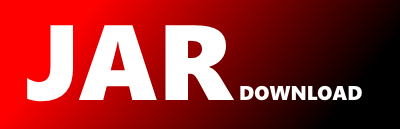
com.powsybl.openrao.searchtreerao.commons.AbsolutePtdfSumsComputation Maven / Gradle / Ivy
/*
* Copyright (c) 2020, RTE (http://www.rte-france.com)
* This Source Code Form is subject to the terms of the Mozilla Public
* License, v. 2.0. If a copy of the MPL was not distributed with this
* file, You can obtain one at http://mozilla.org/MPL/2.0/.
*/
package com.powsybl.openrao.searchtreerao.commons;
import com.powsybl.openrao.commons.EICode;
import com.powsybl.openrao.data.cracapi.cnec.Side;
import com.powsybl.glsk.commons.ZonalData;
import com.powsybl.openrao.data.cracapi.cnec.FlowCnec;
import com.powsybl.openrao.raoapi.ZoneToZonePtdfDefinition;
import com.powsybl.openrao.sensitivityanalysis.SystematicSensitivityResult;
import com.powsybl.sensitivity.SensitivityVariableSet;
import java.util.*;
/**
* This class computes the absolute PTDF sums on a given set of CNECs
* It requires that the sensitivity values be already computed
*
* @author Peter Mitri {@literal }
* @author Baptiste Seguinot {@literal }
*/
public class AbsolutePtdfSumsComputation {
private final ZonalData glskProvider;
private final List zTozPtdfs;
public AbsolutePtdfSumsComputation(ZonalData glskProvider, List zTozPtdfs) {
this.glskProvider = glskProvider;
this.zTozPtdfs = zTozPtdfs;
}
public Map> computeAbsolutePtdfSums(Set flowCnecs, SystematicSensitivityResult sensitivityResult) {
Map> ptdfSums = new HashMap<>();
List eiCodesInPtdfs = zTozPtdfs.stream().flatMap(zToz -> zToz.getEiCodes().stream()).toList();
for (FlowCnec flowCnec : flowCnecs) {
flowCnec.getMonitoredSides().forEach(side -> {
Map ptdfMap = buildZoneToSlackPtdfMap(flowCnec, side, glskProvider, eiCodesInPtdfs, sensitivityResult);
double sumOfZToZPtdf = zTozPtdfs.stream().mapToDouble(zToz -> Math.abs(computeZToZPtdf(zToz, ptdfMap))).sum();
ptdfSums.computeIfAbsent(flowCnec, k -> new EnumMap<>(Side.class)).put(side, sumOfZToZPtdf);
});
}
return ptdfSums;
}
private Map buildZoneToSlackPtdfMap(FlowCnec flowCnec, Side side, ZonalData glsks, List eiCodesInBoundaries, SystematicSensitivityResult sensitivityResult) {
Map ptdfs = new HashMap<>();
for (EICode eiCode : eiCodesInBoundaries) {
SensitivityVariableSet linearGlsk = glsks.getData(eiCode.getAreaCode());
if (linearGlsk != null) {
ptdfs.put(eiCode, sensitivityResult.getSensitivityOnFlow(linearGlsk, flowCnec, side));
}
}
return ptdfs;
}
private double computeZToZPtdf(ZoneToZonePtdfDefinition zToz, Map zToSlackPtdfMap) {
List zoneToSlackPtdf = zToz.getZoneToSlackPtdfs().stream()
.filter(zToS -> zToSlackPtdfMap.containsKey(zToS.getEiCode()))
.map(zToS -> zToS.getWeight() * zToSlackPtdfMap.get(zToS.getEiCode()))
.toList();
if (zoneToSlackPtdf.size() < 2) {
// the boundary should at least contains two zoneToSlack PTDFs
return 0.0;
} else {
return zoneToSlackPtdf.stream().mapToDouble(v -> v).sum();
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy