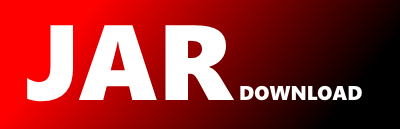
com.powsybl.openrao.raoapi.parameters.ParametersUtil Maven / Gradle / Ivy
/*
* Copyright (c) 2023, RTE (http://www.rte-france.com)
* This Source Code Form is subject to the terms of the Mozilla Public
* License, v. 2.0. If a copy of the MPL was not distributed with this
* file, You can obtain one at http://mozilla.org/MPL/2.0/.
*/
package com.powsybl.openrao.raoapi.parameters;
import com.powsybl.openrao.commons.OpenRaoException;
import com.powsybl.iidm.network.Country;
import org.apache.commons.lang3.StringUtils;
import java.util.*;
/**
*
* @author Godelaine de Montmorillon {@literal }
*/
public final class ParametersUtil {
private ParametersUtil() {
}
public static Set convertToCountrySet(List countryStringList) {
Set countryList = new HashSet<>();
for (String countryString : countryStringList) {
try {
countryList.add(Country.valueOf(countryString));
} catch (Exception e) {
throw new OpenRaoException(String.format("[%s] could not be recognized as a country", countryString));
}
}
return countryList;
}
protected static Map convertListToStringStringMap(List stringList) {
Map map = new HashMap<>();
stringList.forEach(listEntry -> {
String[] splitListEntry = listEntry.split(":");
if (splitListEntry.length != 2) {
throw new OpenRaoException(String.format("String pairs separated by \":\" must be defined, e.g {String1}:{String2} instead of %s", listEntry));
}
map.put(convertBracketIntoString(splitListEntry[0]), convertBracketIntoString(splitListEntry[1]));
});
return map;
}
protected static List convertStringStringMapToList(Map map) {
List list = new ArrayList<>();
map.forEach((key, value) -> list.add("{" + key + "}:{" + value + "}"));
return list;
}
protected static Map convertListToStringIntMap(List stringList) {
Map map = new HashMap<>();
stringList.forEach(listEntry -> {
String[] splitListEntry = listEntry.split(":");
if (splitListEntry.length != 2) {
throw new OpenRaoException(String.format("String-Integer pairs separated by \":\" must be defined, e.g {String1}:Integer instead of %s", listEntry));
}
map.put(convertBracketIntoString(splitListEntry[0]), Integer.parseInt(splitListEntry[1]));
});
return map;
}
protected static List convertStringIntMapToList(Map map) {
List list = new ArrayList<>();
map.forEach((key, value) -> list.add("{" + key + "}:" + value.toString()));
return list;
}
protected static List> convertListToListOfList(List stringList) {
List> listOfList = new ArrayList<>();
stringList.forEach(listEntry -> {
String[] splitListEntry = listEntry.split("\\+");
List newList = new ArrayList<>();
for (String splitString : splitListEntry) {
newList.add(convertBracketIntoString(splitString));
}
listOfList.add(newList);
});
return listOfList;
}
protected static List convertListOfListToList(List> listOfList) {
List finalList = new ArrayList<>();
listOfList.forEach(subList -> {
if (!subList.isEmpty()) {
finalList.add("{" + String.join("}+{", subList) + "}");
}
});
return finalList;
}
private static String convertBracketIntoString(String stringInBrackets) {
// Check that there are only one opening and one closing brackets
if (stringInBrackets.chars().filter(ch -> ch == '{').count() != 1 || stringInBrackets.chars().filter(ch -> ch == '}').count() != 1) {
throw new OpenRaoException(String.format("%s contains too few or too many occurences of \"{ or \"}", stringInBrackets));
}
String insideString = StringUtils.substringBetween(stringInBrackets, "{", "}");
if (insideString == null || insideString.length() == 0) {
throw new OpenRaoException(String.format("%s is not contained into brackets", stringInBrackets));
}
return insideString;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy