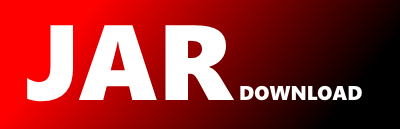
com.powsybl.computation.local.WindowsLocalCommandExecutor Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of powsybl-computation-local Show documentation
Show all versions of powsybl-computation-local Show documentation
A computation implementation to run computations locally
The newest version!
/**
* Copyright (c) 2017, RTE (http://www.rte-france.com)
* This Source Code Form is subject to the terms of the Mozilla Public
* License, v. 2.0. If a copy of the MPL was not distributed with this
* file, You can obtain one at http://mozilla.org/MPL/2.0/.
* SPDX-License-Identifier: MPL-2.0
*/
package com.powsybl.computation.local;
import com.google.common.collect.ImmutableList;
import com.google.common.collect.ImmutableMap;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import java.io.File;
import java.io.IOException;
import java.nio.file.Path;
import java.util.List;
import java.util.Map;
/**
* @author Geoffroy Jamgotchian {@literal }
* @author Nicolas Lhuillier {@literal }
*/
public class WindowsLocalCommandExecutor extends AbstractLocalCommandExecutor {
private static final Logger LOGGER = LoggerFactory.getLogger(WindowsLocalCommandExecutor.class);
@Override
public int execute(String program, List args, Path outFile, Path errFile, Path workingDir, Map env) throws IOException, InterruptedException {
return execute(program, -1, args, outFile, errFile, workingDir, env);
}
@Override
public int execute(String program, long timeoutSecondes, List args, Path outFile, Path errFile, Path workingDir, Map env) throws IOException, InterruptedException {
// set TMP and TEMP to working dir to avoid issues
Map env2 = ImmutableMap.builder()
.putAll(env)
.put("TEMP", workingDir.toAbsolutePath().toString())
.put("TMP", workingDir.toAbsolutePath().toString())
.build();
StringBuilder internalCmd = new StringBuilder();
internalCmd.append("setlocal & ");
for (Map.Entry entry : env2.entrySet()) {
String name = entry.getKey();
String value = entry.getValue();
internalCmd.append("set ").append("\"").append(name).append("=").append(value);
if (name.endsWith("PATH")) {
internalCmd.append(File.pathSeparator).append("%").append(name).append("%");
}
internalCmd.append("\"").append(" & ");
}
internalCmd.append(program);
for (String arg : args) {
internalCmd.append(" \"").append(arg).append("\"");
}
internalCmd.append(" & endlocal");
List cmdLs = ImmutableList.builder()
.add("cmd")
.add("/c")
.add(internalCmd.toString())
.build();
return execute(cmdLs, workingDir, outFile, errFile, timeoutSecondes);
}
@Override
void nonZeroLog(List cmdLs, int exitCode) {
LOGGER.debug(NON_ZERO_LOG_PATTERN, cmdLs, exitCode);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy