeHeight;
public final int maximalSizeWidth,maximalSizeHeight;
public final int colorRegisterDepth;
public final int resolutionX,resolutionY;
public final int pixelSpeed;
public final int fps;
private static TreeMap infos;
public AmigaDisplayInfo(int camg, String name, Dimension textOverscan, Dimension maxOverscan, Dimension minimalSize, Dimension maximalSize, int colorRegisterDepth, Dimension resolution, int pixelSpeed, int fps) {
this.camg = camg;
this.name = name;
this.textOverscanWidth = textOverscan.width;
this.textOverscanHeight = textOverscan.height;
this.maxOverscanWidth = maxOverscan.width;
this.maxOverscanHeight = maxOverscan.height;
this.minimalSizeWidth = minimalSize.width;
this.minimalSizeHeight = minimalSize.height;
this.maximalSizeWidth = maximalSize.width;
this.maximalSizeHeight = maximalSize.height;
this.colorRegisterDepth = colorRegisterDepth;
this.resolutionX = resolution.width;
this.resolutionY = resolution.height;
this.pixelSpeed = pixelSpeed;
this.fps = fps;
}
public boolean isOCS() {
return colorRegisterDepth==4;
}
public boolean isHAM() {
return (camg&COLORMODE_MASK)==HAM_COLORMODE;
}
public boolean isEHB() {
return (camg&COLORMODE_MASK)==EHB_COLORMODE;
}
public boolean isInterlace() {
boolean isInterlace;
switch (camg & MONITOR_ID_MASK) {
case NTSC_MONITOR_ID:
case PAL_MONITOR_ID:
isInterlace=(camg&PALNTSC_INTERLACE_MASK)==PALNTSC_INTERLACE_MODE;
break;
case MULTISCAN_MONITOR_ID:
isInterlace=(camg&MULTISCAN_INTERLACE_MASK)==MULTISCAN_INTERLACE_MODE;
break;
default:
isInterlace=false;
break;
}
return isInterlace;
}
public static Map getAllInfos() {
if (infos == null) {
infos = new TreeMap();
for (Object[] e : infoTable) {
int i = 0;
int camg = (Integer) e[i++];
String name = (String) e[i++];
Dimension textOverscan = new Dimension((Integer) e[i++], (Integer) e[i++]);
Dimension maxOverscan = new Dimension((Integer) e[i++], (Integer) e[i++]);
Dimension minimalSize = new Dimension((Integer) e[i++], (Integer) e[i++]);
Dimension maximalSize = new Dimension((Integer) e[i++], (Integer) e[i++]);
int colorRegisterDepth = (Integer) e[i++];
Dimension resolution = new Dimension((Integer) e[i++], (Integer) e[i++]);
int pixelSpeed = (Integer) e[i++];
Integer fps = monitorToFPSMap.get(camg & MONITOR_ID_MASK).fps;
if (fps == null) {
System.out.println("NO FPS 0x" + Integer.toHexString(camg) + " " + name);
}
infos.put(camg, new AmigaDisplayInfo(camg, name, textOverscan, maxOverscan, minimalSize, maximalSize, colorRegisterDepth, resolution, pixelSpeed, fps));
}
}
return Collections.unmodifiableMap(infos);
}
public static AmigaDisplayInfo getInfo(int camg) {
return getAllInfos().get(camg);
}
public final static int MONITOR_ID_MASK = 0xffff1000;
public final static int DEFAULT_MONITOR_ID = 0x00000000;
public final static int NTSC_MONITOR_ID = 0x00011000;
public final static int PAL_MONITOR_ID = 0x00021000;
public final static int MULTISCAN_MONITOR_ID = 0x00031000;
public final static int A2024_MONITOR_ID = 0x00041000;
public final static int PROTO_MONITOR_ID = 0x00051000;
public final static int EURO72_MONITOR_ID = 0x00061000;
public final static int EURO36_MONITOR_ID = 0x00071000;
public final static int SUPER72_MONITOR_ID = 0x00081000;
public final static int DBLNTSC_MONITOR_ID = 0x00091000;
public final static int DBLPAL_MONITOR_ID = 0x000a1000;
public static int[] getMonitorIds() {
return new int[] {
DEFAULT_MONITOR_ID,
NTSC_MONITOR_ID,
PAL_MONITOR_ID,
MULTISCAN_MONITOR_ID,
A2024_MONITOR_ID,
PROTO_MONITOR_ID,
EURO72_MONITOR_ID,
EURO36_MONITOR_ID,
SUPER72_MONITOR_ID,
DBLNTSC_MONITOR_ID,
DBLPAL_MONITOR_ID,
};
}
public static int[] getGoodMonitorIds() {
return new int[] {
DEFAULT_MONITOR_ID,
NTSC_MONITOR_ID,
PAL_MONITOR_ID,
MULTISCAN_MONITOR_ID,
EURO72_MONITOR_ID,
EURO36_MONITOR_ID,
SUPER72_MONITOR_ID,
DBLNTSC_MONITOR_ID,
DBLPAL_MONITOR_ID,
};
}
public static int getFPS(int camg) {
MonitorItem mi= monitorToFPSMap.get(camg&MONITOR_ID_MASK);
return mi==null?0:mi.fps;
}
public static String getMonitorName(int camg) {
MonitorItem mi= monitorToFPSMap.get(camg&MONITOR_ID_MASK);
return mi==null?null:mi.name;
}
public boolean isDualPlayfield() {
return( camg&DUALPLAYFIELD_MASK)==DUALPLAYFIELD_MODE;
}
private static class MonitorItem {
String name;
int fps;
public MonitorItem(String name, int fps) {
this.name = name;
this.fps = fps;
}
}
private final static TreeMap monitorToFPSMap;
static {
monitorToFPSMap = new TreeMap();
monitorToFPSMap.put(DEFAULT_MONITOR_ID, new MonitorItem("NTSC OCS",60));
monitorToFPSMap.put(NTSC_MONITOR_ID, new MonitorItem("NTSC",60));
monitorToFPSMap.put(PAL_MONITOR_ID, new MonitorItem("PAL",50));
monitorToFPSMap.put(MULTISCAN_MONITOR_ID,new MonitorItem("MULTISCAN", 58));
monitorToFPSMap.put(A2024_MONITOR_ID, new MonitorItem("A2024",60));
monitorToFPSMap.put(PROTO_MONITOR_ID, new MonitorItem("PROTO",60));
monitorToFPSMap.put(EURO72_MONITOR_ID, new MonitorItem("EURO72",69));
monitorToFPSMap.put(EURO36_MONITOR_ID, new MonitorItem("EURO36",73));
monitorToFPSMap.put(SUPER72_MONITOR_ID, new MonitorItem("SUPER72",71));
monitorToFPSMap.put(DBLNTSC_MONITOR_ID, new MonitorItem("DBLNTSC",58));
monitorToFPSMap.put(DBLPAL_MONITOR_ID, new MonitorItem("DBLPAL",48));
}
public final static int COLORMODE_MASK = 0x00000880;
public final static int HAM_COLORMODE = 0x00000800;
public final static int EHB_COLORMODE = 0x00000080;
public final static int PALNTSC_INTERLACE_MASK = 0x00000004;
public final static int PALNTSC_INTERLACE_MODE = 0x00000004;
public final static int MULTISCAN_INTERLACE_MASK = 0x00000001;
public final static int MULTISCAN_INTERLACE_MODE = 0x00000001;
public final static int DUALPLAYFIELD_MASK = 0x00000400;
public final static int DUALPLAYFIELD_MODE = 0x00000400;
public final static int NTSC_320x200_44t52_60fps = 0x11000;
public final static int NTSC_320x400_44t26_interlaced_60fps = 0x11004;
public final static int NTSC_640x200_22t52_60fps = 0x19000;
public final static int NTSC_640x400_22t26_interlaced_60fps = 0x19004;
public final static int NTSC_1280x200_11t52_60fps = 0x19020;
public final static int NTSC_1280x400_11t26_interlaced_60fps = 0x19024;
public final static int PAL_320x256_44t44_50fps = 0x21000;
public final static int PAL_320x512_44t22_interlaced_50fps = 0x21004;
public final static int PAL_640x256_22t44_50fps = 0x29000;
public final static int PAL_640x512_22t22_interlaced_50fps = 0x29004;
public final static int PAL_1280x256_11t44_50fps = 0x29020;
public final static int PAL_1280x512_11t22_interlaced_50fps = 0x29024;
public final static int MULTISCAN_160x480_88t22_58fps = 0x31004;
public final static int MULTISCAN_160x960_88t11_interlaced_58fps = 0x31005;
public final static int MULTISCAN_320x480_44t22_58fps = 0x39004;
public final static int MULTISCAN_320x960_44t11_interlaced_58fps = 0x39005;
public final static int MULTISCAN_640x480_22t22_58fps = 0x39024;
public final static int MULTISCAN_640x960_22t11_interlaced_58fps = 0x39025;
public final static int EURO72_640x400_22t22_69fps = 0x69024;
public final static int EURO72_640x800_22t11_interlaced_69fps = 0x69025;
public final static int EURO36_320x200_44t44_73fps = 0x71000;
public final static int EURO36_320x400_44t22_interlaced_73fps = 0x71004;
public final static int EURO36_640x200_22t44_73fps = 0x79000;
public final static int EURO36_640x400_22t22_interlaced_73fps = 0x79004;
public final static int EURO36_1280x200_11t44_73fps = 0x79020;
public final static int EURO36_1280x400_11t22_interlaced_73fps = 0x79024;
public final static int SUPER72_200x300_68t40_71fps = 0x81000;
public final static int SUPER72_200x600_68t20_interlaced_71fps = 0x81004;
public final static int SUPER72_400x300_34t40_71fps = 0x89000;
public final static int SUPER72_400x600_34t20_interlaced_71fps = 0x89004;
public final static int SUPER72_800x300_17t40_71fps = 0x89020;
public final static int SUPER72_800x600_17t20_interlaced_71fps = 0x89024;
public final static int DBLNTSC_320x200_44t52_58fps = 0x91000;
public final static int DBLNTSC_320x400_44t26_58fps = 0x91004;
public final static int DBLNTSC_320x800_44t13_interlaced_58fps = 0x91005;
public final static int DBLNTSC_640x200_22t52_58fps = 0x99000;
public final static int DBLNTSC_640x400_22t26_58fps = 0x99004;
public final static int DBLNTSC_640x800_22t13_interlaced_58fps = 0x99005;
public final static int DBLPAL_320x256_44t44_48fps = 0xa1000;
public final static int DBLPAL_320x512_44t22_48fps = 0xa1004;
public final static int DBLPAL_320x1024_44t11_interlaced_48fps = 0xa1005;
public final static int DBLPAL_640x256_22t44_48fps = 0xa9000;
public final static int DBLPAL_640x512_22t22_48fps = 0xa9004;
public final static int DBLPAL_640x1024_22t11_interlaced_48fps = 0xa9005;
private final static Object[][] infoTable = {
{0x0, "NTSC OCS:LowRes"
, 320, 200
, 362, 241
, 16, 1
, 16368, 16384
, 4
, 44, 52
, 140
},
{0x4, "NTSC OCS:LowRes Interlace"
, 320, 400
, 362, 482
, 16, 1
, 16368, 16384
, 4
, 44, 26
, 140
},
{0x80, " OCS"
, 320, 200
, 362, 241
, 16, 1
, 16368, 16384
, 4
, 44, 52
, 140
},
{0x84, " OCS"
, 320, 400
, 362, 482
, 16, 1
, 16368, 16384
, 4
, 44, 26
, 140
},
{0x400, " OCS"
, 320, 200
, 362, 241
, 16, 1
, 16368, 16384
, 4
, 44, 52
, 140
},
{0x404, " OCS"
, 320, 400
, 362, 482
, 16, 1
, 16368, 16384
, 4
, 44, 26
, 140
},
{0x440, " OCS"
, 320, 200
, 362, 241
, 16, 1
, 16368, 16384
, 4
, 44, 52
, 140
},
{0x444, " OCS"
, 320, 400
, 362, 482
, 16, 1
, 16368, 16384
, 4
, 44, 26
, 140
},
{0x800, " OCS"
, 320, 200
, 362, 241
, 16, 1
, 16368, 16384
, 4
, 44, 52
, 140
},
{0x804, " OCS"
, 320, 400
, 362, 482
, 16, 1
, 16368, 16384
, 4
, 44, 26
, 140
},
{0x8000, "NTSC OCS:HighRes"
, 640, 200
, 724, 241
, 32, 1
, 16368, 16384
, 4
, 22, 52
, 70
},
{0x8004, "NTSC OCS:HighRes Interlace"
, 640, 400
, 724, 482
, 32, 1
, 16368, 16384
, 4
, 22, 26
, 70
},
{0x8020, "NTSC OCS:SuperHighRes"
, 1280, 200
, 1448, 241
, 64, 1
, 16368, 16384
, 4
, 11, 52
, 35
},
{0x8024, "NTSC OCS:SuperHighRes Interlace"
, 1280, 400
, 1448, 482
, 64, 1
, 16368, 16384
, 4
, 11, 26
, 35
},
{0x8080, ""
, 640, 200
, 724, 241
, 32, 1
, 16368, 16384
, 4
, 22, 52
, 70
},
{0x8084, ""
, 640, 400
, 724, 482
, 32, 1
, 16368, 16384
, 4
, 22, 26
, 70
},
{0x80a0, ""
, 1280, 200
, 1448, 241
, 64, 1
, 16368, 16384
, 4
, 11, 52
, 35
},
{0x80a4, ""
, 1280, 400
, 1448, 482
, 64, 1
, 16368, 16384
, 4
, 11, 26
, 35
},
{0x8400, ""
, 640, 200
, 724, 241
, 32, 1
, 16368, 16384
, 4
, 22, 52
, 70
},
{0x8404, ""
, 640, 400
, 724, 482
, 32, 1
, 16368, 16384
, 4
, 22, 26
, 70
},
{0x8420, ""
, 1280, 200
, 1448, 241
, 64, 1
, 16368, 16384
, 4
, 11, 52
, 35
},
{0x8424, ""
, 1280, 400
, 1448, 482
, 64, 1
, 16368, 16384
, 4
, 11, 26
, 35
},
{0x8440, ""
, 640, 200
, 724, 241
, 32, 1
, 16368, 16384
, 4
, 22, 52
, 70
},
{0x8444, ""
, 640, 400
, 724, 482
, 32, 1
, 16368, 16384
, 4
, 22, 26
, 70
},
{0x8460, ""
, 1280, 200
, 1448, 241
, 64, 1
, 16368, 16384
, 4
, 11, 52
, 35
},
{0x8464, ""
, 1280, 400
, 1448, 482
, 64, 1
, 16368, 16384
, 4
, 11, 26
, 35
},
{0x8800, ""
, 640, 200
, 724, 241
, 32, 1
, 16368, 16384
, 4
, 22, 52
, 70
},
{0x8804, ""
, 640, 400
, 724, 482
, 32, 1
, 16368, 16384
, 4
, 22, 26
, 70
},
{0x8820, ""
, 1280, 200
, 1448, 241
, 64, 1
, 16368, 16384
, 4
, 11, 52
, 35
},
{0x8824, ""
, 1280, 400
, 1448, 482
, 64, 1
, 16368, 16384
, 4
, 11, 26
, 35
},
{0x11000, "NTSC:LowRes"
, 320, 200
, 362, 241
, 16, 1
, 16368, 16384
, 8
, 44, 52
, 140
},
{0x11004, "NTSC:LowRes Interlace"
, 320, 400
, 362, 482
, 16, 1
, 16368, 16384
, 8
, 44, 26
, 140
},
{0x11080, ""
, 320, 200
, 362, 241
, 16, 1
, 16368, 16384
, 8
, 44, 52
, 140
},
{0x11084, ""
, 320, 400
, 362, 482
, 16, 1
, 16368, 16384
, 8
, 44, 26
, 140
},
{0x11400, ""
, 320, 200
, 362, 241
, 16, 1
, 16368, 16384
, 8
, 44, 52
, 140
},
{0x11404, ""
, 320, 400
, 362, 482
, 16, 1
, 16368, 16384
, 8
, 44, 26
, 140
},
{0x11440, ""
, 320, 200
, 362, 241
, 16, 1
, 16368, 16384
, 8
, 44, 52
, 140
},
{0x11444, ""
, 320, 400
, 362, 482
, 16, 1
, 16368, 16384
, 8
, 44, 26
, 140
},
{0x11800, ""
, 320, 200
, 362, 241
, 16, 1
, 16368, 16384
, 8
, 44, 52
, 140
},
{0x11804, ""
, 320, 400
, 362, 482
, 16, 1
, 16368, 16384
, 8
, 44, 26
, 140
},
{0x19000, "NTSC:HighRes"
, 640, 200
, 724, 241
, 32, 1
, 16368, 16384
, 8
, 22, 52
, 70
},
{0x19004, "NTSC:HighRes Interlace"
, 640, 400
, 724, 482
, 32, 1
, 16368, 16384
, 8
, 22, 26
, 70
},
{0x19020, "NTSC:SuperHighRes"
, 1280, 200
, 1448, 241
, 64, 1
, 16368, 16384
, 8
, 11, 52
, 35
},
{0x19024, "NTSC:SuperHighRes Interlace"
, 1280, 400
, 1448, 482
, 64, 1
, 16368, 16384
, 8
, 11, 26
, 35
},
{0x19080, ""
, 640, 200
, 724, 241
, 32, 1
, 16368, 16384
, 8
, 22, 52
, 70
},
{0x19084, ""
, 640, 400
, 724, 482
, 32, 1
, 16368, 16384
, 8
, 22, 26
, 70
},
{0x190a0, ""
, 1280, 200
, 1448, 241
, 64, 1
, 16368, 16384
, 8
, 11, 52
, 35
},
{0x190a4, ""
, 1280, 400
, 1448, 482
, 64, 1
, 16368, 16384
, 8
, 11, 26
, 35
},
{0x19400, ""
, 640, 200
, 724, 241
, 32, 1
, 16368, 16384
, 8
, 22, 52
, 70
},
{0x19404, ""
, 640, 400
, 724, 482
, 32, 1
, 16368, 16384
, 8
, 22, 26
, 70
},
{0x19420, ""
, 1280, 200
, 1448, 241
, 64, 1
, 16368, 16384
, 8
, 11, 52
, 35
},
{0x19424, ""
, 1280, 400
, 1448, 482
, 64, 1
, 16368, 16384
, 8
, 11, 26
, 35
},
{0x19440, ""
, 640, 200
, 724, 241
, 32, 1
, 16368, 16384
, 8
, 22, 52
, 70
},
{0x19444, ""
, 640, 400
, 724, 482
, 32, 1
, 16368, 16384
, 8
, 22, 26
, 70
},
{0x19460, ""
, 1280, 200
, 1448, 241
, 64, 1
, 16368, 16384
, 8
, 11, 52
, 35
},
{0x19464, ""
, 1280, 400
, 1448, 482
, 64, 1
, 16368, 16384
, 8
, 11, 26
, 35
},
{0x19800, ""
, 640, 200
, 724, 241
, 32, 1
, 16368, 16384
, 8
, 22, 52
, 70
},
{0x19804, ""
, 640, 400
, 724, 482
, 32, 1
, 16368, 16384
, 8
, 22, 26
, 70
},
{0x19820, ""
, 1280, 200
, 1448, 241
, 64, 1
, 16368, 16384
, 8
, 11, 52
, 35
},
{0x19824, ""
, 1280, 400
, 1448, 482
, 64, 1
, 16368, 16384
, 8
, 11, 26
, 35
},
{0x21000, "PAL:LowRes"
, 320, 256
, 362, 283
, 16, 1
, 16368, 16384
, 8
, 44, 44
, 140
},
{0x21004, "PAL:LowRes Interlace"
, 320, 512
, 362, 566
, 16, 1
, 16368, 16384
, 8
, 44, 22
, 140
},
{0x21080, ""
, 320, 256
, 362, 283
, 16, 1
, 16368, 16384
, 8
, 44, 44
, 140
},
{0x21084, ""
, 320, 512
, 362, 566
, 16, 1
, 16368, 16384
, 8
, 44, 22
, 140
},
{0x21400, ""
, 320, 256
, 362, 283
, 16, 1
, 16368, 16384
, 8
, 44, 44
, 140
},
{0x21404, ""
, 320, 512
, 362, 566
, 16, 1
, 16368, 16384
, 8
, 44, 22
, 140
},
{0x21440, ""
, 320, 256
, 362, 283
, 16, 1
, 16368, 16384
, 8
, 44, 44
, 140
},
{0x21444, ""
, 320, 512
, 362, 566
, 16, 1
, 16368, 16384
