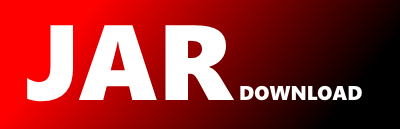
woko.async.JobManager Maven / Gradle / Ivy
The newest version!
package woko.async;
import woko.Closeable;
import woko.util.WLogger;
import java.util.List;
import java.util.Map;
import java.util.concurrent.*;
/**
* Top-level component for managing async {@link Job}s.
*/
public class JobManager implements Closeable {
public static final String KEY = "JobManager";
private static final WLogger logger = WLogger.getLogger(JobManager.class);
private final ExecutorService pool;
private final Map runningJobs = new ConcurrentHashMap();
/**
* Create with a single-threaded executor.
*/
public JobManager() {
this(Executors.newSingleThreadExecutor());
}
/**
* Create with passed ExecutorService
.
*
* @param pool the ExecutorService
to be used
*/
public JobManager(ExecutorService pool) {
logger.info("Created with ExecutorService : " + pool);
this.pool = pool;
}
/**
* Submit and execute a new Job
, and notify all listeners.
*
* @param job the Job
to execute
* @param listeners a list of the listeners to be notified during job execution
*/
public void submit(final Job job, final List listeners) {
logger.debug("Submitting Job : " + job + " with listeners : " + listeners);
pool.submit(new Callable
© 2015 - 2025 Weber Informatics LLC | Privacy Policy