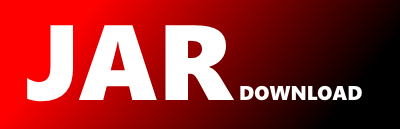
com.powsybl.openrao.data.crac.io.cim.xsd.RemedialActionSeries Maven / Gradle / Ivy
Show all versions of open-rao-crac-io-cim Show documentation
//
// Ce fichier a été généré par Eclipse Implementation of JAXB, v4.0.5
// Voir https://eclipse-ee4j.github.io/jaxb-ri
// Toute modification apportée à ce fichier sera perdue lors de la recompilation du schéma source.
//
package com.powsybl.openrao.data.crac.io.cim.xsd;
import java.math.BigDecimal;
import java.util.ArrayList;
import java.util.List;
import jakarta.xml.bind.annotation.XmlAccessType;
import jakarta.xml.bind.annotation.XmlAccessorType;
import jakarta.xml.bind.annotation.XmlElement;
import jakarta.xml.bind.annotation.XmlType;
/**
* Classe Java pour RemedialAction_Series complex type.
*
* Le fragment de schéma suivant indique le contenu attendu figurant dans cette classe.
*
* {@code
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
* }
*
*
*/
@XmlAccessorType(XmlAccessType.FIELD)
@XmlType(name = "RemedialAction_Series", propOrder = {
"mrid",
"name",
"businessType",
"applicationModeMarketObjectStatusStatus",
"availabilityMarketObjectStatusStatus",
"partyMarketParticipant",
"inDomainMRID",
"outDomainMRID",
"measurementUnitName",
"quantityQuantity",
"registeredResource",
"sharedDomain",
"reason"
})
public class RemedialActionSeries {
@XmlElement(name = "mRID", required = true)
protected String mrid;
protected String name;
protected String businessType;
@XmlElement(name = "applicationMode_MarketObjectStatus.status")
protected String applicationModeMarketObjectStatusStatus;
@XmlElement(name = "availability_MarketObjectStatus.status")
protected String availabilityMarketObjectStatusStatus;
@XmlElement(name = "Party_MarketParticipant")
protected List partyMarketParticipant;
@XmlElement(name = "in_Domain.mRID")
protected AreaIDString inDomainMRID;
@XmlElement(name = "out_Domain.mRID")
protected AreaIDString outDomainMRID;
@XmlElement(name = "measurement_Unit.name")
protected String measurementUnitName;
@XmlElement(name = "quantity.quantity")
protected BigDecimal quantityQuantity;
@XmlElement(name = "RegisteredResource")
protected List registeredResource;
@XmlElement(name = "Shared_Domain")
protected List sharedDomain;
@XmlElement(name = "Reason")
protected List reason;
/**
* Obtient la valeur de la propriété mrid.
*
* @return
* possible object is
* {@link String }
*
*/
public String getMRID() {
return mrid;
}
/**
* Définit la valeur de la propriété mrid.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setMRID(String value) {
this.mrid = value;
}
/**
* Obtient la valeur de la propriété name.
*
* @return
* possible object is
* {@link String }
*
*/
public String getName() {
return name;
}
/**
* Définit la valeur de la propriété name.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setName(String value) {
this.name = value;
}
/**
* Obtient la valeur de la propriété businessType.
*
* @return
* possible object is
* {@link String }
*
*/
public String getBusinessType() {
return businessType;
}
/**
* Définit la valeur de la propriété businessType.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setBusinessType(String value) {
this.businessType = value;
}
/**
* Obtient la valeur de la propriété applicationModeMarketObjectStatusStatus.
*
* @return
* possible object is
* {@link String }
*
*/
public String getApplicationModeMarketObjectStatusStatus() {
return applicationModeMarketObjectStatusStatus;
}
/**
* Définit la valeur de la propriété applicationModeMarketObjectStatusStatus.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setApplicationModeMarketObjectStatusStatus(String value) {
this.applicationModeMarketObjectStatusStatus = value;
}
/**
* Obtient la valeur de la propriété availabilityMarketObjectStatusStatus.
*
* @return
* possible object is
* {@link String }
*
*/
public String getAvailabilityMarketObjectStatusStatus() {
return availabilityMarketObjectStatusStatus;
}
/**
* Définit la valeur de la propriété availabilityMarketObjectStatusStatus.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setAvailabilityMarketObjectStatusStatus(String value) {
this.availabilityMarketObjectStatusStatus = value;
}
/**
* Gets the value of the partyMarketParticipant property.
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the JAXB object.
* This is why there is not a set
method for the partyMarketParticipant property.
*
*
* For example, to add a new item, do as follows:
*
*
* getPartyMarketParticipant().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link PartyMarketParticipant }
*
*
*
* @return
* The value of the partyMarketParticipant property.
*/
public List getPartyMarketParticipant() {
if (partyMarketParticipant == null) {
partyMarketParticipant = new ArrayList<>();
}
return this.partyMarketParticipant;
}
/**
* Obtient la valeur de la propriété inDomainMRID.
*
* @return
* possible object is
* {@link AreaIDString }
*
*/
public AreaIDString getInDomainMRID() {
return inDomainMRID;
}
/**
* Définit la valeur de la propriété inDomainMRID.
*
* @param value
* allowed object is
* {@link AreaIDString }
*
*/
public void setInDomainMRID(AreaIDString value) {
this.inDomainMRID = value;
}
/**
* Obtient la valeur de la propriété outDomainMRID.
*
* @return
* possible object is
* {@link AreaIDString }
*
*/
public AreaIDString getOutDomainMRID() {
return outDomainMRID;
}
/**
* Définit la valeur de la propriété outDomainMRID.
*
* @param value
* allowed object is
* {@link AreaIDString }
*
*/
public void setOutDomainMRID(AreaIDString value) {
this.outDomainMRID = value;
}
/**
* Obtient la valeur de la propriété measurementUnitName.
*
* @return
* possible object is
* {@link String }
*
*/
public String getMeasurementUnitName() {
return measurementUnitName;
}
/**
* Définit la valeur de la propriété measurementUnitName.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setMeasurementUnitName(String value) {
this.measurementUnitName = value;
}
/**
* Obtient la valeur de la propriété quantityQuantity.
*
* @return
* possible object is
* {@link BigDecimal }
*
*/
public BigDecimal getQuantityQuantity() {
return quantityQuantity;
}
/**
* Définit la valeur de la propriété quantityQuantity.
*
* @param value
* allowed object is
* {@link BigDecimal }
*
*/
public void setQuantityQuantity(BigDecimal value) {
this.quantityQuantity = value;
}
/**
* Gets the value of the registeredResource property.
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the JAXB object.
* This is why there is not a set
method for the registeredResource property.
*
*
* For example, to add a new item, do as follows:
*
*
* getRegisteredResource().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link RemedialActionRegisteredResource }
*
*
*
* @return
* The value of the registeredResource property.
*/
public List getRegisteredResource() {
if (registeredResource == null) {
registeredResource = new ArrayList<>();
}
return this.registeredResource;
}
/**
* Gets the value of the sharedDomain property.
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the JAXB object.
* This is why there is not a set
method for the sharedDomain property.
*
*
* For example, to add a new item, do as follows:
*
*
* getSharedDomain().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link SharedDomain }
*
*
*
* @return
* The value of the sharedDomain property.
*/
public List getSharedDomain() {
if (sharedDomain == null) {
sharedDomain = new ArrayList<>();
}
return this.sharedDomain;
}
/**
* Gets the value of the reason property.
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the JAXB object.
* This is why there is not a set
method for the reason property.
*
*
* For example, to add a new item, do as follows:
*
*
* getReason().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link SeriesReason }
*
*
*
* @return
* The value of the reason property.
*/
public List getReason() {
if (reason == null) {
reason = new ArrayList<>();
}
return this.reason;
}
}