, 8
, 44, 22
, 140
},
{0x21800, ""
, 320, 256
, 362, 283
, 16, 1
, 16368, 16384
, 8
, 44, 44
, 140
},
{0x21804, ""
, 320, 512
, 362, 566
, 16, 1
, 16368, 16384
, 8
, 44, 22
, 140
},
{0x29000, "PAL:HighRes"
, 640, 256
, 724, 283
, 32, 1
, 16368, 16384
, 8
, 22, 44
, 70
},
{0x29004, "PAL:HighRes Interlace"
, 640, 512
, 724, 566
, 32, 1
, 16368, 16384
, 8
, 22, 22
, 70
},
{0x29020, "PAL:SuperHighRes"
, 1280, 256
, 1448, 283
, 64, 1
, 16368, 16384
, 8
, 11, 44
, 35
},
{0x29024, "PAL:SuperHighRes Interlace"
, 1280, 512
, 1448, 566
, 64, 1
, 16368, 16384
, 8
, 11, 22
, 35
},
{0x29080, ""
, 640, 256
, 724, 283
, 32, 1
, 16368, 16384
, 8
, 22, 44
, 70
},
{0x29084, ""
, 640, 512
, 724, 566
, 32, 1
, 16368, 16384
, 8
, 22, 22
, 70
},
{0x290a0, ""
, 1280, 256
, 1448, 283
, 64, 1
, 16368, 16384
, 8
, 11, 44
, 35
},
{0x290a4, ""
, 1280, 512
, 1448, 566
, 64, 1
, 16368, 16384
, 8
, 11, 22
, 35
},
{0x29400, ""
, 640, 256
, 724, 283
, 32, 1
, 16368, 16384
, 8
, 22, 44
, 70
},
{0x29404, ""
, 640, 512
, 724, 566
, 32, 1
, 16368, 16384
, 8
, 22, 22
, 70
},
{0x29420, ""
, 1280, 256
, 1448, 283
, 64, 1
, 16368, 16384
, 8
, 11, 44
, 35
},
{0x29424, ""
, 1280, 512
, 1448, 566
, 64, 1
, 16368, 16384
, 8
, 11, 22
, 35
},
{0x29440, ""
, 640, 256
, 724, 283
, 32, 1
, 16368, 16384
, 8
, 22, 44
, 70
},
{0x29444, ""
, 640, 512
, 724, 566
, 32, 1
, 16368, 16384
, 8
, 22, 22
, 70
},
{0x29460, ""
, 1280, 256
, 1448, 283
, 64, 1
, 16368, 16384
, 8
, 11, 44
, 35
},
{0x29464, ""
, 1280, 512
, 1448, 566
, 64, 1
, 16368, 16384
, 8
, 11, 22
, 35
},
{0x29800, ""
, 640, 256
, 724, 283
, 32, 1
, 16368, 16384
, 8
, 22, 44
, 70
},
{0x29804, ""
, 640, 512
, 724, 566
, 32, 1
, 16368, 16384
, 8
, 22, 22
, 70
},
{0x29820, ""
, 1280, 256
, 1448, 283
, 64, 1
, 16368, 16384
, 8
, 11, 44
, 35
},
{0x29824, ""
, 1280, 512
, 1448, 566
, 64, 1
, 16368, 16384
, 8
, 11, 22
, 35
},
{0x31000, "MULTISCAN:ExtraLowRes"
, 160, 240
, 164, 240
, 16, 1
, 16368, 16384
, 8
, 88, 44
, 140
},
{0x31004, "MULTISCAN:ExtraLowRes"
, 160, 480
, 164, 480
, 16, 1
, 16368, 16384
, 8
, 88, 22
, 140
},
{0x31005, "MULTISCAN:ExtraLowRes Interlace"
, 160, 960
, 164, 960
, 16, 1
, 16368, 16384
, 8
, 88, 11
, 140
},
{0x31080, ""
, 160, 240
, 164, 240
, 16, 1
, 16368, 16384
, 8
, 88, 44
, 140
},
{0x31084, ""
, 160, 480
, 164, 480
, 16, 1
, 16368, 16384
, 8
, 88, 22
, 140
},
{0x31085, ""
, 160, 960
, 164, 960
, 16, 1
, 16368, 16384
, 8
, 88, 11
, 140
},
{0x31404, ""
, 160, 480
, 164, 480
, 16, 1
, 16368, 16384
, 8
, 88, 22
, 140
},
{0x31405, ""
, 160, 960
, 164, 960
, 16, 1
, 16368, 16384
, 8
, 88, 11
, 140
},
{0x31444, ""
, 160, 480
, 164, 480
, 16, 1
, 16368, 16384
, 8
, 88, 22
, 140
},
{0x31445, ""
, 160, 960
, 164, 960
, 16, 1
, 16368, 16384
, 8
, 88, 11
, 140
},
{0x31800, ""
, 160, 240
, 164, 240
, 16, 1
, 16368, 16384
, 8
, 88, 44
, 140
},
{0x31804, ""
, 160, 480
, 164, 480
, 16, 1
, 16368, 16384
, 8
, 88, 22
, 140
},
{0x31805, ""
, 160, 960
, 164, 960
, 16, 1
, 16368, 16384
, 8
, 88, 11
, 140
},
{0x39000, "MULTISCAN:LowRes"
, 320, 240
, 328, 240
, 16, 1
, 16368, 16384
, 8
, 44, 44
, 70
},
{0x39004, "MULTISCAN:LowRes"
, 320, 480
, 328, 480
, 16, 1
, 16368, 16384
, 8
, 44, 22
, 70
},
{0x39005, "MULTISCAN:LowRes Interlace"
, 320, 960
, 328, 960
, 16, 1
, 16368, 16384
, 8
, 44, 11
, 70
},
{0x39020, "MULTISCAN:Productivity"
, 640, 240
, 656, 240
, 16, 1
, 16368, 16384
, 8
, 22, 44
, 35
},
{0x39024, "MULTISCAN:Productivity"
, 640, 480
, 656, 480
, 16, 1
, 16368, 16384
, 8
, 22, 22
, 35
},
{0x39025, "MULTISCAN:Productivity Interl."
, 640, 960
, 656, 960
, 16, 1
, 16368, 16384
, 8
, 22, 11
, 35
},
{0x39080, ""
, 320, 240
, 328, 240
, 16, 1
, 16368, 16384
, 8
, 44, 44
, 70
},
{0x39084, ""
, 320, 480
, 328, 480
, 16, 1
, 16368, 16384
, 8
, 44, 22
, 70
},
{0x39085, ""
, 320, 960
, 328, 960
, 16, 1
, 16368, 16384
, 8
, 44, 11
, 70
},
{0x390a0, ""
, 640, 240
, 656, 240
, 16, 1
, 16368, 16384
, 8
, 22, 44
, 35
},
{0x390a4, ""
, 640, 480
, 656, 480
, 16, 1
, 16368, 16384
, 8
, 22, 22
, 35
},
{0x390a5, ""
, 640, 960
, 656, 960
, 16, 1
, 16368, 16384
, 8
, 22, 11
, 35
},
{0x39404, ""
, 320, 480
, 328, 480
, 16, 1
, 16368, 16384
, 8
, 44, 22
, 70
},
{0x39405, ""
, 320, 960
, 328, 960
, 16, 1
, 16368, 16384
, 8
, 44, 11
, 70
},
{0x39424, ""
, 640, 480
, 656, 480
, 16, 1
, 16368, 16384
, 8
, 22, 22
, 35
},
{0x39425, ""
, 640, 960
, 656, 960
, 16, 1
, 16368, 16384
, 8
, 22, 11
, 35
},
{0x39444, ""
, 320, 480
, 328, 480
, 16, 1
, 16368, 16384
, 8
, 44, 22
, 70
},
{0x39445, ""
, 320, 960
, 328, 960
, 16, 1
, 16368, 16384
, 8
, 44, 11
, 70
},
{0x39464, ""
, 640, 480
, 656, 480
, 16, 1
, 16368, 16384
, 8
, 22, 22
, 35
},
{0x39465, ""
, 640, 960
, 656, 960
, 16, 1
, 16368, 16384
, 8
, 22, 11
, 35
},
{0x39800, ""
, 320, 240
, 328, 240
, 16, 1
, 16368, 16384
, 8
, 44, 44
, 70
},
{0x39804, ""
, 320, 480
