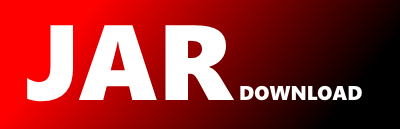
woko.ioc.SimpleWokoIocContainer Maven / Gradle / Ivy
The newest version!
package woko.ioc;
import net.sourceforge.jfacets.IFacetDescriptorManager;
import woko.Closeable;
import woko.persistence.ObjectStore;
import woko.users.UserManager;
import woko.users.UsernameResolutionStrategy;
import woko.util.WLogger;
import java.util.Collections;
import java.util.HashMap;
import java.util.Map;
/**
* Simple implementation of the IOC container for use cases where no heavy IOC is needed.
*/
public class SimpleWokoIocContainer<
OsType extends ObjectStore,
UmType extends UserManager,
UnsType extends UsernameResolutionStrategy,
FdmType extends IFacetDescriptorManager
> extends AbstractWokoIocContainer {
private final Map
© 2015 - 2025 Weber Informatics LLC | Privacy Policy