, 328, 480
, 16, 1
, 16368, 16384
, 8
, 44, 22
, 70
},
{0x39805, ""
, 320, 960
, 328, 960
, 16, 1
, 16368, 16384
, 8
, 44, 11
, 70
},
{0x39820, ""
, 640, 240
, 656, 240
, 16, 1
, 16368, 16384
, 8
, 22, 44
, 35
},
{0x39824, ""
, 640, 480
, 656, 480
, 16, 1
, 16368, 16384
, 8
, 22, 22
, 35
},
{0x39825, ""
, 640, 960
, 656, 960
, 16, 1
, 16368, 16384
, 8
, 22, 11
, 35
},
{0x61000, "EURO72:ExtraLowRes"
, 160, 200
, 164, 200
, 16, 1
, 16368, 16384
, 8
, 88, 44
, 140
},
{0x61004, "EURO72:ExtraLowRes"
, 160, 400
, 164, 400
, 16, 1
, 16368, 16384
, 8
, 88, 22
, 140
},
{0x61005, "EURO72:ExtraLowRes"
, 160, 800
, 164, 800
, 16, 1
, 16368, 16384
, 8
, 88, 11
, 140
},
{0x61080, ""
, 160, 200
, 164, 200
, 16, 1
, 16368, 16384
, 8
, 88, 44
, 140
},
{0x61084, ""
, 160, 400
, 164, 400
, 16, 1
, 16368, 16384
, 8
, 88, 22
, 140
},
{0x61085, ""
, 160, 800
, 164, 800
, 16, 1
, 16368, 16384
, 8
, 88, 11
, 140
},
{0x61404, ""
, 160, 400
, 164, 400
, 16, 1
, 16368, 16384
, 8
, 88, 22
, 140
},
{0x61405, ""
, 160, 800
, 164, 800
, 16, 1
, 16368, 16384
, 8
, 88, 11
, 140
},
{0x61444, ""
, 160, 400
, 164, 400
, 16, 1
, 16368, 16384
, 8
, 88, 22
, 140
},
{0x61445, ""
, 160, 800
, 164, 800
, 16, 1
, 16368, 16384
, 8
, 88, 11
, 140
},
{0x61800, ""
, 160, 200
, 164, 200
, 16, 1
, 16368, 16384
, 8
, 88, 44
, 140
},
{0x61804, ""
, 160, 400
, 164, 400
, 16, 1
, 16368, 16384
, 8
, 88, 22
, 140
},
{0x61805, ""
, 160, 800
, 164, 800
, 16, 1
, 16368, 16384
, 8
, 88, 11
, 140
},
{0x69000, "EURO72:LowRes"
, 320, 200
, 328, 200
, 16, 1
, 16368, 16384
, 8
, 44, 44
, 70
},
{0x69004, "EURO72:LowRes"
, 320, 400
, 328, 400
, 16, 1
, 16368, 16384
, 8
, 44, 22
, 70
},
{0x69005, "EURO72:LowRes"
, 320, 800
, 328, 800
, 16, 1
, 16368, 16384
, 8
, 44, 11
, 70
},
{0x69020, "EURO72:Productivity"
, 640, 200
, 656, 200
, 16, 1
, 16368, 16384
, 8
, 22, 44
, 35
},
{0x69024, "EURO72:Productivity"
, 640, 400
, 656, 400
, 16, 1
, 16368, 16384
, 8
, 22, 22
, 35
},
{0x69025, "EURO72:Productivity Interl."
, 640, 800
, 656, 800
, 16, 1
, 16368, 16384
, 8
, 22, 11
, 35
},
{0x69080, ""
, 320, 200
, 328, 200
, 16, 1
, 16368, 16384
, 8
, 44, 44
, 70
},
{0x69084, ""
, 320, 400
, 328, 400
, 16, 1
, 16368, 16384
, 8
, 44, 22
, 70
},
{0x69085, ""
, 320, 800
, 328, 800
, 16, 1
, 16368, 16384
, 8
, 44, 11
, 70
},
{0x690a0, ""
, 640, 200
, 656, 200
, 16, 1
, 16368, 16384
, 8
, 22, 44
, 35
},
{0x690a4, ""
, 640, 400
, 656, 400
, 16, 1
, 16368, 16384
, 8
, 22, 22
, 35
},
{0x690a5, ""
, 640, 800
, 656, 800
, 16, 1
, 16368, 16384
, 8
, 22, 11
, 35
},
{0x69404, ""
, 320, 400
, 328, 400
, 16, 1
, 16368, 16384
, 8
, 44, 22
, 70
},
{0x69405, ""
, 320, 800
, 328, 800
, 16, 1
, 16368, 16384
, 8
, 44, 11
, 70
},
{0x69424, ""
, 640, 400
, 656, 400
, 16, 1
, 16368, 16384
, 8
, 22, 22
, 35
},
{0x69425, ""
, 640, 800
, 656, 800
, 16, 1
, 16368, 16384
, 8
, 22, 11
, 35
},
{0x69444, ""
, 320, 400
, 328, 400
, 16, 1
, 16368, 16384
, 8
, 44, 22
, 70
},
{0x69445, ""
, 320, 800
, 328, 800
, 16, 1
, 16368, 16384
, 8
, 44, 11
, 70
},
{0x69464, ""
, 640, 400
, 656, 400
, 16, 1
, 16368, 16384
, 8
, 22, 22
, 35
},
{0x69465, ""
, 640, 800
, 656, 800
, 16, 1
, 16368, 16384
, 8
, 22, 11
, 35
},
{0x69800, ""
, 320, 200
, 328, 200
, 16, 1
, 16368, 16384
, 8
, 44, 44
, 70
},
{0x69804, ""
, 320, 400
, 328, 400
, 16, 1
, 16368, 16384
, 8
, 44, 22
, 70
},
{0x69805, ""
, 320, 800
, 328, 800
, 16, 1
, 16368, 16384
, 8
, 44, 11
, 70
},
{0x69820, ""
, 640, 200
, 656, 200
, 16, 1
, 16368, 16384
, 8
, 22, 44
, 35
},
{0x69824, ""
, 640, 400
, 656, 400
, 16, 1
, 16368, 16384
, 8
, 22, 22
, 35
},
{0x69825, ""
, 640, 800
, 656, 800
, 16, 1
, 16368, 16384
, 8
, 22, 11
, 35
},
{0x71000, "EURO36:LowRes"
, 320, 200
, 362, 200
, 16, 1
, 16368, 16384
, 8
, 44, 44
, 140
},
{0x71004, "EURO36:LowRes Interlace"
, 320, 400
, 362, 400
, 16, 1
, 16368, 16384
, 8
, 44, 22
, 140
},
{0x71080, ""
, 320, 200
, 362, 200
, 16, 1
, 16368, 16384
, 8
, 44, 44
, 140
},
{0x71084, ""
, 320, 400
, 362, 400
, 16, 1
, 16368, 16384
, 8
, 44, 22
, 140
},
{0x71400, ""
, 320, 200
, 362, 200
, 16, 1
, 16368, 16384
, 8
, 44, 44
, 140
},
{0x71404, ""
, 320, 400
, 362, 400
, 16, 1
, 16368, 16384
, 8
, 44, 22
, 140
},
{0x71440, ""
, 320, 200
, 362, 200
, 16, 1
, 16368, 16384
, 8
, 44, 44
, 140
},
{0x71444, ""
, 320, 400
, 362, 400
, 16, 1
, 16368, 16384
, 8
, 44, 22
, 140
},
{0x71800, ""
, 320, 200
, 362, 200
, 16, 1
, 16368, 16384
, 8
, 44, 44
, 140
},
{0x71804, ""
, 320, 400
, 362, 400
, 16, 1
, 16368, 16384
, 8
, 44, 22
, 140
},
{0x79000, "EURO36:HighRes"
, 640, 200
, 724, 200
, 16, 1
, 16368, 16384
, 8
, 22, 44
, 70
},
{0x79004, "EURO36:HighRes Interlace"
, 640, 400
, 724, 400
, 16, 1
, 16368, 16384
, 8
, 22, 22
, 70
},
{0x79020, "EURO36:SuperHighRes"
, 1280, 200
, 1448, 200
, 16, 1
, 16368, 16384
, 8
, 11, 44
, 35
},
{0x79024, "EURO36:SuperHighRes Interl."
, 1280, 400
, 1448, 400
, 16, 1
, 16368, 16384
, 8
, 11, 22
, 35
},
{0x79080, ""
, 640, 200
, 724, 200
, 16, 1
, 16368, 16384
, 8
, 22, 44
, 70
},
{0x79084, ""
, 640, 400
, 724, 400
, 16, 1
, 16368, 16384
, 8
, 22, 22
, 70
},
{0x790a0, ""
, 1280, 200
, 1448, 200
, 16, 1
, 16368, 16384
, 8
, 11, 44
, 35
},
{0x790a4, ""
, 1280, 400
, 1448, 400
, 16, 1
, 16368, 16384
, 8
, 11, 22
, 35
},
{0x79400, ""
, 640, 200
, 724, 200
, 16, 1
, 16368, 16384
, 8
, 22, 44
, 70
},
{0x79404, ""
, 640, 400
, 724, 400
, 16, 1
, 16368, 16384
, 8
, 22, 22
, 70
},
{0x79420, ""
, 1280, 200
, 1448, 200
, 16, 1
, 16368, 16384
, 8
, 11, 44
, 35
},
{0x79424, ""
, 1280, 400
, 1448, 400
, 16, 1
, 16368, 16384
, 8
, 11, 22
, 35
},
{0x79440, ""
, 640, 200
, 724, 200
, 16, 1
, 16368, 16384
, 8
, 22, 44
, 70
},
{0x79444, ""
, 640, 400
, 724, 400
, 16, 1
, 16368, 16384
, 8
, 22, 22
, 70
},
{0x79460, ""
, 1280, 200
, 1448, 200
, 16, 1
, 16368, 16384
, 8
, 11, 44
, 35
},
{0x79464, ""
, 1280, 400
, 1448, 400
, 16, 1
, 16368, 16384
, 8
, 11, 22
, 35
},
{0x79800, ""
, 640, 200
, 724, 200
, 16, 1
, 16368, 16384
, 8
, 22, 44
, 70
},
{0x79804, ""
, 640, 400
, 724, 400
, 16, 1
, 16368, 16384
, 8
, 22, 22
, 70
},
{0x79820, ""
, 1280, 200
, 1448, 200
, 16, 1
, 16368, 16384
, 8
, 11, 44
, 35
},
{0x79824, ""
, 1280, 400
, 1448, 400
, 16, 1
, 16368, 16384
, 8
, 11, 22
, 35
},
{0x81000, "SUPER72:LowRes"
, 200, 300
, 228, 306
, 16, 1
, 16368, 16384
, 8
, 68, 40
, 140
},
{0x81004, "SUPER72:LowRes Interlace"
, 200, 600
, 228, 612
, 16, 1
, 16368, 16384
, 8
, 68, 20
, 140
},
{0x81008, "SUPER72:LowRes"
, 200, 150
, 228, 153
, 16, 1
, 16368, 16384
, 8
, 68, 80
, 140
},
{0x81080, ""
, 200, 300
, 228, 306
, 16, 1
, 16368, 16384
, 8
, 68, 40
, 140
},
{0x81084, ""
, 200, 600
, 228, 612
, 16, 1
, 16368, 16384
, 8
, 68, 20
, 140
},
{0x81088, ""
, 200, 150
, 228, 153
, 16, 1
, 16368, 16384
, 8
, 68, 80
, 140
},
{0x81400, ""
, 200, 300
, 228, 306
, 16, 1
, 16368, 16384
, 8
, 68, 40
, 140
},
{0x81404, ""
, 200, 600
, 228, 612
, 16, 1
, 16368, 16384
, 8
, 68, 20
, 140
},
{0x81440, ""
, 200, 300
, 228, 306
, 16, 1
, 16368, 16384
, 8
, 68, 40
, 140
},
{0x81444, ""
, 200, 600
, 228, 612
, 16, 1
, 16368, 16384
, 8
, 68, 20
, 140
},
{0x81800, ""
, 200, 300
, 228, 306
, 16, 1
, 16368, 16384
, 8
, 68, 40
, 140
},
{0x81804, ""
, 200, 600
, 228, 612
, 16, 1
, 16368, 16384
, 8
, 68, 20
, 140
},
{0x81808, ""
, 200, 150
, 228, 153
, 16, 1
, 16368, 16384
, 8
, 68, 80
, 140
},
{0x89000, "SUPER72:HighRes"
, 400, 300
, 456, 306
, 16, 1
, 16368, 16384
, 8
, 34, 40
, 70
},
{0x89004, "SUPER72:HighRes Interlace"
, 400, 600
, 456, 612
, 16, 1
, 16368, 16384
, 8
, 34, 20
, 70
},
{0x89008, "SUPER72:HighRes"
, 400, 150
, 456, 153
, 16, 1
, 16368, 16384
, 8
, 34, 80
, 70
},
{0x89020, "SUPER72:SuperHighRes"
, 800, 300
, 912, 306
, 16, 1
, 16368, 16384
, 8
, 17, 40
, 35
},
{0x89024, "SUPER72:SuperHighRes Interlace"
, 800, 600
, 912, 612
, 16, 1
, 16368, 16384
, 8
, 17, 20
, 35
},
{0x89028, "SUPER72:HighRes"
, 800, 150
, 912, 153
, 16, 1
, 16368, 16384
, 8
, 17, 80
, 35
},
{0x89080, ""
, 400, 300
, 456, 306
, 16, 1
, 16368, 16384
, 8
, 34, 40
, 70
},
{0x89084, ""
, 400, 600
, 456, 612
, 16, 1
, 16368, 16384
, 8
, 34, 20
, 70
},
{0x89088, ""
, 400, 150
, 456, 153
, 16, 1
, 16368, 16384
, 8
, 34, 80
, 70
},
{0x890a0, ""
, 800, 300
, 912, 306
, 16, 1
, 16368, 16384
, 8
, 17, 40
, 35
},
{0x890a4, ""
, 800, 600
, 912, 612
, 16, 1
, 16368, 16384
, 8
, 17, 20
, 35
},
{0x890a8, ""
, 800, 150
, 912, 153
, 16, 1
, 16368, 16384
, 8
, 17, 80
, 35
},
{0x89400, ""
, 400, 300
, 456, 306
, 16, 1
, 16368, 16384
, 8
, 34, 40
, 70
},
{0x89404, ""
, 400, 600
, 456, 612
, 16, 1
, 16368, 16384
, 8
, 34, 20
, 70
},
{0x89420, ""
, 800, 300
, 912, 306
, 16, 1
, 16368, 16384
, 8
, 17, 40
, 35
},
{0x89424, ""
, 800, 600
, 912, 612
, 16, 1
, 16368, 16384
, 8
, 17, 20
, 35
},
{0x89440, ""
, 400, 300
, 456, 306
, 16, 1
, 16368, 16384
, 8
, 34, 40
, 70
},
{0x89444, ""
, 400, 600
, 456, 612
, 16, 1
, 16368, 16384
, 8
, 34, 20
, 70
},
{0x89460, ""
, 800, 300
, 912, 306
, 16, 1
, 16368, 16384
, 8
, 17, 40
, 35
},
{0x89464, ""
, 800, 600
, 912, 612
, 16, 1
, 16368, 16384
, 8
, 17, 20
, 35
},
{0x89800, ""
, 400, 300
, 456, 306
, 16, 1
, 16368, 16384
, 8
, 34, 40
, 70
},
{0x89804, ""
, 400, 600
, 456, 612
, 16, 1
, 16368, 16384
, 8
, 34, 20
, 70
},
{0x89808, ""
, 400, 150
, 456, 153
, 16, 1
, 16368, 16384
, 8
, 34, 80
, 70
},
{0x89820, ""
, 800, 300
, 912, 306
, 16, 1
, 16368, 16384
, 8
, 17, 40
, 35
},
{0x89824, ""
, 800, 600
, 912, 612
, 16, 1
, 16368, 16384
, 8
, 17, 20
, 35
},
{0x89828, ""
, 800, 150
, 912, 153
, 16, 1
, 16368, 16384
, 8
, 17, 80
, 35
},
{0x91000, "DBLNTSC:LowRes"
, 320, 200
, 360, 227
, 16, 1
, 16368, 16384
, 8
, 44, 52
, 70
},
{0x91004, "DBLNTSC:LowRes Flickerfree"
, 320, 400
, 360, 454
, 16, 1
, 16368, 16384
, 8
, 44, 26
, 70
},
{0x91005, "DBLNTSC:LowRes Interlace"
, 320, 800
, 360, 908
, 16, 1
, 16368, 16384
, 8
, 44, 13
, 70
},
{0x91080, ""
, 320, 200
, 360, 227
, 16, 1
, 16368, 16384
, 8
, 44, 52
, 70
},
{0x91084, ""
, 320, 400
, 360, 454
, 16, 1
, 16368, 16384
, 8
, 44, 26
, 70
},
{0x91085, ""
, 320, 800
, 360, 908
, 16, 1
, 16368, 16384
, 8
, 44, 13
, 70
},
{0x91200, "DBLNTSC:ExtraLowRes"
, 160, 200
, 180, 227
, 16, 1
, 16368, 16384
, 8
, 88, 52
, 140
},
{0x91204, "DBLNTSC:ExtraLowRes"
, 160, 400
, 180, 454
, 16, 1
, 16368, 16384
, 8
, 88, 26
, 140
},
{0x91205, "DBLNTSC:ExtraLowRes"
, 160, 800
, 180, 908
, 16, 1
, 16368, 16384
, 8
, 88, 13
, 140
},
{0x91280, ""
, 160, 200
, 180, 227
, 16, 1
, 16368, 16384
, 8
, 88, 52
, 140
},
{0x91284, ""
, 160, 400
, 180, 454
, 16, 1
, 16368, 16384
, 8
, 88, 26
, 140
},
{0x91285, ""
, 160, 800
, 180, 908
, 16, 1
, 16368, 16384
, 8
, 88, 13
, 140
},
{0x91400, ""
, 320, 400
, 360, 454
, 16, 1
, 16368, 16384
, 8
, 44, 26
, 70
},
{0x91404, ""
, 320, 400
, 360, 454
, 16, 1
, 16368, 16384
, 8
, 44, 26
, 70
},
{0x91405, ""
, 320, 800
, 360, 908
, 16, 1
, 16368, 16384
, 8
, 44, 13
, 70
},
{0x91440, ""
, 320, 400
, 360, 454
, 16, 1
, 16368, 16384
, 8
, 44, 26
, 70
},
{0x91444, ""
, 320, 400
, 360, 454
, 16, 1
, 16368, 16384
, 8
, 44, 26
, 70
},
{0x91445, ""
, 320, 800
, 360, 908
, 16, 1
, 16368, 16384
, 8
, 44, 13
, 70
},
{0x91600, ""
, 160, 400
, 180, 454
, 16, 1
, 16368, 16384
, 8
, 88, 26
, 140
},
{0x91604, ""
, 160, 400
, 180, 454
, 16, 1
, 16368, 16384
, 8
, 88, 26
, 140
},
{0x91605, ""
, 160, 800
, 180, 908
, 16, 1
, 16368, 16384
, 8
, 88, 13
, 140
},
{0x91640, ""
, 160, 400
, 180, 454
, 16, 1
, 16368, 16384
, 8
, 88, 26
, 140
},
{0x91644, ""
, 160, 400
, 180, 454
, 16, 1
, 16368, 16384
, 8
, 88, 26
, 140
},
{0x91645, ""
, 160, 800
, 180, 908
, 16, 1
, 16368, 16384
, 8
, 88, 13
, 140
},
{0x91800, ""
, 320, 200
, 360, 227
, 16, 1
, 16368, 16384
, 8
, 44, 52
, 70
},
{0x91804, ""
, 320, 400
, 360, 454
, 16, 1
, 16368, 16384
, 8
, 44, 26
, 70
},
{0x91805, ""
, 320, 800
, 360, 908
, 16, 1
, 16368, 16384
, 8
, 44, 13
, 70
},
{0x91a00, ""
, 160, 200
, 180, 227
, 16, 1
, 16368, 16384
, 8
, 88, 52
, 140
},
{0x91a04, ""
, 160, 400
, 180, 454
, 16, 1
, 16368, 16384
, 8
, 88, 26
, 140
},
{0x91a05, ""
, 160, 800
, 180, 908
, 16, 1
, 16368, 16384
, 8
, 88, 13
, 140
},
{0x99000, "DBLNTSC:HighRes"
, 640, 200
, 720, 227
, 16, 1
, 16368, 16384
, 8
, 22, 52
, 35
},
{0x99004, "DBLNTSC:HighRes Flickerfree"
, 640, 400
, 720, 454
, 16, 1
, 16368, 16384
, 8
, 22, 26
, 35
},
{0x99005, "DBLNTSC:HighRes Interlace"
, 640, 800
, 720, 908
, 16, 1
, 16368, 16384
, 8
, 22, 13
, 35
},
{0x99080, ""
, 640, 200
, 720, 227
, 16, 1
, 16368, 16384
, 8
, 22, 52
, 35
},
{0x99084, ""
, 640, 400
, 720, 454
, 16, 1
, 16368, 16384
, 8
, 22, 26
, 35
},
{0x99085, ""
, 640, 800
, 720, 908
, 16, 1
, 16368, 16384
, 8
, 22, 13
, 35
},
{0x99400, ""
, 640, 400
, 720, 454
, 16, 1
, 16368, 16384
, 8
, 22, 26
, 35
},
{0x99404, ""
, 640, 400
, 720, 454
, 16, 1
, 16368, 16384
, 8
, 22, 26
, 35
},
{0x99405, ""
, 640, 800
, 720, 908
, 16, 1
, 16368, 16384
, 8
, 22, 13
, 35
},
{0x99440, ""
, 640, 400
, 720, 454
, 16, 1
, 16368, 16384
, 8
, 22, 26
, 35
},
{0x99444, ""
, 640, 400
, 720, 454
, 16, 1
, 16368, 16384
, 8
, 22, 26
, 35
},
{0x99445, ""
, 640, 800
, 720, 908
, 16, 1
, 16368, 16384
, 8
, 22, 13
, 35
},
{0x99800, ""
, 640, 200
, 720, 227
, 16, 1
, 16368, 16384
, 8
, 22, 52
, 35
},
{0x99804, ""
, 640, 400
, 720, 454
, 16, 1
, 16368, 16384
, 8
, 22, 26
, 35
},
{0x99805, ""
, 640, 800
, 720, 908
, 16, 1
, 16368, 16384
, 8
, 22, 13
, 35
},
{0xa1000, "DBLPAL:LowRes"
, 320, 256
, 360, 275
, 16, 1
, 16368, 16384
, 8
, 44, 44
, 70
},
{0xa1004, "DBLPAL:LowRes Flickerfree"
, 320, 512
, 360, 550
, 16, 1
, 16368, 16384
, 8
, 44, 22
, 70
},
{0xa1005, "DBLPAL:LowRes Interlace"
, 320, 1024
, 360, 1100
, 16, 1
, 16368, 16384
, 8
, 44, 11
, 70
},
{0xa1080, ""
, 320, 256
, 360, 275
, 16, 1
, 16368, 16384
, 8
, 44, 44
, 70
},
{0xa1084, ""
, 320, 512
, 360, 550
, 16, 1
, 16368, 16384
, 8
, 44, 22
, 70
},
{0xa1085, ""
, 320, 1024
, 360, 1100
, 16, 1
, 16368, 16384
, 8
, 44, 11
, 70
},
{0xa1200, "DBLPAL:ExtraLowRes"
, 160, 256
, 180, 275
, 16, 1
, 16368, 16384
, 8
, 88, 44
, 140
},
{0xa1204, "DBLPAL:ExtraLowRes"
, 160, 512
, 180, 550
, 16, 1
, 16368, 16384
, 8
, 88, 22
, 140
},
{0xa1205, "DBLPAL:ExtraLowRes"
, 160, 1024
, 180, 1100
, 16, 1
, 16368, 16384
, 8
, 88, 11
, 140
},
{0xa1280, ""
, 160, 256
, 180, 275
, 16, 1
, 16368, 16384
, 8
, 88, 44
, 140
},
{0xa1284, ""
, 160, 512
, 180, 550
, 16, 1
, 16368, 16384
, 8
, 88, 22
, 140
},
{0xa1285, ""
, 160, 1024
, 180, 1100
, 16, 1
, 16368, 16384
, 8
, 88, 11
, 140
},
{0xa1400, ""
, 320, 512
, 360, 550
, 16, 1
, 16368, 16384
, 8
, 44, 22
, 70
},
{0xa1404, ""
, 320, 512
, 360, 550
, 16, 1
, 16368, 16384
, 8
, 44, 22
, 70
},
{0xa1405, ""
, 320, 1024
, 360, 1100
, 16, 1
, 16368, 16384
, 8
, 44, 11
, 70
},
{0xa1440, ""
, 320, 512
, 360, 550
, 16, 1
, 16368, 16384
, 8
, 44, 22
, 70
},
{0xa1444, ""
, 320, 512
, 360, 550
, 16, 1
, 16368, 16384
, 8
, 44, 22
, 70
},
{0xa1445, ""
, 320, 1024
, 360, 1100
, 16, 1
, 16368, 16384
, 8
, 44, 11
, 70
},
{0xa1600, ""
, 160, 512
, 180, 550
, 16, 1
, 16368, 16384
, 8
, 88, 22
, 140
},
{0xa1604, ""
, 160, 512
, 180, 550
, 16, 1
, 16368, 16384
, 8
, 88, 22
, 140
},
{0xa1605, ""
, 160, 1024
, 180, 1100
, 16, 1
, 16368, 16384
, 8
, 88, 11
, 140
},
{0xa1640, ""
, 160, 512
, 180, 550
, 16, 1
, 16368, 16384
, 8
, 88, 22
, 140
},
{0xa1644, ""
, 160, 512
, 180, 550
, 16, 1
, 16368, 16384
, 8
, 88, 22
, 140
},
{0xa1645, ""
, 160, 1024
, 180, 1100
, 16, 1
, 16368, 16384
, 8
, 88, 11
, 140
},
{0xa1800, ""
, 320, 256
, 360, 275
, 16, 1
, 16368, 16384
, 8
, 44, 44
, 70
},
{0xa1804, ""
, 320, 512
, 360, 550
, 16, 1
, 16368, 16384
, 8
, 44, 22
, 70
},
{0xa1805, ""
, 320, 1024
, 360, 1100
, 16, 1
, 16368, 16384
, 8
, 44, 11
, 70
},
{0xa1a00, ""
, 160, 256
, 180, 275
, 16, 1
, 16368, 16384
, 8
, 88, 44
, 140
},
{0xa1a04, ""
, 160, 512
, 180, 550
, 16, 1
, 16368, 16384
, 8
, 88, 22
, 140
},
{0xa1a05, ""
, 160, 1024
, 180, 1100
, 16, 1
, 16368, 16384
, 8
, 88, 11
, 140
},
{0xa9000, "DBLPAL:HighRes"
, 640, 256
, 720, 275
, 16, 1
, 16368, 16384
, 8
, 22, 44
, 35
},
{0xa9004, "DBLPAL:HighRes Flickerfree"
, 640, 512
, 720, 550
, 16, 1
, 16368, 16384
, 8
, 22, 22
, 35
},
{0xa9005, "DBLPAL:HighRes Interlace"
, 640, 1024
, 720, 1100
, 16, 1
, 16368, 16384
, 8
, 22, 11
, 35
},
{0xa9080, ""
, 640, 256
, 720, 275
, 16, 1
, 16368, 16384
, 8
, 22, 44
, 35
},
{0xa9084, ""
, 640, 512
, 720, 550
, 16, 1
, 16368, 16384
, 8
, 22, 22
, 35
},
{0xa9085, ""
, 640, 1024
, 720, 1100
, 16, 1
, 16368, 16384
, 8
, 22, 11
, 35
},
{0xa9400, ""
, 640, 512
, 720, 550
, 16, 1
, 16368, 16384
, 8
, 22, 22
, 35
},
{0xa9404, ""
, 640, 512
, 720, 550
, 16, 1
, 16368, 16384
, 8
, 22, 22
, 35
},
{0xa9405, ""
, 640, 1024
, 720, 1100
, 16, 1
, 16368, 16384
, 8
, 22, 11
, 35
},
{0xa9440, ""
, 640, 512
, 720, 550
, 16, 1
, 16368, 16384
, 8
, 22, 22
, 35
},
{0xa9444, ""
, 640, 512
, 720, 550
, 16, 1
, 16368, 16384
, 8
, 22, 22
, 35
},
{0xa9445, ""
, 640, 1024
, 720, 1100
, 16, 1
, 16368, 16384
, 8
, 22, 11
, 35
},
{0xa9800, ""
, 640, 256
, 720, 275
, 16, 1
, 16368, 16384
, 8
, 22, 44
, 35
},
{0xa9804, ""
, 640, 512
, 720, 550
, 16, 1
, 16368, 16384
, 8
, 22, 22
, 35
},
{0xa9805, ""
, 640, 1024
, 720, 1100
, 16, 1
, 16368, 16384
, 8
, 22, 11
, 35
},
};
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy